Introduction to Java Programming
Welcome to the exciting journey of learning Java programming! In this course, we will cover everything you need to know about the fundamentals of programming using Java, as well as delve into data structures and algorithms. This course is divided into two main parts:
- Introduction to Programming: This section will focus on learning the basics of coding, understanding what code is, and how it functions.
- Data Structures and Algorithms: This part will guide you through more complex concepts and applications of data handling and manipulation.
Course Outline
The introductory programming course is designed to be completed in 12 classes over 12 days. Here's a brief overview of what we'll cover:
- Class 1: Introduction to coding and setting up your code editor.
- Class 2: Input, output, variables, and data types.
- Subsequent classes will explore operators, control statements, functions, exceptions, and arrays, among other critical programming concepts.
Understanding Code and Its Importance
In our daily lives, we communicate instructions using natural languages like Hindi or English. However, when it comes to computers, they only understand binary language composed of 0s and 1s. This is because computers are electrical devices that operate on electrical signals, which can be either on (represented by 1) or off (represented by 0).
Why Binary?
- 0 represents an OFF state (no electrical current).
- 1 represents an ON state (presence of electrical current).
To bridge the gap between our human language and binary, high-level programming languages like Java allow us to write code in a human-readable format. The code we write in Java gets converted into binary format by a compiler, which the computer then understands.
Functionality of Java: An Example
Let's consider a simple analogy to explain how programming works:
- Imagine cooking Maggi noodles. You have a step-by-step set of instructions:
- Gather raw materials (water, Maggi, spice).
- Boil the water with added ingredients.
- Check if ready; if not, continue boiling.
- Serve and enjoy your meal.
Similarly, when we write programs, we give the computer a set of instructions through code that tells it what to do.
Pseudocode: A Planning Step
Before diving into the actual coding, it's prudent to create a pseudocode. For instance, if we want to add two numbers and print the sum, our pseudocode might look like:
START
INPUT number1, number2
sum = number1 + number2
PRINT sum
END
Transitioning to Actual Code
Once you have your pseudocode, you can begin writing actual Java code that will perform the intended operations:
import java.util.Scanner;
public class AddNumbers {
public static void main(String[] args) {
Scanner input = new Scanner(System.in);
System.out.print("Enter first number: ");
int number1 = input.nextInt();
System.out.print("Enter second number: ");
int number2 = input.nextInt();
int sum = number1 + number2;
System.out.println("The sum is: " + sum);
input.close();
}
}
Setting Up Your Java Environment
To get started with Java programming, you need to set up your coding environment. Here’s a simple process to do this:
-
Install Java Development Kit (JDK): The JDK contains the tools necessary for developing Java applications. You can download it from the Oracle website.
- Link: Oracle JDK Download
-
Choose a Code Editor: For this course, we will use Visual Studio Code (VS Code), a popular and versatile code editor. You can also use alternatives like IntelliJ IDEA or Eclipse.
- Link: VS Code Download
-
Follow Installation Videos: We’ve created tutorial videos on how to install JDK and VS Code in both English and Hindi. Ensure you follow these to set up your environment correctly.
Exploring Code Editors
After downloading your code editor, open it to create a new Java file. The first Java code typically written by beginners is:
class HelloWorld {
public static void main(String[] args) {
System.out.println("Hello, World!");
}
}
After saving (as HelloWorld.java
), you can run this program to check how Java executes code, producing the output: Hello, World!
The Java Execution Process
All Java programs undergo two main stages:
- Compilation: This is where your source code (
.java
) is compiled into bytecode (.class
). The bytecode is platform-independent, meaning it can run on any device with JRE (Java Runtime Environment). - Execution: During this stage, the Java Virtual Machine (JVM) converts bytecode into native code that the computer system understands, allowing for actual execution of the program.
Key Components of Java Code
- Classes: The blueprint for creating objects, acting as containers for functions (methods).
- Functions/Methods: Blocks of code within classes that perform specific tasks; for instance, to add two numbers or print messages.
- The Main Function: The entry point for execution, where your program begins.
Conclusion
In today’s session, we’ve taken an introductory glance at the world of Java programming. Through understanding code structure, setting up our coding environment, and writing our first Java code, we’ve laid a solid foundation for our coding journey ahead.
I hope you are excited to dive deeper and practice coding. In the upcoming classes, we’ll explore more sophisticated programming concepts and continue building your coding skills. Until next time, keep coding and practicing!
