Introduction to Java Programming
Java is a widely-used programming language known for its simplicity and versatility. It was introduced by Sun Microsystems in 1995 and has become a standard in the IT industry. The following sections will cover various fundamental concepts in Java programming, including its features, objects, exception handling, and collections framework.
Features of Java
Java possesses several key features that make it a preferred programming language:
- Platform Independent: Java programs can run on any machine with a Java Virtual Machine (JVM).
- Simple and Secure: Java syntax is easy to understand, and it offers built-in security features.
- Object-Oriented: Java uses an object-oriented programming model where everything is treated as an object.
- Robust and Maintainable: Java supports strong memory management with garbage collection to reduce memory leaks.
Basic Concepts of Java
Variables and Data Types
In Java, variables are used to store data, and they come in different data types such as int, float, boolean, char, etc. Each data type consumes a specific amount of memory:
int
: 4 bytesfloat
: 4 bytesdouble
: 8 byteschar
: 2 bytes
Operators in Java
Operators are special symbols that perform specific operations on variables and data types. Common types include:
- Arithmetic Operators:
+
,-
,*
,/
- Relational Operators:
==
,!=
,>
,<
- Logical Operators:
&&
,||
,!
Control Statements
Control statements control the flow of the program. They can be categorized as:
- Conditional Statements:
if
,else
,switch
- Looping Statements:
for
,while
,do-while
Object-Oriented Programming (OOP) Concepts
Java is built around the four main OOP concepts:
1. Abstraction
Abstraction involves hiding complex realities while exposing only the necessary parts. This can be achieved via abstract classes and interfaces.
2. Encapsulation
Encapsulation is the bundling of data with the methods that operate on that data. It restricts direct access to some components and methods.
3. Inheritance
Inheritance allows a new class to inherit properties and behaviors from an existing class. For example:
- Superclass: Vehicle
- Subclass: Car, Bike
4. Polymorphism
Polymorphism allows methods to do different things based on the object it is acting upon. It can be seen in:
- Method Overloading: Same method name with different parameters.
- Method Overriding: A subclass redefines a method from its superclass.
Exception Handling in Java
Exceptions are unexpected events that occur during program execution. Exception handling is a technique used to manage these events to ensure the normal flow of the program is maintained. Common types of exceptions include:
- Checked Exceptions: Must be either handled with a try-catch block or declared with a throws keyword.
- Unchecked Exceptions: Runtime exceptions that do not need to be explicitly handled.
- Errors: Serious problems that cannot be handled by the program.
Examples of Exception Handling
try {
int result = 10 / 0;
} catch (ArithmeticException e) {
System.out.println("Cannot divide by zero!");
} finally {
System.out.println("This will always execute.");
}
File I/O in Java
Java provides classes in the java.io
package for performing input and output operations on files. Common classes include:
- File: Represents a file or directory path.
- FileInputStream: Used to read bytes from a file.
- FileOutputStream: Used to write bytes to a file.
- BufferedReader: Efficiently reads characters, arrays, and lines from a file.
Example of File Writing
File file = new File("example.txt");
try (FileWriter fw = new FileWriter(file)) {
fw.write("Hello, World!");
} catch (IOException e) {
e.printStackTrace();
}
Java Collections Framework
The Java Collections Framework provides a set of classes and interfaces for storing and manipulating groups of data. Key interfaces include:
- List: An ordered collection (elements can be duplicated).
- Set: A collection that does not allow duplicate elements.
- Map: A collection of key-value pairs where keys must be unique.
Using ArrayList
List<String> names = new ArrayList<>();
names.add("John");
names.add("Jane");
Using HashMap
Map<Integer, String> map = new HashMap<>();
map.put(1, "One");
map.put(2, "Two");
Conclusion
Java is a powerful and versatile programming language that enables developers to build robust applications. Mastering its core concepts such as OOP, exception handling, file I/O, and the collections framework is essential for any Java developer. This article serves as a guide through these basic yet important concepts. With continued practice, you can advance your Java skills and develop more complex applications in no time!
Java is one of the most fundamental programming languages
that anybody can learn despite being so simple. It is a Marvel what can be achieved with the use of java?
Hi everyone. I welcome you all to this Java full court session by Edureka,
but before we begin, let's look at our agenda for today. So we're going to start out by talking about Java, what it is, and why do we need it?
Then we're going to discuss a few basic concepts like variables and data types in Java followed by which we are going to talk
about operators control statements and methods. We are also going to discuss the meat and potatoes of this session classes objects
and object oriented programming Concepts in Java followed by which we are going to talk about exception handling
then we're going to discuss Java collection Frameworks and finally close this Action by discussing the Dom parser in Java also do click
on the Subscribe button to never miss an update on the hottest Technologies by the Edureka YouTube channel. Okay, before we start off let me introduce myself.
I am vinoth and I have been with this industry IT industry specifically into Java development for last 12 years.
Alright, so the first topic is about introduction to Java. So what are we going to cover in this particular topic? So it's going to be introduction to Java
what Java is all about will have Java installation done as well. So that you guys can use it in future. You guys can try your hands on we'll talk about modifiers
and variables in Java. This is pretty much similar to what we have in other programming languages will talk
about Java data types as well, which is nothing but data type as we have the it's a storage for different data types,
right or the data that is store whatever you want to process. Song is first put into memory
and that is what data type is all about. So you have different storage is like for character you have something else
and for float you will have something else for long. We will have something else sort of data type conversion in Java.
So so you could convert from one data type to other data type in Java. There are implicit conversions done.
We're in the programmer need not take care about it. Java programming by itself would take care about converting it which is implicit conversion and there are few conversions,
which programmers have to take care about they have to explicitly mention that you have to convert from this data type
to this data type. And if you don't do it you would end up in compilation errors or something.
We'll talk about operators operators are nothing but the operators that work upon this data type
and which is nothing but transformation. So you kind of use this operators to add up for say for example Of two integers you want to add it up.
So you would have this operators used. We'll talk about control statements in Java as well control statements.
Like you have if else and for loops and stuff like that, so we'll talk about it.
So Java is pretty much similar to other programming languages thing is it has some unique features
which makes it really important in the current industry the IT industry when it comes to Big Data and stuff like that.
So we'll touch base on all this important features of java, which would help you to understand. Why do we really use it?
Alright. So the first topic is Introduction. So what is Java so Java as we said the programming language
which has object-oriented programming model. So when I say object-oriented programming model everything in Java is taken as an object.
So object is nothing but something that has got State and behavior say for example, you have chair, right?
Jed has got a particular State and it has got a particular Behavior. If you do something if you move it,
it would go this way and that way and stuff like that. So basically any object
if we think about in reality realm any object for that matter would have some kind of state and it would have some kind of behavior
which incorporates on its own. Right? So basically similar to that Java is programming language,
which is based on object oriented programming model. So everything Java could be thought of as an object or you define a class.
So say for example you have house right? So house could be thought of as an object or class rather a house has a class
and it would have certain attributes right like number of rooms or stuff like that. So that is nothing but the state of the house.
So this was actually brought in by Sun Microsystems in 1995, and basically Java is nothing but we have jvm but jvm is nothing but you kind
of kind of can say it's a specification right Sun Microsystems has said that this is how it should be so that any company tomorrow
I can go and create my own version of java, but it has to be aligned to this particular specification that is given by Sun Microsystems.
So Java is nothing but reference implementation of java is provided by Sun Microsystems, but nevertheless
as I said give him itself is a specification which anyone can Implement actually but we will talk about Sun Microsystems talk about the reference implementation
that is nothing but one created by Sun Microsystems. So everything in Java would have a state and behavior and which would be defined by class.
Again, it was started off by a team led by James Gosling and we know it's open source, which doesn't stop anyone from putting in whatever code.
I want to still we have lot of versions coming in Java. So it's not that you just have one version created by Sun Microsystems
and it's been used across the industry. You have like find some issues or if they want to have some kind of enhancement
in the open a particular module the keep doing it. So right now we have like Java is at version 12, which is like we have lot of enhancements coming in.
It's a evolving thing it's ever evolving thing. And as I said, this is open source tomorrow. If you become a good programmer in Java,
you could go and actually write something for Java and which would be moderated by someone and which would be put as open source.
And anyone can use it other thing is Java the very important feature why we use Java or why Java is so widely used is right once and run everywhere.
So you write a class or you write program once and you compile it and this particular compiled version of class.
Be run anywhere on any machine or you could transport this particular compiled version of java, which is nothing but classify we call it as a class file again,
you did not get into what is class file. But just to say that you compile it you get a compiled version of it
and this compiled version of class could be transported anywhere in the network or you could put it anywhere and just run it
so you could take it on your pen drive as well and go anywhere and plug your pendrive
get this class path download this class path or download this class rather and just run it so it right once and run everywhere
and the magic part is it could be run on any environment. It could be run on any platform. That's why it's platform independent.
So I could write a program today on Windows compile it and tomorrow I can just take it on my pen drive and run it on Linux as well.
So you don't have to take care about it. Java by itself has a feature to make it platform independent. So As you could imagine this is one of the reasons why
it's been widely used because since we are getting into a phase of distributed computing,
we cannot have everything run on same platform. You don't know where your so you as a programmer write something
but you don't know where this particular program is going to run. It could be on any platform.
So this is why it is more important. So unlike dotnet, right? You need to have Microsoft
just to give you this thing comparison with dotnet so for.net you need to have Microsoft stack implemented,
but for this you need not could be run everywhere. So Java can be used to create complete applications that may run on a single computer
or can be distributed among servers and clients in the network. As I said, this is more about the application
of platform-independent programming language. So it could be used on any machine you need not take care
about which machine your program is going to run on. All right, so let's talk about Java. Earth so what features do we have in Java?
First thing is Java is pretty much simple when it comes to SDK or when it comes to API application interface.
It's pretty much simple. It's kept as simple as possible. It's pretty much similar to other programming languages
and it's verbose. So anyone can just read through it and understand what it is trying to do
so they have kept it to that level so similar to any high-level languages, right the coach should be such that
as you read through it. You should understand what it is doing and that's what they have tried to do.
Its high-performance. One of the things that I already mentioned about. It's an evolving system.
It's not that it's been just done once and been used throughout its evolving system. So as and when this continuous Improvement done, right,
so as then when if I as the application programmer if I find something not working as expected I can raise a ticket
and they could go through the ticket or there's a Java development. Which has been driven by Oracle.
Now, they would look at the priority of this particular stuff. And if there are a lot
of tickets been raised by other companies as well. And if something could be done on the aspect on the particular aspect
that you raised they would surely Incorporated and notify you in a particular release the enhancement is coming up
and stuff like that. So it's a evolving system and you know, the performance is always
if there's some drawback or if there's something not working as expected they would surely Rectify it in the future releases.
So that way it keep it up to the mark the keep the high-performance thing going it is secured. So Java basically runs in virtual machine sandbox,
and no one can get into this particular space. So no one can intrude it. I would say
so that keeps it free from virus and stuff like that or untrusted process. And other thing
that is important is unlike C. Java doesn't expose pointers so in see There could be malicious user who could get into the memory location through pointers
and can manipulate the contents of a particular memory. Right? Whereas in Java.
It doesn't expose the pointer. It doesn't give you the pointer to a memory location. So you can't actually go and change the content
of a memory location when you are using Java so that keeps it secure. So it's robust.
So Java checks the code during compilation time and runtime. So basically what happens is as I said when you compile it you get a class file
and whenever you try to run this class file on any of the machines, it will ensure that it's properly done
or if there's some issue with the class file. It would say that it's a correctly classify. So basically you have some kind of checks done
during the compilation as well compilation is nothing but if you have something tactical error say, for example,
if you miss the semicolon at the end of a particular statement or an end of any statement, It would prompt you during the compilation time
that this is not what is expected or it would ask you to correct it once you have everything as for the expectation,
it would compile it and create a class file and when you put this glass file to any of the machines during the runtime it checks as well.
And the other thing is about you don't have to take care about memory allocation and releasing unlike in other programming languages.
Java by itself does garbage collection garbage collection is nothing but when a particular memory is not being utilized
by your program of goes out of scope it would Java by itself has a thread I don't want to get into threads
and stuff like that. But Java would enough the memory contains by itself. You don't have to explicitly write a code to clean
it and see you have to write a code to clean. It errors in Java. It's done by itself.
So that makes it robust other thing is it's portable as we already spoke. You can write a programming windows.
You can run the same program or you can write a program in Windows compile it get a class file or the compiled version of the class and just put it
on any platform. It could be Windows. It could be you run it on Unix or Linux or Mac
or anywhere so you don't have to take care about it. So Java by itself does it and that's where jvm comes into picture which we'll talk
about in the the further slides. So other thing is it's Dynamic which means that it has runtime polymorphism.
So all the objects are evaluated during runtime. It's not just that it's done during the compile time. There.
Is this something done during the runtime as well. So basically it's called as runtime polymorphism. But just to keep in mind not everything is linked up
during the compile time is something done during the runtime as well. So it could be distributed as well.
You don't have to have all the code put into one machine and run it from my machine. I could run something written on your machine as well.
If into net in the same network or if if they are exposed to a network we could run programs on different machines. So that's what distributed is all about.
So you could think of big data. So this is one of the major features why Java is been widely used
in big data, right? So big data is nothing but you have chunks of data you have like petabytes
of information coming in every minute or every hour or every day, right and you want to process it.
So it cannot be done in one machine if you want to run everything on one machine as we had earlier, right?
Everything was monolithic everything was done on one machine sort of so that's where you would want to have servers right.
Now. The server thing is it's not being used that much since there's a lot of English.
Intense stuff like that. So distributed computing is nothing but we have like pure computers or normal computers
that we have and programs are actually done on this machines rather than rather than having a high-end machines.
So basically this feature is what helps Java to be used in big data. And as I said,
it's run on commodity Hardware as against server Hardware which needs a lot of investment for companies. Java is multi threaded so multi-threaded is
nothing but so you have multiple cores on your machine, right? You have like four cores and stuff like that.
So you could utilize all this for course say for example, you want to do addition of two numbers and multiplication of two numbers right different numbers.
So you don't have to run everything on One Core. So this could since you have four processors right for course,
you could have addition run on one core and you could have this multiplication going on at the same time at the same instance, right?
You could have this multiplication going on as well. You could run them as a thread right? You don't have to have one waiting for other.
You don't have to do it in a sequential manner when you're operating on different variables, right? So that's what multi-threaded is all about.
As I said you could have this operations carried out on different course in the same instance of time. Object-oriented again as I spoke earlier,
everything is performed using objects and Java can be easily extended since it is based on object model.
Now, let's talk about which sectors or which industry sectors actually use Java. So you have Android apps.
So Android apps Cal could be written on Java. So basically the underneath is Android API, which is similar to jdk so jdk is nothing
but Java development kit. It's widely used in financial services industry. If if you would have some idea about it.
You could see that mostly all Financial companies use Java. So the Legacy systems
that we have in financial companies is mostly developed on Java. So one of the aspects for this is more
about it's pretty secure. So that's why it's been written on Java lots of global investment Banks.
Like Citigroup Barclays Standard Chartered and other Banks use Java for writing front and back office electronic trading system.
So one of the main features why it's been used in financial industry is about security. It provides high level of security
as we spoke earlier Java web applications. So basically you have web servers on which your application would be hosted
and you could request for a particular JSP or JSP is nothing but a dynamic HTML page right there in the contents could change basically you could request
for some particular JSP and you could get it so say for example, you are trying to log on
to a particular application you get a login page that is nothing but a JSP. So basically a mini of the web applications
is developed on Java as well. One of the main features why web applications are developed because you have a lot of web servers supporting,
Java you Have to deploy application on a particular web server and could access it from anywhere across glue.
You should be on internet though. Embedded system when it comes to embedded system. It has to be lightweight, right.
So Java it I remember Java version 8 had lot of feature making it pretty much light weight when it comes to embedded systems.
So basically you have Micro addition j2me. What we are talking here is j2se and the thing that we so there are three flavors of java.
So one is j2se, which is nothing but standard edition and which is standard edition,
which you have like all the data types and stuff like that all four loops and stuff like that. Right that is about j2se.
You have G to EE which is Enterprise Edition, which is nothing but the web application that we spoke about and we have j2me
which is micro Edition, which is nothing but for embedded systems as its name suggests, it is micro Edition,
which is light in weight. And as we can imagine that in embedded system, it has to be lighter since it is burnt into a chip,
right and you cannot have a big application. So that's why we have lightweight when it comes to j2me.
So again as I touch base on this it's about web servers and application servers which makes Java compatible for web applications.
So we have Apache Tomcat which is pretty much widely used which was a web server earlier. Now, they have Apache Tom e or which is Tom double e
which is nothing but application server version of it. So basically when it comes to web servers or application servers,
what you do is you as a client could send a request to our web server and web server
or the application server would just process this particular request and send the response the way the It's written.
So basically it would serve the expected result out. So you have Enterprise applications. Again, this is Java Enterprise Edition,
which makes it favorable for web applications. So all these specifications j2me. J2w.
J2se, this are nothing but specifications exposed by Java. So if you as a developer wants to do something or if you want to write your own version of it you can do it.
So where is Java used again scientific applications? So I as you could imagine in scientific applications, you need to have high level of accuracy
and precision rather. They could be mathematical equations and mathematical computations which needs lot of CPU time
because it's very CPU intensive right? When you do a very precise calculation with high level of high degree.
It needs lot of CPU time, right? So that's where Java is good at. So basically this is about as a spoke about multi-threading
utilizing all the course to the fullest suppose. You have four core processor using all these four cores is been done by Java Java has feature
or through threads you could actually read through or actually utilize this for course to the fullest Big Data Technologies again,
big data is nothing. But as I said, it's distributed you cannot have everything run on one machine,
which was the case earlier. You would have one server put up and everything is done on that particular server.
Now, it cannot be the case. That's where Big Data comes into picture wherein you have distributed architecture
and you have this data being processed across Network rather than just on one machine and mind you as I said,
this machines are commodity hardware and not server level Hardware or the Enterprise level Hardware
which makes Cheaper Internet of Things So Internet of Things is coming up the booming thing wherein you could connect things together.
Say, for example, you have cars, right? So you could connect cars together so you could have sensors in your car
which could emit data and you could connect them together. So basically it's all connecting things together or say, for example, let me talk about internet itself.
So earlier if you remember computers were not connected it were all pure right. It could just be used for your own purpose.
Now internet has started wherein you have all these computers connected and if you are connected to the internet,
you could go onto Google and search something or whatever you want to sort of So basically this is what internet of things is all about.
So consider this instead of computer. It could be any other objects a car for example, as I said so consider car has sensor
and it is emitting this sensor data every minute and we could utilize this sensor data and do something with it or one car
can know where the other car is. So that's what internet of thing is about all about connecting things together.
Let's start off with the setup setup for Java. So basically you could go to this particular site and just download the SDK based on this jdk, right?
So basically based on whatever platform you are at. If you are using Windows, you have to select Windows if you are using something else
if you have mac, you can select Mac. So this is pretty much similar to other softwares you get an exe file download it
and you just have to run that exe file. So basically I can show you what gets downloaded. So I have downloaded version 12 for Java
as you could see here and and since its Windows, it is mentioned as Windows. So this is nothing but an exe file that you get
which you could run and you would have Java installed on your machine. So it's pretty simple.
It's pretty much similar to other software's you have say for example, or I could just show you the location
where it is installed. So if you get into the program files, you can see javabean installed here, right?
And it says jdk 12. So once you install Java, once you download this particular stuff jdk and
once you install this this executable you should be able to see this Java folder in your program files.
So it shows here. It's for you can select whatever you want to see you have as you could see here.
This line X there is Windows Mac OS and stuff like that. You have to select the platform you are
on and should be good to go. This is done. You could see you get a exe which you have to run.
So basically to run Java what you have to do is yeah. So you go to the system you have control panel system you go here
and you have to click on Advanced system settings. Basically, what we are trying to do is we are going to set an environment variable right
and what we are going to set is this path variable. So you have if you want to put it specific to a particular user you
can put it here put it in the upper space or you want it across the system. You could basically put the path variable here
in the system variables. So once you install it you have to make sure that you have this particular
path being put in your path environment variable. So basically tells the OS that you have executables within this particular directory.
So once that is done, once you have this properly setup, let me open a partial.
So partial is nothing but it's pretty much simpler to command prompt but it's a Linux flavor of it.
So basically you could run Linux commands as well. Okay, so I will I will once this is done. Give me a second.
Okay. So with partial you can do LS which would give you a listing unlike in command prompt.
You have to give dir. So it's basically good for programming. So once you have this properly set
once you have this path properly set if you write Java here, if you write Java on your command prompt,
you should be able to see this help coming up here. So if it's not properly set up, you won't see this it would give you unrecognized command.
Yeah, open command prompt and type Java. So once the path is properly set you should be able to see this. So let's set up Eclipse as well.
So it could be eclipse or IntelliJ whatever you are comfortable with so you could use either of them
since IntelliJ has much more features. You could use IntelliJ as well. So you go to Eclipse download
and whatever flavor you can take the latest one Whatever flavor you want to install if you if you want a simple one you can do it.
If you're trying to do something on web. You can download that one as well. So basically for simple purpose
for whatever we are going to do you can have normal ones. So let me quickly open up my so this is nothing but the IDE integrated development environment
that I use with just IntelliJ all the IDS are pretty much similar. So IntelliJ similar to Eclipse, so it's coming up.
So basically what you do is you have to select a workspace first workspace is nothing but all your program
all the class files all the class and everything that you write would be put into this particular Works piece and you can create a new class and start off.
So when we get to the hello world program, which is the first program that we are going to talk about it would give you a clear idea
on what we are talking about. So let's talk about Java internals how it looks like
and all the features that we spoke about. So basically so far what we have covered is more about Java is object
oriented program or it uses object-oriented programming. Paradigm which is nothing but everything is thought of as a class with a particular State
and behavior right is platform independent. That's why it's been widely used it secured as well. That's why it's been used in banking applications and stuff.
It's good for distributed computing since its platform independent. You don't you just have to write it once
and you can run it everywhere. So basically this platform independent aspect is what helps it to be widely used in Big Data applications.
So let's talk about the internals. So what is jvm jvm is nothing. But again, it's a specification right?
You could have your own jvm son by itself gives specification for a jvm. This is what the GBM should be all about so
I could create my own jvm. But we usually we use the reference implementation of sun Microsystems Oracle now.
Right, so jvm is a virtual machine that runs the bytecode. So basically there has to be some level of compilation done
before give him can understand it. So that's where you have Java compiler. And so what this does is nothing but it takes your source code.
It takes the class files as or it takes the class that you write as is so Java compiler would consume it
and kind of compile it into a DOT class file so dot class file is nothing but compressed version of it and compiled version of it as well.
And this dot class file is nothing but it's also known as bytecode and this is what a jvm could understand.
So once you have this class file, it could be sent to the jvm and jvm could understand it and run the applications.
So what makes Java platform independent is this Java compiler is platform. Pendant you don't have to take care about it.
You just have to run the compiler. And whenever you want to run this particular byte code on any of the machines,
you just have to have jvm for that particular machine. Right? So basically if you want to have online X,
right if you want to run it on Windows, you would have jvm installed on Windows or you would download the jvm for Windows.
And if you want to run it on Ubuntu or the Linux or Mac would just have to have the jvm downloaded for that particular platform
and it should be able to sense this particular byte code. So basically this is what makes it platform-independent. Right?
As I said, once you have this bytecode once you compile it this bytecode could be transported or ported into any
of the machines in operating systems and you should be able to run it that is what it is all about.
It's right ones and run anywhere. So as I earlier mentioned you could As get this class file into your pen drive
and take it anywhere and you could just plug your pen drive and just run through
that's how simple it is. So, this is Java runtime environment where in you have set of jvm plus you have libraries libraries,
it does nothing but you know, although programs put together all the programs source code
for Java put together. So our T dot jar is one of the libraries which would have most of the classes
or you have you till classes maybe string class string is a class itself, which is exposed by Java.
So this particular jar file or it's a Java archive jar is nothing but Java archive which is set of java classes or the class files rather
which would have lot of class files within so in our T dot jar you would have something like string class and stuff like that a lot of other classes,
which is been used by the sea. Some are which has been used by Java itself? So basically in JRE this is
what you have jvm plus set of libraries plus other additional files. So as spoke jvm is nothing but specific to environment,
but all these things like Artie dot jar and stuff like that would be independent GRE does not contain tools
and utilities such as compilers or debuggers for developing applets and application. So GRE by itself the runtime environment,
so it doesn't have compilers and stuff like that. So jdk is nothing but it specifically for development you could see here.
It's JRE plus development tools you have compilers and stuff like that as well. So to run a class file,
you just need the JRE but if you are a developer, you would need jdk so what we downloaded earlier if you remember
that was jdk right jdk for Windows or jdk for liners. So basically if you see this one could see here as jdk. So essentially you need jdk only if you are a programmer
if you just want to run the class file GRE would suffice so why is jdk GRE and jvm as we spoke about you could see jdk is a superset.
It has everything right GDK would have your jvm. Plus it would have as we spoke here. It's JRE plus your development tools as well.
As you could see here details it has everything here. It would be really confusing right now. If you directly get into what all this is all about,
but basically you could imagine jdk is a superset and Jerry is nothing but it's your runtime environment which has jvm and some additional files
or RT dot jar as we said which is nothing but set of files set of class files
that is exposed by Java which could be used like string for example, right?
Let's see how it works. So basically you have your source file. So this is what a developer would typically right
would create a DOT Java file. So whatever you write you create a class. You just create a new class and write something to it.
What you get is dot Java you get the extension dot Tower. So basically this is your source code, whatever you write you
as a developer would write this class. Is would be sent for compilation or whenever you are good enough done with your coding you would compile it.
And once the compilation is done once everything looks good enough. Once the compiler
doesn't Mark anything and doesn't flag anything rather, which means everything is syntactically, right? You will get Java bytecode.
Just nothing but dot class 5. Now. This dot class file could be moved across Network
or you could as I said, you could just put it in your pen drive and can take it anywhere
and you could run it on any of the environments. You just have to have jvm. So basically you could run this class file,
which is compiled on Windows. You could run it on any of the environment any of the platform's.
So this is something that we spoke about during runtime. There's a verification as well. So so when classloader would load this particular class file,
it would ensure that it is up to the Mark or it's not manipulated sort of so if it all it sees something wrong,
then it would flag it off as you know corrupted file or something. So this is nothing
but Java class file libraries are T dot jar that we spoke about Java archive. So this is what is happening.
When you run a particular class five, right? So you have this class loaded and then you have this compilers just-in-time compiler actually running it for you.
So basically the part of jvm so jvm is remember jvm is nothing but it's a platform. It is specific to platform.
So basically for Windows you would have a different jvm. And for line X you would have a different jvm. So yeah, you get this class
you load this class using class loader and you run through the application or run through the class files.
So typically this is how Java operates so just to give a just on this one. So you have a source code you have dot Java file written
you compile it you get our class file and you can put this class file anywhere. You can run this class file anywhere on your network
or wherever you want to you just have to take this class file and on the machine on which you run. What would happen is
you load this class file first using class loader and with jvm jvm would have just-in-time compiler which would run a particular class for you.
Right? So what we are trying to do here is you're running the source code
that's written on any environment you are running it on any other environment. So basically you could write this on Windows.
You could write source code on Windows, but you could run it on say for example line X. So that is what makes it platform independent.
So here let's create one class and as we created I will More about it so that you could open up your eclipse
and could create something like a new project so it could be a bit different for you the look and feel might not be exactly the same as mine,
but you just have to create a new project select Java. So basically I could a project name. So whatever you want to so basic nomenclature is
like it has to start with uppercase letter, which is camel casing right? So you should follow camel casing venue.
That's one of the best practices it won't flag you as some error or something, but that's a normal industrial practice that
whenever you create a Java project. It has to use camel casing created record, Java. So it's creating a project for me.
And as you can see here, I have this particular project being created right now. What I do is I create Java class.
So basically as we said everything could be seen as a class, right that's what object-oriented is all about.
So basically what I would do is I would create a class here. So this is my source folder where enormous Source class would be there.
So as you can see here new Java class so say I create calm dot Ed u-- Rica. So this is nothing but your name space
or the package, right? So you could give whatever you want to basically this is to avoid collisions, right?
If we don't have a name space there would be lot of collisions within the class. So there are a lot of people working
on the same project right so I could create a class with name. Class A and the other person might read classy as well. So when this is club together into one particular application
there would be Collision So to avoid this basically what you have is you have a namespace.
So I would write as calm down dear a card dot Class A. The other person would write something else and that would avoid class name collisions Applause collisions.
So this is compounded Eureka is my package or namespace and hello world is my Java class name. So as you could see here,
there's a package calm down Ed Eureka, which is again a namespace and there's a class by name hello world that's been created.
Right? And if you want to see you could actually go and so you can see a folder structure
created here in the source. So this is your Source folder right within your project. There was a source folder where in we created the class.
So if you go to the source folder you could see a packet structure or you could see a directory structure being created
which starts with calm and within calm you would have at Eureka and here you can see Hello World dot Java.
So remember we said that your source code would have all the Java files. So basically this is what I was referring to.
This is a method that the default method that's been called. So when you run this particular class file
jvm would actually look out for this particular method. So you need to have the same signature as has so you would have a main
method wherein your program execution would start, right? So as then when you compile it and run the class file, so basically when you compile it you would get a class file,
right and when you run this class file jvm would check out for this main or a method that has the same signature as this one.
That's a main method and your program execution would start running from here. So basically it's a entry point for your execution, right?
So this would be a simple program wherein we would just print hello world. So basically you have
like system system is a class and this is how you print in Java. So I don't want to confuse right away by saying
what is system worries out and println, but basically what you have is a system is a class as I said everything Could Be Imagined as a class.
So in Java everything is a class. So basically you could just click here and you could see the source code of it, right?
This is the source code and this is coming from our T dot jar remember in jvm. We spoke about in GRE.
We had our DDOT jar. We had Jerry we had jvm plus plus class files. So all this has been written by Java by the Java community.
So basically you can see here. This system itself is a class. So out is instance variable
and println is nothing but a method within out so what this statement particular thing is going as nothing but writing it on to your console.
So let's print hello world. So basically when you build a project it's compiling it as you could see here.
It's saying it's building it. So once it has built it you could see a class file here. Right?
So you could see here hello world dot class. This is because we built it when you build it. Your Java file would be compiled by your compiler
and it would create a DOT class file. And remember as I said, this class file dot class file could be run on any machine.
So this is Windows machine that have written this file Java file and compiled on now.
This dot class file could run on Linux or any platform that you want to run it on. So, let me talk about this class.
Right? So you have a public key word, which is nothing but access modifier
which gives visibility. So basically what we are trying to say here is hello world is publicly could be publicly seen
so it could be seen anywhere within the application. So basically for class level you have public which as the name suggests.
As the name gives out it could be seen anywhere within your application that the visibility key access modifier.
All right, so you have the keyword class here, which is for the class. You need to have it mentioned as class.
If you write something else, then it would give you a compilation error. So it has to be exactly the same.
All right, that's the syntax of it. Hello is nothing but the name of your class, then you have this public static void main,
which is nothing but as I said, this is the entry point for your application or your class file.
So when you run this particular class, it would start off from here. You have a static keyword static keyword is nothing
but it's at the class level. So basically you need not create instance. We haven't reached that point yet.
So basically hello is a class and you could create instance of hello, which is nothing but object right?
So when you have a static keyword here You need not create object of hello to run it if it's not static,
then you need to have object of hello created. So basically what I'm trying to say is see you have one more method here,
which is test. Now this doesn't have a static keyword here. So basically what we can do is we can print So
yeah, this is a non-static method right now. We cannot call it directly. If it's a non-static.
You need to have a instance of Hello World created. So basically how you create instance is nothing but hello world.
That's your hello world object. So when I say instance I'm talking about creating object, right
and you could create new hello world. So object of hello world is created using new keyword right? When you do this you would get object of it or now.
What I do is so basically what I was trying to say is you cannot call test as is if you do it you would get a compilation error saying
that non static method test cannot be referenced from a static context, right? You cannot call it without having your object.
So basically I can call it on Hello World object DOT test Now I can call it now. It doesn't give me compilation error.
I'll create one more static method which would give you some idea. So I will rename this as non static test
and this would be static test. Right and we are printing your static method and here we are printing non static method.
So hello world dot nonstatictest. Now, whereas the static test did not be called using object so we could directly call static test.
Sorry, I haven't written static here. So this is a static method. Okay, so now you could see here.
It compiles fine. Right? So what I was trying to say is
when you have a static method you do not have object to call it. Whereas non-static method you need to have object.
That's what the static is all about. Then you have void is nothing but the return type it's not returning anything from here.
So that's why it's void. You have main method. That's the key word
that the entry point for your application. You have this arguments. You can see string array of strings
which are arguments provided to a particular program. So you could pass on as many arguments as you want or if your program is say,
for example, you want to pass your name as an argument you can do that. So this is arguments then we
have main which represents the Above the program which I already mentioned then we have system dot out dot println is nothing
but the print statement so as I mentioned system is nothing everything could be thought of
as a class system is a class out is instance variable and println is a method within out. So basically you don't have to think much about it just
to understand the structure of it just to understand how a particular class is been written. This is what we have.
This is how we write a class right again, just to brief on this you have access modifier which denotes the visibility of a particular class.
You have the class name you have the main method which is nothing but the entry point for your class you have a static method,
which says whether you need an object to call a particular method or could be called directly from a class.
You have a return type here. So this is how typically any method would be written and you can write
whatever statements you want to within this particular method. This case we have printed out hello world. So basically you could follow this
when you do it on Eclipse, so it might be a bit different based on the eclipse version that you would have
but it should be pretty much similar. All right, so you create a new Java project. So I showed this already on IntelliJ,
which is also widely used IDE. But yeah, you could use Eclipse as well. So basically you
could see the project name being written as hello world, right and here it mentions the JRE to be used. So here you can see class class being created and let's see
how we can run this. So we compiled it and we saw that dot class file was created. Now we can run this.
So as I said main is the entry point so it would start running from here. So your program control would come here.
And the first thing that it would see is you have printed hello world, so it should print this one then I would keep it simple.
I don't want to let's see what's been printed here, right? So you could see here. Hello world being printed first, right?
This is where the program execution started from. So it came to mean so hello world it printed out hello world.
Then you give a call to this non static method. So it printed non-static method which is been printed in this particular method
and then it gave a call to static method and you could see static method being printed here. So one thing to remember is the program execution starts
from Maine and it just you know executes this main method. So whatever content you want to write you would basically write it in the main method
so you could see here hello world being printed out. Let's see how easy it is to coat the same in the J shell. So J shell is nothing but shell prompt
that was created in Java version 9 since nine you would have gesture. So since I have Java version 12,
I should have J shell as well. So yeah, J shell is nothing but a prompt so it's nothing but instead of writing
into integrated workspace. You could have J shell and you could pry out something here.
It's not something for production use as such it's basically to test something right you as a developer could
if you want to see what it does instead of writing everything into the main. So if you write a class you
would have to write things into the main and then run it and stuff like that with J. Shell. It's just a kind of interpreter wherein we could write something
some command and see what the output is. So basically you could say for example we printed hello world, right so system dot out Dot println.
Hello world, right if you remember this is what we typed into our main method plate. So if you want to run this you have to have
all this artifacts created as is right to have a class you need to have main method and then build the class
and then run it basically initial you could run it just to see what's output. As I said,
this is not application as such this is just for the developer to test what the output for a particular command would be
and what a modifiers in Java. So one thing that we saw in the program was access modifier.
We already saw about actually this one right public and these are public here as well. So these are nothing but modifiers right public
as I said this public means the access modifier which shows that hello world could be accessed anywhere throughout your application.
So here we can see as a board or the fries changes. Meaning of other phase in some so basically it just trying to say that one of the aspects are one thing
that access modifier could be used is to control the visibility of a particular class or a method as well.
We have access modifier for your methods as well. So this is just one of the things right we would see other modifiers
as well modifies in general has some other things as well. But when it comes to access modifiers it basically controls the visibility of a class visibility of a class
or a method or so. Yeah modifiers here as you could see there is access and on access modifiers,
let's see access modifiers. So we use public already. So this one is nothing but a access modifier
which is public which says that it could be used throughout the application so visible to the world
as it says public is nothing but visible to the world. If we talked about in a logical order if we talk about the visibility
so default is visible to the package. So default is nothing but within the package it would be visible.
So basically you could default is without any keyword without any access multi-faith. That's a default scope So
within the package it would be accessible only within the package. So you have like calm down Eureka.
That's your package. Right? So this hello world would be accessible
if it has a default scope. It would be accessible only within calm down Ed Eureka. If you try to access it
from some other package you would get an error. So that's the default visibility:visible to the package private is nothing but it is visible to the class.
It is only visible to the class. So this is the lowest visibility only visible to the class. If you try to access it from outside class even
within the same package you would get an error because You won't be able to access it is accessible only within the class public is accessible to the world.
It could be accessed from anywhere within your application or anywhere within your wherever your class file. So basically if you if you have a jar file,
but what I'm trying to say is public is accessible anywhere right protected is again visible to the package and all the sub classes.
So we will talk about sub classes. So subclasses is nothing but in C we have inheritance, right say for example
integer integer is a class as I said in object-oriented Paradigm or in Java everything could be visualized as a class.
So integer itself is a class, right? So there's a number there is a super class which is number which has all the common state and behavior
that a particular number would have right so number is a super class and you have subclasses
like Teacher float would be a subclass of number long would be a subclass of number. Basically.
This is inheritance, right you are inheriting integer float long double and everything from number class.
So number is a super class and all this integer float and everything is a subclass of number. So when it comes to protect it,
it says that it is visible to the package and of the sub classes, so just to keep in mind as of now,
you can think of access modifier as visibility of a particular component or could be visibility of a class or visibility
of a method or instance variable as well. This is what drives the encapsulation factor of object-oriented Paradigm.
So basically we control the access or all this components or we control the access of class variables methods and everything
which makes it encapsulated cannot be breached. Sort of as you could see this is like I could make this one as default as well.
All right, so this is default scope. It would still run because it's within the class. Right now suppose I make this one as private, right?
Just to show you what this encapsulation is all about or what this access modifier is all about suppose. I make this one private right still this one runs
because it within the class you are trying to access it within the class now. I create a new class say for example,
we say axis modified test. So I create a main class here again main method. Now suppose if I try to run this, right?
So basically I create the same stuff here. See I create a hello world object. Okay.
Now this one is private right now. If I try to access it from here, what I was trying to say is if I SEC
as you could see here it says that nonstatictest has a private axis to hello world. So you cannot use it from outside.
That's what I was trying to say. So basically since it has got a private scope you cannot access it from other class.
And if I remove this private now with gives it default scope and as we said default is nothing but it has got access
within package you could see this error going off here now it is accessible, right? So that's what is it is all
about when you have a private scope. It's within a class. There is default is
within a package public is accessible anywhere and protected is visible to the package and just the subclasses.
So let's talk about non access modifiers. This is not controlling the axis of class method or variable when it static you did not create object of a class.
So basically for static test you could see that we didn't create object of a class. We didn't call static test on a particular object of class.
It could be called directly. That's what static is all about the static modifier for calling methods
and variables without an object to which it belongs as we saw we didn't create object of our fellow world we directly cause the static method final is
nothing but you can't change it final as the name suggests. You can't change it once it is created.
So finalizing the implementations of classes methods and so this is nothing but instance variable
which we would be talking about the slides to come just to tell you this is an instance variable
which we have assigned the value 10 now suppose within this particular method I try to change the value to 11. You would get an error saying
that cannot assign a value to final variable. So final is like its final can't change it once you have created it.
You can't change it. But if I remove this final you could see this error would go off.
You don't see this error anymore. Whereas by putting final you would see this error. So that's what final is all about.
So basically this is good enough for constants, right? So if you have constants within your class you would make it final
so that no one can change it abstract is nothing but you could mention it as abstract when it doesn't have implementation
of all the methods. So what I am trying to say is abstract modifier is again a non access modifier and what it tries to say is say,
for example, you have a shape class cheap class is abstract class because shape doesn't say you want to calculate
area of shapes. So basically shape class by itself wouldn't know what the area of the ship would be.
Is a class where in the generic class it doesn't know what the implementation of area would be 4 Square. It would be Side Square
for rectangle its length into breadth or Circle. It's pie R square. So basically shaped by itself wouldn't know
what it's area would be but now shape is a super class and say you have subclasses of it like Square you would have Circle and so on.
So now basically you want to ensure that when you create a subclass of the shape class you want to ensure
that that particular class implements the area that's when you create it as abstract. So the shape method would have
of the shape class would have area method as abstract which would be implemented by the subclasses which has to be ensured its kind of ensuring
or if the subclasses don't actually implement it you would get a error. So basically Circle would have its own implementation of areas
in pie R square square. Would have its own implementation saying Side Square.
So basically what I'm trying to say is area is abstract method for shape class. And when you have abstract method the class
itself is abstract. So you have like shape calm down Eddie record dot shape. I'm creating a class now.
I'm creating a abstract method So when you say abstract, you don't have to provide implementation. It's just a chain break this thing you don't have
implementation of it. You just have the signature of it. So basically as I said,
when you have an abstract method the class itself should be abstract. So we'll have to make this abstract as well.
So you created a shape class which would have a area and which would give you this thing. So basically we want to have subclass and want to show
how the sub classes are created at this point. But basically this is what abstract is all about. You don't have the implementation of it,
but you are ensuring that subclass is implemented. So here you can see Just a signature. You don't have implementation
unlike this implementation is nothing but if you write something within this braces, this is an implementation for this particular method,
whereas in shape class. You just provided the method signature, but we don't have limitation of it.
So that's what abstract is all about synchronized and volatile is much about using threads synchronized is we are saying
that only one thread could get a control at one point. So as we said threads are nothing but parallel execution you could have thread say for example,
you could have read one calling a method. So we have as non static method test right say this is a method.
So what we are trying to say here is if it's synchronized we can make this a synchronized private synchronized.
So this is what id is all about. So when you have this integrated development environment, you don't have to type everything
when you type S why you could see synchronized coming up here. Put selected. So when you put a synchronized here,
what we are trying to say is only one thread could access it at a given point only one thread at one point. So if multiple threads are trying to access it
one thread has to wait for it. So only one thread would get entry to this and the other thread should wait for it.
So that's what synchronized is all about and volatile. It's basically for memory visibility or what we are trying to say is so basically every processor
has got its own cache. So what we are trying to say is when you use a volatile access specifier don't store it
in Cash Store it directly into the main memory so that all the threats would get the most recent value being assigned
to a variable also volatile is not needed when you have using synchronized as such so it's mutually exclusive.
So let's talk about variable. So variables are nothing but it's a holder, right? Holds value and variables are nothing but it's reference
to that particular meal or it's a dress or something that is pointing to a memory location a memory location
where the squirrel use are being stored and you could access it using this particular variable name or you could access the memory location
where the value is stored using a variable name what I'm trying to say here is so this is a variable and you could access
so this 10 is stored somewhere in the memory and you could access this part of memory location where this 10 is stored using this variable
or you can manipulate it as well can change the vat. So basically there are three types of variables in Java this local instance class or static local is nothing
but local to your method whenever you have a method or you kind of create a variable within a method that is nothing but local scope which is
like it has the axis its visibility only within that. Particular method once control goes out of this method you can no longer access is particular variable
that is local. So if I Define something here say, for example, I Define something here.
So this is a local variable since its defined within a method. So once the control you would have this only
within this particular method, once the control goes out of this method. This is no longer accessible.
Basically, this is where garbage collection for Java is useful. Once you control goes
out of this particular method garbage collector would kick in and were dean of this variable or clean of this particular memory location or would make
it available for use. So that's the local scope. The next one is
instance instance is nothing but something that is defined at the instance level. So this is instance variable.
So since it's at the class level, right this is you could see it at the mid-level. This is at the class level.
This is nothing but an instance variable now there is static variable as well. So this is how you define a static variable.
So instance variable is nothing but it's per instance so you could go and change this instance variable to something else suppose.
I assign a value for T here now. I could have one more object created suppose I call this object one
and I have Hello World object. So I create one more object here. So what we are trying to do is we are trying
to change the instance variable through objects. So basically this is what it is when you have an instance variable normal instance variable,
which is non static you could access it through objects, right? So throughout object one you
assign for object one you assign instance variable value to 44 object to rinse you change the instance variable value to 50.
I won't say Changed it. But you assigned it. This is how you deal with instance variable.
It's at the instance level. It's at the object level. Whereas for static.
It's at the class level. It's one per class. It's not one per instance.
It's one per class. So basically what you can do is you could do something of this sort where in its at the class level.
It's not at the object level. You are assigning value hundred to a static variable. So as you could see here,
it's not a particular instance that you're operating on its the class directly just to give you a just we have local variable
which is within a particular method and scope remains within a method. Once the program control moves
out of this particular method Java would or jvm would come in and kick in garbage collector to clean this off Sony accessible within this menu you have instance variable,
which is at the class level, but it's non-static and which means that it has copy.
Per object when you have multiple objects, you could change the values the way you want per object static is nothing
but 1 per class and as you could see here, we change the value of the static variable 202 class. So that's about variables.
We have an instance. So instance variables are declared in a class when the space is allocated.
I will just read through this one. So when a space is allocated for an object in the Heap slot for each instance variable is values created.
We had a your instance variable and whenever you create but basically an instance of a class is created in a part of a memory,
which is called Heap. So whenever hello world object is created a slot for this instance variable
would be treated as well instance variables are created when an object is created with the use of keyword new and destroyed
when the object is destroyed. So unlike local variables, which is within the method scope.
