Understanding Data Structures Through C Language
Introduction
- Welcome to Narish Technologies, presented by Shas.
- The focus is on data structures using the C programming language.
What are Data Structures?
- Data structures are not programming languages but concepts involving algorithms to structure information. For a deeper understanding of the algorithms involved, check out our Comprehensive Overview of Algorithms and Data Structures Course.
- They are implemented using programming languages like C, C++, Java, etc.
- The importance of data storage and processing is highlighted, especially in the context of data science.
Types of Data Structures
- Linear Data Structures: Elements are arranged sequentially.
- Examples: Arrays, Stacks, Queues, Linked Lists.
- Nonlinear Data Structures: Elements are connected in a hierarchical manner.
- Examples: Trees, Graphs.
Arrays
- Arrays hold multiple elements of the same type and are index-based, allowing for faster access. To learn more about variable data types and how they work in C++, see our summary on Understanding Variable Data Types and Operators in C++.
- They are considered derived data types and do not follow any specific algorithm.
- Accessing elements in an array involves pointer arithmetic, enhancing efficiency.
Advantages of Arrays
- Arrays allow for the storage of multiple elements in a single variable, simplifying data management compared to using multiple primitive variables.
- They facilitate faster access and processing of data.
Key Concepts for Implementing Data Structures in C
- To effectively implement data structures in C, one must understand:
- Functions
- Recursive Functions
- Arrays
- Structures
- Pointers
- Dynamic Memory Allocation
- For a more comprehensive understanding of how to handle files and streams in C++, refer to our summary on Understanding Unit 4: Files and Streams in C++.
Conclusion
- The next session will cover the implementation of stack algorithms using both static and dynamic memory allocation. For those interested in Java, our guide on Java Programming: A Comprehensive Guide to Understanding Java and Its Concepts may also be beneficial.
- Viewers are encouraged to subscribe for more content.
[Music] hi everyone welcome to narish Technologies this is
Shas today we are going to start data structures using C language or data structures through C
language so first of all what is this data structures through C language means what so remember data structures is not
a language simply a data structures is the concept
of set of algorithms set of algorithms used to structure the
information it is not a programming language like C C++ Java or net so these are just a set of algorithms we are
implementing these algorithms using any programming language for example in C or C++
languages we have to write the code or we have to write the logic data structure algorithms so but in Java
language right or in a net language so they have given a predefined implementations to all these algorithms
called collections framework but now here so we are developing the logic how to implement an
algorithm right so what is a data structure means to structure the information while
storing we know the importance of a data storage for every application and nowadays so why Hardo data science and
all ruling the market so what is the reason behind that means all these are data processing Tools in early days
right in 1960s only right some of the scientists ident ify whatever what is the importance of a data right in a
future so by that time so they were introduced one very good language that is a Python language that is a Python
language to process the information much faster but by that time the people so doesn't know so what is the importance
of right data analysis and all nowadays right so whenever they understood the importance of data storing and data
processing again python moving to the Limelight so that was right so very important programming language so this
is all about just analysis of data so when you will analyze the data means after you stor is now here our
discussion is a data structures data structures means while storing the information so what are the AL
algorithms we should follow if you follow those algorithms what will happen how effectively we are storing the
information all these are comes under data structures algorithm right so here data
structures data structures through C language data structures through C language so we are implementing
algorithms using the syntax of a c language the syntax so to structure the data n number of algorithms were
proposed n number of algorithms all these algorithms are called abstract data
types abstract data types sir what is abstract data type very simple a set of rules
algorithm is nothing but set of rules right so tell me some of the examples of data structureal
algorithms right so generally in every programming language to structure the information we use one data structure
concept and of course that doesn't follow any algorithm but that is also one way of effect of storing the
information effectively so what is that example Le array what is that example
array using arrays we can store the information effectively how so that we'll
see and some more examples for example stack qes qes linked
lists linked list trees trees Gra graphs
graphs so all these are so comes under algorithms and so many are there sorting techniques searching techniques all
these are comes under algorithms right so now we'll see so one by one and here algorithms divided into
two types nothing but data structures divided into two types so what are the types see data structure algorithm
Ms algorithms divided into two types so what are the two types means first one is a linear data structures second one
is a nonlinear data structure so what are linear data structures and what are nonlinear data
structures linear data structures means the arrangement of elements are in a sequential format so one is connected to
only one element right so that is such type of elements algorithms are called arrays Stacks cues linked
lists all these are comes under linear data structures because in these data
structures one element is connected to another element in a linear form nonlinear form means one element is
connected to n number of elements the best example trees and [Music]
graphs trees and graphs these are nonlinear data structures and these are linear data
structures okay so first of all we'll see a simple data structure arrays right array so we know that what
is the array and why they introduce the concept of array why right simple
thing simple thing it holds more than 1 element it holds more than one element next
one it holds only homogenous elements nothing but same type of elements and next one this is index
based so accessing become faster processing the elements much faster in arrays right and it is also
called derived data type derived data type and next one it doesn't follow any
algorithm it doesn't follow any algorithm so this is a simple data structure they introduced in every
programming language algorithm okay how to access the elements effectively from an
array for example if you declare an array int a RR int AR RoR either you declare like
this error of five some of the values some of the values we are storing
right all these elements get memory allocation all these elements and we are accessing using index number 10 20 30 40
50 The Base address just consider 2046 2048 2050 2052
2054 just consider iner occupies a 2 bytes memory we know that array is an internal pointer variable it holds holds