हाय एवरीवन एंड वेलकम टू अपना कॉलेज और इस वीडियो में हम शुरुआत करने वाले हैं अपने जावा कोर्स के साथ इस कोर्स को हमने दो
पार्ट्स में डिवाइड किया है जिसमें सबसे पहला पार्ट है इंट्रोडक्शन टू प्रोग्रामिंग यानी हम जावा को यूज करके
कोड क्या होता है कोडिंग प्रोग्रामिंग क्या होता है वो सब सीख रहे होंगे जितने भी फंडामेंटल्स जरूरी हैं जावा को जानने
समझने के लिए हम उन सबको सीखेंगे शुरुआत में और इसके सेकंड पार्ट में हम सीख रहे होंगे डाटा स्ट्रक्चर्स एंड एल्गोरिथम्स
को तो जो हमारा प्लान रहेगा इंट्रोडक्शन टू प्रोग्रामिंग का वो कुछ इस तरीके का रहेगा जिसमें फर्स्ट क्लास जो कि आज है हम
सीखेंगे कोड क्या होता है कोडिंग किस तरीके से होती है साथ के साथ इंस्टॉल करेंगे अपने कोड एडिटर्स को अगली क्लास
में इनपुट आउटपुट वेरिएबल एंड डेटा टाइप्स एंड इसी तरीके से आगे के कुछ टॉपिक्स को हम 12 दिन के अंदर 12 क्लासेस में कवर कर
रहे होंगे तो चलिए जावा सीखते हैं अपने शानदार कोर्स के साथ रियल लाइफ में अगर हमें किसी व्यक्ति से बात करनी होती है तो
एक सेट ऑफ इंस्ट्रक्शंस हम उसे देते हैं जो एक लैंग्वेज के माध्यम से देते हैं जैसे मैं आपसे बात कर रही हूं तो मैं
हिंदी लैंग्वेज में बात कर रही हूं हम इंग्लिश में भी बात कर सकते हैं लेकिन अगर हम एक कंप्यूटर या लैपटॉप या मोबाइल से
काम करवाना है तो उन्हें बताना पड़ेगा अब इंग्लिश या हिंदी तो उसे समझ में आता नहीं इसे समझ में आता है बाइनरी यानी ़ और वन
दीदी अब यह ज़ीरो और वन ही क्यों इसलिए यार क्योंकि हमारे लैपटॉप एंड कंप्यूटर इलेक्ट्रिकल डिवाइसेज होते हैं जो बिजली
से चलते हैं तो जब सर्किट के अंदर करंट जाता है उसे हम रिप्रेजेंट कर देते हैं वन से और जब सर्किट के अंदर कोई करंट नहीं
जाता तो उसे हम रिप्रेजेंट कर देते हैं जीरो से या इसे ऐसे समझो कि जब डिवाइसेज हाई वोल्टेज डिटेक्ट कर रही होती है तो
उसे + व से रिप्रेजेंट कर दिया या जब लो वोल्टेज डिटेक्ट कर रही है तो उसे हम जीरो से रिप्रेजेंट कर दें तो ऐसे में मान लो
कि जो हमारे रियल लाइफ डेसीमल नंबर्स होते हैं उनमें से रो को वी कैन रिप्रेजेंट विद रो इन बाइनरी डेसीमल के वन को वी कैन
रिप्रेजेंट विद वन इन बाइनरी डेसीमल के थ्री को वी कैन रिप्रेजेंट विद वनव इन बाइनरी एंड डेसीमल के फोर को वी कैन
रिप्रेजेंट विद 00 इन बाइनरी और इसी तरीके से हम आगे चले जाते हैं ऐसे ही ए बी सी डी ई एफज या स्पेशल कैरेक्टर्स जितनी भी
चीजें हैं उन्हें हम रो और वन के कॉमिनेशन में कंप्यूटर को समझा सकते हैं अब ऐसे में आपको दिमाग में एक चीज आएगी कि दीदी ये तो
बहुत कॉम्प्लिकेटेड लग रहा है कल को अगर मुझे दो नंबर का एडिशन भी करना हुआ जैसे 53 + 72 तो इसको तो 01 में कन्वर्ट करने
में बहुत सारा टाइम लग जाएगा तो मैंने कहा कि यहां आती है हमारी हाई लेवल लैंग्वेजेस जो हमें हेल्प करती हैं कैसे इस कोर्स में
जो हाई लेवल लैंग्वेज हम सीख रहे होंगे वो होगी जावा तो जावा में हम अपना कोड लिखते हैं और कंप्यूटर में एक कंपाइलर बैठा होता
