Introduction
Java is one of the most popular programming languages used worldwide. In this article, we will delve into some basic yet essential fundamentals of Java programming, specifically focusing on how to output data, understand variables, recognize data types, and take user input. These concepts are crucial for anyone beginning their journey in Java programming.
Output in Java
When starting with Java, one of the first things programmers learn is how to display output. The simplest way to output data in Java is through the System.out.println()
statement. Let's explore this in more detail.
The Syntax for Output
To print a message to the console, we use:
System.out.println("Hello, World!");
Here, System
is a class, out
is an instance of PrintStream
, and println
is a method that prints the given message followed by a new line.
Understanding Output Statements
- Use double quotes for strings:
- Correct:
System.out.println("Hello!");
- Incorrect:
System.out.println('Hello!');
- Correct:
- Every statement must end with a semicolon (
;
).
This means that until the semicolon is added, the command won't execute. As good practice, always ensure to terminate your statements properly.
Different Output Methods
print()
: Outputs text without a newline after.System.out.print("Hello, "); System.out.print("World!");
println()
: Outputs text and adds a newline after it.System.out.println("Hello, World!");
- Using newline characters: You can use
System.out.println("Line 1\nLine 2");
Example Code
Here's a simple program demonstrating output in Java:
public class Main {
public static void main(String[] args) {
System.out.println("Hello, World!");
System.out.println("Welcome to Java!");
}
}
Working with Variables
After understanding how to output variables, the next vital concept is understanding variables themselves. Variables are the building blocks of any Java program.
What Are Variables?
A variable in Java is a container that holds data that can vary at execution. For example:
int a = 10;
int b = 5;
In the above code, a
and b
are variable names, while 10
and 5
are the values they store.
Data Types in Java
It is essential to define what type of data a variable holds; this helps in memory management. Java is a strongly typed language, and it requires that every variable be declared with a data type:
- Primitive Data Types:
int
: For integers (e.g.,int x = 5;
)double
: For floating-point numbers (e.g., `double pi = 3.14;")char
: For characters (e.g.,char letter = 'A';
)boolean
: To store true/false values (e.g.,boolean isJavaFun = true;
)
- Non-Primitive Data Types: Strings, Arrays, Classes, etc.
Example Code for Variables
public class VariablesExample {
public static void main(String[] args) {
int x = 10;
double y = 20.5;
String name = "Java";
System.out.println(x + y);
System.out.println("The name is " + name);
}
}
Taking User Input in Java
User input is fundamental in making interactive programs. In Java, the Scanner
class is used to obtain input from the user.
Creating a Scanner Object
To take input, you must first import the class and create a Scanner
object:
import java.util.Scanner;
public class InputExample {
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
System.out.print("Enter your name: ");
String name = scanner.nextLine();
System.out.println("Hello, " + name);
}
}
Methods for Input
nextInt()
: To read integersnextDouble()
: To read floating point numbersnext()
: To read a single word (token)nextLine()
: To read a full line of input
Example Program
Here’s a complete example that takes two numbers as input and prints their sum:
import java.util.Scanner;
public class SumExample {
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
System.out.print("Enter first number: ");
int a = scanner.nextInt();
System.out.print("Enter second number: ");
int b = scanner.nextInt();
int sum = a + b;
System.out.println("The sum is: " + sum);
}
}
Conclusion
Understanding how to output data, manage variables, utilize data types, and take user inputs are foundational skills in Java programming. Mastering these concepts will help you as you continue to explore more advanced topics in Java and programming in general. As you practice coding, make sure to experiment with these features to strengthen your grasp on Java!
हाय एवरीवन एंड वेलकम टू अपना कॉलेज और आज हम बात करने वाले हैं जावा के कुछ बेसिक फंडामेंटल टॉपिक्स की इन टॉपिक्स के अंदर
आज हम बात करेंगे आउटपुट कैसे देते हैं जावा में वेरिएबल क्या होते हैं डेटा टाइप्स क्या होते हैं और एक्चुअली यूजर से
अगर हमें इनपुट लेना है तो वो कैसे करेंगे हम जावा के अंदर इसमें सबसे पहले बात करेंगे पहले टॉपिक की यानी आउटपुट कैसे
लेते हैं जावा के अंदर अब आउटपुट के लिए हमें एक लाइन को शुरुआत से ही याद करके रखना है यह लाइन है हमारी सिम ड आड प्रिंट
हेलो वर्ल्ड जब हेलो वर्ल्ड इसमें एक वेरिएबल चीज है जिसको आपको याद नहीं रखना ब बेसिक सिंटेक्स कीय लाइन कैसे लिखी जा
रही है उसे हमें याद रखना है अब इसका क्या मतलब है यहां पर आउटपुट के लिए हम एक सिस्टम क्लास का यूज करते हैं जावा के
अंदर क्लासेस क्या होती हैं यह हम एक ऊप्स नाम के टॉपिक में बाद में पढ़ेंगे कि क्लासेस क्या होती है ऑब्जेक्ट्स क्या
होती हैं और भी बहुत सारे टॉपिक्स पर अभी के लिए हमें मान के चलना है कि जब भी हम इस लाइन को लिख रहे होंगे मतलब
हमारे लिए जावा में आउटपुट प्रिंट हो जाएगा और क्या आउटपुट प्रिंट होगा कि जब भी ये पैरेंस यानी ये जो ब्रेसेज ये जो