Variable is within the class scope. So basically whenever you create an instance of a particular class,
your memory would be allocated for that particular instance variable. And whenever it's done
whenever it's destroyed by the garbage collector, it would go off access modifiers can be given two instance variables.
You could have like private public protected default all the access modifiers. We assign to students variable.
So basically you could have it as private private is just within this class. You cannot access it
outside this class you could have public as well, which is like would be accessed anywhere within this application you could have protected
which is like within the package or the subclass and you can have the default one which is nothing but package axis,
which would be accessible just within this package that is calm dotted Eureka. So instance variable have default values
for numbers the default value zero for Boolean it is false for all. Big references it is null
so values can be assigned during the Declaration or within the Constructor you could assign values directly while declaring it something
like this instead of 0 is the default one for integer as they have mentioned. But yeah, you could assign
if you put it as 10 here it would be taken as a default value zero zero Constructor is nothing but a method
which constricts an object of a class values can be assigned during the Declaration or within the Constructor instance variables
can be accessed directly by calling the variable name inside the class. However, with static methods
when instance variables are given access ability. They should be using fully qualified name. So what we are trying to say here is this is a static method
from which we are trying to access instance variable in this particular static method you need to have object reference.
This is Object that we created so object reference dot instance variable you need to have as we have mentioned here.
So here as we can see object reference to a variable name. You need to have fully qualified name.
When you try to access it within the static method. You need to have fully qualified name. Whereas here within a method which is non static.
This is non-static here. I can access instance variable without object reference. So here as we can see it could be accessed without but
if I do it here it would throw me an error. It's not accessible here at all. Non-static Fields instance variable cannot be referenced
from static context instance variables are not accessible directly here. It has to have fully qualified this thing,
which is object reference dot instance variable. Whereas when it's called within non static method you could use the direct so static
as we spoke about it's one per class, so it's declared. Using static keyword.
So static variables are stored in static memory. It is rare to use static variables other than declared final
and used as either public or private constants as we said, it's mostly used for constants and static variables are created when program starts
and destroyed when the program stops. So one thing to remember is it's one per class. It's not one per object instance is one per object.
We have a copy of that particular instance variable one per object case of instance, whereas case of static it is one per class.
So the scope remains are the life cycle remains right when the class is loaded in the program is stopped in the class is unloaded static variables are declared public
since they must be available for users of the class. It's not mandatory though, but usually if it's a constant it is declared
as public static variables can be accessed by A calling with the class name. So we saw here last name dot static variable.
So how do we decide what amount of memory is to be allocated? So these are the data types that we have. So each variable in Java has a specific type
which determines the size of memory the range of values that can be stored and the set of operations that can be applied
to the variable data types can be categorized into two major types primitive and non primitive. So basically primitive is not object primitive
is supported by language itself, which is kind of it has got 8 primitive or its pre defined by the language
and named as keywords and it has 8 primitive data types that is byte short integer. Then you have long float double character and Boolean.
This is pretty much similar to other programming languages that you have by it consumes one one bite. These are 8 primitive data type set of predefined non-primitive.
It's nothing but string string is object itself. So that's something that is non pyramid or if we Define
your own class C student for that matter here, right? We have defined student class that's non primitive. So this student class has non primitive you have strings
for storing string, which is again kind of non primitive. We have arrays
or basically it's all as reference variables since referencing a particular memory location through variable name.
This is where we have table showing white consumes one bite and it's range is from - 128127 short is like two bites and you could imagine
that it would be from - 32768232767. So it's pretty much similar to other programming languages that you have like in C as well we have similar to this thing.
One thing is characteristics to bite in C takes one bite. I believe though. It doesn't use all the two bites every time it.
On encoding type. So Boolean is one which is true of all since it has to store 1 or 0.
So it's pretty much similar. So just to talk on the bytes required by test 1 byte short is 2 bytes integer is 4 bytes long is 8 bytes float is nothing
but it has decimal values stored signed decimal values. So it again concerns for bites double is sign again float values
or sign decimal values which consumes 8 bytes character is 2 bytes and bullion is one bit.
So basically so decide whether to use float or double depends on the nature of the application.
So if you want to have more Precision or if you want to have more range you could go with double so non primitive or reference data type is a variable
that holds a particular object or holds bit that represents a way to access an object the pointer to a memory location baisakhi.
So as we spoke Java doesn't expose the pointer directly. It doesn't give you directly pointed to the memory location, but it gives you the reference variable.
So you cannot manipulate memory location directly. You cannot add some values to the memory location through pointers or something
but through reference variable you can access it and assign someone so yeah, it does not hold the object itself,
but it holds a reference address to the object reference type does not have size or bit range. So here we can see string Str is equal to Ed Eureka.
So the one shown in red is actually the memory we're dead Eureka is stored. You have a reference and Str is the reference to it.
So here we can see variables and data types. So we have a main method again and we have bite. We declare a bite here by D is equal
to 10 short s is equal to 20, so, I think it's pretty straightforward. You kind of have this data types created you just have
to assign values to it. These are all Primitives as you could see till here. It's primitive so you could see that values are being assigned
and it's been printed out here. So I think it's pretty straightforward. You can assign a value to a variable n just print.
So again, we use system dot out dot println for printing it you could try it out on your own. You could try it out this data type program.
You could just assign something and just try to print it out. We are talking about data type conversions. So we have implicit
and explicit conversion in some case programmers don't have to actually write explicit conversions from one data type to other
but in many cases programmers need so the arrow in the diagram shows the possible implicit type casting
that a permissible bit primitive data types. It's just with primitive data types right as the diagrams. Those int can be converted implicitly to Long float double
since int X likes less space. It could be applied to any of the numbers for vice versa. They have to be converted explicitly.
Whereas if you want to convert long to integer, you have to mention it explicitly. So basically we are talking about when you're trying
to store integer into long integer takes less space and long takes more space. So it should can be easily accommodated
since long takes more space. If it is more than the range of the integer. It wouldn't know how to assign it to an integer.
So that's why it has to be explicitly convert implicit conversions. We can on the J shell you could type this.
So here what we are trying is we have a character C is equal to a and you could see see is assigned a now integer K is equal
to C. Which could be done you could assign character to an integer. So basically it's a ASCII code for it.
So 97 is the ASCII code for see which would be assigned to K. So now when you assign see to float, which works as well,
so you get ninety seven point zero you could assign character to long as well, which is in bytes integer,
you could assign double as well, which is 8 bytes float and you could see but it cannot be done the other way around
as we spoke. So you cannot have integer or you cannot have double assigned to character.
So it would give you incompatible our possible lossy conversion from double to character. It has to be explicitly done.
If you want to do that need of type conversions. So here we have integer a full 200 initiated a variable with type Teacher then you have a string B,
which is assigned value. Hello. So basically here you can see string being used
which is a reference variable and you have string s is equal to a plus b as equal to a plus b then it adds up like hundred plus since hello is a string it would concatenated
so you could see hundred and hello being concatenated and you could see hundred. Hello the data type of both the variables are different
but to perform any operation we need both the variables to be of same type here integer value is converted into string and gets concatenated with other string.
So basically had we been an integer you would have got edition of it say for example integer B is equal to 200 and if you hundred
plus 200 you would get 300 but since it's a string in this case hundred is converted into string and it is concatenated.
Hello explicit type conversions. We saw the previous case where an double was being assigned to character
which prompted us with lossy conversion. So this is similar to that. So basically here
what we are trying to do is we are trying to assign double to integer. So you have double D is equal to 45 B,
which is 45 right again double is 8 bytes decimal signed decimal number so you assign 45 to it.
And now we are trying to assign this double value to integer. So it's possible lossy conversion, but you have a provision to type Custer.
So basically what we're trying to do here is you're trying to assign again double to integer which is possible if you type casted so
when I say typecasting it's nothing but opening parenthesis, then the destination data type then your data. To a table.
So basically to the right side you could see that through explicit type casting we can assign double to integer.
So costing may lose information. For example, floating Point values are truncated when they are cast two integers example the value of D.
That is 45.5 when converted to integer gives 45 so we could see here the bottom like the double we assigned the value of 45.5 to double
but when we convert it into integer, we got 45.5 Force truncated since double since integer since the destination data type
that's integer dozens to decimal values. Now, this is like type conversion methods which is there any wrapper classes?
What we are trying to do here is we are trying to convert 23, which is string into integer. So you have a string.
As is equal to 23 you assign the value 23 so mind you this is this is string right now. We have integer dot parse int and we pass the string
which would be converted into integer. So this integer class that you see here right here is nothing
but butter wrapper class you have string into an integer, which is nothing but integer dot value of string which converts it into digital data type itself.
So the you could see the destination is is integer. Now integer to string you have integer. We have a tostring method
which with converts integer into string. So basically you have integer I which is been assigned 23. Now you have integer dot tostring I
which would convert this integer into string. We have one more method which is string dot value of which would also convert.
Integer into string. Let's talk about operators operators are nothing but it operate on this data types.
So you have unary which is kind of pretty much similar to what you have C C++ you have post fix and you have prefix post fixes.
I plus plus or expression plus plus and the operator is after the variable which means that it would be assigned value
and then it would be added prefix is before the operator which would be added first and then assign or the operation would be done first
and then assign that's prefix arithmetic is pretty much similar like multiplicative you have like multiplication division and mod you have additive which is
plus and minus you have shifting operation, which is bits shifting to the left and bit shifting to the right you have relational operators less
than greater than less than equal to greater than equal to. Instance of Quality quality of two data types
or two variables which check whether they are equal and not equal. We have a bitwise
and bitwise xor bit wise inclusive or which is happening at the bit level. We have logical and logical or so.
Basically, this is for conditions, right? If you want to have like two conditions, like if int I is greater than 0
and int J is greater than zero sort of you add or you have logical anding between two conditions logical conditions
or illogical or pretty much similar to other programming languages. Like it's or between two conditions.
We say that either this or that we have a ternary operator, which says that if the condition matches we would have a condition followed
by a question mark followed by colon, and we would have some value Followed by colon followed by some other value.
So what we are trying to say here is if the condition is true assign value 1 if the condition is false then assign value to so
instead of having it written in if else you try to put it within turn your printer. So basically this is when you have simple assignment operator,
if you have a logic if you have some particular logic been written it would be a better practice to have
if else so that it's readable right because ternary operator it would be very difficult to actually
through we have assignment operator which is equal to which is plus equal to is nothing but adds the value on the right hand side
to the left hand side and assigns it to the left hand side table - equal to does the same thing.
It kind of subtracts value to the the left hand side from the value to the right hand side and assigns it to the left hand side.
So I believe this is pretty much similar to other programming. Languages there's nothing different in this we might have used it somewhere
or the else should be pretty much simpler for you guys. So let's see unary operator example when X is equal to 10,
so you could see X been assigned value 10, you have X plus plus as we said it assigns first and then increments it
so you could see value 10 here again. So now if you after this if you print the value of x, you should see the incremented value.
That's 11. So that's post-increment. So, let's see pre-decrement.
So you have a been assigned the value of 10. All right. Now, you do - -
A which is pre-decrement you would see the value 9 directly. And now if you print the value of a it would be nine again. So basically this is
like decrement and then assign this is like a sign and then operate this is like operate and then assign Let's see the negation operation like a has a value of true now,
we negate it and assign it to the variable D and you could see that it's changed to false.
Now. Let's see the arithmetic operator. You have B which has value of 20.
We have D which has value of 30. So when you add it gives you value of 50 when you multiply it gives you the value of 613 220
which is 600 when you divide it 30 by 20 you get value 1 and when you have mod,
which is like remainder, which gives you value 30 by 20, which is equal to 10 shift operators are
so it shifts to the left so shift left shift, right? So basically you have integer value of 20 and if you convert this into binary
if you convert the 22 binary which comes out five digits, right? So when you convert this binary and when you shift,
To the left you would get the value of 80. So you have value 20, which is nothing but if you convert this 20 into binary,
which would give you one zero one zero zero right. Now, what we're doing here is kind of Shifting it to the left by 2.
This is what you do and this is what it shows up as value of DT. So this is nothing but 64 plus 16. So that's what this left shift does.
Basically it shifting to the left by 2. Again, you could have shifting to the right by 2 by 3, which would give the value of to just shifting
to the right by 3 would be something like this. Right? So you're basically it shifts it and you could see that.
Yeah, basically it would end up to 2. So now we have like relational operators, which is pretty much simple like a less than b.
It gives false a greater than b equals equals P. Since you have a is equal to B. In this case.
We have a equal to B. That's why we have a less than b as false and a greater than b as false.
But whereas a equal to equal to B is equal to 2, we have arithmetic operators pretty much similar. Basically you have ad you have
multiplication you have division you have mod. So here we have a is equal to 20. So what we are trying to do is assignment so a is equal
to 20 now B is equal to 40 a plus equal to B, which is nothing but as I said,
your left hand side would be added up to the right hand side and would be assigned to the left hand side, which is nothing but 20 plus 40 would be assigned to a so
when you print a it would print 60 ternary operator, which is nothing but if a is less than b here we can see that if a is less than b then the value
of Should be assigned to tr or the value 200 in this case. The value 200 is been assigned is greater than b logical operators is nothing but anding
and o-ring of conditions. Yeah, here we are saying that a is less than B
and D is less than b then return false and it's like kind of ending logical conditions. Now, let's talk about the control statement in Java.
Now again, this one is pretty much similar to other programming languages nothing different nothing different in Java.
So you have control statements you have I treat if statements you have jump statements. So one is selection or decision making statements,
which is like if else if else ladder you have if if this particular condition satisfies do some things do some execute group of statements
and if it doesn't then execute other group of statements that is about if else Which is nothing but based on the value of a variable
like you to execute different step of statements like say for example, you have you write a switch
on Integer value integer variable X. Now when X is 1 you do something or if x is 0 you do something or you can have a default statement as well
when X is either 0 or 1 do something else that supports which I tradition is you repeat the same set of statements again,
and again till the condition till a particular condition is met that's a titration. So you have four Loops,
which is like after every iteration the value of variable or the iterating variables would be changed and would be checked against a condition and
if it meets it would come out or if it made it would execute it again, and if it doesn't mean then it would come out.
So yeah, this is pretty much similar to other. Other programming languages. So for for INT I is equal to Z 0 is less than 10.
I plus plus and you write something within it. So this Loop would go on for 10 times from 029.
It would break it would come out when eyes greater than 9 which is 10 when it goes to 10. It would come out
while is again pretty much similar to that. You have do while construct which is guaranteed to be executed once
because the condition is checked after the execution is done once so basically no matter what the condition is.
It has to be executed once for sure. That's when if that's the behavior you want to put into your program you would use
to Y is equal to once for sure jump statements you have like break which come out of for Loop Komodo for Loop
or any attractive statements you have continued which would again continue and return is again coming out. Of a method or are mostly
from the methods you written something so the control leaves the method now just to give you what if Wells is if if the condition is true,
then then execute the conditional code. If the condition is false come out of the execution or come out of the conditional code.
Skip the conditional code. That's what if else is so here you have int I is equal to 10 and int b equal to 20
if I is less than b so it's pretty much similar to the ternary operator that we had so you could see here
TR is equal to a plus b so basically the value if a is less than b which is in this case a is equal to 10 and B is equal to 20 in that case.
You would have PR as 30 since it is true. So basically it checks the condition and if the condition is true it executes the code with now
let's talk about If else if it if else construct is nothing but you have a is a set of statements to be executed
when the condition is in satisfy succeed. So in this case if a is less than b TR is equal to a plus b else TR is equal
to B minus a so in this case it was less than a is less than b so you got the value of 30 whereas if a is greater than b we have again changed it.
So if a is greater than b then TR is equal to a plus b else TR is equal to B minus a so in this case a is less than b so
which means CR should be B minus a which goes into the else condition statement and here you have 20 be as 20 and a as 10
which is 20 minus 10. So you should have PR as 10. So here it's pretty much same.
It does the same thing nested if-else now, we are talking about the switch statement. So you have switch expression and you would have case written
against it whatever case you want to execute. So basically you would have for Value 1. So yeah,
what we are trying to say here is we have a switch expression and we have a value for Value 1 as its value then execute this piece of code
and then break out and if it has value to then execute this piece of code and then break it doesn't match
with value 1 and value to then executes the default cases. So one thing to note here is it's mutually exclusive only one of it would be executed out of the cases.
Make sure you break out of all the the conditions so to the right hand side you could see integer CH has a value of 40 now.
We have a switch for 40 now. Is when the value of CH is 20 it would print case 20 executor
when its value is 30 then we would have case 30 executed when its value is 40 then we would have case 40 executed
and then break out and if it's neither 20 30 or 40 we would have a default case is executed with says
that 20 30 40 not executed. So in this case since it has a value of 40 you would see
that case 40 executed as output I'd frisson is nothing but it is basically I treating code if a condition is met the the code would be executed
until the condition is true. The code would be executed and once once it is false, it would come out of the loop.
So basically it's a looping statement. So after every Loop the condition would be checked and if the condition is true it would loop again.
And if not, then it would come out. There are three types of for Loop in Java simple for Loop similar.
See like for INT I is equal to 0 I is less than 10. I plus plus would be a simple for Loop for each
or an enhanced for Loop and labeled for Loop for each is basically I treating on array list suppose you have some collection
or suppose you have a re right? You don't have to manipulate the indexes since the typical scenario
with arraylist is I treating through all the elements within the list. So we have an enhanced
for Loop wherein we don't have to actually deal with indexes. Java has come up with a enhanced for Loop where and what each iteration it would assign the value
of each element within the array to a variable which could be used within the loop. So we don't have to deal with indexes
like we don't have to Loop through till the sizes met in the loop through in the size of an array is Matt,
basically it Done by Java itself. We have an example which would clarify it. So simple for Loop is
as I mentioned you have for INT I is equal to 10 is greater than 1 I minus minus so it would print from 10 to 2.
That's a simple for Loop. Now. What's the Syntax for it is for then open up round brackets.
Then you have initialization. Then you have a condition and you have increment or decrement whatever you want to do
or you could even add up like I plus equal to 2 even that is good enough. It's like for each even number not even I would say it's
like incrementing by 2. Now. This is what I was talking about.
So this is for each Loop or enhanced for loop. We're in a typical scenario with array. You kind of eye to it through each element
within the array and then do something within the loop, right? So this is what Java has made it easier for us.
So basically you don't have to deal with indexes here if we wouldn't have had for each Loop. What you would do is you would tell the size
of the array is Matthew would I trade through and read from each indexes right? But in this case
as you could see there's an array and for each Loop the value within the array would be assigned to this variable
and you could use this variable within makes it pretty much simpler for us. And as I said,
this is typical thing that we do with arrays now labeled for loop it's not recommended to use this often
but still we have this construct here. So basically it's like go to we can have a label of each for Loop.
It is useful we have nested for Loop so that we can break or continue a specific for Loop normally break
and continue keywords continues the innermost Loop innermost for the loop only to give Behind why I mentioned that this should be used often is
because it becomes very difficult to debug right? If you dry run it if you use this labels, it becomes very difficult to actually understand
what's happening within so so we should avoid it and all this could be done by writing a proper construct. Sorry using simple for loop as well.
So we should avoid it somehow so basically as per my experience is not a good practice to use them. So type of while loop we
have SIMPLE while loop we have do-while Loop. All right. So simple wire Loop is first the condition is checked
if it meets then the looping statements are executed whereas do-while Loop is kind of the statement is executed once for sure and then the condition is checked
if it is met then it is looped again. If not, then comes out. So this is a simple while loop
so you have conditioned as you could see here while a is greater than 1 you print the value of a and you increase
decremented Within Here it's a do while loop so no matter what you would have this to printer and it would be incremented.
So it's 2 to 9. So no matter what some value would be printed for sure when you use do I now we have jump statements,
which is breaking slyke coming out of a loop. So as we mentioned it's the innermost Loop that it comes out of so that's what Brave does
if a particular thing is met or you could write a condition for a particular Loop to be broken rather than continuing it.
So basically you could have like this would be more or less like you could have infinite Loop say for example running through and
if a particular condition is met you could break out of it a typical scenario where it would be used.
So here you could see that you have a loop from 10, which I traits from where I is equal to 10 and where I is greater than V i- -
which we are decrementing each Loop. Whereas you're saying that if I is equal to equal to 7 we should break out.
So here you can see though eyes greater than 5 you can see the loop has broken out after 8:00 since the value has reached seven.
It's not printing the other indexes because it broke out of the loop. So basically this is like
if a particular condition is met and you don't want the loop to go ahead you can break out of the loop continue is similar
when you write continue, it goes to start of the loop again, it starts the loop again
and it skips the messages or it skips the code that you have after continue. So in this case you could see
that we have I equal to in case when I is equal to equal to 5 We are continuing the loop which means the print statement
for I when I is equal to Phi wouldn't get executed since you're continuing it. So basically as you could see the News being printed here.
You can see the 01234 and there's no 5 here then we have 6789. So basically what we are saying here is
when I is equal to 5 don't execute the statement within the loop. Just continue.
Just keep it. Okay. So what a methods in Java So method is
if you would have written function or if you would have used any programming languages if you want to perform some operation
if you want to do something or if you want to do something on some particular data, you would write a method.
So basically in Java methods are defines the behavior of a class. So remember I told you a class is all
about State and behavior. So methods are nothing but it defines the behavior of a class. So what are methods or method has a group of statements?
Of course, as I said, since it defines the behavior of a class you need to have some operations done, right?
So you define the operations through group of statements. It is much more used to have reusability of a block of code that minimize redundancy.
So imagine if you didn't have methods then you have written the same piece of code again and again, Say for example,
you have a method which adds to number right? And if we write the piece of code to add two numbers directly into the main method
if you want to write it again, or if you want to again add two numbers, you will have to write the same piece of code again
in the main method. Whereas if you have a method what you would do is you would refactor
and take this add method or functionality which adds two numbers into a method and this method could be invoked from anywhere
within the application which is nothing but re usability right rather than writing the same piece of code.
Now, what you are doing is you are defining this add method into some other method which could be called anywhere within the code.
So that's about redundancy that minimizes redundancy and increases the reusability of a code a class can have multiple methods
as you could imagine. You could have multiple behaviors introduced into a class which means
that you would potentially have multiple methods within a class. A method returns a null or a value using the written statement.
So basically the intent of the method is to perform some operation, right? So what method would take is it would take parameters
and do some operations on these parameters and it may or may not return something back to the calling program.
So if you don't want written anything you have to return null or if you want to return some value you could have say for example,
you want to return string you would return a string of a method you could have void as well, which means that the method is not returning anything back
to the calling program. It is doing something within itself and just the control would be back to the calling program,
but it won't return anything out of the method that's about method. So let's talk about so what the syntax
of method So the first one that you see so this is a method which is public int at Eureka integer a GB
and it is just concatenating the two strings or two numbers that we have sent a cross. So basically let's an itemized it
so what is public so public is nothing but again access modifier so public means this particular method
could be accessed from anywhere within the application. So that's what public means similarly you could have private.
You could have default scope or you could have protected scope. So public is accessible anywhere within the program private is within the class default is within the package
and the protected is within the subclass and the package. Okay.
What is this int it is nothing but the return type so this particular method is going to take two. Arguments and do something and written integer,
right name of the method is you can see ID Rekha. That's the name of the method. Okay.
So what do you see within the parentheses after the method name is nothing but the arguments that this method takes so
what this particular method is doing is it is taking two arguments which it is acting upon right?
So it takes integer a and integer B, that's the parameter list. So whenever you want to invoke this particular method you have
to pass arguments in this order. Now, what do you see within this curly braces is nothing but the behavior or this is
how you introduce a behavior to the class or this is how you add functionality to a method. So what you are doing here is kind of adding
these two numbers. So what is the written statement? What does it do?
So written statement is nothing but you do something you take the arguments whenever controlled comes into a particular method.
It does something and you return it back to the calling program. So the written statement is a control flow statement
that terminates the execution of method and written control to its caller. So when return type of any method is void,
then the method does not return anything if it is void, you won't return anything out. But if it is written in string
or something you would return string or null if you land not to return anything, it could be null as well.
So here we can see a method by the name work which is returning void. So you can see that it is not taking
any arguments as such or parameters, but it is just printing out saying that I do recall welcomes you
in the second case you can see it is written integer. So it's taking int A and B. Okay, and then it is printing out
and it's returning integer as well. So let's write a program sample program to add two numbers and we would be writing all this logic within a method.
So let's start off by defining a class. All right. So we define a class say calm dot edu,
Rica Dot and a typical name for all arithmetic operation glass with all arithmetic operation would be calculator, right? So I would write it as calculator.
For example. Alright, so here you can see the naming convention again. There's a package which is calm down
at Eureka and you have a class has calculator and it follows camel casing so it starts with upper case followed by lower case.
So you can see the class is being created here. Okay. Now what we are trying to do is you are going to have a method
which takes to number. Okay. So say I Define let's keep it public.
All right. So this is access modifier. We want to keep it public.
So you want to access this particular method from anywhere within the application. So I'm keeping this public.
So typically addition would return results, right? You would add two numbers and return results of two numbers.
So that's your written type which is integer. So you could either write the return type as primitive or you could write it as a wrapper class.
So basically wrapper class is nothing but integer has its own everything in Java could has to be realized in terms of classes.
So integer has its own class int is a primitive data type and integer that you see here. Is a class corresponding int so let's put the return type
as integer and add is a method name. Okay. Now this takes two arguments integer arguments,
which is arg1 and let's put the second one as arg2. All right, so we start and ended by curly brace. Okay.
Now we have to write logic within here. So what you see here, it's throwing an error saying that you
are not returning integer out. There is a missing written statement because in the signature this
is known as method signature this particular thing that we have defined here is Method signature. So in the method signature you have mentioned
that this particular add method is intended to return integer out of the body, but still you haven't written integer.
So basically to get rid of this I could put written null which means it's doing nothing now you can see that Got rid of the error.
So let's do one thing just to make it more verbose. I will write integer result is equal to ARG 1 plus ARG 2. So this is a method body that you are defining here.
So you are adding a behavior, right? You're giving Behavior to the calculator class. So by defining add method this certain behavior
that you are introducing now, you could have a multiplier method as well which would take two numbers and would multiply it
which is again giving some sort of behavior to calculator. So that's the reason we say that methods add Behavior to the plus.
Okay. So here it is taken two arguments arg1 arg2 adding it and we will return the result from here.
All right. So this is a method that you have defined. But so far we are not invoking this method right?
We are not calling this method you want to call and check whether it's running as expected. So that's where we would have main method right?
When you type mean it should give you suggestions saying that are you trying to write main method? So if once you click on it it would this is done by
IDE by the IntelliJ similarly in Eclipse. You would get an option, you know, it would give you suggestions saying
that we want to introduce main method. All right, so we have a main method here. So again, it's public static void Main
and it's taking arguments here. All right. So remember I said static and non-static
Method you can see here add is non static method when it's a non static method you have to create instance of this class to invoke it.
So basically first thing that I do is I create instance of Later, so how do you create instance of calculator?
It's with new keyword. All right, you create new and it would create an instance of calculator.
Now I give a call to add method and suppose I want to add like 10 and 30. Okay.
So these are the arguments and it has to be in order in this case since both are integer.
It doesn't matter but had it been some other data type. You have to ensure that say for example, I write string here
what this would return is typically a result right addition of two numbers. Now, let's print it out system dot out dot println.
This is for printing result of addition is flits print out the result that we get here.
All right, and let's end it by semicolon. So basically you have a package defined here within the package you have calculator
and actually it's my bad. I should have defined it right here. So it gives my it gives the right package name here.
So earlier I had defined calculator with income dot Ed u-- Rica again. I defined a package computed Eureka which is not required.
So yeah, you can see here packages calm down said Eureka which is nothing but the namespace we have public class calculator.
So public is nothing but the access specifier for this class plus is the keyword used for defining class
and calculator is the name of your class. Also one thing to note is the name of the Java file should be same as the name of the class.
So that is something that IDE or IntelliJ would do it for you. You just have to create class and it would create class.
So basically if you go to the source folder you would have something like calculator dot Java right? Then you have public method which is again,
here's the method that we have defined public is the access modifier for this method integer
is the return type add is the name of the method. It's taken two arguments arg1 arg2 within the body you are adding two numbers and you are returning result
which is integer which aligns to the signature that you have put here. All right in the main method just to check
whether this add method is functioning properly. What you do is you create the instance of calculator. You add two numbers you pass two numbers to it then
and 30 and we are going to print the results and see if it returns as expected. So here basically it should print the result as 40
since adding 10 and 30. So remember while it builds it it would compile into a class file.
Okay, the dot Java file would be compiled if it's properly written if it's in tactically, correct, it would read dot class file.
All right, let's wait for it to run. Okay, so it's prompting me errors that we have an other methods.
So let me do one thing. Let me delete other classes that we defined yesterday. So I get go to the source folder Comdata.
Directa. I So I am just deleting this classes that we defend yesterday.
So that we don't spend time in actually correcting it, right? So put it into a backup folder. All right, so
Okay, so we have only this calculator class now I run this. So yeah, you can see here.
It has been tested result of addition is 40. So basically what it has done is adding these two numbers 10 and 30.
All right, so that's what we have here. So we are adding two numbers. So let's talk about the sequence of how all this is being carried
out by Java right when you run a particular program how things work out when you run a class.
The first thing that's been called is the main method. Okay jvm is nothing but Java virtual machine
which runs the program for you which is part of JRE Java runtime environment. When you run a particular class,
it would search for a main method and if it doesn't have a main method then it would give you an error saying
that a class can't be run. So it's pretty much similar to executable file, right? So if you're trying
to run a class it has to have a main method or you won't be able to run the class directly. So that's where the execution starts from.
That's the entry point for your program. Okay. So once it encounters Main
and everything is good your jvm would start executing the statement. That are there within your main method.
Okay. So in the edition case that we saw within the main method we invoked we created
the instance of calculator and we invoked add method right? So when we ran that particular class you could see that the addition was done.
So basically what's happening behind the scenes is, you know jvm would execute the sequence of statements that you have with a name.
So again coming back to the example add example So within the main you invoke the add method right when you invoke add method the control would go
to the add numbers method and it would again execute whatever functionality you have put within that particular method this case
it was addition of two numbers. So it would execute once the execution is done. It would return whatever is been written out.
So in this case, I was was addition of two numbers, so it would return addition of two numbers back to the calling.
Program so you're calling method was the main method wherein you called this add numbers from? Okay, so it would come back to the main method and that's
how it prints it out. So following the invocation of add numbers you could see that we have printed out the visual
that comes out of this add numbers and that's how it gets printed onto your console so that the sequence of execution that happens behind the scenes.
Now what are the ways in which you could call a method? There's call-by-value call by value is nothing but instead of passing the memory location,
it passes the value of a particular variable. Okay. So this is similar
to call-by-value and call-by-name reference that we have in see okay, instead of passing
the memory reference or instead of passing the reference. It's just a value that's been passed. So if you change something within the method or
if we change the value that's been passed within the method only the local copy would change
whereas the main copy would remain as is but basically call by value is just passing the value but not the actual reference.
All right, so to understand call-by-value you could so this would give you some idea.
So say for example, I have integer ARG 1 which is equal to 10 integer ARG 2 which is equal to 30.
Okay. Now I pass ARG 1 comma arg2. So what's happening here is it's passed by value.
So suppose you change. What I'm trying to say is here if you change arg1 to something else 300.
Okay. So what we are trying to do here is we are trying to pass this to arguments arg1 arg2 to add method
and within the add method we are changing this arg1 200. Basically we would check whether this hundred is been reflected in arg1 here.
Okay. Arg1 here would change 200 as well. Let's see what happens here.
Okay, so I print it. Okay before passing it by value. So I print arg1 here.
Okay, and after I give a call I would again printed printing arg1 after busting it a value to a method add Method All right,
so let's run this so basically since its passed by value arg1 would remain as 10. Okay, so that's what pass by value is all over.
So here you can see arg1 before passing was 10 and arg1 after passing was again 10. Alright, so the change
that you made here doesn't reflect here. So that's pass by value. All right.
So this one more concept of method overloading with in Java. You could have same methods with different number of parameters same method
when I say same method it has The same method name which would take variable number of parameters. Okay, the overloading happens at the compile time itself.
So during compile-time jvm understands to which method you are actually giving a call based on the actual parameters you pass on so just to give you
a fair idea about this I Define one more method. C ad which takes say integer arg1, let's keep this adders.
So I defend three parameters here. So instead of two I Define three parameters here. This is nothing but method overloading.
All right. So here I Define ARG 1 plus ARG 2 plus arg3. All right, so we sum it up here
and we return it back to the calling program. So one thing to note here is it's not giving you compile time error, right?
So you can see that it has accepted this add method as well. So you can see here.
The signature is pretty much same only thing is we have one more extra parameter, which is a RG3.
So it is treating this true methods as a different method. That's what method overloading is all about suppose I Define one more ARG pee I say 40.
Alright, so we have three parameters here. I am giving a call to add arg1 arg2. If I put arg1 arg2 the first add method would be called
if I have one more ARG 3 now the second add parameter or the second add method would be called. So let's define result from new add method, right?
So here we say. Okay. So as I said,
we have overloaded the add method overloading is nothing but same method name but different arguments or different number
of arguments or it could be different types as well. You could have only two arguments but one is integer and one is something else
if for example string that also works so basically different data types A number of arguments
but different data types or different number of arguments. Alright, so here we are adding three numbers and let's run this.
So it's going to add 10 30 and 40, which is nothing but a tea. So this is done at the compile time.
As I said this linking is done by GBM at the compile time. So here you can see results from new add methods 80 during runtime.
You can see that it invoked this particular method and not this one. Alright now, let's start off with arrays, right?
What is the concept of arrays? So again, this is similar to other programming languages arrays are nothing but grouping data
or grouping values of same data-type. Okay. So arrays are used to solve the problem
of storing multiple elements of the same data type. Okay, an array is group of like typed variables that are referred to by a common name.
So you define a name for the array and in the future you can use this name to access it. Okay specific element in an array is accessed by its index
as you could imagine since we are grouping you would have multiple elements, right you could add something to it
or you could delete something or you could add it to the end insert it in the between of the array
or just so something or just search by index rate you would give some specific index and get element from that particular index.
So we would be looking at the Which would give you a clear idea about this but basically array is nothing but group
of or its multiple elements of the same data type array type is fixed and cannot be changed.
So in Java when you define an array you either give the number of elements that you can store in the array,
which is nothing but the array size or you have to give the values that a particular array would contain right
when you define it. Okay, but all in all you have to make sure that when you define an array you give the size as well
which cannot be changed the feature the size of the array has to be mentioned during the Declaration itself. So here's an example of array this array has around
for which has four subjects with stores marks of four subjects for a student. Okay?
Here you could see indexes in the white box, which says 0123 it's always in sequence. It starts with 0 and it ends with n minus 1.
So basically this array is of size for which starts from index ranges from 0 to 3. All right the value that is stored is nothing
but the that's a marks in a particular subject which is like 87 60 70 and 80. That's the value
that when stored into this array. Okay, we can access any of this indexes suppose you want to access
at index 1 you could do it. Do you need to define a front you need to Define on the size of the array of front?
This is how it's been done. Okay. So this is how you declare array.
So we saw how we usually declare an integer and stuff like that in Java. But here we are declaring an array.
All right. So this is an integer array and you can see square brackets here.
Alright, this tells jvm that we are trying to Define array of type integer. Alright.
So again, we use the same keyword which is nothing but Nu new keyword is used to create a particular array.
All right, and here we are defining an array of size five. So this is one way of declaring an array.
The other one would be who initializer itself when you declare it you give what are the values that particular array is holding up the first case
you're not giving the values you are just defining an array which Those some some amount of memory right jvm would reserve some amount
of memory or in this case. It's four bytes right array. So for integer, it takes four bytes.
So it would Reserve 20 bytes for array of size five. All right. So in the second case as I said,
it's directly initializing the array it's putting these values directly into the memory location still it would be consuming 20 bytes,
but the second variant would have values directly initialized. So when you declare an array of size 5 the range
of indexes would be from 0 to 4. If you try to access index 5 you would get this exception exception is nothing
but a anomalies situation right which or something which is not expected as occurred in your program
and Java expresses such event by throwing out an exception. You can't access index fi. So different ways of declaring an array.
We saw that it's with new keyword. All right, so you have new int 10 which would declare array of size 10 again.
You could either have the square bracket at the end of the variable or you could have it in between the type
and the variable name. So behind the scenes it everything is the same. It doesn't do anything different for both
but these are different ways in which erase could be declared. All right, the third one is similar to the last one where in we have BR initializing it directly.
Alright, we are putting this value 12345 into this array. This one is again same you are so within the square brackets,
you can see that you are not defining the size of the array, but you have an initializer at the end by which you express
that you want to put in 1 2 3 4 5 into this array. So basically one and two That you see here does the same thing it declares the array of size.
The first case is 10 the second case is five though, but behind the scenes it's doing the same thing. It's nothing different when it comes
to actual preserving memory and stuff like that. It's doing the same stuff and three and four does the same stuff
only thing is syntactically it's varying but what I'm trying to say is GBM doesn't do anything different to actually executed.
So the length of the array is set when it is declared and when an array is declared array index gets initialized.
All right, so if you define an array and if you try to print the length of it you could see that it prints out in this case.
We have declared an array of size 20 and when you do x dot length, when you print it out you could see that it prints 20.
All right. So what are different types of arrays that we have again similar to what we have in Programming languages,
we have single dimensional array and multi-dimensional array single dimensional array is what we saw earlier like you define array
of integer of size 5 that is a single dimensional array. So in this case, you could see a array been initialized with value
2 4 6 8 and 10 that's a single dimensional array since we have just one dimension one row right? Say for example,
you want to store marks scored by a student or marks towed by a particular student just one student. That would be a single dimensional array.
All right. So say for example, the first one is the marks code in math.
The second one is the sign and so on and so forth. All right, so that single dimensional array. So now what's multi-dimensional array so taking the same example
of storing marks code by a student. So by a particular student it would be a single dimensional array,
but suppose you want to show your marks code by all the students in all the subjects. If you want to store it,
it would be multi-dimensional array. So one particular row that you see here would be a mosque secured by a student
by just one student in all the subjects. The first row would be by student won. The second row would be by student to the third row
would be by student 3 and so on right? So that's where you have this application of multi-dimensional array.
So how you access it is nothing but you want to access the first element the first index it would be a of 0 0
and this would be of 0 1 and so on so as I said if the First Column is for math if it's a marks secured in math this entire The First Column
that you see would be marks secured by all the students in math. That would be the First Column
whereas the first row is the Mach secured by student a in all the subjects. So basically This Could Be Imagined as
a table in a database, right you have a table where in you have rows and columns so columns are nothing
but you have Fields within a table and the rows that you have our different entries that you have within a table.
So This Could Be Imagined in the same way. So you could see how the index is you could see how the indexes are aligned so X increases
when you go down and Y increases as you go to the right the memory allocation of array, so for single dimensional array of type integer,
1 integer value takes four bytes, right? So now when you declare an array of size 5, you would have 20 bytes Reserve.
That's how memories been allocated right if you have a character array of size 5, it would be ten bites
since each character takes two bites in Java. So you would have 10 bytes reserved up front. All right, so that's
about memory location a location in single dimensional array and when it goes to multi-dimensional array this case you have like five elements,
right you have array of 55, which means that you have 25 elements stored within the array
right 25 integer element stored within the array and each integer value takes four bytes for storage. So it would be a hundred bytes.
All right, so if you have array of if you define a multi-dimensional array of 5 into 5,
you would have basically a hundred bytes allocated for it. Let's write some programs and understand more about how this arrays operate.
So we have an array of five elements. All right, as you could see here 2 4 6 8 and 10 write a program to access
element at a specific index. Okay, so you have an array of five elements 2 4 6 8 and 10 and we want to access it.
We want to access particular element. All right, so let's write so I create a class. All right, I Define this as our a demo,
so I don't give a package name here because I am defining a class within a package so you could see here the package came directly
because I right clicked here right and then created a new class. So yeah, you get this particular class here.
I Define a main method as a type main it would give me a suggestion. So this is the entry point again for this particular class
and suppose I Define the jar. Ray and suppose I Define 2 4 6 8 and 10, right so you could see here.
We have an array defined of size five. Okay, and you have initialized it as well. So what I was trying to say is you need to have length
of the array given up front right? If you don't do that, it would show you an error
so you can do something like array of integers is equal to Nu so you could see here array initializer expected. All right.
So either you will have to initialize it or give some value to it. So if you put here 10 you could see
that it compiled right? So either you will have to mention some indexes here or the length size
of the array or the ways to initialize this okay. Now you could see the compilation error as gone. So what I'm trying to say is it jvm has
to know about the size of the array of And if it doesn't know then it would flag you with an error. All right, so we have defined this array of let me keep it
as is okay. So now we have defined an array of size here five and we have initialized values as well.
Now, let's try to print out values, right? So just to keep it simple suppose. It ranges the index would range from 0 to 5,
in this case. So this value to is stored at index 0 value for is stored at index 1 value 6 is stored
at index 2 value 8 is stored at index 3 and then is to that index for all right. So let's try to print 0 by the way.
I want to copy it. Yeah, so I'm just sprinting 0 1 and 4 and let's see what it gives up.
Right so it should print 2 4 and 10, right? So you could see here to 4 and 10. Now.
The other thing that I wanted to show is if you try to access so let me show you what happens there.
All right, so if you try to access at index 5 which doesn't exist at all. No, it should give you a exception So
in Java exceptions could be nothing but God and you could do something with it. So here you could see
that it gives it gave you array index out of bounds exception since five doesn't exist at all. The index five doesn't exist at all for this array.
Okay. Now let's define multi-dimensional a write code to find the length of rho 0 right.
So let's define a multi-dimensional array. Alright, so we have a multi-dimensional array here. So how you define multi-dimensional array is
something like 1 2 3 4 Alright, so this is a multi-dimensional array that you have. Okay, so we have defined a multi-dimensional array
the size of this array would be 2 by 2. Right 2 by 2 is the size of this array since you have two elements here
or let me put it to remove the confusion. Let me put it this way. So right now we have like 2 by 3,
right so you have like two columns and 3 rows. All right. So now let's print actually it has to be 3 by 2 rather.
Okay. Now, let's print the size of this array 0 right. So how we do it is
again println now you have multi-dimensional array and you have 0 so 0 to 1 refers to this 0 1 and 2, okay.
Dot length, so as you could see here that individual row that you have within multi-dimensional array
itself is an array is a single dimensional array. So multi-dimensional array is nothing but it's a array of single dimensional array.
Okay. So let me print here size of first array. I miss Plus.
So as you could see here, there was an exception and since there was an exception you won't have this particular statement executed
since there was exception here. So basically to execute this particular statement, you should handle this particular exception,
but we are not talking about handing the exception yet. So I'll have to comment this to pass through. So now you could see
that it has printed out to which is your size of first array. So this is a program that we actually did right now create an array having
many characters write a program to copy elements from created array to another array and also write a program to delete an element from an array.
Okay, so I will just talk about this right here. So we have an array here of characters, right? So basically when you want to delete something from my array,
what you will have to do is you will have to shift the indexes, right? You can't delete something directly from an array.
So what you will have to do is if you want to remove something then you will have to shift the indexes
what happens is suppose you have an array of five integers and suppose you are deleting the second element right. Now.
What you would do is you would shift if you are deleting the second element within the array you would shift all the elements
that follows the second element to the left, right? So the third element would become the second element. What would become third
and the fifth one would become the fourth. So basically you shift it. That's how you delete something from an array.
All right. So other thing is about copying elements from the array, we have a utility class
which is nothing but system system is a class itself. So as you could have seen that when I'm printing out something I do system dot
out dot println, right? So this system it's a utility class in Java where an IT exposes lot
of functions within a lot of methods utility methods. So one of them being our a copy, so here you have to give source
and the starting index and the destination and what we are trying to do here is copy 5, so this is how we copy
from one array into another starting from index 0. So this is copying and the other one that you see here is about delete.
So as I said deleting is nothing but we have A logic written here to shift the indexes to the left.
So here we are trying to delete one at index 3 and we are just shifting elements after this index 3 to the left. That's how we delete something from an array.
Now. Let's see what our strings so string is nothing. But again the data type with in Java.
So why do we have strings at? All? Right.
We have array of characters then why do we have string at all? So here's an example where in you have lot of loads of data?
So you could imagine a role of a data analyst who has to analyze through lot of data coming in right? So nowadays, it's like petabytes
of information being processed throughout a day, right which would be very difficult to handle if you had to deal with character array.
So that's where that's where Java has come up with strings, which is nothing but a group of characters,
but you don't have to deal with it Java by Itself has a class which would take care of this strings and
since strings are widely used within a program you could imagine that name or anything that any identify
that we have is mostly we have to store it as the string. So one of the data types that widely used within industry or which is widely used
in programming is string, right. So Java has some kind of string management as well to make sure
that programs run or make effective use of strings. Okay. So here what's been mentioned is James is a data analyst
and he's finding is really difficult to actually deal with character array to store patients names every time so that's where we have string.
So string a solving the problem of actually dealing with character array. So Java is actually
having character array is touring characters or the characters in the string in a form of character array, but It doesn't expose it to the outside world.
You can just use this class to deal with it, but you don't have to deal with character arrays. So Java string is nothing but sequence of characters,
they are objects of type string class. Once the string object is created. It cannot be changed.
This is the immutability functionality of java. We're in once you write to it. You can change the value.