है जो इसे बाइनरी फॉर्मेट में कन्वर्ट करके देता है जो कंप्यूटर को समझ में आने लगती है और यहां से शुरुआत होती है हमारे
कोडिंग सफर की तो आपने बात तो करना सीख लिया पर हमें कंप्यूटर को कुछ कुछ सेट ऑफ इंस्ट्रक्शंस भी देने हैं यह इंस्ट्रक्शंस
देने के लिए हमें प्रोग्राम करना पड़ेगा तो हम यूज कर रहे होंगे जावा लैंग्वेज को टू गिव कमांड्स या फिर इंस्ट्रक्शंस टू द
कंप्यूटर टू परफॉर्म समथिंग अब ये चीज अगर समझ में नहीं आ रही तो एक एग्जांपल लेते हैं अब डे टू डे लाइफ में अगर हमें कोई
काम एग्जीक्यूट करना है तो उसके लिए कोई सेट ऑफ इंस्ट्रक्शंस देते हैं जैसे हम अभी एक फ्लो चार्ट से समझेंगे जैसे सबसे पहले
मैं शुरुआत करूंगी स्टार्ट से मुझे कुछ रॉ मटेरियल चाहिए है जैसे मैगी मसाला और पानी उसके साथ एक बर्तन और एक स्टोव चाहिए उसे
पकाने के लिए नेक्स्ट स्टेप होता है कि हम पानी मसाला मैगी उस बर्तन में डालें और उसके बाद उसे पकाने लगे उसे बॉईल करते हैं
और चेक करते हैं कि क्या हमारी मैगी बन गई है अगर मैगी नहीं बनी तो हम कहते हैं कि इसे और बॉईल करो और अगर बन गई तो कहते हैं
कि गैस बंद कर दो फिर हम मैगी को सर्व करते हैं और उसे खाते हैं फिर हमने एग्जिट कर दिया अपने फ्लो चार्ट से सिमिलरली जब
हम कंप्यूटर को कोई बात समझाना चाहते हैं तो हम ऐसे ही सेट ऑफ इंस्ट्रक्शंस को यूज करते हैं इनको कोड में लिखने से पहले हम
देख लेते हैं कि फ्लो चार्ट की हेल्प से इन्हें कैसे समझा रहे होंगे इससे हमें पता चल जाएगा कि रियल लाइफ में हमारे
कम्युनिकेशंस कितना सिमिलर हो रहे हैं और इसी सेट ऑफ इंस्ट्रक्शंस को जो हमारे राइट में लिखे हैं कंप्यूटर साइंस की भाषा में
हम सूडो कोड कह देते हैं अब हमें क्या करना है मान लेते हैं कि हमारा काम है कि दो नंबर्स हमें किसी से लेने हैं और उनका
सम कैलकुलेट करके हमें प्रिंट करवा देना है उस सेम लॉजिक को हम फ्लो चार्ट की हेल्प से कैसे रिप्रेजेंट करेंगे हम सबसे
पहले शुरुआत करेंगे तो इसीलिए हम ड्रॉ करेंगे सबसे पहले अपना स्टार्ट उसके बाद इनपुट के लिए हम अपने वेरिएबल को इनपुट ले
लेंगे पहले वेरिएबल को स्टोर कर देंगे नंबर वन में दूसरे वेरिएबल को स्टोर कर देंगे हम नंबर टू में यानी नंबर वन और
नंबर टू हमने यूजर से इनपुट ले लिए हैं उसके बाद दोनों का सम हम एक सम नाम के वेरिएबल में स्टोर करा देंगे यानी सम में
नंबर वन प्लस नंबर टू हमने ऐड करके डाल दिया उसके बाद हम प्रिंट करवा देंगे अपने सम को एंड फाइनली हम कर जाएंगे एग्जिट तो
कुछ इस तरीके से फ्लो रहेगा जो काम हमें करना है उस काम का अब सिमिलरली अगर हम सूडो कोड की बात करें तो सुडो कोड के अंदर
सबसे पहला स्टेप क्या होगा कि हम स्टार्ट कर रहे हैं उसके बाद हम इनपुट ले रहे हैं दो नंबर्स को उसके बाद हम सम कैलकुलेट कर
रहे हैं नंबर वन प्लस नंबर टू की हेल्प से फिर हम प्रिंट करवा रहे हैं उसी सम को एंड फाइनली हम अपने कोड से कर जाते हैं एग्जिट
तो सूडो कोड एक तरीके से जो भी फ्लो होता है प्रोग्राम का उसको सिंपल इंग्लिश की भाषा में रिप्रेजेंट करने का तरीका होता
