Introduction
In the realm of computer programming, understanding how data is represented is fundamental. Particularly when working with a powerful language like C, it is crucial to grasp the concepts of data representation, which can greatly affect how we write and compile programs. In this article, we will dive deep into data representation, instruction encoding, and the implications of numbers on computer programming.
Data Representation in Computers
When we think about computers, we often picture them as devices that process text, numbers, and commands. However, at a fundamental level, computers consist of a Central Processing Unit (CPU), which includes an arithmetic and logic unit, as well as memory for storage. The way data is represented in these components can profoundly influence computational tasks.
The Importance of Data Representation
Data representation is essential because:
- Computers operate with binary systems: At the most basic level, computers use two digits, 0 and 1. This method of representation needs to be efficient and effective for various types of data, including integers, floating-point numbers, and more.
- Context for interpretation: Just as humans need context to understand language, computers require clear definitions of data types to interpret binary values correctly.
How Do We Represent Data?
When representing numbers in a computer, we often think in terms of various bases. The two most common representations are:
- Decimal (Base 10): Familiar to humans, consisting of digits from 0 to 9.
- Binary (Base 2): Used by computers, consisting solely of the digits 0 and 1.
This leads us to a key question: How do we convert human-familiar numbers into a format that a computer can understand?
A Closer Look at Numbers
To illustrate how computers manage numbers, let’s consider how we can store the values of a quadratic equation solver (for instance, coefficients A, B, and C).
-
Integers: To store integers like 5, 27, and 956, we must convert them into binary form:
- 5 in binary: 101 (base 2)
- 27 in binary: 11011 (base 2)
- 956 in binary: 1110111100 (base 2)
-
Binary Representation: In binary, each digit (or bit) has a specific weight, depending on its position:
- The rightmost bit represents 2^0 (units)
- The next bit to the left represents 2^1 (twos)
- Continuing this pattern gives context to each sequence of bits, allowing our computers to represent and manipulate numbers effectively.
Instruction Encoding and Machine Code
Besides data, computers must also understand instructions. Just like numbers, instructions are encoded in binary, meaning they also require representation in a format that can be executed by the CPU.
- Compile Process: When we write a program in C, it must be compiled into machine code—another binary format which the CPU can understand and execute.
- Role of the Operating System: Operating systems manage how programs are executed, ensuring that the right hardware resources are allocated appropriately based on the compiled code.
Trade-offs in Data Representation
When designing systems, programmers must consider trade-offs:
- Registers and Memory Size: The amount of memory available will influence how numbers are stored and manipulated. For instance, larger registers can represent larger values but may slow down computations.
- Standardization: Using a defined data type ensures portability across different systems. In C programming, integers may have different sizes (e.g., 16-bit, 32-bit), but a standard definition allows programs to run across various machines without modification.
Conclusion
Understanding data representation is crucial for programmers looking to expand their skills in C and computer architecture. By breaking down complex ideas into manageable concepts like binary numbers and instruction encoding, we can build a solid foundation. As we continue to work with languages like C, appreciating the significance of data representation will pave the way for more effective programming practices and a deeper understanding of how computers function.
With data being at the core of computational tasks, knowing how to represent, interpret, and manipulate data accurately not only enhances our programming skills but also prepares us for further exploration in computer science and related fields.