So this is specifically important when it comes to multi-threading when you have multiple threads accessing a particular string
or same string. So that's when immutability helps us to make sure that you know,
multi-threading is it's thread-safe we call it as thread safe because multiple threads can access the same string just
because it is immutable. All right. So what are different ways in which you declare a string?
So you have string Str is equal to new string which we are not initializing the value of the string here which could Any value and you have string str1 is equal
to Ed Eureka wherein you are initializing the value of the string right away. The other one is the character array
similar to the integer array that we discussed. We have character array as well. So immutability of the string.
So why do we have immutability one is for security. So string stores lot of useful information, like even the credentials and stuff like that,
which shouldn't be accessed by external users. So this immutability factor of string helps us to keep it secure
so that no one else can see it synchronization. So this is what I spoke about when you have multiple threads accessing the same string,
you don't have to synchronize it Java by itself through its immutability feature would ensure that multiple threads can access the same string
without hampering it or it would run as it's expected. Shing so caching is nothing but you have a string pool.
So basically if you have two strings with the same value, it won't store it. It won't store redundant copy of it.
There would be just one copy in the string pool and both this references would be pointing to that value. If you happen to change one of these references it would point
or it would create a new value in the swimming pool and point the other reference to it sort of so basically what we do is why caching is required is
as you could imagine in application, as I said string is widely used data type and you could have multiple references pointing
to the same value of a string. So we don't want to have duplicate values stored. That's where this caching comes into picture to utilize
memory efficiently. So what is shrinking pool as I said string pool is all the constants
that you define within the string within the application would be stored in the string pull string tool used in Java is a spool
of string stored in Java Heap memory. So we have heat memory. We're in the objects are created and that's
where string pool resides as well. String pool is Possible only because strings are immutable. You can't change once it is defined.
You can change it. If you change it, then you can change that particular memory location.
It creates one more entry in your string pool and the reference would point to the new entry. So the actual memory location is not getting changed.
That's what immutability is all about string pool helps in Saving lot of space for Java runtime. We are breaking on the redundancy Factor here.
So if you have multiple strings or if you have multiple strings holding up the same value, it won't create.
People copies of it there would be just one value in the string pool, which would be accessed by all the references.
So basically string pool as we discussed is or resides in Heap memory. So string is not a primitive data type
unlike character character CH AR is a primitive data type string is not a primitive data type
is the wrapper class to array of characters and this is specifically done so that Java has a mechanism to manage strings.
Well, which is widely used it arrived within any application or any programming language for that matter.
Della strings are stored in string to love Heap area which we already discussed so we can see a string pool. So we have stack memory and Heap memory.
So when you have a local variable when you define something locally, the reference is created in the stack.
All right, and it points to the actual object is created in the Heap, but the reference is created in the stand
so you could see as pointing to hello in string pool. Okay, so s is nothing but a reference reference to this ring.
Okay, so here we can see that we are concatenating. Right? So this would give you fair amount of idea.
What immutability is all about, right? So here we have string S1 is equal to happy. All right,
and what we are printing out the original string that is happy, right? So you could see here S one is a reference
that's been created in the stack. You have a string pool within your HEAP memory which would store all the strings all the constants
or literals that you have defined here. So you could see happy been stored in the string pool and S1 pointing to it.
Now you are trying to concatenate S1 with learning, right? So it's happy learning.
So you could see that the memory location that s one is pointing to is not changed right still pointing to happy.
But this one more constant that's been created in the string pool that second category. The nation of happy
and learning so you could see happy learning also created but what's important to note here is s 1 is still pointing to happy.
It's not pointing to happy learning. All right, so if you want S1 to actually point to happy learning this is
how you do so you have S 1 which has the value happy. All right. So you do S1 dot concat learning and you assign it to S 1.
All right. So you are assigning to the same reference as S1 and you are printing out s 1 in this case,
what would happen is unlike the previous example that we saw there in S1 is still pointing to happy you would see that as one is pointing to happy learning.
So here you could see that this one is pointing to happy learning. So basically you
move the reference from happy to have Peter. All right. So, let's see what our strings how do we operate on strings?
Right? So let me create a new class here. Just ring demo.
I create a main main method again. All right. Now what we do is string s is equal to and Eureka.
All right. So let's first print the length of the string. All right.
So this is how this utility class or this is how this wrapper class helps us. So it has all these utility methods right you just have
to do else dot length. It would give you length of the string so had we not had strings and
if you want to deal with the character arrays, you would have to print the size of the array here. Alright, so when we run it,
it should print it it is the size of the string at Eureka. Let's have substring.
So it's a beginning index. So suppose we give to so it would give you substring from index 2.
So basically we are getting substring. We are getting part of the string substring is nothing but part of a string right
from from a particular index. So since we have given to hear it starts from index to so you have 0
he's at 0 and x 0 D is at index 1 and U is at index 2 so it starts from you till the end of the string you have compared to you.
Could Define two strings and you could actually compare them and see the value so basically So
you could have one more string defined here something of this sort. Alright, so here we compare it.
So we are doing s 1 dot compared to To him, it's true. So we are comparing S1 with S2. So it compares the given string with current string.
So here you can see it as shown minus 1 right since B is one ahead of a so if you have same string it would show you zero.
So if it's exactly the same it would give you zero. You have he's empty suppose. You don't have anything within your spring.
You could check it whether it's empty. He's empty returns a Boolean variable which means if it's true it is empty and if it is false,
it has some value then we have to lower case we have to upper case in this case suppose you want to have could be scenario
in which you want to change or you want to change the casing. So I'll just take one of that I make it to uppercase.
Okay, so s 1 is nothing but we have Ed Eureka stored within and we are so when I run this
you should have said Eureka printed in uppercase. So here we have written camel casing and let's see the output. So here you can see the string was converted
or translated into upper case. So similarly you could do lowercase which would print string in lowercase.
Now we have value of is nothing but the value of a string value of some of the data type so you could pass integer and you could do value
of and it would give you a convert integer into string, right so value of is a method within string which takes different data type and converts it into a string.
So just to give you an example you could have like teacher I is equal to a hundred String dot value
of and you give I it converting this I into string. So it would print the same value hundred but it's converting it into spring
you could replace something within the string. If you want to replace particular character within the string
which could be done with replace method. So replace method takes two parameters replaces a method within string
which takes two parameters. First one is the character that you want to change and the second one is
the new character that you want to change replace to. Okay so here to show S1 dot replace I could replace e Within a be alright,
so we are making it lowercase and as you can see here, it's printed in uppercase E was changed to lowercase e so that's about replace.
We have contains which again gives you Boolean type result, which says whether a particular value
of particular character is present in a string. All right. So in this case we check
whether we have a stew with a TLD. Oh and you replace t with L, which becomes hello,
and now we are checking with the replaced string contains D, which it doesn't contain it, right so it would give you false.
So that's about contains equals is basically checks for the equality of string. It takes one argument
and it Compares it against the string object against which you invoke the equals against. All right, so to give you an example,
so you have S 1 which is at Eureka Eureka now to print it. So here we do S1 Dot. printing equality of string So
since it's the same it would give you true if had it been different it would give you false.
So you can see here. It's printing both the strings are the same you have different methods,
like compare ignore case where in this case we compared with case, right? If you would have had Eureka in lowercase, right?
If it would have start with lowercase e you wouldn't have got 0 here. All right, whereas this one more method which is s 1 dot
compared to ignore case when it doesn't consider the case, right? So even if there is a case change
if this how one in uppercase and one in lowercase still it would match all still it would give the value as 0
which means both the strings are the same we could get some character out from a string. Okay.
There is a method character at there's ends with which again returns a Boolean value saying that That a string ends with a particular character.
Alright, so here what we are doing is we are checking whether the string P
which holds the value happy learning is ending with you, which doesn't right. That's the reason it gave you false.
Had it been G here it would have given it true. Now that's about strings. Now.
Let's talk about different variant of strings. And why do we need it? So there are like three variants
of string one is the string class itself. The other one is stringbuffer and you have a string Builder. So we'll talk about why do we need this variance
at the first place? So string buffer is nothing but good for multi-threading when you have multiple threads.
Usually it's good to have string buffer because all the reads and writes that you do on the string. It is synchronized.
So when I say synchronized there's only one thread that could access a particular method within a class at any given point
so you can't have multiple threads going in and changing the value or doing something. So basically if you have a string within your application,
which is been accessed by multiple threads it's better to go with stream buffer or if there is lot of not accessed as such access.
One factor, but of course if there are a lot of modifications done and stuff like that like with stream
before you could append a pain to a particular string which cannot be done in string right in stream buffer.
You could actually do lot of things it cannot be done and string and just to make it thread safe. They have made all the methods that modify the string contents
as synchronized so that only one thread can access it at any given point. So see you can see insert here right in string.
You don't have all these methods you don't have methods to manipulate the strings in string, right? Whereas in stringbuffer you have methods
to manipulate the strings. So when I say strings those are literal strings, right not the string class.
All right. So here we have string buffer and we are trying to append something to the string buffer.
So we define a class we Define stringbuffer. So you could have like S1 dot append and when you see a pendant you could see
that it appends any data type. This is the important factor in stringbuffer you have lot of utility methods
within or you have lot of methods to manipulate the string which you don't have within string class. All right.
So this is mutable, right you are changing the values suppose. I append three exclamation marks do it, right.
So what we are doing here is we are just printing out the new string. All right.
So basically you could you should see at Eureka and the three exclamation marks. As you could see here it got upended.
So basically it's a mutable string change the value with them insert is nothing but inserts a new character at the given position.
So here we are saying that inside w at position zero. You could replace it replace particular or replace substring with a new string.
So here what we are saying is replace index starting from 0 till to with this new character sequence. You could delete something from it delete sequence of it.
So here we are saying from index 0 delete one character. So if you have two then it would delete two characters. So basically when you
say two characters even this e would have been gone. Okay, in this case, we are just deleting one character.
So basically this delete method takes the starting index and the number of characters following the starting index. Zebras you have like you could reverse the entire string.
Okay, so just to show you again new string dot if I reverse it. So basically this is a kind of utility methods
which we often use right so you can see it's been reversed here. All right, you can see the capacity
of the string capacity of the string buffer is nothing but I think it reserves 16 characters initially when you declare it
and it keeps on incrementing it so it has a growth factor defined within which you did not take care of at this point,
which you did not think about it at this point because those are internals to Java but initially when you declare a string string buffer storage space
for 16 characters, which is 32 bytes. If you have stringbuffer by do we have string Builder, right?
So string buffer as I said has some drawbacks. What are the drawbacks is more about string buffer is synchronized when I say synchronized which means
that only one thread can enter it only one thread can process it. So basically if you have a multi-threaded application,
you should go with synchronization because synchronization has some overhead, right? It has its own trade offs.
So when you when you synchronized what happens is when you move out of the synchronized methods
DVM internally has to do some operations which takes some time. So basically it's good to avoid synchronization
and which would make the application much more faster and that's where stringbuilder comes into picture
if it's a single-threaded application wherein you know that you don't have multiple threads which are going to access a particular string
in that case. You should go with string Builder as against ring. First because stringbuffer would give you a slightly
lower in performance compared to stringbuilder. Most of the things that string buffer does is done by stringbuilder as well.
As far as the functionality is concerned but it's not thread safe stringbuilder is faster, but it's not thread safe
which means that you cannot have multiple threats accessing it. All right, so to keep it simple, if you have multiple threads accessing or string buffer,
then you should go with stringbuffer. But if you have a single-threaded application, you should go with string Builder which would make
the application much more faster than stringbuffer again with stringbuilder. The default capacity is 60 when you initialize it stores
as you could see it allocated 16 space for 16 characters initially. So as far as the demo is concerned,
it's pretty much similar to stringbuffer. As far as the outcome is concerned. It's pretty much similar to stream buffer is no difference
as such but as far as the performance is concerned stringbuilder is faster than string buffer because it's not synchronized.
Okay, so you define a string Builder with happy and you append learning to it? Okay, and if you try to print s B1
which is appended with learning you will see happy learning would see it been upended. Okay similar to stringbuffer
when you delete character from index position zero and one character from that position you would see
that the H which is the first character has been deleted out so you could see that at index 1 you could insert welcome.
You can see the entire string that is welcome being inserted between okay, then you have reverse Could reverse the entire string
pretty much similar to string buffer. So again, this one is very similar. You have you are appending you're deleting then you
are inserting here you have reversing here. This is pretty much similar to what we did for stringbuffer as far as the syntax
and semantics is concerned and the outcome is the same as well. Just a performance change in terms of speed right?
So, when do you stringbuffer and stringbuilder as I said if you have multiple threads you should go with string buffer to make it thread safe,
but it would be slower as compared to stringbuilder. Okay stringbuilder is specifically good enough and you have single-threaded application
and it would be faster since it's not synchronized. So to make it thread-safe you have synchronization
which adds over it to the performance which takes tool on the performance. That's why stringbuffer is slower why to use
object-oriented Concepts? So let's talk about classes and objects. So classes are nothing but it has got a street
and behavior right as you could see you have a class and you have different objects, right?
There's a phone which is a class and which has got different types of phones right rotary phone. Then you have a touch-tone phone and you have a cellular phone
this are objects basically, right? So you define phone which is pretty much Jen Rick and which has got State and behavior class is something
that is generate that has got State and behavior but objects is something that is an instance of a class
which would have specific State and behavior. So phone by itself phone is a Class by itself. Which may or may not have specific behavior,
but you could see that the specific phones will have its own behavior to could Define its own behavior.
So all these three things that you see here three types of phones and nothing but objects objects of one class state is something
that is defined by instance variables, right? It's a class level variable right at the class level you define something
that gives State and behavior is nothing but something that is defined by methods like calculator was a class where in we had add method and add method
basically gave some Behavior to the calculator class, right? So what are attributes so you have a class and you have attributes attributes
and nothing but a property of object and in Java, this is defined by your instance variables basically class will have properties which is nothing but the state
which would be defined by instance variables and you could imagine every instance will have its own set of properties.
It won't be the same right in terms of the phone that we saw earlier. There were three different types of phones you could imagine
that it would have different properties right? It's not the same. So what are the naming conventions in generally used
at the industry level for Java. So this is not something that is actually taken care by compiler.
So even if you follow this naming conventions still the compiler would pass through but these are best practices
or this is good to have thing in the industry conventions is more about to keep On the same page and make it easier for other developers to understand more right
or keep things simpler. So that's why we have conventions. If you wouldn't have conventions then people would prefer
to write in their own way which would make us difficult to understand. Alright.
So for class name it start with uppercase as we saw in all the examples you would see that I have always used started with the uppercase
or it's rather camel casing. So in this case you could see stringbuffer demo, right? So it starts with upper case you have B,
which is again upper case you have B, which is again uppercase. This is nothing but camel casing it starts with upper case.
Basically it follows camel casing interface name is again similar to class. It starts with upper case you have method name
which would be lower case always which should start with lower case, but you could have it follows
camel casing but it has to start with lowercase. So maybe it's a predefined method with in Java. Right?
So you could see main starting with lowercase or I created a dry Tad is again put C uppercase.
If you would have say for example at numbers you would have something of this sort adverse. If you want to append numbers to it,
it should start with uppercase. So basically it's camel casing but starting with lower case where as class name.
It's a camel casing which starts with upper case variable name is again camel casing we should start with lowercase
and constant is always like it has to be everything in uppercase. So basically if I Define a constant final string,
I would write something like this. okay, so and if you want to add more words to it, it should be like this underscore
So it should be separated out band score. All right. So these are the conventions
that followed in Industry by Java community. So mostly even if you go to the source code if you want to see something dig into the source code
of java you would see same naming convention being followed types of variables. We spoke about the types of variables again to brief you
through we have local variables local variables are nothing but the variables that are defined in a method. So if you define something within a method
that's local variable so ARG 100 or suppose I Define and T local where is equal to a hundred.
So this is a local variable right? If you remember this is within the scope of a method once the control goes
out of a method your variable is no longer accessible and also it gives space for the jvm for garbage collector to kick in and clean up this particular memory space.
Variables are nothing but it's defined at the instance level so here for that matter. This constant is also instance variable.
All right, so I can Define integer variable starts with lower case and it follows camel casing. So this integer is nothing but an instance variable.
It's at the class level right when your class is loaded when you refer to this particular class, you will have your instance variable initialized by Java.
So instance variables are declared in a class, but outside a method Constructor or any block. Okay class variables are nothing but static variables
and it is one copy per class. So as against instance variable, which is one per object, whereas static variable or class level variable
are it's just one per class. So if you define static, this is just one per class and this could be accessed
directly using calculator calculator dot constant example, you would be able to access it but for instance variable, you need to have an object created.
This is non-static basically non static instance variable. So one of the reasons why you need to access instance variable is
because different instances might have different values for a particular instance variable, right? I could Define calculator one right?
Let me put it as calculator and we have like normal calculator. You could have one more class which is
like scientific calculator. It's one more object. Now a normal calculator can have its instance value
as 10 and scientific calculator can have its instance value as a hundred you have different values,
but whereas in static since it's one per class, it's not at the object level. So you have mod is equal to new demo,
which is an instance of a class demo. And you print message right d dot print message and it should print this message.
So basically there's a class demo which has got a method print message and we are trying to invoke that message
from the main method. Okay, let's talk about the constrictor constrictor is nothing but when you invoke new keyword new calculator,
there is a Constructor implicit Constructor for each class, which is without any parameters. So we'll talk about that.
But when you do new calculator, basically there's a method which could be used by the programmers to initialize
variables if they want to initialize something. So for each instance, if you want the same value for instance variable,
you could initialize it with in Constructor. So basically Constructors are for initialization or if you want to do some kind
of pre-processing break on invocation if you want to initialize something or do some kind of pre-processing
that could be done through Constructor Constructor is used in creation of an object. It is a block of the code used to initialize an object.
So when I say initialize an object, it's nothing but initialize some property or variable within your class Constructor
must have the same name as the class. It is in and it does not have any return type. So unlike method,
which has got a return type Constructor won't have a return type at all because what we are trying to do
through Constructor is just to initialize objects within but you can't return something out of it. So basically you Constructor is a special method
which you don't have control over you are not doing anything within it you're not trying to actually return something out of it,
right? You don't have control over its invocation. It's done by jvm itself jvm calls this special method
when you invoke knew or when you create an instance of it by using this new keyword Constructors are of two types default Constructor
and parameterised Constructor. So when I say default Constructor and parameterised Constructor,
this is nothing but so I am defining a Constructor now calculator as I said, it has to have the same name as the class.
Okay. So this is a Constructor. And as I said,
this could be used for initialization of variables. Say I initialize this 200. So for all the objects you would see this
being initialized to 200. That's the initialization. Okay, so I have created two instance of it to instance
of calculator class that is normal calculator and scientific calculator. Let me do normal calculator dot instance variable.
Okay. So here we are printing the value of the instance variable. Let me copy the same thing again,
but in this case. the second case I would be As you could see here. We have a Constructor.
I defined a Constructor here Constructor for a class which is initializing the instance variable to Value 200.
So as in when you call this new calculator a new instance of calculator would be created. The first thing that would be called.
Is this Constructor and which would initialize it. So basically what we are trying to do here is we are right after the construction of this particular object.
We are going to print the values of instance variable to see if it assigned 202 it. So here you can see the value 200 being printed out.
So basically if I wouldn't have Constructor then the value wouldn't have been 200. So let me comment out this one.
This is how we comment in Java. By the way. This is a block comment which starts with Slash
and asterisk and which ends with asterisk and smash. So I commented out that one I comment out the Constructor and you would see the value being initialized to 0
so you could see value been initialized to 0. All right, so that's what Constructor is all about. Now.
This is a default Constructor. You could have parameterised Constructor, which is like integer you provide some value right
and you assign this value to variable. So instead of hard-coding this value of 200 for the instance variable you could pass on some value
during the construction of the object. So basically what we are doing here is I pass 30 here and bypass 40 here.
Alright, so I am passing 30 and I'm passing 42 the Constructor. And as you could see here,
we are initializing the instance variable to that value. So basically for normal calculator,
it should be 30 and for scientific. It should be 40. Yeah, as you could see here you have 30 and 40
min printed out and this is the parameterised Constructor. That's what this default and parameterised Constructor and that's what it is all about.
So default Constructor is you don't even have to specify any Constructor. So I commented this calculator constructed still implicitly.
There is the Constructor put in by jvm, which is the default Constructor. So it's not mandatory to have Constructor each time
unless you want to initialize something. So what's the difference between Constructor and Method constructive must not have a return type?
Whereas method may or may not have a return type here you could see that unlike the methods add integer, which return something.
This doesn't say anything. This doesn't return anything because we are not doing anything within it.
You're just initializing it initializing some instance variables right Constructor name must be same as the class
so that This is a contract this has to be followed Constructor has to have same name as the class, since you are not invoking the Constructor by yourself.
It's done by the jvm. You don't have control over invocation of Constructor and you have to follow the naming pattern that Java
recommends to or Java forces us to you have to make sure that instructor is given the same name as the class name. Whereas method can have any name
as we saw the add method you're free to use whatever method name that you want to Constructor is used to initialize the state of an object.
We saw the instance variable being initialized within the Constructor method is basically it gives some Behavior to a class right
we saw add method which is giving some Behavior to the calculator method, which is nothing but adding up two numbers.
Similarly. You could have multiplied you could have divided and stuff like that
which adds more Behavior to it Constructor is invoked. Implicitly so you don't have control over invocation of Constructor,
which is done by Java itself. When you try to instantiate a class or when you use the new keyword,
it's implicitly invoked by Java by jvm. Whereas method you have to invoke it manually. So basically when you
do this Constructor is called by itself. Whereas when you want to call add numbers, you have to invoke it explicitly.
How does Constructor work the moment object of a class is created The Constructor of the class is called which initializes the class attributes, right?
So we saw about this. So when you use the new keyword jvm by itself would give a call to the Constructor
which could be used for initializing initializing instance variables within your class. So type of Constructors
this default Constructor and this parametrize Constructor, which we already spoke about the Constructor, which is created by the compiler
without any parameters is the default Constructor and the Constructor with specific number of parameters is called parameterized Constructor.
So here we spoke about this already. So we have integer value, right? This is a parameterised Constructor,
since you are passing parameters to the Constructor, whereas if you don't Define anything Java by itself, so in this case you could see that we didn't have
a Constructor for this class straight. I read them all we don't have Constructor for this class. So Java by itself puts her default Constructor
for array demo, which is known as default Constructor. So it's not mandatory
that you need to have Constructor every time since Java takes care of it. That's the differentiation between default Constructor
and parametrization. We use parameterised Constructor for passing values to the Constructor
and initializing something based on the value that's been passed out past in brother. So the default Constructor is used to provide
the default values to the object like 0 null depending on the type right when I didn't have any Constructor at all
for a moment. I am going to comment this. So this is a line comment when you put two slashes.
It's a line comment. Okay, it's commenting just this particular line This is a block comment
and this line comment block comment is slash asterisk and ends with asterisk. / whereas line comment is two slashes forward slashes.
Okay. So basically a comment or disc instructors as well. Alright now we have this calculator class now lets me.
Let me print the value of the instance variable. value of instance variable initialized by default Constructor.
All right, so we don't have a Constructor at all. So that's what I am trying to say here. I commented out this Constructors, right?
So we don't have a Constructor at all. And when you create a new calculator jvm by itself would have a default Constructor
which is used by jvm to initialize variables within so you would see this instance variable has zero value as 0 because that's
what the default Constructor does. It assigns some value to it has like specific values for integer it 0
if it's a class it would be null so you could see the value being printed as 0 if it's a class it would assign value null.
So in this case you have string right and you could see 0 null depending on the type of instance variable that we are dealing.
We saw how we pass parameters and initialized it. Constructor overloading is just like method overloading without written type Constructor overloading
in Java is a method of having more than one Constructor with different parameter lists. Like we defined add we had
to add methods and now I have renamed this to add numbers, but basically this was add method right so you could have multiple methods with the same name
but different parameter lists, which is known as overloading similarly for Constructor. You could have the same Paradigm.
So basically what we are trying to do here is we have a Constructor with 1 parameter that sing teacher I'll Define one more Constructor here
with two parameters. So we Define one more parameter here and I say for example, I put something like this.
So we are adding up these two parameters and putting it into instance variable. Okay, this is Constructor overloading.
So here you have single parameter been passed here. You have multiple parameters being passed So based on whatever you pass here suppose I say 10 and 20.
Or say I say? So on construction you should C-130 as the value of the instance variable.
Okay, as you could see here, you have the instance variable initialized to 130, which is nothing but edition of hundred and ten and twenty
and you could have something like this just hundred and ten, which is also K because you have a single distinct defined
as well Constructor taking a single value as well. And in this case, it would be just a hundred and so Constructors are nothing
but methods which helps in construction of object. All right, so you get a hundred and ten here? So Constructor overloading is in Java is a technique of having
more than one Constructor with different parameter lists. So we had a demo about it as I showed there were multiple Constructors
one with one parameter and the other one with integer parameters and during runtime based on whatever you
pass a particular instructor would be called in this case. You could see that in the first case
which is shop s 1 you could see the first Constructor been called which has two integers as being passed. Whereas when you create
object S 2 which has two integer parameters and one string you could see that second Constructor is being called.
What's Constructor chaining so Constructor chaining is the process of calling one Constructor from another Constructor with respect
to the current object? The real purpose of Constructor chaining is to pass parameters to a bunch of different Constructor,
but the initialization should be done at a single place Constructor chaining can be done in two ways within the same class from the base class
and Constructor chaining occurs through inheritance. So basically for Constructor chaining, there are two key words that we have.
So one is this this itself is a keyword in Java this pH is this is a keyword and the super keyword.
Let's see how we how we do it for this particular Constructor. I have this Constructor which has this value, right? So instead of doing this directly,
what I could do is so here. I was initializing this value directly to the instance variable.
What I could do through Constructor chaining as I could do this and I could pass the value comma zero.
So basically you're chaining here, right? You could see that what I am trying to do here is I am trying
to invoke this Constructor from this Constructor. That's what the chain is all about. So when you say this it tries to find Constructor
within your class which takes two parameters to integer parameters and this is the one it finds out, right?
It has two parameters. And basically what we are doing is we are keeping the second parameter is 0
which means it would initialize the instance variable to whatever value you have here, right?
This is what Constructor chaining is all about. It's more about calling Constructor of the same class within the Structure of the same class.
So basically from this Constructor you are giving a call to this Constructor that is constructive change. Super should be the first
it gives a call to the super Constructor. So you have a subclass and superclass, right? What do you do here is you give a call to superclass?
For Constructor chaining. I think they should be good enough where and you use this keyword to call Constructors
within the same class. You basically chain them together, right? We have demonstration on Constructor chaining here.
When you create student you could see there's a Constructor without any parameter and we could see this Meghan been called.
So basically what we are trying to do here is the default name would be Meghan, right? So and again we have one more Constructor,
which is overloaded Constructor, which takes name and in that case it would give a call with the specified name
and Mark as 70. So basically 70 is a default marks that we are signing and you can is the default name here.
If you don't provide name, it would be Meghan. All right. So this is what Constructor chaining is all about.
Static keyword static keyword again, we have spoken about in couple of instances, but I'll just walk you through this slide again.
The static keyword is used for memory management static is non access modifier used in Java accessible applicable for blocks methods class variables static keyword
is used to refer the common properties of an object. So as we spoke it's at the class level, it's not at the object level.
We have it common across all the objects right when the static keyword is used to declare any parameter. Then memory is allocated only once for that parameter.
So in this example, we could see that since it is one per class. So you would have this constant example
Define just once right it would be allocated. Just once whereas the non-static ones like this in. This variable you would have memory allocated
for each and every instance. So basically for this instance, you would have memory allocated for this instance variable
for again this normal calculator that we had earlier you would again have a memory allocated for instance variable.
All right, so it's one per object whereas the static ones are one per class. So yeah memory is allocated only once for that parameter.
All right. So here we can see that this we have a static string company
which has some company named put in there and we are just displaying it right we can see
that we are displaying ID salary and Company and you could see that for both the instances for even and E2 you have two employees here
with ID 25 and salary 25,000 and we have employee to with salary 30. Sorry with ID 30 and salary 3000 right?
So we are defining two instances of employees and you can see here. We are not passing the company name as a parameter
to the Constructor right still when you display it look at the output at the right side. You can see
that it has displayed twenty five twenty five thousand and SRT Traders the company name came by itself because it's one per class.
So what we are assuming is this class is basically designed just for one company, right that is SRT creators.
So all the instances of the class are all the employees that you create here.
All the instances of employees that you create here will have the same company name that is defined here
since it is one per class. Whereas their ID and salary would be variable which would differ as
per the value that you passed to the Constructor. here we can see that company is a static variable little allocates memory only once So
here it could be seen that you as I mentioned earlier you have reference variables being created out in the stack.
And in the Heap memory, you could see values being stored. So ID is tri-five salaries 25,000 for E2 ID is 30
and salaries 3000 and you can see the static variable. So basically static variable is stored in a different space which is permanent generation memory.
That's where the static variables are stored. So method could have static as well. When a method is static you did not create instance
of a method to call that method instance of a class to call that method pretty much similar to static variables, right?
So basically I can have one more static method here. So say for example I have private static all right, so So what I was trying to say here is you
could have calculator. . And you could directly call the display method. So you do not have instance
of a class created its at the class level. This is at the class level rather write one per class. Let's talk about this keyword
that this keyword is used as a reference variables to the current object. So basically what what it is trying to say here is so
if I want to print something here, let me print the instance variable, right? So within the add method
what I am trying to do is I am printing the value of instance variable Within add numbers, right? So I'm trying to print the value
of the instance variable within add numbers which would be basically this is where we are calling at numbers.
So basically it should be a hundred and ten, right? When we have such scenarios where and we refer to an instance variable
within a method we could see here printing the value of instance variable within add numbers gives you a hundred and ten right the implicitly
what happens is it puts this dot here this dot instance variable. All right.
So what we are trying to say is the instance on which the add numbers was called on the same instance, whatever the value of instance variable is just print
that thing out. So this is implicitly put in here you need not use this keyword.
This keyword is usually used when you have something like this right when you have instance variable suppose.
You have something of this sort. Right? One of your parameters is same as
as the instance variable, right? So you want to differentiate which is the instance variable and which is this variable right?
So that's when you use this When you say this dot instance variable, this is referring to this instance variable
and this instance variable to the right hand side is referring to this one. Alright, so that's
when you have to explicitly put this or in other cases. It's done by the jvm itself. You did not take care of it.
All right, so So, this can be passed as an argument in the Constructor call.
This can be used to invoke current class method. This can be passed as an argument the method call and this can be used to invoke current class Constructor.
This could be past basically if there is one more. so say for example, you have something of this sort which is pretty big though,
but Admitted taking calculator as its parameter. Okay, so I'm just trying to demo you could actually take calculator as an argument for any of the methods within.
All right. So you have ADD method which takes calculator as its parameter.
Alright, so here say for example within this method don't think about the logic it's not logical though. I'm just trying to show you it could be done.
Right? So I have ad and I put this within your method you could have you could pass as an argument you could pass
this as an argument. So basically you are invoking this one. So now if I print it
you can see in add numbers you have this method being called. So yeah here you can see printing out the add method taking calculator has its parameter.
So basically it's calling this on by passing this. So here we spoke about the Constructor earlier. We saw how this could be used in The Constructor and one
of the use cases as I said here you could see that similar to the example that I spoke regarding the instance variable.
You could see the parameter the name of the parameter is rule number which is same as the name of the instance variable.
This one is pretty much similar to that. You could see that the name of the parameter that is passed to the Constructor is same
as the instance variable name the rule number two. The left hand side is nothing but instance variable within the class info whereas to the right hand side.
It's a rule number that is passed as an as an argument to the Constructor similarly for the name you have this dot name,
which is referring to the name the instance variable in the class info and to the right hand side. It's a parameter that's passed to the Constructor.
That's a typical use of this. So we are creating a message here and you can see that we are giving a call within the Constructor.
This is within the Constructor actually, so we saw this in the previous example. Basically, this is
what it does write this value of 0 that is what this is doing within the Constructor you have this and you are giving a call.
So in this case this Annie would give a call to message string n and would print it out. So how does it work the main function is executed.
So again, this is a sequence in which the things execute behind the scenes. The main function is executed first,
when an object of the class is created a default Constructor is called okay, in this case, the one without parameter is called
right then you have this dot any which is nothing but you're giving a call to the Constructor within the same class,
but with the parameter any so the jvm would check whether you have a Constructor with parameters as string, so it finds it and it would give a call to that Constructor,
which is nothing but the Constructor that you see here in a Blue Block right, then it would similar to any method
after the method invocation is done. It comes back to the calling method, right? So after this and is called it comes back here then it prints.
Welcome to edu Rica. And once this is done, it would go back to the main method in general.
What happens is whenever a method is invoked the local variables and everything is pushed
onto the stack similar to C. Write in C. Also you have a calling stack. So your local variables go into the stack.
The calling method is invoked after the method is being invoked and is completely done.
It would come back to the stack and start off from where it had left earlier. So typically how the subroutines
and everything work in programming language. That's how it's done in Java as well. So that's why we have calling stack.
So that's the sequence of execution that happens. So let's start off with object-oriented programming Concepts.
This is pretty much generic. This is not something that is just for Java. So this is object oriented programming concept,
which usually all the object-oriented programming languages use it or adhere to it.
So we have Which we'll be covering up in the topics to come polymorphism, which is we have like static polymorphism
and we have Dynamic polymorphism cover it up in details. The coming slides will talk about what is abstraction and also
how Java has encapsulation or how Java is aligned words the encapsulation feature of object-oriented programming.
So let's start off with inheritance just to give you an analogy you could think of inheritance anything that is derived have some super class
or you have something from which other subclasses are derived when you have some feature of your parents. We say that you inherited right
because you have got it from your parent. So you have a parent so this could be an illogical to something of that sort.
Maybe you are looks is inherited from your parents sort of that is exactly what inheritance is all about. You have a parent class
and you could have subclasses out of it and this subclass is would inherit. Features or inherited State or the behavior?
I would say of your parent class. So to give you an example as you could think of vehicle right vehicle is generic thing
which could be a super class so vehicle could be thought of as a superclass. Now, there are subclasses to Vehicles you have bike
which is a type of vehicle you have car which is a type of vehicle you have buses, which is again a variant of vehicle and truck
of course is again a vehicle. So basically this defines easier relationship by if you read it out you could Clearly say
that bike is a vehicle car is a vehicle but it's a vehicle and similarly truck is vehicle. So basically this is something like it defines.
He's a relationship. So let's see about how actually this classes or the sub classes inherit
from the parent classes just to read out inheritance is a powerful feature object-oriented programming through which one object acquires some
or all properties. Behavior of parent object. So as I said, it's an easy relationship.
So he's a relationship is represented in Java through inheritance. It could also be seen as a parent child relationship.
So subclasses are actually child of parent classes in the previous case you have by which is a subclass of superclass vehicle.
So vehicle is a superclass and henceforth we would be talking in this terms of we would be using this terminology is so
just wanted to be sure you get it so vehicle is nothing but a superclass and by could be henceforth referred
as subclass child classes would be referred as subclass. So you have method overriding what do you achieve by inheritance is more
about method overriding to achieve runtime polymorphism. And the second thing is code reuse ability as I mentioned the subclass would inherit
properties and behavior from your superclass. Your parent class so there could be some method defined in your super class
which need not be redefined in your subclass. It inherits by itself. That is the property of inheritance.
If it has got a common Behavior like say you have two kinds of calculator different types of calculated the example that we spoke about yesterday.
There could be a parent class calculator, which is adding numbers as is you provide two numbers. It adds up and it gives you maybe this add
Behavior could be added into the calculator Claus now Suppose there is a subclass of calculator
where in you have this scientific calculator or something which is modifying this behavior of our
which is using the same behavior of add right basically a normal calculator or a scientific calculator will have the same add
Behavior adding up two numbers. Whatever you send it as a parameter, it would add it up and give you the result.
So basically you would Define this ad in the calculator in the scientific calculator. That would be the subclass
of your calculator you need Redefine this add method. It would inherit from the calculator method. So that reduces would redundancy
or it helps you in code reuse ability. You need not redefine the add method we have lot of slides to come about method overriding
and Method overloading so I won't talk it right away. This is inheritance.
You have a manager class and you have like employee which is extending manager. So what we are trying to show here is e dot salary
as you could see here. There's a salary property in a manager and this employed to is extending manager
and we could see here that employee to in Harrods salary property though. It doesn't have a salary property.
We are able to access the salary property from employee to let me give you an example. You just have to click on create new project
and give some project name. I'll give a dude a car modules 3 so it opens up a new window you could go
to the source folder and you could right click on it and Create a new class. So basically I'll do one thing.
I'll create an employee class. I'll follow the same example that's been mentioned here. I create calm down at Eureka dot manager class.
So calm down Eureka is nothing but the package name and manager is your class name. So now I Define a property here say salary in that case,
right suppose. I say maybe I will put it as wrong salary. So I Define a property within your manager,
which is salary. Now I Define one more class. So you could either Define it with in the same file
or you could create a new class for Simplicity. I create a new class. It will create employee.
So it created an employee class within calm down Eureka package. Now, let's do main which is your entry point for the program.
Now as you could see I haven't defined salary within employ. The first thing that I'll have to do is we
are talking about inheritance. So employ extends. This is a keyword used for inheritance.
So employ extends manager. So now you could see there is a property which is salary
which is defined in the manager and this employee which is the subclass of manager and let's see if salary is accessible to employ.
This is a place of manager. So I Define employ EMP is equal to Nu employ. This is how we create instance of the class using new now.
Let's see if just for Simplicity. I will give this e MP dot salary you could see that right just to be sure I print out so
as we can see here salary though. It's not defined in employ. It has been inherited from rather from your manager.
We can have one more instance created which is like manager is equal to new manager. This is instance of manager class.
Now, I do manager dot salary is equal to salary of manager. Is it just printing it out? So what we have done here is again,
I repeat the same thing. So we have a manager within manager. We have defined a field salary and we have employee
which is extending manager and the employee class. We don't have the field or we don't have the property salary defined here,
but we could see here in this example wherein we created the instance of employ and we are accessing salary property from here though.
It's not defined specifically in employee. It's been inherited from the parent class that's manager and manager of course has salary field
which is been access of here and we could assign some value to it. I run the program it should compile
and create a class file out of it and it runs the class file using jvm. So yeah, as you could see here,
it's printing out values for salary of employee and manager as well. So that's about inheritance it see inheriting
the property salary from parent loss. Now, what are different types of inheritance that we have this Bill inheritance wherein you
have a parent class and you define a subclass out of it so similar to what we spoke.
We had a manager class and we had employee class which was inheriting manager. That's about single inheritance.
You can have hierarchical inheritance wherein you could have multiple classes inheriting from a same class. So you could have a
and be inheriting from a single class. You could have multi-level inheritance wherein you have one class inheriting from another and again,
you could have subclass of the subclass parent class you inherited or you subclass it or extended whatever you say so there is one level.
So you have a child class. Now, you could have one more sub class of this child class.
That's what multi-level inheritance is all about. So as we go through the example, it should give you a clear idea about what I'm talking
about so single inheritance, so we have vehicle and we have bike which is extending vehicle.
So this Similar to what we defined here. So what we did here is single inheritance. You had a manager and you subclass debt
and this is just single inheritance one level. So what is hierarchical inheritance? So you have a vehicle
and you have two subclasses created out of vehicle. So there are two child's of a parent class. So this could be pretty much similar
to a parent having two kids right there could be two cats and they inherit some property from your parent. So it's pretty much similar to that.
So you have a vehicle class which is the super class and you have subclasses to it. So, let's see how this works.
So I create a vehicle class which is the super class. I have a vehicle class and civically class has a property
which says integer say number of Wheels. This could be one of the property of a vehicle class. You have say for example, you have the mileage.
So these are common properties that you have in vehicle. Say you have Motion just to add a behavior to this particular class.
I Define method move and I just print this thing out here. I defend two properties here for vehicle class,
which is number of wheels and the mileage and add a behavior to the class by introducing method
which is move which is nothing but I'm just printing it out. So ideally you would have some functionality written within or you would have lines of code written within just
because we are talking about inheritance just to show the properties and behavior is been inherited by the subclass
for Simplicity sake we don't have any logic written within move. It's just printing it out saying
that just to indicate that you know, there's a move method within the vehicle class is being called.
We're just printing it out there but in reality as I said there would be actual logic written with them. So now I Define car class and I defined by class.
So now I say that car is extending which is nothing but sub class of vehicle.
So remember we said easy relationship. Chip car is a vehicle. So what we are saying is subclassing
or we are inheriting properties of vehicle into car suppose. I change the property of any you method within or say I can have its own moment
when car cross. So basically we are overriding it. What we are trying to do is we are trying
to whatever method you had within your car class or the vehicle class. You are overriding it for the car class.
You are trying to change something similarly in your by class. You could overwrite the move.
So first thing that we'll have to do is bike should extent your vehicle. So bike is a vehicle we are extending it now.
We are again overriding this move method to say move in by class. So now basically we can have seven more just
for simplicity's sake we have just a class wherein you would write the main method. So this is generally
Practice were in such classes are called as client classes where in you have a main method and you do something with it? I just call it as automobile management class.
So you have a main method so suppose you want to change the number of wheels for a car. So what you would do is car car is equal to Nu
or it won't be carved would be like suppose I put Mercedes is equal to new car by create Mercedes.
I create BMW is equal to new car. So now what we do is I want to overwrite the property that is number of wheels for Mercedes to for similarly.
I could have a bike class say r 1 is equal to Mu by something of this sort you could have Ducati is equal to Nu by so this is just to give you an idea of
how we have classes and objects right now. You could imagine what objects is all about. So Mercedes is object of car BMW is object of car.
Arvin is object of bike and Ducati is again an object of by so now I could overwrite the properties here say number of vehicles
as to so yeah, this is what it is all about. This should give you some idea
about how we use Java you have a class you have subclasses, then you kind of create objects and you could override the properties with them
and you could change when I say override is nothing but you could modify the values within your subclasses and similarly,
you could change the behavior vehicle by itself would have some Behavior defined but Mercedes and BMW,
which is a car would have its own kind of behavior, which is not inherited from parent you're overriding it when you say
this this could be an illogical to something like kid kid doesn't have the same features as a parent. It is something like it's been overridden right?
It's peculiar to that particular kid, and that's what this is. About so you could override it.
Now. Let me print move or let me call the move method on BMW so you could see a move method
and when I run it it should show move method in car class since it's a movement that called on car BMW is instance of car.
So this should give you some idea about how are we inheriting properties and behavior from parent class. So here we can see that move in car class.
So again, you could Define your own method within your subclass, which is not something
that is inherited from your parent class. So BMW by itself or car by itself could have something like sector board
which is not in vehicle. Maybe this is specific to car. So this is what we spoke about is hierarchical inheritance.
Now, let's talk about multi-level inheritance. So basically it's nothing but say you have a super class category.
So let me use the same example. You have a vehicle class you Have a car which is extending vehicle.
Now I Define one more class, which is nothing but superclass Supercar which is extending car.
Basically, I can put this turbo in Supercar rather that would make more sense. This is like kind of multi-level.
So I am putting this turbo here in supercars. You have a vehicle class you have a car which is surplus of vehicle.
You have a Supercar which is a subclass of car. So all the properties that you have in vehicle class could be accessed
in car as well as Supercar. That's what we want to see here. So you could have something like say change Wheels
which is void which is not returning anything here. I just want to show you that we can still access number of Wheels here though.
It's not defined in car or Supercar but in vehicle class you could actually it is still visible here
and you can modify it. That's the beauty of it again. Brief, you have a vehicle class you have a car class,
which is extending vehicle class. You have a Supercar class, which is extending car class. So basically there is a multi-level
of inheritance and all the properties and behavior that you have in vehicle class would be visible to car class as well as Supercar cross
and Supercar class can access properties and behavior that is there specific to car class. What I'm trying to say is if you define a new method here,
so I just mention here Behavior specific to class to car. So there's some behavior that is specific to car that we have defined within the car class.
Now Supercar class can access this one as well. So you could see here you could access Behavior specific to car as well.
If you look it as a tree. This is a leaf node. This is super car class can access everything
from vehicle and car class. Whereas. Our class can access everything from vehicle class,
but it's not the other way around car cannot access something that is specific to Supercar. There's a turbo method
that we have put in into the Supercar class. Now. If you try to access Turbo from here,
you won't be able to access it. All right, so it's not the other way round. So you cannot access something
that is specific to your subclass in your super class. Whereas you can access anything
that is there in your superclass into your subclass. So it's a one directional flow from superclass to subclass. Now, let's talk about has a relationship has
a relationship is nothing but you have properties within your class, which is has a relationship.
All right. So say for example employee, right? We defined the employee class
and employee would have an ID specific to employ and it can have salary and stuff like that which is has a relationship
which is nothing but employ as ID sort of right so a department in a class Let's talk about this example a department in a college
has several professors without existence of departments. There is good chance for the professor's to exist hence professors and Department
are loosely associated. And this loose Association is known as aggregation. So one can exist without other which is loose Association
and such thing is nothing but aggregation. So here you could see employ here. You could see name name class itself,
which has got first and last name. So basically you have a name class which has got property as first
and last and which is nothing but the first name and the last name of any entity. It's not associated with anything right now.
It's a different entity name is a different entity which would have first and last name now this employee info class,
which has got ID and which has got name so you can see name hear this name property is nothing but The instance of name class
that is there the left hand side. So this is has a relationship. This says that employee info has name.
So basically this is what it is when you talk about has a relationship its containment. Basically you have class as a property in a class.