ब्रैकेट्स लगे हुए हैं हमारे सेंटेंस में इसके अंदर अगर हम डबल कोट्स लगा दें और डबल कोट्स के बीच में हम जो भी चीज
लिखेंगे वो हमारे लिए हमारी विंडो में यानी कंसोल स्क्रीन में जब हम कोड लिख रहे होंगे उस टाइम पर हमारे यूजर के लिए
प्रिंट हो जाएगी उसके साथ के साथ इस लाइन के बाद एक सेमीकलन आता है तो जावा में सारे जो सेंटेंसेस होते हैं सारी कमांड्स
जो होती हैं वो सेमीकलन से खत्म होती हैं जब तक आप सेमीकलन नहीं लगाते तब तक आपकी लाइन चलती रहेगी जैसे इंग्लिश में अगर
हमें किसी लाइन को टर्मिनेट करना होता है खत्म करना होता है तो हम वहां पर डॉट लगा देते हैं हिंदी में हम एक डंडा लगा देते
हैं उसी तरीके से जावा के अंदर या बहुत बत सारी प्रोग्रामिंग लैंग्वेजेस के अंदर हम सेमीकलन लगाते हैं यह दिखाने के लिए कि
यहां पर हमारी लाइन यहां पर हमारी कमांड खत्म हो गई है अब बात करें इस आउटपुट की तो यहां पर डबल कोट्स की जगह बहुत सारे
स्टूडेंट्स पूछते हैं कि क्या अगर हम सिंगल कोट्स लगाने तो भी क्या हमारा जो आउटपुट है वो प्रिंट हो रहा होगा तो इसका
जवाब है हां अगर आप डबल कोट्स की जगह सिंगल कोट्स यूज करते हैं तो भी आपका आउटपुट प्रिंट हो जाएगा लेकिन यूजुअली बाय
कन्वेंशन लैंग्वेजेस में ज जावा में जब भी हम प्रिंट करवाते हैं तो डबल कोट्स यूज़ करते हैं स्ट्रिंग्स के लिए क्योंकि यहां
पर एक तरीके से हेलो वर्ल्ड एक स्ट्रिंग है कैरेक्टर्स की मतलब कैरेक्टर्स को जोड़ के हमने एक सेंटेंस बना दिया एक वर्ड बना
दिया टोकेंस का कलेक्शन बना दिया जिसे अब हम प्रिंट करवा रहे हैं अब ये हमने काफी सारी बात कर ली है इस पूरी की पूरी
स्टेटमेंट के बारे में इसको बहुत अच्छे से समझ पाएंगे जब हम कोड कर रहे होंगे तो इसको एक्चुअली एडिटर में हम लिखकर देखते
हैं यहां पर जो हम एडिटर यूज कर रहे हैं दैट इज इंटे लीजिए अब इसके अलावा आप नेट बनस एक्लिप्स जैसे कोई और एडिटर भी यूज कर
सकते हैं अब इस कोड के अंदर हम देख पाएंगे कि यहां पर कुछ पैकेज जैसी स्टेटमेंट है कुछ लिखा हुआ है यहां पर एक क्लास हमें
दिख रही है इस क्लास का नाम हम समझ सकते हैं कि मेन है यहां पर कुछ पब्लिक स्टैटिक वॉइड लिखा हुआ है इसका नाम भी मेन है इसके
अंदर कुछ-कुछ लाइने लिखी हुई हैं तो इस पूरे के पूरे कोड को जो बाय डिफॉल्ट आता है किसी भी एडिटर के अंदर इसको बॉयलर
प्लेट कोड कहते हैं बॉयलर प्लेट कोड यानी जो कोड पहले से बाय डिफॉल्ट हमें मिला होता है इस कोड के बारे में हम धीरे-धीरे
आने वाले लेक्चर में और डिटेल में पढ़ रहे होंगे कि क्लासेस क्या होती हैं ये फंक्शन क्या होता है पैरामीटर्स क्या होते हैं
पैकेज को इंपोर्ट कैसे करते हैं पैकेज को डिफाइन कैसे करते हैं कौन से पैकेज के अंदर हमारी फाइलें हैं तो ये सारी चीजों
के बारे में हमें अभी टेंशन लेने की जरूरत नहीं है हमें मानना है कि एडिटर पर हमें जो ये बॉयलर प्लेट बाय डिफॉल्ट कोड दिख
रहा है वो ठीक है हम इसी के अंदर अपनी प्रोग्रामिंग करेंगे जितना भी हम कोड करने वाले हैं जब तक हम बाकी चीजें सीख नहीं
लेते वो सारा का सारा कोड हम इस मेन फंक्शन के अंदर लिखेंगे यानी इतनी स्पेस में यहां से लेकर अगर नेक्स्ट लाइन तो इस
पूरी स्पेस के अंदर इस मेन के अंदर जब ये कर्ली ब्रेसेज से शुरू हो रहा है कर्ली ब्रेसेज से एंड हो रहा है इस स्पेस में हम
सारा का सारा अपना कोड लिखेंगे और उसको एग्जीक्यूट करेंगे तो हम बात कर रहे हैं जावा में आउटपुट की आउटपुट के लिए हमने एक
स्टेटमेंट सीखी है अभी उसको लिख कर देखते हैं स्टेटमेंट थी सिस्टम ड आउटड प्रिंट और प्रिंट में हमने लगाए थे डबल
कोट्स हमने लिखा था हेलो वर्ल्ड और बाद में लगाया था सेमीकलन यह दिखाने के लिए कि यहां पर हमारी लाइन
टर्मिनेट कर गई खत्म हो गई इस कोड को हमने लिख लिया अब यहां पर इंटेलीज में ऊपर जाकर ग्रीन कलर का एक रन बटन होता है कि आपका
कोड रन कर जाए जितना भी आपने लिख दिया इस ग्रीन बटन को हम क्लिक करेंगे तो हमारे लिए नीचे एक आउटपुट स्क्रीन हमें दिख रही
होगी इसको थोड़ा एक्सपेंड कर लेते हैं हम और इस स्क्रीन के अंदर कुछ-कुछ आउटपुट प्रिंट हुआ है ऊपर कुछ हमें लोकेशन जैसा
कुछ दिखाई दे रहा है बट बीच में जो प्रिंट हुआ है वो है हमारा एक्चुअल आउटपुट यानी हेलो वर्ल्ड अब हेलो वर्ल्ड प्रिंट हुआ है
उसके बाद प्रिंट हुआ है प्रोसेस फिनिश्ड विद एग्जिट कोड जीरो ये क्यों प्रिंट हुआ है ये तो हमने डबल कोर्स में नहीं लिखा था
अब इसका मतलब है कि हमारा जो कोड है वो बिना किसी एरर के चल गया है उसमें कोई एरर कोई परेशानी नहीं आई और हमारा जो आउटपुट
है वो हमारे लिए प्रिंट हो गया अब इस डबल कोट्स के अंदर हम हेलो वर्ल्ड विद जावा भी अगर लिख देते और उसे दोबारा हम एग्जीक्यूट
कराते तो आप देखेंगे कि अब हमारी स्ट्रिंग चेंज हो गई है और अब हमारे लिए प्रिंट हो गया है हेलो वर्ल्ड विद जावा तो बेसिकली
इन डबल कोट्स के अंदर हम जो भी कुछ प्रिंट करवाना चाहेंगे वो हमारी स्क्रीन पर प्रिंट होकर आ जाएगा एज अ स्ट्रिंग एज अ
सेंटेंस अब इसके अलावा बात करें तो प्रिंट जो चीज है वह एक फंक्शन है जावा के अंदर मतलब इस फंक्शन के अंदर आपने स्ट्रिंग पास
कर दी है और एक तरीके से जो भी आप इस फंक्शन के अंदर पास करेंगे वह हमारे लिए प्रिंट हो जाएगा इस विंडो में अब बहुत
सारे स्टूडेंट्स जो अलग-अलग लैंग्वेजेस से आते हैं जिन्होंने पहले c+ प या पाइथन सीखी है वो समझ पा रहे होंगे कि फंक्शन के
अंदर पास किया तो प्रिंट हो गया नए स्टूडेंट्स को शायद कोई परेशानी हो पर वह धीरे-धीरे टाइम के साथ हमें आदत हो जाएगी
अभी के लिए हम समझ सकते हैं कि ये एक फंक्शन है ये एक क्लास है और ये है हमारी स्ट्रिंग जिसे हमने प्रिंट करवाया था अब
इसके अलावा एक और तरीका होता है जावा में प्रिंट करवाने का उस तरीके को समझने के लिए सबसे पहले हम क्या करते हैं इसी लाइन
को कॉपी कर देते हैं कंट्रोल सी कंट्रोल v हमने इस लाइन को कॉपी कर दिया अब अगर इस कोड को हम रन करें तो हमारे लिए आउटपुट
स्क्रीन पर जो प्रिंट होगा वो कुछ ऐसा होगा हेलो वर्ल्ड विद जावा एंड हेलो वर्ल्ड विद
जावा अब यह तो सेम लाइन में प्रिंट हो गया बट हमें क्या चाहिए हमें अलग-अलग लाइन पे चाहिए तो हम क्या चाहते हैं कि जब भी पहले
हमारा प्रिंट हो जाए उसके बाद हम नेक्स्ट लाइन पर अगली चीज प्रिंट करें और यह चीज कैसे पॉसिबल होगी यह पॉसिबल होगी जावा के
