Introduction
Welcome to the course on advanced placement, where we dive deep into programming concepts with a positive attitude and a grateful mindset. In this lecture, we focus on Conditional Statements, which are essential for making decisions in code. Today, we will cover the if
, switch
, and break
statements in Java. Understanding these concepts will help you manipulate and control the flow of your programs effectively.
What are Conditional Statements?
Conditional statements allow our programs to make decisions based on certain conditions. They are crucial for executing different blocks of code depending on whether a condition is true or false.
The if
Statement
The if
statement is the fundamental conditional statement.
- Syntax:
if (condition) { // code to be executed if condition is true }
- Example:
int age = 20; if (age >= 18) { System.out.println("Adult"); } else { System.out.println("Not Adult"); }
In this example, the program checks if the age is 18 or greater. If true, it prints "Adult"; otherwise, it prints "Not Adult".
The else if
Statement
Sometimes, you may need to check multiple conditions. For these scenarios, you can use else if
to chain conditions together.
- Syntax:
if (condition1) { // code for condition1 } else if (condition2) { // code for condition2 } else { // code if none of the conditions are true }
Using the switch
Statement
When you have a variable that can take on multiple values, the switch
statement can be a cleaner alternative to multiple if
statements.
- Syntax:
switch (expression) { case value1: // code for value1 break; case value2: // code for value2 break; default: // code if none of the cases are matched }
- Example:
int button = 2; switch (button) { case 1: System.out.println("Hello"); break; case 2: System.out.println("Namaste"); break; case 3: System.out.println("Bonjour"); break; default: System.out.println("Invalid button"); }
In this example, depending on the value of button
, a different greeting is printed. The break
statement prevents the execution of the subsequent cases once a match is found.
Understanding the break
Statement
The break
statement serves two main purposes:
- To terminate a loop prematurely.
- To exit a switch statement.
Example of Using break
in Loops
for (int i = 0; i < 10; i++) {
if (i == 5) {
break;
}
System.out.println(i);
}
This loop prints numbers 0 to 4 and stops when i
is equal to 5 due to the break
statement.
Practical Implementation
Input and Output in Java
We will now see how to take user input and implement conditional statements based on that input using the Scanner class.
- Import Scanner Class:
import java.util.Scanner;
- Create a Scanner object:
Scanner scanner = new Scanner(System.in);
- Take user input:
System.out.print("Enter your age: ");
int age = scanner.nextInt();
- Use conditional statements to make decisions:
if (age >= 18) {
System.out.println("Adult");
} else {
System.out.println("Not Adult");
}
Practicing with Examples
Let's practice with a small exercise. Create a calculator program that takes two numbers and an operator as input and prints the result. Here’s a quick layout of what to implement:
- Take two integers as input from the user.
- Take an operator (+, -, *, /) as input from the user.
- Use conditional statements to perform the operation based on the operator and print the result.
Example Code of Simple Calculator
import java.util.Scanner;
public class Calculator {
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
System.out.print("Enter first number: ");
int num1 = scanner.nextInt();
System.out.print("Enter second number: ");
int num2 = scanner.nextInt();
System.out.print("Enter operator (+, -, *, /): ");
char operator = scanner.next().charAt(0);
switch (operator) {
case '+':
System.out.println("Result: " + (num1 + num2));
break;
case '-':
System.out.println("Result: " + (num1 - num2));
break;
case '*':
System.out.println("Result: " + (num1 * num2));
break;
case '/':
System.out.println("Result: " + (num1 / num2));
break;
default:
System.out.println("Invalid operator");
break;
}
}
}
Conclusion
In this lecture, we have discussed the fundamental concepts of conditional statements in Java, including if
, else if
, else
, switch
, and break
. Conditional statements are crucial for controlling the flow of your programs based on dynamic user input or other conditions. We encourage you to practice implementing these concepts through exercises such as the calculator example. Keep learning and practicing to solidify your understanding and proficiency in Java programming!