foreign [Music] hello everybody and welcome to unit 2 of
the course on introduction to programming in c what we are going to be looking at in this set of videos is the
problem of data representation right in particular when we work with a computer one of the things that we assume is that
there is the computers are processing data right processing meaning that maybe they are performing computations maybe
they are working with text you know whatever it is there is something that is required as a fundamental step over
there which is how do we get the data into the computer in the first place right
and we now have a rough idea of how a CPU works right there's an arithmetic and logic unit there are some registers
for temporary storage of information and there is memory which actually stores the data that you're working with
what we are going to be looking at over here is how does that data how should that data be represented in such a way
that a computer can make use of it so the outline of this ah set of lectures is roughly going to be
along the following lines right the first thing that we are going to look at is how do we represent data inside a
computer right we will then get into another section which is on instructions because it
turns out that instructions can also be encoded as some kind of numbers which means that we can use a very similar
kind of representation what we do for data in order to store instructions as well
that naturally takes us to the next stage which is how do we what is what does it mean to compile a program in
other words when we write a program in a high-level language like C right and C compared to SMD at least is
a high level language how do we then go about generating the machine code that actually needs to sit
in the memory of the computer and get executed and finally we will also look briefly at
the role of an operating system right now a word of caution over here many of
these topics are entire courses in themselves so for example if you get into the problem of instruction encoding
you could pretty much get into how instruction set architectures need to be designed and that would in turn lead
into an entire course on computer architecture right compilers once you start looking at how
does a compiler work How does it go about taking the input of a program and converting it into the machine code and
more importantly once you start looking at the kind of optimizations or improvements that it can make in the
process of generating that machine code that's another course in itself right and similarly of course operating
systems are at the very least one full course on them on their own right so naturally what that means is that the
level at which I'm going to be addressing them in these videos is going to be at a somewhat superficial level
it's enough to sort of give you a flavor for what lies underneath these topics right and hopefully will also you know
ignite some interest in terms of learning more about them unfortunately
learning more about them is pretty much beyond the scope of this course right so it's not really too much that we can do
over here what I am going to be sort of trying and doing as we go through even the data representation
is to focus on Parts where we basically say okay these are things that are essential for you to know as a
programmer working with a language like C and beyond that if you want to dig
deeper into the subject yes there are ways that you can go further and learn more about it but many of those topics
happen to be beyond the scope of what you need for an introductory course in C all right so let's start by trying to
understand what we mean by data and what exactly it is that we are trying to represent over here right there's an
interesting quotation from Leopold Chronicle right some of you may have heard of the chronicle Delta function
which is you know something that comes up in advanced math the quote essentially says God made the
integers all else is the work of man and partly the reason why that comes up is that integers or counting is something
that comes naturally right it is something very fundamental if at all you want to even keep track of
let's say you know how many pets you have or how many cattle you are have as a farmer you need to be able to count
but anything beyond that which starts getting into fractions real numbers more complicated representations complex
numbers of course are pretty much things that come out only when you start digging deeper and deeper into trying to
find out about mathematics now of course this statement is an exaggeration it's not that you know man just created all
of these things these are things which help us to understand nature now why is all of this relevant because
we use computers in order to enhance our understanding of nature right we want to be able to solve problems
and for that we need to have ways of representing all the different kinds of numbers and techniques and data in
general right that we have to deal with so the first question of course is why do we need to look into this problem of
data representation right and even by just looking at these slides one thing should pop up you know fairly
obviously which is humans use a variety of symbols right now I have written out these symbols in this case by hand right
but you could have typed them out right or you could have had the computer generate something in what looks like a
good handwriting right any different ways are possible of generating symbols the point is that humans are very good
at recognizing symbols which means that we can use a very large number of symbols in order to represent the
different kinds of values that we are interested in computers on the other hand must build
everything out of just two basic data points right 0 and 1 right so when people say that everything inside a
computer is just zeros and ones it's a bit of an exaggeration right that's not really a helpful or meaningful statement
but on the other hand at a very fundamental level it's also true because the only sort of states that can be
represented within a computer are zero and one what the computer does with those is
that by grouping them together appropriately and by interpreting them appropriately they are able to do a lot
more with those values so as an example let's look at this memory map that we had in the earlier
discussion on the quadratic equation solver where essentially what we said was we assumed that the three inputs ax
square plus BX plus C the A B and C are the inputs to our solver and their numbers right and I'm going to
store them in three memory locations that I have labeled 0 1 and 2. so far so good right the idea of the
memory location with a label or an address attached to it was I think well motivated and we kind of understand why
we need something like that right we need to be able to put something in a place and then get it back later when we
would like to use it now the question that was not answered at that point or rather was not even
raised at that point was what are these a b and c right we said numbers fine but I can't actually write a number into a
