Introduction
In today's lecture, we will embark on understanding variable data types and operators in C++. This is crucial as it forms the foundation of programming in C++. We will dive into the type of variable data types, their practical uses, and how to effectively utilize operators in programming.
By the end of this article, you will gain a comprehensive understanding of how to implement these concepts in your coding practices.
Types of Variable Data Types
C++ supports various data types. Here are some of the primary ones:
Primitive Data Types
- Integer (
int
): Used to store whole numbers. - Character (
char
): Used to store single characters such as 'a', 'b', or '1'. - Float (
float
): Used to store single-precision floating-point numbers (decimal numbers). - Double (
double
): Used to store double-precision floating-point numbers, which is more precise thanfloat
. - Boolean (
bool
): Can hold eithertrue
orfalse
values.
Example of Variable Declaration
int age = 25;
char grade = 'A';
float price = 99.99f;
double pi = 3.14159;
bool isCPlusPlusFun = true;
Memory Allocation for Data Types
Understanding how much memory each data type occupies is essential for memory management:
int
occupies 4 byteschar
occupies 1 bytefloat
occupies 4 bytesdouble
occupies 8 bytesbool
occupies 1 byte
This understanding helps in effective memory usage and management in larger applications.
Operators in C++
Operators are special symbols that perform operations on variables and values. C++ has several types of operators:
Arithmetic Operators
Arithmetic operators are used to perform basic mathematical operations:
- Addition (
+
) - Subtraction (
-
) - Multiplication (
*
) - Division (
/
) - Modulus (
%
) – finds the remainder.
Example of Using Arithmetic Operators
int a = 5;
int b = 2;
int sum = a + b;
int product = a * b;
float division = (float)a / b; // Type casting to float
int remainder = a % b;
Relational Operators
Relational operators are used to compare two values:
- Less than (
<
) - Greater than (
>
) - Less than or equal (
<=
) - Greater than or equal (
>=
) - Equal to (
==
) - Not equal to (
!=
)
Example
if (a > b) {
cout << "a is greater than b";
}
Logical Operators
Logical operators are used to combine multiple relational expressions:
- AND (
&&
) - OR (
||
) - NOT (
!
)
Example
if (a > b && b > 0) {
cout << "Both conditions are true.";
}
Unary Operators
Unary operators operate on a single operand. Key examples include:
- Increment (
++
) and Decrement (--
) operators that add or subtract 1 from a variable. - Postfix and Prefix Operators
a++
(postfix)++a
(prefix)
Example of Increment/Decrement
int counter = 10;
cout << counter++; // Outputs: 10, then counter becomes 11
cout << ++counter; // Outputs: 12
Input and Output in C++
C++ provides a way to handle user input and display output. We use cin
for input and cout
for output.
Example
int age;
cout << "Enter your age: ";
cin >> age;
cout << "Your age is: " << age;
Conclusion
Understanding variable data types and operators is essential for programming in C++. These foundational concepts help you manage data efficiently and perform operations necessary for creating functional programs. As you practice creating programs using these data types and operators, you'll become proficient in C++ programming.
Feel free to experiment with the examples provided and explore more complex operations, which will further enhance your programming skills.
Homework
To solidify your understanding, create a simple calculator program that can perform addition, subtraction, multiplication, and division based on user input. Happy coding!
हाय एवरीवन एंड टुडे वी आर गोइंग टू स्टार्ट विद आवर नेक्स्ट चैप्टर व्हिच इज गोइंग टू बी अबाउट वेरिएबल डेटा टाइप्स
एंड ऑपरेटर्स नाउ अपार्ट फ्रॉम दिस चैप्टर अगर हमें डीएसए के कॉन्सेप्ट्स में कोई और कांसेप्ट भी सीखना है तो वह हमें इसी चैनल
के ऊपर इस प्लेलिस्ट के अंदर अवेलेबल मिल रहा होगा तो वहां से जाकर हम सीख सकते हैं आल्सो यह जो हमारी पूरी डीएसए सीरीज रहेगी
इससे रिगार्डिंग जितने भी हमारे अपडेट्स होंगे जैसे लेक्चर का शेड्यूल हो गया तो वह सब हम इस
आपको नीचे डिस्क्रिप्शन बॉक्स के अंदर दिया गया है तो हम चाहे तो इसे भी जाकर चेक कर सकते हैं आल्सो हमारी सीरीज से
रिलेटेड जितनी भी आपकी प्रोग्रेस होगी उसे भी आप इसी सेम अकाउंट के साथ शेयर कर सकते हैं तो शुरुआत करते हैं अपने लेक्चर टू के
[संगीत] साथ हाय एवरीवन एंड वेलकम टू अपना कॉलेज और आज हम स्टार्ट करने वाले हैं अपना
लेक्चर टू इन आवर डीएसए सीरीज जिसके अंदर अब हम c+ प के अंदर प्रोग्रामिंग करना सीख रहे होंगे यानी फाइनली अब हम अपने कोड को
लिखना स्टार्ट करेंगे अब कोड को लिखने के लिए सबसे पहली चीज़ जो हम करने वाले हैं दैट इज़ टू राइट आवर फर्स्ट c+ प
प्रोग्राम अब इस प्रोग्राम को लिखने से पहले कुछ बेसिक चीजें हैं जिनका हमें पता होना चाहिए सबसे पहले तो हमें पता होना
चाहिए कि c+ प के अंदर आउटपुट कैसे प्रोड्यूस करते हैं आउटपुट प्रोड्यूस करने का मतलब है कि अगर मुझे अपनी कोड के थ्रू
अपनी स्क्रीन पर कुछ प्रिंट करवाना है तो वह मैं कैसे करा सकती हूं तो उसके लिए हम एक स्टेटमेंट यूज़ करते हैं व्हिच इज़ दिस
स्टेटमेंट वी बेसिकली यूज़ दिस सी आउट सी आउट कहने का मतलब है कि हम कुछ आउटपुट कराना चाहते हैं तो हम सी आउट लिखते हैं
उसके बाद हम इस तरीके से दो लेस दन के सिंबल लगाते हैं फिर हम डबल कोट्स के अंदर जो भी चीज लिखते हैं वो वैसे के वैसी
हमारी स्क्रीन पर आकर प्रिंट हो जाती है ये जो स्टेटमेंट यहां पर हमें दिखाई दे रही है इसे हम एक आउटपुट स्टेटमेंट कहते
हैं तो इस आउटपुट स्टेटमेंट को हम अपनी एक c+ की फाइल के अंदर लिखने वाले हैं अब c+ की फाइल के अंदर कोड लिखने से पहले एक
बहुत इंपॉर्टेंट चीज है जिसका हमें पता होना चाहिए कि c+ प एक केस सेंसिटिव लैंग्वेज है है केस सेंसिटिव कहने का मतलब
है कि अगर मैंने यहां पर यह c स्मॉल में लोअर केस में लिखा हुआ है तो हमें कोड के अंदर भी इसे लोअर केस में ही लिखना है हम
अगर कैपिटल c लिख देंगे स आउट की जगह तो हमारे पास एरर आ जाएगा तो जो भी चीज जैसे भी दिखाई जा रही है जो चीज लोअर केस में
है उसे लोअर केस में लिखना है जो चीज अपर केस में है उसे हमें अपर केस में लिखना है हमें केस चेंज नहीं करना नहीं तो हमारे
कोड के अंदर एरर आ जाएगा तो इस चीज पर हम पहले ही ध्यान देते हुए चल सकते हैं c+ प हो गया जावा हो गई पाइथन हो गई ये सारी
केस सेंसिटिव लैंग्वेज है और अगर एसक्यूएल जैसी लैंग्वेज की बात करें या फिर html5 टाइम स्टार्ट करते हैं तो हमारे पास
इस तरीके की स्टार्टिंग विंडो आती है ये जो नीचे वाला रीसेंट सेक्शन है यह आपके पास नहीं आ रहा होगा यह जनरली तब आता है
जब हम काफी टाइम से वीएस कोड को यूज़ कर रहे हैं तो इस वेलकम विंडो को यहां से जाकर हमें क्रॉस कर देना है एंड वी
बेसिकली हैव टू गो टू दिस एक्सप्लोरर आइकन लेट मी एनलार्जमेंट पे आइकन होता है इसको हम एक्सप्लोरर आइकन
कहते हैं और हमें इस पर क्लिक करना है जैसे इस पर क्लिक करेंगे हमारे पास कुछ ऑप्शंस आ जाएंगे हम बेसिकली इस ओपन फोल्डर
पर क्लिक करके अपने फोल्डर को ओपन करेंगे फोल्डर को ओपन करने के लिए आई एम गोइंग टू ओपन दिस फोल्डर कॉल्ड य सीरीज इस ओपन
फोल्डर पर क्लिक करने के बाद हम कोई भी फोल्डर है उसे सेलेक्ट कर सकते हैं हम चाहें तो कोई नया फोल्डर भी अपने लिए
क्रिएट कर सकते हैं लेट्स सपोज मैंने अपने लिए एक नया फोल्डर क्रिएट किया कॉल्ड youtube's आप चाहें तो इसको कुछ भी नाम दे
सकते हैं और यह मैंने क्रिएट कर दिया अब जैसे ये फोल्डर मेरे लिए क्रिएट हो गया चाहे आप मैक पर हैं चाहे आप विज पर हैं यू
कैन क्रिएट अ न्यू फोल्डर एंड यू कैन ओपन दिस फोल्डर तो फिर क्या होगा वीएस कोड के अंदर यह फोल्डर ओपन हो जाएगा कुछ ऑप्शंस
हमारे पास आएंगे हम इसको यस कर सकते हैं नो भी कर सकते हैं कोई फर्क नहीं पड़ेगा एंड यह वेलकम विंडो को भी हम यहां से
क्रॉस कर देंगे तो यहां से एक्सप्लोरर को क्लिक करके हम लेफ्ट वाली ये जो पैनल है इसे बंद या ओपन कर सकते हैं तो पैनल में
हमारे फोल्डर का नाम हमारे पास आ रहा है और इस फोल्डर के अंदर अब हम चाहे तो कोई नई फाइल भी क्रिएट कर सकते हैं बाय क्लिंग
ऑन दिस आइकन हम नया फोल्डर भी क्रिएट कर सकते हैं अब क्योंकि हमें डायरेक्टली कोड लिखना शुरू करना है तो हम एक फाइल क्रिएट
करने वाले हैं इस न्यू फाइल पर क्लिक करके आई एम गोइंग टू क्रिएट अ फाइल कॉल्ड कोड ड सप इस कोड ड सप में यह जो कोड है यह मेरी
फाइल का नाम है जो फाइल का नाम कुछ भी हो सकता है जनरली इसे हम इंग्लिश के अंदर रखते हैं तो आप इसको अपना फर्स्ट कोड भी
बोल सकते हैं कुछ भी बोल सकते हैं और यह जो डॉट सीपीपी है यह मेरी फाइल की एक्सटेंशन है c+ प की जितनी भी फाइल्स
होती हैं उन सारी फाइल्स की एक्सटेंशन होती है डॉ सप जैसे टेक्स्ट फाइल्स जो होती हैं उनकी एक्सटेंशन ड
html.com मैंने इस तरीके से दो लेस दन के सिंबल लिखे और मैंने डबल कोड्स के अंदर अपना कॉलेज लिखा और जैसे ही हम कोई भी
स्टेटमेंट लिखते हैं कोड के अंदर हमें हमेशा इसे एंड करना होता है विद अ सेमीकलन अब ये सेमीकलन का क्या मतलब है सेमीकलन को
मैंने यहां पर भी दिखाया है सेमीकलन को बेसिकली हम स्टेटमेंट टर्मिनेटर कहते हैं अब टर्मिनेटर सुनके खतरनाक लग रहा होगा बट
सेमी कोलन इन c+ प इज वेरी सिमिलर टू फुल स्टॉप इन इंग्लिश इंग्लिश के अंदर जो पीरियड होता है जो सेंटेंस के एंड पर लगता
है उसका सी + इक्विवेलेंट होता है हमारे पास सेमीकलन या हिंदी में जो पूर्ण विराम लगता है तो उसकी जगह हमारे c+ प का जो
पूर्ण विराम होता है उसको हम अपना टर्मिनेटर कहते हैं तो यहां पे मैंने अपनी स्टेटमेंट को खत्म कर दिया है अब ये तो हो
गई मेरी आउटपुट स्टेटमेंट इसे अगर मैं सेव भी कर लूं लेकिन अभी ये जो स्टेटमेंट है ये वर्क नहीं करेगी और इनफैक्ट आपके वीएस
कोड में ये रेड लाइंस आ रही होंगी जो हमारा विजुअल स्टूडियो कोड का सेटअप है वो हमें पहले ही बता रहा है कि हमारे कोड के
अंदर कुछ एरर है इनफैक्ट इस पर अगर हम हर करेंगे तो हम देख भी पाएंगे दिस डिक्लेरेशन हैज नो स्टोरेज क्लास और टाइप
फायर ये कुछ एरर आ रहा है पर यह कोड अभी इसलिए वर्क नहीं करेगा क्योंकि हमें कुछ-कुछ एडिशनल चीजें हैं जो अपने हर एक
c+ पलस कोड के अंदर लिखनी पड़ती है काम तो मुझे सिर्फ ये करवाना है प्रिंट करवाने का पर मुझे और भी स्टेटमेंट्स लिखनी पड़ेंगी
इस काम को कंप्लीट करवाने के लिए जिसमें से सबसे पहली चीज जो हम अपने c+ प के कोड के अंदर ऐड करते हैं दैट इज आवर मेन
फंक्शन अब ये मेन फंक्शन क्या होता है जैसे फ्लो चार्ट के अंदर जो भी हमने सॉल्यूशन लिखे थे तो सॉल्यूशंस को लिखने
के लिए हमने हमेशा स्टार्ट के साथ शुरुआत की थी तो कोड के अंदर जो मेन फंक्शन होता है दैट