अंदर एक और फंक्शन की हेल्प से जिसको हम नाम देते हैं प्रिंट एल अब यहां पर अगर हम प्रिंट की जगह प्रिंट एन लिख द तो क्या हो
जाएगा अब इस कोड को दोबारा रन करें तो हमारे लिए आउटपुट कुछ हमें ऐसा दिखेगा जहां पर हेलो वर्ल्ड विद जावा पहली लाइन
में प्रिंट हुआ और प्रिंट की जगह प्रिंट एलन यूज करने की वजह से पहली जब लाइन प्रिंट हुई होगी उसके बाद नेक्स्ट लाइन आ
गई होगी अब यह वाली लाइन प्रिंट हुई अगर हम एक और थर्ड टाइम कुछ प्रिंट करवाना चाहते तो इसको भी नेक्स्ट लाइन में लाने
के लिए हम यहां पर कर देते प्रिंट एलन और इसे भी दोबारा रन करें तो हमारे लिए जो तीनों लाइंस हैं वो एक के बाद एक के बाद
एक प्रिंट होंगी जिस तरीके से हम चाहते हैं तो हमने दो मेथड्स देख लिए जावा में आउटपुट प्रिंट करवाने के एक यूज करता है
प्रिंट जिसमें नेक्स्ट लाइन नहीं होती एक यूज करता है प्रिंट एलन जिसमें नेक्स्ट लाइन वो दे देता है आउटपुट कुछ भी प्रिंट
करवाने के बाद इसके अलावा एक लाइन का गैप छोड़ने के लिए एक और तरीका होता है जावा में वो ये होता है कि यहां से अगर हम
प्रिंट एलन हटा दें और अपनी इस स्ट्रिंग के अंदर मतलब डबल कोट्स के अंदर हम लिख दें बै स्ल ए बै स्ल
ए यानी नेक्स्ट लाइन मतलब आप इस आउटपुट को प्रिंट कर दीजिए और उसके बाद एक नेक्स्ट लाइन प चले जाइए एक नेक्स्ट लाइन प आपकी
आगे वाली जो चीजें हैं वो सारी हो रही होंगी हमारी स्क्रीन के ऊपर सेम चीज हम नीचे वाली लाइन में कर देते हैं बै स्ल ए
अब इस कोड को दोबारा से रन करें तो हम जो आउटपुट ऑब्जर्व करेंगे वो सेम आउटपुट होगा यानी पहले जैसा अब हमने प्रिंट एल एन यूज
नहीं नहीं किया सिर्फ प्रिंट यूज किया लेकिन बै स्ल ए की हेल्प से हमारे पास एक नेक्स्ट लाइन आ गई जिसकी वजह से हमारा जो
आउटपुट है वो नेक्स्ट लाइन के अंदर चला गया तो इस तरीके से जावा के अंदर तीन तरीके होते हैं आउटपुट को प्रिंट करवाने
के इन तरीकों के बारे में और थोड़ा समझे तो इनमें से सबसे पहला तरीका आता है हमारा प्रिंट का प्रिंट करने का मतलब हम लाइन
लिखेंगे सिस्टम डॉट आउट डॉट प्रिंट इस प्रिंट के अंदर डबल कोट्स या सिंगल कोट्स में हम जो भी हमें प्रिंट करवाना है उसको
लिख देंगे और एक सेमीकलन लगा देंगे तो हमारे लिए आउटपुट प्रिंट हो जाएगा बट उस आउटपुट में नेक्स्ट लाइन की जगह नहीं होगी
मतलब उस आउटपुट को लिखने के बाद आप कुछ और प्रिंट करवाना चाहते हैं तो वो भी सेम लाइन में प्रिंट होगा लेकिन आप चाहते हैं
कि मैं जो भी प्रिंट करवा दूं उसके बाद उसी लाइन में कुछ ना प्रिंट हो सब कुछ नेक्स्ट लाइन में प्रिंट हो उसके लिए हम
यूज करते हैं प्रिंट एलन प्रिंट एलन को भी इसी तरीके से यूज करते हैं सिम आड प्रिंट ए ए फिर लगाते हैं डबल कोट्स जो
हमें लिखना होता है वह हम लिख देते हैं फिर लगाते हैं टर्मिनेटर यानी हमारा सेमीकलन और इसकी वजह से हमारा जो भी
आउटपुट है वह प्रिंट होने के बाद हमें एक नेक्स्ट लाइन दे देता है इसके अलावा एक और तरीका होता है नेक्स्ट लाइन प्रिंट करने
का जिसमें हम लिखते हैं ब n जब भी किसी सेंटेंस में बै स् n लगा देते हैं तो वहां पर हमारा उससे पहले जो भी प्रिंट हुआ है
और उसके बाद जो भी प्रिंट हुआ है सपोज करते हैं पहले प्रिंट होना था ए बाद में प्रिंट होना था बी तो a के बाद बी जब भी
प्रिंट होगा तो वो नेक्स्ट लाइन में जाकर प्रिंट होगा सेम लाइन में प्रिंट नहीं होगा इसका एक एग्जांपल कोड में देखते हैं
अगर हम अपनी दोनों लाइंस को हटा दें और यहां पर लिखें हेलो वर्ल्ड विद जावा फ्रॉम अपनी
कक्षा और इसे अगर हम रन करें तो आउटपुट में देखेंगे कि हेलो वर्ल्ड विद जावा तो पहले प्रिंट हुआ और
फ्रॉम अपनी कक्षा प्रिंट हुआ नेक्स्ट लाइन में तो यही मैजिक है ब n का जो भी उसके बाद होगा वो नेक्स्ट लाइन में प्रिंट होता
जाएगा अगर हम यहां पर दो ब n लगा दें तो क्या होगा तो हेलो वर्ल्ड विद जावा प्रिंट होगा
फर्स्ट लाइन में फ्रॉम प्रिंट होगा सेकंड लाइन में और बै स् ए के बाद जो भी है वो प्रिंट हो रहा होगा उससे नेक्स्ट लाइन में
अब यहां पर एक ये जो छोटी सी स्पेस आई है अपनी के a से पहले य आई है क्योंकि बैक स्ल ए के बाद हमने एक स्पेस दिया था वहां
पर तो उसकी हमें टेंशन नहीं लेनी स्पेस को हम ऐसे करके हटा सकते हैं तो इस तरीके से हमने आउटपुट करने के तीन तरीके देख लिए
हैं अब इसके बाद बात करते हैं एक क्वेश्चन की एक सिंपल सा क्वेश्चन सॉल्व करके देखते हैं आउटपुट के लिए क्वेश्चन है प्रिंट द
पैटर्न पैटर्न क्या दिया है हमें स्टार्स प्रिंट करने हैं स्टार्स कुछ इस तरीके से प्रिंट करने हैं कि पहली लाइन में हमारे
पास सिंगल स्टार हो दूसरी लाइन में हमारे पास पास दो स्टार हो उसी तरीके से तीसरी लाइन में हमारे पास तीन स्टार्स हो चौथी
लाइन में चार स्टार्स हो यह थोड़ा सा हमें एक पैटर्न प्रिंट करना है जो आपको यहां पर बहुत अच्छे से
डिस्प्ले भी किया गया है इस पैटर्न को हम किस तरीके से प्रिंट करेंगे हम देख पा रहे हैं कि इस क्वेश्चन में सबसे पहले तो हमें
एक स्टार प्रिंट करना है यानी प्रिंट स्टेटमेंट जिसको शॉर्ट में हमने साउटरपेट भी एक प्रिंट स्टेटमेंट और उसमें दो स्टार
लगाने पड़ेंगे वैसे ही एक तीसरी लाइन लिखनी पड़ेगी जिसमें प्रिंट स्टेटमेंट और उसमें कुछ हमें तीन स्टार लगाने पड़ेंगे
सारे के सारे डबल कोट्स के अंदर तो ऐसा सा कुछ हमें करना पड़ेगा जिसकी हेल्प से हम प्रिंट कर पाएंगे अब इसी चीज को अगर कोड
की हेल्प से करने की कोशिश करें तो अपना पुराना कोड हम हटा देते हैं अब इंटेलीज के अंदर एक शॉर्टकट होता है system.out
प्रिंट एलन या हमें पूरी की पूरी स्टेटमेंट ना लिखनी पड़े उसकी जगह तो जब भी हम साट प्रिंट करते हैं जब भी हम साट
लिखते हैं उसके बाद कीबोर्ड पर एक टैब होता है लेफ्ट साइड में उस टैब को जब हम प्रेस कर देंगे तो हमारे पास सिस्टम डड
प्रिंट पूरा का पूरा आ जाता है इंटेलीज पर उसमें हम अपने आउटपुट को लिख सकते हैं उसमें हम लिखेंगे स्टार अभ हमने एक स्टार
लिख लिया तो एक लाइन में यह प्रिंट हो जाएगा उसके बाद नेक्स्ट लाइन में हमें प्रिंट करवाना है तो यहां पर लिख देंगे दो
स्टार अभी हम यहां पर एल ए यूज कर रहे हैं एलएन इसलिए क्योंकि पहली लाइन में हम सिर्फ एक ही स्टार चाहते हैं उसके बाद
नेक्स्ट लाइन चाहते हैं फिर नेक्स्ट लाइन में अगले स्टार्स चाहते हैं अब सेम चीज हम चार बार कर दें तो यहां पर प्रिंट