So in this case name is a property within your employee info and similarly this name could be used somewhere else as well.
It's not that it has to be used just in employee info. That's a kind of Detachment they have right. It's not tightly coupled.
It's Loosely coupled or decoupled. So basically you have name which is altogether a different entity defined as a class
which would have first and the last name and you would have an employee in for which is containing name which has name.
So this is has a relationship and also to give you idea about has a relationship. It's not just it has to be object.
Right so vehicle has number of Wheels vehicle has Uh, this could be thought of as a relationship. So let's talk about polymorphism.
Now when one task is performed by different ways, then it is called as polymorphism Polly is multiple of ism is forms.
Basically, that's the meaning behind it. So multiple forms. That's what polymorphism means
and when one task is performed by different ways, then it's called as polymorphism this person which is the super class.
You have a subclass student is again person millionaire is a person these are super classes. So you have a person class you have super class student
and millionaire you have a behavior, which is nothing but P Bill and which would be specific to both of them.
So as we move on you would get much more idea about the different kinds, but basically you have multiple forms.
That's what polymorphism is all about. So, what is Method overloading so we spoke about this yesterday if you remember we spoke
about Constructor overloading we're in you Had multiple Constructors Define one was integer integer, and the other one was
like it was taking three parameters integer integer n 3 integers, right? So basically this is what method overloading is all about to have
the same name of a method but you have different number of parameters passed to the method or you have different type
of parameters passed to the object to the method and based on that. It would understand which is a method
that needs to be called. So suppose you have ad with two integer parameters Define and you have ADD with three integer parameters defined now
when you invoke add method on a particular instance of calculator and you pass two parameters to it, it would appropriately call add method with two parameters
and if you pass three parameters to it, it would call one with three parameters or it would resolve it
during the runtime which one to be called rather. It's not overloading doesn't happen during runtime. It's done during the linking of the resolving operation.
Ben's during the compile time itself. That's why it's static polymorphism method overloading is nothing but static polymorphism.
So return type is not taken into consideration when it comes to Method overloading. It's just the parameters that a particular method takes.
So Alex wants to write a code which can be used to find out the area of triangle as well as of circle.
So generic code which would have area of triangle and circle so such are the instances wherein you could use method overloading.
So what's the thought behind is I want to find area of both the shapes here area is the common method. It's there for both the shapes.
It's therefore rectangle. It would be there for square. It would be there for Circle and so on and so forth.
So basically you would have a shift method which would have area which is a common method. So instead of writing two methods of different names.
I will write area as the method name for both shape. And a path different arguments according to the respective shape.
So instead of defining multiple area methods you would have just a general area method defined in your shape class
and your subclasses would overloaded as per the type of shape. So here we can see that this area class there's area method
which is taking integer since it's a triangle its base and height. So it's taking base and height as parameter.
So Java doesn't understand base and height. This is user defined by the way. It understand.
There are two integer parameters passed through the area Whenever there are two integer parameters passed to area method. This one would be called.
The other one is again, we have one more method which is area which is by the same name
and which is taking just one integer parameter, which is radius. So this is supposedly for circle Pi R square.
So during runtime when you call it, so I'm not repeating this exercise again, because we did the same.
Yesterday if you remember we kind of had multiple add methods in the calculator and we saw that it was getting resolved to the proper one
based on the number of actual parameters that you pass. So I will just read
through the slides to make you give more explanation on this but it's pretty straightforward. So here you have area method which is ticking for six
which is nothing but it would get resolved during compile-time this particular area method invocation would get resolved
to this one since it is taking two integer parameters. Second one is taking just one integer parameter,
which is 5 so it understands that it has to call this area method the second area method that's been defined
in method demo and it would call it accordingly. So here you could see that when you run this particular program you could see
that the first one resolved it to Triangle and it said that the area of triangle is nothing but 4 into 6 into point five,
which is nothing but 12 the second one goes 2 pie R square. And it gives answer as seventy eight point five.
So you could see there are two different invocations done based on the number of actual parameters. Now, let's talk about type of parameter the method
overloading can happen on type of parameter as well. So you could have multiple methods with the same name taking same number of arguments
but different data types one could be taking integer as a parameter. The other one could be taken string as a parameter
and during runtime ordering compile time itself. It would resolve that, you know, this one should be resolved to this invocation
and the other one should be resolved to the other one. So basically it tries to resolve based on the parameter based on the data type of the parameter
that you pass. So here's the example wherein you have two different methods. I will take this one.
Say for example, I Define public integer. So it's a dummy value to be printed one of the best practices
when you write Java code is to express it, right. So you can have a big variable name you shouldn't be restricting on variable name
which makes it difficult for other programmers to understand. So it's good to have big variable name versus small one,
which is not that expressive. So here I said dummies string value to be printed. I'm printing integer value is nothing but dumb integer value
and here I am printing so we have two methods with the same name but it's taking different data types as argument
and we are just printing it out. So I Define a main method here and I Define manager. So we have instance of manager senior manager grade
2 dot display I pass integer to it. I pass hundred to it and I pass so I have to Method invocations here.
One is first one. We are passing hundred the second one. We are passing a string to it and it gets resolved.
You could see that the first one would go to this display method which says printing integer value the next one
would go to this. Display method which print string value so you could see here.
Right? So first one is printing integer value. The second one is printing string van.
So based on the type of the parameter that you pass through are passed to the method particular method would be called This is static polymorphism.
Now what's runtime polymorphism. So since it is resolved during the compile time, it is static polymorphism.
There are few things that gets resolved during the runtime or the compiler cannot judge it up front what the instance
would be and that's when it does it during the runtime and that is nothing but dynamic polymorphism
or method overriding so method overriding is mostly used in an easy relationship. So in one of the methods or we saw in vehicle and car example,
we have a move method here and here we overrode it. You can see override annotation here for the move because you're overriding it overriding the behavior
of vehicle in car class. So that's where it says that this Easy relationship method overriding must be used
in case of inheritance that is easy relationship method must have the same name as in the parent class.
We saw in the move in the example move. We have the same name as in this super class. So huichol has a method move and car has a method move
as well method must have the same parameters as in the parent class as against method overloading which has different parameters method overriding
you have to be sure that it takes the same parameter if I change this to integer if I add an integer parameter to it,
so it's not taking it as overriding you could see it. It's not been taken as overriding because you have integer parameter to it.
So basically it has to have same number of or it has to have the same signature as your parent class. So that's about method overriding.
Let's have a demo on this so which we already saw but I will again A trait on that so basically this example you could see there's a man class
which has got a pay method which is adding Behavior to the man method and you could see it's a dummy printer
and you have a millionaire is a man and you could see it overrides the pay behavior and you run this program.
One thing to note here is you can assign me linear to man. You can assign unlike all the examples that we saw we are creating instances of the same class
or assigning the instance of a class to the same class. But in this case you could see the instance of millionaire is being assigned to reference of man.
This is something that happens only in Easy relationship or inheritance. So what I am trying to say here is a go-to
automobile management I have car I have a new car which is Mercedes I could assign this Mercedes to vehicle
since Mercedes is a vertical it should be a sign you should be able to assign it. This is something
that you need to keep in mind your subclass object could be assigned to superclass. So here we have Mercedes being assigned to vehicle.
But one thing to note here again is the tricky part is vehicle itself has its own move method, which Moving vehicle class
and Mercedes which is car itself has its move method with this move in car class. So the tricky part here is
when you would do something like vehicle dot move just give a thought about it which one should be called. So you have two variants of move method here.
You have one in car and one in vehicle. So the one in vehicle is printing out moving vehicle class and one
in car is the overridden version of vehicle with says move in car class. Now, what we are doing here is we are creating
an instance of a car class and we are assigning that instance to vehicle and we are calling the move method.
So just give a thought whether the move method on the vehicle class would be called or a move method
on the car class would be called. So this is where method overriding comes into picture so method
overriding is nothing but it's a runtime polymorphism which is done at the runtime. So during the runtime jvm would see
that Mercedes is nothing but the instance of car though, it's assigned to vehicle which is super class still an instance of class.
So during compile-time jvm wouldn't have a friend knowledge that which move are you calling? Is it on vehicle class or is it on something else
or some sub class of vehicle? So it doesn't decided during the compile time it delays it or there's a lazy binding
that happens delays it till the time you run it. So when you run it, it understands that this vehicle is actually pointing to car.
That's Mercedes. So when you run this you could see that I will comment out this piece of code.
So this is how we comment out that's a block comment. So when I move this you could see that move glass
on car has been called and not vehicle that is what is if you get this concept, you're pretty good in terms of object oriented.
By the way. This is an important Concept in object-oriented Paradigm. So you could see here move in car classes.
Called similarly vehicle could have I call it as vehicle one, right? I Define one more vehicle here,
which is like new vehicle and I invoke the method on vehicle to so the second variant
would call the move method in your vehicle class. The first one calls on the curved glass the second one calls on the baker was
since its object of vehicle itself. So basically you have to see on the right side, which is the object
that's been assigned since its object of vehicle. It's vehicle. But since this one is object of car,
that's the reason you have car class being called. So we had some discussion on this keyword yesterday. So super keyword is similar to that super keyword is just
that it is called on superclass. This is called on the same class super is called on the immediate subclass
immediate parent class you could have multi levels, right? But this one is calling the immediate parent used to refer immediate parent.
Us instance variable used to invoke parent class method and there's one more Super keyword one more form in which you could use is to have class Constructor.
So basically whenever you create a class whenever you call a class whenever you call Constructor of a class by default jvm
by itself would give a call to the superclass Constructor, which we would be looking at the further slides. So here we have vehicle leaven,
which is a super class which defines string Wheels vehicle moves because of wheels so that the string
that the property of a vehicle you have a truck which extends vehicle and then within truck you have your overriding the wheels property saying
that truck has four wheels. This is kind of your overriding the property. It's not called overriding though
when it comes to properties. But basically you have changing the values within your truck class print wheel is nothing but a method
that's defined in. Truck which would bring the number of wheels in your truck class and it has got super dot Wheels
which would print the number of wheels in your or print the value of the Veals not number of Wheels value of the wheels property within your vehicle class.
So we will look at this one. So we have a vehicle class and we have string Wheels which is a property within a vehicle class
which has some value put in right? We have a truck class again, which has got wheels and it is overriding the value.
It is just changing the value as truck has four wheels with interrupt class you have print will method
which is printing the wheel or the value that this particular Wheels property holds it would print it first one would be the value
that this particular truck class would hold and the other one that you see here the second print statement, which is doing super dot Wheels is actually printing the value
of wheels in vehicle class. So, let me show you a quick demo on this thing. So we Have a vehicle class
which were in we are defining the default number of Wheels as for say for example, and now we have a bike class,
which is overriding vehicle class. And what we are going to do here is we are going to change the value of number of Wheels.
I have a method to print number of fields, which would give suppose I change your number of Wheels to say to so here we print number of Wheels first
and I would have one more print statement which would be printing super DOT number of Wheels within a by class.
We have a print number of bills method first. We are changing the number of Wheels to 2 and then we are printing the number of wheels for bike
and number of wheels on the superclass. All right, and by default we have set the number of wheels in the vehicle class
to before so we have a bike class now I remove this to so now I print the number of Wheels. So I create the instance
of bike and I invoke print number of Wheels. Now you could see that first it would print to and on super dot.
Basically. This is what it's going to call so first it will print to so basically here
we need to have one more teacher number of fields when it comes to instance variables. It's not overridden as such so I have like number
of Wheels defined here again and here I change number of Wheels to to so let me run it again.
So when it comes to instance variable when you define one more field here of the same name, it's not overriding it.
It's rather creating a new instance variable. So now you could see that when you print number of Wheels it's printing out
to which is nothing but the value here value of this instance variable. Whereas when you do super DOT number of Wheels,
you have a default value of 4 here which is been printed out. So on super it gives a call to superclass. So here you are creating.
It's at the Constructor level. And we can see that so it's more about as I mentioned within your Constructor.
The first thing that is called when you create an instance is a super Constructor is called first
and then your sub class Constructor or I mean to say is when you create instance of car. So your first thing
that would be called is the Constructor of vehicle and it would do all the initialization that's required in vehicle.
And then it would call the cars Constructor. So what I mean to say is for example, I create a Constructor here and I print saying
that we are within Constructor of fecal of superclass vehicle. Now we have this car class and say we have Constructor for car class as well.
Remember we said that there's a default Constructor implicit Constructor that's already been put by GBM itself.
You don't have to take care about it. So I don't do anything here. So basically you need not create you do.
Right Constructor jvm by default right Constructor, unless you want to write something or you want to initialize something.
That's when you would write explicitly you would write Constructor. But in this case since we are not initializing anything
as such I won't put a Constructor car. What I'm trying to say is we are creating an instance of car here.
And let's see when we create the instance of car. There is a super Constructor that's been called. That's what I wanted to show.
So that's why we created a Constructor here for vehicle. This is not a method. This is constructed by the way,
since it has got the same name as your class and it doesn't have any written value. So so I have printed it out saying
that we are in the Constructor when we call this now when we create the instance of car you could see that this Constructor of superclass that's vehicle
that's been called. So this is done implicitly by compiler. You don't have to care about it.
So here you could see whenever you create the instance of car you would see that we are in construction.
Of superclass vehicle and the second print statement that you see is directly for the vehicle sensation. So even for car you could see
so this will get rid of confusion. So there's only one instance of car being created and we can see that it is calling vehicle Constructor
and it's implicitly put we don't have to explicitly put it jvm by itself operates that way
and how it calls is nothing but by Super Final keyword we again touch based on this one earlier in the session. Number one final keyword is non access modifier applicable only
to variable method or a class when it's applied to a variable. We say that the variable content cannot be changed usually use final when you want to define a constant
within your application. So that's final a method could be defined as final you define a method as final when you say
that your subclass cannot overwrite it that's when you define it as final you define a class. As final when you say
that there cannot be any subclass of that class. So you cannot subclass it like if you try to extend on a final class you
would get an error compile time error saying that you cannot extend it. So just to show you maybe
if I make this as final your car would show an error saying that you cannot inherit from final class. That's Comdata recorded pickle.
So that's about final class. And if you make this method final you would again get an error in the class saying
that you cannot overwrite this the car class you are trying to override the move method since its final it showing you an error saying that move
cannot overwrite move in calm dot Ed u-- recorded vehicle overridden method is final. Let's remove this finer
and you should see the error is gone. And also if you want to see at the instance level if you define this as for number
of Wheels as for now within this vehicle, if you're trying to modify this number of Units to to it would throw you an error saying
that cannot assign a value to final variable. All right, so that's what it does it. It's not access modifier,
but it regulates it in a way that you can't change the value in case of variables you can change the value in terms of class.
You can't subclass it or you cannot create a child class of a final class and in terms of methods,
if you have final method you cannot override that particular method in your subclass. So final modifier can be applied to class method instance
variable class variables local variable and Method parameters. So final variable is used to create constant variables when it comes to variable
as I said, usually you use final variable when you want to have constants, right
and it would be final static. By the way. If you have a constant usually final and static
static final final methods is used to prevent method overriding in terms. Method the demo that I showed you previously we saw
that we cannot override it in terms of class. When you have a final class, you won't be able to create subclass of it which we saw.
All right, it gives you an error some examples of final clause in string class. So system class
in Java dot Lang dot package is final as well string class that we have been using string class that's been exposed by or Java itself has
or Java by itself defines it that class is final as well. If you try to extend string class, it would show you an error it would give you an error.
So system is kind of putting a contract or Java by itself is putting a contract or is saying that cannot extend the string class.
You cannot extend Its Behavior. You can't change anything any of the behavior within your string class by yo,
that's why they have marked it as final if a method is declared as final then it cannot be overridden in your child class.
If a Variable is declared as final then you cannot change the value of that particular class or cannot change the reference of that particular class
when it comes to objects. So we will see that later a Constructor cannot be declared as final.
So that's the rule you cannot declare the constructors final and blank final variable should be initialized in Constructor. So maybe in this case
if you wouldn't have any value here, you declared this as final if you don't have any value here. It has to be assigned an Constructor right now.
It's giving an error saying that it's not initialized if you mark it as for it should be gone.
So it's mandatory that if you put instance variable as Final in class, it's mandatory that you assign some value in the Constructor.
If you don't then compiler would throw an error so here you can't override it. So basically you have a final method void run
and you have a sub class of vehicle and you're trying to override Run method, it shows you an error.
So this is what we saw in case of was Final and when we try to override it in car we got an error and it's all compiled sign
if you declare any class as final you cannot overwrite that class which we saw it gives you a compile-time error.
So when it declared a vehicle as final and when you try to extend when you try to create car class, which extends vehicle class it gave you an error
because vehicle class was fake lettuce final again. This is a compile time. Now what's Dynamic binding
which is also known as runtime polymorphism is nothing but during runtime it decides which instance of which instance
of a method should be called or which method should be called. So this is in case of again inheritance
and when you override the method in your subclass, so we saw this earlier again to just to show you we had this move method here
and in I have this move method and in automobile management, we did this thing we have a Mercedes that is assigned
to vehicle class class reference rather vehicle class reference of reference named by vehicle 1 and in the second thing we have vehicle itself.
So first one is pointing to an object of car, which is Mercedes and the second one is referring to the object of vehicle itself.
So this is what dynamic polymorphism is all about. The first one gives a call to the move method in car the second one second one gives a call
to the move method in vehicle. So yeah, you could see here. So move in car class
and then it calls a moving vehicle class and it happens at run time. That's why it's runtime polymorphism.
So abstraction is a mechanism of hiding the implementation details from the user and only providing the functionality of a user.
So basically you could have abstraction. We're in on the first day. We spoke about the shape class and the circle class
and Wrangle and square and stuff like that. Right? So basically shape is a class which is abstract class,
which doesn't know all the functionality up front door. It doesn't have all the behavior of front. It doesn't know
what would be the behavior of a circle class or it doesn't know. What is the behavior of a square class
when I see Behavior. It's nothing but calculation of area calculation of area is nothing but it adds Behavior to the class
and calculation of area in your Square class would be different calculation of area in your circle class would be different.
So shape by itself doesn't know what the implementation of area method I would say. So that is what is attraction.
There are two ways in which you could provide abstraction in Java one is by abstraction class, which is not hundred percent abstract,
which could have like abstract methods like area in this case is about Track but there could be some methods
which is same throughout or common throughout all the subclasses of shape say for example printing area. You just have to have a print statement
which is printing area for a particular shape from the implementation per se it's same throughout all the classes area method
would be something that is abstract. Whereas display area could be something that is not an abstract method.
So in such cases wherein you have blend of abstract as well as non abstract methods, that's where you use abstract class.
So it's not hundred percent abstract. Whereas in interface it has to be interface is something which says that it has to be a hundred percent abstract.
So interface is altogether a new construct that we have in Java when it comes to abstract class abstract is just
a modified to a class. So you write class and you just prepend it by abstract which makes that class and abstract class.
Whereas interface is something that is a new construct that we are going to see in the coming. Slides upset class
and Abstract methods so abstract method is as I said area would be abstract method in this case wherein you don't have a concrete implementation
of area in your shape class. That's the scenario in such cases wherein you don't have a complete concrete implementation
of a particular method you would declare it as abstract method and if you have an abstract method, if you have at least one abstract method
in your class, you have to declare that class as abstract class or else compiler would throw an error.
So whenever you have a abstract class, it means that there is at least one method within that particular class which is declared as abstract.
So when you suppress this abstract class so shape for that matter when you subclass shape shape has got a area method
which is abstract. Now when you subclass it when you create Circle, which is nothing but class Circle
which extends shape now you are creating surplus of it. That case you have to ensure that you implement area. If you don't Implement area,
it is still kept as abstract and you will have to make circle class as abstract as well. If you don't give the actual implementation.
So an abstract method is a method that is declared without implementation any class that contains one or more abstract methods
must be declared with abstract keyword and abstract class is a class that is declared with abstract keyword
and abstract class may or may not have all abstract methods. So as I said,
it's a mixture of abstract and on abstract methods and abstract class is mostly used for inheritance. So let me take the same example of shape and shapes.
So we Define a shape which would have abstract area. This is what I was saying. So when you have at least one abstract method the compiler
will throw an error. So in this case it is saying that you have abstract method but your class is not abstract.
So we have to make this one as abstract. And as I said abstract class is nothing but a modifier you just have a modifier
that you need to prepare into the class. This is abstract method and you say you have a method which is public void.
It's not returning anything. We are just displaying area now rather than defining it here. Maybe I will put something like So we have a shift method
and now I declare Circle method now, this circle method is extending shape now. It's throwing an error and it says you have to implement
the abstract methods shape as got abstract method if you don't implement it as I mentioned earlier,
if you don't implement it if you choose not to implement the area method then you will have to make this abstract.
If you make this abstract error would go off. But in this case since we know the concrete implementation of area
for Circle will have to implement it when I say we will have to implement it we say area and we override it.
So for Simplicity reason, I'll just put some value here for now since we are talking about abstraction.
I'll just keep it simple. So basically what we are trying to do here is we have implemented area
which would be nothing but your Pi R square actually, so here we have a shape class and we are overriding the idea method here.
And this is how you abstract it. When you run it, so basically you could have something
like suppose I create a shape utility class. So this is pretty much like overriding methods. So you could have a shape class shape circle is equal
to New Circle and you could call Circle dot area. So basically what you would get is nothing but a float value, which is your area area
of let me put it this way area of circle when I want to print it. What I would do is circle dot display area
and I print area of circle what we have seen here is there was abstract method area which was implemented
in your subclass that Circle and when I give a call to it and here you could see that again similar to vehicles that we spoke
about instance of circle is being assigned to shape and when you give a call to it you could see that method on circle is being called and not on shape
because shape by itself doesn't have any implementation of area. So one thing to note here is when you have Class. So here you could see this value being printed operate abstract
class would have one or more abstract methods for sure. And when you subclass it, you have to ensure that you give implementation
of your abstract methods. If you choose not to implemented, then you will have to make the subclass
as abstract as well. So execution of abstract method. This is very much similar to normal exhibition
that we have. So the main method you have instance of mobile class created this case then there's a default Constructor
of mobile class gets created gets executed. So pretty much similar to normal execution when you create an instance of Nokia,
which is subclass of mobile. As I said the first statement that you have the Constructor of subclass is nothing
but super or it calls the super Constructor. So here we can see that the default Constructor for super is being called
when you try to instantiate Nokia as we are running run method, but in Mobile class run is an abstract method so
run method from Nokia class gets executed similar to the example that we saw so shape area class area method on Circle got executed and not on shape.
What is the encapsulation that encap solution is the methodology of binding code and data together into a single unit.
So basically everything it's a encapsulation. It's put as one so you could imagine class being put as one right classes called this for that matter.
You see everything been encapsulated as one right you group these together you group this integer and long
which is a property of vehicle and it has got some Behavior as through this move. It's all encapsulated as one
or it's all put together as one entity that is nothing but encapsulation. So basically you could imagine a capsule right
which has got multiple ingredients which has multiple made sense or chemical components which are put together into one capsule
and you have you think capsule has one mixing but it's basically combination of chemical components Within Thing is for encapsulation.
There is access modifier comes into picture you have this access modifiers or private and you have public
you have protected you have default this access modifiers restrict or it restricts the visibility
of regular component of a class say method or variable or anything. So that's encapsulation feature of object-oriented programming.
You can't see everything. It's not that everything is open for everyone. So you can have restriction you have
different levels of restriction when it comes to visibility of this components that is a part of encapsulation as well.
So to achieve encapsulation in Java declare the variables as private usually the best practices not to expose everything
because once you expose something as public or once you expose it for the application to access you have to ensure that you maintain it right because anyone can access it.
So your class is becoming much more fragile a I won't talk about this topics right now because fragile D is something that is related to code quality
or coupling we say so I don't want to touch on that. But basically the best practice
is to have less visibility restrict visibility or different visibility as much as possible. Try to make it as much less as possible make it private.
Basically if you could make all the variables as private it would be good thing. So basically all non private
or public variables are liability for application. So it increases the maintenance of the application and it's easy to break that way
if you make some changes to a class it becomes very difficult the future to maintain it. So usually the general practice is to keep the methods variables
as private not methods variables as private and we have Getters and Setters method which exposes which are public methods or mutator methods
which would expose this variable to the outside world through public. So usually we don't have Setter methods.
We restrict Setter methods again. Usually you have a private since variable and you have a getter method
which is a public method which is nothing but Returns the value of the instance variable to the outside world.
So data hiding the user will have no idea about the inner implementation of the class. What are the advantages of data hiding user
need not know the core implementation of the class. It increases flexibility. We can make variables and methods read only
and right only as per the requirement. So imagine if you didn't have all this access modifiers, there's only one that is public which is exposed to everyone
so it would create a Havoc. So this is basically based on your requirements. You could have different patterns
of this access modifiers used within the application. It makes testing easy. So basically Hotpoint is reuse ability easy to reuse
and easy to change with the new requirement. So with reusability, even the maintainability
of the particular application is improved when you have this encapsulation and easy to reuse in the sense if imagine The way around
where in you have only private methods so a class could access only the instance variables within a class nothing from outside world.
It cannot be accessed from outside as well. Imagine such a scenario right in that case. You would have a lot of code redundancy right everywhere.
You would write the same code. It might be the same thing. We have already implemented in some other class,
but you will have to re-implement it since it's not accessible outside the class. So that's how encapsulation increases the reusability of who
and it makes testing easier. Of course. So here you could see that employ one.
So this is the encapsulation mutator method that I was talking about. So basically you have employee one
which has got string name, which is private you could see here. It's defined as private when I say private this name property
would be used only within this class. If you try to access it from outside class you would get an error
but there might be scenarios in which you want to Says this name from outside class you want to understand what its value is in that case.
You would have something like this right? You would have getter method get name, which is nothing but it's written type is string
which is the data type for name and it's returning the name. Now one thing to observe here is this is public.
This one was Private name was private but get name is public which means it could be accessed from outside as well. Similarly.
You could have Setters which is not a good practice. By the way, you shouldn't have Setter methods. So again Setter is something like you pass the value
that you want to set this particular name variable. So basically you are saying that whatever you send through this method is going to set to the name variable.
So we are referred to this particular example in Maine. So you create an instance of employee one
who have edad set name you're sitting at well X and when you access it you do e dot get name. So basically what we are trying to say here is
a demo end caps is a Glass it's not the same class. So you have employee one and you
have demo end caps as a different class though. It's a different class till you are able to access your name employee name
from this class. This is again, a private instance variable you are able to do this just
because you have this Getters and Setters, let understand interfaces interfaces are nothing but could imagine interface as a blueprint
or it is something that it's a specification rather, right and interface could be thought of as a specification. This is how it should be.
So basically it could be a company manufacturing bottles or remote rate. It would say that this is
how it should be basically there should be so-and-so buttons. There should be this button should be here. This button should be there and something of that sort.
So basically through interphase what we do is we specify the contract we say that this is how it should be now.
For example, you could have specification mentioned in a piece of paper. This is how Remote should be
and there could be different vendors for this remote who would be actual manufacturing remote and which would be aligned
to this particular remote specifications. Then you could check that. Okay, so and so specifications are met which means
that this this particular thing could be used as a remote. So basically these are nothing but the specifications for the system so through interphase you say
that this is how it should be and you would have different vendors or you would have different implementations
that align to the specifications and if they match up to the specification, it means that it's a correct one.
So hence an interface contains all the specifications and can be used for creating a new remote. So all you see here,
there's a joystick this AC remote this TV remote and everything. It has got different things
but there's something in common and that's what you specify through interface and interface contains variables and methods
but the methods declared inside interface or by default abstract methods. As we saw in the earlier slide interface is
hundred percent abstract. So all the methods that you have within interface is abstract and interface is used to achieve abstraction.
It is used to achieve loose coupling when I say loose coupling. It's like you are not binding everything into a class.
Basically you say that fits a remote. It has to have this features, right? So basically your kind of decoupling it or you can see
that all the implementations of remote will have so-and-so specifications, which is been introduced by interface also in Java,
you cannot have multiple inheritance. You cannot have a class which extends multiple classes cannot have car
which extends vehicle and which extends the for example locomotive locomotive is one of the classes if for example,
so, you cannot have a class which extends to classes that is multiple inheritance. Do you could have levels
and you could have hierarchical inheritance, but you cannot have multiple inheritance. But through interfaces you can have multiple inheritance
you could parlament multiple interfaces, but not multiple classes again, since this is
inheritance interface is hundred percent abstract class. And basically you create sub classes from or you implement an interface is nothing
but it follows he's a relationship. So what's the difference between interface and a class and interface can never be instantiated
just to give you an example, I'll show you what interface is. So instead of this class.
I will make this interface. So this is how we Define an interface we Define interface, but as I said,
the interface is hundred percent abstract. Everything has to be attract. You cannot have methods something of this sort.
You cannot have a concrete implementation of method though. You could have a default method which we would be looking at in the in the slides to come but at this point,
let's imagine that you have a ship class and whatever methods you have you declared within your ship class has to be Track a by default.
It is abstract. You did not even mention it by default jvm puts it has abstract.
Even if you mention it abstract, it's not a problem. It is abstract. So now when I unlike extends
that we do on glass or for the face you implement it so you implement shape class. So you'll have to make it public by default the access modifier
for a method the face is public. So here you can see that we have implemented it we could see
that it has a hundred percent abstract. So with this you could see most of this differences being listed here and interface should contain abstract methods,
which we saw class can contain only concrete methods. We are talking about normal class here not the abstract class.
The members of the interface are always public which we again saw when I didn't implement
this one as public Circle it gave me an error. So by default it is public the members of the class can be private public
or protected and interface can I have a Constructor since we are not creating instance. The first point you can see that interface is
cannot be instantiated since we cannot instantiate interface. There's no need of Constructor a class can have constructed to initialize the variables
which we met yesterday implements keyword is used for inheritance and whereas in terms of class, we have extends
which we saw now right for interfaces you we changed it to implements rather than exchange after extends keyword.
Any number of interfaces can be given this is multiple inheritance that we spoke about whereas after extends
you can have only one and only one class. You cannot have multiple classes cannot contain instance Fields. The only field
that can appear in our interface must be declared both static and final can contain instance Fields. So only the constants would be declared
when your interfaces whereas with in class. You can have all levels of all the instance variables. Classes have implementation
whereas this is between class and abstract class. So classes have implementation abstract classes have no implementations or they can have implementation as well.
So it's a mixture of abstract methods and non abstract methods as we saw.
So abstract class is not hundred percent abstract right unlike interfaces. You could have concrete implementation
as well concrete classes are instantiated to create object abstract classes cannot be instantiated similar to interfaces
abstract classes cannot be instantiated a concrete class can be final and abstract class can never be final as it has no defined functions
for abstract class to be a complete. It has to be extended. That's what the focus is abstract class
and interface is an abstract class can be extended with class using keyword extends and whereas interface
can be implemented to a class using implements which we saw. Abstract class can extend only one class at a time
and interface can extend number of interfaces at a time. So yeah, this is one thing we're in your interface can extend other interfaces, right
and you could have multiple interfaces extended abstract class can have private default protected and public members
and interface members are default or by default public in abstract class keyword abstract is mandatory to declare a method as an abstract method
which we saw okay in interphase. It's not mandatory because by jvm by default put set abstract classes
are to achieve zero to hundred percent of abstraction, which means that you could have some concrete implementation as well.
Whereas interfaces or hundred percent abstract. You cannot have anything you cannot have any implementation within but you could have a default methods abstract class
can have abstract and on abstract methods again here abstract class can have Constructors.
Interface cannot have Constructors which we saw earlier abstract class can have abstract and non abstract methods
since it's not a hundred percent abstract. Whereas interface can have only abstract methods by the way from java it as I said,
there's a default method being introduced which is nothing but you could have a concrete implementation written
within interface class interface relationship you have class which extends other class you have class
which implements interface you have interface which can extend other interface if there's an interface already defined and you want to inherit
some methods or you want to inherit the methods that you have within the other interface would do it using extends a class extends another class
while implements an interface and interface extends another interface class cannot extend multiple classes,
but can Implement multiple interfaces. So here you could see that this one class. Which is extending the class on the top
and this one at the right side. It's time to extend it. If you try to extend multiple classes,
it would give you a compile-time error. It won't allow you to inherit from multiple classes. Whereas in this case,
you could see it's implementing multiple interfaces on the top. You could see one interface been implemented by this class and there's one more interface to the right
which is being implemented by this class class cannot extend multiple classes, but it could Implement multiple interfaces
which gives an opening for multiple inheritance when any interface gets compiled compiler automatically adds access modifiers to the members.
So this is done by default its internal Edition. So here you could see interface demo which has got one variable
that is int count is equal to 10 and it has got a method which is output now when this demo dot Java,
which is an interface, which gets compiled you See that by default. It has put it as public static final so count 10 would be given
access modifier public static final so four variables jvm by itself puts public static final and four methods it would put it as public abstract.
So that is the reason when we had the area method when we try to override it it gave an error saying that it has to be public.
So John went to a bank he wants to credit some money to his account. So let's see this one.
So basically here you could see to implementation of it you have money interface and you have operation as its method. So now you could see two implementations of it
one is debit and the other one is credited with in debit. We are just saying that we are writing a dummy statement.
They're saying that we are debating money from this account whereas credit is nothing. But again, we are printing saying
that we are crediting money to this account. So now when you create instance of money or you create in It's off credit
and assign it to money and money dot operation. You would have operation method on credit being called in this example.
We can see that there's a shape interface which has abstract area and there's a circle which returns this value right and say I declare one more class
which is square which implements shape and its again three main error saying that you will have to declare it.
You will have to implement the method so I will read this and say I written some dummy value like hundred you have a square and said Circle say circle area.
We are just hard coding it right now. We don't have to implement it. So it gives you two hundred.
It's returning 200 as float. And this one is returning hundred. So what we can do here is we have this shape utility.
So here we have this thing called on Circle. So I will just print it out here saying And now I have one more say for example,
I create one more shape which is square nothing but Nu Square now what we are doing is we are going to print out
where dot area so instead of assigning to a variable you could do it this way as well area of a square. What we did is we have interface shape
and we have a area method which is abstract. We have two concrete implementations of it Circle and square
and we are instantiated circle and square here and we are calling area method on it. By the way,
you could see here that this is dynamic polymorphism Circle and square is been assigned to the shape reference. It's not directly to the circle.
You would see hundred and two hundred mean printer know so Circle was 200 and square was hundred and you could see two hundred
and hundred so it's basically Dynamic polymorphisms followed in interface as well. You could extend an interface with another interface.
So suppose you have one more Switch is for now just too. So I create an interface test interface
which has like test method. So you have a test interface which has void test and you could have shape which is extending test interface.
Now your circle and your Square would give an error because there is one more method that you need to implement. If you don't implement it you will have
to either make this abstract. If you make it abstract, it should go off but in this case suppose
we want to implement it then you will have to ensure that you implement test method as well once implemented the error should be gone similarly in square.
You will have to find it. So that's what is extending one interface with another right? So basically here you could see there's walkable.
There's a runnable and when you do it when you implement runnable, you will have to ensure that you implement Walk and Run
both the methods. It's a class extending one class and implementing more than one interface.
There's two interfaces Walk and Run so you could see animal which implements walk and So then it will have to implement
both the methods Walk and Run whereas there's a human glass which extends animal. So this is basically there's a class
which is implementing multiple interfaces and which has to ensure that it implements both the methods.
So in this case walk and run it has to ensure that it implements Walk and Run and this one class which is extending from this particular class
and you could see here that you could assign human which is extending which is a subclass of animal
we could see that human could be assigned to walk and run as well since it is implemented from there.
So you could have H 1 dot walk and H 2 dot run sort of you have given a call to this methods.
So basically this is again if you understand the idea of runtime polymorphism or dynamic polymorphism,
which is nothing but assigning the instance of subclass to superclass you should get idea of me. All this thing we are pretty much talking the same thing
all this is talking about Dynamic polymorphism. If you get that thing, right you have good enough. So we are talking about default method right
since Java it you could have default implementation within your interface. You could say that you cannot have a concrete method
but you could have if you put a concrete methods something like this, it would give you an error.
Okay, it gives you an error saying that it's not allowed but you could have it as default. Once you define is default.
It's good enough. So all this methods are all these classes circle square and all these classes would get would inherit
this they can't override it. They can't do anything with it, but they would inherit this we can give a call though,
but he can't override it. So basically I'll just show it here. So basically you have default method
which is say say and which is printing out. Hello. Welcome to edu Rekha, right
and you can see here. Flash interface demo, which is implementing welcome and you could see here.
Hello bean concrete implementation of L, which is nothing but it's printing out the message that is passed to the hello method so
though default class interface demo doesn't declare say method it inherits from welcome. When you do out dot say you could see the default method
being invoked here. We could see that you know, hello welcome to edu Drake has been printed out
which is nothing but the output of default method so basically default methods are not but method which cannot be overridden
but is available for all the classes that implements a particular interface or implements that interface
so rules for using private methods in interfaces following all the rules for using private methods in interfaces private interface method cannot be abstract
private method can be used only inside interface private static method can be called from other static.
Non-static interface methods private non static methods cannot be called from private static methods. This might look confusing at this point,
but you could think that first of all private interface method cannot be abstract because we want it
to be implemented in some other implementation, right? We cannot keep it private since interface by itself doesn't have the implementation of it.
You want to implement that method in some other class. So it cannot be private private method can be used only inside interface.
So if we Define a private method within an interface, it can be used only within the interface. So basically you could have a default method
which is private, right? So basically default method itself cannot be private but within default method,
so what I am trying to say is so here you could have private void which is doing nothing but here your default method would call this private method.
So private method can only be used within the interface and it's usually used 24 get private static method can be called from other static.
This is pretty much similar to other classes normal classes private non static methods cannot be called from private static methods.
This is a common rule that we have private non static methods cannot be called from private static method.
So if you have a static method within the static method, you cannot call a non static method. Remember we mentioned it earlier
unless we create the instance of it. We can't call it. But in this case you cannot call it at all.
So it's not on a class you're invoking it basically within an interface. So private methods is specifically you could call
Private methods from within your default method in interface and since private methods are not accessible from outside. That's the only reason you could have private method
near interface static methods in an interface. So you could have something like this where in you have interface one and you
have a static method display? You remember static methods are more of a class level method, right?
You need not have to create instance of a class to invoke a static method if an interface has a static method you could call
it directly by interface dot the name of the interface does the name of the method so we can have a static method like static.
So this method could be called so you could have something like this method and you could call it directly using the interface name.
So we have this so now within shape utility you could wreck akahl, like as you could see here,
you could call the static method directly by the name of the interface. You cannot have normal methods within it
unless it's a default one interface features four different jdk version. So interface has evolved throughout the versions
of JD case. So just to brief on that. We have jdk 121 .7
having normal interfaces with constant static variables as we said the variables that you would have in a The freezer typically constants
which is public static final and with abstract methods, of course in Java 8 it evolved and we have a default method as I said,
there would be one default method which would be inherited by all the interfaces extending that interface and you have static methods as well,
which was not there earlier in Java 9 and later versions. So in Industry right now, it's mostly Java 8 that's been used across
very few have gone to Java 9 all the big data Frameworks and everything we have is supporting Java it at this point.
So with the latest version that's Java 9 and above we have static constant static variables abstract methods default method static methods
and they have come up with this two methods which are nothing but private methods and private static method,
which was not there earlier. Now you could have this things as well. It makes very little sense to have all this scope variables,
but maybe they might Have or something as a part of evolution, but at this point,
I believe since this private methods cannot be called from outside it just that you have control over the default method.
So basically maybe if you have a big logic to be written within your default method
just to modularize it just to break it into multiple methods not to have one monolith big function or big method you could have this private methods.
That's what I can think. What is the package we have been using package since day one. So package is nothing but it's a namespace
it's for avoiding Collision. So basically I could write a name class with name shape and maybe someone else
within my team would write the same thing. Right? So basically at the end when we archive it together
like we have a jar file, which is Java archive when we archive this files together there is going
to be named Collision, right? You wouldn't know which shape class. Are you referring to?
So basically namespace is nothing but it adds. Stewart and it avoids Collision classes in the same package can access each other's members.
So basically you can have within a package. So we'll talk about the import statements that will give you a clearer understanding
of what we are talking about. But basically within a package as you could see here, we have not done anything to refer any of these classes.
So it's within a package we have calmed said Eureka and we have all these classes within this package so you could see that employ or maybe manager
which is a different class in the same package. We are accessing it directly. We are not doing anything different for it.
But when you want to access it from a different package you have to do something else which is nothing but importing the package
the normal living convention is usually domain name here. You could see we wenshan calm down at Eureka. So it's usually something
of that sort dorji dot example dot hyphenated name. It has to be lower case usually written in lower case companies use their reversed.
Net domain name to begin their package names. So basically if you have my package or example.com, you would have it in reverse order.
This is typically a one of the best practices of practices that's been followed across industry to define the package but it doesn't have any constraints
from the Java side as such you could do anything. So for example,
if I make it uppercase, so basically it's saying that it's not showing error because of its in uppercase, but basically you don't have a directory starting
with uppercase Aspire Java or from the Java side. You don't have any constraints. For example, I could do
something like So basically it does convert it into lower case, even if you give uppercase. I don't know whether this is a feature in IntelliJ IDE,
or it is of course it has to do something with the ID because Heidi is converting it into lower case as far as the Java naming convention is concerned.
This is what we do but it doesn't have constraint Java predefined packages. So Java itself has many packages.
So you could imagine the entire language been written in Java source code. So basically all this you would find in the source code
when we refer to the string when we refer to string string is a class which resides in Java dot Lang.
There is Java dot language is nothing but Java dot language. So string is something that resides in Java dot Lang object is a class
which is a super class for all the classes. So, you know, what super classes are after we went through the inheritance thing.
So you have super class and you have subclasses. So the superclass All the classes no matter what class you use with in Java.
All the classes are inherited from a class called object. There is a class object in Java from which all the classes have inherited
from our all the class inherit from this particular class thread is used for multi-threading.
Java dot length of thread is used for multi-threading multi-threading is nothing but 2 lightweight processes in order to leverage the potential
of your processors. So if you have multi-core, then you could have multiple operations happening
at the same instance of time. That's what thread is all for you make it faster you have exception class
you have system class Java dot language system. This package is used to achieve the language functionality such as conversion
of data from string to fundamental data display the output on the console and many more the package is quoted by default.
So you don't have to specifically explicitly import. This particular package is by default voted into your application Java dot util.
This is like utility classes you have Elections and stuff like that into your Java dot util this package is used for developing quality
or reliable applications in Java or J2 ee this package contains various classes and interfaces which improves the performance of j2me application.
So basically these are utility classes. It does something in terms of performance or does some conversions or something of that sort.
Java dot IO is a package which contains something like file input stream file output stream and stuff like that,
which is basically for interacting with your files with your I/O. So basically if you want to load something into your memory,
if you want to read something from your file or write something go file in your local directory, you would use something like Java dot IO package
if you want to do some kind of socket networking or some kind of network programming you would use java.net which has something like socket datagrampacket
datagram socket Etc. We have applet tablet is nothing but application that gets loaded into your browser.
It's not you. So as of now earlier it is to be used but now there are a lot of other technologies
that have come up but this is something like an applet is a Java program which runs in the context of w WR world wide web for browser.
You have Java dot e WT which is like event-driven like you could have UI applications. If you click on your checkbox
or button there would be even triggered and you would have an event handler handling it if you click on a mouse
or something you would have a even triggered. So basically all these classes are grouped into this package, which is Java dot AWD
so you could see the Java dot w dot even which has like Mouse listener. This is specific to event without this classes.
It is impossible to handle events generated by G UI components. So as far as the front end
is concerned as far as all this a WTF that is concerned. It's not in use basically.
Java is much more useful in terms of back and program which means you take data and Do something with your data and stuff like that,
but it's not well suited for UI applications and it's not used to that extent in Market or industry. You have Java dot SQL
which is nothing but your database related stuff. So we want to connect to your post gray or if you want to connect to Oracle.
You have all the stuff written in Java dot SQL. So mostly in Java data skill, you have interfaces and this interfaces
as I said, it's nothing but specifications and this specifications are implemented by database vendors.
So it's nothing but interface is just an abstract method it would have like four five abstract methods within so all this concrete implementation.
Someone will have two women to use this interfaces or someone will have a class which will implement this interface has and we
as developers can use those classes to interact with databases. So Wenders like Oracle will have its own set of implementation
to the Patients that are mentioned in Java dot SQL as I said most of the thing in Java dot SQL or interfaces.
So about the access modifier. We have spoken earlier. We have public we have protected private and default modifier
public is nothing but it could be accessed from anywhere within your application. So you could have your methods as public you could have
your class as public you could have your instance variables as public which means that particular method could be accessed
from anywhere within your application. You could have protected modifier protected modifier is nothing but your subclass is can access it
and your current package whatever the package in which a particular method or particular class resides in which you have protected method.
You could access it from that class or any class from that particular package access the protected methods defined within a class that package
so I will give you an example since we have spoken about inheritance now, so I will give you a demo on protected private.
Modifier is basically it's private. It's within a class. You cannot access it from outside.
If you try to access it, you would get a compile time error saying that it's not visible default modifier is package is public
within package So within package any class can access it. So basically it's like protected right protected can be accessed
within the package plus it has like if a sub classes are outside the package, even though subclasses can access it
whereas in default. It's just within the package. So protected has wider scope then default modifier.
This is kind of public so public has almost like everything so why didn't scope then comes protected then comes default and then comes private so access modifiers public word Republic.
So when you have Public Access modifier within a class in this case, you have two packages.