है और इसी सूडो कोड को बाद में हम कन्वर्ट कर देते हैं एक्चुअल जावा या c+ प या पाइथन कोड के अंदर अब अपने कोड को लिखने
के लिए सबसे पहले जावा के अंदर हमें इंस्टॉल करनी पड़ेंगी कुछ-कुछ चीजें इसमें से सबसे पहली चीज जो हमें इंस्टॉल करनी है
व है जावा का जावा डेवलपमेंट किट ये एक ऐसा किट है जिसके अंदर वो सारी चीजें भरी हुई हैं जो तो आपको चाहिए आपके सिस्टम पर
जावा चलाने के लिए तो जब भी आपको जावा का कोड लिखना होता है जावा का प्रोग्राम लिखना होता है और उसे किसी भी सिस्टम पर
रन करना होता है तो हमें जावा डेवलपमेंट किट की जरूरत पड़ती है कुछ जरूरी टूल्स लेने के लिए उसके साथ-साथ हमें डाउनलोड
करना पड़ेगा एक कोड एडिटर या आईडी यानी इंटीग्रेटेड डेवलपमेंट एनवायरमेंट ये कोई भी हो सकता है हम अपने कोर्स में यूज कर
रहे होंगे विजुअल स्टूडियो कोड यानी वीएस कोड इसके अलावा आप इंटेलीज भी यूज कर सकते हैं आप इ क्लिप भी यूज कर सकते हैं यह कुछ
सॉफ्टवेयर्स हैं जैसे गेम के लिए हम गेम्स डाउनलोड कर रहे होते हैं वैसे ही कोड लिखने के लिए हम एक सॉफ्टवेयर डाउनलोड
करना पड़ता है अपने लैपटॉप या कंप्यूटर के ऊपर तो इन सॉफ्टवेयर्स को डाउनलोड करने के बाद हम अपना कोड इनके ऊपर लिख रहे होते
हैं और यह हमारे कोड को रन करके हमें कुछ दिखा रहे होते हैं कि एक्चुअल रिजल्टेंट आउटपुट हमारा क्या आने वाला है अब हमने
ऑलरेडी दो वीडियोस बनाए हुए हैं इंग्लिश और हिंदी में वीएस कोड को डाउनलोड करने की इनमें से सबसे पह पहला वीडियो है जो आपको
c+ प सीरीज में दूसरे इंडेक्स पर मिल रहा होगा उसके अंदर c+ प के अंदर हमने डाउनलोड करके दिखाया था आपको c+ प के कंपाइलर्स उन
सबको हमें डाउनलोड नहीं करना हमें सिर्फ डाउनलोड करना है जावा डेवलपमेंट किट को साथ के साथ विजुअल स्टूडियो कोड को अब
चाहे आप विज पर काम कर रहे हैं या आप मैक पर काम कर रहे हैं ये दोनों चीजें डाउनलोड करना सफिशिएंट है इसके लिए जाकर आप उन
दोनों वीडियोस को रेफर कर सकते हैं साथ के साथ अगर आपको इंटेलीज को डाउनलोड करना तो उसके लिए भी हमने एक ऑलरेडी वीडियो बनाया
हुआ है जावा वन शॉट के नाम से जिसके अंदर एक मिनट पर जब आप जाएंगे तो वहां पर जाकर आपको पूरा इंस्टॉलेशन प्रोसेस मिल जाएगा
कि जावा डेवलपमेंट किट को कैसे डाउनलोड करना है और इंटेलीज को कैसे डाउनलोड करना है उसके साथ-साथ हम आपको अभी दिखा भी देते
हैं कि जैसे ही आप अपने कम पर जाएंगे तो कम या जो भी ब्राउजर आप यूज करते हैं उस पर जाकर आपको सर्च करना है
जावा डेवलपमेंट किट के बारे में और कुछ इस तरीके से जो पहला ओरेकल का लिंक आ रहा होगा उस पर आप जाएंगे तो वहां पर
आपको डाउनलोड करने के लिए डिफरेंट डिफरेंट फॉर्मैट्स मिल जाएंगे अगर आप इसके अलावा एक और ऑप्शन है जिसमें पूरा का
पूरा जावा डेवलपर पैक आपको मिल रहा होता है तो माइक्रोसॉफ्ट ने क्या किया है विजुअल स्टूडियो कोड के साथ कुछ एक्सटेंशन
मिला दिए हैं जावा डेवलपमेंट किट मिला दिया है जिसकी वजह से एक ही लिंक में आपको सारी की सारी चीजें डाउनलोड करने को मिल
जाएंगी तो उसके लिए भी हम सर्च कर लेते हैं विजुअल स्टूडियो कोड इंस्टॉल पैक जावा तो यहां पर यह जो पहला लिंक आएगा