करवाएंगे तीन स्टार्स यहां पर प्रिंट करवाएंगे चार स्टार्स तो इस तरीके से हमने चार स्टेटमेंट्स लिखी हैं अब इसे रन करके
देखते हैं तो हमारे लिए आउटपुट निकल कर आया है ये जिसमें पहली लाइन में वन स्टार दूसरी
लाइन में टू स्टार्स तीसरी लाइन में तीन और उसी तरीके से फोर्थ लाइन में चार सात तो हमने अपने क्वेश्चन को सॉल्व कर दिया
है चार प्रिंट स्टेटमेंट्स को यूज़ करके अब आगे जाकर हम जब लूप्स वाला टॉपिक पढ़ रहे होंगे उसमें जब पैटर्स पढ़ रहे होंगे
तो उसके अंदर और भी ऑप्टिमाइज तरीके हम सीख रहे होंगे बट अभी हम बिगिनर्स स्टेज में हैं इसीलिए हम बार-बार सेम लाइन को
लिखकर इस चीज को प्रिंट करवा रहे हैं धीरे-धीरे हम अपने कोड्स को ऑप्टिमाइज करेंगे बेटर टाइम
कॉम्प्लेक्टेड में हम बहुत सारा आउटपुट डिस्प्ले करेंगे इस इसके बाद एक क्विज की बात करते हैं सेम आउटपुट के लिए अब इतने
सारे क्विज एंड क्वेश्चंस आपको इसलिए कराए जा रहे हैं ताकि ये जो टॉपिक है ये इंपॉर्टेंट टॉपिक हैं जिन्हें हमें शुरुआत
से ही याद करते हुए चलना है और बार-बार इनके थोड़े क्वेश्चंस हम प्रैक्टिस कर लेंगे तो हमारे दिमाग में थोड़ी चीजें बैठ
जाएंगी तो हमें काफी हेल्प होगी जब आगे जाकर हम और एडवांस चीजें सीख रहे होंगे तो ये बेसिक चीजें हमें याद होंगी बैक ऑफ द
माइंड पे होंगी तो क्विज की बात करते हैं क्विज में एक क्वेश्चन है हमें प्रिंट करवाना है स्टार और ने लाइन में दो स्टार
ठीक है उसके लिए हमें तीन ऑप्शंस दे दिए कोड के हमें बताना है इन तीनों ऑप्शन में से कौन सा कोड करेक्ट है मतलब अगर इन
तीनों की बात करें तो इन तीनों में से कौन सा जो है कोड वो एक्चुअली आउटपुट में यह हमें दे देगा उसकी बात करते हैं अब सबसे
पहले एनालाइज करते हैं ए को ए को जब हम एनालाइज करेंगे तो उसमें हमें दिया है सिस्टम ड आड प्रिंट एलन प्रिंट दे दिया
अंदर लिखा है स्टार ब n उसके साथ-साथ दो स्टार्स अब इसे प्रिंट करवाने की कोशिश करते हैं तो सबसे पहले तो प्रिंट होगा
स्टार स्टार प्रिंट हुआ उसके बाद आ गया बै स्ल n यानी अब इस सेम लाइन पर कुछ भी प्रिंट नहीं होगा सब कुछ इसके बाद प्रिंट
होगा तो बै स् n का मतलब हम नेक्स्ट लाइन पर आ गए नेक्स्ट लाइन पर हम प्रिंट करवाएंगे दो स्टार्स तो दो स्टार्स हमारे
लिए प्रिंट हो गए इस चीज को अगर आउटपुट से मैच करें तो यह कंप्लीट मैच हो रही है मतलब आंसर ए ही हमारा करेक्ट आंसर है
लेकिन हम बाकी दोनों स्टेटमेंट्स को भी एनालाइज करेंगे अब b की अगर बात करें तो b में है हमारे पास स्टार स्टार फिर बै स् n
फिर एक और स्टार तो सबसे पहले तो हम प्रिंट करेंगे स्टार स्टार सेम लाइन में क्योंकि बै स्ल n नहीं आया तो दो स्टार
प्रिंट हुए फिर आया बै n यानी हम नेक्स्ट लाइन में चले गए फिर आया एक और स्टार तो हम नेक्स्ट लाइन में जाकर स्टार प्रिंट कर
देंगे अब यह जो आउटपुट आया हमारे लिए यह हमारे इनपुट के मतलब जो क्वेश्चन में दिया था उसके सेम नहीं है इसीलिए इसे हम कर
देंगे डिस्कार्ड क्योंकि यह गलत कोड है उस आउटपुट के हिसाब से जो हमें चाहिए था इसके बाद बात करते हैं c की c के अंदर हमें
दिया है स्टार स्टार स्टार n बस तो इसमें क्या होगा स्टार स्टार स्टार तीन स्टार सेम लाइन पर प्रिंट हो जाएंगी एंड उसके
बाद आएगा बै स् n यानी हम नेक्स्ट लाइन पर चले जाएंगे पर उसके बाद कुछ नहीं है मतलब ये लाइन हमारी खाली रहेगी तो हमारा आउटपुट
आएगा ये जो कि गलत आउटपुट है जो हमें चाहिए था उसके हिसाब से इसीलिए हमारा जो करेक्ट आंसर है वो है ए तो हमारे क्विज
में जो हम करेक्ट आंसर लिखेंगे वो होगा ए तो इस तरीके से हमने क्विज को भी सॉल्व कर लिया एंड आई होप कि काफी अच्छे से हमें
समझ आ पा रहा होगा कि हम आउटपुट कैसे देते हैं जावा के अंदर कौन से दो से तीन पॉपुलर तरीके हैं जिसके अंदर हम आउटपुट दिखा सकते
हैं जावा के अंदर अलग-अलग चीजों के लिए अब बात करते हैं जावा के अंदर वेरिएबल की वेरिएबल एक बहुत ही इंपॉर्टेंट टॉपिक है
जावा का सारा का सारा जो कोड होता है इवन आप बेसिक प्रोग्रामिंग के बाद जब कंपटिंग करते हैं डीएसए करते हैं तो वेरिएबल काफी
इंपॉर्टेंट है बेसिकली बिल्डिंग ब्लॉक्स हैं जावा के तो वेरिएबल क्या होता है जैसे कि 11 12थ के अंदर हम मैथ्स में पढ़ते थे
कि हमारे वेरिएबल होते थे जैसे कि हमें फॉर एग्जांपल एक क्वेश्चन दे दिया इवन सिक्सथ सेवंथ क्लास में जब हमें रेक्टेंगल
स्क्वायर सर्कल इन के एरिया निकालने होते थे सपोज करते हैं हमें दिया है यह रेक्टेंगल जिसमें एक साइड की लेंथ है a
दूसरी साइड की लेंथ है b अब a की वैल्यू हमने ले ली 25 b की वैल्यू हमने ले ली 10 इसका अगर हमें पेरीमीटर निकालना है तो
उसका फार्मूला होता था 2 * a + b तो 2 * a + b फॉर्मूला है तो इस फॉर्मूले के अंदर मैथ्स की अगर याद करें मैथ्स को याद करें
तो उसके अंदर a जो था वो एक वेरिएबल था b एक वेरिएबल था ये वेरिएबल कैसे थे क्योंकि इनकी वैल्यू फिक्स्ड नहीं थी चेंज होती
रहती थी अब अगले क्वेश्चन में एक और रेक्टेंगल ले सकते हैं उसमें दो और तीन रख सकते हैं लेंथ इसको आप a ले लीजिए इसको b
ले लीजिए तो जो वैल्यू है वो अब चेंज हो गई नाम तो a और b है लेकिन इनकी वैल्यू चेंज हो गई इसीलिए इन्हें वेरिएबल कहा
जाता है तो अगर इस पेरीमीटर को ध्यान से देखें तो इसमें a और b दोनों की वैल्यू फिक्स नहीं है चेंज हो सकती है तो इन्हें
हम कह देते हैं वेरिएबल लेकिन कुछ चीजें ऐसी होती है जिनकी वैल्यू फिक्स्ड होती है इन चीजों को हम कह देते हैं कांस्टेंट
जैसे पेरीमीटर में टू एक कांस्टेंट है हमें पता है कि टू की वैल्यू टू ही रहती है जैसे अगर पा की वैल्यू ले यानी
3.14 वो हमेशा 3.14 यानी 22/7 ही रहेगी इसीलिए उसे कांस्टेंट कहते हैं या फिर कैरेक्टर्स के एग्जांपल ले ले a b c d अब
d की वैल्यू कभी 35 या d की वैल्यू कभी माय नेम या d की वैल्यू कभी ट दरे d की वैल्यू f नहीं हो सकती d की वैल्यू d ही
रहेगी इसीलिए इन सारे वर्ड्स को इन सारे कैरेक्टर्स को इन सारे नंबर्स को हम कहते हैं कांस्टेंट्स जिनकी वैल्यू कभी चेंज
नहीं होती तो अब समझने की कोशिश करते हैं कि जावा के अंदर वेरिएबल को डिफाइन करना मैथ्स के अंदर वेरिएबल को डिफाइन करने से
डिफरेंट कैसे हैं हम सबसे पहले बात करते हैं कंप्यूटर के अंदर की मेमोरी की ये मेमरी है हमारे पास जिसके अंदर सारा का
सारा डाटा हमारा स्टोर होता है किसी भी कंप्यूटर के अंदर इसके अर अंदर डेटा को मान लेते हैं कि वह स्टोर होता है