इज लाइक द स्टार्ट इन फ्लो चार्ट सारा का सारा जो कोड एग्जीक्यूट होना रन होना शुरू होता है वह हमेशा इस मेन फंक्शन से रन
होना शुरू होता है वैसे फंक्शंस के बारे में बाद में हमारा एक डेडिकेटेड चैप्टर आएगा तो अभी ज्यादा टेंशन लेने की जरूरत
नहीं है कि फंक्शन क्या होता है उसको हम बाद में पढ़ेंगे अभी के लिए बस यह याद रखना है कि एक मेन फंक्शन नाम की चीज होती
है जो हमें हमेशा लिखनी होती है और मेन फंक्शन को कैसे लिखते हैं मेन फंक्शन को लिखने के लिए हम लिखते हैं
इंट मेन बीच में स्पेस आ रहा है उसके बाद हमारे पेंसिस एंड उसके बाद हमारे कर्ली ब्रेसेज
और जो भी हमें काम करवाना होता है वो हम इन कर्ली ब्रेसेज के बीच में लिख रहे होते हैं तो इस तरीके से हमारा मेन फंक्शन कोड
के अंदर लिखा जाता है लेट अस राइट द मेन फंक्शन मैंने लिख दिया इंट मेन एंड इस तरीके से ये जो सी आउट वाली लाइन है इसको
कट करके हम यहां पर पेस्ट कर देंगे इसे और थोड़ा सा ज़ूम इन कर देते हैं तो यह मेरा पूरा का पूरा मेन फंक्शन हो गया तो मेन
फंक्शन को लिखने के पीछे हमारा जो ऑब्जेक्टिव होता है वो ये होता है कि कंपाइलर को हम बता कि एग्जीक्यूशन यहां से
स्टार्ट होगा यह स्टार्टिंग पॉइंट है हमारे पूरे के पूरे प्रोग्राम का पर इसके साथ में दो और एडिशनल लाइंस हैं जिनको
हमें लिखना पड़ता है और इन लाइंस को हम लिखते हैं अपने कोड के अपने प्रोग्राम के अपनी फाइल के टॉप पर सबसे पहली लाइन होती
है हैश इंक्लूड आई स्ट्रीम अब ये हैश इंक्लूड आईओ स्ट्रीम क्या होता है बहुत ही टेक्निकल भाषा में मैं आपको बताऊं तो हैश
इंक्लूड आ स्ट्रीम को हम अपना प्रीप्रोसेसर डायरेक्टिव कहते हैं पर प्रीप्रोसेसर डायरेक्टिव के मैं उतना
डिटेल में नहीं जाऊंगी बेसिकली ये एक स्टेटमेंट है जो हम कंपाइलेशन से पहले ही अपने कंपाइलर को कुछ बात बता रहे हैं और
ये बात हम ये बता रहे हैं कि एक आयो स्ट्रीम नाम की फाइल है जिसके अंदर ये सी आउट का लॉजिक लिखा हुआ है अभी c+ प को
नहीं पता सी आउट का लॉजिक कहां है सी आउट कैसे काम करेगा सी आउट का काम प्रिंट करवाना है या इनपुट लेना है या क्या है वो
कंपाइलर को नहीं पता कंपाइलर को कब पता चलेगा जब हम इस आई स्ट्रीम को इंक्लूड करेंगे अपनी फाइल के अंदर तो इसीलिए सब
सबसे ऊपर हमने इसे इंक्लूड कर लिया तो यह सिंटेक्स होता है इंक्लूड करने का और ये ऐसी लाइन है जिसको हम हर एक सिंगल कोड के
अंदर लिखेंगे तो अब इसको याद करने की भी जरूरत नहीं है आपको धीरे-धीरे खुद ही आदत हो जाएगी सेकंड लाइन जो हम लिखते हैं इट
इज कॉल्ड यूजिंग नेम स्पेस एसटीडी अब नेम स्पेस का क्या मतलब होता है बेसिकली जब भी हम कोड लिख रहे होते हैं ऐसा तो है नहीं
कि सी आउट बहुत ही यूनिक चीज है हो सकता है हमारे पास एक फाइल हो जिसके अंदर एक सी आउट लिखा हुआ है हमारे पास दूसरी फाइल हो
जिसके अंदर एक सी आउट लिखा हुआ है हमारे पास तीसरी फाइल हो जिसके अंदर एक सी आउट लिखा हुआ है या फिर जैसे सी आउट होता है
वैसे आगे जाके हम पढ़ेंगे हमारे पास एक सी इन भी होता है तो लेट्स सपोज सी इन भी अलग-अलग फाइल्स के अंदर लिखा हुआ है अब
कंपाइलर को कैसे पता कि उसको ये वाला सी आउट यूज़ करना है ये वाला यूज़ करना है या ये वाला यूज़ करना है तो बेसिकली हम उसे
बताते हैं कि c+ प के अंदर एक एसडीडी नाम की नेम स्पेस है नेम स्पेस क्या होता है उसे अभी के लिए हम फाइल की तरह इमेजिन कर
सकते हैं ज्यादा उसमें डीप जाएंगे तो अभी हमें कंफ्यूजन हो सकता है उसको बाद में जाके हम डिटेल में पढ़ सकते हैं कि
नेमस्पेस का क्या मतलब होता है अभी के लिए इमेजिन करना है कि वो सिर्फ एक फाइल है जिसमें कई सारी चीजों का लॉजिक लिखा हुआ
है तो यहां पर जिस नेम स्पेस को हम यूज़ करना चाहते हैं उसका नाम एसडीडी है तो हमने सिंपली बताया है कि हम यूज करना
चाहते हैं पूरे कोड के अंदर एक नेम स्पेस जिसका नाम है एसटीडी वैसे अगर हम चाहे तो ये लाइन अपने कोड से हटाना चाहे तो
बिल्कुल हटा सकते हैं बस फिर हर बार जब भी हम सी आउट लिखेंगे या कुछ और लिखेंगे तो हमें इस तरीके से एसटीडी लिखना पड़ेगा और
बताना पड़ेगा कि एसटीडी वाली नेम स्पेस का सी आउट हम यूज़ करना चाहते हैं पर बार-बार हम ऐसे एसटीडी नहीं लिखते हैं हम सिंपली
क्या करते हैं हम यूजिंग नेमस्पेस एसटीडी एसटीडी बेसिकली c+ प का स्टैंडर्ड नेम स्पेस होता है जिसको हमने ऊपर ही इंक्लूड
कर लिया है ये दोनों लाइनों का मतलब अगर शुरुआत में कॉम्प्लिकेटेड लगे तो बहुत ही नेचुरल सी चीज है बट धीरे-धीरे बार-बार हम
इनका सिंटेक्स लिखेंगे तो हमें आदत हो जाएगी तो यह जनरली इतना हमारा कोड होता है जिसको हम हर बार हर एक c+ प फाइल के अंदर
लिख रहे होंगे इसके साथ में एक और एडिशनल चीज होती है वो एडिशनल चीज होती है कि जो हमने पूरा का पूरा अपना काम लिख लिया काम
मतलब कुछ भी प्रिंट करवाने का काम उसके बाद हम हमेशा एक रिटर्न जरो लिखते हैं रिटर्न जरो के बिना हमारा कोड बिल्कुल रन
कर जाएगा लेकिन क्योंकि इस फंक्शन का काम है एक इंटी जर वैल्यू को रिटर्न करना तो लास्ट में हम एक इंटी जर वैल्यू यानी
ज़ीरो को रिटर्न कर रहे हैं ये जो स्टेटमेंट मैंने बोली इसका मतलब अगर हमें कंप्लीट समझ नहीं आ रहा तो भी कोई परेशानी
की बात नहीं है उसको बाद में डिटेल में पढ़ेंगे कि इंटी जर क्या होता है पर ये रिटर्न ज़ीरो स्टेटमेंट लिखना एक अच्छे
प्रोग्रामर की निशानी होती है अगर यह आप स्किप कर रहे हैं तो मतलब हमें अच्छे से प्रोग्रामिंग नहीं आती है तो हमने कंप्लीट
सारी चीजों को लिख लिया है तो यहां से सबसे पहली चीज जो हमें पता चली होगी कि अगर हमें सिर्फ प्रिंट करवाने का भी काम
करना पड़ता है कोड के अंदर तो भी हमें काफी सारी एक्स्ट्रा लाइने लिखनी पड़ती हैं ताकि कंपाइलर हमारा काम समझ सके हम जो
करवाना चाहते हैं उसे समझ सके और उसे एग्जीक्यूट कर सके तो ये तो हमने अपना कोड लिख दिया अब इसे कंट्रोल s या कमांड एस
प्रेस करके हम सेव कर सकते हैं सेव करने के बाद इसे एग्जीक्यूट कैसे करना है एग्जीक्यूट करने के लिए हमारे वीएस कोड पर
ये हमारे पास नीचे की तरफ ऑप्शंस आ रहे होंगे हमें इस पर क्लिक करना है क्लिक करने के बाद यहां पर कई सारे डिफरेंट
डिफरेंट ऑप्शंस होंगे डी बग कंसोल आउटपुट हो गया हमें इस टर्मिनल पर जाना है टर्मिनल पर हमारे पास हमारे फोल्डर का नाम
भी आ रहा होगा हमारे पास हमारी पूरी मशीन का नाम भी आ रहा होगा तो जब आपके फोल्डर का नाम सही दिखा रहा है मतलब टर्मिनल में
सारी चीजें ठीक चल रही है अब सबसे पहले जब भी हम किसी भी फाइल को रन करना चाहते हैं जब भी हम किसी भी कोड को रन करना चाहते
हैं व दो स्टेजेस के अंदर रन करता है कैसे रन करता है सबसे पहले तो हमें उस कोड को कंपाइल करना पड़ता है तो सबसे पहली स्टेज
होती है जिसमें कंपाइलर उस कोड को कंपाइल करता है कंपाइल करने का मतलब होता है कि कोड के अंदर बेसिक चीजें देखना आपने सारी
चीजें सही तो लिखी है ना कोई गलती तो नहीं है जो c+ प् के रूल्स होते हैं उन सारे रूल्स को हम फॉलो कर रहे हैं ना कोई रेड
लाइन तो नहीं आ रही कोड के अंदर तो कंपाइल करने के लिए हमें यहां पे एक स्टेटमेंट लिखनी होती है टर्मिनल के अंदर g+ प जिससे
हम अपने c+ कंपाइलर को इवोक कर रहे हैं एंड उसके बाद हम लिखते हैं अपनी फाइल का नाम हमारी फाइल का नाम है कोड . सप तो हम
इसे एंटर कर देंगे अगर कोई भी एरर नहीं आया सक्सेसफुली एंटर हो गया तो मतलब हमारा कोड सक्सेसफुली कंपाइल कर गया जैसे ही
हमारा जो सी प का कोड होता है कोड ड सप फाइल है जैसे ही कंपाइलर ने उसे कंपाइल कर दिया तो कंपाइलर उसके लिए बना देता है एक
नई एग्जीक्यूटेबल फाइल इसका लॉजिक हमने लेक्चर वन में भी डिस्कस किया था कि कंप्यूटर को c+ प समझ में नहीं आती
कंप्यूटर को सिर्फ 0 और वन बाइनरी समझ में आती है रो और वन को बाइनरी कहते हैं प्रोग्रामिंग में और बाइनरी कहने का मतलब
है कि इस पूरे कोड को फिर मुझे एक बाइनरी फाइल के अंदर एग्जीक्यूटेबल फाइल के अंदर कन्वर्ट करना पड़ेगा और वह कन्वर्ट करने
का काम होता है कंपाइलर का तो अब आपके सिस्टम में उसी सेम फोल्डर के अंदर एक एग्जीक्यूटेबल फाइल बन गई होगी उस फाइल को
हम देख भी सकते हैं जैसे यहां पर मैं एक्सप्लोरर पर अगर क्लिक करूं तो मेरे पास एक नई फाइल बन गई है एड आउट आपके पास
मोस्ट प्रोबेबली जो फाइल बनी होगी उसका नाम या तो a.exe होगा या समथिंग डसी होगा डॉटसी फाइल विंडोज यूजर के लिए बनती है और
डॉट आउट फाइल मैक यूजर्स के लिए बनती है तो डिपेंडिंग अपॉन द सिस्टम हमारे पास अलग एक्सटेंशन की फाइल बन गई होगी पर
एवेंचुरा हो ये एक एग्जीक्यूटेबल फाइल है जिसको रन किया जाता है तो अगर अब मुझे इस फाइल को रन करना है हम रन किसको करते हैं
हम रन अपनी एग्जीक्यूटेबल फाइल को करते हैं तो इस फाइल को रन करने के लिए हमें लिखना पड़ता है / a.out अगर आप विज सिस्टम
पर हैं तो आपको डॉट लिखना पड़ेगा एंड फिर हम कर देते हैं एंटर जैसे ही हमने एंटर किया हमारे पास ये सेम चीज प्रिंट होकर आ
गई अपना कॉलेज तो यहां पर हमने अपना कॉलेज लिखा था एज इट इज हमारे पास यहां पर अपना कॉलेज प्रिंट होकर आ गया अब वैसे तो हमारा
कोड दो स्टेजेस में रन करता है फर्स्ट स्टेज इज कंपाइलेशन सेकंड स्टेज इज एग्जीक्यूशन या रनिंग का स्टेज लेकिन इन
दोनों को हम कंबाइन भी कर सकते हैं कंबाइन करने का मतलब है कि हम सिंपली लिख सकते हैं g+ प कोड . सप एंड एंड ये एंड एंड का
सिंबल है मतलब हम दो कमांड्स को एक साथ रन करना चाह रहे हैं और सेकंड कमांड है / a.out
तो इसको मैंने एंटर किया तो इस बार एक ही लाइन में मेरा कोड पहले कंपाइल हुआ उसके बाद मेरा कोड रन हो गया और उसका जो आउटपुट
है मेरे पास आकर प्रिंट हो गया अगर टर्मिनल को कभी भी हम क्लियर करना चाहते हैं तो उसके लिए हम सिंपली क्लियर कमांड
लिख सकते हैं और हमारे टर्मिनल से सारी कमांड्स गायब हो जाएंगी अगर हम पुरानी कमांड को वापस लाना चाहते हैं तो हमारे
कीबोर्ड के ऊपर जो एरो कीज होती है उसमें से ऊपर वाली अपवर्ड्स वाली एरो की अगर हम प्रेस करेंगे तो पुरानी कमांड आ जाएगी एक
बार और प्रेस करेंगे उससे पुरानी आ जाएगी एक बार और प्रेस करेंगे उससे पुरानी एंड उससे पुरानी डाउन वर्ड्स प्रेस कर तो नई
वाली हमारे पास कमांड आ जाएगी तो दोबारा कोड को रन करना है तो दोबारा हम इसे प्रेस कर सकते हैं अब कुछ और प्रिंट करवाने की
कोशिश करते हैं लेट्स सपोज इस बार अपना कॉलेज की जगह मुझे प्रिंट करवाना है हेलो वर्ल्ड तो मैंने इसे सेव किया सेव करवाने
के बाद इसे क्लियर करते हैं एंड दोबारा रन करते हैं तो इस बार मेरे पास हेलो वर्ल्ड प्रिंट होकर आ गया यहां पर एक बार लेक्चर
को पॉज करना है और यहां पर हेलो वर्ल्ड की जगह अपना कंप्लीट नाम आपको प्रिंट करवाने की कोशिश करनी है लिखना है चेंज करना है
कोड को और उसे एग्जीक्यूट करना है तो अगर उसे हम रन कर पाए हैं मतलब अभी तक चीजें हमारे लिए कंप्लीट क्लियर है अब जब भी हम
यह प्रिंट करवा रहे हैं आउटपुट स्क्रीन पर यह परसेंटेज जैसा अजीब सा साइन हमारे पास आ रहा है यह साइन ये सिंबल बेसिकली हमारे
पास इसलिए आ रहा है क्योंकि हमने लास्ट में कोई एंडलाइन कैरेक्टर नहीं दिया एंडलाइन कैरेक्टर कहने का मतलब है कि जब
हम पड़ता है तो उससे हम क्या हो जाते हैं नेक्स्ट लाइन पे आ जाते हैं वैसे ही जब हम
कुछ भी प्रिंट करवाते हैं और प्रिंट करवाने के बाद अगर हम नेक्स्ट लाइन पर आना चाहते हैं तो नेक्स्ट लाइन पर आने का
तरीका होता है कि हम लिखते हैं सी आउट और लेट्स सपोज हमने यहां पे कुछ प्रिंट करवा दिया उसके बाद हम एक और सेट ऑफ लेस दन लेस