जावा इन विजुअल स्टूडियो कोड का उसके अंदर हमें जाना है थोड़ा सा स्क्रॉल करना है और यह इंस्टॉलिंग द कोडिंग पैक फॉर जावा विज
अगर आप विज यूज करते हैं तो या फिर इंस्टॉलिंग द कोडिंग पैक फॉर जावा मैक ओ अगर आप मैक यूज करते हैं तो तो इस तरीके
से आप जाकर विजुअल स्टूडियो कोड को सेटअप कर सकते हैं अपने सिस्टम के ऊपर एंड आगे के कोड जो हम लिखेंगे जावा के साथ वह हम
उसी कोड एडिटर पर लिख रहे होंगे अब बात करें कि अगर यह कोड एडिटर कैसा दिखता है तो डाउनलोड करने के बाद हमें विज स्टूडियो
कोड कुछ इस तरीके से दिख रहा होगा जिसमें हम क्रिएट करेंगे अपनी एक नई फाइल तो नई फाइल आप क्रिएट कर सकते हैं क्रिएट करने
के बाद अब इस फाइल में हम लिखने वाले हैं अपना पहला जावा कोड अब इस कोड को लिखने से पहले हम एक छोटी सी बात कर लें कि शुरू की
दो-तीन क्लासेस में हम जो जो कोड लिख रहे होंगे वो अगर आप प्रोग्रामिंग कोडिंग में नए हैं अगर आप पहली बार कोड को देख रहे
हैं तो आपको थोड़ी सी परेशानी आ सकती है समझने में लेकिन हमें हार नहीं माननी है हमें चीजों को समझने की कोशिश करनी है
शुरू शुरू में जैसे अगर हम अरेबिक या फिर फ्रेंच सीखने जाएं तो हमें चीजें काफी अलग लगेंगी और समझ नहीं आएंगी लेकिन थोड़े
टाइम के बाद हमें चीजें समझ आनी शुरू होगी तो वो फेथ वो ट्रस्ट हमें दिखाना पड़ेगा कोड के ऊपर हमें थोड़ा टाइम जब कोड को
देखते हुए हो जाएगा तब हमें चीजों के मतलब समझ में आने शुरू होंगे तो पहली क्लास के अंदर हम कोड लिखेंगे कुछ चीजें समझ नहीं
आएंगी बहुत सारी चीजें समझ आएंगी तो हमें उन चीजों को समझने पर ध्यान देना है सबसे पहले जावा कोड में जो हम लिखते हैं वो
बनाते हैं एक क्लास तो क्लास का नानाम हम लेते हैं फर्स्ट क्लास हमने इसका नाम दे दिया इसके अंदर हम बनाएंगे एक पब्लिक
स्टैटिक वॉइड मेन नाम का फंक्शन जिसमें स्ट्रिंग आर्गुमेंट आ रहे होंगे तो कुछ इस तरीके से जावा में कोई भी
कोड लिखने का यही टेंप्लेट रहता है जिसमें आप एक क्लास डिफाइन करते हैं और आप एक फंक्शन डिफाइन करते हैं अब ये क्लास और
फंक्शन क्या होता है यह हम थोड़ा सा देर बाद समझेंगे पर अभी के लिए मान लेते हैं कि जब भी जावा कोड लिखना होता है हमें यह
चीजें करनी होती है एंड इस फाइल को सेव करने का क्या तरीका रहेगा जो भी आपने जावा के अंदर फाइल के अंदर क्लास का नाम दिया
है वही नाम आप अपनी जावा फाइल को देंगे जैसे यहां पर क्लास का नाम दिया था फर्स्ट क्लास तो हम अपनी जावा फाइल को सेव करेंगे
एज फर्स्ट क्लास डॉट जावा तो जावा के अंदर जितनी भी फाइलें हम सेव करते हैं वो डॉट जावा के नाम से सेव होती है जैसे हम 11th
12th क्लास में या फिर जब भी कंप्यूटर साइंस पढ़ते थे स्कूल में तो ड txt.gz सबसे पहली चीज सबसे पहला कोड जो यूजुअली
सारी की सारी प्रोग्रामिंग लैंग्वेजेस में कोडर्स लिखते हैं वो होता है हेलो वर्ल्ड जिसमें आप पहली बार वर्ल्ड को हेलो कह रहे
वर्ल्ड इसे सेव कर दिया अब हम इस कोड को रन करेंगे रन करने के बाद आपको पता चलेगा कि एक्चुअली कोड से होता क्या है जावा कोड