memory location I need to convert it into some form of zeros and ones that is the first step towards you know
understanding why we need why there is a problem with data representation okay there are a few questions we need to
answer before we get into you know even the different ways by which a b and c can be represented as numbers the first
thing is can a b and c be any arbitrary numbers right what do I mean by that well obviously
when I say A is a number you can imagine that you know one two three four yes certainly numbers okay
what about minus 1 right is a negative number allowed to be used for a in this context of course we would
naturally say yes right but at least for a person who's starting out from just counting it is no
longer it is not really obvious that negative numbers matter right in the case of a quadratic equation of course
that matters but not for a person who is just starting out Counting the next question comes what about 3.14
right in other words we know that we have decimal values right we understand what 3.14 means it's a decimal value it
is actually some kind of a fraction between three and four right but on the other hand is represented using the
decimal notation should I be able to have a equal to 3.14 right
what about 3.14 plus 2i right where I is basically the complex unit right which basically says 3.14
plus 2i would be a complex number which has a real part and an imaginary part should I be able to use arbitrary
complex values for a right the quadratic equation solver in general works for all of these
conditions right it doesn't really care what kind of number you use and that equation is a very general equation
but how we the kind of constraints that we put on a b and c will affect how we build a computer to solve the problem
the next question that we need to sort of address over here is how are these numbers a b and c stored in memory right
clearly I've been talking about zeros and ones we need to have some idea of how they will actually get stored
and another interesting question that comes out which is not very obvious until you start actually looking at the
zeros and ones inside the memory of a computer is how do I know when a number starts and when it stops
because after all inside the memory of a computer I don't have a space I don't have punctuation I don't have anything
to break the memory into different parts so how do I recognize that this corresponds to a number this corresponds
to a punctuation mark this corresponds to something else all of those are interesting questions that we do need to
address so let's look into the problem of numbers to start with okay as an example
I am going to continue with the quadratic equation solver of course I'm not actually going to go
and find the solutions because that would probably end up you know for most certainly it would
bring in negative numbers it might possibly also bring in you know complex numbers right
and possibly also decimal values right so right now I don't want to get into that
so I am just going to leave that out all I'm saying is okay if a is equal to 5 b is equal to 27 and C is equal to 956 how
do I even represent these values inside the memory of a computer so let's first look at the decimal
numbers right I mean 5 27 and 956 we understand them because we are used to the decimal system of representing
numbers and what that means is there are 10 digits 0 1 2 3 4 5 6 7 8 and 9. okay five is one of those digits in this case
I know when I say A is equal to 5 what I actually mean is that 5 is in the so called units place which effectively
means that it has a value of 5 into 10 to the power of 0 right 5 units five ones
27 on the other hand is more slightly more complicated number right it has two digits over there the first
or rather the right most digit corresponds to what we call the units place or the ones place and what we are
saying over here is that effectively this means that the units place 10 power 0
7 is multiplied by 10 power 0. and in the tens place I have two right so the tens place corresponds to
the factor 10 power 1. I multiply that by the digit that's over there which is 2 so I get 2 into 10 power 1. so 2 into
10 to the power of 1 is 20. plus 7 gives me 27. that is how I am interpreting these numbers of course by now we have
been using these numbers for so long that we no longer think explicitly about the place values right but it's good to
sort of recollect that in order to understand how we are going to do the same thing with computers
so taking the same thing one step forward I would say that 956 has six in the ones place so six units
five tens and nine hundreds okay so 9 into 10 power 2 900 plus 5 into 10 power 150 plus 6 into 10 power 0 6 956.
so this is the natural sort of place value system that we use in order to represent numbers that
they are all familiar with right from you know the start of school okay how is that relevant to what we would
like to do inside a computer so the assumptions that we implicitly make when we write down numbers like you
know 5 27 956 and so on let's just examine those because that will help us to for you know move forward from here
the first thing we assume is that we have a few symbols zero one two up to nine which we call the digits right and
we assume that these are available to us now how do I know when a number starts and when it stops the moment I start
seeing digits I sort of say Hey you know this is the start of a number but then I know that if there is some kind of a
space or some other kind of punctuation right maybe a comma or parenthesis or maybe
some other kind of symbol right which sort of separates two numbers I can sort of say that hey this looks like
these are two different numbers because you have to be a little bit careful because we do also tend to use
commas and decimal points and sometimes even underscores in the middle of numbers okay
now right now I'm going to ignore that I'm going to assume that we have some way to distinguish when one number stops
and another number starts and most of the time it is obvious to us from the context in which we are looking at those
numbers the point is all of these are human recognizable symbols they are not zero
or one which after all is the only thing that a computer can understand now if you are restricted to the symbol
0 and 1 right and let's say that you end up with some kind of a long string of zeros and ones like this and if I just
put this set of values in front of you and ask you hey what is this right
is this a number does it correspond to Punctuation is there you know some indication of a space or a break
somewhere in the middle of those numbers if it is a number how big is the number what is the value of the number right
all of those are questions that I can naturally ask if I am just presented with this value out here
right and in general there's no way to know whether you know do these four symbols correspond to a number do these
four correspond to punctuation does the entire thing correspond to a number does this part correspond to let's say an
encoding of some character does this correspond to a question mark we don't know