दन कैरेक्टर लिखेंगे और हम लिखेंगे डी एल और फिर सेमीकलन तो इसका मतलब है यह जो आउटपुट प्रिंट हो जाए उसके बाद मुझे एक
एंड ऑफ लाइन चाहिए मतलब मैं चाहता हूं या मैं चाहती हूं कि मेरा जो आगे का सारा आउटपुट है वह सब नेक्स्ट लाइन में चला जाए
तो इसको भी एक बार करके देखते हैं और जैसे ही मैंने यहां पर एंड ऑफ लाइन किया वैसे ही क्या होगा ये परसेंटेज साइन गायब हो
जाएगा लेट अस क्लियर दिस एंड रिर तो इस बार हेलो वर्ल्ड आ रहा है विदाउट अ परसेंटेज साइन क्योंकि लास्ट में हमने
कैरेक्टर को क्लियर कर दिया और हम नेक्स्ट लाइन पर आ गए अब ऐसे ही नेक्स्ट लाइन लिखने का एक और तरीका होता है कि हम यहां
पर लिख दें बै n हम डबल कोट्स के अंदर ये बैक स्लैश सिंबल होता है पीछे से आगे की तरफ आता है एंड ये n हो गया तो बै स् n
कहने का मतलब है कि हमें एक नेक्स्ट लाइन कैरेक्टर चाहिए इसका भी आउटपुट बिल्कुल सेम होगा कोई डिफरेंस नहीं है अब वैसे
बहुत ज्यादा अगर डीप डाइव करें तो जनरली जो बैक स्ल ए होता है वो थोड़ा सा हल्का सा फास्टर होता है एज कंपेयर टू एंड लाइन
तो जनरली जब हम डेटा स्ट्रक्चर्स एल्गोरिथम्स पर मूव कर जाएंगे और जब या कंपीटेटिव प्रोग्रामिंग या कोई और चीजें
कर रहे हैं तो यू कैन प्रेफर बै स्ल n एज कंपेयर्ड टू एंड लाइन पर अभी के लिए सीखते टाइम कोई फर्क नहीं पड़ता और मेजॉरिटी
टाइम्स में एंड लाइन नहीं लिखने वाली हूं क्योंकि उसको मेरे लिए टाइप करना ज्यादा इजी है तो ये जो ब n है हम चाहे तो इसे
अपने इस आउटपुट के साथ भी लिख सकते हैं कि मैंने अपना कुछ भी काम लिखा आउटपुट करवाने के लिए और उसके बाद बै स्ल ए लिख दिया तो
इससे भी हमारा जो आउटपुट है वो कंपलीटली सेम होने वाला है अगर हम बै स् n के बाद कुछ प्रिंट करवाते हैं लेट्स सपोज मैंने
लिखा फ्रॉम अपना कॉलेज ये मेरा हेलो वर्ल्ड हो गया ये मेरा बै स्ल एन हो गया फिर मैंने लिखा फ्रॉम अपना कॉलेज तो बै
स्ल एन के बाद जो जो भी हम लिखेंगे वो नेक्स्ट लाइन पर प्रिंट होगा लेट्स चेक इट तो हेलो वर्ल्ड प्रिंट हो गया तो बै स् n
आ गया फिर यह छोटी सी स्पेस आई तो ये स्पेस यहां पे प्रिंट हो गई फिर हमारे पास फ्रॉम अपना कॉलेज आ गया और क्योंकि लास्ट
में हमने बै स्ल ए नहीं दिया तो इसीलिए परसेंटेज साइन दोबारा हमारे पास आ गया तो ये ट्रेलिंग कैरेक्टर को अगर हम हटाना
चाहते हैं तो उसके लिए हमेशा लास्ट में हमें या तो एंड लाइन देना पड़ेगा या फिर हमें बै n देना पड़ेगा हम चाहे तो कुछ भी
दे सकते हैं आल्सो अगर हमें मल्टीपल चीजें एक ही लाइन पर प्रिंट करवानी है तो उन्हें हम इस तरीके से भी प्रिंट करवा सकते हैं
सी आउट मैंने किया अपना कॉलेज उसके बाद मैंने एक स्पेस दिया और नेक्स्ट जो मैं चीज प्रिंट करवाना चाहती हूं दैट इज हेलो
वर्ल्ड एंड उसके बाद एंड ऑफ लाइन तो ये सारी की सारी चीजें सेम लाइन पर प्रिंट होगी क्योंकि बीच में ना एंड ऑफ लाइन आया
ना बीच में बैक स्ल ए आया एंड इसे क्लियर करेंगे पहले अपना कॉलेज आएगा स्पेस फिर हेलो वर्ल्ड आएगा एंड देन नेक्स्ट लाइन अब
यहां पर एक बार पॉज करना है एंड व्हाट यू हैव टू डू आपको अपना जो फर्स्ट नेम है व्हाट एवर योर फर्स्ट नेम मैं अपना नाम
लेकर एग्जांपल देकर बता देती हूं कि मेरा फर्स्ट नेम मैंने एक लाइन पर प्रिंट करवाया और नेक्स्ट
नेम लास्ट नेम नेक्स्ट लाइन पर प्रिंट करवाया और आपको सी आउट स्टेटमेंट एक ही लिखनी है एक सिंगल स्टेटमेंट से आपको इस
तरीके से दो लाइन में आउटपुट लेकर आना है एक बार पॉज करना है और खुद से इस तरीके से अपने फर्स्ट एंड लास्ट नेम को प्रिंट
करवाने की कोशिश करनी है आल्सो जब हम अपने फर्स्ट प्रोग्राम की बात कर रहे हैं तो एक और चीज भी डिस्कस कर लेते हैं बेसिकली अगर
हम इस लाइन को हटा दें तो ये जो बचा हुआ पूरा का पूरा कोड है इस कोड को हम एक स्पेशल नाम देते हैं हम इस कोड को कहते
हैं बॉयलर प्लेट कोड बॉयलर प्लेट कोड कहने का मतलब है कि ये स्टार्टिंग कोड है जो हमेशा लिखा ही लिखा जाएगा चाहे आप कोई भी
सवाल क्यों ना सॉल्व कर रहे हो तो जनरली हर एक डीएसए क्वेश्चन के अंदर हम इस तरीके के एक बॉयलर प्लेट कोड के साथ स्टार्ट
करते हैं आल्सो ये जो चीज मैंने लिखी है इसे प्रोग्रामिंग के अंदर एक कॉमेंट कहते हैं हम चाहे तो अपने कोड के अंदर कॉमेंट्स
लिख सकते हैं कॉमेंट्स कोड का पार्ट नहीं होते कॉमेंट्स को कंपाइलर इग्नोर कर देता है कॉमेंट्स में हमें कोई रूल फॉलो नहीं
करने पड़ते बेसिकली कॉमेंट्स को कंपाइलर के लिए नहीं ह्यूमंस के लिए लिखा जाता है जैसे एक बड़ी ऑर्गेनाइजेशन है बड़ी कंपनी
में अगर बहुत सारे डेवलपर्स काम कर रहे हैं और हर कोई अपना कोड लिख रहा है अब कोई नया डेवलपर अगर टीम के अंदर आता है तो उसे
समझना पड़ेगा पुराने लोगों का कोड अब पुराने लोगों का कोड समझने में हमें कॉमेंट्स हेल्प करते हैं जैसे मान लो किसी
ने लिख दिया य इंट मेन है तो उसके ऊपर कमेंट करके लिखा जा सकता है दिस इज द स्टार्टिंग पॉइंट ऑफ
एग्जीक्यूशन तो यहां से किसी नए डेवलपर को पढ़ के समझ आ जाएगा कि हमारा जो एग्जीक्यूशन है वो यहां से स्टार्ट हो रहा
है तो कॉमेंट्स बेसिकली इंग्लिश में लिखे जाते हैं हम चाहे तो किसी और दूसरी लैंग्वेज में भी लिख सकते हैं जो वीएस कोड
को समझ में आती हो पर जनरली प्रेफर टू राइट देम इन इंग्लिश एंड किसी भी इंग्लिश की स्टेटमेंट को कॉमेंट बनाने के लिए हमें
सिंपली अपनी स्टेटमेंट लिखनी होती है जैसे मैंने लिख दिया बॉयलर प्लेट कोड अब जैसे ही इसके आगे मैं ये दो स्लैशेस लगा दूंगी
वैसे ही कंपाइलर को समझ में आ जाएगा कि एक कमेंट है तो कमेंट्स को जनरली वीएस कोड ग्रीन कलर से दिखाता है और जली मोस्ट ऑफ द
एडिटर्स ग्रीन कलर से ही अपने कमेंट्स को दिखा रहे होते हैं वैसे कमेंट करने का एक और शॉर्टकट भी होता है हम एक साथ अगर
कंट्रोल स्लैश को प्रेस करें तो लाइन ऑटोमेटिक कॉमेंट हो जाएगी या मैक यूजर्स के लिए कमांड स्लैश को अगर प्रेस करें तो
लाइन ऑटोमेटिक कॉमेंट हो जाएगी और ऐसे ही दोबारा प्रेस करें तो अनकमेंट हो जाएगी अगर मुझे पूरे की पूरी फाइल को एक साथ
कॉमेंट करना है तो सिलेक्ट करना है और कंट्रोल स्लैश या कमांड स्लैश दबा देना है तो इस तरीके से हमारे कॉमेंट्स वर्क करते
हैं अब ये तो हमने बात कर ली कि बॉयलर प्लेट कोड क्या होता है हम आउटपुट कैसे करा सकते हैं हम कॉमेंट कैसे करा सकते हैं
नेक्स्ट इंपॉर्टेंट चीज जिस पर हम बढ़ने वाले हैं तो वो होते हैं हमारे वेरिएबल तो वेरिएबल काफी इंपॉर्टेंट क्रक्स होते हैं
c+ प्रोग्रामिंग का अब वेरिएबल कहने का क्या मतलब है वेरिएबल वैसे हमने ऑलरेडी यूज़ किए होंगे मैथ के अंदर मैथ के अंदर
अगर हमें कभी डिफाइन करना होता है a की वैल्यू पांच है तो हम लिख देते हैं a = 5 हमें कभी लिखना होता है x की वैल्यू 10 है
तो हम लिख देते हैं x = 10 y = 5 तो ये जो a होता है ये जो x होता है ये जो y होता है इन्हीं को मैथ के अंदर वेरिएबल कहते
हैं और इन्हीं को c+ के अंदर भी हम वेरिएबल कहते हैं जैसे अगर मुझे एज है मेरे पास मुझे एज 25 स्टोर करनी है तो मैं
एक वेरिएबल बना सकती हूं एज और उसके अंदर इक्वल टू 25 करके अपनी वैल्यू 25 को स्टोर करा सकती हूं अब जब भी हम कोई वेरिएबल
डिफाइन करते हैं उसके साथ उसका डेटा टाइप भी बताना पड़ता है पर डेटा टाइप को अभी हम बाद में पढ़ेंगे पहले वेरिएबल पे फोकस
करते हैं तो बेसिकली मैंने एक एज वेरिएबल बना दिया जिसके अंदर वैल्यू स्टोर हो जाएगी 25 तो वेरिएबल क्या होता है वेरिएबल
एक कंटेनर होता है जिसके अंदर हम कोई भी डटा स्टोर करा सकते हैं जरूरी नहीं है ये डेटा कोई नंबर हो ये डटा कोई कैरेक्टर भी
हो सकता है लेट्स सपोज मुझे अपना ग्रेड स्टोर कराना है तो ग्रेड इज इक्वल टू a जब भी कैरेक्टर्स लिखते हैं c+ प के अंदर तो
सिंगल कोट के अंदर लिखते हैं सिंगल कैरेक्टर जब भी बहुत सारे कैरेक्टर्स लिखने हैं ए बी सी डी तो उन्हें डबल कोट्स
के अंदर लिखने हैं ये चीज याद रखनी है तो ये जनरल कन्वेंशन है जो सिर्फ c+ प नहीं हम जावा के अंदर या दूसरी लैंग्वेजेस के
अंदर भी फॉलो करते हैं तो इस तरीके से से हम अपने वेरिएबल को क्रिएट कर सकते हैं अच्छा जब भी हमने कभी कोड के अंदर लिखा
जैसे लेट्स सपोज दिस इज माय कोड इस कोड के अंदर मैंने लिखा ए इ इक्वल 25 यह मैंने एक वेरिएबल को क्रिएट किया
अभी वैसे इसके अंदर एरर इसलिए आ रहा है क्योंकि मैंने वेरिएबल का टाइप नहीं बताया परट लेट्स सपोज मैंने ये वेरिएबल बना दिया
ए = 25 जैसे ही हम ये लिखेंगे कोड के अंदर वैसे ही क्या होगा कंप्यूटर की मेमोरी के अंदर मतलब ये जो 25 है ये कहां जाके स्टोर
होगा बेसिकली कंप्यूटर की जो मेमोरी है यानी कंप्यूटर की जो रैम है उसे हम एक बॉक्स की तरह इमेजिन कर सकते हैं इस बॉक्स
के अंदर डिफरेंट डिफरेंट स्लॉट्स होते हैं डिफरेंट डिफरेंट स्पेसेस होती हैं या फिर इसे इमेजिन कर सकते हैं बड़ी सी कोई जगह
जिसके अंदर किसी ने प्लॉट्स बहुत सारे दे दिए तो इसके अंदर मल्टीपल कंटेनर्स होते हैं लेट्स सपोज एक यह कंटेनर हो गया एक यह
कंटेनर हो गया एक यह कंटेनर हो गया यह जगह है सारी की सारी मेमोरी के अंदर और ये फिजिकली एजिस्ट करती है कंप्यूटर की
मेमोरी तो जैसे ही मैंने लिखा ए 25 एक कोई जगह होगी जो एज नाम के वेरिएबल को एज क्या है वेरिएबल का नाम है एलोकेट हो जाएगी और
उस जगह पर 25 वाली वैल्यू जाकर स्टोर हो जाएगी जैसे ही मैंने लिखा ग्रेड इ इक्वल ट a तो एक जगह होगी ग्रेड जिसका नाम ग्रेड
पड़ जाएगा एंड साथ में इसके अंदर हमारा जो ग्रेड ए है उसकी वैल्यू जाकर स्टोर हो जाएगी तो जब भी हम वेरिएबल को डिफाइन करते
हैं प्रोग्रामिंग के अंदर मेमोरी के अंदर वो इस तरीके से जाकर एक्चुअली स्पेस जो है उसको ऑक्यूप करते हैं तो वेरिएबल का
इंग्लिश के अंदर मतलब होता है जो चीज वेरी करती रहती है जो चीज चेंज कर सकती है मतलब हो सकता है कल को यह एज बढ़ के 26 हो जाए
या 28 हो जाए लेकिन हम इस एज वेरिएबल का नाम सेम रख सकते हैं हम उस कंटेनर का नाम सेम रख सकते हैं उसके अंदर की वैल्यू कल
को हो सकता है चेंज हो जाए अब जो हमारी वेरिएबल हैं उनको जनरली हम कुछ भी नाम दे सकते हैं हम चाहे तो एक वेरिएबल बना सकते
हैं कॉल्ड फुल नेम तो मैंने फुल नेम नाम का वेरिएबल बना दिया हम चाहे तो एक वेरिएबल बना सकते हैं कॉल्ड प्राइस तो इस
तरीके से अलग-अलग हम वेरिएबल बना सकते हैं और उनके के अंदर कुछ-कुछ वैल्यूज को हम स्टोर करवा सकते हैं अब जब भी हम वेरिएबल
का नाम डिफाइन करते हैं वो नाम या तो अंडरस्कोर के साथ स्टार्ट होना चाहिए या वो a से लेके z तक
किसी ना किसी इंग्लिश कैरेक्टर के साथ स्टार्ट होना चाहिए ऐसा नहीं है कि हम डिजिट के साथ स्टार्ट कर देंगे और फिर
अपने वेरिएबल को नाइन एज इस तरीके से कुछ नाम लिख देंगे हमें हमेशा या तो अंडरस्कोर के साथ स्टार्ट करना है या इस तरीके से
स्टार्ट करना है तो तभी हमारा जो वेरिएबल का नाम होगा वो एक वैलिड नाम होगा वेरिएबल नेम्स को c+ के अंदर हम एक स्पेशल टर्म
देते व्हिच इज कॉल्ड आइडेंटिफिकेशन को हम
आइडेंटिफिकेशन कंपाइलर जान सके कि आप अपने वेरिएबल में किस टाइप का डाटा स्टोर कराना चाहते हैं जैसे फॉर एग्जांपल मुझे अगर
वैल्यू स्टोर करानी है 25 और मैंने उसके लिए एक एज नाम का वेरिएबल बना दिया तो मुझे बताना पड़ेगा कि ये वैल्यू किस टाइप
की है अब मुझे ऑलरेडी पता है 25 इज व्हाट 25 इज एन इंटी जर वैल्यू तो मैं यहां पर लिख दूंगी इंट इंटी जर वो वैल्यूज होती
हैं जो स्टूडेंट भूल गए हैं जिसके अंदर डेसीमल वैल्यू नहीं होती जो कंप्लीट नंबर्स होते हैं वो नेगेटिव भी हो सकता है