का मतलब क्या होता है ये कुछ लाइनें हमने अपनी फाइल में सेव कर दी अब यहां पर राइट में जाकर रन जावा अगर हम क्लिक
करें तो हमारे लिए कुछ ऐसा प्रिंट होगा हेलो वर्ल्ड अब यह चीज हम कैसे समझ सकते हैं जैसे हम देखते हैं कि कोडर्स
बहुत सारा कोड लिखते हैं और फिर उसके आउटपुट में क्या आता है एक वेबसाइट हमें दिखने लगती है वैसे ही यहां पर जावा का ये
जो छोटा सा कोड हमने लिखा है इसकी वजह से हमें अपनी स्क्रीन पर यह हेलो वर्ल्ड दिखना शुरू हो गया है तो यह कुछ जादू है
जो कोडिंग में होता है जिसमें कोड की हेल्प से आप स्क्रीन पर कुछ भी दिखा सकते हैं अब स्क्रीन पर दिखाने को हम आउटपुट
कहते हैं इसके अलावा अपने कोड की हेल्प से आप यूजर से कोई भी डाटा ले सकते हैं जिसको हम इनपुट कहते हैं इनपुट आउटपुट के बारे
में हम अगली क्लासेस में समझ रहे होंगे अभी के लिए हमने समझ लिया कि कुछ इस तरीके से जावा का कोड रन करता है और हमें एक
आउटपुट दिखा देता है अब थोड़ा थोड़ा सा समझते हैं कि जावा कोड एक्चुअली रन कैसे करता है जावा कोड को रन करने के लिए जो
हमारा कोड होता है वह दो स्टेप्स के थ्रू जाता है जिसमें से सबसे पहला स्टेप होता है कंपाइलेशन का और दूसरा स्टेप होता है
एग्जीक्यूशन का अब ये थ्योरी समझना थोड़ा सा इंपॉर्टेंट है क्योंकि ये थ्योरी कभी-कभी आपसे बाद में जाकर आपके प्लेसमेंट
इंटरव्यू में पूछ ली जाएगी या फिर अगर आपके कॉलेज में आप जावा एज अ सब्जेक्ट पढ़ते हैं तो वहां पर एग्जाम में आ जाएगी
अब जो जावा डेवलपमेंट किट हमने डाउनलोड किया है यानी जेडी के उसके अंदर जे आरई नाम का एक कंपोनेंट है जिसे हम कहते हैं
जावा रन टाइम एनवायरमेंट जे आरई के साथ-साथ इसके अंदर काफी सारे डेवलपमेंट टूल्स भी अवेलेबल हैं
और यह जो जे आरई है इसके अंदर भी एक और कंपोनेंट है जिसको हम कहते हैं जेवीएम जेवीएम यानी जावा वर्चुअल मशीन तो जावा
वर्चुअल मशीन जे आरई और जेडी के ये एक के अंदर एक लेवल वाइज फॉर्म होते हैं लेवल वाइज स्टोर्ड होते हैं और इसी की हेल्प से
हमारा पूरा का पूरा जावा जो कोड है वह रन हो पाता है अब इन दोनों स्टेजेस को अगर हम समझे तो कंपाइलेशन की स्टेज में क्या होता
है जो भी कोड हमने लिखा है उसे हम कह देते हैं सोर्स कोड सोर्स कोड को हम डॉट जावा से सेव करते
हैं और ये सारा का सारा कोड एक कंपाइलर के पास जाता है ये जो कंपाइलर है ये जेडीके के अंदर होता है और इस कंपाइलर के पास
सारा का सारा सोर्स कोड जाता है और यह कंपाइलर इस सोर्स कोड को कन्वर्ट कर देता है बाइट कोड के अंदर जिसका एक्सटेंशन होता
है डॉट क्लास और यह जो वाइट कोड है यह किसी भी ऑपरेटिंग सिस्टम पर रन कर सकता है एज़ लॉन्ग एज़ उस ऑपरेटिंग सिस्टम के अंदर
भी जे आरई जावा रन टाइम एनवायरमेंट हो तो अगर आपने कोड यह चीज आप c+ के साथ नहीं कर सकते इसीलिए
जावा को एक पोर्टेबल लैंग्वेज कहा जाता है क्योंकि इसमें बनाई हुई एप्स को आप बाइट कोड में कन्वर्ट करके किसी भी सिस्टम के
ऊपर रन कर सकते हैं तो यह हमने पहली स्टेज कवर कर ली जो थी कंपाइलेशन की स्टेज अब अगर अपनी दूसरी स्टेज की बात करें जो है