Are you Ricardo back one and a new record out back to and you have some public members within your class one,
which we can see here that all this public. Members could be accessed by Class 2 class 3, which is there in the same package as class 1 that is
at Eureka dot Pack 1. So now there are two classes in a do record back to one is a class
which doesn't have any relationship with glass one but still it can access it though. It's there in a different package.
But still it can access the public members of class one. You can see class for which is a child of last one since it extends class one,
but it's there in a different package but still it can access the public members of class 1 so protected protected is nothing
but it could be accessed within the package in which the class is defined and it could be accessed by members that are outside the package but child of that particular class.
So in this case we can see that there are few protected members in class one which could be accessed by Class 2 and class 3
since they are in the same package as class 1 plus 3 and class two are defined in Eddie cannot pack 1 we have class 5 Is not related
to class 1 which means it's not a subclass or it doesn't have any relationship with class 1 in that case class
5 cannot access any protected members within class 1. So here we have class for which extends class 1 which is nothing but plus 4 is a subclass of class 1 which is there
in a different package at your record back to but it can access the protected members of class one
since it is there in a different package private member is nothing but it can be accessed just within the class in which it is Define.
So in this case you could see that this is private members. So you have class one which has got private members and you can see that class to contacts
is it class three contacts? Is it either class 4 or plus 5 no one can access it. It's just that class one can access private members.
So we have seen this in examples that we took in session one default package. So default package is nothing
but only within the package in which it is defined. It so in this case you have class one which has got default members with default scope
and you can see that class 2 and class 3 can access it but not class 4 and Class 5 since they are out of this package
since Class 2 and class 3 are defined in the same package as class 1 that is a deal-breaker DOT back one. That's the reason you could access from there.
This is same for attribute or method. There's a table here, which is summarizing whatever we discussed so far you
have modified public. So yeah, it's within the class. Yes.
It's within the package. Yes, if it's within the subclass. Yes and within the world days
so everywhere it could be accessed right? That's why you have public protected is within the class. Yes, within packages within subclass.
Yes, but not within world. So just within package and subclass, but if you have
different package or if you have different package, but it's not a subclass. Then you can't access it.
No modifier, which is a default scope within the classes within the Yes,
but we saw that it cannot be accessed within the subclass if the subclass is in a different package
when we save world world is nothing but any package and they are not related in this case the previous example that we should saw last five is something
that could be thought of as laws that are unrelated. But reside in a different package private is just within the class you can see
that it's not with package within the package. It's not within the subclass. It's not within the world.
So I think we have spoken about private public and default scope earlier. Let's start off with protected.
So in the meanwhile when it starts, let's talk about this. So here we can see package demo
which shows public it has got a public method that is message. There are three ways to access the package from outside.
So let me talk about how we actually recorded. So there are three ways to access the package from outside. The package one is with import this case
like you had a direct a 1 and enter a car to right there. Sample that we saw earlier. I do record Pack 1 and add
you taken right back to these are the two packages you have if you want to access something you want to access class 3 from class 5 you would have to import
a new record Pac-12 class 3, since it is outside the package of back one if it's within the package so far
the examples that we were taking was all within the same package, so we didn't have to import it now.
I will just show you an example wherein you would have something that is outside the package and I will show you how to access it.
So we have to import it. So whenever you have it in different package, then you have to input that particular package
within one thing is importing the entire package. The other one is importing a particular class. So in this case you have a package demo,
and we can see that within package demo. There's a pack demo, which is nothing but a class defined within it.
So, let's see how to access package from another package you have a Demo here
that's a class that is defined within the package demo as you could see here. There is one method which is MSG and
which is taking two parameters as input TJ Ryan integer J. So what are the ways in which you could access this particular class
that is packed demo from outside the package. So the first thing that you could do is you could import
the entire package itself. So demo dot star or demo dot asterisk as you could see here,
which says that all the classes within this particular package can be accessed from this particular class. So you have a package demo class defined here,
which says import demo dot star and which would input everything all the classes within demo. So in this case back the more you could see
that pack demo class could be accessed from within package demo class that's there in different package.
So basically this package demo class that you see here is defined in some other package other thing is See
if you want to import just a class and not the entire package. You could do that thing as well.
So here what we are doing is import demo dot pack demo. So we are importing a specific class and not the entire package that could be done as well.
And the third one is instead of importing you can do one thing you can have fully qualified path name or fully qualified name like demo dot pack demo.
That's your class name. You can access it within your class. So basically you would use the fully qualified name
who might use it. You did not do it, but you might use it in case where you're just using it once
within your class, but you would go with this options demo dot pack demo or demo dot star in which you class has multiple is referring
to the class in different package multiple times. So in that case, you just do it once and could be used
within your class. You need not do it again and again, so I create a new class
which is Calm down at Eureka new DOT. So I created a new package. So there is one that is calm down Eddie Rekha,
and the other one that is calm dot ed u de canoe this are different packages you have
if you want to use something within this package Comdata Rekha suppose I want to use Vehicles, right? So this is what I was talking about.
When you try to access it. You can't do it you can access vehicle from here because it's there in a different package.
Now you are not able to access it because you haven't imported it. So what I do here is import calm dot Ed u--
Rica dot vehicle once I do this I am able to access it now. Now what I was saying is
if you are accessing multiple classes from multiple classes within the package like car also you
are accessing so instead of writing it like this. So what you would do is calm down at Eureka the car now the compilation error has gone.
So basically instead of doing this like instead of writing. Multiple times the other option that we have is like calm down at Eureka dot star.
So now if I get rid of this 2 inputs still it would work. I don't need these two inputs
because we have putted all the classes within the package. So it's able to access both the classes vehicles and Ed Eureka from calm dotted Eureka.
The other one was you could get rid of this now it's showing up error. What you could do is calm down Eureka dot vehicle.
You could access it directly with the fully qualified name so you can see the error has gone. So these are the three ways in which you could access
different packages or access classes from different packages. Now, let's understand.
What is the regular expression so regular expression pretty much similar to other languages that you have regular expression is nothing but an expression
through which you could extract some sequence of characters from your string or you could check
whether a specific Fake regular expression or whether a string is aligned to a particular regular expression. So you could Define a regular expression saying
that if for example email ID, right one of the use cases of regular Expressions could be like you have email IDs and you might have seen on websites
where in you do some kind of registration or something and if you don't give a proper syntactic 1 then what it would do is it would give you an error
at the red gmail.com. If you don't give that it would throw you an error showing
that it's not proper. So it's not checking against the Gmail server to see whether your email ID is proper or not.
So it is just checking syntactically. You might have seen it. So if you give a wrong syntactic email ID and
if you go to the next tab, if you tap to the next column or to the next space what it would show is you haven't entered it correctly.
So basically what it's doing is its checking it against a regular expression. It has got a regular expression saying that This is
how an email ID could be it should end with so-and-so characters. It should be at the rate.
It should have at the rate. It should have Gmail or it should have some characters in between then
add followed by.com or something of that sort. So basically to have this syntactic this thing done just to ensure
that you have given it properly you have a regular expression. So yeah, one of the use cases could be to have this pattern Checker usually to see
if you're giving value which aligns to what is expected. So maybe other thing could be
if you're typing something or if you have been asked to put some amount and if you type characters
there it would give you an error saying that it's not allowed those type of checks could be done through regular expression or
if you want some value to start with a particular character and end with a particular character. You can check it against a regular expression
regular expression is a pattern used for searching and manipulating strings. It could be used for manipulating strings as well.
So one of the use cases is Ching and the other one is for manipulating the regular expression either matches the text
or fields to match. So basically it would validate and say whether it's a match or it's feeling
so we call regular expression as Rex which is nothing but abbreviation for regular expression. So Java supports regular expression,
and these are the pluses that we have with in Java, which is in Java dot util package. So remember we spoke about Java dot util package
which has got utility classes. So these are among them. So here you can see a pattern class you
have mature class and you have patterns in tax and tax exception. So basically if you have some exception
if it's not proper, then you would get some exception as well. So we would be taking an example clarify what this is all about.
So here are some matching patterns used in regular expression. So it just about to read through it.
So you have like ABC It means it has to exactly match ABC if the string that you are matching it against has the content
ABC it would match when you put it into square bracket, which is any letter a b or c should match any character.
And again if there's a negation if within the square bracket, if you have this negation Mark, which says that any character except these three characters
should be matched. So if you put D it will match the second case if you put a or b
or c it will match and here it would be from D to Z. If you put anything it will match not just alphabets. It could be anything any character A to Z.
So when you say A to Z, it says that we are giving a sequence of it, right? So we are saying from a it's a range basically
so it understands range as well. So when you say a - Z which means that it is from A to Z here we are saying that
any one letter or digit. Ben sequence so basically you could have uppercase and lowercase as well.
Basically it is saying it should be in sequence. It should end with basically digit at the N dot is some kind of a wild-card character saying
that any one character except line Terminator must be in sequence. This one indicates beginning of line.
So we say that line should begin with so and so you could put some character here and we can say
that if a line begins when Sue and Sue character sequence or whatever regular expression that we have put in then the entire line matches.
The other one is end of the line with check if any character present at the end of the line other thing is word boundary.
We have an example about this so which would give you a clear idea about it, but it's more about it cannot be part of a word here
when we put slash B to the front and the end or to the start and the end of a particular character sequence, which means that it has to be separate word, but it
I'd be part of some other word /b to verify that any character is not present at the word boundary /g to check
that the character is present at the end of the previous match metacharacters. So we have /t instead of writing 029.
You could use metacharacters. /d to define DJ when you want to Define on digit which is
like you can see here negation of 029 set, which is non digit which could be like alphabets peace or anything
which could be grouped as /t / s is a whitespace character who space tabs and stuff like that could be enter for that matter is whitespace character
/s is a non whitespace character which means the actual character rather than white space / W is a word character and / uppercase
W is a non word character you have quantifiers, which is a quantifier defines how often an element can occur. So star, which means
that zero or more times plus which means that it has to occur one or more times question mark is it could be no or just one time you could mention the number
of times you want a particular sequence to occur, which could be put into your curly braces occurs X number of times x
you could put a range within within your curly braces X comma Y which means that it should occur somewhere between X and Y.
So it's a range you have asterisk question mark it tries to find the smallest match. This makes the regular expression stop
at the first match. So as and when it finds the first match it would stop so we will have a quick demo on regular expression.
So here before we start off we Define a pattern here. Basically, then you could see it is basically saying from A to Z and followed by character
one or more character then we have a check here, which is nothing but a string again. Which we are going to check first.
We compile the pattern. This is a regular expression pattern that you have you're going to check against.
So the first thing that we have to do is we have to compile this pattern to see if it's properly done or not.
So first thing is to compile it against patent and if it's correct up to the mark you would get the instance if it's wrong then there would be some kind
of exception thrown then there is a matcher. So once you have the pattern you do pattern matcher and you provide the string against which you want to check
in this case you have happy learning welcome to Eddie break is the string that spin past and when you do see dot find
if this is fine, it would return the result true and if you remember the while construct it would get in only
if this is true, so it's a loop so it would basically I trait through
and you could see here that it's displaying all the characters. I'll quickly run this.
Let's name the class has pattern Checker now, I write a main method now if Remember, we had a pattern there
which was like a to z followed by actress. Then we have string against which you want to check or you could say simple string to check.
So we just put this one. So once you have this string to check now, what we want to do is we want to compile this particular pattern.
So what we do patten.com pile, so one thing to see here is that's the beauty of using the IntelliJ or Eclipse.
So you could see here. This package is already been imported when you use it. Unless you have multiple classes with name patterns,
it would ask you to specifically explicitly mention which one you opt for. But in this case there was just one pattern class.
So it imported it automatically then we give the pattern here. So basically if this pattern is correct, if it's rightly formed syntactically you would get
a pattern here or so. Pat and I believe we have a compiled pattern now now what we do is we take this compile pattern
and I think we have matured here. We get the matcher. No so matcher would check again string.
So you have a string to check which would give you matcher. So here it's asking me which mature do I want I'll select this one
and you see this one getting in put it here. So this is what we have done. We have compiled the pattern and we have provided the argument
against which we have to check as a part of nature. We get the instance of matcher. So everything is in terms of class.
This is what object oriented programming is all about. You have a pattern which is a class. You have a method
within pattern which is compiling your pattern. So this is what object oriented programming is all about. Everything is realized in terms of classes and objects.
This is nothing but the object of pattern class company. So now what I do is matcher Dot Find I just print this one match her dot check.
So basically what we are trying to do here is once the entire string passes through we are trying to get the substring out of it.
So when we get the substring from matcher, you would get the string indexes begin index and you would get matched end as well.
So when we run it, you should get a PP y n so the first character would be trimmed off so you could see first character being trained of each line.
So I'm putting regular expression. We saw that Java dot util don't reg ex gets imported. Then you have patent then you have string to be checked
against the pattern and the sentence which is to be matched are given A 2 Z means any character from A to Z and plus means one or more the sentence check is checked
whether it matches the pattern or not the strings from the sentence which match the pattern are printed. So basically here we could see
that Echizen uppercase and we don't have uppercase here. So that's the reason I dreamed of the first character. So basically if I add H hear you now eh would match as well
and it won't cream H or it won't remove edge. You should be able to see H as well. So yeah,
as you could see here you have H appearing as with previous case. It was getting primed of so this one is
for the word boundary. As I said, if you want to have something like you want to be sure it's a separate word
and it's not a part of any word you could put something like this. So basically what we can do here is
so we can mention here something like special are we and then in so what we are trying to do here is you could see here.
What we are saying is we want to separate word as in we don't want it to be a part of any other word. So here in learning do you have iin?
It didn't pass it because it's word boundary whereas Just a separate word here. It just passed this one.
So if and I remove this one you could have seen two ions. That's what I mean to say. So now what's exception exception handling is nothing
but typically so here you could see two ions when it's / / be it would be just the ones that are complete word instead of matching the word boundary.
Let's talk about what are exceptions and exception is an event which occurs during the execution of a program
that disrupts the normal flow of the program instruction. So to give you an example yesterday, we spoke about arrays,
right if array size is 5 and if you try to access something more than four like if array size is 5 which means
that the index is range from 0 to 4, if you try to access something more than four like if you try to access Sixth Element within the array
it would give you an error saying array index out of bounds or if a particular Their object is assigned null value. And if you're trying to access that method
within that particular object, you would get null pointer exception since it's not instantiated as yet
when you instantiate it that's when the object gets created. But if your reference is pointing to null and if you're trying to access something within that reference
or a class you would get null pointer exception similarly you have like / 0 and stuff like that. It's basically something that is not expected
or something that would disrupt the normal flow of program execution. When an exception occurs the jvm creates an exception object
to identify the type of the exception that has occurred. So basically within itself,
it would create it or the gold would create it so I can create my own custom exception tomorrow. If I am writing an application usually in applications.
That's how we do. We write our own custom exceptions. I can Define my own exception as
well if My application something is not working or something is not running as I would expect it to I would throw an exception
so that within my application it properly logs or it properly prints out in my console saying that this is the exception
that's been triggered due to so-and-so condition. So an exception is often referred to as runtime error. So here we can see divide by zero exception as an example.
Now there are different types of exceptions first one being checked exception the other one being unchecked exception and the third one being error.
So checked exception is something that happens in the compile time. What happens is when you have a checked exception when you
are invoking some method, which is throwing a checked exception and okay. I don't want to get into the catch block
as yet because we haven't covered it. So this is also called as compile-time exception. It means if a method is throwing a checked exception then it
should handle the exception using the try Clash block. It should declare the exception using throws keyword. Otherwise, the program will give
a compilation error whenever there's a checked exception that's been thrown out thrown out in the sense not at the runtime.
There could be methods which is throwing an exception. So whenever there is a checked exception you have to ensure when you give a call to that particular method say
there is a method X which is throwing some checked exception which is throwing some checked exception from your application.
You are trying to access this method X when you try to access this method X you have to ensure that you are taking care of the checked exception
that is thrown from X. If you don't take care of it, it would give you a compilation error
when I say when you don't take care of it. How do you take care of exception? That would be the first question
that would have come to your mind. So when I say you have to take care is nothing but you have to catch it.
You have to handle that exception. So try and catch is a mechanism through Which you handle the exception.
So what you would do is Method call or the call to X you would put it into a try block and the catch block.
You would say that if this particular exception occurs execute this block of code,
that is a Handler for exception X or checked exception that is thrown from method X. So basically you write a Handler for in the catch block saying
that this is what I want to do when this exception occurs. Once you have that when your program runs even if the exception occurs,
it won't stop at that point it would go and check whatever is written and whatever piece of code that you have written and it can continue from there
on it's basically a decision made by the developer whether to carry from their own or whether you want to break it there whenever there's
a checked exception you have to ensure that you handle it either handle it or you say
that I'm not going to handle this. I am just going to throw this off from my method or throw this off from the Well,
I'm executing this method X method X which we spoke about which is throwing checked exception. I can choose not to handle it and just to throw it off
to the calling program wherever the execution initiated from. So if you don't do that, you would get a compilation error unchecked exception
unlike checked exception. It's not mandatory that you catch it's not mandatory
that you handle it. So runtime exception basically it could occur any time and it's not mandatory
that you go and actually handle it. So even if you don't handle it jvm would be okay with it and it won't give you compile time error.
So basically these are nothing but runtime exception so divide by 0 that we spoke about his nothing
but a runtime exception it's not mandatory that you go and actually, you know handle it during the compile time.
You can either handle it or you can leave it as is and during the runtime you would get an exception. So compiler doesn't check for the unchecked exception.
It's done at the runtime now error. These are Conditions that are external to the application and that application usually cannot anticipate
or recover from it. For example, if stack Overflow occurs and error will arise
they are also ignored during the time of compilation. So even the error condition cannot be anticipated during the compilation time.
It's done during runtime. Right? So one of the classic example is the stack Overflow,
we're in see for example, you call the same methods like thousand times not thousand time is too less
for the stack to get overflowed. But suppose you call it like million times you call same method right in that case.
Remember when I said when you give a call to the method it goes to the call stack, right?
We have a call stack. So it would push something it would push state of class or it would push the properties
that's been passed as an argument the class or to the method to the stack. I treatable if you call it like million times,
what would - you would get stack Overflow because basically everything would get into the stack and it would basically, you know,
give you a memory or float or even memory for the Heap memory for that matter if you create lot of classes
if you create a lot of instances of classes, you are Heap memory would get overflowed and you would get out of memory error.
Those are classic examples of error. So what the hierarchy of exception in Java when it comes to classes you have a stable interface
and then you have error and you have exception. So exception is further.
It has got runtime exception. The other ones are the checked exceptions. So ioexception SQL exception.
These are the checked exception. This has to be handled if there's a method which is throwing one
of these checked exceptions. You have to ensure that when you give a call to such methods you handle it
or you throw it from your method to the right side its runtime exception, so it need not be handled as I said.
Divide by zero and stuff like that would be runtime exception even array index out of bounds and Class cast exception arithmetic exception.
Then you have null pointer exception that we spoke about all these exceptions are unchecked exception
and you need not actually handle it during compilation time. So why to use exceptions when we execute a given program will get an exception exception
handling is performed in order to get the output. So basically, why do we handle exception is nothing
but to get the program going rather than breaking it at that point use of exception handling process exceptions
from program components handle exceptions in a uniform manner in large projects. So basically, why do we do it?
So it's done basically to get the process going rather than stopping it at that point and you could handle it the way you want
to that 's the reason you have this exception handlers give you a simple. Example suppose 29 by zero
what I am trying to print here result after dividing 29 by zero. So basically when you run this,
it's not showing up any compile time error. It's compiling fine. Now when I run it
since it's a runtime exception it's compiling fine. But when I run it, let's see if it throws an exception.
So as you could see here it throws arithmetic exception which is divided by 0 and this is happening at the runtime.
That's why it's a runtime exception or unchecked exception when a runtime exception occurs program gets crashed
and control comes out of the program as we saw here when we ran it. It didn't run this part of it.
You could see that it broke out just right from here. So whenever there is an exception
if you haven't handled it program control would come out from there exception handling is done to execute the program without getting an exception.
So handling is done basically to handle the exception whenever the exception occurs do some action it. It's flexibility to the developer to handle
it the way they want to and get it going mainly try catch and finally our keywords for exception handling. So we have a trike at as I said,
there's a try block where in all the suspicious one or wherever the exception you think that there could be exception occurring
could put it into a trike block and in the catch block, you would say that if a particular exception occurs, this is what I want to do.
All right, that's the catch block. The finally block is nothing but no matter the exception is thrown or not.
A finally block is going to execute at the end. So basically finally block is usually used to release resources,
right when you deal with databases and stuff you create connections against database or you create some socket connections and stuff like that.
When you do some socket programming finally would be a place where in once the execution of the method is done
or execution of something is done you say that we can release these resources of rather than Still establishing the connection,
right which picks up some of your resources CPU resources or which takes up memory. So basically it's to release of the memory exception handling.
So let's talk about try try block is nothing but code that could generate so suspicious could as I said that could generate an error is written
into the try block. So the cache block could be more about catching a specific exception
and doing something with it. So you could opt out to just break at that point or do something
with it say for example want to profile an application or you want to have some instrumentation set for the application to see
how much time what are the exceptions that occurring during runtime, right if you want to see
that may be a typical way in which it is done is you write all this exceptions into a database
and we're in later could apply some analytics to see you know, what kind of Errors have occurred in the application you could see that later on so that
one of those cases where a new Let Whenever there is a particular exception you put it into a database you make an entry in the database saying
that this is the exception that was caught in so-and-so time. And you could later on have some analytics to see
how it could be improved how the application could be improved. So finally block is nothing but with a successful
or unsuccessful the block is going to get executed as I said, this is nothing but mostly it's used to release
resources such as connections and stuff like that. Let's see a demo of how we can handle exceptions to give you an example
of try catch us with this was this is a suspicious code which we are running. Maybe you could put this in to try catch block and save
and there is any kind of arithmetic exception that occurs just printing It Out Printing any kind of rhythmatic.
So what we are doing here is nothing but we are catching exception and we are printing it
so everything is in terms of classes again see exception itself. A class so you could click on it control click
and you could see it as a class. This is source code for arithmetic exception. So you could see extends runtime exception which means
arithmetic expression is a runtime exception. So it's a subclass of runtime exception you could see super
and everything being used here whatever we discussed about. So arithmetic exception is nothing but it's a Constructor right and you could see super been called here.
So that's how you could get into exception and you could read through it to understand how the source code is being written
that would give you a good idea about how flexible Java is it's very much when it comes to flexibility
and when it comes to object-oriented Paradigm, it's very much cleanly followed code so you could go
and actually see at the the way the programs are being written. If you remember when you'd we did 29 by 0 the program bro,
it came right from there and it didn't execute the following code. Now we have Handled it.
We are saying that whenever there is an arithmetic expression in this piece of code.
That's here. We are saying that whenever there is an arithmetic exception.
We are going to just print it and we are going to continue it. Don't stop at that point. So you could see here
the program didn't break right it give you an opportunity for you to actually handle it and it didn't break it continued.
So you could see this statements been executed as well. That's why you have this results. So we had printed here.
So as a part of handling exception handling, what we did is we just printed out saying that what kind of exception had occurred.
So exception dot get message that gave like divide by zero this divided by 0 that you see here is nothing
but is printed out by this message. So it give you an opportunity to handle it and get the program running
as is so try catch finally block. Basically. What I can do here is
let me Define integer denominator is equal to So as I said finally block is going to execute no matter of pot is going to get executed.
So I just print the message here saying I will say here. I'll put denominator when the denominator is 0 we would get exception
and it would come to the exception block here or the catch block here and it would print it
and then it would come to the finally block. So let me run this and see so as you could see here, it came to the exception Handler it caught arithmetic expression
that is divided by 0 and then we printed out saying that printing the typical message or the actual exception that we got and it came to the finally block here.
Let me put a scenario which is not going to throw exception in this case. My denominator is 1
which means 29 by one we shouldn't throw any exception. So what I want to show is still it would execute the finally block
so you could see here 29 been printed out which this got executed successfully this time because the denominator is not
0 it's one it got successfully executed still the finally block is been executed why to use multiple Catch blocks so you could have multiple exceptions
and you could write multiple catch blocks. There are different exceptions that we spoke about it could be like a pointer exception as well which you want to handle right?
So it depends upon your program logic or what you are trying to do here. So there could be null pointer exception then you have finally
so you have multiple catch blocks here. So you usually don't handle null pointer exception but this is just to give you an example.
So basically you have this arithmetic exception here been handled then you have null pointer exception being handled here
then you have finally so if you want to have different exceptions to be handled within your application,
you could have multiple cache blocks since all the exceptions are derived from exception catch exception. He should be placed at the end.
So basically if you put the exception this exception, right if you remember the hierarchy that we spoke about all the exceptions are derived
out of exception class so you could have something of this sort. So if it is
not an arithmetic expression not a null pointer exception then is of course going to be some kind of exception which is going to be handled in this exception block.
So basically we are catching all the exceptions here first is specific to edit mattock exception. The second one is null pointer exception
if it's not a null pointer exception or arithmetic exception then this particular cache block is bound to get executed
and it has to be last you cannot place it first because if you place it first this two blocks exception Handler blocks will never get executed
because even this two exceptions are a subtype of exception class itself. So it has to be it is mandatory that you place it at the end
or else it would be be compiled time exception so you could have something like array index out of bounds and stuff.
So just to give you this is integer array. I put one two three I Define an array now I say integer array of Three elements right
and if I try to access or just choose so when there are three elements the index ranges from 0 to 2. So now I put three here.
So this is generic exception Handler for all the exceptions. We would have this Handler when you run it. This is going to give you a rain dicks out of bound
because you are trying to access element at index 3 which doesn't exist. So you would see a generic exception
and so it came here. What I was trying to say is so you could have arraigned X Out of bound here, right?
So instead of null pointer exception now, I have arranged index out of bounds now, it would be handled by this block
and it wouldn't come to the generic exception Handler. So here you saw it came to the generic exception Handler. Now what you would get is array index
out of bounds exception Handler because it's been handled by this particular cache block and not the last catch block.
So this one is as you go down it becomes generate. So here you could see it's been handled by array index
out of bounds exception as you go down. It becomes generic more. Generally it is multiple catch block.
So this is pretty much similar. We have re we have arithmetic exception that occurred array has four elements 023
and you are trying to access the 10th element. That's when you would get array index out of bounds exception as we saw there could be nested try the rain.
You could have try and inside try. So basically when you have prai inside try if there's an exception that occurs in the nested one
or the innermost one, it would try to see if there's a catch Handler for that particular exception innermost one.
If it doesn't find then it goes to the outer one. So in many cases it may happen that a part of the block may cause an error
and the entire block may cause another such cases. You're going s to try block. So what I was trying to say is you have this one.
We have one more like exception or shouldn't be done. So you have a nested one within this try you have one more wire. This thing with says array index which is handling
null pointer exception sending just the null pointer exception. It's not handling array index out of bounds. So what I was trying to say is if such scenario occurs,
it would check the innermost one if it finds the exception Handler, it would execute it.
If it doesn't find then it would come to the outer one. So basically though this try doesn't have Handler for arraigned X Out of pound still it would be taken care of
so you can see here. It came to the outer one. So if you would have some piece of code right after this,
it won't get executed. So this piece of this code will not execute if inner try so basically
if there's a reception which occurs in the inner block and if it's not handled it would come to the outer block but this piece of code whatever you have in between this catch
and this catch would At x equal to Y to use throw keyword the Java through keyword is used to explicitly to throw an exception
while executing the program. So if you want to throw something as I said, you could create your own custom exception and
if you want to throw something you could throw it out you could throw an exception from your program. It can be used to throw checked or unchecked exception any kind
of exception could be thrown using throws up using through and the Java through keyword is used inside a method so you could see here.
Basically if B is equal to 0 you throw new exception / 0 causes an exception. So before it goes to actually dividing it by a by B,
you are kind of handling it prior to that you are checking whether denominator is 0 if the denominator is 0 you're throwing Exception by yourself, you are creating
a new exception. This is kind of your own exception, right you are you're creating your own exception and saying
that it's divided by 0 so for any condition so basically Denominator is equal to equal to 0 you could throw it from you're saying or denominator.
So basically if I keep this denominator has zero and this should be prior to this just cut this and this should be done prior
to this event to use throws throw this something as I said, when you have a checked exception, you can either handle it using prai catch
or you have to throw it out of the method saying that I am not going to handle this let the collar handle it you could do
that using throws keyword. The method is not taking the responsibility to handle a checked exception and it is,
you know, asking the collar of the method to actually handle it. This is in terms of just checked exception
when you have a checked exception. So basically since you're throwing it from there, but since you have Handler here is coming to this
so you have this denominator you through an exception from here, but this exception is been handled here, so it came here saying That generic exception Handler.
All right. So if I remove this that's the reason you have generic exception Handler here.
So I remove this Handler I'm not going to handle exceptions whenever you throw an exception. It has to be handled
since exception is a checked exception. So basically you could have run time exception which need not be handled.
So basically the Handler that you see here, we just have two handlers which is for arithmetic exception and array index
out of bounds exception, but we don't have a Handler for runtime exception. So that's the reason it gave you an error
saying denominator is 0 so, this is an exception that we through here using throws. So it's giving you an error right here
because this is a checked exception. The exception itself is is a checked exception. Now, you could either handle it
like the way we had handled it here. You could write catch exception and you could handle the way you want to so you have two options now
when you get into this scenario into this situation, You have two ways to handle it either right exception and handle it.
This would be a Handler. If you see the compilation error has gone or this is one thing that you could do or the other thing
that you could do is you could ask this method this method can say I'm not going to handle it. I'm going to throw it for my collar to handle it.
So the main method is saying I am not going to handle it. I'm just throwing it off from my side from my code or the caller to handle it.
So see the exception has again the error has gone. So basically Whenever there is a checked exception you have to option
and this is happening at the compile time, by the way, so you have to ensure that you either handle it or you throw it throw
in the sense throws its through throws me anything that I am not going to handle this at the collar handle him as you could see
the throws keyword was added in the method signature. We saw how we Throw exception. We throw exception from here you check
for some condition you throw exception from Why uvu's throws throw is basically to throw an exception and a method saying
that I am not going to handle the exception. So what's the difference between through and throws the throw keyword is used to explicitly throw an exception.
The throws keyword is used to declare an exception. The through keyword is followed by an instance. The throws keyword is followed by throwable class.
Alright, so here you could see this is throws keyword is actually by instance you create an instance of exception.
Whereas throws is the class itself through keyword is used within a method and throws keyword is used with the method signature.
So you could see through is used within a method within the method body and you could see throws at the signature level.
So you have throws and throw so throw is nothing but whenever you want to check whether there is an exception and you want to throw it out
you want to actually trigger the exception that's when you would right through and you would This exception. So basically here we are checking for a condition
if denominator is equal to zero, which is potentially which would cause error further down the line.
So we are checking it up front saying that if the denominator is 0 then we are not passing it further write in such scenarios.
We could throw the exception right away. That's how you figure the exception from the program Rose is nothing but it's at the signature level
of the method that you could see and what you mention out by throws this
that main is saying here that I'm not going to handle the exception and just going to throw it off.
So this are unchecked exceptions, by the way. So whatever is not been handled here if there's some exception handled here it would get
to the exception Handler or the catch block. But if there is something that is not been handled here would be thrown
out of this main method main method won't handle it. That's the reason why we have throws through keyword is used within the method
and throws keyword is used with the method signature. Through keyword can throw only one exception whereas throws keyword can be used to declare
multiple exception. Basically, you could have something like this one exception say ioexception
so you could have multiple exceptions here. So the main is saying I'm not going to handle ioexception. I'm not going to handle exception or something of that
so you could have multiple exceptions after throws user-defined exceptions. You can create your own exception and it is called
as user-defined exception or custom exceptions user-defined exception class can be created by creating a class child class of exception.
So basically create exception. I create a clasp which is SE custom exception. Now, this custom exception is extending exception
so you could have your own classes defined and it would have like this. So what you exception
you get you go you could pass that exception directly here. So this is See, what I'm trying to do here is overloading of Constructor.
So you could see if you are passing string. It would come to this. If you are passing exception to come to this Constructor.
So you could create your own exception here. So basically to give you example of this instead of exception. What I throw is I can have a new custom exception.
So initially I was throwing exception. Now what I am saying is new custom exception. This is the exception that I have created.
It's my own exception when I run this now, you can see that it should throw custom exception so you can see here exception
in thread main thread main is nothing but your main method where your program is executing and it shows the exception type which is custom exception which is created by me
and I have passed the strings in the denominator is 0 and at what point or at which line this particular exception occurred can see it
in the stack trace. This is a stack Trace basically. Whatever you get here
when an exception occurs is nothing but stack Trace. So hi everyone, welcome to module number four before I start off L.
Just briefed on what we covered in module number 3, so we covered on Hoops Concepts in general. What are the Hoops Concepts
what are different concepts or terminologies that we have in oops? Then we saw how Java is aligned to those Hoops Concepts
how Java is known as object oriented program, right or how Java is aligned to object oriented programming Paradigm.
Then we spoke about abstract classes, which is a different modifier all together, right and then we spoke about a hundred percent abstraction,
which is nothing but interface which is a construct in Java which helps you to specify something and you could have different implementations as
per the specifications then we spoke about exception, right? What are different exceptions
and why do we have exceptions at all? And how do we catch exceptions or what is the need to catch exception
and what are different types of exceptions right? Have like checked unchecked exceptions checked exception has to be caught by your application
or a particular method my top to just throw it off rather than actually handling it through the throws keyword, right? We touch base on regular expression as well.
And why do we need regular expression and what are different classes that Java has to support regular expression?
Alright. So today we would be covering file handling and Java Collections framework.
All right. So file handling is nothing but you have external files you might have something
on your network or it could be on your local machine itself and you want to access it
and do something on it or you might want to have data that's been coming to your program.
You might want to write it into a file so that you could kind of analyze it later. I spoke about exception right?
If you get an exception, you could write it into a file so that you could later on go through it
and understand at what time a particular exception occurred and you could act upon it. It's a kind of profiling
and instrumentation turn on application to understand more on the behavior of the application and to improvise on it.
Such activities could be done using file handling will talk about Java Collections framework. Java Collections framework is nothing but you have
a re array list and everything. That is we spoke about arrays in the previous topics. But Java Collections framework itself exposes array list,
which is a dynamic list. You don't have to manage it. So remember in list we said
that when you take Claire a list or declare an array, you have to mention the size of it whereas array list is dynamic.
You don't have to mention it. It keeps growing. So these are the topics
that we are going to cover file input output of operation in Java wrapper classes in Java. We are going to talk about Java collection Frameworks.
We are going to talk about list and its classification in Java Q in Java and sets and their classification in Java. So as you could relate all this Action all this components
within the collection Frameworks are nothing but data structures in general. That's you straight.
So maybe in see you might have used different data structure and whereas in different languages, you have different data structures, right?
This is just to handle data data structures are nothing but to store data efficiently and you could select one of them based on the use cases
that you're handling within your application. So let's start with file I/O file I/ O is nothing
but you have a file on your disk and if you want to do something if you want to write to that or if you want to read from that file,
you would ideally use file I/O API. All right. So file I/O is used to process the input
and produce output for a specific file. All right, so you could access some file within your local disk do something
and you can write it on to a different file as well. Write Java dot IO package contains all the classes required for input
and output operation for a Phi. All right, so As we said package is nothing but namespacing right?
So everything is grouped. All the I/O classes are all the classes in Java that is handling.
IO is grouped into Java dot IO package. Okay, that makes it easier for programmers to understand as well.
Right? So if you're doing a lot of I/O operations, you could directly say import Java dot IO dot star
which would in put all the classes within your Java dot IO package. All right, the files can be text file or it
could be binary file, right so you could imagine not always you deal with a text file, right you have other files as well.
So nowadays. There's a lot of things going on on iot right internet of things.
So you get data from different systems. It could be like your mobile phones. It could be from the car sensors or elsewhere, right?
You have a real-time raw information coming in into your system for analytics. So such data cannot be a text file.
Txt file as such is a heavy file, right so such iot applications. What is been passed between systems our intercommunication
between systems is done through a binary file, which is kind of lightweight right compared to text file txt files are heavier.
So you could imagine this files not being just the text file. It could be binary files and so on when I say binary files, it could be it could be images
as well write different images that could be shared among system or some image that is there on your local disk,
which you want to read into Java and do something on it some kind of graphical processing on it right stream is a sequence of data.
So you convert this file into a stream and you use it with in Java. Okay, we'll look at example,
which would give you a clear idea of what I'm talking about. Okay, so here you can see an example where in you have a file
which is student dot txt into your local system into your local disk where you are running this program right then you
have a pretty Traitor this is nothing but a printwriter is a class that is exposed by Java
which is there in Java dot IO package you would pass on this file. So initially you have new file
and you pass on the path of this file that you are going to read or do operations on not just read it could be right operations as well.
So basically you have new file and you specify the path name or initialize the file. The next thing that you do is you have printwriter wherein
you pass this file. All right. Now what we are trying to do here is we are trying to write
into a Phi, right? We are trying to write the name or the some ID or something. We're trying to write this content into a Phi right
into your local file will write a program on this which would give you a clear idea and the next step that we are doing here is kind of we are reading it from a Phi.
Alright. So the first step that you do is write it into a file The Next Step
that you do is read from the same file and print the contents of it. All right, so it's not mandatory
that you have to specify the same file for reading right? This is for just for the convenience or just to demo it.
We are using the same file you could have different file as well. All right, so let me show you how this is done.
So again, I create a new project for today, which is All right, so we create a new project and Eureka.
module 4 Alright, so we have this project now. Let's create a new class. Calm down daddy.
Rekha is nothing but the package name and I give up. input output demo Alright,
so before we start off I can do one thing. I can create a folder here. Say said, Eureka.
All right, so we'll put all the files here. So this is the empty folder that I have created right now or let me put it here.
So for Simplicity, I'm going to put it on C drive itself. Alright, so on see have created a director IO directory
where in we are going to dump all the files, right? Whatever we are going to do is going to come into this particular directory.
Alright. So the first thing that we are going to do is so now we are thinking of writing
into a file first right? So I create a main method right? The first thing that I do is create a file.
All right, and I create a new file. Which is nothing, but I am calling the Constructor of a class of the class file,
which is with in Java. Right? It's not something that we are writing.
So Java itself has this internal file class, which is file. All right.
So now a point to this particular directory. All right, calm down Ed Eureka IO and we say so I call it as employee info dot txt.
Alright, so we are going to write into this file now from java program. So now it's showing up an error saying
that which file I want to refer to as we said the all the I/O related files all the all the I/O related classes are placed
in Java dot IO package. So I select this package once selected you can see an import statement here.
Being up. So why do we have input statement because file is a class
which resides in a different package then calm down Eureka had it been within calm down Eureka. You need not import it it's outside.
So you have to put it right then there are different ways in which you could write it, but now we'll follow the same example.
So I write printwriter printwriter. Is there within Java dot IO and you could see it imported here.
All right employ info writers if example, okay now we create a new printwriter and if you see the Constructor of printwriter,
so we spoke about Constructor overloading and stuff like that. Right? So you could see if you go into the source code.
If you want to Deep dive more you could go into the source code and see it takes file. Alright, so here you could see an option
which is nothing but it consumes file. So the file which we created above. I can put it as e employ info file.
All right. So there's a file that's created here. So I put the same file here right
since the Constructor supports file itself. Now this is checked exception. All right, you could see here.
It is saying Java is explicitly saying that you are not handling this exception. All right.
So ideally the better practice is we shouldn't be writing everything into the main because it would become cumbersome to understand
later on right you shouldn't be dumping everything to the main method. So as a good practice,
what you should do is you should create a new method right to file. All right.
So this is best practices, right? It's not mandatory. Java is not going to tell you there is an error or something.
But as a part of best practices, this is how you should write programs which would make it readable for others
because tomorrow some other developer is going to handle this particular class. It's not that you have going to be there
throughout right so basically so that other developers can easily read It and maintain it.
Well, this is how we write it. I move this content. Whatever the writing part is.
I move this content here. Alright, so now it's showing up an error saying that it's not been handled right file not found exception
is not been handled because it's a checked exception. So remember in the checked exception we said that there are two options one is to handle it
within your method all this right to file method can hop to throw it off saying that I am not going to handle it let the caller handle it.
So when I say caller caller is nothing but this method alright, so I am going to create say here input demo. Sam trading in instance here.
Alright and demoed right to file and I'm going to pass the file that we created here. All right, so there was one file that we created here
which is pointing to an employee info Dot txt. And we are calling this method to write it if you think about the reusability part of it.
So this is how you make the method reusable. If you put it into Maine you cannot reuse it. If you're taking the right logic outside the main and refactoring
and putting all the code that is specific to writing to a file do particular method. You could reuse this method you're making
it generic right now. I opted to throw it off or let's handle it. All right when I say let's handle it.
It's nothing but you have try catch block. All right, so I say Catch so what exception was that? It was filenotfoundexception.
So you need to have file already in place. Alright, so here I print saying that given file was not found on the local disk.
All right, and once that file Maybe we can print the name of the file as well. Alright, so here we have chosen to handle it.
Okay, so we remove this. Alright, so we have a try catch block written now and we say that Okay,
so we are handling this error. So we say that it just print a message saying that given file was not found on the local disk.
And as a good practice, I don't want further processing right if I don't have a file I don't want to process it further.
So I say through runtime exception and I so runtime exception is unchecked exception. It won't give you error even if you don't handle it,
right whereas this was a checked exception. That's why you are getting error. Basically.
What I'm doing is I'm catching the checked exception and wrapping it into unchecked exception. All right, because I don't want this to be processed further.
So once we have this printwriter set, so I'll have to take this print right outside or I'll get here.
Alright, so what I do is employ Dot println and I write some content to it and say in this case I am writing vinod.
I write some ID to it as well. Anything you could write. Okay one side right into the file.
I have to ensure that I close the file. So basically you could have finally block so you would do this.
I'll have to take so this if this is within try so it's not accessible within the finally block.
So to make it accessible what you will have to do is you'll have to take it outside. All right.
So I Define it outside Dry block so that it gets accessed in finally. Alright, so now
what I do is employ info dot writer Dot close I close it. So that's one of the use cases of finally as I said,
it's basically for closing the resources. So this finally would execute even if this doesn't throw
or don't throw any exception and it would get executed even if it throws an exception. All right, so
that's what the use of finally block is. So let me quickly run this program to see I have to create a file here.
If I create a text file here Dot txt. All right before I create a file maybe I will I would want to show you what happens
if we don't have a file, right? So if you don't have a file I run it so we don't have anything in to see at Eureka
I oh, all right. Basically, you're trying to write into a file which doesn't exist at all.
So it's building. And it's running now. Even if you don't have a file,
it doesn't throw file not found exception rather. It creates one on its own. So remember we didn't have anything here.
Now. Let's see the content of this file. You could see whatever we rode right the employee name
and you could see the employee ID, which is hundred. So whatever you wrote here came into that file.
All right. Now, let's write a program to scan or read through this file.
All right, so maybe I can use the same file or I can use something like All right. So I write something like BMW cities howdy.
All right, I I write something like this. It's a vehicle info and say you have something like let's keep it
as is okay. So you have vehicle info in here. So now we are writing a program to read from a file.
All right, so we pass on the file parameter here. There are different ways in which you can do. All right, so we create scanner now scanner takes
if you see instructor you could see that scanner takes file as a parameter. All right.
So I put scanner file again. It is saying that file not found exception is to be handled.
So in this case, you have to be sure the file is present, right, since you are reading the file the earlier case
it was writing to the file which can create a file but reading to the file you have to be sure
that the file is present or else you would get a file not found exception. So in this case, I choose to throw the exception.
The read file is saying that I don't want to handle this exception i instead threw it off.
All right, you could see throws here. Okay. Now what we do is we read lines from it, right?
So we have like Okay, so we read line from it and we just print it out. basically, you would have something like So let
me just print line read from the input file, right? So this is nothing but we are just printing whatever we read from there.
Basically, this should have something like it should Loop in until it's end of the file. Alright, so this is
how you use the while loop similar to what you do in C and C++ right till the end of the file till yo F character
is encountered you kind of read the Lines within the file. All right. So this is a method
that we have written but we haven't invoked this method yet. So I create file since it's a different file we have to create
a file vehicle info is equal to new file. We give the path of it, which is nothing but a Eureka IO vehicle info dot txt.
All right, we created a file here. Now, what we are going to do is we are going to call read file on this file
or vehicle info file and we should be able to see the contents of it. All right.
So now one thing here is since you have thrown exception it's saying that exception has to be handled here.
All right, I can choose to not handle the exception here as well and I am adding it to the method signature. Sure, so main would throw as well.
So basically what would happen is if you don't have a file which is being read your program would stop at that point
because you're not handling it anywhere, right? You're just throwing it off up the ladder. So basically at the end
if you don't have a file you're going to get an exception your program is going to stop there. I run this file and let's see what we get.
All right, so you could see BMW Mercedes and Audi being read out from vehicle info. This was the content that we had within the file
and which you could see it's been bred. So file writer and file reader so similar to print this one is a different way of writing it.
So what's written within this program is nothing but we create an instance of file writer and we pass the string the path of the file rather as an input
to the file writer now file writer is nothing but it's character-oriented right print was not as such character oriented.
This one is character oriented which means two bites right character is nothing but two bite-sized with in Java.
So this one is not like it doesn't work at the byte level. Basically, whenever
you're dealing with character-oriented file, you could use this one rather than printwriter. All right, objectify lighter is being created
which is a character oriented file or which is a character oriented place. All right, and you write to a file you're writing
to the file here. All right, and similarly when you use file reader, it's a character oriented reader reads character one character
at a time from the file that you have mentioned here. So maybe I will take just an example of writing it because it's going to be pretty much similar.