the Base address of the memory block the Base address a RR is holding the Base address of the block is a
2046 it holds the Base address of the block it holds next one how to access the
elements simply if you access a RR of three a RR of three then how it will execute so directly it will give the
value a r of three value is a 40 simply it is giving but internally what is happening means internally it
uses the concept of concept of pointers a r r + 3 sir pointer
arithmatic how it will increase very simple it will be increased by the size of integer every
time the size of integer of course exactly not we are writing like this because so whenever we are increasing
the ordinary variable that will be increased by one integer variable but whenever we increase a pointer variable
using modify operator that will be pointing to the next memory location in the array that will be pointing to next
memory location in the array right see so now pointer a RR value is 2046 plus 3 into size of integer is a 2 bytes
now again pointer 2046 + 6 is a pointer to 2052 what is pointer to 2052 the value
which is inside the location so what is that value 40 40 if you are new to the programming
we are accessing the array elements with the help of index number but one once you are perfect in a Pointer's concept
right you should understand what is the internal concept right how it is providing the information effectively so
with the help of some of the formulas we can access the information of every array much faster of course using index
number but in the background of every index number so there is a concept of pointers and pointer arthamatic concept
so many Concepts we will use okay so this is right what is the advantage of array when compar with the
ordinary primitive data if it is a primitive variable if you declare two variables if you declare two variables
in AAL to 10 and in Bal to 20 consider a gets memory allocation at some location and B gets memory allocation at
another location at another location so so we cannot access the information
effectively using programs so whenever we are declaring two variables all these variables get memory allocation in two
different locations so in such kind of situation right if you want to access
the information effectively that is not possible because these are random memory locations we can't use any equations or
any Expressions to access the information so faster so this is the advantage of arrays instead of using
right variables and one more important thing using arrays right we can store n number of elements with the help of a
single variable whereas if you go for ordinary variables for example if you want to store right some mobile numbers
100 mobile numbers 100 variables you have to declare right for example 100 students maths marks again 100 variables
you have to declare so working with 100 variables either declaring right or assigning processing anything is much
complex in a programming so that's why it is better to use arrays instead of using primitive data types this is one
type of a data structure of course it doesn't follow any algorithm but using array we can store the information
effectively and we can process the information effectively so right so now we are using right some of the algorithm
based data structures right to store the information nothing but to structure the data right see so one important thing
you should understand that right if you want to implement all the algorithms stack algorithm Q algorithm linked list
trees and graphs right using any programming language for example here we are using C language you should be
perfect in some of the areas of a c language so what are the areas so first one first one functions we should
understand functions and one more thing recursive function
also second one arrays arrays third one structures structures fourth one
pointers and the last one is a dynamic memory allocation these five concepts are very
very important right that you should know to learn a data structure algorithms using a c
language because if you want to implement any algorithm into code to store the information in a structural
format We are following all these Concepts logic right functions arrays structures
pointers dynamic memory allocation right hope all of you people good at all these concepts of a c
language right if you are not perfect just go through right all the previous videos in a c language so there already
we discussed about all these Concepts perfectly okay right so in the next session we'll start right how to
implement a stack algorithm right using static memory allocation and using dynamic memory
allocation right so hope you will enjoy this video for more videos please subscribe to Nar Channel thank you
oh [Music]
Heads up!
This summary and transcript were automatically generated using AI with the Free YouTube Transcript Summary Tool by LunaNotes.
Generate a summary for freeRelated Summaries
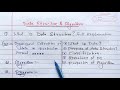
Introduction to Data Structures and Algorithms
This video provides a comprehensive introduction to data structures and algorithms, explaining key concepts such as data, data structures, their purpose, classifications, and operations. It also covers algorithms, their properties, and practical implementation examples.
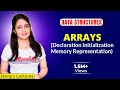
Understanding Arrays in Programming: Declaration, Initialization, and Memory Representation
This video provides a comprehensive overview of arrays in programming, covering their declaration, initialization, and how they are represented in memory. It explains the need for arrays, the types of arrays, and the importance of data types in array declarations.
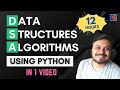
Comprehensive Overview of Data Structures and Algorithms Using Python
This video provides an in-depth exploration of data structures and algorithms using Python, covering essential topics such as linked lists, stacks, queues, and sorting algorithms. The session includes practical coding examples, theoretical explanations, and insights into the efficiency of various algorithms.
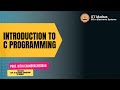
Understanding Data Representation in C Programming
Explore how data representation works in computers, focusing on integers and binary systems in C programming.
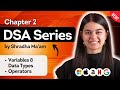
Understanding Variable Data Types and Operators in C++
Learn about variable data types and operators in C++. Discover syntax, examples, and functions for programming in C++.
Most Viewed Summaries
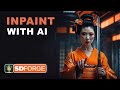
Mastering Inpainting with Stable Diffusion: Fix Mistakes and Enhance Your Images
Learn to fix mistakes and enhance images with Stable Diffusion's inpainting features effectively.
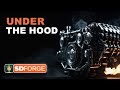
A Comprehensive Guide to Using Stable Diffusion Forge UI
Explore the Stable Diffusion Forge UI, customizable settings, models, and more to enhance your image generation experience.
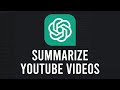
How to Use ChatGPT to Summarize YouTube Videos Efficiently
Learn how to summarize YouTube videos with ChatGPT in just a few simple steps.
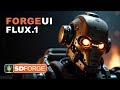
Ultimate Guide to Installing Forge UI and Flowing with Flux Models
Learn how to install Forge UI and explore various Flux models efficiently in this detailed guide.
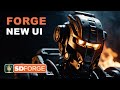
How to Install and Configure Forge: A New Stable Diffusion Web UI
Learn to install and configure the new Forge web UI for Stable Diffusion, with tips on models and settings.