था अब जावा वर्चुअल मशीन इस बाइट कोड को लेती है और उसे नेटिव कोड में कन्वर्ट करती है नेटिव कोड यानी वो कोड जो मशीन को
समझ में आए अभी हमने बात की थी कि सबसे लो लेवल कोड जो मशीन को समझ में आता है वो आता है रो और वन का तो नेटिव कोड वैसा ही
कुछ कोड होता है जो मशीन को समझ में आता है अब ये बाइट कोड नेटिव कोड में जब कन्वर्ट हो गया तो चाहे आपकी मशीन विंडोज
हो या मैक हो अब उसे आपका जावा कोड समझ में आने लग जाएगा उसी तरीके से जैसे आपको हेलो वर्ल्ड प्रिंट करवाना था तो आपकी
मशीन में वो कोड कन्वर्ट होते-होते नेटिव कोड में कन्वर्ट हुआ और फिर आपके सिस्टम में आपको हेलो वर्ल्ड दिखने लग गया अब अगर
हम अपने कोड के कंपोनेंट्स की बात करें तो इसमें से सबसे पहला कंपोनेंट आता है फंक्शन फंक्शंस या मेथड्स कोड का वो पार्ट
होते हैं जिसको हम कुछ काम परफॉर्म करवाने के लिए बोलते हैं जैसे अगर हमें दो नंबर्स को ऐड करवाना था तो वो सारा का सारा कोड
हम एक फंक्शन के अंदर लिखते अगर हमें हेलो वर्ल्ड प्रिंट करवाना था तो उस सारे के सारे कोड को हमने एक फंक्शन के अंदर लिखा
और वो फंक्शन क्या था वो फंक्शन था यह वॉइड मेन नाम की चीज जहां पर फंक्शन का नाम था मेन और उसका रिटर्न टाइप था वॉइड
वॉइड यानी खाली यह फंक्शन कुछ रिटर्न नहीं करता इसने सिर्फ हमारे लिए हेलो वर्ल्ड को प्रिंट करवाया था तो जो भी कोड हमें लिखना
होता है जावा के अंदर वो हम फंक्शंस के अंदर लिखते हैं और इन फंक्शंस को हम क्लास के अंदर लिखते हैं तो क्लास एक बड़ी
एंटिटी है जिसके अंदर काफी सारे फंक्शंस आ सकते हैं क्लास को कैसे समझे जैसे हमारा एक बड़ा सा किचन होता है घर के अंदर
जिसमें बहुत सारे छोटे-छोटे डब्बे होते हैं जिसके अंदर कुछ-कुछ स्टोर्ड होता है जैसे किचन के अंदर एक डब्बा चीनी का है एक
डब्बा नमक का है वैसे ही जावा के अंदर बड़ी सी एक क्लास होती है जिसके अंदर छोटे-छोटे फंक्शंस होते हैं एक फंक्शन में
कुछ काम हो रहा है दूसरे फंक्शन में कुछ और काम हो रहा है एंड जावा की क्लासेस में जो मेन नाम का फंक्शन होता है वो पूरे के
पूरे कोड का पहला पार्ट होता है जो एग्जीक्यूट होता है तो जावा के अंदर जो मेन फंक्शन में पहली चीज लिखी है सबसे
पहले वो एग्जीक्यूट होगी बाद में सारी की सारी कोड की चीजें हमारे मेरे पास एग्जीक्यूट होकर आ रही होंगी तो आज की
क्लास में हमने काफी सारी चीजें समझने की कोशिश करी कि जैसे कोड क्या होता है कोडिंग क्या होती है मशीन को चीजें कैसे
समझ में आती है और हम अपने जावा कोड को एक्चुअली कैसे लिखते हैं आई होप कि नेक्स्ट क्लास से पहले आप अपना कोड एडिटर
यानी विजुअल स्टूडियो कोड या फिर जो भी एडिटर आपको पसंद है साथ के साथ जावा डेवलपमेंट किट को आप डाउनलोड कर लेंगे
ताकि आगे की क्लासेस में हम क्लास के साथ-साथ कोड को लिखकर देख सके रन करके देख सके कि हमें कोई प्रॉब्लम तो नहीं आ रही
या फिर सारे की सारी चीजें हम क्या अच्छे से सीख पा रहे हैं कर पा रहे हैं क्योंकि कोडिंग में प्रैक्टिस करना ज्यादा