right and it's impossible to know in general and what happens is that the only real
solution for it is to use conventions okay what do we mean by conventions I
will be talking more about this as we go along but the basic idea is that at some point you should be able to look at a
set of values somewhere and say given the context right this is where I
see these values this is where they start this is where they stop this is how I should interpret them
okay because you know that context and you know that this is how you should interpret them in the context you are
able to make sense of those values right if on the other hand these numbers were just sort of stored you know they
were fossilized and seen by you know whatever humans have evolved into a million years from now a human looking
at or a creature looking at it at that point we'll just see a string of zeros and ones and does not have context
cannot make out whether this corresponded to a number a symbol or anything else
so that context right what is it that you are trying to represent is important and pretty much everything that
computers do and the how and how we make use of them relies on the fact that we can understand the context in which we
are looking at a number or a value and make sense of it in that way right so please keep this in mind there
are a number of places where I will say this is how an integer is represented or this is how a character is represented
and it's important to remember that those are conventions what that means in particular is it is
entirely possible that someone else could come up with a different set of conventions
right and effectively what would happen at that point is what you have written down will mean something different to
them and what they have tried to put down will mean something different to you or more likely will not mean
anything useful Okay the reason why conventions become important is so that we can communicate
from one person to another if two people decide that apple means different things right then it's impossible to have a
conversation about eating a kind of fruit right
but on the other hand as long as people have a certain image that comes into their mind when they
hear the word Apple of course in this case that could be confusing because you might end up thinking of a laptop or an
iPad or an iPhone hopefully you think of a fruit instead right as long as that happens
yes the conversation makes sense so if someone says I ate an apple yesterday you know what they are talking about
because the word apple has a certain visualization a certain meaning that comes into your mind when you hear it
and in the context saying I ate an apple you are reasonably clear that this must refer to the fruit
okay now in the same way what computers do is they rely on conventions I have a certain amount of
memory where I can store these symbols 0 and 1. okay and depending on where in the memory they are stored what is there
before them after them and so on I will interpret them appropriately yeah
so let's get into a little bit of detail on how numbers in particular could be represented using this binary system and
why am I saying binary because I have only two symbols out here 0 and 1 right
so anything which works with two symbols is can be referred to as a binary system by basically the prefix for the number
two right if you write three symbols it would be called ternary if it was four it would be quaternary decimal basically
refers to the fact that there are 10 symbols right for the digits at least we don't have a similar thing for the
alphabets and so on but we are talking about numbers out here so if I have only 0 and 1.
the question then becomes how can I for example go about representing a number like five now when I say five obviously
I mean the decimal number five right because in binary there are one there is only zero and one there is no 5
as such what does what can I say 5 is equal to I can sort of break it up and say that 5
is actually equal to 4 plus 1 right and the reason I've written it as 4 plus 0 plus 1 is that once again I'm
trying to translate this into a place value kind of a system right I basically say okay you know I have 2 to the power
of 2 to the power of 1 2 to the power of 0 right ideally I should be starting from
the right side so that I know exactly when to stop right I start 2 plus 0 2 power 1 2 power 2 2 power 3
point is to I don't need to go as far as 2 power 3 because that is eight which is already greater than this
number that I am trying to represent so I know that I can stop at 2 bar 2 and if I try breaking this up it basically
looks like 2 power 0 plus nothing out here in the sort of twos place right I don't want to
call it the tens place it's the two's place over here and this well we again can't call it the
hundreds place maybe we should just call it the two power two place okay so 2 power 0 and 2 power 2 have a
have to be added together how do I indicate that I put a 1 in that location because after all I have only those
these two symbols zero and one right if I put a 1 over there it means yes I need to add that 2 power 2 if I put a 0 it
means skip this 2 power 1 and if I put another one over here it means add the 2 power 0.
which finally gives us this value 1 0 1 as a binary notation for the decimal value 5.
okay this is some notation that we'll be seeing quite a lot of as we move forward
right which is usually that we say that we put the number 5 within parenthesis and just put this subscript out here
this value at the bottom right when I put the number 10 essentially what I mean is that this is the number 5
in decimal that is the base that I am using in order to do this
representation is 10 meaning that everything is in 10 power 0 10 power 1 10 power 2 right the units place the
tens place a hundreds place and so on similarly when I give the subscript 2 out here it means that the number is
binary right and when I say base 2 it basically means that this is now 2 power 0 this is
2 power 1 this is 2 bar 2 and so on right this notation is important because remember that 1 0 1 could also have been
a decimal number which has the value of 101 right so unless I explicitly put in something to
indicate what that number is it can be confusing now 5 on the other hand is very clear it cannot be a binary number
right but 1 0 1 could be a decimal number so you have to be a little careful and
make sure that you put the right notation to indicate what it is once again this is part of convention
right now 5 was easy right what about 27 right now once again I'm going to skip the
decimal representation part and straight away go for you know place values in the base 2 right and I'm going to say I'm
interested in these values 2 power 0 2 power 1 2 power 2 2 power 3 2 power 4. now you might ask the question why did I
stop there why did I stop at 2 power 4. right and the reason is I actually want to find which is the largest power of 2
that is less than this number that I am trying to represent right and it turns out that 2 power 4 is
equal to 16 Which is less than 27 but if I go to 2 power 5 I go to 32 which is greater than 27.