पॉजिटिव भी हो सकता है ज़ीरो भी हो सकता है तो मैं हमेशा लिखूंगी इंट ए इज इक्व 25 जैसे यहां पर मैंने यह जो एज वेरिएबल
बनाया था इसके अंदर अभी एरर आ रहा है पर मैंने जैसे ही इंट लिख दिया एंड इसे सेव कर दिया अब कोई एरर नहीं है अब यह कंप्लीट
स्टेटमेंट है और यह बिल्कुल एग्जीक्यूट करेगी मेरे कोड के अंदर कोई एरर नहीं आएगा तो इस तरीके से हम अपने वेरिएबल को डिफाइन
कर सकते हैं और इस इंट से मुझे पता चल रहा है कि यह जो एज नाम का वेरिएबल है यह एक इंटी जर वैल्यू को स्टोर करता है हम चाहे
तो इस वेरिएबल को भी प्रिंट करवा सकते हैं वेरिएबल को प्रिंट करवाने के लिए अगर मैंने डबल कोट्स में लिखा इस तरीके से एज
तो एक बार देखते हैं क्या प्रिंट होगा तो हमारे लिए एज ही प्रिंट हो जाएगा मतलब डबल कोट्स के अंदर जो चीज लिखी जाती है वह
वैसे के वैसे प्रिंट होती है पर मुझे तो एज की वैल्यू प्रिंट करवानी है तो उसके लिए मुझे इन डबल कोड्स को हटाना पड़ेगा जब
मैं इन डबल कोड्स को हटाऊ तो c+ का कंपाइलर समझ जाएगा कि ये जो एज की मैं बात कर रही हूं इस पर हर भी करें अगर तो मैं
इंटी जर एज की बात कर रही हूं और इंटी जर एज के अंदर वैल्यू 25 है लेट अस सेव इट अगेन एंड रन द कोड तो इस बार हमारे पास
आउटपुट में आया है वैल्यू 25 के इक्वल तो इस तरीके से से वेरिएबल को हम प्रिंट भी करवा सकते हैं उनको हम कैलकुलेशन के लिए
भी यूज कर सकते हैं तो ये तो हो गया हमारा इंटी जर डेटा टाइप अब इंटी जर डेटा टाइप इंटरनली मेमोरी के अंदर चार बाइट्स की जगह
लेता है यह जो मैंने चार बाइट्स बोला इसका क्या मतलब है अभी जो मैं बताने वाली हूं यह बहुत इंपॉर्टेंट है इसको याद रखना है
बेसिकली कंप्यूटर्स पहली बात कर ली हमने 10 बार बात कर ली होगी कि कंप्यूटर्स 0 व नंबर सिस्टम पर चलते हैं कंप्यूटर को
सिर्फ इलेक्ट्रिकल सि सल 01 समझ में आता है तो कंप्यूटर के जो दिमाग की मैथ होती है ना कंप्यूटर के जो अंदर की मैथ होती है
वो हमारी वाली नॉर्मल मैथ नहीं होती कंप्यूटर की जो मैथ है वो बाइनरी नंबर सिस्टम को फॉलो करती है बाद में बाइनरी
नंबर सिस्टम्स प हम पूरा चैप्टर पढ़ेंगे पर अभी के लिए ऊपर ऊपर से हम चीजें देख रहे हैं थोड़ी सी कंप्यूटर्स बाइनरी नंबर
सिस्टम को फॉलो करते हैं और बाइनरी नंबर सिस्टम में एक जो सिंगल डिजिट होता है वो या तो जीरो हो सकता है या फिर वो वन हो
सकता है और इस सिंगल डिजिट को हम कहते हैं अपनी बिट सिंगल डिजिट को बिट कहा जाता है ये याद
रखना है अगर हम आठ बिट्स ले लें तो उसे हम अपनी एक बाइट कह देते हैं आठ बिट्स मतलब यहां पे एक बिट आएगी यहां
दूसरी आएगी तीसरी चौथी पांचवी छह सात और आठ जब हम ये आठ बिट्स का कलेक्शन लेते हैं मतलब जब हमारे पास इस तरीके का कुछ बाइनरी
नंबर आता है जिसमें सिर्फ रो और वन है इसको हम एक बाइट कह देते हैं तो चार बाइट्स कहने का मतलब है कि हमारे पास इस
तरीके के चार सेट्स हैं मतलब हमारे पास 8 * 4 32 बिट्स की जगह है एक इंटी जर नंबर को स्टोर
कराने के लिए अब अभी अगर यह मैथ आपको उतनी ज्यादा क्लियर ना हो तो घबराने की बात नहीं है बाद में बाइनरी नंबर सिस्टम हम
पढ़ेंगे तो और डिटेल में इन सारे टॉपिक्स को डिस्कस करेंगे पर अभी के लिए हम यह समझ सकते हैं कि इंटी जर के अंदर हमारे पास
चार बाइट्स की जगह होती है तो जब भी इंटी जर डिक्लेयर होता है मतलब जब भी हमने कोड के अंदर लिख दिया कि मेरे पास एक इंटी जर
वेरिएबल है ए जिसके अंदर वैल्यू स्टोर्ड है 25 तो मेमोरी के अंदर क्या होता है मेमोरी के अंदर इस तरीके की बिट्स की 32
स्पेसेस रिजर्व हो जाती हैं चाहे उनको सबको आप यूज मत करो पर वो रिजर्व हो जाती हैं और फिर उसमें जाके 25 को हम स्टोर
करवाते हैं इन द फॉर्म ऑफ रोज एंड वनस 25 ऐ ऐसे का ऐसे 25 स्टोर नहीं होता सिर्फ रो और वन की फॉर्म में 25 को कन्वर्ट करके
स्टोर किया जाता है अगर एगजैक्टली हमें 25 का बाइनरी फॉर्म जानना हो तो वो होता है 11001
ये 25 का बाइनरी फॉर्म होता है इसको कैसे निकालते हैं वो बाइनरी नंबर सिस्टम वाले चैप्टर में पढ़ेंगे तो मेमोरी के अंदर 25
स्टोर नहीं होता मेमोरी के अंदर 111 स्टोर होता है तो इस तरीके से हमारी कंप्यूटर की जो मेमोरी है वह काम करती है तो हर एक
डेटा टाइप का अपना मतलब होता है और वो मतलब यह होता है कि कितनी जगह मेमोरी के अंदर रिजर्व होनी चाहिए इस पर्टिकुलर
वेरिएबल के लिए इनफैक्ट इस चीज को हम चेक भी कर सकते हैं चेक करने के लिए लेट अस क्लियर दिस तो चेक करवाने के लिए हम लिखते
हैं साइज ऑफ एंड फिर अपने पैरेंस तो साइज़ ऑफ़ एक फंक्शन होता है जो किसी भी वैल्यू का साइज़ प्रिंट करवाता है बाइट्स के अंदर
तो इस चीज़ को हम प्रिंट करवा सकते हैं फंक्शंस क्या होते हैं उसको बाद में देखेंगे एंड इसे अब अगर मैं प्रिंट करवाऊं
तो मेरे पास वैल्यू प्रिंट होकर आएगी फोर फोर का मतलब है कि यह जो एज नाम का वेरिएबल है क्योंकि इसका डाटा टाइप इंटी
जर है तो यह चार बाइट्स की जगह लेता है मेमोरी के अंदर अब यह तो हो गया इंटी जर इसके अलावा और भी हमारे पास डिफरेंट
डिफरेंट डेटा टाइप्स होते हैं नेक्स्ट जो हमारे पास डेटा टाइप होता है व होता है कैरेक्टर कैर डेटा टाइप कैर
डेटा टाइप के अंदर हम सिंगल कैरेक्टर्स को स्टोर करवाते हैं यह जो मेमोरी के अंदर जगह ऑक्यूपाइड इज इक्वल टू 1 बाइट
कैरेक्टर को स्टोर करवाने के लिए हम सिंपली लिख सकते हैं लेट्स सपोज मुझे कैरेक्टर ग्रेड स्टोर करवाना है तो ग्रेड
और फिर सिंगल कोड्स लेट्स सपोज माय ग्रेड इज इक्वल टू a तो मैंने कैर ग्रेड इज इक्वल ट a को स्टोर करवा दिया हम चाहे तो
इस ग्रेड को भी हम प्रिंट करवा सकते हैं तो हमारे पास हमारा ग्रेड ए प्रिंट होकर आ जाएगा लेट अस क्लियर एंड रन दिस तो हमारे
पास a आ जाएगा a हमारे पास प्रिंट होकर आ गया हम चाहे तो यहां पर लोअर केस में भी a लिख सकते हैं तो हमारे पास लोअर केस वाला
a प्रिंट होकर आ जाएगा अब नंबर्स के लिए तो काफी स्ट्रेट फॉरवर्ड होता है कि जैसे 25 को हमने कन्वर्ट कर दिया 11001 के अंदर
तो हमें पता है कि नंबर को नंबर के अंदर ठीक है बाद में हम सीख लेंगे बाइनरी नंबर सिस्टम में कैसे कन्वर्ट करते हैं जो
हमारे कैरेक्टर्स होते हैं जैसे कैपिटल ए हो गया कैपिटल b हो गया z तक इन सारे के सारे कैरेक्टर्स को मेमोरी के अंदर स्टोर
कराने से पहले इन सब की एस् काई वैल्यू निकाली जाती है और फिर उस एस्का वैल्यू को मेमोरी के अंदर स्टोर कराया जाता है यह
काम हमें नहीं करना पड़ता यह काम ऑटोमेटिक हो रहा है अभी बस मैं आपको उसके पीछे की स्टोरी दिखाने की कोशिश कर रही हूं
बेसिकली एस्का होता है अमेरिकन स्टैंडर्ड कोड ऑफ इंफॉर्मेशन इंटरचेंज जिसके अंदर हर एक कैरेक्टर के लिए एक नंबर डिसाइडेड होता
है कि भाई ये जो a है इसका नंबर है 65 ये फिक्स है इसका नंबर हमेशा 65 ही रहेगा चाहे आप c+ में कोड कर रहे हैं चाहे आप
जावा में कोड कर रहे हैं ये जो b है इसका नंबर है 66 जो c है उसका नंबर है 67 उसी तरीके से अगर हमल ए b c d इनके नंबर्स
कंसीडर करें तो ये जो स्ल a होता है इसका नंबर होता है 97 स्मल b का नंबर होता है 98 स्मल c का नंबर होता है 99 एंड सो ऑन
तो जब कंपाइलर इस a को मेमोरी के अंदर स्टोर करा रहा होगा तो मेमोरी के अंदर वो a को स्टोर नहीं कराएगा वो इस 65 को 01 के
अंदर कन्वर्ट करेगा और फिर उस वैल्यू को स्टोर करा रहा होगा तो ये जो नंबर्स होते हैं इन सब की एक एस काई वैल्यू एसोसिएटेड
होती है जिसको बाद में हम डिटेल में समझने वाले हैं कि एस्का वैल्यूज को हम कैसे यूज़ कर सकते हैं हमारा एक डेडिकेटेड
चैप्टर होगा स्ट्रिंग्स के बारे में जिसमें हम बहुत सारे ऑपरेशंस करेंगे इन कैरेक्टर्स पर यूजिंग दीज एस्का वैल्यूज
पर अभी के लिए एक छोटा सा ग्लिम्स हमें मिल गया कि मेमोरी के अंदर हमारे जो कैरेक्टर्स होते हैं वो किस तरीके से
स्टोर्ड होते हैं आल्सो मेमोरी के अंदर पॉजिटिव नंबर्स एंड नेगेटिव नंबर्स के स्टोर होने का तरीका भी अलग-अलग होता है
उसको भी हम बाइनरी नंबर सिस्टम वाले चैप्टर के अंदर पढ़ेंगे नेक्स्ट जो हमारे पास डेटा टाइप होता है दैट इज़ फ्लोट डेटा
टाइप अभी तक इंटी जर के अंदर हम किसी भी नंबर जैसे 25 हो गया जैसे 100 हो गया उसको स्टोर करा सकते हैं लेकिन अगर हमें ऐसा
कोई वेरिएबल बनाना हुआ तो प्राइस जिसके अंदर वैल्यू है 1.99 डेसिमल वैल्यू आ गई तो जैसे ही हमारे पास फ्लोटिंग वैल्यूज आ
जाती है इनको हम फ्लोटिंग वैल्यूज कहते हैं डेसिमल के साथ वाली वैल्यूज को तो फ्लोटिंग वैल्यूज के लिए हमारे पास एक और
डेटा टाइप होता है कॉल्ड फ्लोट जैसे फॉर एग्जांपल आई वांट टू क्रिएट फ्लोट पाई प पाई पाई हमारे पास क्या होता है पाई
मैथ के अंदर होता है 3 14 तो इसमें डेसीमल वैल्यू आ जाती है तो इस तरीके से हम अपना फ्लोटिंग वेरिएबल बना सकते हैं अब जब भी
हम फ्लोट वेरिएबल को डिफाइन करते हैं उनकी वैल्यू लिखने के बाद हमें हमेशा वहां पर f लिखना होता है स्ल f लिख सकते हैं कैपिटल
f लिख सकते हैं बेसिकली जब तक हम ये नहीं लिखते तब तक कंपाइलर अज्यू करता है कि हम कोई डबल वैल्यू लिखना चाह रहे हैं पर हम
डबल नहीं लिखना चाह रहे हमारा डेटा टाइप फ्लोट है तो हम यहां पे f लिख देते हैं तो ये हमने फ्लोटिंग वैल्यू को डिफाइन कर
लिया यहां पर प पा लिख सकते हैं मैंने इसे कैपिटल इसलिए लिखा है क्योंकि जनरली पाई एक कांस्टेंट होता है और जनरली
प्रोग्रामिंग अच्छे जो सॉफ्टवेयर इंजीनियर्स होते हैं वो अगर कोई भी कांस्टेंट वेरिएबल डिफाइन करते हैं जिसकी
वैल्यू चेंज नहीं हो सकती क्योंकि पाई की वैल्यू हमेशा 3.14 ही रहेगी तो वो जो वेरिएबल होते हैं उनको कैपिटल से जनरली
कोड के अंदर दिखाया जाता है इसे क्लियर कर लेते हैं एंड रन करते हैं तो इस बार मेरे पास 3.14 प्रिंट होकर आ गया तो यह हमारा
फ्लोट डाटा टाइप हो गया फ्लोट भी चार बाइट्स की जो स्पेस है वो ऑक्यूपाइड है
बूल बूल इज गोइंग टू बी समथिंग इंटरेस्टिंग क्योंकि शायद ये प्रोग्रामिंग के अंदर ही हम देख रहे होते हैं बूल का
मतलब होता है बुलियन डेटा टाइप बुलियन का मतलब होता है या तो यह ट्रू स्टोर कराएगा या यह फॉल्स स्टोर करवाएगा इसके अंदर दो
ही वैल्यूज हो सकती हैं ट्रू या फॉल्स और यह जो मेमोरी लेता है वो होती है एक बाइट की मेमरी जैसे फॉर एग्जांपल मैंने एक बलिन
वेरिएबल बनाया बूल एंड इसके लिए मैंने लिख दिया इज सेफ इज इट सेफ इज इट सेफ के लिए या तो वैल्यू ट्रू हो सकती है तो उसके लिए
हम स्मल से ट्रू लिख देंगे या फिर वैल्यू फाल्स हो सकती है अच्छा जब भी हम अपने बुलियन वेरिएबल को प्रिंट करवाते हैं
लेट्स सपोज मैं इस सेफ को प्रिंट करवाऊं तो हमारे पास ट्रू या फाल्स प्रिंट होकर नहीं आता
इंटरनली जो ट्रू वैल्यू होती है इट ट्रांसलेट्स टू वन एंड जो फाल्स वैल्यू होती है इट ट्रांसलेट्स टू जीरो मतलब जब
हम फॉल्स को अब ये फॉल्स है इससे इसको जब हम प्रिंट करवाएंगे तो हमारे पास जीरो प्रिंट होगा या फिर इसको अगर हम ट्रू करके
प्रिंट करवाएंगे तो हमारे पास वन प्रिंट होगा क्योंकि जब मेमोरी के अंदर ट्रू या फाल्स स्टोर होता है तो ट्रू या फाल्स
स्टोर नहीं होता उसमें तो रो वन स्टोर होता है तो जब मेमोरी के अंदर ट्रू स्टोर करना होता है तो कंप्यूटर वन स्टोर करता
है जब मेमोरी के अंदर जीरो फॉल्स स्टोर करना होता है तो कंप्यूटर जीरो स्टोर करता है तो दैट इज व्हाई जब हम इने प्रिंट
करवाते हैं हमारे पास ट्रू फॉल्स नहीं आता हमारे पास रो और वन वाली वैल्यूज प्रिंट होकर आती है तो ये हमारा बुलियन डेटा टाइप
हो गया जिसको बाद में डिटेल में यूज करेंगे और एक हमारे पास डेटा टाइप होता है कॉल्ड डबल डबल का साइज फ्लोट के डबल होता