अब आधे हाय एव्रीवन वेलकम टो थे ज्यादा प्लेसमेंट कोर्स इसमें सीखेंगे हम लेक्चर थ्री यानि कंडीशनर स्टेटमेंट्स क्लास की
शुरुआत करते हैं पॉजिटिव एटीट्यूड के साथ लेटेस्ट प्लांट डे विद ग्रिटीट्यूड एंड थैंकफूलनेस ना इसके सी पानी ऐड डेफिनेटली
ऐड नहीं स्किल जितना सीखेंगे चीज़ें सीखते रहेंगे उतना ही हम लाइफ में आगे बढ़ रहे होंगे तो शुरुआत करते हैं लेक्चर थ्री की
जिसमें सीखेंगे कंडीशनर स्टेटमेंट आज जो टॉपिक हम कवर कर रहे होंगे उसके अंदर आएंगे
f1s स्विच और ब्रेक अबे कुछ समझ सकते हैं जावा के जिनके बारे में सीखेंगे तीव्र क्या होते हैं अब जैसे इंग्लिश के अंदर
हमारे पास सिक्स डिक्शनरी होती है जिसके अंदर जितनी भी वोट लिखे होते हैं वह सारे के सारे इंग्लिश के वैलिड वर्ड्स होते हैं
बाकियों को इंग्लिश कपाट नहीं माना जाता वैसे ही ज्यादा की भी अपनी एक डिक्शनरी है जिसके अंदर कुछ बोर्ड सपोर्ट है और उन्हें
वर्ष के वृक्ष मतलब है उन वर्ष के कोई और मतलब हम नहीं ले सकते ना ही उनके नाम के हम देरी बना सकते हैं ऐसे इफेक्ट बोर्ड को
जाऊंगा कि अगर कीवर्ड्स कहते हैं तो यह सारे के सारे जावा के कुछ कि वह इस ऐज अब इससे पहले हमने इनपुट आउटपुट ठीक है
ज्यादा में वेरिएबल सीखेंगे क्या होते हैं और डाटा टाइप सी किए हैं इन सारी चीजों का यूज हम इस क्लास के अंदर कर रहे होंगे तो
आपको अच्छे दिन है इस क्लास में तब ज्यादा समझ में आ रही हूं जो आपने पहले वाली क्लास है देखिए हो विच बेसिक जब अब शुरुआत
करें इस पर स्टेटमेंट ऑफ अकाउंट स्टेटमेंट के तो क्या होती है कंडीशनर स्टेटमेंट्स जैसे अगर हमारा इंग्लिश का कोई लॉजिकल
स्टेटमेंट हो कि आपकी जो ऐज है अगर वह 18 से ऊपर है तब आप एक डाइट है नहीं तो आप एक एडल्ट नहीं है हमने हिंदी में एक सरल सी
बात कह दी अब इसी को इंग्लिश में ट्रांसलेट करें तो एक यूअर शोल्डर दिन 8:00 एग्जैगरेटेड एंड यूअर रिजल्ट इन
यूनाइटेड अल्ट कि हम निकलेंगे इसको इंग्लिश में यानी इस और इसको यूज करके अब इन्हीं कंडीशनर स्टेटमेंट को हम जावा के
अंदर इस ऐज दो कि वर्ष की हेल्प से लिखते हैं इंटरनेशनल स्टेटमेंट्स एंड यहां पर इस
कंडीशन आगे कंडीशन किस प्राइजेज पर अगर एज 18 से ऊपर है यह कंडीशन है अगर यह टू है तो आप पेड सिम नहीं तो आप एडिट नहीं है तो
इन्हीं कंडीशनर स्टेटमेंट को हम जावा के अंदर भी लिखते हैं अगर इसको लिखने का सेंटेंस देखें तो कुछ इस तरीके से
रिप्रेजेंट करते हैं जैसे जानवर के अंदर नॉर्मल कोड के अंदर कुछ सुच स्टेटमेंट्स लिखेंगे और बीच में आ जाएगा टपक पड़ेगा
हमारा एग्स उसको ऐसे लिखेंगे कि पेरेंट्स इसके अंदर कोई भी एक कंडीशन यहां पर कंडीशन हो सकती है सम नंबर एंड ग्रेटर
2018 या फिर हमारी ए ग्रेट कर देंगे 30 या फिर अगर हमारा एबल टू बी है अब जावा के अंदर सिर्फ एक उल्टा एक बार नहीं लिखते दो
बार लिखते हैं तो एग्जीक्यूटिव इज इक्वल टू अगर बी है तो वह भी एक कंडीशन हो सकती है अगर हमारा कोई बुलियन अवेलेबल है मान