So quickly write this program. You have a main method you create a method to say you have public or it could be a private method
as I said, the best practice would be to restrict as much as possible rate.
If you don't want this method to be called from outside, you could have private method so it's going to be right to file.
So you need to give string or you can have file as well. Whatever you prefer to would have file and file to write to okay.
So basically we were talking about file right? All right, so I create file right to object. Okay, and again say it's a bike info.
And I pause this file to which you have to write. All right. So again, you could see now you could see a checked exception
that is nothing but issuing that ioexception has to be handled now I choose to throw it off. I'm not handling it here.
Bike in four thought I write to the file and I write some content. Basically, this should be writer just to make it more elaborate.
All right, I say right Road close close this particular file. Okay.
Now I give a call to this method which is nothing but file writer demo is equal to Nu we are creating instance of it.
Okay, let me create a file which is nothing but file bike info file and which is new file and within the same directory,
which is nothing but calm dot Ed u-- record at I/O. I create by info Dot txt.
Alright, so what we are doing here is using file writer. We are going to write into biking for DOT txt. And we are going to write this content
that is Ducati and maybe after this we could have whatever you have in buffer that that would be flushed off to or disc.
Alright, so when I run this basically we don't have a file now bike info and I think it should create it so you could see here.
We don't have a bike info file. Okay. So again, let me talk about this program.
So what we have written is we have a file. So what we are trying to do is we are earlier the program we wrote using printwriter.
Now we are going to use five writer which is a character stream based I/ O so we are going to write into bike info dot txt file
and we have a method right to file in which we are creating a file writer object which is taking up this parameter
or taking this pile, which we are going to read through as the input. All right and not read through its rather, right?
So the file to which we are going to write is taken as an input parameter to the file writer and we are writing into this file.
So right now we are just writing to cart either. All right. So I invoke this method like file writer demo dot right
to file and I pass on this file here. All right shows up an error that you have to handle iOS. Option which is been thrown from right to file.
So I choose not to handle it here as well. Which means if you don't have that file or if there's some ioexception
that's been triggered you would program would stop. All right, so I run this program. So basically, it should create file
and should write to it as well. All right, so I go to the directory. So you see bike info file has been created here.
We didn't have it earlier. It got created after we ran this program now, I just open it up
and you should see the value Ducati been written there. All right, so that's about file writer and similar to that.
You have to just read through it. You have to pass the file that you want to read as a parameter
and you just have while loop to iterate through till the end of the file and you can print the character. So basically it's pretty much similar to what we did
for the printwriter, but I just wanted to demo for one of these cases so we took an example of why writer.
Alright, so now let's move towards stream stream based I /O so Java streams are used to perform input and output operations on eight bits.
A bite right screams is basically operating on a bite input stream. It is used to read the data
from The Source it could be file it could be keyboard or it could be anywhere across across the network, right?
It could be a socket as well. So basically it is used to read the data from the source that is input stream and output stream is nothing but
if you want to write something from your program to any resource on a network, that would be nothing but write
that would be nothing but output stream. All right. So with output stream you would typically write data
to a destination and with input stream. You're going to read it from a source. All right, just to walk you through so we have object
as I said all classes or every class in Java is inherited from a class. That is object.
All right. This is a super class for all the objects all the classes in Java.
Okay. So you have object and you you could see that this input stream and output stream.
So let's talk about the input stream. There's file input. Beam, there's byte array input stream this filter input stream
and this object input stream. So we'll talk about this in the coming slides. Why do we have object input stream
and stuff like that. But right now you could imagine that we have multiple classes or you could think
that there are multiple classes with it input stream, which are used for different use cases. Like you have buffered input stream,
which is nothing but it buffers it. It doesn't read or write it in one go. It's sort of buffers it and then it flushes it over.
All right output stream similar to input stream. We have an output stream. It's an interface.
I believe which has got several implementations like file output stream. You have byte array output stream.
You have filter output stream. You have object output stream and filter output stream is further categorized as
or has further sub classes as buffered output stream and data output stream. All right, so in further slides,
we are going to discuss file input Stream file output stream object input stream and object output stream.
Okay, so file output stream class and file input stream class. Let's talk about it.
The file output stream class is used to write to a file right you you could take a bite array and you could write to a Phi.
All right, so you could take something. If you have a string you could convert it into a byte array and you could pass it
and it would write to a file. So remember we said it's a byte oriented, right? So basically it's going to write take array as input
and it's going to write to it write to the file that you have mentioned in there. Right so you could take integer as well.
It is used to write the specified bite to the file output stream. So you could provide integer as well.
There's a close method which is nothing but closing the Stream So streams are nothing but it is hooked up to the resources.
It is taking up resources and there is a Action between your program and the file established rate when you create three times.
So when you close it you close that connection and it is free for garbage collector to remove that particular object from memory.
So basically freeze memory as well right unless you close it. It's going to stay in the memory.
So it's a good practice to close it right after you use it. Okay rights, you could write to a particular offset,
which could be done using right method. So right length bites, whatever length you have mentioned in as third argument,
it would write it to a particular offset. It would write the bite that you have even or bite that you have passed in as input.
It would write that bite do a particular Offset you have flush method, which is nothing but flushes the output stream
and forces any buffered output bytes to be written out. All right, so it could be buffered as I said, so not writing it each
and every time You have bite basically it buffers it and you could have flush method to flush it off to the Phi. So basically we have buffered stream,
which is this Method All right. So here we have file output stream and using file output stream.
We rewrite it to the file and then we close the file. All right, as far as the syntax is concerned. It's pretty much similar to file writer example
that we took but just to give you a demo just to show you how it is. We'll have fun demo done here.
All right, so I create a new class which is nothing but file output stream demo. Alright, so we have a main method
and I create a new method which is like file write to a file. Which could be like private method
private void write to a file? Alright, so we are going to write to this file using what using file output Stream So it showing up this
so I select Java dot IO dot fight. All right. Okay now I create file output stream.
Create an instance of it right similar to how we did for other programs. Now, it says file not found exception has to be handled.
I choose not to handle it and I throw it back to the calling program. All right.
So now what I do is I do file dot right file output stream. I am going to write it to this file. So I just write a text here.
All right. So write a text here. Hello folks.
Hope you're enjoying the session and I just closed it off again it throws ioexception when you close it. So remember we said there could be multiple exceptions
that could be thrown out of a method. So I again I choose to throw this one as well. So basically what I'm trying to do here is I throw
IO exception as well. Okay, so it doesn't take a string as a as an input. So what I have to do is I have to do not get bites.
As we saw the Constructor of it constructed isn't takes string as input in case of output stream or in case of file output stream.
It interacts in terms of byte array and stuff like that. So I converted this string into byte array.
So when you see get bites get bites method is a method on a string class which converts the string into array of bytes.
All right. So now what I do is I give a call to this particular. I'm writing to a file
and I'm going to take create a new file. So I can take the same file, right? I am going to take a b or else I will create a new one.
All right, so I create a file which is like message dot txt or you need not even create it. It will create by its own.
I will delete it may be okay on the thing is I love to create see a Eureka yo. All right.
And here we say message dot txt. All right, so I call a method to write to the file using file output stream night choose to throw it off
from here as well. Alright, so we have a method which is going to write into a file using file output stream.
All right, so I run this program. Okay, it ran successfully. Let me open this message and see
if we have the message written there. All right, so you could see here. Hello folks.
Hope you're enjoying the session which is written from your program. All right, so this file input stream as well,
which is pretty much similar it again operates in terms of bytes. As you could see the signatures of the methods within it
would read the contents of the file into the byte array that you have passed as a parameter. The second one is to read
from a particular offset the length that you have mentioned or passed as a parameter close is pretty much similar.
It would close the connection that's been established and it would make the class available
for garbage collector to clean it off. Okay garbage collector is nothing but unlike see where in you have to delegate the memory location
that's been used garbage collector is parallel thread that is run by jvm to remove the or to free up the memory space that's been acquired by classes.
So it runs sporadically it's not within your control. It runs per radically and it clears of the memory locations. We have a reed method which is nothing
but reads the next bite from the input source. So on the file input stream instance on which you read it, it would read out the next bite and if it's end of the stream,
it would return -1. So the return value is integer as you could see here you could skip specified number
of bytes from the input stream and available Returns the number of bytes that can be read from the currents tree,
right? So maximum number of bytes that could be read out. So I'm just going to read through this light
or if you want. Yeah, I can take an example maybe since we didn't take example on reader.
I'll take one example and reader as well. Okay, so here I to file input stream demo All right, so we create a class here
and I have a main method here. Okay. I have a private method
which is void and it's not returning anything and I said read from file. All right.
which takes file Again, I'll have to import file. Okay, so Java dot you could see the input statement coming up here.
So now I create file input stream. Okay. I create a new instance of it.
Which would typically take for that matter it would take string as well. Just to show you a different variant of it.
It's not that you have to always pass file it you could pass string as well. Alright, so we are passing a string here.
It says file not found exception has to be handled since its read you have to ensure that you have the file there.
Right? So I choose to again throw off this exception. So I'm not handling it.
So typically you would handle exception when you want to do something on it. Right when file not found
if you're trying to read a file and that file doesn't exist in your drive. I wouldn't handle it.
I would just throw it off. I want program to break right there. Right?
I don't want it to proceed because our program at this particular method is trying to read from a file
and if it doesn't exist it, it doesn't make sense to proceed further. All right, so I have input stream.
Now what I do is not read and as you could see it's written in integer, right so This has to be looped.
All right. This has to be looped until you get minus 1 right. So basically you would have like say teacher and you have like
so this is integer read from file. All right, and I keep this as integer, which is so whatever you read I need to assign this because I
want to write that as well. All right, so I'm assigning to this and unless this is minus 1 you are going
to read through write it again shows exception, which I need to handle. So this time it's ioexception
and I'm going to throw this one as well. All right, so you have a reed method you are going to read it and basically till the time its minus 1 it's going
to be red right now. What I'm going to do is within the loop. I'm going to just type.
So basically I'm going to just type cuz this to character. It's read as integer. So you have to convert it into character.
If you want to see in terms of character. If or else you would get the ASCII value of it. Alright, once this is done.
Basically I need to close off the stream as well. Okay. Now what I do is I create the instance
of file output stream demo. Just nothing, but creating instance of it. Right demo dot read from file.
But remember this time we are not creating a file instance. We are just passing the string. All right, so you could take any of this file
since we are reading it we could take like let me take bike in for it just got valued Ducati. So I'm passing this as a string this time.
All right, and it's a different variant. You could pass it as a file as well. You could pass it as a string as well.
But since I have been passing as a file in previous examples this time I choose to pass it as a string. Alright, so now I am throwing it off
from here as well since I don't want to handle it right. So if I get an error, I want the program to break. So that's the reason I am throwing it off
from here as well. So coordinator, let's see. What's it?
Okay, so I have to put double slash here. All right. I miss that one.
So basically if you don't put double slash it would take it as a regular expression sort of again. It's not bike in Foods dot txt,
which I missed I believe so it says that bike info file doesn't exist. Right since it's a text file, right?
You have to provide the extension as well. All right, so you could see it read in terms of characters, right?
So you could see the first character read as D then then you could see it followed till till it red Ducati. All right, so that's about file stream
or file input stream. That's how you write programs. Now what serialization
and deserialization I will quickly cover on this one since this is something that's been used widely in big data when it comes
to Big Data or when it comes to distributed computing. Just not to if you understand it's good. If you don't understand you don't have to think much
but I'm just talking in terms of distributed computing. Right? So when it comes to Big Data,
it's not that you have server farms and everything. Your program doesn't run on server Farm anymore. It's more about you have commodity Hardware
on which a program is run and that's what Big Data Frameworks are good about the investments in terms of infrastructure
by having server Farms has been reduced a lot has been cut down a lot and you could have commodity Hardware
on which you could have this distributed Frameworks running. So say for example, you might have heard about Hadoop or Apache spark,
which could actually run on normal Hardware right or you could Need to on cloud as well. So you have like Amazon web service
which is cloud like you you have Google as well Google services, which exposes as a cloud you have a seizure,
which is nothing but Microsoft's Cloud. So all these are kind of not big Hardware not server Farms that you have.
It's a normal Hardware that you could configure and you could run through your chunks of data or petabytes of data
that comes in from cloud or from elsewhere, right? So that's the beauty of it. That's the beauty of java and one
of the main things are main feature that Java has got a serialization and deserialization
which helps in having this distributed framework going. Okay. So serialization is nothing but you have class
right and you you create instance of a class, which is nothing but object right now this instance of a class
if you want to pass it over to some other computer or some other computer on your network, right?
This could be done just because this Serialization there's a concept of serialization right suppose.
I create some object of a class today on my computer. And if I want to pass the same state, right it's about passing the street
not the behavior behavior is of course just methods, right? So it's about passing the state of the object. So whatever state my object is
or whatever properties my object bolts at some particular point if I want to pass it over to some other computer
on the network. This could be done just because you have serialization was
what you would do is basically if you remember the object stays in Heap right Heap memory, which is protected
which no other process can get into that particular. He process Heap memory, right? That's a security that Java provides
if you remember in the first slide we covered it, right. So what Java does by serialization is whatever contains of a particular object
or whatever contains a particular object whole set any could be written. To your disk as a binary file
and you could send it over to some other computer via wire, or you could take it plug in your USB drive and you could just take that particular file the binary file
that's created and you can go elsewhere and just play that file or just deserialize it to see the contents of it.
Right? So you could imagine this is something that is needed in terms of distributed computing
since not everything is been carried out on a single computer you you would process something on your computer you would you would write it to a file
and you need to pass on this file toward or transport this file to different computer right or typical example in which you
would think of serialization is more about you. Could you could have say for example one operation carried out on one computer
and same operation or different operation on a same file carried out on some other computer now you
want to move Just them together and that's where you would have serialization and deserialization coming into picture basically to understand
the concept serialization is nothing but writing the state of the object to a file and deserialization is nothing
but again taking the state of the object that's been written to the file again putting it back to a class format
or when you want to use this serialized class. You would basically deserialize it use it. So serialization is a process of converting an object mrs.
To sequence of bytes, which can be persisted to a disk or file and can be sent through streams or can send across wire
or could be you could actually take it on your drive and transport it elsewhere the reverse process which will convert data bytes into object
is called D serialization. All right. So how do we have this serialization
and deserialization done or what classes we have for supporting it. Let's talk about that.
All right in this example. You have a student info which is having ID and name, right?
And basically you could see that we have created an instance of student info which which has value of 9 and John.
All right. And now what we are doing is we are writing the state state is nothing but the value
that this particular object holds right which is nothing but ID of 9 and name value of John, right?
So we are writing this into a do a file which is nothing but student Dot txt. And and we are just writing that to a particular file.
Okay, and this is nothing but the student dot txt file that you see it should be txt. Actually.
It's it's a binary file. So you should I would rather prefer to have student dot Pi n or binary and you would write it to that file
using right object. So the supporting class for serialization is object output stream
and object input stream. All right, so I will quickly take a demo of this. So the first thing that we do is serialization serialization
is done by object output stream. All right. So again, we have a main method suppose.
I want to serialize it. So I would do something like say I have a private method which is void,
which is serialize. Okay. So the first thing I would want to create
is nothing but Java class which will hold the state right. So this is a class which you want to serialize or which you want to send it
across two different computers. So such losses are known as model classes, right? Basically such classes are nothing but classes
which holds chunks of data. So for Simplicity, we have taken small data like ID and name,
but in general you would have like chunks of data. They're all right. So here I say Vehicle info.
Maybe this is the class that I have here. Alright, and now what I do is suppose I have Suppose I have
two parameters here. All right, so I have two parameters here, which is nothing but integer
and suppose I have like string name of equal. Alright, so now I Define a Constructor for vehicle info which takes T three parameters for Simplicity number of Wheels.
First. Say for example, I take name of vehicle. then it's number of wheels and say I have one more parameter,
which is mileage. All right. Remember we use of this keyword
since the name of the arguments and the name of the instance variables are the same we need to use this this DOT number of Wheels.
Is equal to number of Wheels? All right, and this dot mileage is equal to mileage. All right.
So this is a simple model class which is supposed to hold values. Basically in reality.
This would be holding like chunks of values. Okay. Imagine like could be like megabytes
of information or even huge gigabytes of information. All right, but for Simplicity here, we are taking this simple class right.
Now. What we want to do is we want to see realize this into a GFI. We are going to create an instance
of this particular class and we are going to dry this state or whatever values we have that particular instance.
We are going to write it to a file so that it could be read from somewhere else or it could be used by any computers on the network.
All right, so I have vehicle info. Again suppose I write BMW is equal to new vehicle info. Now, what I have to pass is name of the vehicle,
which is BMW right number of and I pass some value like Like 10, all right. Okay.
Now we have created instance of vehicle info and we have passed the values that we want to pass it and now we want to see realized this.
Okay. So how do we see realize is nothing but we use object output stream, right?
So what does it take? So basically you could go into the source code and if you want to deal more you could actually
see what other Constructors that it takes. You could see it takes output stream.
So one is output stream as a Constructor. If it takes output stream, then I'll have to create file or stream.
Basically. I will name this as object stream and this one would be filestream.
Okay, I create a new file output stream and you could pass the file on which you want to write it. All right.
So in this case the file on which I want to write a particular class. I will pass it as file
and I'll be passing it over here. All right. So what I am trying to do here is resolve this okay.
So you take the file or to which you want to see realize so basically this vehicle info would be serialized into this file
and this file would be transported anywhere across your network. So it shows file not found exception again,
I choose to throw it. It shows my exception now, I've passed this file stream here.
Alright, so this is how we usually write programs. You have file input stream file output stream, which is nothing but the file
to which you want to write write the state of the object and you instantiate object output stream. Okay.
Now let me write to it. Okay. So basically what are you going to write is nothing
but vehicle info just instance of okay. So you're going to you want to write this instance of vehicle info particular instance of vehicle info
into your into the file? Okay. So I write something like this.
Okay. So so basically what we'll have to do here is whenever you have this thing
whenever you want to write or whenever you want to write the state of a class to a file you need to implement one interface,
which is serializable. All right. It's a do-nothing interface.
It's just used by it's a mock-up interface as well marker interface rather. It is just used by jvm to say
that this particular class could be serialized but it doesn't have any methods within it as you could see if it would.
I've had some methods it would have shown an error saying that you should implement it. But in this case it didn't because it doesn't have
any methods within right? So basically when you have object streamed, right? Okay, so it takes all right.
So we we create objects stream and we pass the file that we want to write to and we have right object method which would take the instance
of the class that you want to write and we have like we flush it and we close it. So one thing to note here is vehicle info class has
to implement serializable. That's a marker interface serializable is nothing but a marker interface
and it doesn't have any method within it is just an indicator as you could see it's an empty interface. All right.
This is the source code of it. You could see it's an empty interface. It is just a marker
or it is just a notification to the jvm saying that this class is something that could be serialized. Alright, so we have this class
and we are going to serialize the state of BMW object into a Phi. Alright, so now
what I do is I create Object output stream demo and I call serialized within it now this serializes taking two parameters.
So if the first parameter is this is other way of passing it. We are not actually creating instance. We are passing it directly.
All right, so I pass this and I say serialized file. Okay, maybe dot bin and the other parameter that it's taking is nothing but the instance of class
that you want to serialize. Alright, so here we are again getting exception which needs to be thrown off.
Alright, so this is a simple class that we have we have vehicle info which carries BMW instance
or we have created instance of vehicle info with BMW values and we are trying to serialize this to the to a file which is serialized file dot bin.
Okay. Let me see if it runs. Alright, so I hope it ran now.
This is a binary file. If you see you are not going to understand everything that you have within this file.
Okay, so I just Okay, so here you could see it's not something that could be completely readable,
but you could get some idea about it. It's during game W. All right, and this is not meant
for you to read it writes not in a human readable form. This is basically for passing this over a network and at the other end you would deserialize it.
Alright, so this is how we see realized it now. We will check on how we deserialize it. All right.
So you you saw the contents right? It's something that is not readable. Okay again, I'll open it up.
Not everything would make sense. But you could see that this is for BMW. So basically you could see the value BMW here, right?
So now what I do is I want to see see realize this right. So this is how you see realize it. We spoke about it
how we see realized it now we are going to deserialize this. So basically this deserialization won't happen on the same computer.
It might or might not or it could be saved different application within the same computer as well. So if you have two applications running
and want to interact between the two applications running on the same computer could use serialization and deserialization or you could majority of the use case
for this feature is more about passing it over the network. All right.
So, let's see how we deserialize it. Okay. So when you easy realize it you use object input stream.
Okay, so in the same class or I'll just for Simplicity sake I'll change this to object stream demo because this is not just all right,
so I Defector it and I change we name this too. Okay, so this is been renamed to object stream demo now
create a private method, which is nothing but void deserialize. Again First DC realization you need to have the file right
you could imagine of you having the file and file should be okay. Okay.
So now what we do is basically we could have this as a file rather since we are going to send the same file.
Same file for DC realizing as well. So it's better to have it defined here. And you pass it as CD list.
Okay so far we have serialized it now we are going to deserialize it. So what you do in D serialization is nothing
but you use object. So the first thing that you will have to create similar to be created
file input stream about now, we are going to file output stream in the serialized case, but here we are going to create a file input stream.
And a provide the fight here. Alright, so we have file input stream created now we have object input stream.
Alright, we are creating instance of it and it would be new object input stream and you would be passing the fill input stream
that you created the step above all red showing you for exception. You have to could either throw it or you could handle it.
So this case I'm throwing it off. Alright, so we have so here you could see we had bright object now,
I'll have read object. So read object throws filenotfoundexception or class not found exception
that you need to handle right again through it. So here you could see IO exception and class not found exception right single method
can choose to throw multiple exceptions from within. All right, so we have chosen to throw it now we have vehicle info or we don't know
whether it's BMW at that point. So I say d serialized object. All right, so object streamed read object by default.
It returns object type. So we need to we need to type casted. This is how we typecasted one form to the other.
So basically we know that object streamed or read object is going to give us vehicle info object. That's why we can actually type cast it.
All right. So now what I do is once I get this D serialized object,
I'm going to print out the values that I have within. All right, so I'm going to print the name of the vehicle. So I say All right put us.
Right, so name of the vehicle the serialized file similarly. I just copy paste. It will print number of Wheels Alright
and we'll print mileage of we go I need this one. Alright, so basically we are serializing it and then DC realizing it after deer is realizing it.
We are printing the value of the contents or we are printing the contents of a particular object to see if it is what we expect.
All right, so maybe I can do one thing. I can delete this off. Let me close this and let me delete this off.
Okay. I'm deleting serialized info. I'm going to run this program again
and this program will serialize and deserialize it. Okay, it will see realize it in the first case. And now I'm going to write would be serialization deserialized
and the serialized file. All right now here as well. I am going to throw away.
Okay. So first we are going to serialize it and then we are going to deserialize it and see
whether we get the contents that we expect. All right, so it's running. Let's see if the file has been created.
So we deleted the files. It's not yet come. So we can see that a file was created here.
Right which is a serialized file and we printed the value from be serialized file as well after DC realization. We printed the value of the contents
in the serialized file just like we got the expected value which was BMW 4 and 10, which you had said.
So basically what we are doing here is we are storing the state of a class or state of the object
to a disk and this could be transported anywhere when I say it could be transported is nothing but this is just a binary file,
right so you could take it into your text file or you could take it into your pen drive and you could take it anywhere.
All right. So that's the beauty of serialization and deserialization.
I spent more time on this because this is something that is very important for Big Data
since big data is nothing but all the big data Frameworks that we have they follow distributed computing and this is one of the important internals
of distributed computing though you need not. Take care of it.
It's all taken care by the Big Data framework. But this is an important concept to understand. All right, so let me quickly talk about wrapper classes.
It's not an important topic to but I will quickly cover on this thing. So wrapper classes are nothing but you have
primitive data types that we discussed the first day, right? So initially we didn't have this wrapper classes in picture
when the initial version of java was created. So data types were dealt in terms of primitive data type itself.
Then since Java is object oriented, right? It has to be completely object-oriented so they came up with a concept
that we shouldn't be dealing data types as a primitive data type rather. We should be having some sort of object to it
which is rapper, right which is wrapping this primitive data type. So in order to make it completely object-oriented
they have come up with this wrapper classes, right so int when you define int right if you define int something of this sort,
it's Native whereas you would have something like this. Inti is going to be primitive data type and whereas you would have something like this
which is nothing but wrapper class, right? So if you go into the wrapper class, you could go into the source code and see it's nothing
but it's doing the same thing. It has got some utility methods as well on the top of it, but basically it storing your value.
So for now they have changed the source code quite a bit. Basically, it's touring in terms of characters, I believe. All right, so you need not take care of this.
But what I am trying to say is wrapper class is nothing but a rapper built upon the primitive data type so that everything is taken care in terms of classes since it's
completely object-oriented programming language, right? So Java has a class dedicated to each of the Primitive types. These are known as wrapper classes
because they wrap the primitive data type into the object of that class. All right, so you could see for bite you have a wrapper class
corresponding wrapper class as bite with starts with capital. Be all right, since it's a class it has to start with uppercase
or it has to follow camel casing short has again short int has integer long has long float. You could see a corresponding wrapper class
with name float double will have double character CH AR will have character which starts with a bouquet see again Boolean again has
camel cased Boolean as its wrapper class. All right, so when it comes to classes you could have hierarchy.
So basically you could see that all this byte short integer long float double. Everything is subclassed from number.
So basically in the source code that we saw for integer you could see it's extending number right?
So that's what it is saying here is a subclass of number integer is a subclass of number. So how do we reduce wrapper class?
It's basically you just have to pass value to it. You could have as a part of Constructor, you could pass some kind of value to it.
So you could either assign value of hundred right or you could have something like Something like this. Okay.
So you have different variants of it. You could either create it directly assign literal value to it,
or you could have something like this but as you could see this is deprecated. This is no longer required.
Actually. It is still accepting it. It's not throwing compile time error,
but it's deprecated. Which means if you're writing a new code, you shouldn't be writing it this way.
So there's also concept of Auto boxing which is nothing but if you have like integer a could be assigned value K directly.
All right, or could be assigned value J directly. So it could be converted from wrapper class into your primitive type without any kind of conversion.
That is known as Auto boxing. It does it by itself? What is generic in Java generic is nothing but template in C C++
in C++ rather you have you define a template which is which is a general. Thing you're not putting in
a specific data type you are saying that this is what it is. It's a template
and you could have any data type during runtime. So basically what you're trying to say is this particular method or this particular class is a generic class,
which could handle any data type that is passed. So it's not specific. All right.
So generics in Java is similar to templates in C++ generique enabled type parameters when defining classes interfaces and methods, right?
So the type parameter is nothing but a generic type and it's not saying that it's going to deal just with integer or with character
or with string. It is saying that it could handle anything that comes anytime that you would send it any type
that you would instant. She ate that class with. All right.
So when we take example, you should get some more idea about this generate provide compile time.
Type safety allowing programmers to catch invalid types at the compile time. So generics work on type Erasure,
which is nothing but which is done at the compile time itself. Basically, it's done at the compile time.
It can catch exhibitions during the compile time itself, or it could prompt you during the compile time itself, right? We can specify the type the angular brackets.
So whatever type you want to do, whatever type is that class going to handle we can put it into angular brackets,
which makes it generate right? So here you could see that this is a method which is print array
which is taking e as type. So as you could see he is the type here. All right, he is a type here and we are not mentioning
that it is going to take print integer array or string array or something of that sort. We are seeing that it generates.
So whatever you pass or whatever you instantiate it with it's going to take that array and print elements within it.
All right, so this Method could be thought of as a generic method, right you could think
of this printer as a generic method not a method which is just taking integer and printing it. It could be string array or it could be anything else.
So that's what generic means advantages of generics type safety objects or single type can be stored in generics.
We would be looking at it in the next example typecasting is not required. There is no need to type casters
because it knows that what kind of objects that particular data structure would carry or hold compile time checking it checks type mismatch error
in compile time, which avoids runtime errors. All right.
So imagine a list which we are going to talk in further slides what is list, but imagine a list which is a collection.
All right, and you have like imagine a list which is holding strings. All right, so you have list
dot add and you you add a direct cut to it. Now when you get 0 typically get Zero would have object the return type would be object
since it doesn't know that it is just carrying string know where you have mentioned that it is a string of
it's a list of string right? It's a generic list so it could hold integer value as well.
Alright, so in that case, what you need to do is when you do list dot get 0 you have to Typecast to the string here.
The next element could be integer. We don't know right since we haven't said that it's a list of string.
So that's that the reason you need to have typecasting done. All right now using generics you could Define that this list is a list of string.
All right, it's going to carry only string nothing else. It's going to hold now. It makes it simple right you add either a car now.
If you try to during the compile time itself, if you try to add integer to it, like list dot add hundred.
It would throw an error saying that it cannot pulled integer because it's meant for string. So that's the advantage that you get over.
Rick's you could hold it you could catch errors during the compile time itself. All right, so it won't allow you to add
or it won't allow you to put a hundred in to your list of string. All right, and
since we know it's going to hold only string there's no need of typecasting it as well. You can see here.
It's not typecasting it in this case you had to put this string here, right? So basically in this case, you need not type custard.
There's no type question required because we know it's a list of string now what a collection Frameworks collections framework is nothing
but you have data structures in terms of classes. Again, it's a wrapper for data structure. All right, it's nothing
but like you have array which is normal primitive arrays, but this one would be sort of growing array. So basically you need not take care of actually,
you know adding up indexes to it. It would automatically It's a self growable array. So you could imagine
when it comes to array you could imagine collection. You could imagine one of the classes within collection is list,
which is nothing but growable array of any data type. All right, so Java Collections framework provides an architecture
to store and manipulate a group of objects or Java Collections framework includes the following it has interfaces classes
and algorithm interfaces is nothing but again specification. This is how it should be. So Java says that you know,
when you have a list it should have so-and-so methods like adding to the list setting to the list removing
some elements to the list something of that sort. So it defines collection you have a collection you should have so-and-so methods
and list should have so-and-so methods and so on and so forth. So basically it's for specification classes is nothing but concrete implementation
so list by itself would have Would have all the methods that you just the it's an interface, right? So it would have all the methods
that you want without any implementation. But this classes would be actually implementing it or rather.
It would be implementing this interfaces and would be giving a concrete implementation for methods. Right?
So as you could read here, it's concrete implementations of collection interfaces in essence.
They are reusable data structures, right algorithm is nothing but there are utility algorithms that you have like
if you want to sort a particular collection or if you want to sort a list list of string you could do it using using your algorithm using a predefined algorithms
that already comes pre-loaded with Java. All right when I say algorithms and where are these algorithms residing?
It is a class which is collections. So you have a collection which is an interface top level interface
and you have a collections class which is class. Which is holding all the utility methods or algorithms, whatever you call
who basically you could just read through this hierarchy. These are different types of data structures that has been supported by Java.
You have a collection. So the top level interface is as you could see there's collection,
which is extending I treble all right, extending iterable interface collection itself is an interface you have a list as interface,
which is extending collection. All right. So list is extending collection Q is extending collection set
is extending collection. So we have three distinct type of data structures Year's list queue
and set list is nothing but you could say it's a ordered one, right it maintains the insertion order write the order in which you inserted data into the list
Q is nothing but first in first out and whatever you push in first would come out first and set is nothing but To sit in mathematical form right over
in you have unique values. You cannot have duplicate values. So that's what set is but it's it need not be ordered.
It need not maintain the insertion order, right? So you have the concrete implementations for list our array list,
which is nothing but again similar to array that we have right the normal primitive array that we use.
So it's a wrapper class for it. We have a linked list. Alright, so this linked list is nothing but doubly linked list.
All right, so you could insert from you could Traverse the either direction you have Vector as well which is which is Legacy Forum,
which is not used to that extent yet as of now. So we have vector and we have a stack which extends this Vector redstack is nothing
but last in first out and it adds some more capability on the top of this Vector, which is nothing
but it's a subclass of Victor. All right, so these are Implementations of list we have a Q and as you could see here,
there's one interface here DQ which is nothing but it is extending Q. Alright and we have an array D Cube which is implementation of TQ.
All right. It's a class which is implementing DQ interface. All right, this is a priority queue as well,
which is a class which is implementing Q when it comes to set we have a sorted set which is again an interface which is extending set
and as you could see there is a tree set, which is a concrete implementation of sortedset and it sorts the element that you put into a set.
It sorts it into a specific order. All right, again, the concrete implementation of set f is our hash set
which is nothing but which does some kind of hashing which is a default implementation of set and which doesn't maintain insertion order
as set is nothing but it is indexed to for fast reversal or Want to go get something out of a set,
its kind of indexed set. You could say right it has buckets and everything. It follows hashing algorithm,
right which is which is basically for indexing or which is basically meant for retrieving data faster
from the hash set from the set and that implementation. That's the default implementation which is has said, all right,
there is a linked hash set which maintains the insertion order. All right. This has said doesn't maintain the internet insertion order.
So if you put X & Y into your hash set and if you retrieve it or if you Traverse through it and print the values,
it's not mandatory that you would get X and Y in the same order whereas in the linked has set its pretty much you could be
a hundred percent sure that X would come before why all right. So it maintains insertion order what is less list has
nothing but an ordered collection of elements which can contain duplicate son like sect it can contain duplicates,
but it has ordered an unlike say State has ordered write lists are further classified into following arraylist linked list and vectors, right?
So based on the use case, you could select one of these data structures. Let's talk about array list arraylist is nothing
but similar to arrays that we had and here you could see element stored within of size 5-a released
of size 5 and you could see value stored within right. So this is how you instantiate an arraylist array list object is equal to new arraylist
and you have an arraylist created one of the main things about arraylist is as I said itself growable
or its Dynamic you unlike array the normal primitive array where in you had to within your square brackets, you had to mention the size of the array
during the Declaration itself. Whereas in Array list, you need not have to mention this sighs.
All right, it grows by itself as then when you insert data into it, it would go So basically you could imagine a normal
in general scenarios or in most of the programming cases or in most of the real use cases. You don't know up front.
What is the size of data that a particular array is going to hold right. Now the number of elements that a particular array
is going to hold you cannot know it up front because things are Dynamic right? So suppose you are reading from the database.
You don't know what that size of the data or what what are the number of elements that a particular database is going to have.
So typically you could imagine real cases you would go with arraylist and not primitive arrays.
All right. So also you could imagine this is this is this is saving us in terms of memory
because we are not hard coding the values of it. So by default, it starts with 10 size of 10 and then it keeps growing as
and when you insert it. So again, it has a logic it doesn't keep going for each and every That you do
but it has a logic within to grow it by particular size. So which is all optimized
which is good for your for your cases use cases. So these are the methods that we have within our A-list. It's very straightforward.
When you do add it appends the specific. It's usually add and not collection for collection. You have a doll methods.
So it's a typo here you have ad and you you mention an element that you want to insert into a list
if it's a string you would have string string element or if it's something else if it's an integer,
you would have integer element you could add it to a particular index you could clear it removes all the elements
from the list you could have last index of a written the index of this list of the last occurrence of the mentioned object.
So if you have multiple as we said they could be duplicate values within but when you give last index of it's giving you the last index
All right last index of the object that's been passed you could clone it. So it's basically coming clone is nothing but a method
that they're within your object class and it would shallow copy the array list. So whatever list you have there would be a clone of it.
Right so clone is as we could see it's it's it's from the object class. So any class that you create could be clone.
All right, then you have to array so you could have an array list and if you want to convert it into a primitive array and use it
in some form you could do that thing as well by doing two array you could trim to size. So trim to size is nothing but prims the capacity
of this array list instance to the list current size. All right. So whatever is the size
it would trim to that so moving on let us understand how we can Traverse through a list of collection. So travel cell is done through I traitor interface.
Okay. So basically you have a nitrate interface and that's how you Traverse it.
Basically you have implementations of it since it's an interface it is it is just the specification you would have an implementation of it.
So I traitor is an interface that I traits the elements. It is used to Traverse The Collection access the data element
and remove the data elements of the collection. All right, it's used for traversal as well as removal of data elements within the collection.
Okay. So what are the methods that we have within I traitor first is has next.
So it's it's nothing but as you could imagine it is written in Boolean. So if it has a next element it would return you true.
Okay, and if it doesn't then if the arraylist is already exhausted then it would give you false.
So you would I basically would have a loop wherein you would see if it has a name next right
and if it has next you could This is the next element using next Method All right, so next would return the actual object
and to remove it you could do I treated or remove which removes the last element written by the I traitor. Alright, so here you could see
how I treat her is being used you have you declare an array list and you you I trade through it
and you print the elements so quickly we can take this. So basically I do calm dot edu Rekha dot I create one more package now for arraylist.
Okay, Isaac collections, demo dot array list. All right, so I have this particular class created now. I create a main method and I create private void.
I write a method which is create array list for demo. All right,
as you could imagine when I see create it's going to return an arraylist. All right, we want it to return list.
Okay, what I do here is I would create list and this list is going to be list of string. Okay, imagine generic
that we spoke about you are saying that it is going to be list of string now, it's three mirrors saying which list I want it's going
to be Java dot util so you could see Java dot util doll wrist here. Now.
I say it's strings dot new. I didn't list. You're saying that here
what we are doing is we are instantiated. So we have a list of strings and we are instantiated it since we want arraylist.
It's going to be a release. So here you could see array list being imported as well. All right.
Now what we are going to do here is we are going to add some values to it strings dot add or maybe I will put again
since we have been talking about cars and bikes I will cars right? So we are creating here cars list of car.
All right, so I put BMW But what? all right, so all German cars and then we Then we written it. We are returning this.
So we are creating a collection. Right and we are inserting data into it and we are returning it.
All right, so this would create a list for me right now. I want to I trade this list, okay. So I create a private method which would return void
and which food print arraylist or demo. All right. So as you could imagine
this is going to take a list as parameter. All right list list to be printed or it's pretty much implicit
or implied that list is whatever list you pass as a parameter is going to be taken for printing. Okay.
So here what I would do is list dot I traitor I take the I traitor. Okay, so I get the list I traitor
I say I traitor and it's a list I traitor All right. So I take this list I traitor
and I say list right while while it has any elements within so, how do we check with
whether it has elements within is nothing but dot has next if you remember while it has any elements within what we are going
to do is we are going to print out print the value the list. Alright, we are going to print it using I traitor dot next. Okay.
So I've created two methods one is create a released for demo and the other one is print arraylist for demo create arraylist is doing nothing,
but it's creating a list and it is returning the list and printer a demo is printing the list
that you have passed as a parameter. Okay, so I create a new element of array list demo dot create arraylist.
All right, and whatever I realize that I get over. Will is that I get I would be putting it here.
Right now I create one more this and I print it. All right. Now when I print it I am going to pass
this returned array list. All right, I think it's pretty clear now. So we have created a released in this method
and we have returned it and in the second case we are just going to print it. We are going to take any array list
and we are going to print it. All right. So let's see.
So rest of the collections most of the collections you do it pretty much similarly. It's just that you know,
it's used for different use cases. All right, I am getting some error I think okay. Yeah, you could see here.
It has printed BMW Mercedes and Audi which we had put into the arraylist. Alright, so that's
how you kind of I trade through it. Similarly. You could just remove it.
You could use list I traitor Don't remove will remove the current element that's penetrated.
All right. So yeah, this is I don't need to actually create one dot remove you could remove it.
All right Drew. So that's how you remove it if you want to so you you have the same syntax.
So basically we don't use I traitors nowadays more. It's more about we have Java 8 there are different constructs. Like we have Lambda expressions and stuff.
We have screams stream processing, which is you have I traitors. All right.
So this is what we have we have a hydrator, which is I creating through all the elements right and you could remove it as well using
the I traitor usually nowadays. We use Java 8 streams which helps us to I'd rate through which is pretty much
like the underneath is I traitor and stuff but there are some some kind of abstractions done
so that we don't actually deal with I traitors there's some kind of abstractions are it's less verbose in this case.
You have to write a lot of code to print through and everything. Whereas in streams. It would be just one method call
which would do lot of things right. So that's what has been used as of now, but this should be good enough to start off with to understand
the basics of it. Alright other thing is I was talking about the best practices.
So it's good to have things broken down in methods. So that could reuse it rather than putting everything into the main method which makes it difficult
for any other developers to understand mode. So basically it's a good practice. Make it modular,
right and which enables reusability as well which reduces the lines of code within your program. So tomorrow if I have some other list,
which I want to I trait I can just pass the list to this and it would right fit and give me the results. So I don't have to write the same piece of code again.
Alright, so moving on so we have a released arraylist is nothing but abstraction done on a primitive array.
So what are the advantages of using array list? It's more about it's faster in terms of retrieval right index based retrieval suppose.
If you want to get to the index to just write like arraylist get to and it would give you the one at the end x
2 or the element at the index to whereas when it comes to insertions and deletions. If you want to insert a particular object
at index 2 what it has to do is it has to move that was there at the index to to the index 3 and it has to increment the entire array by one
or move to the right by Position which is pretty cumbersome, right which takes a lot of time so insertions and deletions are not good when it comes to arraylist,
whereas when it comes to retrieval it's faster. So based on the use case you have to decide which data structure you should go for right?
So simply put we think of Big Data applications. It's mostly about you don't have insertions and deletions as such it's not transaction,
right but typical online transaction processing this thing would be application or say, for example, you have Amazon right Amazon or something
where in you have a lot of transactions happening which needs insertions and deletions right in that case arraylist is not a good option.
Whereas in terms of big data, which is like analytics right online analytical processing which doesn't have transactions as such right.
It doesn't have insertions and deletions. It is one-time load you have data you load it into your data structures and you do some kind of processing
or you do some kind of analytics on This data, but you don't manipulate this data. You don't actually have
insertions or deletions done in such cases. It's better to go with a realist. All right, so
which is a variant which is good for insertions and deletions that is linked list. All right, when it comes to insertions and deletions
and you want to maintain the order of the list insertion order of the list. That's when you would go with linked lists.
All right. So linked list is a sequence of Link's which contain items each link contains connection
to another link and that's how insertions and deletions are simpler. You don't have to shift elements to the right
when you insert it or you don't have to shift elements the left when you delete it.
There are two types of linked lists to store element singly linked list and doubly linked list singly linked list is nothing
but it has pointers in One Direction doubly linked list is like it has previous and the next its stores.
Both each node would store previous element pointer to the previous element and the next element. So yeah, this is a singly linked list wherein you see
that it has pointed to the next. It doesn't have pointed to the previous one, right? It's a one directional traversal, right?
Whereas in doubly linked list, you could see that it is looping back to the previous element as well.
So it has a pointer to the next and it has pointed to the previous one. It's a bi-directional traversal.
So linked list has pretty much similar to arraylist you have ad you could add a particular object. You could check
whether a particular object is contained within the linked list and that object has to be passed as a parameter to contains. You could add a certain element at a particular index.
You could add it to the to the head of the list you could add to the last you could check the size of the list. You can remove the element from the list and you
could get the index of a particular element so you could pass some element and get the index of it since linked list is again,
it can have duplicate elements. If you want to get the last index of a particular element you could do that thing as well.
So if you have multiple elements, you would get the last one right the index of the last one. So linked list example here you could see
that a linked list of string is being created and you add values to it, which is fragile Rahul
and Richard you add things to it. And and yeah, this is just about adding elements to the list.
All right. So arraylist versus linked List released internally uses Dynamic array to store the elements since I said,
it's Groove able it grows on its own linked list internally uses doubly linked list to store the elements to add an element
in between or to remove an element from the array list slow because it internally uses array
if any element is removed from the array list, then the rest of the elements should be shifted to the left. All right, similarly
when you add something to the list you have to move everything to the right adding an element in between or removing
an element with linked list is faster than arraylist because it uses doubly linked list. So no element shifting is required, right?
You don't have to shift any address. Or something. It's just about moving the pointers.
Right? So you would just move it skip one element and move to the next in terms of deletion.
Whereas in terms of insertion, you would insert a node and you would manipulate
the pointers according T. Alright arraylist can act as a list only it is normally for list but linked list could be thought of as a list as well as Q right
since it's a doubly linked list you could have like first-in first-out arraylist is better for storing and accessing data. All right.
So as I said, this is pretty much good enough for analytical sort of application analytical nature of application.
Whereas when it comes to transactional linked list would be better. So linked list is better for manipulating data
and it is slow in searching an element because it needs to compare the elements from the first note when it comes to you know,
index based search. It is slower because it has to start all the way from the start.
Was it goes through links? Right? It has links to next element and that's
how it traverses through vectors are similar to erase but the Legacy form of it. It's a dynamic array again similar to arraylist.
All right, it could be visualized very much similar to array list, but just that it's a legacy of java.
It's not used to that extent or I would say it's not used at all at this point. But what is important to note is Vector is synchronized
when it comes to multi-threading it's better to go with Vector, but nowadays we have concurrent arraylist arraylist that is supporting concurrency.
So there are a lot of optimized versions of it which gives lot of performance Improvement. So vectors are no more used as such an industry.
It's a Legacy form of arraylist. There are concurrent versions of arraylist that that has been evolved
which could be used in multi-threaded applications. All right, Victor. Contain many Legacy methods
that are not part of collections framework. So it doesn't even fit into collection framework because it contains some Legacy methods
which are no longer used or which are not part of collection framework at all. Now Vector is again taken into collection framework
and it implements list and that's the reason it has the implementation for this methods
because this methods are typically coming from collection and list collection interface and list interface and all the concrete implementations
have to implemented. And since Victor is one of the concrete implementations of list.