right just like when I know that let's say my number is 27 do I need to have anything in the
hundreds place no because I know that 100 is already greater than the number that I'm trying to represent
so in the same way 2 power 5 is greater than the number that I am trying to represent so I know that the coefficient
right that value that is going to be there multiplying the 2 power 5.
is essentially going to be 0 for sure okay
by the way as a word of notation these values 1 0 1 and so on are usually referred to as bits
right where bit is essentially a short form for binary digit okay so I'll be using the term bits
whenever we are talking about binary numbers right so please again keep that in mind when I say 1 0 1 only if I have
also confirmed that it is in binary then it makes sense to call these bits otherwise they are actually digits
so 27 I know is greater than 16 but less than 32 so I only need to go up to 2 power 4.
okay and in fact I can pretty much clearly see that this coefficient of 2 power 4
the bit value over here must probably be a one not probably must be a one right otherwise I'm not going to get something
adding up to the value that I want okay is it possible to have some kind of a
notation where you know even if I skip this is there another Way by which you know I could have
represented the same thing not in the normal binary notation you can actually prove that you know the representation
of any number in binary using place values is unique right but I'm going to skip over that
for now I'm not going to try and prove it out here the important point is as soon as we
know this value that you know the number lies between 16 and 32 I know that I must have a 1 over here
okay so let's put that in right and proceed what else do we need to do after that I
have already got a 16 and I need to add something else to get the remaining part of the number
right what's the remaining part of the number I subtract 16 from 27 and I get 11.
so in other words these four bits out here now need to represent the value 11. okay because this one has already taken
care of the first 16. okay so let's proceed 11 happens to be greater than 2 to the
power of 3 right and less than 2 to the power of 4 right it lies between those two so I know that
this value this bit over here must be 1. good let's do that right I subtract it out and I take the
remaining number which is 11 minus 8 is equal to 3. right now here's something a little bit interesting
what were we checking for when 11 was greater than or equal to 2 power 3 I put a 1 out here but now in this case I am
finding that 3 is less than 2 power 2. right because I mean that's the next location that I'm looking at right 2
power 2. so when I look at 3 and I look at 2 power 2 I find that it's less if I were to add 2 power 2 I would
overshoot I would have two bigger number for what I am trying to represent
okay so I need to put a 0 in here right but it also happens to be greater than 2
power 1. okay so that's good means that I need to put a 1 in the two power one place
and finally subtract that out 3 minus 2 power 1 is equal to 1 that is greater than or equal to in fact
it is equal to 2 power 0 so my zeros place or rather the 2 bar 0 place also has a 1 in it
okay so the way that I can represent 27 is all add up the corresponding values the
powers of 2 right only the ones that are needed in order to add up to 27. okay in fact what we can see is it
basically 16 plus 8 plus I skipped this one and two and one and when I add all of
these I get 27 as hoped for okay now one important observation at this
point is that what would have happened if I put another 0 out in front over here
right or another one and zero like keep adding zeros in front after 16 right so in other words apart from the zero if I
had 0 into 2 power 2 power 5 that is 32 plus 0 into 2 power 6 Plus 0 into 2 power 7 plus 0 into 2 power 8. it
doesn't change the value right leading zeros don't change the value of the number so this zero zero zero as many as
I want followed by one one zero one one is still the number 27.