है यानी आ बाइट्स होता है और इसके अंदर भी हम फ्लोटिंग पॉइंट यानी डेसिमल वाले नंबर्स को स्टोर कराते हैं बाय डिफॉल्ट
कोई भी नंबर अगर हम लिखेंगे जैसे मैंने लिख दिया 1.99 तो ये जो नंबर है ये बाय डिफॉल्ट एक
डबल नंबर होगा तो हम लिख सकते हैं डबल प्राइस इ इक्वल टू 100.9 तो इस तरीके से मैंने एक डबल वेरिएबल को क्रिएट कर दिया
तो मेजॉरिटी कोडम जो लिखते हैं वो इन्हीं डेटा टाइप्स को यूज करके लिख रहे होते हैं और ये जो हमारे सारे के सारे डेटा टाइप्स
होते हैं इन्हें एक स्पेशल टर्म दी जा आती है इन्हें हम बोलते हैं अपने प्रिमिटिव डेटा टाइप्स बाद में हम नॉन प्रिमिटिव
डेटा टाइप्स भी पढ़ेंगे जिसके अंदर हम अरेज पढ़ रहे होंगे जिसके अंदर हम स्ट्रिंग को पढ़ रहे होंगे एंड और भी डेटा
टाइप्स पढ़ेंगे पर ये हमारे प्रिमिटिव यानी सबसे बेसिक वाले डेटा टाइप्स होते हैं तो इन्हीं पांच डेटा टाइप्स को हम
बार-बार बार-बार अपने प्रोग्राम्स के अंदर यूज़ कर रहे होंगे तो ये तो हो गया ऑल अबाउट वेरिएबल एंड डेटा टाइप्स इसके बाद
नेक्स्ट चीज जो हम करने वाले हैं दैट इज टाइप कास्टिंग तो कभी-कभी डाटा को हमें एक टाइप से दूसरे टाइप के अंदर कन्वर्ट करना
होता है जिसके लिए हम टाइप कास्टिंग के कांसेप्ट को यूज़ करते हैं अब टाइप कास्टिंग के दो तरीके होते हैं सबसे पहला
होता है टाइप कन्वर्जन और दूसरा होता है टाइप कास्टिंग कन्वर्जन कैसा प्रोसेस होता है कन्वर्जन एक इंप्लीड प्रोसेस होता है
इंप्ली मतलब वह प्रोग्रामर को हमें नहीं करना पड़ता वह कंपाइलर ऑटोमेटिक कर देता है ऑटोमेटिक कहने का मतलब है जब भी हम एक
छोटे टाइप की वैल्यू को किसी लार्ज टाइप की वैल्यू के अंदर कन्वर्ट करना चाहते हैं तो यह प्रोसेस ऑटोमेटिक है इसमें कोई डटा
लॉस तो हो नहीं रहा लेट्स सपोज मैंने फ्लोट के अंदर 3.14 स्टोर कराया था और ये 3.1 कितनी मेमोरी के अंदर स्टोर हुआ था
फ्लोट की जो मेमोरी होती है वो होती है चा बाइट्स की तो ये चार बाइट्स की मेमोरी के अंदर स्टोर हो गया था अब इसे मुझे अगर डबल
के अंदर कन्वर्ट करना है तो डबल तो बड़ा डटा टाइप है अब आठ बाइट्स की मेमोरी के अंदर 4 बाइट का डाटा तो बहुत आसानी से
जाके स्टोर हो जाएगा आधी मेमोरी बच भी जाएगी तो ये जो कन्वर्जन है ये बहुत आसान होगा तो इसको हम इंप्लीड कन्वर्जन कहते
हैं यानी ये ऑटोमेटिक कंपाइलर हमारे लिए कर देता है और क्या एग्जांपल हो सकता है इसका जैसे मान लो मुझे किसी कैरेक्टर की
वैल्यू को कन्वर्ट करना है टू सम इंटी जर वैल्यू तो वो प्रोसेस बहुत आसान है क्योंकि कैरेक्टर एक बाइट लेता है इंटी जर
चार बाइट लेता है एक बाइट के डाटा को चार बाइट की जगह में फिट करना बहुत आसान है इसका एग्जांपल भी देखते हैं लेट्स क्रिएट
टू वेरिएबल या इनफैक्ट एक सिंगल वेरिएबल क्रिएट करते हैं मैंने लिया अपना ग्रेड और अभी हमारा ग्रेड है a के इक्वल अब इस a को
हम एक इंटी जर के अंदर कन्वर्ट करना चाहते हैं तो इंटी जर वैल्यू ले देते हैं और सिंपली लिखेंगे इंटी जर वैल्यू इज इक्वल
टू ग्रेड जब हम इस तरीके से लिखते हैं तो c+ प के अंदर क्या होता है राइट साइड की जो वैल्यू होती है वो लेफ्ट में आकर स्टोर
हो जाती है जैसे जब हमने ग्रेड डिफाइन किया तो ये राइट का ए जो है ये इस वेरिएबल के अंदर जाकर स्टोर हो गया तो अब हमारे
पास इंटी जजर वैल्यू आ गई होगी जब हम सी आउट करवाएंगे इस वैल्यू को यू विल सी समथिंग इंटरेस्टिंग एंड हमारे पास आकर
प्रिंट हो गया 65 मैंने शुरू में भी बताया था कैपिटल ए की एस काई वैल्यू क्या होती है कैपिटल ए की एस काई वैल्यू होती है 65
के इक्वल वही वैल्यू मेरे पास आकर प्रिंट हो गई है अगर यहां पर स्ल a होता तो स्ल a की ए काई वैल्यू 97 होती है तो जब इस
कैरेक्टर को हम इंटी जर के अंदर कन्वर्ट करेंगे तो आई एम गोइंग टू गेट 97 इन आउटपुट तो इससे हमें एक चीज और कंफर्म हो
रही है कि हां मेमोरी के अंदर एस काई वैल्यूज ही जाके प्रिंट हो रही है सही बताया था उसके साथ में हमें ये भी दिख रहा
है कि इंप्ली सिट कन्वर्जन हमें कुछ करना नहीं पड़ा हमें तो बस बताना है कि इसकी वैल्यू भाई इसमें जाके स्टोर कर दो
कंपाइलर ने ऑटोमेटिक स्टोर कर दी अब जैसे टाइप कन्वर्जन इंपलीसिट हो होता है हमारे पास एक और टर्म होती है कॉल्ड टाइप
कास्टिंग जो एक्सप्लिसिट होता है एक्सप्लिसिट मतलब टाइप कास्टिंग प्रोग्रामर मैनुअली करता है ऑटोमेटिक
कंपाइलर नहीं करता जब भी हम किसी एक टाइप से दूसरे टाइप के अंदर कन्वर्ट करना चाहते हैं तो उस चीज को हम एज अ प्रोग्रामर
फोर्स भी कर सकते हैं भले कंपाइलर अलाउ कर रहा हो या ना कर रहा हो और जनरली टाइप कास्टिंग जो यूज होती है व बिग डटा टाइप
से स्मॉल डेटा टाइप के अंदर डाटा को कन्वर्ट करने के लिए यूज होती है जैसे फॉर एग्जांपल हमने बनाया एक डबल वेरिएबल कॉल्ड
प्राइस तो प्राइस के अंदर हमने स्टोर कर दिया 1.99 अब हम एक इंटी जर वेरिएबल न्यू
प्राइस के अंदर प्राइस की वैल्यू स्टोर करना चाहते हैं तो अब हम चाहते हैं कि ये जो डबल है ये इंटी जर में टाइपकास्ट हो
जाए तो बेसिकली बड़ी वैल्यू को हम छोटी वैल्यू में टाइपकास्ट करना चाहते हैं तो इसके लिए हम लिख सकते हैं यहां पर इस
तरीके से इंट तो ये हमारी एक डबल वैल्यू है जिसको हम इंट में टाइप कास्ट करने की कोशिश कर रहे हैं और ये टाइप कास्ट करने
के लिए हम पैरेंस के अंदर अपना नया डटा टाइप बताते हैं अब जब हम सी आउट करेंगे न्यू
प्राइस विदाउट स्पेस तो हमारे पास हमारी नई प्राइस की वैल्यू आकर प्रिंट हो जाएगी व्हिच इज 100 अब अनलाइक मैथ मैथ के अंदर
तो क्या होता है मैथ के अंदर अगर किसी ने लिख दिया 1.99 तो उसको राउंड ऑफ करके हम 101 लिख
सकते हैं लेकिन c+ प में ऐसा नहीं होता c+ प में चाहे 100 के पॉइंट के बाद कितने भी नाइन लगे हो वो सारे के सारे कट हो जाएंगे
और सिर्फ डेसीमल से से पहले वाला जो नंबर है उसको इंटी जर के अंदर स्टोर किया जाता है यह बहुत इंपॉर्टेंट चीज है c+ प के
अंदर चाहे आप 100.0 ले चाहे आप 1.99 लें दोनों को जब इंटी जर के अंदर
टाइपकास्ट किया जाएगा तो दोनों की ही वैल्यू 100 निकल कर आ रही होगी तो ये जो डेसीमल के बाद वाली वैल्यूज होती हैं
कंप्लीट इग्नोर होती है सो दिस इज़ एन एग्जांपल ऑफ टाइप कास्टिंग टाइप कास्टिंग हम जनरली काफी सारे डिफरेंट डाटास के लिए
कर सकते हैं और टाइप कास्टिंग को हम आगे देखेंगे कि कैसे हम यूज़ कर सकते हैं प्रैक्टिकली टाइपकास्टिंग को पर इस तरीके
से कन्वर्जन एंड टाइप कास्टिंग हो सकती है इंपॉर्टेंट चीज याद रखने वाली यह है कि कन्वर्जन इंप्ली होता है कंपाइलर करके
देता है टाइप कास्टिंग एक्सप्लिसिट होती है मतलब एक्सप्लिसिट मतलब अलग से जिसको हमें एज अ प्रोग्रामर करना पड़ता है तो ये
तो हमने टाइप कास्टिंग को पढ़ लिया नेक्स्ट हम पढ़ने वाले हैं कि कैसे c+ 10 के अंदर हम इनपुट ले सकते हैं शुरुआत में
फर्स्ट लेक्चर में हमने बात की थी कि हमारा जो भी प्रोग्राम हम लिखते हैं c के अंदर उसके अंदर कुछ ना कुछ लॉजिक लिखा
होता है और इस लॉजिक का काम होता है कि इसे कुछ इनपुट लेना है उस परे कुछ ऑपरेशन परफॉर्म करने हैं और फिर कुछ आउटपुट
रिटर्न करना है तो अब हमने आउटपुट रिटर्न करना सीख लिया हमने थोड़ा बहुत लॉजिक करना भी सीख लिया है कि किस तरीके से हम टाइप
कास्ट कर सकते हैं किस तरीके से वेरिएबल बना सकते हैं अब हम बात करते हैं हम इनपुट कैसे ले सकते हैं वैल्यूज को वैल्यूज को
इनपुट लेने के लिए जैसे हमने सी आउट लिखा था आउटपुट के लिए वैसे ही हम लिखते हैं सी इन फॉर इनपुट और हम फिर लिखते हैं अपने
ग्रेटर दन ग्रेटर दन वाले दो सिंबल और फिर जिस जिस भी वेरिएबल के अंदर हम वो डाटा स्टोर कराना चाहते हैं हम उस वेरिएबल का
नाम लिख देते हैं फॉर एग्जांपल हमारे पास एक एज वेरिएबल है पर अभी हमारे पास एज की वैल्यू नहीं है तो हमने यहीं पर सेमीकलन
लगा दिया और बस कोड को बता दिया कि एज नाम का एक इंटी जर वेरिएबल एजिस्ट करता है अभी इस एज के अंदर कोई वैल्यू नहीं है अभी
इसके अंदर कह सकते हैं गार्बेज वैल्यू है इनफैक्ट इसकी वैल्यू अगर अभी मैं प्रिंट भी करवाऊं तो इफ आई डू सी आउट एज तो कोई
भी रैंडम वैल्यू है वो आकर प्रिंट हो सकती है हो सकता है जीरो भी प्रिंट हो जाए हो सकता है रैंडम गार्बेज वैल्यू प्रिंट हो
जाए तो यहां पे मेरे पास रैंडम कुछ भी वैल्यू आगे प्रिंट हो गई है इसको हम गार्बेज वैल्यू कहते हैं तो अब अगर एज की
वैल्यू मुझे यूजर से चाहिए यूजर से वैल्यू लेने का मतलब होता है जैसे हम कोई वेबसाइट यूज़ करते हैं हम
google-chrome एज अ सॉफ्टवेयर एज अ प्रोग्राम हमसे इनपुट ले रहा है तो वैसे ही हम भी ऐसा प्रोग्राम बना सकते हैं जो
यूजर से इनपुट ले तो यूजर कुछ भी वैल्यू इनपुट कर सकता है तो वैल्यू इनपुट लेने के लेट्स सपोज मैंने पहले सी आउट किया एंटर द
एज इस तरीके से मैंने सी आउट कर दिया एंड इनफैक्ट यहां पर नेक्स्ट लाइन नहीं देते एंड यहां पर हम सी इन कर सकते हैं एज
तो बेसिकली सी आउट करके हम बता रहे हैं यह चीज एंटर करो और सी इन में हम उस जो भी डाटा आया होगा एंटर होके उसको एज नाम के
वेरिएबल में स्टोर करवा रहे हैं और फिर सी आउट करवा देते हैं योर एज इज एंड यहां पर हम अपनी एज की वैल्यू को
प्रिंट करवा सकते हैं आई होप यह इतना पार्ट हमें समझ में आया होगा क्योंकि सी आउट को हम बहुत बार लिख चुके हैं तो एक
बार इस कोड को रन करते हैं मेरे पास पहले तो प्रिंट होकर आया है एंटर द एज अब जब तक मैं कुछ इनपुट नहीं एंटर करूंगी कोड आगे
नहीं बढ़ेगा तो मुझे कुछ इनपुट एंटर करना पड़ेगा लेट्स सपोज मैंने एंटर किया ए = 25 यहां पे टाइप करके जैसे इसको एंटर करेंगे
वैसे ही अब ये लाइन एग्जीक्यूट हो जाएगी योर एज इज 25 तो इस तरीके से हम इनपुट आउटपुट एंड कुछ ऑपरेशन के साथ अपना पूरा
का पूरा एक प्रोग्राम बना सकते हैं अब जैसे हमने यह प्रोग्राम बनाया लेट्स सपोज मुझे प्राइस स्टोर करवाना है किसी चीज का
तो हम लिख सकते हैं एंटर द प्राइस तो यहां पर हमारे पास प्राइस एंटर हो जाएगा और प्राइस कोई फ्लोटिंग या कोई
डबल वैल्यू हो सकती है और फिर हम क्या कर देंगे हम लिख देंगे योर या यू
एंटर्ड प्राइस इक्वल टू एंड यहां पर हम प्राइस की वैल्यू को प्रिंट करवा देंगे लेट्स सेव इट क्लियर इट एंड रन हमारा
प्राइस लेट्स सपोज हमने 99.99 एंटर किया तो यू एंटर्ड प्राइस = 99.99 तो इस तरीके से इनपुट भी लेना बहुत आसान होता है सिर्फ
सीन लिखना पड़ता है आउटपुट भी देना बहुत आसान होता है सिर्फ सी आउट लिखना पड़ता है ये जो हमारे ऑपरेटर्स होते हैं ये
ओवरलोडेड ऑपरेटर्स इनको हम कहते हैं पर ओवरलोडिंग हमें अभी समझ में नहीं आएगी वो ऑब्जेक्ट ओरिएंटेशन का कांसेप्ट है
ऑब्जेक्ट ओरिएंटेशन भी अभी समझ में नहीं आएगा उसका काफी बड़ा हम डेडिकेटेड चैप्टर करेंगे जिसमें डिटेल डि में बात करेंगे ये
जो सी इन सी आउट होते हैं कुछ स्टूडेंट्स को कंफ्यूजन होता है जिन्होंने ऑलरेडी थोड़ी बहुत प्रोग्रामिंग की होती है कि ये
फंक्शंस होते हैं पर सी इन सी आउट एक्चुअली फंक्शंस नहीं होते ये ग्लोबल ऑब्जेक्ट्स होती हैं ऑब्जेक्ट्स क्या होती
हैं उसको भी हम अपने ऑब्जेक्ट ओरिएंटेशन वाले चैप्टर में करेंगे तो ये सारी चीजों के बारे में अभी काफी सारी ऐसी चीजें हैं
जिनको आपको बस भरोसा करके फेथ करके सीखना पड़ेगा कि हां ठीक है ये मैंने कर लिया और ये चीज ऐसे ही होती है क्योंकि अभी हम
रूल्स सीख रहे हैं अभी हम ए बी सी डी सीख रहे हैं अभी हम ए एंड द सीख रहे हैं अभी हम स्टॉप लगाना सीख रहे हैं बाद में
सीखेंगे कि काफी सारी चीजें जो हमने की थी उनका क्या लॉजिक था वो हमें डिटेल में बाद में समझ में आ रहा होगा तो अब यहां तक