लीजिए इंसिडेंट पहले से हमें पता है क्या उसकी वैल्यू ट्रू है वह वेकेशन हो सकती है तरीके से इसके अंदर कोई भी कंडीशन अगर
ट्रू हो जाए तो उसके बाद जो कर ली ड्रेस है उसके अंदर जितनी भी स्टेटमेंट है वह सारी लाइन करेंगे लेकिन अगर वह कंडीशन
स्पॉइल्स हो जाए तो उस केस में रन करेंगे इस वाले कर ली ड्रेसेस कि स्टेटमेंट स्टेटमेंट 5th एंड 6th कुछ इस तरीके से इस
काम करता है जहां केंद्र हम इसको एक सिंपल एग्जांपल समझने की कोशिश करते हैं सबसे पहले हम क्या करेंगे यूजर से इनपुट ले
लेंगे तेज हमने सीखा तलाश तलाश में इनपुट कैसे लेते हैं ज्यादा के अंदर पहला सेट अप होगा यह
दूसरा सैफ चेक करें कि अगर हमारी ए ग्रेटर 19th है तो हम प्रिंट करवा देते डिफिसिट नहीं तो मतलब बैलेंस कम प्रिंट
करवा दे नोट एडल्ट बहुत ही सिंपल काम अभी सिंपल काम को कोड की हेल्प से करते हैं सबसे पहले क्या करेंगे स्कैन अ क्लास की
ऑब्जेक्ट बनाएंगे स्कैनर क्लास का यूज करते हैं जहां हम इनपुट लेने के लिए इसके अलावा प्रॉपर लीडर करके भी होता है उसको
हम थोड़ी एडवांस क्लासेस में पढेंगे उससे टाइम जो है कम हो जाता है मतलब कोड आपका फास्ट हो जाता है पर अभी हम basic तेल में
तो धीरे-धीरे काम करेंगे तो ज्यादा चीज समझ में आयेंगे अंदर लिखेंगे सिस्टम डॉट इन
अब यह स्कैनर जो है इसको यूज करने के लिए हमें इंपोर्ट करना पड़ेगा इकबाल कुछ जावा डॉट डॉट डॉट
सब कुछ लेते हैं वैसे डौ टायर होता इनपुट आउटपुट के लिए स्कैनर क्लास का हमने ऑब्जेक्ट बना लिया
उसके बाद क्या किया ऐज को हम कर लेंगे इनपुट इनटेजेसिक विंडो एसी डॉट एक्सटेंट एक हमने कलियां इनपुट और लिखेंगे अपना इस
ऐज तो ए ग्रेट 18th यह हम यहां जो लिखी है यह कंडीशन है
प्रिंट करवा देंगे हम कि अल्ट अब आप सोच रहे होंगे कि मैंने बस एस्पायर टो टाइप किया और फिर यह पूरी स्टेटमेंट कैसे आगे
तो विजुअल स्टूडियो कोड के अंदर शॉर्टकट होता है कि आप ऐसा एप्स सोर्सेस टो टाइप करें और फिर कीबोर्ड पर टांग दबाने तो
आपके लिए पूरी स्टेटमेंट है वह प्रिंट को जाएगी है तो यहां पर इसके बाद लिखेंगे एंड
स्पेसिफिकली ब्रिग्स एंड यहां पर प्रिंट करवा देंगे हम नोट एडल्ट तो कुछ इस तरीके से हमारे 1 इंच की हेल्प से हम प्रिंट
करवा रहे होंगे कि अगर यूजर नेम इज देवी तो अडल्ट है या नहीं है इस ए ग्रेट धुंध प्रिंट वैल्यू सिस्टम डॉट प्रिंट एंड नॉट
रिजल्ट तो इस पूरे के पूरे कोड को सेव कर लेते हैं कंट्रोल इस दबाकर या ऊपर फाइल में जाकर और अभिषेक करेंगे रन रन करने के
बाद इसे डीबग नहीं करना तो हम इन फूड देंगे अपनी एप्स क्या लिखना चाहते हैं सबसे पहले मिट्टी ने एंटर कर देते हैं तो
₹15 इसलिए नोटिफिकेशन से इसको रन करें अगर और इस बारे में क्या बात करेंगे एक में बात कर
रहे होंगे हम 2121 पास किया तो एडल्ट तो कुछ इस तरीके से एक इस काम करता है जहां केंद्र अब एक