okay this is a useful observation we'll be making use of it later when we talk
about negative numbers right it's obvious right I mean it's just like in decimal we say that 9 and 0 9 and 0 0
9 are all the same we almost never write it as 0 9 or 0 0 9 but we know that if you put a 0 in front of it it doesn't
change the value of the number that's exactly what we are saying here as well
so what that in turn means is if I ask you the question what is the minimum number of bits required to represent the
number 27. the answer is 5. because I know that 27 happens to be
greater than 2 power 4 but less than 2 power 5 which means that I need only at most these five bits
and I'm done I have a representation of 27. right
later on we'll see why this could potentially be a problem if I try representing it using just 5 bits but
the fact of the matter is I mean it's good enough I have an answer out here okay
what if I change the question and ask you what is the maximum number of bits required to represent 27
well the answer is there is no answer actually right because as you can see over here I can
keep adding zeros in front and make the number bigger
right but they are not changing the value of the number we are just changing how many bits are being used in order to
represent it what this means is I can represent 27 as a 5 bit number
I could represent it as an 8-bit number a 10 bit number a 20 bit number a 64-bit number right any of those would
ultimately I just apply the place value system find out what the value is if it's equal to
27 yes this number represents 27. using the binary number system right one more number right 956 now how
would I go about doing this I am not going to work through each and every step I'm sort of going to jump ahead and
say okay this is what the answer looks like I can straight away you know break this down and say that 956 is greater
than 2 power 9 which is 512 and less than 2 power 10 which is 1024
so 2 power 9 is required okay then what do I do I take 956 minus 2 power 9 9 56 minus 5 12. I get that
value I compare it with 2 power 8. it turns out that that result is greater than 2 power 8 so that bit also needs to
be set the remainder after that is greater than 2 power 7
with the remainder after that is not greater than 2 power 6 right and I would encourage you to work through this keep
doing the subtraction business which incidentally is exactly the procedure that is normally used when we
try to convert decimal numbers to minor right we sort of talk about it as a division but if you think about it it's
basically just subtracting out the powers of 2 that you want right the division would be sort of
approaching it from the opposite direction this is one way by which I can approach it from the most the you know
the larger side of things I basically look for do I need a coefficient of 512 to be one or zero in this case I can say
it has to be one I can also say that the coefficient of 1024 must be 0 because that would be greater than what I'm
trying to represent so when I go through this step and you know keep on taking subtracting out and
finding the remainder at this point I find that hey this you know the remainder that I've got is less than 64
which is 2.6 so skip that bit but then I find that 2 power 5 2 power 4
2 power 3 2 power 2 are all required once I hit this point I actually find that I'm done I already have a
representation for 956. okay so 2 power 1 and 2 power 0 are not required what does that mean
there will be zeros in the corresponding representation so let's write that out I basically say
that you know I have a one here one here one here a 0 here again 1 1 1 1 and
two zeros corresponding to this missing two power one and two power zero this in other words is the binary
representation of 956 right how many bits did I need 10. right and how do I get that 956 happens to be greater than
2 power 9 but less than 2 power 10. and any number in that range essentially requires 10 bits to be represented
now previously we saw that 27 required 5 bits and was one one zero one one five on the other hand was just 3 bits
one zero one okay so what should I do can I say that you
know 5 will be represented with three bits 27 will be represented with 5 bits 956 with 10 bits and how am I going to
recognize them because they all look the same they look like zeros and ones right if I just put them down next to each
other I wouldn't know where one number was starting and the other number was ending
right so the question then becomes you know the sort of natural and obvious solution
to this is hey use the same number of bits for all of them in that way at least when I look at the
thing I can say that you know if I know that a number starts here I know where it ends because I've already been told
how many bits are required to store one number and I can assume that maybe the next
number starts immediately after this one which means that I can now start interpreting the bits that I see in
front of me okay and the problem is if we ask that
question how many bits do we need right there's no unique answer to that and the reason for that is obvious right
like I said I can add zeros in front over here or here or for that matter even here without changing any of these
values okay so we make our choice based on other
deciding factors so what are these other deciding factors we'll have to sort of you know uh
look at you know what are the other possibilities that can come in over there right so the other deciding
factors are going to be things that actually are related to our physical constraints we have a computer that we
are designing it has a CPU an ALU which can perform certain kinds of additions and so on
it has memory registers right that can store values and memory that can actually store the values from which I
need to fetch data okay all of those are going to be parts of my decision making process
now one more word about binary numbers right if we just look at what we can do with for example 8-bit values
right the numbers would start like this right it would be zero zero zero all zeros
essentially right now if I talked about decimal I would not normally write number if I asked you how many three