They have to ensure that they Implement these methods as well. All right.
So these are the methods pretty much similar to what we spoke earlier add clear and add to a particular index remove
and then you could have size and you could have last index of and last index of object. So it's a vector of string then you add element you add.
Issued fun and Kumar and you just write great through it to print out the message from a chiffon and Kumar would be the output of this application
or this program. All right, so you could see I traitors use here as well. Like we did it in list.
So let's talk about QQ is nothing but first in first out whatever goes in first would be first to come out a priority queue allows you
to initialize the queue. These are implementations of Q. So you have a priority queue you have
as we said linked list is list as well as Q so you could have linked list assigned to a queue right linked list is the list and linked list is a queue right is a relationship
which means it's extending it right. So here we can see double ended queue lets you to a door and remove so double-ended interface DQ DQ interface
allows you to Add and remove elements from Peak as well as from the bottom. That's what DQ is double ended queue,
right and array d q is nothing but the concrete implementation of DQ EQ is an interface and the concrete implementation is array d
q. So what are the Q & DQ methods you have ADD method which is nothing but it adds to the top of the queue
and it returns a Boolean as you could see here if it is successful if it has added to the top of the queue
it would give you true. And if not, then it would give you false right you have offer
which inserts the specified element into the queue again, it does the similar stuff. It inserts to the cube.
All right, you have removed which removes the head of the queue we have pull which retrieves and removes the head of the queue again.
It does the same thing retrieves and removes the head of the queue and returns null if the queue is empty.
All right, bullying is nothing but getting the top of the queue. And you have element
which retrieves but does not remove. All right. So when you do Q dot element it would give you the top
of the queue of the head of the queue, but it would just give you but it would still be there. So if you want to check value that is on the top of the queue
if you want to check it and do something with it based on the value that is there on the top of the list or top of the queue.
If you want to perform some actions we can do it using element Peak again does the same thing it retrieves but it does not remove the head of the queue
if you want to actually retrieve and remove the head of the queue you would either use remove or pole. Basically you would use pole
not remove right remove is not a good operation to do when you want to retrieve and do some actions on it. The best practice would be
to use piec to retrieve and use Pole to actually retrieve and remove Alright, so let's take an example of Q.
So right here in the package I create. Secretary main method, right? So I create private.
Q v8q for demo All right, so it's asking me which Q I will go with this one, which is Java dot util dot Q.
All right. Now I am creating a new priority queue which is a concrete implementation
which would be say for example of integer, right? So I create a queue of integers. So we are putting integers within all right,
and what we are doing is new. I am instance eating it. Alright, so this is a q
which is going to hold just integers. That's what Jen Rick's come into picture, right? Queue of integers dot add suppose.
I put a hundred and then I put ad could is used to as I said to insert right and I put 50. So I've created a queue and I written this Q.
So I've created a q so far. Let me print this queue. Alright, so we have
which is going to return void and print queue for demo and which is going to take you. All right Q of Cl you don't even put it here.
I so now, let's see how we can retrieve elements from it one way is to I trade through it and let's see how pole
and other things work right? So let me get the head of the list. So this is just retrieving it right retrieving
head of the list. We are not removing it it is still there in the queue. We are just trying to check what it is.
So when I say when it's retrieving it speak right now if I want to remove it if I want to kind of retrieve and remove it.
So basically what I'm trying to say here is when you have q dot Peak it's going to return the top of the list which is nothing but 50.
All right. So the first one the peak is just retrieving it. Whereas when I pull it it's going to retrieve
and remove as well. So I have q dot pole is going to now when I do s out retrieve
and remove Say this is the first attempt. All right, and we again do it which is the second attempt. This is just to show you
that when you pull it it's actually removing it as well. Whereas Peak is not removing it. All right.
So Peak is just retrieving it and this one is removing it. So the first pole is going to give you 50 whereas the second pole is not going to give you 50.
It's going to give you 200 because it has removed 50 from the queue. All right, so this is what I wanted to show.
So let me Q demo Alright, so here I'm going to do demo Dot. So when it comes to generic we can make this generic Q program
by doing something of this and instead of hard-coding it you could put a Ste here. Right now, let's keep it as is.
Alright, so the first case Q is being created the second case. We are going to see how we can pole works. Retrieving is just ringing
and it is still keeping that element at the top of the queue whereas polling is actually removing it as well.
All right, so you could see here Peak is giving you the last one here. It's a double-ended one.
So it's giving you the last one here and pull is giving you this one. It's based on Friday.
I think. All right, so you can see pole is giving you 50 as well and when you pull it again,
it's giving you a hundred. All right, let's move on to the double ended queue. All right.
So here we have something like you could remove first. All right, you know, so it's a double ended queue you could in fact
in both the directions you you could actually remove first and add to the last and stuff like that.
So you could see here that there's a double ended queue created with four elements initially 2142 63 and 84 and you could see
that remove first is removing 4221 rather from here since 21 is the first one so it's removing 21 and remove lost or add
last it's adding to the last which is nothing but it's adding hundred and five to the last So what is set is nothing but
as we said it's a representation of mathematical set which is unique which holds unique values right?
You cannot have duplicate values within set set has its implementation in various classes such as hashtag preset and linked
as set so it's a mathematical set abstraction. We have variants of set in Java which is has said linked hash set
and we said hash set is nothing but it hashes it or basically there's this indexing done and it's good for retrieval
as you could imagine since it's indexed. It's good for retrieval. Okay.
So the analogy behind this could be our it is analogous to index page that you have in books,
right you have index page you just go to the index page and see at what page is the content that you are looking out for this one is
pretty much similar to that. So when you do hashing you would be able to retrieve it much faster then sequential retrieval.
All right, so that's what has said does and it has unique values of course, which is a property of a set.
You can't have multiple values within or duplicate values within it doesn't sort automatically. This is a typo here has set is
acid doesn't maintain maintain any order at all its internal to The order in which the retrieval would be done is pretty much internal
to the runtime or what I mean to say is if you have a has set with the same content and if you run it multiple times you would see
that the retrieval is different in different instances. So it doesn't maintain any order your application might demand something or demand a data structure
that is set as well as it maintains just the insertion order right? That's a scenario
in which you would go with linked hash set. So link - there is nothing but set which has
its insertion order maintained. All right. So the third one is tree set,
which is nothing but it so these are the methods that gets inherited into hash set and but similar to other collections
that we have, but just to talk about this one so you have ad which is just adding object into hazard
or linked has set you have contains which is basically checking whether a particular.
Object is present in a set. You can clear the contents of the set. You can check
whether a set is empty using he's empty method. You can remove a particular object from a set using remove method and pass.
The object that you want to remove clone is nothing but a method that is inherited from object class,
which is super class and it is basically meant for cloning any data structure or any class or any instance of a class.
All right. So when I say cloning it's not but making a copy of it and it's a shallow copy.
There is a steep coffee and there's a shallow copy shallow copy is nothing but the properties within the set.
So the references remain the same basically you would have a value say for example string right? If you have a value say at Eureka you would basically
have both the set pointing to the same instance of a dareka. All right. So it's a shallow copy and deep copies were
in you would have different instances or together. So when A Change Is Made in new set A particular element it won't be reflected in the other set.
So that's deep copy, but by default the shallow copy that's done. If you want to deep
copied then you will have to use some other utility or you some other class. All right, there's an iterator similar to other collection
you can have I traitor to iterate through the set and if you want to check the size of the set you could use the size method.
All right. So let's see an example of hash set and linked has set. Alright, so here I have created two methods create hash set
which creates the instance of has set and add some integer values to it, which is 130 340 and 440
and you see there is one more method created which is create linked hash set which is again inserting three elements into it,
which is hundred three hundred and five one. So I return this. All right now I write a method to 848 through it.
Which is displaying nothing and Print set and which would hit s. All right.
What you do is you have like set to print dot you could have I traitor right which would give you I traitor
which would give you an instance of I traitor for set. Alright, so we have I traitor created for set now. Let's iterate through it.
so remember how we I treated list so you would say set I traitor dot has next if it has next then print the value
of So basically we have this then you have set I traitor dot next which would give you the actual value.
Alright, so we have a method which would I trade through the set and display its content?
All right. Now what I do is I create instance of set demo. I create has set and whatever value I get.
I put it as as set. That's it for demo. All right, keep it I set.
All right. Now I have against it. I say linked has set for demo.
So basically we just creating the set. All right, then we create the linked hash set now said demo we are going to print it.
The first one to be printed is is Hash set for demo. And this is what is reusable ET right? See I'm not fighting the method to print it multiple times.
It's just once and which could be used for printing has set as well as linked has sit. So this is what reusability is all about.
If you don't expose it as a method and if you write it within your main method, you won't be able to reuse it
and you would have to write the same piece of code multiple times which adds to the redundancy and which is not a good practice.
And this also makes it much more readable by looking at it. You can understand that. Okay, it's creating a hazard.
The second method is creating a link has said and the third one is printing asset. So it makes much more readable, right?
So that's an important factor as well when it comes to programming. Alright, so here we can see
that when I printed the hash set it gave me 133 4440. Now the thing is it's just three elements. So it has maintained the insertion order.
But basically if I have more elements you can just copy this multiple. Okay, I remove this.
Okay. Now you can see that you won't get it in insertion order
since it was it was just three elements its by fluke that you got it in the same order as which you inserted.
So if you see it now. So now you can see that, you know, the first element that got retrieved is 4401
which was basically you put it at the last ride. So it's pretty much random. As I said linked has set.
The retrieval would be pretty much random. You could see 4401 then 130 which was the first element inserted than 340 then 440 then you saw 40,
which is again out of order. So basically this is what linked has said does and suppose I put
the same values in the link has set you would see that insertion order is maintained. All right, so from here
if you see from 130 in from here, it's printing linked has it and you could see the insertion order being maintained
as is 4410 and when you see Hazard, which is very much like this one, you can see that it's random.
All right, so that's what link has set an asset differs own preset is nothing,
but it Sorted set and you have a retain all method retain all method is nothing but intersection between two sets.
So basically you could pass one more set to the retain all method and you would get an intersection of two sets.
So what I mean to say is if you set has value one, two, one, two, three four and you pass one more set to it
or you invoke retain all method and pass one more set to it which has values 1 and 2. All right.
So your Source set on which retain all method is being called that has got four elements 1 2 3 & 4 whereas the set
that is passed to retain all contains only two elements. That is one and two. All right.
Now when you invoke it your Source will have only one and two your 3 & 4 would be removed off from the source set
because the retinol method is nothing but it's an intersection between two sets. Okay?
So size and hashcode, so all these methods remain the same as other ones that you have in different collections, right?
So you have like size which would give you size. Hash code is nothing but a unique integer that's written for any object in Java.
It's not just free set. Any object in Java when you invoke hashcode, it would give unique integer.
All right, because this particular method is inherited from object class of java contains is nothing
but pretty much similar you pass element and check whether that element is contained in the source set contains.
All is something like retain all it would return true only if all the elements in the collection that's passed here
as an argument is present in the source set. All right, it has to have everything all the elements. So the previous case wherein I said the source has one two,
three and four and if you do collect contains all on a set Are you pass a set to contains all with values 1 and 2
it would return true. Whereas if you pass a collection with values 5 & 6 it would say false
because you don't have values 5 & 6 in your Source collection. All right, I traitor is nothing but it Returns the iterator that we checked on you
can convert it to to array any collection could be converted to to array which would give you object array.
You could check equals basically checks if the collection is I think it has to be empty here it checks whether your collection is empty.
So there's a typo here. Just to give you an example. I will create one more.
So I copied the same method and I said create rehash set. All right. So what I do here is I will make these changes.
I will keep it as this could be kept as set and this is like free. All right, so tree set and and we are set.
Now I print this one. Okay, basically set the dough dot create rehash set. All right, so I'm printing this so prior to that.
Maybe I will put a statement here printing stre set. All right, so I created a tree set with this random values 133 4440 and stuff like that.
Now let's check whether it has sorted it or not. Right? So I created a tree set and I'm just printing it here.
So what you can see here there's we have created reset now if you see it right after printing tree set all the values
that you see here then 3044 41 3440. It's all sorted efficiency. Right and it's Unique as well.
So if you insert it multiple times like if you insert n multiple times still in it would take Just one value of 10.
All right, so you cannot have multiple values if you put 10 unlike array list or linked list, which will have multiple values.
This would take just one 10. All right, it would ignore the other one. Enunciate is a special type of set which creates genome
is nothing but a constant it's a replacement for constant in Java. So basically the replacement
for public static final and basically for constant earlier. It was like we used to use public static final
but now it's advisable to use enums four constants. All right, we'll see an example which would give you much more clear idea about it.
So first one is all of all of method is nothing but creates an enum set containing all of the elements in the specified element type.
So it would create enum set with all of the elements that you have copy of is nothing but creates an enum set initialized
from the specified collection. All right, so you could pass a collection and it would create an enum set out of it.
None of is creates a MD enum set with the specified element type or this creates a in mm set initially containing the specified element.
Can you could initialize it as well? So basically here we are giving a specific class with which it would be created
or the enum set would be created range creates a enum set initially containing the specified elements, right and clone is nothing.
But again, it's pretty much similar. As I said clone is a is a method that is inherited from object class
and is meant to clone or have a shallow copy of the data structure against which it is invoked or object against which it
which the Clone method is invoked. All right. So here we can see there's the enum
which is months. Alright, so here you could see a in a months which has like three months declared
within Jan Feb and March. All right. So now what you do is, you know him set off
and you just wait set out of two of these elements, which is Fab and March. All right, so this is what Them office
so you could have some enum values put in and it would create set out of it. Now when you write rate through it,
you would see that you have Fab and March into your genome set. All right.
So this is something that is required when you have enums with multiple constants and if you want to have
or you want to create set out of it and do something with it, right? So it would be pretty much common
or you imagine of enums as exhaustive list of constants, right which is used throughout your application. Now there might be instances
where in you want to have you want to put them into set and do something with it. Right?
So basically this is mint because the values within your enum cannot be put into a data structure as of now or prepare to enum set.
We were not able to put the values within the enums into a data structure, right? It was considered as a separate entity.
So now this is That you could put it into a data structure as object and you could play around with it.
So that's the reason we have enum set. What is map map is nothing but it's a key/value pair and it is unique Keys you it holds unique keys.
So suppose if you try to insert same key with different value, you would have your value updated or overwritten with the new one.
All right, so but it won't duplicate it. So what I mean to say is it maintains a key value pair,
but the key is going to be unique across. All right. So This Could Be Imagined as a table, right?
You have a table in data structure, which has got one primary key say employee ID and there's a name
which is like name of the employee right? Imagine. This ID is a primary key, right?
So you cannot have multiple values. So if you try to put some multiple if you try to put one more value to it,
it won't allow you to put in. The only thing that you could do is update the value of existing ideas.
He's our add a new ID. So this is pretty much similar to that. Right map is specifically for maintaining key value pair
and we're in Keys would be unique and if you try to map and existing key with a new value,
you would see that the value is been overwritten, but you won't have to kick it keys. Right again.
There are different variants that we would look at map has hash map which is similar to Hash set
which is based on indexing or hashing. All right, you have linked hash map, which is pretty much similar again.
Hashmap doesn't maintain the sequence of insertions linked hashmap maintains the sequence of insertions. There is a sorted map which is again free map
and which is sorted on based on Keys. All right. So the structure
that you see here is pretty much similar to set right we had hash set we had linked hash set and we had preset
so this one is pretty much on the same lines as set So how do you put data into your hash map? It's with put method,
right you have put method and you you put some key and value. All right, we have put all we can have an existing map. And if you want to put all the key value pairs
that they're into a map into a new map, you could use put all or you could remove some key. You could get some key.
All right, you could check whether a particular key is contained within hashmap. You could get your key all the keys
in the hashmap as a set, which is key set give you extract all the keys as set and as their unique already,
then you would get a new set returned out from he said entry said Returns the set view containing all key value keys and values.
So basically you would get all the keys and values as a set when it comes to entry set
from the entry said you could get key and value distinctly. All right, so get key is nothing but obtained
a key get value would give you a value against the key. Now. What are the typical exceptions
that you get when you deal with map some exceptions Throne while using map interface is no such element exception. So when you are trying to invoke some item from the map
if you're trying to invoke a key that doesn't exist. This is what you would get. Okay, this is more about when there are no items
if there is no item that exists in the map at all if it's an empty one, and if you are trying to retrieve something
that's when you would get no such element exception. All right Class cast exception is pretty much. It's a generic exception
that you get when you try to cast it against an object or suppose value is a string. All right, and if you try to cast it to integer you
would get a Class cast exception since string cannot be mapped into integer. All right.
Now pointer exception is the runtime exception which we saw that if You haven't initialized your hash map.
And if you try to put something into it, you would get null pointer exception. So you have to make sure
that you instantiate the map first and then start using it unsupported operation exception. This occurs when an attempt
is made to change a map which is unmodified unmodifiable. So you can get unmodifiable version of a map. And if you try to change something within it this is
what you are going to get unsupported operation. Hashmap linked hashmap and free map. So this is pretty much similar to the examples
that we saw for the set write Java hashmap class implements the map interface by using hash tables.
It inherits abstract map and implements map interface. It contains only unique elements hash map contains values based on the key.
It may have multiple null values, but only one real key. So this is important to note.
You can have multiple null values. But only in one alky you can't have multiple in alkies sonal is also treated as a key as a valid key
when it comes to hashmap, right? Whereas in hash table this one more variant of key-value pair,
which is Hash table, which would throw you an error if you have G as null but hashmap take ski as null so that's an advantage of it.
So hash map doesn't maintain the order of the element linked hashmap maintains the order of the elements. In which they were entered
and treemap sorts based on the key. So these are pretty much the same thing that we discussed for map earlier.
If you see entry set key set and all those things we have already discussed about and it's the same this something that we have in specific to tree map.
You could get first key. You could get lost key. All right,
since it is sorted you could get it now. There's a hashmap example. I create a main method first.
Then I create private map and then create. hashmap And I create a new map here say app of integer string.
Typically you would have integer as your ID right employ map. All right, and which would be new suppose. I create a new hash map.
Read so I'm creating a new map here, which is like the key would be integer your value would be string.
not put Buddhism method that we use and say employ map dot put Alright, so this is how you put into it put into map.
All right, so I'd written this map from here. So we so we'll take an example of hash map. Where in we would be putting some ID
and we would be putting some value as a string and we would check how it gets I treated
or how we can hydrate through or get something out of the set out of the map. Okay.
So here you can see that I created a hashmap. I created this method which is create hashmap and I put some value
within which is nothing but and you could see here. It's a map of integer and string. So I put a value of one
and I put value as X I put 2 and value as Y and I put a hundred and value as a right. So I created a map of employee ID and employ name
so to say right? Okay, so I've created this and so what we are going to do here
is so you could even print out a map. See if you want if I print it out like this like map to print you would see all
the values contained within the map. All right, so this is all right. I print the hash map right here.
So basically I created demo dot create hashmap. Okay, demo dot print map and let's print this map here. All right, so we have inserted three entries into this map 12
and hundred with ID 1 to 100 and which has got value of XY and a respectively And what we are doing is we are just printing the map contains
of the map right here. So you could see here is 1/2 and hundred been printed out here. Alright.
So again, this is a hash map and you could have it's based on hashing. So it doesn't maintain any order.
So if you have multiple values within you would see that the order is not maintained similar to what we had in hashmap.
So if I put something like this Alright now when I run it you would see that insertion order is not being maintained
and when you retrieve it, you will get it in any order. All right here we can see that the order
is not been maintained 112 is displayed first, which is second last actually. All right, so it gives you all the entries within the map
but it won't maintain the insertion order. So that's if you want to maintain the insertion order you have used linked hashmap.
All right, if I change this to link to map linked hashmap just one single change would sigh copy the same stuff here.
All right, and I put a link hashmap. All right and suppose I create one more which is 43 map. All right, so we have created three Maps
here one is your tree map. So the other one is linked hashmap link - map now. Let's print one by one, right?
The first one is hashmap that's already been printed here. Now, I create linked hashmap. Right?
And the third one that I print is demo dot create remap. All right, so we have all the three maps created and we are printing it here.
Alright, so here you could see that when it comes to hashmap. The key value pair is not maintained. Right?
Whereas when it comes to link the hashmap. This is a link hashmap and you could see that the order is exactly the same as the order
in which we put in. All right, and when it comes to tree map with the third one and here you can see
that it's sorted one. So sorted by your key. Not the value.
Alright, so see the key to the left hand side when it says 1 equal to X1 is the key and X is the value if you see it will see that
12 Levin 2,100 hundred and eleven hundred and twelve 1010 and 1100 so you could see that it's been sorted / the key.
All right. So this genome map in a map with specialized map implementation for enum Keys.
All right, which we saw here. We can see that there is a enum which is nothing but constants and you could see three constants been put
into the months, which is Jan Feb and March and you could see here is a in a map created
for all the months or for all the months that you have within your genome that is months right you have this in a map
and you could actually put in values something like this. So basically in a map is nothing but you could use the enums or the value within the enums as your keys.
All right, when you use in a maps and you could just write rate through it to see that you know,
you have this Keys put in and values put in properly. All right. So, so just to give you an example we can have
we can create an enum here. I'll choose to create them same one and I create enum here, right?
So I create in a month and which has got say for example, Jan Feb March. All right, so we have created this now
what I do is I create a new in a map with months and with string as its value, so I create a main.
Gina map fifth month and maybe we could put into your head. I create this in a map here.
All right in a map of our you have month and integer. Alright, so here we will have to give a month dot or even months.
Okay? Ticks class 1 class. All right.
So in the Constructor we could see that it takes class as a parameter. So we put one thought class as a parameter now I put ma'am
don't put I put like the the key is your it is depicting something like seals ton per month.
All right it basically it refrains you from putting anything but the enum value as your key. All right, so that's
what we are trying to do here and you could just print. In a map calendar and you should be able to see the values.
All right, so it's basically restricting the key as one of the values or one of the constants declared within your enum.
See how you can see here. Jan is equal to hundred fehb is equal to 200 you could. Now I will quickly talk about comparable and comparator
comparable and comparator are the two interfaces or it's used when you want to have sorted set or if you want to sort a collection say for example,
you have lists of integer and you want to sort it a list of integer is something that is by default could be done
because integer itself implements comparator, but if you have a self-defined class or if you have your own class say for example,
if you have your own defined class something like this vehicle info, which has like a number of Wheels mileage and name
of the vehicle put in now if you want to sort this particular class if you give it to a sorter,
it won't understand because this is a self-defined class straight. It won't understand
what you want to sort in this right. Basically when you have something of this sort when you have your own defined class and you want to sort it,
that's when you use some variable and comparator. All right, so I'll just walk you through it so comparable. Phase is used to sort
the objects of user-defined class in an order. So to give you an example suppose you have an employee class
which has got ID and say salary, right? If you want to sort it by default. Java wouldn't know
what you want to sort within that particular class, right? You want to sort it by the identifier or the ID field within the employ
or if that's the use case, then it could be like you want to understand the order in which employees
joined the company and the other case. Could you want to sort it by salary to understand which employees taking the most salary
or to understand the sequence in which salaries been paid to the employees, right? So to make Java understand
which use case you are trying to solve based on which identifier or based on which property
or instance variable within your class. You want to sort to just indicate Java that this is what we want to do express it
in a form of implementing comparable interface. It is in Java dot length package and it contains only one method that is compared to it provides single sorting sequence only
that is you can sort the elements based on one data only you can't have multiple data consort based on multiple data.
All right. So I'll just walk you through this which would give you an example.
So so basically you have a student which implements comparable now here you could relate to the example
that I took wherein you have roll number and name now you want to sort it. Now what we are trying to sort here is by rule number.
So that's how you express using comparable. Right? You have comparable student, right?
And you could see here. There's a compareto method that this comparable student has got all the comparable interface
has got now compared to will take student as a parameter. All right. Now you could see
that we are comparing rule number right you're comparing the rule number of the parameter student that's been passed to the compared to
if it is equal then it's 0 right you don't have to do anything if it is greater,
then you written as 1 and if it is less then you return as minus 1 so basically based on this compared to Java will do sorting All right,
it will sort it based on whatever you provide here. So what you are trying to tell Java is if it is equal we are returning 0 so this is a contract.
Okay. This is what we need to actually this is how it's been coded or this is a contract
that you need to follow whenever you compare to. So if it is a real number then you say it's rule number 0 or return 0 if if both the real numbers match
if the one in your instance is greater than the one being passed here. Then it would written one or else - one.
All right. So how do we use this? So basically you can see here you created student.
All right, so they were like three students created 101103 and 102. You could see three IDs being created here.
All right. Now what you're doing here is collections dot sort and you're passing your array list of student
and you use collections dots Not right when you do collections dot sort what it would do is it would arrange it
or it would sort the array the order of rule number so 1 0 1 would be first 102 would be second and 103 would be the last.
All right, so there's comparable and this comparator. All right. There are two interfaces comparator is nothing
but it is used to order the object of use again. It is used to order the object of user-defined class. But what is different
in comparable is comparable takes compared to which takes just one parameter as input and the other parameter is nothing but the instance
within the class itself. All right, although this instance rather the instance
on which this particular method or instance on which compareto method was called. All right.
So basically comparable will have only one method compared to all right, whereas comparator
when you use comparator interface it will it will take two methods or it will take two parameters in the compare method.
All right, and it will compare both the parameters that are passed. So basically it's
for customized sorting in the first example, that is comparable. We are putting the logic of comparing
within the class itself, but you have a separate class which implements this particular comparison logic.
So basically you could see here right you have a class student and student is just holding the values of roll number name. All right, you don't see
how you don't see the comparison logic with them. Now you have another class which is named comparator which implements comparator.
Alright this name comparator which implements comparator now if you see the compare method, it takes two parameters,
the earlier one the compareto method incomparable was taking just one parameter here. It is chicken two parameters, right?
And what you could do is you could simply compare based on name right since name is a string you could do S1 dot name dot
compared to S 2 dot name. This would compare based on name. There's another one you could have one more class created
which is nothing but rule number comparator which implements again comparator. Now here you would use the same logic
that you defined earlier for rule number. All right. If S1 dot roll number is equal to equal to S
2 dot roll number return 0 else if S1 dot roll number is greater than x 2 dot roll number written one or else
if it is less if S1 dot roll number is less than S2. Total number written minus 1 So
based on this logic you would see that the compare or you would see the Sorting Happening Now, how do we use this?
So we have defined a class. We have defined a model class. So model class is nothing but a class
that has got just the state right student. If you see student doesn't have any Behavior as such it doesn't have any method with them.
It is just a state. So this is a model class. Alright, so student is a model class
which has record roll number and name and we have two separate comparators defined here named comparator, which is comparing the name and we have
a rule number comparator, which is comparing based on rule number. Alright now, how do we use it?
This is not sorting at yet. Right? How do we use it?
So how do you use it is nothing but you have collections dot sort. All right.
This is pretty much similar to proceed other part of the program. This is just about putting the data into your class.
All right, so you have 1 0 1 put in as Vijay 106 put in as a J1 05 put in as Jay. Alright, and now we are trying to sort this
based on rule number as well as name. So both this can't happen in one go by the way. All right.
This is like first you can have something done on rule number and so it's not in one go. All right, you could do it in step five manner.
So as you could see here, how are we using this comparator is nothing but you have collections dot sort
and then comes the collection that you want to sort. In this case.
It is a L2. All right, and then you provide the comparator. So here you are providing new name comparator.
Alright, so after This particular statement is called you would see that the collection is been sorted based on name.
All right, and the first one here as you could see collections dot saute L2 and here you are passing roll number comparator
in the first statement or here the first collections dot sort statement would sort based on Roll number.
Whereas the lower one would sort based on name. All right. So this is basically offloading
or just decoupling your comparison logic out of your class. That's when you would go with comparator.
Whereas you would go with comparable when you want to put the logic within the class itself. All right, so it depends on what use case you want to do.
But as far as the performance is concerned, it's pretty much the same. All right, even
if use comparable or comparator, it's pretty much the same. It just depends on how you want to write it the programmers wish so few people refer
to have modular programming rather than coupling everything into one class. They would go with comparator
and few people like to add things to the class itself, which they would go with comparable. So what is comparator
and comparable comparator provide single sorting sequence? That is we can sort the collection on the basis of single statement such as ID or name
as we saw that you would have just one compareto method and based on that. You would have the sequencing done
or the Sorting done. Whereas comparator you could have multiple learning since you could have multiple classes
implementing this comparator and you could write your own logic like in the previous example,
we saw that we had a comparator based on rule number and we had a comparator based on name as well comparable effects original
class comparator does not affect the original class. So we saw the student class right the student class within the student class itself.
You define the compareto method when you use comparable, whereas when you use comparator you wrote different classes. So that's about decoupling
that I mention comparable provides compare to method to sort the elements and comparator provides compare method to sort.
All right comparable is found in Java dot Lang package and comparator is found in Java dot util package. We can sort the list of comparable type
by collections dot sort lists and we can sort list of elements of comparator type by collections dot sort lists comma comparator method.
All right, when I say list, it's a list of some type, right? So in the previous case, it was list of student
and since student class itself at the logic for comparison. We don't have to mention it explicitly taken care of by itself.
Whereas in this case, where an you use comparator and you had different classes where in you put the comparison logic in that case,
you have to explicitly give the comparator right? Like we give something for rule number. We instantiated rule number comparator
and gave it for sorting by a rule number and we instantiated name compare. There for sorting by name.
Alright, so that's how we sort user defined classes. So basically why do we have comparable and comparator just to reiterate but jvm or Java wouldn't know
how to sort a user-defined class, right? It could be based on rule number. It could be based on names.
It won't know by itself what you're trying to do. So that's how you using. This interfaces you express your logic of comparison.
So what is XML so Henry handles the database of a college but the data is stored in a form of XML file. He wants to extract information from this now.
He is learning XML so that he can handle it easily. So what is XML all about? It's extensible markup language.
It is designed to store and transport data XML has hierarchical human readable format XML is platform independent
and language independent. Why did XML come into picture at the first place? So it's basically you have different systems Mauro
you might develop some system or you might have some service that is exposed to the outside world. So what happens is they has to be some contract you as a client
or you as a service provider. First of all would expose a contract saying that if you give input to my service in so-and-so format,
I will give you output in Swann Format, so basically when you want to send data from a client the service you would send it in a specific format
that the client understands or the server understands. So the server exposes or the server expresses the input format
in a XML form and this is platform independent. This is like you might have a service tomorrow in created in.net which can use the same XML
as used by Java program as well. So it's a platform independent thing. Basically, it's used for carrying data
as I said store and transport of data, which we'll see in the coming slides but you could imagine this as nothing but a file
which is used to send data from your client to the server for communication. It's pretty much human readable.
It's not like yesterday we saw but serializing and serializing the state of the object which was not human readable.
So this one is pretty much human readable you could imagine like you would have an employee ID or You want to start something in the employee directory
and there's a service which is exposed for that you could imagine that you could send the ID to it
or you could send an employee name to it and format in which it is sent would be pretty much human readable.
You could see that okay, there's an ID there is a name which is sent to the server and the server is doing so
and so things so it's hierarchical and it's pretty much human readable again, as I mentioned platform independent
and language-independent. It's agnostic of all these Technologies. So there are a lot of existing Services
which use xml's as a part of input data and output data. So as we go through the examples, you should be clear to you guys about what XML is all
about why we need XML XML is an industry standard for delivering content on the internet. So it's a standard.
So most of the services most of data entry communication that's happening within in Annette is done in the form of XML.
They communicate with each other in the form of XML XML is designed to store and transport data XML is the extensible
because it provides a facility to Define new tags. It's not that once you define it. It's all done.
So it's extensible. It's extensibility feature wherein I can say today, my server is accepting employee ID and employee named tomorrow.
My server want to accept employee salary as well. So you could add that to it add tags to it, which makes it extensible.
So it's not one time Define. You could change it. You could evolve the xml's XML is used to describe the content
and structure of data in a document. So it has got its own schema and you can say that my XML is going to contain just email ID
and employee named nothing else and you could validate VL XML or the actual XML that you have against the schema
and if you Some extra parameters put in it. Would throw you an error. So that's about white need XML.
Let's talk about what are the features of XML? Why is it so widely used writing XML is pretty much easy. As I said,
it's human readable and you could actually write it and there's a lot of API or lot of sdks that are exposed for reading
and writing with xml's and very optimized version of it. So you could see parses, right? We had multiple parses.
It's for Performance Based on the needs of your particular application or the nature of your application.
You would select one of the parsers. So writing xml's is very easy. The other one is XML data can be extended with DTD and xsd.
It's a schema description. As I said, you could extend it. It's not that it's one time Define.
You could extend it XML can work on any platform this platform agnostic. You could run it on any platform either Dot.
Or it could be different languages or different platform. It could be different operating systems as well. I could run it on line X tomorrow
or I could run it on dotnet any tool can open XML file and can parse it in programming language. So simple editor or even notepad you could open it
in notepad plus plus and just you through it and there are different tools available in the market as well, which would give you kind of format your xml's
so that it's much more readable. So there are a lot of tools available already XML separates data from HTML.
It separates the actual data from HTML code XML simplifies data sharing. So basically as I said it
pretty much intercommunication standard between systems, you just have to put it into XML and you share it across systems XML simplifies data transport.
What is the difference between XML and HTML so XML is used for storing data and data communication. So as I said inter system communication standard
and HTML is used for display. So whatever you see on the web is something that is you have a HTML page you have coded it in HTML format
and it's used for displaying it XML uses user-defined tax HTML has its own predefined tags. So when you define your XML, it's a user-defined XML.
As I said today. I might have a service which is taking employee ID and employee name
which is like user defined which is not predefined. Whereas when you use HTML HTML is nothing but it has to get parsed
into or it has to get past into a page which has been visualized by clients. So that's why you have a predefined tags.
You cannot have anything you cannot put something of your own or if you put your own tags, it would show up an error.
So it's validated reason being you have to compile it or you have to run through to show it as a view or show it as a HTML page with the plants.
There's an interpreter which has to understand what you're trying to do. So it's predefined
tags XML is case sensitive and HTML is case insensitive in XML. It is mandatory to close all the tags. So you cannot keep any tags open.
So we'll talk about the tags. Once you see the format of the xml's but basically if you have a every property or every data
that you want to send out will be enclosed in a tag you'll have to ensure the tag is closed. So for example employee ID right employee ID will have
opening tag and it will have an employee ID as a value and you will have to close the tag you have to ensure that employee ID tag is closed right after the value in HTML.
It's not mandatory to close the tax all the time XML is dynamic because it is used to Transport data HTML is static
because it is to display it it. So basically when I say, it's Dynamic it's extensible. So tomorrow you could change it.
You could change the format or you could change add few more elements or few more tags or add some attributes.
So it's pretty Dynamic would change it whereas HTML is aligned to a proper predefined tags and you can't change return.
You cannot add some tags additional tags of your own XML preserves white space and HTML does not preserve white space.
So what are the rules let's talk about XML rule XML considers white space as the actual data. So whatever you are sending data through XML.
So imagine you are sending data between two systems, right? So space is also a character when it comes to XML. So suppose you want to send some data
that is client is typing. So of course you need To have Space Center as well. You cannot have spacing node.
It's actual data between two systems communication between two systems and since space is valid data. It's been considered as data by XML ordering
and nesting of XML document should be proper. As I said, you have to ensure that once you open
a tag you have to close it there could be nesting so there could be a tag with says employ and within employed tag,
you might have employee ID tag, and you might have employee name tag. And once you have this employee ID
and employee name tag, you have to ensure that you close the employee tag. So that's nesting.
So your nesting ID and name within employee tag. So your this might be really confusing at this point. If you have no idea
about what the structure of XML is all about, but don't worry in the coming slides. We have examples
and it's pretty straightforward XML tags are case sensitive every opening tag must. Have a close tag else XML be not correctly function.
What does an XML file consists of it has one root element that is one tag, which encloses the remaining tags.
So it has one root element within which the entire content would be and each elements consist of start tag content tag
and an end tag. So there is one of the components of XML is nothing but element
within an element you would again have some content expressed in form of element again, which will have start tag content tag again
and end tag. This is about nesting it will see in the example which is pretty much like readable format XML tracks
are case-sensitive opening and closing tags should be exactly the same without any difference in the case.
So you have to ensure that it's been enclosed with the same case as it was started off.
This is an XML. So the first That you see is nothing but XML declaration. The first line that you see here rather is nothing
but XML Declaration of prologue. So this is nothing but kind of saying what the XML is or the format in which it is encoded here.
You could have your dtds which we'll see in the coming slides but so kind of summarizing your XML what the XML is all about.
That's your Declaration of prologue and that's mandatory. If you don't put it it would throw you an error then comes the student tag,
which is user defined you could imagine this as a user-defined XML, right and you could imagine this as data being
or you could have a service a for example, which is doing something with this data may be inserting to a database right?
So you could imagine this being sent from client or you have a webpage say for example, wherein you enter first name last name
and email address of a student and say for example that comes to a server and it's loaded. To your database.
So the root element of the document is nothing but student here, you can see a student tag.
And as you can see here, it's an element which starts with angular bracket then the name
of the element and it ends with the angular bracket. So this is student and then the next line is about you define three tags within it.
This is nothing but the content of student and here you can see it's following same strategy or it is following the same structure.
You have was named Henry and disclosed the first name element. You can see the second element as last name and with starts
with angular bracket last name and and the angular brackets. Then there is actual value lie, and you could see that it ends with the tag
or with the element last name. So one thing to remember here is about start and ending of a tag ending of element.
You can see the right after the value. The element is closed and you can see This thing here as well. So student is a element
and you can see first name last name and email being nested into student element nested or they are the child elements of the root element.
So you have child elements defined here and you have the last line which defines the end of the root element here.
We have XML which has doors or which is used to transport. First name last name and email address of a student across systems.
Let me show you the tree structure of this XML so it could be imagined as student being the root element and you can see that first name last name
and email is nothing but your child or is the child for student since it's a root element and you could see the values
the actual content of it within your first name tag, you saw the value Henry within the last name you saw the value lie
and within the email address you saw Henry 123 at gmail.com. So the leaf node is nothing but the content of elements or the actual contents of the XML
that you're trying to send across systems. This is pretty straightforward. Like you could realize XML in a form of free format
where in your root node is nothing but element of your XML and the leaf nodes or the leaves are nothing
but the actual value stored in the XML. So let me talk about three rules in this given example student is a root element.
Then first name last name and email are descendants of student. This is pretty much straightforward
when it comes to tree so you have a root node and you have descendants and we can see from the structure
and sisters in this example student is ancestor of all other elements, so root node,
so it's ancestor of all other elements within this tree order XML attributes you can have attributes.
Which are common across or you could have some attributes defined within an element.
So here we can see that message. That's nothing but an XML with the root node messages and you could see individual messages within so
there are two messages here within messages Tab. And the first one is to any and it's from John and you have a body there the actual body of the message
what you want to send and you can see here this ID or this something that has been provided as an attribute,
which is nothing but you could see Mi di is equal to 1 that's attribute has to be enclosed within single quotes or double quotes.
So whatever you see within the element and closed within codes that is nothing but attribute so modifier,
you can say some kind of a modifier to your element. So attributes add more information about the element it is adding some more Formation
to the element XML attributes must always be coated either. It should be within single quote or you could have it within double quotes here.
We have it within double quotes for our XML comments. So to make it more readable or to increase the maintainability of an XML
so that someone else can understand what you are trying to do you could have comments put in so this is pretty much similar to comments in other languages.
The reason why we rationale behind why we put the comments it's more about make it much more verbose and understand what bag is all about how we comment
is nothing but you have opening angle bracket. Then you have exclamation mark then two hyphens and after the - you have the comment and
once you have the comment you close it with double - and then closing angle bracket. So one thing to note here is exclamation mark,
is there only when you start the comment and at the end you don't have Exclamation mark double - and then closing angle bracket similar comments are just
like HTML comments comments are used to make the code more understandable. What are the rules?
Do not Nest a comment inside the other comment? Okay, you can't Nest it. And I don't see any need
to nested was your just expressing it or we are just adding comments to make it more readable. So if you nested it would give you an error do not use comments
before and XML declaration XML declaration should be the first line. You shouldn't be using comment before that comments can be used
anywhere in XML file except attribute value. So you can't put comment within an element or you can't put it right
after the element is defined or say, for example, you have this message tag, right which starts with angular bracket and then message
and right after the message. You can't have comment your element tag has to be closed after this closing angular bracket you could Any comment
but it cannot be within this space wherein you define the attribute so basically comment can be put anywhere
but it cannot be as attribute value. So let's see a well-formed XML and what do we call well-formed XML
as there must be exactly 1 root element and XML should have exactly one root element. You cannot have multiple every start tag has a match intact.
So we saw in the previous example, like if you start student you have to ensure have an intact for student as well.
So that's what it is about every start tag must have an end tag attribute must be coated either with single quotes or you could have double quotes comments
and processing instructions may not appear inside a tag cannot be inside a tag and you have opening angular brackets or
and must not occur inside a data element. Your data cannot have this as value or your data element cannot Contain this as a part of the name of element.
So let's move towards XML validation. So well-formed XML can be validated against DTD or xsd.
So communication between multiple systems you have to ensure plant is sending data or is kind of you know, preparing an XML
which is aligned to what server expects right? So that's where you have decreed in the and xsd. Let's see.
What is D TD. E TD is a EB NF grammar defining XML structure. It's a normalized form at grammar,
which defined XML structure a DTD defines a legal element of an XML document. So it says what XML can contain what an element could be
or what the attributes could be and it's a legal element. Okay. So basically when you define
a DTD you can expose the service today, which could be used by Tomorrow right? Google can be using it for some purpose.
So this is a legal document saying that my service expect or accepts the times 1 so format, so you have to send it in so-and-so format
for us to process it through. All right. So it's a legal document between multiple organizations
XS T is nothing but used to address the shortcomings of DTD uses name space to allow for reuse of existing definitions.
So you could Define an xsd and you could just use it or through name spacing you can have it within other pieces of xml's as well.
So, let's see. What is GT D PT d stands for document type definition. DTD is used to define structure of an XML document
or DTD defines the legal elements of an XML document as we spoke. It defines legal elements,
or which cannot be Accepted so basically you define what your XML is all about through DTD so you could see a student DTD here.
So you have XML which is nothing but which has student which has first name last name email and marks.
All right. This is what you have within your XML. Now you define a DTD
which could be put into your doctype. So the first tag that you see is nothing but declaration
and it's linking your kind of tying it against a DTD with that's defined external DTD file. All right, you define this
and you have a DTD file below which is student dot DT D. And here you can see that it says that within student element you can have
for other elements or as a nesting of for other elements, which is first name last name email and marks. All right, if you
since this DTD is linked with your document or with your ex. Mel document now if we try to add something if you have one more element
here which could be like a dress right? If you have one more element here, which is address.
It would throw you an error because your ETD says that student can have only first name last name email and marks address is not a valid element within student.
All right. First name now, let's define. What can we have in first name?
Alright. So first name is nothing but character data again. Last name is a character data email is again a string
and Marx is again taken as character sort of so this is how we Define DTD. Alright, so we are trying to establish the structure
or we are trying to say that student can have a nice one so fields and if there's something else then it's invalid.
So here we can see ETD contains root element and declare the child elements we can see That it says student can have only first name last name
and email and marks. It defines last name child element and data from this element is possible right
when it is PC data. It's possible again you have first name, which is possible you have last name,
which is possible you have email address which is possible and you have marks which is possible.
All right. So what is XML schema XML schema is used to express constraints about XML document.
All right, it's pretty much it does the same purpose as DTD but it's much more advanced than DTV. It has much more features XML provides more control
on XML structure a well-formed XML document can be validated against xsd. So you have an xsd again similar to DT
which defines the structure and a well-formed XML should be validated or should be Against a particular axis T.
So here we see an xsd, which is much more verbose or which is much more clearer
to understand compared to DT DT D was something like was not expressing to that extent but X is T is pretty clear
in terms of what the content could be. Again. It defines the root node and declare the child elements.
So here you can see that exists is defined in a form of XML itself. All right, it follows the same pattern as the XML.
So it says element which is a keyword. All right element is a keyword for your xsd and you have student as a attribute.
So this you could realize xsd as I said as an XML itself, it's a valid XML cracker, which says that you have an element with name student now
what can students hold is again a sequence when you say sequence it has to The same sequence break the sequence it's again going to be invalid
then it says first element first child element within student should be first name. The next one to follow should be last name.
The next one should be email and the last one should be marks. All right, and it defines what type is
particular elements hold. So first name is a string. Last name is a string.
You may raise a string and Marx is a string as well. So this is how it is you define a structure to the XML and you have to ensure for your XML to be valid.
It has to be valid against this exists e it's a similar scheme are descriptive. So what's the difference between DTD and Xs T DT
v stands for document type definition XS d stands for XML schema definition. DTD is not extensible access T is X Zebra dpv
provides less control on XML structure xsd provides more control on XML structure. ETD does not support data types xsd supports data type.
We sort the data types like string and stuff which is like it supported just in exist. You don't have DTD supporting
and DTD does not define the order of child elements as we could see it just says that you could have four elements within but it
doesn't have any specific order as such whereas when it comes to xsd. You could Define a sequence right as we saw
in the previous example, we said that the first one should be the first name, then the last name then email ID and then marks
so you could Define the sequence in which your sub elements should occur XML CSS file CSS is used to add more tiles to the XML document.
So if you want to color coded or do something that's when You CSS pretty much similar to HTML document. So here we can see that you could Define student
which has got a background color of pink. Then you have first name, which has different font sizes here.