हमने इनपुट को खत्म कर लिया है तो अब नेक्स्ट एक और बहुत ही इंपॉर्टेंट कांसेप्ट जिसको हम कवर करेंगे यह है हमारे
ऑपरेटर्स ऑपरेटर्स का काम होता है कुछ ऑपरेशन को परफॉर्म करना ऑपरेटर्स को हमने मैथ के अंदर भी किया हुआ है मैथ के अंदर
हम लिखते थे a + b तो इसमें प्लस क्या होता था ये प्लस एक ऑपरेटर होता था हम लिखते थे a - b तो ये माइनस एक
ऑपरेटर होता था प्लस का काम होता है दो वैल्यूज को ऐड करना माइनस का काम होता है एक वैल्यू में से दूसरी वैल्यू को सबस्टैक
करना तो इस तरीके से मैथ के अंदर हमने बहुत सारे ऑपरेटर सीखें वैसे ही c+ प के अंदर भी काफी सारे ऑपरेटर्स होते हैं
जिसमें से फर्स्ट टाइप ऑफ ऑपरेटर होते हैं एरिदमेटोसस एरिदमेटोसस होता है मैथमेटिकल कैलकुलेशंस
को परफॉर्म करना एरिदमेटोसस में एक प्लस हो जाता है एक माइनस हो जाता है एक मल्टीप्लाई होता है जिसको हम ये स्टार
वाले सिंबल से दिखाते हैं इस स्टार सिंबल को हम एस्टरिस कहते हैं और एक होता है डिवाइड यह
चारों तो हमने मैथ में पढ़े हुए हैं इनको यूज करना बहुत आसान है इनफैक्ट जल्दी से एक डेमोंस्ट्रेट भी ले लेते हैं लेट्स
सपोज हमारे पास दो वैल्यूज हैं इंटी जर a = 5 एंड इंटी जर b = 10 इनफैक्ट इन वैल्यूज को हम यहीं पर लिख सकते हैं b =
10 एक सिंगल लाइन में कॉमा से सेपरेट करके हम मल्टीपल वेरिएबल डिफाइन कर सकते हैं सेम टाइप के तो अब मुझे सी आउट करना है
सबसे पहले तो a + b तो मैं इस तरीके से पेंसिस में या सिंपल तरीका लेते हैं पहले इंट सम इ a + b मैंने क्या किया a + b दो
वेरिएबल लिए और उनका सम कैलकुलेट किया और अब मैं इस सम को प्रिंट करवा सकती हूं लेट्स रन दिस कोड तो मेरे पास 15 आकर
प्रिंट हो गया अब यहां पर सम क्रिएट करने की बजाय मैं डायरेक्टली यहां पर a + b भी लिख सकती थी पेंसिस के साथ तो डायरेक्टली
a + b की वैल्यू मेरे पास प्रिंट होकर आ जाती तो यहां पर पहले तो प्रिंट करवा लेते हैं सम इज इ
एंड फिर a प् बी लेट्स क्लियर इट लेट्स रन तो सम इ इक्वल टू 15 तो ये प्लस हो गया ऐसे ही हम माइनस कर सकते हैं माइनस को
क्या कहेंगे माइनस को कहेंगे डिफरेंस इ इक्वल टू a - बी या a को 10 कर लेते हैं बी को 5 कर लेते हैं तो फिर वैल्यू
पॉजिटिव आ जाएगी 10 - 5 क्या होगा 5 के इक्वल होगा तो सम इस बार 15 हो गया डिफरेंस हमारे पास फ हो गया ऐसे ही हम
दोनों को मल्टीप्लाई भी कर सकते हैं मल्टीप्लाई कर करने के लिए मल्टीप्लिकेशन को प्रोडक्ट कहते हैं a * b लिख सकते हैं
चाहे तो स्पेस भी दे सकते हैं चाहे तो बिना स्पेस के भी लिख सकते हैं यह एस्टरिस स्टार सिंबल होता है जो हमें दोनों का
मल्टीप्लिकेशन देता है तो प्रोडक्ट हो गया 5 * 10 10 * 5 50 के इक्वल ऐसे ही हम डिवाइड भी कर सकते हैं डिवाइड जब हम
करेंगे तो हमारे पास क्श आएगा या डिवीज़न कह लेते हैं इसको ज्यादा कॉम्प्लिकेट नहीं करते a / बा b के लिए हम
सिंपली स्लैश लिखना होता है तो 10 / 5 हमारे पास टू प्रिंट होकर आएगा इसको क्लियर कर लेते हैं रन करते हैं तो डिवीजन
में हमारे पास टू प्रिंट होक आ गया अब ये तो चार काफी बेसिक मैथमेटिकल ऑपरेशन है इसके अलावा एक और हमने सीखा था लास्ट
क्लास के अंदर व्हिच इज द रिमाइंडर ऑपरेटर रिमाइंडर ऑपरेटर को हम मॉड्यूल कहते हैं तो अगर हम a मॉडलो b लिखते हैं तो यह हमें
a को जब हम b से डिवाइड करेंगे तो हमारे पास क्या रिमाइंडर बचेगा उस रिमाइंडर की वैल्यू लाकर देता है तो जब हम 10 को फ से
डिवाइड करेंगे तो रिमाइंडर जीरो बचता है तो एक बार देखते हैं मॉडलो का क्या आंसर हमारे पास आएगा दिस इज सपोज टू बी मॉडलो
मॉडलो के लिए हम लिखेंगे a मॉडलो बी तो ये डिवाइड वाला ऑपरेशन हो गया और इसका आंसर आना चाहिए जीरो के इक्वल क्लियर दिस एंड
रन तो मॉड्यूल आ गया है जीरो के इक्वल अगर यहां a की जगह 11 होता 10 नहीं होता तो 11 / बा 5 का जो रिमाइंडर होता है वो वन के
इक्वल होता है तो इस बार हमारे पास वैल्यू आ जाएगी वन के इक्वल तो इस तरीके से हम हमारे डिफरेंट डिफरेंट जो एरिथ मेे िक
ऑपरेटर्स होते हैं वो काम करते हैं तो हमारे पास ये मेजर पांच एरिथ मेे ऑपरेशंस होते हैं अब इसमें से जो हमारा डिवीजन
ऑपरेटर होता है उसको एक बार थोड़ा सा और डिटेल में देखते हैं जैसे लेट्स सपोज अगर हम मैथ के अंदर फाइव को टू से डिवाइड करते
हैं इंटी जर फाइव को इंटी टू से डिवाइड करते हैं तो आंसर हमारे पास आता है 2.5 या फिर अगर हम 10 को थ से डिवाइड करते हैं तो
आंसर हमारे पास आता है 3.33 इस तरीके से लेकिन c+ प् के अंदर ऐसा नहीं होता c+ प के अंदर अगर हम फाइव को टू से डिवाइड करें
तो हमारे पास आंसर आएगा टू के इक्वल ये जो डेसीमल के बाद वाला हिस्सा है इसको कंपलीटली कट कर दिया जाता है जब हम 10 को
थ से डिवाइड करेंगे तो आंसर आएगा थ्र के इक्वल डेसिमल के बाद वाले हिस्से को कंपलीटली कट कर दिया जाता है उसको देखते
हैं एक बार होते हुए लेट्स सपोज हम सी आउट कराना चाहते हैं वी वांट टू सी आउट 5 डिवाइड बाय
2 तो यहां पर जब हम हर करेंगे मुझे पता है फ क्या है इंटी जर वैल्यू है टू क्या है यह भी इंटी जर वैल्यू है तो जब इनको
डिवाइड किया जाएगा तो जब इंटी जर को इंटी जर के साथ डिवाइड करते हैं c+ प के अंदर तो आंसर भी एक इंटी जर वैल्यू ही आता है
तो इसीलिए इंटी जर के अंदर डेसीमल नहीं होंगे तो इन डेसीमल को पूरा इग्नोर कर देंगे राउंड ऑफ नहीं करेंगे मैथ की तरह
इसे जब हम रन करेंगे तो वी आर गोइंग टू गेट अ वैल्यू ऑफ टू नॉट 2.5 लेकिन लेट्स सपोज अगर हमें किसी केस में 2.5 चाहिए
होता 2.5 लाने के लिए हमें फिर इंटी जर को इंटी जर से डिवाइड कर करने की जगह या तो हमें फ्लोट को इंटी जर से डिवाइड करना
पड़ेगा या हमें डबल को इंटी जर से डिवाइड करना पड़ेगा या फिर इसका अपोजिट करना पड़ेगा यानी या तो इंटी जर को फ्लोट से
डिवाइड कर दो या इंटी जर को डबल से डिवाइड कर दो तो जब भी डिवीजन होता है जब भी ऐड होता है सबस्टैक होता है मल्टीप्लाई होता
है तो दोनों अगर डिफरेंट डिफरेंट डेटा टाइप्स हैं जैसे यहां डिफरेंट डेटा टाइप्स हैं तो उनमें से जो बड़े वाला डेटा टाइप
होता है रिजल्ट उसी के अंदर आएगा तो अगर इंटी जर को फ्लोट से डिवाइड करेंगे तो रिजल्ट फ्लोट होगा फ्लोट वैसे साइज वाइज
बड़ा नहीं होता दोनों चार बाइट्स ही लेते हैं लेकिन क्योंकि फ्लोट के अंदर डेसिमल पॉइंट ज्यादा होते हैं प्रेसीजन ज्यादा
होती है तो इसीलिए इसको हम बड़ा डाटा टाइप लेंगे तो इनका रिजल्ट भी फ्लोट होगा इस तरीके का डिवीजन होगा तो रिजल्ट डबल होगा
इस तरीके का डिवीजन होगा रिजल्ट फ्लोट होगा इस तरीके का डिवीजन होगा रिजल्ट डबल होगा और ये सिर्फ डिवीजन के अंदर नहीं
मल्टीप्लिकेशन या दूसरे ऑपरेशंस के अंदर भी ऐसा ही होता है तो बेसिकली अब 5 डिवाइड बा 2 को या तो हम यहां पर इस तरीके से
लिखें इंट a = 5 एंड इसको डबल कह देते हैं डबल ब
इ 2 या तो हम इस तरीके से लिखें फिर a और b को डिवाइड करें तो एक तो यह तरीका है आंसर के अंदर 2.5 लाने का a / बा b तो
आंसर के अंदर इस बार 2.5 आ गया एक और तरीका है कि अगर कभी हमारे पास डायरेक्ट वैल्यूज होती है 5 / बा 2 तो किसी भी एक
वैल्यू को हम टाइप कास्ट कर सकते हैं यहां हमें समझ में आएगा टाइप कास्टिंग क्यों इंपॉर्टेंट है जैसे लेट्स सपोज ये जो
फ्लोट है फ्लोट के आगे मैं लिख देती हूं डबल तो मैंने क्या किया टू के डायरेक्टली आगे मैंने डबल लिख दिया तो मैंने टू को
टाइप कास्ट कर दिया टू डबल तो अब जो मेरा आंसर होगा मुझे अब ये a और b यूज करने की जरूरत नहीं है अब जो आंसर होगा वो फिर से
2.5 आएगा क्योंकि इस बार इंटी जर को डबल के साथ डिवाइड किया जा रहा है तो इस तरीके से टाइप कास्टिंग हमें हेल्प करती है जब
हमारे पास इंटी जर वैल्यू होती है या किसी दूसरे डेटा टाइप की वैल्यूज होती हैं पर हमें आंसर किसी और डेटा टाइप में चाहिए तो
उनको फिर हमें टाइप कास्ट करना पड़ता है अच्छा जब हम टाइप कास्टिंग करते हैं तो अगर हम ऐसा कुछ कर रहे हैं इंटी जर आंसर
इज इक्वल टू इसी वैल्यू को ले लेते हैं और आंसर को प्रिंट करवाते हैं लेट्स सेव इट एंड रन इट तो यहां पे आंसर टू आएगा यहां
पे आंसर 2.5 नहीं आएगा और उसका रीजन क्या है उसका रीजन यह है कि ठीक है राइट साइड में तो आपने अपना डबल वैल्यू का आंसर
निकाल लिया था 2.5 लेकिन जब लेफ्ट में उस 2.5 को इस वेरिएबल के अंदर स्टोर किया जाएगा तो ये वेरिएबल तो इंटी जर टाइप का
है तो जब 2.5 को आप एक छोटे कंटेनर इंटी के अंदर स्टोर करोगे तो डेफिनेटली डेसिमल पॉइंट हट जाएंगे तो इसीलिए आंसर के अंदर
डेसिमल पॉइंट प्रिंट होकर नहीं आए तो जब भी चीजें हो रही होती हैं प्रोग्राम्स के अंदर उनके पीछे हमेशा एक लॉजिक होता है और
वो लॉजिक समझना बहुत इंपॉर्टेंट होता है सिर्फ ऐसे ही ले नहीं लेना कि ठीक है हां आउटपुट आ गया बस ठीक है इसका कोई लॉजिक
नहीं होगा हमेशा चीजों का लॉजिक होता है अब क्योंकि हम धीरे-धीरे जर्नी के अंदर बढ़ रहे हैं तो कुछ चीजों के लॉजिक हो
सकता है हम इस लेक्चर के अंदर ना कवर करें हम अगले लेक्चर के अंदर कवर करें या उससे अगले लेक्चर के अंदर कवर करें लेकिन हर एक
चीज का प्रोग्राम के अंदर कुछ कुछ ना कुछ फिक्स्ड लॉजिक होता ही होता है तो यह तो हो गए हमारे एरिम ऑपरेटर्स इसके बाद बात
करते हैं अपने रिलेशनल ऑपरेटर्स की रिलेशनल ऑपरेटर्स भी मैथ में हमने कहीं कहीं देखे होंगे रिलेशनल ऑपरेटर में एक
आता है लेस देन एक होता है लेस देन इक्वल टू एक होता है ग्रेटर देन एक होता है ग्रेटर दन इक्वल टू तो ये तो चार मेन होते
हैं जब भी हमें इक्वल टू चेक करना होता है मैथ के अंदर तो हम लिखते हैं a क्या बी के इक्वल है लेकिन c+ प् के अंदर हम लिखते
हैं क्या a बी के इक्वल है हम डबल इक्वल टू यूज करते हैं क्योंकि जो सिंगल इक्वल टू होता है वो तो यहां पर असाइनमेंट के
लिए यूज होता है असाइनमेंट कहने का मतलब है जब हम वैल्यू असाइन कर रहे हैं जब राइट साइड की वैल्यू लेट्स सपोज आंसर 20 है तो
जब राइट साइड की वैल्यू लेफ्ट साइड में असाइन की जाती है भेजी जाती है तो उसे असाइनमेंट कहते हैं तो चेक करने के लिए हम
डबल इक्वल टू यूज करते हैं तो एक रिलेशनल ऑपरेटर डबल इक्वल टू होता है और एक होता है नॉट इक्वल टू नॉट इक्वल टू के लिए हम
लिखते हैं एक्सक्लेमेशन और इक्वल टू तो इसको हम पढ़ते कैसे हैं को हम पढ़ते हैं नॉट एक्सक्लेमेशन का मतलब है नॉट और आगे
इक्वल टू तो ये हमारे डिफरेंट रिलेशनल ऑपरेटर्स हैं जो एजिस्ट करते हैं रिलेशनल ऑपरेटर आंसर में या तो ट्रू रिटर्न करते
हैं या फिर फाल्स रिटर्न करते हैं यह फिक्स होता है जैसे फॉर एग्जांपल रिलेशनल ऑपरेटर्स को एक बार यूज करके देखते
हैं मुझे अगर प्रिंट करवाना है 3 < 5 तो इस स्टेटमेंट को जब हम लिखते हैं तो इसे हमें ऐसे पढ़ना है क्या 3 लेन 5 है
ओबवियसली 3 लेसन 5 है तो आंसर क्या होना चाहिए आंसर होना चाहिए ट्रू आंसर होना चाहिए ट्रू और ट्रू का मतलब क्या होता है
वन तो हमारे पास प्रिंट होकर आ जाएगा वन पर इसी का अगर हम ऑपोजिट लिख दें क्या 3 ग्रेटर दन 5 है तो ओबवियसली 3
ग्रेटर दन 5 नहीं है तो इसका आंसर फाल्स होना चाहिए और फाल्स कैसे प्रिंट होकर आएगा फाल्स प्रिंट होकर आएगा एज जीरो तो
इस बार हमारे पास वैल्यू प्रिंट होकर आएगी एज जी तो इस तरीके से हमारे लेस दन और ग्रेटर दन काम करते हैं जैसे लेस दन
ग्रेटर दन होता है वैसे ही लेस दन इक्वल टू ग्रेटर दन इक्वल टू हो सकता है जैसे अगर मैं एक स्टेटमेंट लिखूं क्या 3 लेसन
इक्वल टू 3 है लेस देन तो नहीं है पर इक्वल टू है तो आंसर तो ट्रू ही होना चाहिए इसे सेव कर लेते हैं रन करते हैं तो