digit numbers exist you would not normally start by writing zero zero zero okay you don't consider zero zero zero
to be a three digit number you just say that it's zero right and you would say that the first three
digit number is actually 100 right where there is something other than 0 in the hundreds place in binary we don't
exactly work that way we just say if it's an 8-bit number you know even if there were leading zeros I still
consider it an 8-bit number and I have to write all the eight bits okay so an 8-bit number of zero the
representation for 0 as an 8-bit number is all the bits are zero
if the first seven bits starting from the left are 0 and the bottom bit is a one then I call that a I
say that that's one if I have zero zero zero zero zero zero one zero then this corresponds to the two power one place
right and that's basically a the total value decimal value is 2. I go all the way down this would be equal to
255. right you can work that out you can basically see it is 128 plus 64 plus Etc
finally I'll get 255. okay the interesting thing is 2 to the power
of 8 is 256. so it turns out that you know when you have added all of these things together
what you get is 255 which is 2 to the power of 8 minus 1. right and what that means is when I get
all of these ones out here and I add one to it I'll basically get the zeros all over
here and a 1 in the most significant place right another way of looking at this in other
words would be that if I was to add 1 to this I would end up getting 0
right how we do addition is exactly the same way that we would do it in decimal numbers right except that we
carry whenever you have one plus one okay I'm not going to go into the details of
how we do arithmetic because we assume that the computer is already doing it for us
right in a course on digital systems you will actually sort of look into that in a lot more detail so I'm going to skip
over that over here what does end up happening is that I'll get a number that looks like this
which is basically 256 decimal right
so this is just an observation one other piece of notation which I had not mentioned so far is that this bit is
usually called the most significant
bit or the m s b and the one out here is usually called the least
significant bit or the LSB okay so that's just notation which or
rather terminology that we tend to use when we are talking about such values okay
in general what we have over here of course is that an N bit value can take
decimal values all the way from 0 up to in this case 2 to the power of n minus 1
to power n being 2 power 8 or 256 minus 1 which is 255. that's always true right if I had something else which was
let's say a 16 bit number I would go from 0 up to 2 power 16 minus 1. so now let's look more closely at what
are these other factors that we talked about right the fact that I have a CPU the CPU has registers inside it and that
those registers are in turn going to be getting data from some external memory right
all of these things are going to build up out of physical circuits which means that they occupy space they
have a cost associated with them they consume power ultimately what it means is that I there
are reasons why I might need to restrict the size of each of these memory registers and the CPU itself right I
can't use an infinite number of bits over there I need to make a call at some point
the second thing as we saw was a uniform size for all numbers is preferable right if I say that all numbers are going to
be 8 Bits yeah I can only represent numbers from 0 up to 255 but at least I can look at an 8-bit value and straight
away say hey this could be this you know start and end of a number and the other thing like I said is you
know you have these registers inside the CPU those are in turn feeding into the arithmetic and logic unit
right and the larger those registers the slower the circuitry is going to become right the reason for that is not
entirely obvious intuitively you can sort of understand right larger circuits are probably going to be slower they
sort of more work needs to be done the exact details of why they are slower you probably need to go into a course on
digital systems in fact a slightly more advanced course on how they are implemented in order to understand
exactly what the implications are but at an intuitive level it is obvious that larger is likely to mean slower
okay and that is in fact what happens and once we take all of these things into account the fact that we have
physical circuits we would like to have uniform sizes for the numbers and that there are all these registers that need
to be implemented and in turn are feeding into the ALU they come across this
what is called a trade-off right and in engineering a trade-off basically happens when you have to make
a choice there are two possible solutions or two or more possible solutions
Each of which has its own merits and demerits right so having just eight bits probably means that your CPU is going to
be small and fast but it also means you have only a few numbers that you can represent having 32 bits means that your
CPU can represent a lot more numbers up to at least you know 10 power 9 or so but is going to be slower than an 8-bit
CPU what about having 1024 bits right now that
will probably be very good from the point of view of the range of values of numbers that you can represent but is
going to occupy an awful lot of space for normal numbers which are probably in the smaller range and is going to be
slow so each of these has pros and cons and Engineering is all
about making the right kind of trade-offs at some level you have to make a choice and say this is why I made
the choice there are these benefits there are these drawbacks overall I think this
is a good reason to make a choice in a certain way okay now
you might be wondering at this point why is all of this relevant to you because you are only interested in learning
about C programming right but the way that I'm looking at this is you are interested in this course
because you're just you are not just interested in C programming by itself you are presumably interested in
understanding C because it helps you to understand a little bit more about how a computer works