इंपॉर्टेंट है तो हम जितना प्रैक्टिस करेंगे उतना हम कोड को और अच्छे से समझ पाएंगे आज के लिए इतना ही मिलते हैं
Heads up!
This summary and transcript were automatically generated using AI with the Free YouTube Transcript Summary Tool by LunaNotes.
Generate a summary for freeRelated Summaries
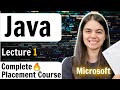
Java Course Introduction: Mastering Coding Fundamentals and Data Structures
Kickstart your Java programming journey with our guided course covering basics to algorithms for aspiring developers.
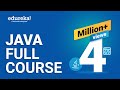
Java Programming: A Comprehensive Guide to Understanding Java and Its Concepts
Explore Java programming concepts including OOP, exception handling, and collections. Learn how to build robust applications!
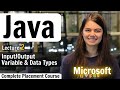
Java Basics: Outputs, Variables, and User Input Explained
Learn Java's fundamentals: how to give output, use variables, data types, and take user input effectively.
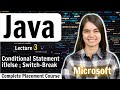
Mastering Java: Understanding Conditional Statements for Beginners
Dive into Java's conditional statements, including 'if', 'switch', and 'break', to enhance your programming skills.
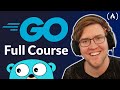
Unlocking the Power of Go: A Comprehensive Programming Course for Beginners
Learn Go programming with our comprehensive course for beginners. Master the fundamentals and build real-world projects!
Most Viewed Summaries
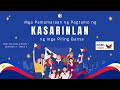
Pamamaraan ng Pagtamo ng Kasarinlan sa Timog Silangang Asya: Isang Pagsusuri
Alamin ang mga pamamaraan ng mga bansa sa Timog Silangang Asya tungo sa kasarinlan at kung paano umusbong ang nasyonalismo sa rehiyon.
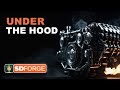
A Comprehensive Guide to Using Stable Diffusion Forge UI
Explore the Stable Diffusion Forge UI, customizable settings, models, and more to enhance your image generation experience.
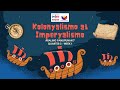
Kolonyalismo at Imperyalismo: Ang Kasaysayan ng Pagsakop sa Pilipinas
Tuklasin ang kasaysayan ng kolonyalismo at imperyalismo sa Pilipinas sa pamamagitan ni Ferdinand Magellan.
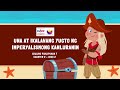
Imperyalismong Kanluranin: Unang at Ikalawang Yugto ng Pananakop
Tuklasin ang kasaysayan ng imperyalismong Kanluranin at mga yugto nito mula sa unang explorasyon hanggang sa mataas na imperyalismo.
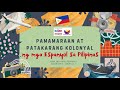
Pamaraan at Patakarang Kolonyal ng mga Espanyol sa Pilipinas
Tuklasin ang mga pamamaraan at patakarang kolonyal ng mga Espanyol sa Pilipinas at ang mga epekto nito sa mga Pilipino.