लास्ट में हमारे पास प्रिंट होकर आएगी ट्रू वैल्यू जैसे लेस दन इक्वल टू होता है ऐसे ग्रेटर दन इक्वल टू होता है अब डबल
इक्वल टू देखते हैं अगर मैं सी आउट करूं 3 क्या थ्री के इक्वल है तो इस केस में ट्रू प्रिंट होकर आना चाहिए इन्हें कॉमेंट आउट
कर देते हैं क्या थ के इक्वल है यस ्र इज इक्वल टू 3 तो वन आएगा ट्रू आएगा पर क्या 3 फ के इक्वल है फाइव के इक्वल नहीं होता
उस केस में फाल्स आएगा क्या थ फ के नॉट इक्वल है क्या थ्री और फ अलग-अलग हैं यस थ्री और फाइव अलग-अलग
है तो इस केस में हमारे पास ट्रू आएगा कि 3 फ के इक्वल नहीं है ये सच बात है तो इस केस में ट्रू प्रिंट करो करवा दो लेकिन 3
3 के इक्वल नहीं है यह तो गलत बात है ये तो फाल्स बात है तो इसके लिए जीरो प्रिंट करवा दो तो उसके लिए हमारे पास प्रिंट
होकर आएगी वैल्यू जीरो के इक्वल तो इस तरीके से हमारे रिलेशनल ऑपरेटर्स काम करते हैं एंड दे आर प्रिटी स्ट्रेट फॉरवर्ड
बिल्कुल मैथस जैसा ही लॉजिक काम करता है तो कभी भी अगर हम वैल्यूज को कंपेयर करना चाहते हैं उनके बीच में रिलेशन देखना
चाहते हैं कौन सी वैल्यू छोटी है कौन सी वैल्यू बड़ी है तो हम इन ऑपरेटर्स को यूज करते हैं और ये ऑपरेटर्स हमेशा एक बुलियन
वैल्यू को रिटर्न करते हैं तो ये तो हो गए रिलेशनल ऑपरेटर्स इसके अलावा हमारे पास लॉजिकल ऑपरेटर्स भी होते हैं लॉजिकल
ऑपरेटर्स में हमारे पास तीन लॉजिकल ऑपरेटर होते हैं एक को हम कहते हैं लॉजिकल ऑर एक को हम कहते हैं लॉजिकल एंड एक को हम कहते
हैं लॉजिकल नॉट लॉजिकल र होता है यह दो सीधी लाइन इसको हम लॉजिकल और कहते हैं इसको टेक्निकल भाषा में हम पाइप ऑपरेटर
कहते हैं क्योंकि स्ट्रेट लाइन है तो वी कॉल इट अ पाइप तो डबल पाइप जब हम यूज करते हैं तो लॉजिकल और होता है डबल एम परसेंट
क्या बोला मैंने एंड को मैंने एंड को बोला एम परसेंट इसको एम परसेंट सिंबल बोलते हैं पर इसको एंड भी बोल सकते हैं तो डबल एंड
जब हम लिखते हैं तो उसको लॉजिकल एंड कहते हैं और जब हम एक सिंगल एक्सक्लेमेशन लिखते हैं इसको हम लॉजिकल नॉट कहते हैं अब
लॉजिकल नॉट सबसे आसान होता है इसको देख लेते हैं सबसे पहले लॉजिकल नॉट क्या कहता है लॉजिकल नॉट कहता है कि जो अगर आपका
आंसर ट्रू है तो मैं इसको फाल्स कर दूंगा और आपका आंसर फाल्स है तो मैं इसको ट्रू कर दूंगा जैसे फ फॉर एग्जांपल मुझे पता है
कि 3 ग्रेटर दन वन होता है मुझे पता है कि 3 ग्रेटर दन व होता है इसका आंसर ट्रू होना
चाहिए जो बहुत लॉजिकल भी है वन आ गया लेकिन जब मुझे इसका ऑपोजिट चाहिए कि नहीं मुझे तो अगर ये ट्रू होता है तो मुझे
फॉल्स चाहिए तो मैं आगे नॉट लगा देती हूं और नॉट के बाद आंसर जीरो में कन्वर्ट हो जाएगा या फिर मुझे पता है कि 3 लेसन व
नहीं होता इसका आंसर फाल्स होना चाहिए तो एक बार आंसर देखते हैं इसका आंसर फॉल्स आ गया लेकिन मुझे अगर फॉल्स की जगह ट्रू
चाहिए तो मैंने आगे नॉट लगा दिया और इस बार आंसर ट्रू के इक्वल आ गया तो इस तरीके से लॉजिकल नॉट जो भी आंसर होता है उसको
रिवर्स करके दे देता है उसका नॉट करके दे देता है ऐसे ही हमारे पास लॉजिकल ऑर होता है अब लॉजिकल ऑर एंड लॉजिकल एंड इनका जो
लॉजिक है वो हमें अगले चैप्टर के अंदर मतलब जब हम कंडीशनल स्टेटमेंट्स पढ़ेंगे कंडीशनल स्टेटमेंट्स पढ़ेंगे तब हमें
क्लियर होगा कि क्यों इनको हम यूज़ करते हैं अभी के लिए बहुत थोरेट्स लॉजिक आपको बता देती हूं लॉजिकल और को
क्यों यूज करते हैं बेसिकली लेट्स सपोज अगर हमें बहुत सारे हमारे पास स्टेटमेंट्स है एक मेरे पास ये स्टेटमेंट है एक
स्टेटमेंट कह रही है कि थ भाई ग्रेटर दन व होता है दूसरी स्टेटमेंट कह रही है 5 ग्रेटर दन 3 होता है यह दो स्टेटमेंट्स है
मेरे पास मैं चाहती हूं कि दोनों में से अगर कोई भी स्टेटमेंट सच हो जाए तो मेरे पास फाइनल आंसर ट्रू आ जाए ठीक है एक
फॉल्स हो गई पर दोनों में से कोई भी अगर ट्रू हो जाए तो फाइनल आंसर ट्रू होना चाहिए अगर इस तरीके की मेरे पास सिचुएशन
होती है तो उस केस में हम लॉजिकल और का यूज करते हैं तो मुझे पता है 3 ग्रेटर दन 1 होता है यह तो मुझे ट्रू दे देगा 5 ग्र
द 3 होता है यह भी मुझे ट्रू दे देगा तो तो फाइनल आंसर ट्रू आना चाहिए और अगर यहां पर 3 ग्र 5 भी होता लेट्स सपोज यहां 3 ग्र
दन 5 होता है तो मुझे पता है ये तो फाल्स होता है 3 ग्र 5 हो ही नहीं सकता पर फिर भी फाइनल आंसर क्या आएगा फिर भी फाइनल
आंसर मेरे पास ट्रू के इक्वल आएगा तो और तब यूज होता है जब हम कहना चाहते हैं जब मल्टीपल स्टेटमेंट्स हो और मल्ट में से
कोई भी एक अगर ट्रू हो तो फाइनल आंसर ट्रू हो जाए मतलब और को यूज करना है इसको देख भी लेते हैं कैसे यूज करते हैं जैसे मैंने
लिखा 3 लेव होता है तो यह ओबवियसली एक फाल्स स्टेटमेंट है एंड और और मैंने लिख दिया 3 ले 5 होता है एंड इन सब को एक बड़े
पेंसिस के अंदर लिख देते हैं तो 3 लेव यह फॉल्स है 3 ले 5 ये ट्रू है एक फॉल्स है एक ट्रू है तो मेरा फाइनल आंसर क्या आएगा
मेरा आंसर ट्रू के इक्वल आएगा और अगर दोनों ही फाल्स हो गए यहां पर भी लेस दन वन हो गया तब फाइनल आंसर जीरो आएगा फाल्स
आएगा लेकिन दोनों में से एक भी सच हो जाता है तो फाइनल आंसर ट्रू आएगा एंड इसका ऑपोजिट होता है लॉजिकल एंड कहता है कि
सारे के सारी स्टेटमेंट्स जब सच होंगी तभी फाइनल आंसर ट्रू होना चाहिए लेफ्ट भी सच हो राइट भी सच हो जैसे थ ग्रेटर दन व होगा
एंड 3 ग्रेटर दन टू होगा दोनों स्टेटमेंट सच है तो मेरे पास फाइनल आंसर एम पर एम परसेंट में ट्रू आना
चाहिए तो ट्रू आएगा लेकिन अगर एक को भी हमने झूठ कर दिया 3 ग्रेटर दन 4 लिख दिया तो उस केस में आंसर जीरो हो जाएगा उस केस
में आंसर फाल्स हो जाएगा तो इस तरीके से हमारे डिफरेंट डिफरेंट ऑपरेटर्स काम करते हैं इनफैक्ट और और एंड की मैं एक टेबल भी
बना सकती हूं जिसके अंदर पूरा का पूरा लॉजिक हमें और समझ में आएगा वैसे और एंड को डिटेल में हम नेक्स्ट चैप्टर के अंदर
बात करेंगे लेट्स सपोज और के अंदर अगर स्टेटमेंट वन अगर हमारा इनपुट वन ये अगर हमारा रिजल्ट है यह हमारा इनपुट वन है य
इनपुट टू है अगर स्टेटमेंट वन ट्रू है स्टेटमेंट टू भी ट्रू है तो भी रिजल्ट ट्रू आएगा अगर ये ट्रू है और ये फॉल्स है
तो भी रिजल्ट ट्रू आएगा अगर ये फॉल्स है ये ट्रू है तो भी रिजल्ट ट्रू आएगा जब दोनों फॉल्स होंगे तभी फाइनल आंसर मेरा
फॉल्स के इक्वल होगा और एंड का लॉजिक क्या कहता है एंड का लॉजिक कहता है कि अगर यह हमारा रिजल्ट हो गया दोनों ट्रू
हैं तब तो ट्रू आएगा लेकिन दोनों में से एक भी फॉल्स हो गया तो ये फॉल्स हो जाएगा दोनों में से एक भी फॉल्स हो गया तो ये
फॉल्स हो जाएगा दोनों के दोनों फॉल्स हैं तो भी ये फॉल्स रहेगा तो ट्रू सिर्फ तब आएगा जब दोनों के दोनों ट्रू हो तो ये
डिफरेंस होता है ऑर एंड एंड के अंदर अब ऑपरेटर्स में एरिथ मेे िक रिलेशनल लॉजिकल ऑपरेटर हमने पढ़ लिया इसके अलावा एक और
ऑपरेटर होता है जिसको हम कहते हैं बिट वाइज ऑपरेटर तो बिट वाइज ऑपरेटर्स को हम बाइनरी नंबर सिस्टम पढ़ने के बाद पढ़ रहे
होंगे तो इनको अभी के लिए टेंशन लेने की जरूरत नहीं है इनको बाद में डिटेल के अंदर हम कवर करेंगे दीज आर द मेजॉरिटी ऑपरेटर्स
जिनको हम पढ़ते हैं अब एक सवाल छोटा सा हम सॉल्व करने वाले हैं हमें कैलकुलेट करना है सम ऑफ टू नंबर्स सम ऑफ टू नंबर्स
कैलकुलेट करने का लॉजिक हमने ऑलरेडी फ्लो चार्ट सूडो कोड में देख रखा है बेसिकली पहला स्टेप क्या होगा कि हम इनपुट करेंगे
ए एंड बी अपने दो नंबर्स सेकंड स्टेप होगा कि हम सम कैलकुलेट करेंगे a + b थर्ड स्टेप होगा कि मुझे प्रिंट करवाना है अपने
सम को तो यह तीनों के तीनों यह हमारा सूडो कोड होता था बस इसको कोड के अंदर कन्वर्ट करना है कैसे कन्वर्ट करेंगे बहुत आसान
होने वाला है इनफैक्ट यहां पे आप लेक्चर को पॉज भी कर सकते हैं और खुद से इसका पूरा लॉजिक लिख सकते हैं सबसे पहले तो दो
वेरिएबल डिफाइन करेंगे a और b पहले a को इनपुट लेंगे एंटर
a तो सीन हो जाएगा a फिर इसी को कॉपी कर लेते हैं फिर हम b को एंटर करवाएंगे तो b एंटर
हो जाएगा फिर एक वेरिएबल ले लेते हैं सम = a + b कुछ नहीं किया बस सूडो कोड में जो लाइनें थी उनको एज इट इज बस c प् प् के
लॉजिक में कन्वर्ट करते जा रहे हैं पूरा लॉजिक बहुत आसान है बस जो सिंटेक्स है सिंटेक्स मतलब सिंटेक्स एक शब्द है जो आप
बार-बार सुनेंगे मुझसे सिंटेक्स का मतलब है रूल्स ऑफ प्रोग्रामिंग रूल्स ऑफ c+ प् बस उसको c+ प के रूल्स के अकॉर्डिंग हम
लिख रहे हैं एंड फाइनली सी आउट कर देंगे सम इज इक्वल टू सम एंड फाइनली एंड लाइन
लेट्स सेव इट लेट्स रन इट तो एंटर ए ए में मैंने एंटर किया थ्री एंटर b b में मैंने एंटर किया 10 तो सम = इ 13 हमारे पास 13
प्रिंट हो गया आकर तो हमने ये पूरा एक प्रोग्राम बना लिया है जो इनपुट भी लेता है जो कुछ ऑपरेशन भी परफॉर्म करता है सम
करने का और जो कुछ आउटपुट भी रिटर्न करता है तो ओवरऑल यही प्रोग्राम का स्ट्रक्चर होगा प्रॉब्लम जो है व चाहे कितनी मुश्किल
से मुश्किल हो जाए स्ट्रक्चर वही रहेगा कुछ इनपुट लेना है कुछ काम करना है और कुछ आउटपुट रिटर्न करना है यही चीज यही काम है
जिसको हम और नेक्स्ट लेवल एडवांस चीजों तक लेकर जाने वाले हैं अच्छा जाने से पहले एक और चीज ऑपरेटर्स के अंदर हम एक और स्पेशल
टाइप ऑफ ऑपरेटर होते हैं जिसको पढ़ते हैं वी कॉल इट यूरी ऑपरेटर यूनिरी ऑपरेटर्स क्या होते हैं यूरी ऑपरेटर से पहले बाइनरी
ऑपरेटर समझते हैं बाइनरी में बाय का मतलब होता है टू यूरी में य का मतलब होता है वन बाइनरी
ऑपरेटर वह ऑपरेटर्स होते हैं जो दो नंबर्स को यूज़ करके कोई ऑपरेशन परफॉर्म करते हैं जैसे अगर मुझे ऐड करना है तो ऐड करने के
लिए मुझे a और b दो नंबर्स चाहिए होंगे सिर्फ एक नंबर को तो ऐड कर नहीं सकते दो नंबर्स तो चाहिए होंगे तो प्लस क्या हो
गया प्लस बाइनरी ऑपरेटर हो गया मुझे माइनस करना है तो भी दो नंबर चाहिए मुझे मल्टीप्लाई करना है तो भी दो नंबर चाहिए
मुझे डिवाइड करना है तो भी दो नंबर चाहिए तो इन सारे ऑपरेटर्स को जो अभी तक हमने पढ़े इनको हम बाइनरी ऑपरेटर्स कहते हैं तो
अब यूनिरी ऑपरेटर्स क्या होते हैं यूनिरी ऑपरेटर्स को एक ही ऑपरेंट चाहिए होता है मतलब एक ही नंबर चाहिए होता है इन्
बेसिकली जब हम प्रोग्रामिंग कर रहे होंगे यानी जब हम लूप्स वाला कांसेप्ट पढ़ रहे होंगे लूप्स जब हम पढ़ेंगे नेक्स्ट चैप्टर
के अंदर तो जब हम लूप करते हैं तो एक ऑपरेशन है जो कोडिंग के अंदर हमें बार-बार करना पड़ता है और वह ऑपरेशन होता है a = a
+ 1 का या i = i + 1 का या समथिंग कोई वेरिएबल है इज इक्वल ट वेरिएबल + 1 का तो बार-बार बार-बार हमें वेरिएबल के अंदर + 1
करना होता है क्यों करना होता है क्योंकि कई सारे सवाल होते हैं जिनके अंदर कंडीशन वैसी होती है जैसे सूडो कोड वाले चैप्टर
में हमने सम ऑफ़ n नंबर्स निकालने का लॉजिक सीखा या प्राइम नंबर का सीखा तो वहां पे बार-बार हमें किसी वेरिएबल के
अंदर + व करना पड़ रहा था तो जब हमें इस तरीके की स्टेटमेंट लिखनी होती है बार-बार + व करना होता है तो हम उसको लिखने का एक
शॉर्टकट अपनाते हैं व्हिच वी कॉल a + प् मतलब प्लस प्लस को हम एक साथ लिख देते हैं तो a + प् का मतलब
होता है कि a के अंदर हम प्लव करने की कोशिश कर रहे हैं तो यह प्लस प्लस क्या होता है यह प्लस प्लस मेरा इंक्रीमेंट
यूनिरी ऑपरेटर होता है इस प्लस प्लस को पहले से ही पता है कि इसका सेकंड जो नंबर है वह वन ही होगा हमेशा तो यह जब हम a
प्लस प्लस लिखते हैं तो प्लस प्लस को सिर्फ एक ही नंबर चाहिए तो इसको हम यूनिरी ऑपरेटर कहते हैं अब प्लस प्लस जो है वह दो