छोटे-छोटे ब्लॉक्स में यानी इस मेमोरी को बहुत सारे पार्ट्स में डिवाइड कर दिया गया है एक तरीके से और हर पार्ट का अपना एक
एड्रेस है मतलब इस पार्ट का कुछ और एड्रेस है इसका कुछ और एड्रेस है इसका कुछ और एड्रेस है हर एक डिफरेंट एड्रेस पर कोई
डिफरेंट डाटा स्टोर होता है तो इस मेमोरी के अंदर छोटे-छोटे ब्लॉक्स हैं जिनके अंदर अलग-अलग टाइप का डाटा बहुत सारा डाटा
स्टोर होता है कंप्यूटर के अंदर अब जब भी हम जावा के अंदर वेरिएबल को डिफाइन करते हैं यानी फॉर एग्जांपल हम लिख देते हैं
इंट a = 25 इंट b = 10 अब यहां पर हम थोड़ी देर के लिए इग्नोर करेंगे कि ये इंट क्या होता है
इसके बारे में बात करेंगे थोड़ी देर में बट एक तरीके से a को हमने बता दिया है कि a एक चीज है एक वेरिएबल है जिसकी वैल्यू
25 है b एक वेरिएबल है जिसकी वैल्यू 10 है यह कहने का क्या मतलब हुआ इसका मतलब हुआ कि मेमोरी में आप आप एक ब्लॉक पर जाइए उस
ब्लॉक में आप 25 स्टोर कर दीजिए तो 25 स्टोर कर दिया और उस ब्लॉक को आप नाम दे दीजिए
a इसी तरीके से वेरिएबल बस डिफाइन हो गया जावा के अंदर कुछ सेम तरीके की चीज b के लिए हुई होगी मतलब मेमोरी के अंदर हम गए
होंगे उस मेमोरी लोकेशन को हमने नाम दे दिया होगा b कि वेरिएबल b है तो मतलब इस मेमोरी को इस वैल्यू की लोकेशन को हम नाम
दे रहे हैं b और यहां पर इस लोकेशन के अंदर हमने स्टोर करा दी उसकी एक्चुअल वैल्यू यानी कि 10
तो अब अगर हमें कुछ और भी स्टोर कराना है जैसे मान लेते हैं अभी तक हम नंबर स्टोर करा रहे थे अब हम कोई स्ट्रिंग स्टोर
करवाना चाहते हैं कि हमें कोई नाम स्टोर कराना है तो हम क्या करेंगे कुछ स्ट्रिंग जैसा लिख देंगे स्ट्रिंग नेम इ इक्वल टू
लेट्स से टोनी स्टार्क तो अब क्या होगा इस स्ट्रिंग को भी थोड़ी देर के लिए इग्नोर करते हैं हमने
कुछ नेम वेरिएबल बनाया है नेम नाम का उसके अंदर वैल्यू स्टोर कराई है टोनी स्टार्क तो री के अंदर यहां पर इस लोकेशन को मतलब
किसी भी रैंडम लोकेशन को हम नाम दे देंगे नेम और इसके अंदर हमारी जो स्ट्रिंग थी वो पूरी की पूरी आकर स्टोर हो जाएगी अब यहां
पर ये जो स्क्वायर्स या रेक्टेंगल हैं ये सेम साइज के दिख रहे होंगे लेकिन मेमोरी के अंदर ये सेम साइज के नहीं होते इन सब
का साइज डिफरेंट होता है जैसे अगर आपको कोई नंबर स्टोर करवाना है इंट टाइप का तो उसका जो साइज है वो अलग होगा एक स्ट्रिंग
के साइज से तो हमें रेक्टेंगल को स्क्वायर्स को देख के ये नहीं सोचना कि सेम साइज में ही स्टोर करा रही है चीजें
सारे के सारे जो डिफरेंट टाइप्स ऑफ डाटा होता है वो सब डिफरेंट साइज के ब्लॉक्स में स्टोर होता है मेमोरी के अंदर बट बात
यही है कि हमारे जो वेरिएबल हैं वो कुछ इस तरीके से हमारी मेमोरी के अंदर स्टोर हो रहे होते हैं अब थोड़ा कोड की हेल्प से
समझते हैं कि वेरिएबल एक्चुअली होते कैसे हैं दिखाई कैसे देते हैं कोड के अंदर क्योंकि थ्योरी हमने डिस्कस कर ली है कोड
में इसको लिखना बहुत जरूरी है तो हम बात कर रहे हैं हमारे कोड में वेरिएबल की स्क्रीन को थोड़ा सा नीचे कर लेते
हैं तो सबसे पहले हम क्या करते हैं एक नेम डिफाइन करते हैं मतलब हमें कोई नाम लिखना है तो वेरिएबल बनाएंगे नेम अभी के लिए इस
वेरिएबल का एक तरीके से टाइप है स्ट्रिंग बट अभी हम बात नहीं कर रहे स्ट्रिंग की हम जावा में वेरिएबल कुछ इस तरीके से डिफाइन
करते हैं नेम हमने लिख दिया उसके अंदर हम कोई भी नेम लिख देंगे तो यह हमने नेम लिख दिया ऐसे ही अगर
हमें रेक्टेंगल की उसकी साइड्स a और b डिफाइन करनी होती तो वह कैसे करते a = 25 b = 10 तो इस तरीके से हमने अलग-अलग
स्टेटमेंट्स लिख दी हैं वेरिएबल को डिफाइन करने के लिए अगर हमें यही एज देनी होती तो वोह हम दे देते उसके अलावा और भी
बहुत सारी चीजें हैं अब मान लेते हैं कि हमने मार्केट से कोई चीज खरीदी कोई पेंसिल खरीदी जो ₹2500000 पैसे की है तो उसका
टाइप कुछ और होगा उसका नाम होगा प्राइस यानी वेरिएबल का नाम प्राइस और उसकी वैल्यू हम लिख देते हैं
25.25 तो इस तरीके से बहुत सारे वेरिएबल हम अपने कोड के अंदर डिफाइन कर सकते हैं अब इन वेरिएबल की खास बात यह है कि इनकी
वैल्यू को हम चेंज कर सकते हैं यानी b की वैल्यू अगर अभी 10 है तो बाद में b की वैल्यू को हम 20 भी कर देंगे कोई एरर नहीं
आएगा उसके अलावा अगर हमारा नेम अभी यह है तो बाद में हम इस नेम की व को भी चेंज करके कुछ और कर सकते
हैं तो हमारी वैल्यू चेंज हो जाएगी कोई एरर नहीं आया अब इसके अलावा बात करते हैं हमारे कोड में किय इंट डबल स्ट्रिंग यह सब
हमने क्या लिखा है वेरिएबल के साथ तो इन सारी चीजों को जावा के अंदर हम कहते हैं डेटा टाइप्स अब डेटा टाइप्स क्या होते हैं
तो बात करें अगर जावा की तो जावा इज अ टाइप्ड लैंग्वेज टाइप्ड लैंग्वेज यानी इस लैंग्वेज के अंदर
कोई भी वेरिएबल आपको बताने से पहले यह बताना पड़ता है कि उस वेरिएबल का टाइप क्या है अगर आप नंबर लिख रहे हैं तो आप
कौन से टाइप का नंबर लिख रहे हैं क्या आप इंटी जर लिख रहे हैं क्या आप फ्लोटिंग वैल्यू वाला नंबर लिख रहे हैं यानी डेसिमल
पॉइंट है उस नंबर के अंदर क्या आप एक स्ट्रिंग डिफाइन कर रहे हैं या क्या आप एक बुलियन या एक कैरेक्टर तो बहुत सारे
टाइप्स होते हैं तो इन टाइप्स को हमें थोड़ा याद रखना है तो जावा के अंदर कोई भी वेरिएबल डिफाइन करने से पहले हमें बताना
पड़ता है कि कि उस वेरिएबल का टाइप क्या है ताकि उसी हिसाब से उसी साइज का ब्लॉक मेमोरी में उसे मिल सके अब बहुत सारी
लैंग्वेजेस होती हैं जैसे जावास्क्रिप्ट एक वीकली टाइप लैंग्वेज है उस लैंग्वेज में आपको बताना नहीं पड़ता कि आप कौन से
टाइप का वेरिएबल बना रहे हैं वहां पर आप वर लिख दीजिए लेट लिख दीजिए तो आपके लिए वेरिएबल अपने आप वो लैंग्वेज डिटेक्ट कर
लेगी लेकिन जावा के अंदर बताना पड़ता है c+ प के अंदर बताना पड़ता है तो बात करें तो डेटा टाइप्स की तो डेटा टाइप्स दो
टाइप्स के होते हैं इनमें सबसे पहले हैं हमारे प्रिमिटिव टाइप यानी बेसिक डेटा टाइप्स जिन्हें हमें याद रखना है उसके
अलावा होते हैं नॉन प्रिमिटिव डेटा टाइप्स नॉन प्रिमिटिव डेटा टाइप्स को हम कस्टमाइज कर सकते हैं अपने हिसाब से बना सकते हैं
हम खुद एज अ प्रोग्रामर भी इनको क्रिएट कर सकते हैं लेकिन जो प्रिमिटिव डेटा टाइप्स हैं वो पहले से जावा के अंदर होते हैं