First name last name and email ID and marks which has got different font sizes. So we want to have font size of 25 for this child elements.
You want to display it as a block and color as white and the margin left as 50 pixels. All right,
when you have such thing defined if you want to link it up if you want to link CSS to XML, you need to have a XML stylesheet tag defined
and you would type as text or css and you put H ref as your CSS name to make the changes in the styles
of the XML file CSS file is linked to XML file through this statement. So this is how you link your CSS file to your XML file.
Now. What is XS L XS L stands for extensible stylesheet language XSL navigates
all the nodes and elements and display XML data in a particular format. All right, it's basically
for parsing sort of it navigates through all the nodes and elements queries can be specified in XSL. If you want to query some particular data
or like we had message this thing in a couple of slides back. We saw multiple messages within message, right?
So if you want to read some messages extract the messages that are sent by the users you could wear it out using excessive it displays data
on the browser as per the format given in the Excel file. So, let's see what is accessible file all about.
Okay. So here what you're doing is nothing but you're extracting data.
All right, you are. Acting first name. So the first name here would be taken from your student
and would be displayed here. And again the second one you have last name you select the last name particularly.
This is acting upon student dot XML. All right, how do you link up accessible to XML is pretty much similar to CSS.
You have style sheet and you have type as text / XSL and not CSS, right and you have H ref as student accessor.
So that's 4X s l-- and again, let's see. What's the difference between CSS and Xs L fi CSS files are easy to understand
Excel files are difficult to understand CSS is less powerful than accessible CSS is specifically for display right changing the font size changing the colors background colors
and foreground color and everything XSL is more. Full then CSS since you can extract data or do something with it.
Basically, it's a processor. You actually can process the XML using Excel CSS does not use XML notations Excel uses XML notations,
which helps in writing code. CSS can be read by modern web browsers XSL is not supported by many web browsers.
Now, let's talk about XML parsers. Okay. So XML parser is nothing but you parse through you
have an XML and you want to read data from it or XML parsers could be used for writing the XML as well. Okay, creating an XML could create an XML
using XML parsers as well or you could read through the existing xml's. So an XML parser is a software Library
which provides client applications to work with XML document and XML parser is used to check whether the Comment is in well format or not.
It is basically used for compiling our parsing your XML as well. It is used to validate an XML document
and parsing XML refers to going through XML document to access data or to modify data in one or the other way.
All right, you could actually read through the xml's or you could extract data or you could modify data using XML parsers.
What a bar says here. You could see XML document right being sent to the parser parser is nothing but API
so you send the XML The Source XML or the XML that you are trying to pass is going to be sent to the XML parser
and this API is going to be used by client application right to parse the XML files. So XML parsing could be broadly classified
into two different types one is object-based and one is event-based right object-based is nothing but document object model or it is also known
as Dom even base. We have two different parses when it comes to even based one is sacks
and the other one is tax. All right, so let's talk about object based model Dom parser. It stands for document object model.
It is an object-oriented representation of an XML parser tree. So remember we assign XML
and we passed it into a tree like structure where in your root node of the tree was the root element from your XML
and we had all the elements and at the end the leaves were nothing but the values or the data
that the XML stores actual values right or XML stores or care. Rather, so that's what trees all about.
It defines the standard way to access and manipulate documents Dom is a common way for traversing the tree. So as we look through the examples,
you should get Fair idea about it creating an XML document using Dom parser. So what is required to use Dom parsers?
How do we do that? First is you have to import all the parsers. You have to import all the classes
that you have within partial Java x dot XML dot parsers. These are all input statements that you have.
You have to import different packages which when you use IntelliJ or Eclipse, it would prompt you to import
it it would suggest you to import it. So you did not actually write this input statements, but it's very important
to understand what we are trying to do here. So these are different packages that we are trying to import and as you could see all your XML related classes.
Are put into Java x dot XML dot transform or basically G of x dot XML package.
So once you import it, you can start using the classes within the first thing that you do is when you generate an XML,
you have document Builder Factory, so you have document build a factory dot new instance. You create a new instance of it
and you get document build a factory that's instance of it. So now with the document Builder Factory instance that you got you would create a new document Builder Okay.
So this is a factory design pattern. We have a design pattern in Java and document Builder factor is nothing but it's following
a factory design pattern, right? You don't have to think much about it. But basically what we are trying to do is instead
of creating the instance of something on our own which is like we usually do with new and the class name, right? Sort of doing it on our own Factory design pattern is
nothing but the creation logic is written within a class which is not exposed to you. So you need not create it there's some class
which creates instance for you. All right, and that is nothing but a factory class then you create a document Builder.
So it's nothing but F dot new document Builder. Okay, you handle exceptions and everything here. So now let's start with creating the actual document.
You have the root element as students. All right, and within student you have student within students
you create student and within student you have like first name last name email and marks and you could see first name put as Henry.
So how do you create element is nothing but using dog create element, how do you create X node or text node
or the actual value that you have? Then your element you would do it with create text node, and you could Nest it using appendchild.
So basically you have first name appended to T1, which is nothing but this one right T1 is appended to the first name first name element
that you have T2, which is the text node, which is holding. The last name is appended
to the last name element p 3 is appended to email element and the for which is your marks which is appended to Mark's element.
So basically this is how you make nesting of your nesting within the xml's. Alright.
So once you create this document you append all the child's and everything you create a well-formed document here. Are you create an XML here and all the XML
that you would get is within this dog, since we are creating element you could see here we are. Getting elements within doc creating elements and text node
and everything within dog. So at the end once you have all this done, you would have a well-defined XML stored as a form
of document class. So we want to print this particular document in a text format,
right which could be readable by us or do you want an XML out of it? So that's when we use Transformer.
So there is a transform Factory dot again since it's a factory design pattern. It's exposing a method
which is nothing but new instance. So you create a new instance of Transformer factory and you create a new Transformer.
So at then what you get is nothing but a Transformer and what are we trying to transform is nothing but we are trying to transform the object
or the document object that we created. We are trying to transform it into a human-readable text file or the XML file all So
that's a transformation that we are trying to do here. So here you could see that you transform it TDOT transform
and you pass the document object that you created and put the element into element and text notes
and everything into which is holding a valid XML. You are trying to transform it into a XML file. So how you do that is T dot transform.
Then you have the new Dom source and you specify the instance of document which holds your XML then you have stream result
and new stream result class which takes file output stream as its parameter. So you have to pass the parameter where you
want to write this XML to and when you run this at the end, we are just printing that XML file is been generated. The first thing that we do is we are creating a document
and using the document. We are creating elements the wave that we want. So in this case,
We have a students as the root node and within student you have student and within student you have first name
last name email ID and marks, right? We created this structure till here. And as you could imagine this is just dealt
in the form of object. Right which is stored in dog. So now since you want to write it into a file,
which is into a human-readable form or which could be passed across Network. You have to transform this into XML file,
which could be read. So that's what we do here through Transformer. We are transforming your document or the XML
which is in the form of document into a file. So, let's see this program. In the meanwhile, we can do one thing.
I will create one more directory here. At Eureka XML. Okay, and we don't have any files with them.
Yeah, let's hold on for a minute. Yeah, I just come up. All right, so it's the same program that we spoke about.
So I have loaded into my ID. So we do the same steps here. We create all the elements all the required elements and stuff.
So we are writing it into a file right so you could have your own file here, whatever file you want to write into.
okay, so I right into the directory that we created which is nothing but Ed Eureka XML we are writing the XML file there.
All right, let me execute this program. It's building it and if it's generated you should get XML file generated at the end.
So this is about using Dom. All right. So when you say Dom it's nothing but document object model
its building it. So hold on for a minute. In that see I think it should come up soon.
Right. I think it's coming up. Alright, so we have this program here,
which is kind of creating XML document. This is same as what we spoke in the slide. Right what I walked you through.
So basically you have students you're creating students elements within students element you are going to have student
and then first name last name email and marks. Alright, we are going to create a text node within this elements.
So this is nothing but your first name, which would go into the first name element. This is the last name
which would go into the last name element that we created earlier. Then we have email
and marks going into a prospective element. Alright. So basically we are nesting it here at the end.
We have the Transformer which is kind of taking the document and converting it into XML file that we want.
So let me go and delete this off and run the program. In this folder, we don't have any file right I run this program from here.
So you could see that it says XML file generated. All right. So let's go to the folder and see up there is a file
that's generated that is student studying similar. Now I can open this with any tool say I open it with notepad
and I see something like this right now. I want to format this I want to format this into because this is like one single string,
which is Big right? So if I want to format it, we have tools that are online,
even you could format it online, right? So I put this and I say I want like three spaces or indent level which is good
and I formatted okay when I click the format. You can see
that it formatted the entire XML for me. So the basic thing that it's doing is just about formatting this XML.
All right, this is much more readable right after formatting. You can see that it's readable can see first name as Henry Lee
and Henry 123 at gmail.com and you have 70 put in here, which is what we expect out. Okay.
So we saw this output after running the program Now quickly. Let me run through what's Dom parser and how do we use Dom parser for parsing and existing XML?
All right. So these are the classes that you need to import which would be suggested by your IDE as well
either IntelliJ or Eclipse. So you have document Builder Factory you use the same thing get the new instance from the factory
where in you get an instance of document Builder Factory with this you create document Builder and once you have the document Builder you parse it
Boca parse method The document Builder and pass the XML file name, right? So whatever XML you want to pass you would send it across now.
Once you have this past if you want to get the node name if you want to display something like what's the root element within your XML you could do something
like this talk dot get document element and get no name. So this would basically display the first element that you have within your XML,
which is nothing but the root element right you could read this on the name. You have the tag names or element name.
So you have methods like get Elements by tag name and you could give the name of the element
and it would retrieve it as a node list. Now, you will have to I trait through this node list to display the contents of it.
All right. So here what you do is you I trade through it. So when you get a node list,
basically you would get list of notes. All right. So now what we are doing is we are printing it out one by one.
Printing the nodes are the contents that we have within one by one. So in this case,
you can see that when you do student dot get Elements by tag names and you can see student here. So this would give the number of student
that you have within a document the previous example that we took the number of element is just one right we just had entry for one student.
So it would I try it. Just once in this case it would I try it just once all right. And what we are doing here is we are kind of taking
again element by tag name and these are nothing but your actual values or the first name last name email
and marks this Loop is going to run just once since we have only one student and this is how you display the content of an XML.
So basically what we are doing is we are parsing it when we say parse we are trying to get data or we have trying to load data into Dom or document.
And we are printing it out. Why do we have parsers is nothing but to retrieve data if you want to query out particular data,
you could do it. You could write some logic to read out a particular data from an XML.
Say for example, you might have a long XML and you want to read data pertaining to student Henry.
So what you could do here is you could write some logic here saying that if student is equal
to Henry then written mock secured by Henry. So that's why we have XML parsers in picture to have it loaded into memory and play around with it.
Alright, so let's see the example of XML reading using Dom I give the path here as see Give the same file.
All right at Eureka XML. Student dot XML that we created or let me do one thing.
So I create one more student here. Say for example. Just for Simplicity purposes.
I'm just putting test last name as the last name test email and I put test Mouse. All right.
So these are the values that I have put for one more student that I've created
or maybe I will put this one as Just to be consistent. So we have two students now within so first one is Henry D. And the other one is
like we have created a test to let me show you when we run this program. What we are trying to do is we are going to read this XML
that we have created and it's going to parse it parsing is nothing but it's going to display the contents of it.
So it's going to Loop through its going to understand there are two students within and it's going to display first name last name email
and mock secured by each student. All right. It says the processing instruction.
Okay, we can see the output being displayed here. All right, so we can see two students here the current element and really calm
and re 1 2 3 gmail.com and 70 and there is one more student that we added directly into the XML and we can see it displayed as well saying that test first name,
press last name test email and test marks. So that's how we bars it. Okay using Dom parser and what Dom parser does is nothing
but it creates a tree if you remember I said it creates a tree like model that was there in one of the slides
that we discussed earlier. It creates a tree-like structure with a root element as your root node,
and your leaves are nothing but this values Henry Lee and all this stuff whatever is been displayed here or nothing but leaves so of your tree advantages
and disadvantages of using Dom it is preferred when we have to randomly access separate parts of the document.
So if you don't want to do it sequentially its preferred that you use Dom it supports both read and write operation. So we created XML using Dom
and we read XML using Dom as well. So the first example that we took about creating student dot XML was using Dom as well since it was document.
Remember we created object of document and then we inserted all the elements within that is nothing
but Dom it supports both read and write operation as we saw and what are the disadvantages of using it. It is slower than other parsers since it has to create free.
So it's a one time activity that it does. So remember in the second example that we took we saw parse here,
right the sparse here document Builder dot parse this is going to take lot of time because it's going to create a tree
if you XML is big enough based on the size of the XML it would take time because it's going to create tree.
Parsa's something that is going to take time and it's not very memory efficient since you could imagine if you have a big XML
so xml's in general are like it could have like a hundred million records within they are humongous.
They are not small examples that we are dealing right now. It's coming from different systems, right? So you could imagine their system pushing data
in the form of XML and sending it to some other system to process it. So it could be humongous could grow up to like
a hundred million records or something of that sort. So imagine that you want to load all this into memory, right? I'm create a tree.
So this parse method is going to take a long time to create a tree and the other thing is it's not memory efficient because it's going to load this entire document
into your memory, right which is going to take like lot of achebe's of memory right to load it.
So it's not pretty memory efficient when it comes to parsing using Tom. So that's the reason we have other variants
of parsing which is not document object model, which is basically event-based. So we have like two parses even base parser one is like
for push parsing it is sacks and whereas for will parsing it is Stacks. All right, so we'll just go
through what is sax parser and sax parser sax parser is nothing but it stands for simple API for XML sax parser reads XML file. Sequentially.
It's an event-based right? So when I say even based what happens is when a parsing event occurs,
the parser invoke the corresponding method of a corresponding Handler. So basically you define a Handler you say
that if I get so and so tag or if I get start of element tag go to this particular Handler. All right,
and this handlers are nothing but user-defined handlers so you could say that Not get a start of element tag. Go to this Handler and do something, right?
It could be as simple as printing it or it could be as simple as filtering it. So it's up to you to write the logic for the Handler
but what the framework does is nothing, but when you see a opening tag, it would give a call to your method.
Alright you Handler method sax parser is used when we have a large XML document. As I said in the previous example of you know
hundred thousand records that you have within XML file document object model is not that efficient
because it's going to load everything into the memory which is going to take a lot of time as well as memory, which is going to consume a lot of memory.
So it's not good in terms of time and space complexity. Whereas Sachs does a good job when it comes to large xml's when it comes to parsing large xml's
because it's not storing or it's not parsing everything into a object in one go. It's an event.
Just so basically you would see that it sequentially goes through the XML and as and when it sees something right start
of element start of an attribute or anything of that sort. It would give a call to Handler and Handler would process it. So basically it's not storing anything.
It's not retaining any information into memory. It is just calling the event handler and just leaving it right there.
So since it's not consuming memory, it's good when it comes to large xml's but it's not good for random search right random parsing
because it goes sequentially so for sax parser again, you could see the files present in Java x dot XML dot parses dot sax parser earlier,
it was document and here it's sax parser again. You could have this Imports done by your ID. This is what you event handler is all about.
Right? So you are defining a an event handler here, which is nothing but a default Handler
so Your XML reader using Sachs is nothing but a default Handler is a relationship is a default Handler you extended your extending default Handler,
which is maybe an internal interface to your sax parser. Once you extend it. It's not an interface or it
since it's extending it has to be class. All right, it's a class. So the default parser or the default Handler
would have this methods within which is nothing but start element you have characters you have end element and end document.
So these are the methods within right when you give a big XML in this example or when I say this one, so when it comes to student,
which is your root node, it would go to start document when it reads this XML. It understands that it's a start of document.
So it says document begins here. It would go sequentially this parser what it would do. Is it would read this entire file
and it would go sequentially when I say sequentially then it would understand that there is an element again
and it would give a call to start element and here what you could do is you could print it. So what we are doing here is nothing
but we are printing the XML document using sax parser. All right, which is event-based whenever a particular event occurs in your XML.
It would give a call to the particular Handler. So whenever there is a start of document in this case a student is a start of element again,
which would go into start element. All right, when does it come into characters is nothing
but when it encounters Henry when it encounters Henry, it would go into characters. All right, you see a character's method here.
It would go into characters and you can see that it's a character array. Alright, so here it
would print the entire array which is nothing but the value. Lou Henry and all this Leaf nodes. All right, Henry Lee and whatever values
that you have would be printed by this characters method whereas the tag names would be printed by your start element and end element.
This would be your end element. All right. So this is pretty much like it's an event-driven one,
whenever it encounters something framework gives a call to a particular method and this method is user defined
you could do whatever you want to so this is how you create a instance of it. So you have sax parser Factory dot new instance
dot new sax parser. You create the instance of sax parser. Now you parse it P dot parse
and you provide the Handler as well then let that you have provided which is nothing but XML reader using sacks.
That's your Handler. So you have to create the instance of it and pass it over.
Let's see this program now also you could see it is reading students dot XML, right? So I'll have to change this name to see.
Ed Eureka XML All right. So let's run this program which is the same program that I spoke about in the slide,
which is even based you could see methods implemented here and you could see it extending default Handler. All right.
So there's a default Handler this start document basically start document and everything is coming from your default Handler.
All right, and in the start element, we are just printing It characters and again when it comes to end we are ending it
and when it comes to end of the document we are just printing saying that document ends here.
Alright, so we are reading the same student file and this time it's using sacks. So you can see here
when it encountered start of the document. It printed document begins here. Then you could see elements printed sequentially.
So this is what it is. This is like event-driven right and at the end when it reaches the end
of the document you can see document and C are being printed out. So basically this is
as I said even driven and this is sequential, right it goes line by line. So if you say you have like a hundred thousand records
within your XML and say the last entry that you are searching for it's going to go
through the entire XML delete encounters the last any when it comes to random search or if you want to search randomly
if it's not well suited but when it comes to Big XML, it's much better than Tom since it's not loading anything. So it's not keeping anything into the memory.
It's just parsing it it just printing it out and it leaves it right there. So what are the advantages of Sacks, it is simple.
It is memory efficient. Sax parser is faster than any other parser. It handles large document.
We spoke about all this right here disadvantages of using is land will be unable to understand the whole information because data is broken into pieces.
So it's not storing it in one place. It's broken into pieces. It's not even storing it into memory just flushing it off.
It's just writing it and leaving it. So what's the difference between Dom and sax parser Dom stands for document object model Sixth
and four simple API for XML Dom reads. The entire document sacks reads node by node, which we saw sequential.
It would go Dom is useful when reading small to medium-sized xml's Sachs is used when big XML files need to be parsed.
Sax is good for big and this one Dom is used for Two medium sized Dom is tree-based parser. We saw it creates a tree and sax is event-based far sir.
We saw how it has event handler and you saw that it calls a particular method based on the event that it encounters Tom is little slow as compared to Saks and
because it has to create the entire tree and sax is faster than Dom once the tree is created.
I think Tom would retrieve it much faster than sacks. But the tree creation itself takes longer time, right when you parse it.
It has to create a document model which takes a lot of time Dom can insert and delete nodes
since it has entire tree it pre-loaded. You could actually go and delete it you have the entire tree loaded
so you can Traverse to any particular node and delete a particular node or delete a part of a tree itself,
which means that you could do insertions and deletions whereas sack Doesn't store anything in memory just reads there
and just pushes it off. So you cannot insert or delete nodes into your Source XML.
So basically it's meant for just doing something or retrieving information from your XML. It cannot manipulate the source XML sax parser is
cannot manipulate the source XML now we have one more which is Stacks. So in terms of sacks,
if you imagine sex, it's a push API. All right, when I say push API it's nothing but it reads through scans
through the XML and when it encounters an event, it pushes the Handler to handle it. So there's a push
from your API to the handler do perform some actions whereas Stacks it's a cool API, lets go through it to understand more stack stands
for Java streaming API for XML sax parser pulls the required data from the XML this Sax parser
maintains a cursor at the current position in the document allows to extract the content available at the cursor.
So basically it maintains the cursor at the current position in the document which allows it to extract the content available
at the cursor again, you could see Java x dot XML dot stream in the first one the Dom we had document the second one.
We had sacks. Now the third one you could see stream. So that's the beauty
of packages you could understand what files are there with them? Okay. So using Stacks you could see here.
We create an event handler XML element Factory dot create XML event handler and new file reader. So you pass a new file reader
and it's nothing but the XML file that you want to pass. Now, you could see that you get all the elements.
So basically you have even Trader and you get the elements of front or you get all the events that you have.
Then and XML. All right and UI thread through it. Now you have a switch statement
which would do the processing for you which would say, for example, you have a start element similar to
how we saw in the sax parser. So you have a start element and if it's a start element and even would be passed
and you could actually get the element value. So basically what we are doing here is we are getting all the events and we are iterating through it
and we have a switch statement which is handling the event. So instead of having the event Handler written in a separate class.
We are writing the event handler in the same class. This is kind of again decoupling right? So imagine yesterday.
We spoke about comparable and comparator. So comparable was like compareto method was written within your class right within your model class,
which was student yesterday. All right. And again, we had other example of comparator
which had a compare method which was Into student objects as an argument. So that's the difference.
Right? So we are decoupling it. We are writing the entire comparison logic
to a different class so we can we can imagine the same here. So that's what the difference between Stacks and stacks is Stacks is writing it within the same class.
Whereas sacks you have a separate class for event handling so you could see here pretty much similar to how we did in the sacks
so you can have like if it's a character then you have even - characters you print the characters when you
encounter and document you have event as in document and so on basically you get all the events
and you I trade through it and you have switched treatment handling the event. All right, so I take this example.
So the first thing that I'll have to do is change the file name. Ed Eureka XML and I just run through it.
Alright, so here we can see start element student. You have first element as Henry and so and so forth again, first name Henry and stuff like that again.
You can see the same second student being parsed as well which was a dummy student that we inserted
and at the end you will see end of the element student. Alright, so this is how we parse using three different parsers
and we saw about advantages and disadvantages and which one would be apt one per your use. All right.
So you have to understand the nature of the application or nature of the XML is that you are dealing with and based on
that you could decide which parser to go for so that's about the parsers. Now, let's talk
about XPath XPath expression is a query language used to select path of XML document based on the query string. You can create a query string
and you can retrieve Wait using X bar X power threatens a node set representing XML nodes at the end of the path red. So this is basically like wearing language
or you have X path expression, which you could write query in this example. If I want to get first name of a student with so-and-so marks
if I want to get a first name of student with 80% marks, right? I could do that using XPath.
So basically it's for querying right and it returns a node representing the XML nodes at the end of the path.
It is used to Traverse elements and attributes of an XML document XPath defines the structure and provides XPath expression.
So the 7 type of nodes that can be output of the execution of XPath expression
is root element text attribute comment processing instructions and namespace. We'll take a look at it expert defines a pattern
or path expression to select nodes or node sets in XML document what I expect Expressions you have node name,
which is used to select all nodes with the given node name you define a particular node name and you could select all the nodes within an XML document
that has named as node name. So it could be either element. It could be attribute or anything,
but it could be just element named rather. It specifies that selection starts from the root node. If you have 1/4,
it says that it starts from the root node. It specifies that the selection starts from the current node that match the selection.
All right, so / scans the entire document / / is nothing but the current node dot it is again select the current node.
So whatever node you are at during parsing, it would print it out dot dot is parent node. And at the rate is it selects attributes.
So if you want to carry out certain attributes like at the rate ID is equal to 10 say the employee IDs been stored as an attribute
and if you want to have employee ID with value hundred you could query out saying that at the rate ID is equal
to hundred steps to use XPath again. You have expert related packages. You have to import you have to create document filter.
You have to create an XPath object and XPath path expression create a document from a file or a stream.
So basically when you are creating document is nothing but it's a dom-based parser. Oh, basically it's not well suited for big xml's
for small or medium-sized XML. It's good enough because it's creating document out of your XML then fourth
create an XPath object and an XPath expression. Let's do you have to create object and you have to pass the expression
that you want to retrieve compile the XPath expression first, you have to ensure
that the XPath expression that you have provided is up to the mark and it doesn't have any error and you're following the contract
that XPath expression has I'd rate over the list of nodes. Once you get the result of the XPath expression you have to I'd read through the list of nodes,
which is nothing but the result and examine attributes and examine sub-elements, right? That's a typical way in which you would use XPath
so you could see here. It's again Java x dot XML dot X path. There is a separate package for it
and it will have all the classes related to it like expat Factory you have constants and you have like exception
and you have expert class itself here. We are again parsing students XML we pass it into Document first
as we mentioned the steps that we spoke about we create the document and then you apply the XPath you have expression here /
class / student. All right, and then you compile this expression what you expression
you have here you compile it and you then evaluate it so evaluation is nothing but you are retreating to the result and you are printing out the result
that you got to broadly classify. What we are doing here is we are creating a document object out of the XML file and the next step
that we do is we compiled the expression and then we are evaluating it evaluation is nothing but doing something with the result.
There are a couple of checked exceptions that you will have to take care of so you could see that it's been handled here.
It's not specifically handling. It is just printing out the stack Trace. So let me quickly.
The take this example. Alright, so from here go to resources. So let me go to documents downloads.
And it's let me run through the same example and see if it runs. Alright, so we have I take
the same example c letter c at Eureka XML Okay, so, let's see if it runs. It in fine dot txt size change this to XML.
Okay, let's try to run. So what I am doing here is pretty much passing this XML and then reading it through.
So basically just to again tell you I love to see why it's not running at this point, but it's more about you just created document model.
You have your XPath set or you have the XPath expression you compile it and then you evaluate it which is nothing but
whatever results you get. You can do something on it. All right, let me move on to the next slide.
Dom4j is open source, Java based library to parse XML document dom4j is flexible and memory efficient.
It integrates. Well with Tom Sachs apis, it should be used when you need the information
in an XML document more than once. So basically with sacks if you want to pass through more than once
if you want the information from the same XML more than once it's not that efficient because it will have to parse it again.
So it doesn't store anything. It has to go through it again. Whereas this one is something
that integrates well with dom and sax apis, which allow developers to use the information in an XML document more than once.
So what are the steps? So basically you have to download this dom4j dot jar from the website or from the GitHub.
So there's a GitHub mentioned above so you'll have to go and download the latest version from there. So once you have that Library,
this is a common thing that you do in Java. You download a jar file. You can go and download any particular jar file
that you opt for when I say jar file. It's nothing but the third party Java archive, which is nothing but it's a group of classes
together doing something. You can download any jar into a specific directory and you can add it into your application.
There could be a third party say for example, I want to add two numbers or say, for example, you want to do some big data processing a Java
by itself doesn't have a big data processing library or framework. So what it would do is Big Data framework
will have a jar file exposed. Right which is z for example Apache Apache is a provider which is providing solution for big data, right?
So you would have the libraries or you would have everything coded into a jar file for big data processing now you need to import that jar file
into Are you need to link up your application to that particular jar file to leverage big data processing in application.
So in that typical scenario, what you do is you would have this jar files downloaded into your local directory
and have you could add those external jar files or this is how you link to your applications.
All right. So you go to Java you right click on your application go to build path.
Then you click on Java build path here. And then there's a button here to the right which is ADD external jars.
You would have a jar file in this case dom4j 2.1 dot o you have this jar files here. Now you link up to your application
and you click on that and you click on open that would add the jar file to your application and you can apply and close.
So now you could leverage that particular jar file or the functionality that is exposed by the jar file into your application.
All right, in this case, it was dom4j introduction to Jack's be Jack's b stands for Java architecture for XML binding.
It's a specification. Actually Jack's be is used to write Java objects into XML and vice versa.
All right, so you could write it or read it. What are the features of Jack speed supports for all w3c schema features read
all the standard w3c standard and all the features that you have in w3c. It has a support for it.
It reduces a lot of generated schema derived classes. It cut Downs rather on the generated classes scheme are derived classes
small runtime libraries in terms of size. It's pretty lightweight it provides additional validation support by jxp,
1.3 validation API. It steps to convert Java object into XML create a pojo or bind the scheme.
And generate the classes. So basically Jags B is nothing but from the schema, you can create your class.
All right, if you schema says like remember we had an excess T wherein we saw
that it was like an XML which you could read through like you could have the schema if you remember we had a student schema
wherein we declared this is what the format of XML would be you could have the name you would have student as a starting tag.
We defined the sequence as well. Like first name should be first then last name then email address and then marks right?
We Define the sequence as well. So that is nothing but that's a schema. That is XS T.
So when use Jack's be what you do is from the schema, you can create Java classes. So basically schema is nothing but schema tells this is
what an XML can contain and from schema. You can have Java classes created so there would be a Java class
by the name student and within student there would be spring so We had the data type Putin in the exist as well. Like first name
would be string last name would be string and stuff. There's a mapping between this data type and Java data type.
What it would do is nothing but it would create all these properties or all the properties
that you have all the elements of elements that you have within student. There would be a corresponding property
created in a Java class. So basically this Java class could be thought of as a container for your XML,
right you could parse them into this Java class and that's where Jack's be comes into picture. It's a binding it binds your XML into this Java classes.
All right. So create Jack's be context object the so the next step is to create Jack's be context object.
Then create the Marshal ER objects. You have to Marshal and unmarshal. So in this case,
since we are going to do from pocho into XML, it's going to be Marshall method. All right.
Create the content free by using set methods. All right, whatever. You want to set your going to set it into Java object.
So unlike earlier in the Dom example that we took we were actually creating the tree. If you remember weapon child and stuff like that,
which makes it very difficult to understand right which is difficult to maintain and if you miss something it's cumbersome to actually maintain it.
Now, you have a student class wherein you just have to set into the student class. All right, so create the content free by using set methods
and then call the Marshal method to convert this class into an XML. We have a student class and within student class.
We would have all the properties created which is derived from your schema class from your schema xsd
or rather scheming existing mean the same and what you are doing here is you are going to set it in to set values
that you want in the XML in. The instance variables in this class student and we are going to Marshall it to convert this into XML.
So basically rather than creating XML on your own what you are doing here is you're using the Marshall method to do it.
So once you Marshall it, you should be able to get the XML. So let's understand Jason.
Jason is nothing but JavaScript object notation. We will look at the format of it very soon. It is easy to read and write than XML XML is bit cumbersome
when it comes to reading and writing and it takes occupies lot of memory as well. Jason is language-independent similar to XML.
It's language independent. It is lightweight compared to XML and which is nothing but lightweight data
interchange format XML is a data interchange format as well. And Jason is pretty much like the same used for data interchange.
The medium of communication between two different systems Jason supports arrays of objects strings numbers and values. So most of the rest API is restful apis,
which is nothing but a web service built upon HTTP. Mostly they use Json for communication between client and rest API systems.
So this is because it's pretty lightweight and it's lighter compared to XML and as you could imagine
since it is across networks since communication is happening across networks. You need to have lightweight system
or lightweight medium or else it would occupy lot of the bandwidth between communication just
because it is lightweight. It's preferable interchange format difference between Json and XML Json stands
for JavaScript object notation XML stands for extensible markup language. Jason is simple to read
and write XML is difficult to Then rate so we have been looking at the examples which were not pretty straightforward.
Right? We had a lot of code to be put in so it is difficult to read
and write Jason is data-oriented XML is document-oriented. Jason is less secure than XML XML is more secure than Jason Jason supports only text and number data type XML
supports many data type as text number images charts graphs Etc. Alright, so it has restriction with the number of data types
that can hold Json object holds key value pair. We took example of student earlier the form of XML, right? We're in new head.
First name last name and marks. This is the same example, but in Jason, so here you could see opening brace and closing brace
and you could see student is a key and followed by colon and again, There's opening brace and closing brace for student
which means it is saying that student is object if you have value as which starts with opening brace,
which means that the object the second one is as you get deep into this particular object wherein you see first name, right?
First name is the key and you see a literal which is Henry as object as the value rather and as you can see here I Henry is not object.
That's why you don't have it within braces, which means it's a literal value. Alright again, you have last name and D'Souza
which is a literal value you have marks and 50, which is a literal value just to summarize whenever you have: to the left side of the colon
is nothing but the key the right side it's a value and if you have a value starting with coats, which means that it's objects a complex object
or you have multiple things with So in this case, you could see that within object.
You have like three things put in like first name last name and marks basically student can be mapped to some object in Java
which will have first name last name and mouse. So each key is represented as string in Jason and key is always string in Jason
and the value can be of any type Json array represents order list of values. It is always ordered.
Json array can store string number Boolean or objects. It can have string it can hold number it can hold Boolean or any other object as well.
So here you can see the first example is array representing days of a week and you could see Monday Tuesday Wednesday, Thursday,
Friday Saturday and Sunday put in there, right you could see another example is students and within students you could see it.
Students is nothing but array of student and each student has first name last name email. So basically this is how you could interpret it.
So students is nothing but it's an array. So the key is to dance here. Let's see how to run it.
So the first thing that you do is Jason simple, so you can see here jar file is already downloaded which I would be using so we have like Jason simple.
All right, so it's not there. Let me see. So whenever you want to deal with Jason's the first thing
that you would do is you have multiple apis though, but the slides to come we are referring to Jason simple jar.
So the first thing that I would do is I would download Jason simple jar. All right, so I go.
So you could go and download this Jason simple jar. Okay, usually nowadays. We don't do this
because we have other we need tools like mavin mavin is a build tool and you have a file XML file wherein you provide all the jars
that you want and it would download it by itself. So it has got a repository from which it would download all the jars.
You don't have to explicitly put it but I don't want to confuse at this point with Maven. Let's do it this way downloading it and then linking it.
All right, so it's connecting. Basically, we are downloading the jar, right and you could see it has downloaded it.
It's pretty small like when dkb jar because those are all class files. It's Java archive,
which is nothing but class file. So I take this and I kind of unzip it. Okay, so I get this directory here
and you should be able to see a jar file. Now. This is nothing but an executable jar file
you could see it here would see the type as executable jar file. All right. So now I put it into on let's for Simplicity.
Let me put it here itself. All right, so I create a new folder. Which is Ed, Eureka?
Jason and I put it here. So now we'll have to link up our application with that. So what I do.
So when we had this dom4j downloaded and linked up we'll have to do the similar stuff for this thing as well.
So since I am using IntelliJ, the steps would be different than Eclipse. But basically what you do is you go into let
me see the option here. There's open module settings. So you go to the open module settings
and you would see something of this sort coming up. Alright, so now I want to add something right. Add a particular Library.
So I go to libraries first. I click on add Java and I've Traverse to the path that I put the jar file into
so I put it into Ed Eureka Jason. I select this jar file and click on OK. So this is basically you're trying to link
your application to a particular jar file. All right, so you could leverage it. So here you can see that this is jar file
that will link to your application. All right, I apply it which I already did and I will click on OK
OK, so that's how you link any executable jar to your application. So you go to the build path you go to this is
how you do it in Eclipse. Like in IntelliJ. I did module settings where as in Eclipse.
You would go to the build path. You would go to the add external jars and you would select the path and then apply and close.
So this is how you do it in Eclipse. So encoding Jason in Java, how do we encode Jason in Java?
How do we write a Jason? How do we actually create Jason in Java? All right.
So I had to comment this earlier now I uncomment this so there was some are earlier now. Let me try to resolving it.
Okay, it's taking So it's asking me which Java class to resolve. So there is a Json object class. We'll have to see so let me select this one.
So I have a Json file. So similarly, I would prefer just to keep best practices. I would say.
Json object and this is like private method the return type is Json object and I say create Jason.
Andre and I would prefer to put this here rather than in main method that's one of the best practices by the way,
it's not something that you have to do. But as I said, I prefer to write something like this give a meaningful name.
Something like this. So I'm just iterating through this so that you get to understand.
What are the best practices it's not mandatory. So I say student. Jason then I say student.
So this is to make it more readable tomorrow if I come and look at it. I would be able to understand and maybe
as I said there would be many other developers who would be looking at the same code that you have written in order
for them to understand and to increase the maintainability and reusability Factor as well. It's better to write like this.
Alright, and then I could even say private void. Bring Jason. And I take the Json object.
You have a print method which is taking Json object and say I call it as Jason because it could be any generic Jason
and I print it out saying Jason. All right. So you have new Json encode a Creator instance of it
and I create Jason now this Json object. Which is nothing but student Jason. It's going to be here and I'm going to call new.
Json encode dot print Jason and I'm going to pass the student Jason here. So we have created a Json here with first name last name
and email address and we have marks as well and we are printing this Jason there so I have run it. Let's see what it shows up.
So Jason is pretty much light weight as compared to XML as you could imagine right XML has all this start tag in tag and stuff like that.
So when it comes to a humongous big chunk of data, like millions of data, you're processing you could imagine the size
of Jason is size of XML would be pretty much high as compared to Jason since Jason is just doing it in the form of key value pair.
You don't have to end it you don't have the end tags and stuff like that when it comes to readability. I think in terms of readability XML
could be better but when it comes to size, this one is better. Jason is better.
So machine to machine interaction. Jason is better whereas Deputy I think XML could be at times more readable
to humans compared to Jason's. Alright, so here you can see Jason being created. So again if I want to format this there are online tools
since this have widely become standard for data exchange standards you have lot of tools dealing with it.
All right, if I want to do online tools itself rather if I want to do Jason for matter, I have Jason for matter as well,
which is online. And it's validating as well. If you miss something if you don't have a empty braces,
or if you don't have a closing brace, it would give you an error, right? So if I do this,
you can see that it formatted it and it has given you to collapse and expand so you could parse through
and understand more about this Jesus. Also, it validates it. So if I remove this
Cody Breeze and if I try to do it it would say it's a invalid Jason. So if I scroll down it shows invalid Jason
since it was expecting closing Breeze at the end. I put this braces and it should be back up again. Be able to parse it.
All right, so that's about Jason. So what do we have next is creating Json file. So you could write it into a file.
Basically what you could convert it is into a string and you could write it into a file. All right, so if I want to write here Yeah,
I want to write to file and I said Json object for right this Json object into a file. What I would do is say I do it using file writer and I
take the file name as well. Say for example or string. It should be absolute filename.
All right, so it's absolute path of the file name. So I do F or maybe I can use it within the file writer, right? So I create a file writer, which is nothing but Jason.
File writer since we know that it's going to write just Jason's. All right.
I create the instance of it. What does it take? So we have created a file writer for writing.
It is showing some exception. Remember we have to add exception to throw it. Now.
What I do is Json file writer. . Right I'm going to write this thing Json object as a string.
Okay, so Jason dot to Json string. All right, I got a string and I would write it into a file. Now.
What I do is Jason brighter dot flush. I would flush whatever is been buffered. And last thing that I would do is close it.
So one thing to remember is you should always close it. If you don't close it. It's going to remain open
and which is going to consume lot of your memory at the end which might become a bottleneck for your application. So remember you whenever you deal with file,
you have to close it at the end. Now what I do is new I create a new instance of Json encode and I kind of write to a file.
Okay write this to a file and I need to provide file path as well. So I say Ed Eureka.
Jason died say Student Jason I have to handle the exception here as well. If I want handle I can handle it
or else I can choose to throw it off. So in this case, I have thrown it off. So you could see the program executed successfully now.
Let me go to a director Jason and I see student Jason here. Okay, go to see I go to a to record Jason. I just open it up and you should be able to see students
that Jason has all the fields that we have gotten right first name marks email and this thing so
yeah, we saw how to write it into a Json file, right? So we have created a Json file and we have similar thing like how to read it
from a Json file you have like Json parser, which is again, you need to pass your file reader
and which would pass it into a particular Json object. So from your file, you are basically converting it into a Json object
and from Json object. You can read whatever data you want to again give you an example you could have private void read Json
and you could have string say absolute. Alright, so you have this now what I'm trying to do is I'll create a new Json parser.
You can see here. There's a parse method which takes filereader. All right, so I do dot bars.
All right, and you could see there's a second method here, which is taking reader All right. So what I do is bars
new file reader file reader is nothing but is a reader that's why you can use it there and I give absolute path name. All right, so this should pass your Jason.
So it's asking me to import it. I am putted it. So this file not found exception
that I need to handle which I will rather throw it off. Alright, so as you could see here, I have thrown of filed all these other exceptional swell
that I need to handle which is nothing but IO exception which is again, I've thrown it off.
Alright, so we have Parts this o on parsing what do you get is nothing but object You get Json as object right here.
You have passed it. And you have got Jason as object. Now.
What I'm going to do is I'm just print going to print say one of the attributes not all since it's going to be the same.
I'm going to print the first name. So it says there's no method. Let me see.
So you have this it cannot be taken as object directly. It has to be taken as Json object so that we could read it.
All right. So this has to be typed costed to Json object. This is basically typecasting.
All right, what is typecasting is nothing but you know what is going to be written doubt.
But from the pass is going to be Json object so we can do it something of this sort. All right,
once you do this, you can get based on the key. So I give first name key. Let me print the last name as well.
All right, and let me print the email as well to for convenience. So basically we are going to read from this so
its first name last name and email as we can see first name is lowercase. So this would give an error.
So we have passed it as well, right we have read it from Json file. Now.
What I do is I'll have to give a call to this which is nothing but Jason and quote dot read Jason and I passed the same file here.
All right. Now it is asking me to handle the exceptions. So I add it to the method signature
as I choose not to handle it. All right. So what we have done here is nothing but we are we
are parsing the Json file. All right. So we have a read Jason method
which is taking absolute file name as its parameter. We have a jsonparser class, which is one of the classes from the jar
that we downloaded Jason simple jar that we downloaded and since it's put into your application
since we have attached it with our application. We are able to use those classes, right? If you wouldn't have done that step of linking the library
with the application you won't be able to use this classes. Okay, because these are third party classes. This is not as a part of the standard Java kit or SDK
that comes with Java. We are to download it and then link it up with our application using module settings
or if you are in an eclipse, then it would be configured build path. Then you parse it and you provide the reader instance
of reader in this case. I passed file reader and I give the absolute file name. Once this is done you should be able to read messages
or read the content or read the keys that you have within your file. You get this an object then I do Json dot get first name then I
do Jason don't get last name. And the last thing that I am printing is email. All right.
Let's see if this works. So I'm running this. You could see the value being printed here.
First name that came out as John then the last name that is Lee and the email that we printed. That is John at the right one two, three.
All right, so that's about XML and Json which is nothing but it's a standard set across industry for data entry exchange.
So yeah having said that one of the main differences between XML and Json is its lightweight
and most of the companies are the industries moving towards using Jason's rather than xml's but xml's our Legacy
and they have lot of weed they carry a lot of weight in the industries lot of systems at this point. I call the financial systems.
They have Legacy systems and they deal with xml's and less of Jesus. But Jason is something that is upcoming
and lot of systems have started migrating to or started using Json speak since they are lightweight
that's pretty much it from my sight and thanks a lot for Sting and I hope you guys all become an emerging coders
and practice a lot on coding. All right, so all the best thank you. I hope you have enjoyed listening to this video.
Please be kind enough to like it and you can comment any of your doubts and queries and we will reply them
at the earliest do look out for more videos in our playlist And subscribe to Edureka channel to learn more. Happy learning.
Heads up!
This summary and transcript were automatically generated using AI with the Free YouTube Transcript Summary Tool by LunaNotes.
Generate a summary for freeRelated Summaries
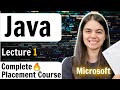
Java Course Introduction: Mastering Coding Fundamentals and Data Structures
Kickstart your Java programming journey with our guided course covering basics to algorithms for aspiring developers.
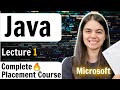
Java Programming Course: Introduction, Structure, and Setup Guide
Learn about Java programming fundamentals, data structures, and how to set up your coding environment.
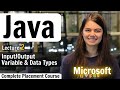
Java Basics: Outputs, Variables, and User Input Explained
Learn Java's fundamentals: how to give output, use variables, data types, and take user input effectively.
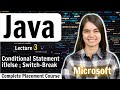
Mastering Java: Understanding Conditional Statements for Beginners
Dive into Java's conditional statements, including 'if', 'switch', and 'break', to enhance your programming skills.
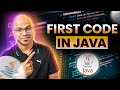
Getting Started with Java: Writing Your First Code in VS Code
In this tutorial, we explore how to set up Visual Studio Code for Java development and write your first Java program. We cover the basics of creating a project, using the terminal, and writing a simple 'Hello World' program.
Most Viewed Summaries
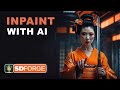
Mastering Inpainting with Stable Diffusion: Fix Mistakes and Enhance Your Images
Learn to fix mistakes and enhance images with Stable Diffusion's inpainting features effectively.
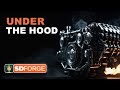
A Comprehensive Guide to Using Stable Diffusion Forge UI
Explore the Stable Diffusion Forge UI, customizable settings, models, and more to enhance your image generation experience.
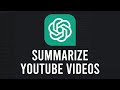
How to Use ChatGPT to Summarize YouTube Videos Efficiently
Learn how to summarize YouTube videos with ChatGPT in just a few simple steps.
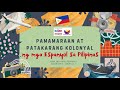
Pamaraan at Patakarang Kolonyal ng mga Espanyol sa Pilipinas
Tuklasin ang mga pamamaraan at patakarang kolonyal ng mga Espanyol sa Pilipinas at ang mga epekto nito sa mga Pilipino.
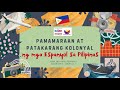
Pamamaraan at Patakarang Kolonyal ng mga Espanyol sa Pilipinas
Tuklasin ang mga pamamaraan at patakaran ng mga Espanyol sa Pilipinas, at ang epekto nito sa mga Pilipino.