तरीके से काम करता है दो तरीके से कैसे काम करता है एक तो हम लिख सकते हैं a + प् और एक हम लिख सकते हैं + + a दोनों का
मतलब थोड़ा सा अलग होता है a प्लस प्लस लिखने का मतलब है क्योंकि इसमें में प्लस प्लस बाद में आता है इसको लिखने का मतलब
है कि पहले हम काम करेंगे बाद में हम अपडेट करेंगे इसको मैं भी करके दिखाऊंगी लॉजिकली
कि और डिटेल में और क्योंकि इसमें प्लस प्लस पहले आता है तो इसका मतलब होता है कि पहले हम अपडेट करेंगे क्योंकि प्लस प्लस
प्लव अपडेट करता है और बाद में हम काम करेंगे जैसे फॉर एग्जांपल कोड के अंदर देखते हैं लेट्स सपोज मैंने एक वेरिएबल
बनाया a = 10 इसे क्लियर कर लेते हैं अब मैंने लिखा इंटी जर b = a + प अब ये जो स्टेटमेंट है इसमें दो चीजें हो रही हैं
सबसे पहला तो यहां पे काम हो रहा है काम क्या है काम है राइट साइड से b के अंदर कोई वैल्यू आ जाए ये मेरा काम है दूसरी
चीज जो स्टेटमेंट के अंदर हो रही है वो हो रहा है अपडेट होना अपडेट मतलब कि a के अंदर + व हो जाए a के अंदर अगर 10 वैल्यू
थी तो वो + व हो जाए तो प्लस प्लस अगर बाद में लगाए तो क्या होगा पहले काम होगा फिर अपडेट होगा पहले काम होगा मतलब पहले इस b
के अंदर वैल्यू जाएगी तो b के अंदर अगर वैल्यू पहले जाएगी तो जब हम सी आउट करवाएंगे b को तो b के अंदर a की पुरानी
वैल्यू जाएगी तो b के अंदर a की पुरानी वैल्यू मतलब 10 जाकर स्टोर होगी उसके बाद a अपडेट होगा एंड उसके बाद a अपडेट होके
10 से 11 बन जाएगा तो जब हम सी आउट करवाएंगे a की वैल्यू तो इस बार हमारे पास 11 प्रिंट हो कर आएगा तो b में 10 प्रिंट
होगा a में 11 प्रिंट होगा इसको इनफैक्ट हम प्रिंट करवा कर भी देख सकते हैं a इ इज इक्वल
टू एंड b इज इक्वल टू लेट्स सेव इट लेट्स रन तो b तो 10 है a 11 है तो आई होप इसके
पीछे का हमें लॉजिक समझ में आया होगा कि जब मैंने लिखा इंट b = a + + तो यहां पे दो काम हो रहे थे तो दो काम में से काम
सबसे पहले हुआ ये मेन वाला काम मेन वाला काम मतलब पहले b नाम का वेरिएबल क्रिएट होगा उसके अंदर a की पुरानी वैल्यू आएगी a
की पुरानी वैल्यू है 10 के इक्वल जैसे ये a है इसके अंदर पुरानी वैल्यू है 10 के इक्वल तो यही सेम वैल्यू यहां आएगी
फिर अपडेट होगा तो अपडेट होगा तो यहां 11 आ जाएगा तो फाइनली बी के अंदर 10 होगा a के अंदर 11 होगा यह तो हो गया a के बाद
प्लस प्लस लिखने का मतलब प्लस प्स ए यहां पर हम इसका उल्टा भी लिख सकते हैं कि हम लिख दें इंट b = प् प् a अब जब इंट b = प्
प् a हमने लिखा है तो उस केस में क्या होगा अगर मेरे पास इंटी जर a = 10 है यह मेरी मेमोरी है दिस इज a जिसके अंदर 10 है
मैंने लिखा इंटी जर b = + + a तो यहां पे काम क्या हो रहा है काम हो रहा है कि मैं b वेरिएबल क्रिएट करना चाह रही हूं अपडेट
किसको कर रहे हैं अपडेट a को करने की कोशिश कर रहे हैं तो प्लस प्लस ए कहता है पहले अपडेट करो a को अपडेट करना था तो
पहले a को अपडेट करके 11 करो उसके बाद काम करो काम क्या है b को क्रिएट करना तो b फिर मेमोरी में जाके क्रिएट होगा और अब b
के अंदर a की जब वैल्यू आएगी तो ब के अर अर 11 वैल्यू आएगी और इसे वेरीफाई भी कर सकते हैं जब मैंने प्लस प्लस ए लिखा तो
पहले अपडेट होगा उसके बाद काम होगा तो अपडेट होके पहले ए की वैल्यू 11 बनेगी फिर बी के अंदर
जो वैल्यू असाइन होगी वो भी 11 होगी तो इस बार जब प्रिंट करवाएंगे तो बी भी 11 है और ए भी 11 है तो यह प्लस प्स ए एंड ए प्स
प्लस के बीच का डिफरेंस होता है इनमें से जो a प् प् होता है इसे हम कहते हैं पोस्ट इंक्रीमेंट ऑपरेटर
और जो प्लस प्लस ए होता है इसको हम कहते हैं प्री इंक्रीमेंट ऑपरेटर पोस्ट का मतलब होता है बाद में क्योंकि प्लस प्लस बाद
में आ रहा है प्री का मतलब होता है पहले क्योंकि प्लस प्लस पहले आ रहा है तो यह हमारे पोस्ट एंड प्री इंक्रीमेंट ऑपरेटर
होते हैं अब जैसे इंक्रीमेंट ऑपरेटर्स होते हैं वैसे ही डिक्रिमेंट ऑपरेटर होते हैं इनका काम होता है -1 कर देना इनको भी
देख लेते हैं जैसे मेरे पास पोस्ट डिक्रिमेंट जो ऑपरेटर है व होगा a - माइनस a - माइनस का मतलब होता है a = a - 1 और
जैसे प्री डिक्रिमेंट ऑपरेटर है इसका मतलब है - - a - - a का भी मतलब a = a -1 होता है बस अगर यह किसी स्टेटमेंट में यूज़ हो
जाए तो यहां पे पहले काम होता है फिर अपडेट होता है यहां पे पहले अपडेट होता है फिर काम होता है यह डिफरेंस आ जाता है
इसको भी एक बार देख लेते हैं और इस बार प्रिंट करने से पहले ही हम अपने आउटपुट को प्रिडिक्ट करने की कोशिश
करेंगे लेट्स सपोज a10 है इन्हें हटा लेते हैं a अभी 10 है a को अगर मैंने किया - - a तो जब माइनस माइनस पहले आएगा पहले अपडेट
होगा तो a अपडेट होके क्या हो जाएगा a अपडेट होके नाइन हो जाएगा तो यहां आ जाएगा नाइन तो जब a की अपडेटेड वैल्यू b के अंदर
असाइन होगी तो b भी नाइन हो जाएगा तो यहां भी नाइन आ जाएगा जब प्रिंट करवाएंगे तो दोनों के अंदर हमारे पास नाइन आएगा ऐसे ही
इसका ऑपोजिट लें a प् a माइनस माइनस ले तो पहले काम होगा तो b के अंदर पहले a की वैल्यू जाएगी तो b के अंदर 10 जाएगा फिर
अपडेट होगा बाद में अपडेट होगा तो a अपडेट होके नाइ हो जाएगा सेव करेंगे रन करेंगे तो इस बार 10 एंड 9 प्रिंट होंगे a की
वैल्यू तो हमेशा सेम ही रहेगी अपडेट तो होना ही होना है चाहे अपडेट पहले हो चाहे अपडेट बाद में हो अपडेट तो हमेशा होगी
वैल्यू लेकिन पहले काम पहले होगा या बाद में होगा वो ज्यादा इंपॉर्टेंट होता है तो डिपेंडिंग अपॉन द सिचुएशन हम प्री
इंक्रीमेंट प्री डिक्रिमेंट को भी यूज कर सकते हैं या हम पोस्ट इंक्रीमेंट पोस्ट डिक्रिमेंट को भी यूज कर सकते हैं अभी के
लिए इसका आपको यूज समझ नहीं आ रहा होगा पर जैसे ही हम लूप्स को पढ़ लेंगे इसका यूज़ आपको परफेक्टली क्लियर हो जाएगा कि क्यों
हमने यह सारी पूरी जो कहानी है उसको इतना डिटेल में पढ़ा है यह टॉपिक जनरली काफी लोग स्किप कर देते हैं पर यह मैंने आपको
सेकंड लेक्चर के अंदर ही इसलिए बता दिया क्योंकि ये बहुत इंपॉर्टेंट टॉपिक है और ऐसे ही लॉजिक हैं जिनके ऊपर पूरी
प्रोग्रामिंग बेस्ड है इसको बाद में कराने का कोई पॉइंट नहीं है यह अभी से ही हमारा दिमाग उस डायरेक्शन में प्रोग्रामिंग की
डायरेक्शन में खुलना जो है वह शुरू होना चाहिए तो ये हमने अपने यूनिरी ऑपरेटर्स को कवर कर लिया है तो एक बार देख लेते हैं
हमने आज क्या-क्या पढ़ा हमने आज पढ़ा कि c+ प के अंदर इनपुट आउटपुट किस तरीके से लिया जा सकता है हमने
पढ़ा हमारे वेरिएबल कैसे काम करते हैं हमारे डेटा टाइप्स किस तरीके से काम करते हैं हमारे ऑपरेटर्स कैसे काम करते हैं जब
हम वेरिएबल डेटा टाइप्स को यूज करते हैं तो मेमोरी के अंदर किस तरीके से हमारा डाटा स्टोर हो रहा होता है जाके साथ के
साथ ऑपरेटर्स के बाद हमने यूनिरी ऑपरेटर्स को भी कवर किया है तो यह डिफरेंट डिफरेंट
चीजें हैं जो आज के सेक्शन में हमने पढ़ी हैं प्लस एक तरीके से हमने एक कंप्लीट प्रोग्राम बनाना सीखा है कि कैसे इनपुट
आउटपुट ऑपरेशंस का जो एक मिक्सचर है वह एक कंप्लीट प्रोग्राम हम लिख सकते हैं सी प्स प् के अंदर तो आई होप किय जो लॉजिक है यह
हमें समझ में आया होगा आज का जो लेक्चर है व हमें समझ में आया होगा एक दो चीजें हैं जो मैं आपको ए होमवर्क प्रॉब्लम दूंगी
सबसे पहला तो जैसे हमने सम ऑफ टू नंबर्स किया आपके लिए होमवर्क प्रॉब्लम है कि यू हैव टू बिल्ड अ कैलकुलेटर प्रोग्राम एक
तरीके से जो डिस भी कर पाए जो प्रोडक्ट भी निकाल पाए मल्टीप्लिकेशन भी कर पाए जो डिवीजन भी
कर पाए और जो मॉड्यूल भी कर पाए तो सम तो हमने कर ही लिया था आपको इन चारों के लिए ऑपरेटर्स हमें ऑलरेडी पता है एरिथ मेे िक
ऑपरेटर्स यूज़ करने हैं तो यू हैव टू राइट अ कैलकुलेटर लाइक प्रोग्राम जो हमारे लिए सारे के सारे ऑपरेशंस परफॉर्म कर पाए अब
यहां पर लेक्चर टू हमारा खत्म होता है तो इफ यू हैव सक्सेसफुली कंप्लीटेड लेक्चर टू तो आप जाकर लिख सकते हैं कमेंट्स के अंदर
अपनी आज की डेट और आपने लेक्चर टू को सक्सेसफुली कंप्लीट कर लिया है इसके साथ में यू कैन आल्सो लेट मी नो कि लेक्चर टू
के अंदर कौन-कौन सी चीजें हैं जो हमें अच्छी लगी कौन-कौन सी चीजें हैं जो हमें उतनी अच्छी नहीं लगी या व्हाट वाज आवर
बिगेस्ट लर्निंग फ्रॉम दिस लेक्चर कौन सी नई चीज है जो हमें सीखने को मिली साथ के साथ यू कैन आल्सो टैग मी ऑन
Heads up!
This summary and transcript were automatically generated using AI with the Free YouTube Transcript Summary Tool by LunaNotes.
Generate a summary for freeRelated Summaries
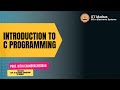
Understanding Data Representation in C Programming
Explore how data representation works in computers, focusing on integers and binary systems in C programming.
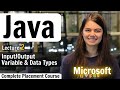
Java Basics: Outputs, Variables, and User Input Explained
Learn Java's fundamentals: how to give output, use variables, data types, and take user input effectively.
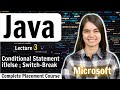
Mastering Java: Understanding Conditional Statements for Beginners
Dive into Java's conditional statements, including 'if', 'switch', and 'break', to enhance your programming skills.
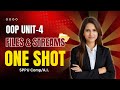
Understanding Unit 4: Files and Streams in C++
In this comprehensive video, we explore Unit 4 of the OP course, focusing on files and streams in C++. The session covers data organization, file handling, and the differences between text and binary files, along with practical coding examples.
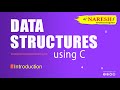
Understanding Data Structures Through C Language: A Comprehensive Guide
This video introduces the concept of data structures using the C programming language, explaining the importance of algorithms in structuring information. It covers various types of data structures, including linear and nonlinear types, and emphasizes the significance of arrays, stacks, queues, and linked lists in effective data storage and processing.
Most Viewed Summaries
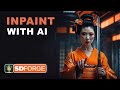
Mastering Inpainting with Stable Diffusion: Fix Mistakes and Enhance Your Images
Learn to fix mistakes and enhance images with Stable Diffusion's inpainting features effectively.
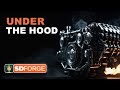
A Comprehensive Guide to Using Stable Diffusion Forge UI
Explore the Stable Diffusion Forge UI, customizable settings, models, and more to enhance your image generation experience.
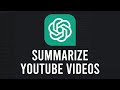
How to Use ChatGPT to Summarize YouTube Videos Efficiently
Learn how to summarize YouTube videos with ChatGPT in just a few simple steps.
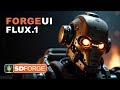
Ultimate Guide to Installing Forge UI and Flowing with Flux Models
Learn how to install Forge UI and explore various Flux models efficiently in this detailed guide.
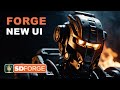
How to Install and Configure Forge: A New Stable Diffusion Web UI
Learn to install and configure the new Forge web UI for Stable Diffusion, with tips on models and settings.