जावा के अंदर आठ प्रिमिटिव डाटा टाइप्स होते हैं जिनके नाम है बाइट शॉर्ट कैर बुलियन इंट लॉन्ग फ्लोट और डबल अब इन सबके
अलग-अलग काम हैं इन सबके पास जो मेमोरी का साइज है वो अलग-अलग है अगर इनके बारे में बात करें शॉर्ट में तो बाइट के पास जो
मेमोरी का साइज है वो है एक बाइट एक बाइट यानी आठ बिट्स अब हमें पता ही है कंप्यूटर के अंदर कुछ भी चीज स्टोर हो रही थी है
मेमोरी में तो वो रो या वन की फॉर्म में स्टोर होती है एक रो या वन मतलब एक बिट तो इसी तरीके से आठ 0 वन ले ले तो वो एक बाइट
यानी कि आठ बिट्स हो जाएगा तो वन बाइट जो मेमोरी लेता है दैट इज व बाइट उसी तरीके से अगर बात करें तो कैर एक इंपॉर्टेंट
डाटा टाइप है जो दो बाइट्स ले लेता है बुलियन हमारा फिर से एक बाइट लेता है इंट हमारा चार बाइट्स लेता है लॉन्ग आठ लेता
है फ्लोट भी चार लेता है डबल आठ लेता है तो इस तरीके से अलग-अलग जो डेटा टाइप्स है वो अलग-अलग नंबर ऑफ बाइट्स ले रहे होते
हैं मेमोरी के अंदर अब आप बोलेंगे कि हमारे सिस्टम के अंदर तो लेट्स से कि हमारा जो इंट है वो चार नहीं ले रहा दो ले
रहा है तो देयर इज अ फिर डिफरेंस बिटवीन द सिस्टम क्योंकि ये जो बाइट्स हैं ये 64 बिट के हिसाब से होती हैं कुछ-कुछ
सिस्टम्स जो 32 बिट के हिसाब से चलते हैं उनमें मेमोरी एलोकेशन थोड़ा सा कम होता है तो वो सिस्टम टू सिस्टम डिपेंड कर सकता है
बट यूजुअली जब भी हम नंबर्स की बात कर रहे होते हैं तो हम 64 बिट्स यानी इन नंबर्स की बात कर रहे होते हैं अब ये आपको नीचे
डिस्क्रिप्शन बॉक्स के अंदर जो भी लिंक है हमारे नोट्स का उसके अंदर आपको पूरा डिस्क्रिप्शन मिल जाएगा कि इसमें कितनी
बाइट्स हैं तो अभी आपको याद करने की जरूरत नहीं है सिर्फ समझना है कि कितनी बाइट्स हैं अब इस वीडियो के अंदर हम फोकस कर रहे
होंगे मोस्टली अपने प्रिमिटिव डाटा टाइप्स पर तो हम इन्हीं के बारे में मोस्टली बात कर रहे होंगे इन्हीं
के एग्जांपल्स यूज कर रहे होंगे लेकिन धीरे-धीरे आने वाली क्लासेस में हम इन सब को एक्सप्लोर करेंगे इन सब को यूज करेंगे
एंड एक पॉइंट ऐसा आएगा जब आप मतलब सोचेंगे भी नहीं कि आप इन चीजों को यूज कर रहे हैं बस आप ऑटोमेटिक दिमाग से आएगा और आप यूज
कर लेंगे इन चीजों को काफी अच्छे से अब इन्हीं डाटा टाइप्स को थोड़ा सा कोड की हेल्प से प्रैक्टिस कर लेते हैं तु हम
क्या करेंगे अपने दो वेरिएबल बनाएंगे पहला होगा इंट टाइप का यानी इंटी जर टाइप का उस उसम हम वैल्यू स्टोर करा देंगे 10 दूसरा
होगा दूसरे टाइप का उसमें वैल्यू स्टोर करा देंगे हम 25 तो यह हमने स्टोर करा दिया अब हम क्या चाहते हैं हम एक तीसरा
वेरिएबल बनाना चाहते हैं इंट टाइप का ही जिसमें हम सम स्टोर कराएंगे तो कर देंगे a प् बी तो मैथ्स की तरह यहां पर भी a प् बी
हो जाता है इसे अगर हम प्रिंट करवाना चाहे तो s आउट करके टैब इसमें प्रिंट करवा देंगे सम देखें क्या आउटपुट निकल कर आता
है तो हम लिए जो आउटपुट निकल कर आया है दैट इज 35 यानी 10 प् 25 उसी तरीके से अगर यहां पर इसे कर देते हैं कॉमेंट आउट अब
जावा के अंदर हमने कॉमेंट्स ऑलरेडी डिस्कस किए थे कॉमेंट्स वो पार्ट होता है कोड का जिसको हम एग्जीक्यूट नहीं करना चाहते इसको
जो कंपाइलर है वो इग्नोर कर देता है इस पार्ट में अगर आप कोई गलती कर देंगे मान लेते हैं हम सेमीकलन हटा देंगे तो कोई एरर
नहीं आएगा लेकिन ऊपर वाली लाइन में सेमीकलन हटा दिया तो ये रेड लाइन आ गई मतलब यहां एरर है तो
इसीलिए कॉमेंट्स में हम चीजें लिख देते हैं जिनको हम अपने कोड के में इंक्लूड नहीं करना चाहते अगर यहां पर हम ले ले
डिफरेंस डिफरेंस यानी माइनस तो हम यहां कर देंगे b - a और उसे हम प्रिंट करवाना चाहे अगर तो कोड को रन करते हैं तो हमारे लिए
प्रिंट हो गया 15 यानी 25 - 10 तो ऐसे ही वेरिएबल पर जो हमने डिफरेंट डिफरेंट वेरिएबल बनाए हैं कोड के अंदर उन पर आप
डिफरेंट डिफरेंट ऑपरेशंस लगाएंगे तो उनकी वैल्यू इधर-उधर जाती रहेगी अब अगर बात करें वैल्यू इधर-उधर जाने का
मतलब तो कंट्रोल जड कर कर अगर हम वापस अपनी इनिशियल स्टेटमेंट पर आ जाए जो कि यह थी मतलब हमने सम निकाला था a
और बी का तो एक बार एनालाइज करते हैं कि इसका मतलब क्या हुआ सम निकालने का मतलब क्या हुआ सम निकालने का मतलब यह हुआ कि
यहां पर इंट a = 10 करने से एक मेमोरी लोकेशन क्रिएट हुई होगी जिसमें हमने स्टोर किया होगा 10 को एक मेमोरी लोकेशन क्रिएट
हुई होगी जिसमें हमने स्टोर कि होगा 25 को तो हमने जो वैल्यूज है अपोजिट कर दिए हैं अब a + b जब हुआ होगा मतलब 10 + 25 जब हुआ
होगा तो इसका आंसर आएगा 35 जिसे हमने स्टोर किया है एक इंट टाइप की लोकेशन पर जिसका नाम हमने दिया है सम अब सम इ इक्वल
ट 35 तो यहां सम नाम का एक और वेरिएबल बना होगा मेमोरी के अंदर जिसके अंदर हमने स्टोर करवा दिया होगा 35 को तो इस तरीके
से ऑपरेशन परफॉर्म हुआ होगा ए और बी को और फाइनली हमारे पास जो सम है वो एक नया बल क्रिएट हो गया मेमोरी के अंदर जिसके अंदर
वैल्यू स्टोर्ड है 35 तो इस तरीके से जो डिफरेंट कैलकुलेशंस है एक वैल्यू दूसरे को असाइन करना या ऐड कर देना सबकट कर देना
मल्टीप्लाई कर देना यह सारी चीजें हमारे कोड के अंदर हो रही है और मेमोरी में कुछ इस तरीके से हो रही है अब इसके बाद बात
करें अगर मल्टीप्लाई की तो इसे कर देते हैं कॉमेंट आउट और एक और वेरिएबल बनाते हैं
मल्टीप्लाई तो हम लिख देंगे ए अब मैथ्स में ए हम ऐसे ही ख लिख देते थे कि ए बट जावा के अंदर ऐसा नहीं होता इसलिए
ये पोर्शन रेड हो गया है जावा के अंदर आपको मल्टीप्लाई का सिंबल देना पड़ेगा तो आप यहां मल्टीप्लाई लिखेंगे तभी वह करेक्ट
होगा आप फिर प्रिंट करवा सकते हैं अपना मल्टीप्लिकेशन का आउटपुट जो कि हमारे लिए निकल कर आएगा 250 अब मल्टीप्लाई के अलावा
भी डिवाइड क्वेशन बहुत सारे ऑपरेटर्स होते हैं जिनको हम वेरिएबल पर लगा सकते हैं अपने डिफरेंट डाटा टाइप्स के लिए अब इन सब
को हम आने वाले सेक्शंस में बाद में डिस्कस करेंगे जब हम ऑपरेटर्स पढ़ रहे होंगे जब हम टाइप कास्टिंग को डिस्कस कर
रहे होंगे तो व थोड़े से एडवांस्ड हो जाते हैं अभी हम बेसिक चीजें कर रहे हैं तो इस तरीके से बेसिक तरीके से हमने अपने
वेरिएबल को डिफाइन कर लिया है अब इसके बाद बात करते हैं क्विज की तो डेटा टाइप्स पर ही एक क्विज है जिसको हम सॉल्व करेंगे
हमारा क्वेश्चन ये है हमें कैलकुलेट करना है a * b / a - b a की वैल्यू दे दी है 10 b की वैल्यू दे दी है 5 अब ये क्विज थोड़े