and possibly because you might also want to implement custom embedded type of systems right where C gives you the most
control over what actually happens in the hardware right and once you take that into
account the all these discussions that we had how do
we represent numbers how do we decide the number of bits and so on they have a direct impact on the programming
language right in particular
we need some kind of a primitive data type some kind of a way of representing numbers
right that is going to be portable meaning that once I represent it in a particular system
right if I if somebody goes and builds another new processor or a new computer I don't want to have to change all my
definitions everything from scratch I should be able to still say hey this is what I mean by a number I don't care
whether you use processor from company a or from Company B they should both be able to interpret a
number the same way right and that in fact is one of the key things right that the reasons for going
towards a higher level language like C right and when I say higher level language obviously I mean compared to
Assembly Language and machine code because arguably C is not a very high level
language it's not one of those things where you can express great abstractions but when you look at it from the point
of view of the hardware it's a tremendous advancement right you are no longer tied to the individual sizes and
numbers of each register you can just assume that there is a primitive data type
right which in the case of C is usually defined as the integer right and modern variance of C basically
assume that an integer is 32 bits wide why do I say modern variance because it was not always the case right there were
scenarios where you had 16 bit integers right in fact the C language references sort
of vague on this point you could potentially even have a larger integer if you go towards processors with bigger
registers right so
like I said this is part of convention the place where it is a big win is that it says that as far as the C language is
concerned there is some kind of standardization which tells us that you know this is what a number is going to
be like unfortunately that's also not a very solid standardization right it is
slightly vague and that's partly to do with how the C reference itself has been written there are other languages that
are a lot more strict in this regard and within C itself there are other kinds of data types which we will see later where
you can precisely specify how many bits you are interested in okay the important point is the language
allows you to do something like that and why that is crucial is once you are able to do something of that sort you
can then write a program get it working on one particular CPU one particular machine
and there is a very good chance that if somebody creates another new machine somewhere else whatever program you
wrote in C will most likely compile and run correctly on that as well okay so that is sort of the important
point the reason why we go for a language like C the impact of all this discussion that we had in terms of
number representations is that it affects how the language itself is designed
Heads up!
This summary and transcript were automatically generated using AI with the Free YouTube Transcript Summary Tool by LunaNotes.
Generate a summary for freeRelated Summaries
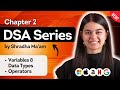
Understanding Variable Data Types and Operators in C++
Learn about variable data types and operators in C++. Discover syntax, examples, and functions for programming in C++.
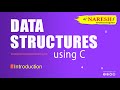
Understanding Data Structures Through C Language: A Comprehensive Guide
This video introduces the concept of data structures using the C programming language, explaining the importance of algorithms in structuring information. It covers various types of data structures, including linear and nonlinear types, and emphasizes the significance of arrays, stacks, queues, and linked lists in effective data storage and processing.
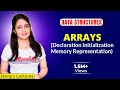
Understanding Arrays in Programming: Declaration, Initialization, and Memory Representation
This video provides a comprehensive overview of arrays in programming, covering their declaration, initialization, and how they are represented in memory. It explains the need for arrays, the types of arrays, and the importance of data types in array declarations.
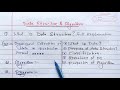
Introduction to Data Structures and Algorithms
This video provides a comprehensive introduction to data structures and algorithms, explaining key concepts such as data, data structures, their purpose, classifications, and operations. It also covers algorithms, their properties, and practical implementation examples.
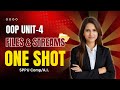
Understanding Unit 4: Files and Streams in C++
In this comprehensive video, we explore Unit 4 of the OP course, focusing on files and streams in C++. The session covers data organization, file handling, and the differences between text and binary files, along with practical coding examples.
Most Viewed Summaries
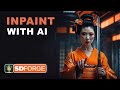
Mastering Inpainting with Stable Diffusion: Fix Mistakes and Enhance Your Images
Learn to fix mistakes and enhance images with Stable Diffusion's inpainting features effectively.
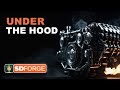
A Comprehensive Guide to Using Stable Diffusion Forge UI
Explore the Stable Diffusion Forge UI, customizable settings, models, and more to enhance your image generation experience.
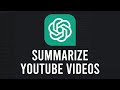
How to Use ChatGPT to Summarize YouTube Videos Efficiently
Learn how to summarize YouTube videos with ChatGPT in just a few simple steps.
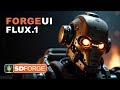
Ultimate Guide to Installing Forge UI and Flowing with Flux Models
Learn how to install Forge UI and explore various Flux models efficiently in this detailed guide.
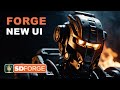
How to Install and Configure Forge: A New Stable Diffusion Web UI
Learn to install and configure the new Forge web UI for Stable Diffusion, with tips on models and settings.