आपको 11 12 टाइप्स लग रहे होंगे बट इस तरीके की चीजें जब हम करते हैं तो एक तरीके से हम स्कूल सिस्टम में अपने कोडिंग
सिस्टम में शिफ्ट हो रहे हैं हम कोड को भी देख पा रहे हैं मैथ्स को भी थोड़ा एक्सप्लोर कर पा रहे हैं तो काफी लॉजिकल
कनेक्शन है इन दोनों का तो यह हमें क्वेश्चन दे दिया हमें दो ऑप्शंस दे दिए हैं कि इन दोनों में से कौन सा करेक्ट
आउटपुट हमें दे रहा होगा अब सबसे पहले मैथमेटिकली कैलकुलेट करते हैं 10 * 5 / 10 - 5 इसका आंसर आना चाहिए 50 / 5 यानी कि
10 अब इन दोनों ऑप्शंस को देखेंगे क्या इन दोनों का आंसर 10 आता है या नहीं आता पहले ऑप्शन को देखते हैं पहले हमने लिखा है 10
* 5 / बा 10 - 5 अब जावा के अंदर बॉर्ड मास रूल नहीं चलता बॉड मास यानी मैथ्स वाला रूल जावा के अंदर प्रायोरिटी होती है
ऑपरेटर्स की कि सबसे पहले प्रायोरिटी में आता है मल्टीप्लाई फिर डिवाइड फिर एक मॉड्यूल नाम का ऑपरेटर होता है जिसके बारे
में बाद में बात करेंगे बेसिकली इस ऑपरेटर से हम रिमाइंडर निकाल रहे होते हैं इन तीनों की प्रायोरिटी माइनस और प्लस से
बड़ी होती है यह चीज हमें याद रखनी है अब यह हमने याद रख लिया उसके बाद अगर हम कैलकुलेट करें इस चीज को कैसे करेंगे जावा
के अंदर कैलकुलेशन लेफ्ट टू राइट चलती है तो हम भी लेफ्ट टू राइट चलेंगे सबसे पहले मल्टीप्लाई करेंगे 10 को 5 से 50 आ गया
डिवाइड बा 10 - 5 ये आ गया उसके बाद 50 / 10 हम कर रहे होंगे 10 - 5 नहीं 50 / बा 10 उससे हमारे पास आएगा 5 -5 यानी कि रो
तो पहले वाले ऑप्शन का जो आंसर आया है वो हमारे पास आया है जीरो अब उसी तरीके से दूसरे वाले ऑप्शन को अगर एनालाइज करें तो
इसमें हमारे पास क्या होगा पैरेंस होंगे 10 * 5 डिवाइड बाय 10 - 5 तो सबसे पहले जो वैल्यू सॉल्व होगी वो
सॉल्व होगी पैरेंस के अंदर वाली तो 10 * 5 यानी 50 / 10 - 5 यानी 5 तो 10 / 5 इज 10 अब इस तरीके से हमारे पास आ गया 10 अब आप
सोच रहे होंगे कि यह तो बहुत आसान सा क्विज था ये तो बहुत मैथ्स है इसमें कोडिंग का कोई लेना देना नहीं है तो हमारे
टाइम पर एक टेस्ट हुआ था amazon2 राउंड में amazon2 पर बेस्ड थे तो जैसे कि ऑपरेटर्स
वेरिएबल टाइप्स या फिर उसके अलावा पॉइंट्स भी आ जाता है c+ प में तो ये बहुत बेसिक माने जाते हैं टॉपिक्स इनसे रिलेटेड
क्वेश्चंस उन्होंने पूछ लिए थे और उस उसमें फिर थोड़े बहुत जो स्टूडेंट्स है वो कंफ्यूज हो गए थे जिनके बेसिक फंडामेंटल्स
क्लियर नहीं थे इसलिए फंडामेंटल्स क्लियर रखने हैं और ऐसी चीजों को इग्नोर नहीं करता जो भी चीजें हर बेसिक चीज का हमें
पता होना चाहिए कि एक्सप्रेशंस कैसे कैलकुलेट हो रहे हैं वैल्यू कैसे असाइन हो रही है टाइप कन्वर्जंस कैसे होते हैं जो
हम आगे जाके सीख रहे होंगे तो इस तरीके से हमारा जो आंसर है कोड में वो हमारे लिए निकल कर आया है बी ऑप्शन अब बात करते हैं
जावा में वन ऑफ द मोस्ट इंपोर्टेंट टॉपिक्स की जो है जावा के अंदर इनपुट लेना जावा के अंदर इनपुट हम एक स्कैनर क्लास की
हेल्प से लेते हैं तो एक स्टेटमेंट लिखते हैं तो स्टेटमेंट हमने लिखी है स्कनर एसी इ इक्टू न्यू स्कनर system.in
हमने इंपोर्ट किया है java.jar क्लास अब इस क्लास के अंदर हमने पास कर दिया system.in तो जिस तरीके से
आउटपुट के लिए यूज करते थे सड आउट इनपुट के लिए यूज करते हैं system.in यानी हमारे कंसोल से हमारे टर्मिनल से हमारी इनपुट
विंडो से हमने इनपुट को ले लिया है अब इनपुट को लेने के लिए बाद हमें उसे कहीं पर स्टोर कराना
पड़ेगा मान लेते हैं हमें इनपुट लेना है किसी का नेम तो यहां पर डिफाइन करेंगे वेरिएबल स्ट्रिंग स्ट्रिंग में हमारे पास
आने वाला है नेम नेम को हम कुछ इस तरीके से लेंगे एडनेट यानी ये जो स्कैनर का हमने ऑब्जेक्ट
बनाया है स्कैनर क्लास का इसमें एक फंक्शन है नेक्स्ट इस नेक्स्ट फंक्शन की हेल्प से हम इनपुट को लेने वाले हैं ये इनपुट आएगा
और हम क्या करेंगे इसी इनपुट को हम प्रिंट करवा देंगे यानी नेम को हम प्रिंट करवा देंगे तो इसको रन करते हैं कोड को इससे
ऊपर ले आते हैं थोड़ा सा कोड को जब रन करेंगे तो यहां पर हमें अपना इनपुट पास करना पड़ेगा इनपुट पास करते हैं टोनी हमने
एंटर किया तो हमारे लिए क्या हुआ सबसे पहले उसने हमारा इनपुट लिया फिर उसी इनपुट को आउटपुट करके दिखा दिया और एग्जिट करके
कोड से तो स्कैनर क्लास की हमने ऑब्जेक्ट बनाई वहां से हमने ने इनपुट लिया एड नेक्स्ट करके फिर उसी इनपुट को हमने
प्रिंट करवा दिया अब एक खास चीज देखते हैं नेक्स्ट से यानी नेक्स्ट हमने इनपुट ले लिया यहां पर अगर हम दोबारा अपने नाम को
एंटर करवाना चाहे उसे करवाते हैं पर यहां पर पूरा नाम पास करते हैं पूरा नाम हमने पास किया लेकिन हमारे पास प्रिंट सिर्फ
फर्स्ट वर्ड हुआ ऐसा क्यों हुआ क्योंकि जब भी हम नेक्स्ट फंक्शन यूज करते हैं तो वो एक ही टोकन लेता है अब सेंटेंस की अगर बात
करें माय नेम इज टोनी स्टार्क तो इसमें बहुत सारे डिफरेंट वर्ड्स हैं इनमें हर एक सिंगल वर्ड एक सिंगल टोकन है अगर आप
नेक्स्ट फंक्शन को यूज कर रहे हैं तो एक सिंगल वर्ड यानी एक टोकन आएगा अगर आपको पूरी लाइन को इनपुट लेना है तो यहां पर
हमें यूज करना पड़ेगा फंक्शन नेक्स्ट लाइन जब हम नेक्स्ट लाइन लेकर रन करेंगे अपने कोड में तो क्या होगा यहां पर हम लिखते
हैं पूरा नाम और इस बार हमारे लिए पूरा नाम प्रिंट हो गया आउटपुट में तो यानी नेक्स्ट लाइन
को यूज़ करने से हमारे पास पूरी लाइन इनपुट हो गई अब उसी तरीके से जैसे नेक्स्ट और नेक्स्ट लाइन होता है वैसे ही स्कैनर
क्लास के और भी बहुत सारे फंक्शंस होते हैं इन फंक्शंस के अंदर आ जाता है हमारा नेक्स्ट इंट नेक्स्ट इंट यूज़ होता है
इंटी जर टाइप को इनपुट लेने के लिए उसके अलावा आ जाता है नेक्स्ट फ्लोट नेक्स्ट फ्लोट होता है फ्लोटिंग टाइप के इंटी
फ्लोटिंग टाइप के नंबर्स को लेने के लिए फ्लोटिंग टाइप यानी जिसमें आपका डेसीमल आ जाता है तो उस तरीके के नंबर अगर आपको
इनपुट लेने हैं तो उनको इनपुट करने के लिए लिए आपका नेक्स्ट स्लोट यूज होते हैं उसी तरीके से नेक्स्ट डबल नेक्स्ट लॉन्ग ऐसे
बहुत सारे आपके टाइप्स जो है वो आ जाते हैं जिनको आप इनपुट ले सकते हैं अपने कोड में अब इनपुट की हमने बात कर ली इस पर अब
एक क्वेश्चन कर लेते हैं और जितने भी कांसेप्ट हमने आज सीखे हैं उनको थोड़ा सा रिवाइज कर लेते हैं तो साथ में हमने मीम
भी डाल दिया है जो शायद काफी स्टूडेंट्स को रिलेटेबल लग रहा हो जावा पढ़ते हुए अब बात करते हैं क्वेश्चन की तो हमें लेने
हैं दो वेरिएबल यूजर से ए एंड बी और हमें प्रिंट करना है उनका तो कुछ इस तरीके का कोड होगा कि हम स्कैनर
क्लास बना रहे होंगे ए और बी को इनपुट ले रहे होंगे उसके बाद सम नाम का एक वेरिएबल बनाएंगे जिसमें a प् बी कर देंगे एंड
फाइनली प्रिंट करवा देंगे अपने सम की वैल्यू को तो हम देख पा रहे हैं कुछ चार पांच लाइनों का हम कोड लिखेंगे जिस कोड से
हम इस क्वेश्चन को सॉल्व करने वाले हैं तो कोड को अगर देखें तो सबसे पहले बनाएंगे अपनी स्कैनर ऑब्जेक्ट
स्कैनर ऑब्जेक्ट को हमने बनाया इसके बाद क्या करेंगे इनपुट ले लेंगे ए और बी तो इंट ए जो हो जाएगा वो हो जाएगा एडॉट
नेक्स्ट इंट हमने अभी डिस्कस किया था कि नेक्स्ट इंट फंक्शन इंटी जर टाइप की वैल्यूज को इनपुट लेने के लिए यूज होता है
उसके बाद इंट बी वो भी नेक्स्ट इंट से तो दो इंटी जर वैल्यूज को हमने इनपुट ले लिया अब क्रिएट करेंगे एक तीसरा वेरिएबल जिसका
नाम है सम तो इंट सम की वैल्यू हो जाएगी a प् b तो हमने a को इनपुट ले लिया स्कैनर ऑब्जेक्ट से b को इनपुट ले लिया स्कैनर
ऑब्जेक्ट से अब इंट सम नाम का एक वेरिएबल बनाया जिसमें a + b हमने कर दिया अब हमें आउटपुट करना है इसी सम को तो अपने आउटपुट
वाले कांसेप्ट को यूज़ करेंगे और प्रिंट कर देंगे अपने सम को अब आप देखेंगे कि काफी टाइम से हम जो वेरिएबल हैं उनके लिए
डबल कोड्स नहीं यूज़ कर रहे हम सीधा सीधा उन्हीं वेरिएबल को लिख देते हैं पहले भी जब हमने एक स्टेटमेंट लिखी थी कि नाम को
इनपुट लेके नाम को ही आउटपुट करा दिया था तो हमने वहां पर इस तरीके से डबल कोट्स नहीं डाले थे ऐसा क्यों हुआ एक बार इस कोड
को रन करके देखते हैं कि डबल कोट्स डालेंगे तो क्या होगा अगर हम इस कोड के अंदर डबल कोट्स
डालेंगे तो मान लेते हैं a हमने एंटर किया 10 b एंटर किया फाइव हमारे लिए प्रिंट क्या हुआ सम प्रिंट हो गया सम प्रिंट होने
का मतलब है कि डबल कोट्स के अंदर जो चीज़ लिख दोगे वो वैसी की वैसी प्रिंट हो जाएगी पर हमें ये वाला सम प्रिंट नहीं करवाना
हमें दोनों वैल्यूज का सम प्रिंट करवाना है तो उसके लिए हमें डबल कोट्स यूज़ नहीं करने सीधा का सीधा अपने वेरिएबल को इन
पैरेंस के अंदर लिख देना है तो वहां पर सम नहीं प्रिंट हो रहा होगा सम के अंदर स्टोर्ड वैल्यू प्रिंट हो रही होगी मेमोरी
की इसको दोबारा रन करते हैं दोबारा हम अपना इनपुट पास करते हैं व्हिच इज 10 एंड फ और अब हमारे लिए प्रिंट
हुआ है 15 तो 10 एंड 5 का सम हमारे लिए प्रिंट हो गया हमने यहां कांसेप्ट यूज़ कर लिया इनपुट का वेरिएबल का डेटा टाइप का
उसके साथ-साथ हमारे आउटपुट का तो आज हमने चार जो बेसिक फंडामेंटल्स ऑफ प्रोग्रामिंग होते हैं फंडामेंटल्स ऑफ जावा होते हैं
उन्हें डिस्कस कर लिया है इसके बाद और भी चीजें डिस्कस करेंगे तो एक बार हम जावा को कंप्लीट कवर कर लेंगे उसके बाद स्टार्ट
करेंगे डेटा स्ट्रक्चर्स एंड एल्गोरिथम्स तो आई होप कि आज की जो क्लास है उसमें चीजें क्लियर हुई होंगी नीचे जो नोट्स है
उसके अंदर और भी एडिशनल कुछ कुछ कांसेप्ट हमने आपको दिए होंगे तो उन नोट्स को आप जाकर रेफर कर सकते हैं उन नोट्स में जो
मेंशन क्वेश्च उन्हे हमें प्रैक्टिस करना है नेक्स्ट क्लास से पहले मिलते हैं नेक्स्ट वीडियो
में टिल देन कीप लर्निंग एंड कीप एक्सप्लोरिंग
Heads up!
This summary and transcript were automatically generated using AI with the Free YouTube Transcript Summary Tool by LunaNotes.
Generate a summary for freeRelated Summaries
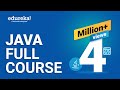
Java Programming: A Comprehensive Guide to Understanding Java and Its Concepts
Explore Java programming concepts including OOP, exception handling, and collections. Learn how to build robust applications!
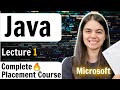
Java Course Introduction: Mastering Coding Fundamentals and Data Structures
Kickstart your Java programming journey with our guided course covering basics to algorithms for aspiring developers.
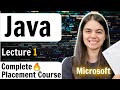
Java Programming Course: Introduction, Structure, and Setup Guide
Learn about Java programming fundamentals, data structures, and how to set up your coding environment.
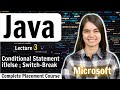
Mastering Java: Understanding Conditional Statements for Beginners
Dive into Java's conditional statements, including 'if', 'switch', and 'break', to enhance your programming skills.
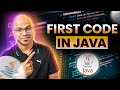
Getting Started with Java: Writing Your First Code in VS Code
In this tutorial, we explore how to set up Visual Studio Code for Java development and write your first Java program. We cover the basics of creating a project, using the terminal, and writing a simple 'Hello World' program.
Most Viewed Summaries
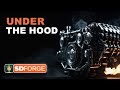
A Comprehensive Guide to Using Stable Diffusion Forge UI
Explore the Stable Diffusion Forge UI, customizable settings, models, and more to enhance your image generation experience.
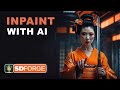
Mastering Inpainting with Stable Diffusion: Fix Mistakes and Enhance Your Images
Learn to fix mistakes and enhance images with Stable Diffusion's inpainting features effectively.
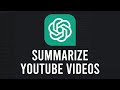
How to Use ChatGPT to Summarize YouTube Videos Efficiently
Learn how to summarize YouTube videos with ChatGPT in just a few simple steps.
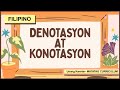
Pag-unawa sa Denotasyon at Konotasyon sa Filipino 4
Alamin ang kahulugan ng denotasyon at konotasyon sa Filipino 4 kasama ang mga halimbawa at pagsasanay.
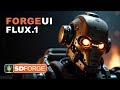
Ultimate Guide to Installing Forge UI and Flowing with Flux Models
Learn how to install Forge UI and explore various Flux models efficiently in this detailed guide.