और एग्जाम पर लेते हैं अगले वाले एग्जांपल जो होगा हम लेवल आफ अच्छी तरह अब जो एग्जांपल होगा उसमें क्या करेंगे हम चेक
करेंगे यूजर से इनपुट लेंगे एक नंबर है अच्छा ठीक है अब एक नंबर के लिए चेक करेंगे कि क्या यह नंबर और है
या फिर यह नंबर इवन है हल्दी और डोरेमोन कैसे चेक करते हैं जो आपके अंदर यह तो हमने सीखा ही नहीं तो और जीवन कैसे चेक
करते हैं हमारे पास जावा के अंदर एक ऑपरेटर होता है जिसको नाम देते हैं मोलू मोलू ऑपरेटर क्या करता है यह कैलकुलेट
करता है रिमाइंडर जैसे अगर क्लिक करना है तो प्लांट ऑपरेटर होता है - करना है तो - ओपरेटरों है
मल्टिप्लाई करना तो मार्किट आपरेटर होता है देखते ही जावा के अंदर यह मॉडल ऑपरेटर होता है अगर एक्स मॉडल ओं व्हाय हम ले लें
तो एक्स डिवाइडेड बाय बाय का जो भी रिमाइंडर आएगा रिमाइंडर वह हमें आउटपुट में मिल रहा होगा
तो जब भी एक डिवाइडेड बाय बाय का रिमाइंडर चाहिए तो आप एक मॉडल उपाय लिख दीजिए ज्यादा के अंदर प्लेन मोड लेने से ऑड-इवन
का क्या लेना-देना तो यहां पर हम करेंगे लॉजिक की बात लॉजिक की बात यह है कि हमने मैथ्स में सीखा हुआ है कि किसी इस नंबर को
दूसरे डिवाइड कर दें और वह कंप्लीट लीड हो जाए और रिमाइंडर जीरो आ जाए तो इस नंबर को हम क्या कहते हैं उस नंबर को कहते हैं यह
बन मतलब एक्स डिवाइडेड बाय टू अगर कहीं भी हमारा जो रिमाइंडर है वह गुरुदेव तो मतलब वही वन नंबर है लेकिन एक डिवाइडेड बाय टू
अगर जीरो नहीं दे तो वह नंबर दिया है वह नंबर है और अभी एंड डिवाइड है यह तो हमें क्वेश्चन देता है ज्यादा के अंदर लेकिन
मॉड्यूलों की हेल्प से हम रिमाइंडर निकाल सकते हैं तो हमें क्या करना है सिंपली लिए हमें सिंपली यह करना है कि अगर हमें एक
नंबर दिया हुआ है जो हम गुर्जर से इनपुट लेंगे मान लेते हो नंबर है एक्स तो एक काम ऑडियो मी टू चेक कर लेंगे अगर वह जीरो हो
जाता है तो मतलब वही वन नंबर है लेकिन अगर गुर्जरों नहीं होता मतलब ऐसी कंडीशन तो वह एक और नंबर है इसी सेम लॉजिक को अब कोर्ट
की हेल्प से लिखते हैं कोर्ट में क्या करेंगे पहले हमने इनपुट लिया था वेज इस बार इनपुट लेंगे हमारा नंबर टू है
कि एक सा गया हमारे पास अगर एक SIM और डिलाइट टू इज इक्वल टू इज इक्वल टू जीरो पुलिस केस में प्रिंट कर दीजिए आप चीन
यानी एक कंपनी के साथ प्रिंट करेंगे और कुछ इस तरीके से और इसके बाद हमने देख
लिया कि हमने जो नंबर को सेव करते हैं इसको करते हैं करने के बाद हमारे पास नंबर हमें देना है तो सबसे
पहले नंबर बिल्कुल सही नंबर इंटर करके देखेंगे
इस बार एंटर करते हैं नंबर है बिल्कुल ठीक है इस तरीके से इसको यूज करके कि हमारी स्टेटमेंट लाइफ में
कैसे प्रेगनेंट होती है और करके देखेंगे इस क्वेश्चन से थोड़े से नए टिप्स ठीक रही होंगे सबसे पहले इस
क्वेश्चन को आसान तरीके से करेंगे तो हमें जो क्वेश्चन गिवर है वह यह है कि आप क्या करिए यूजर से इस बार दो नंबर इनपुट नीचे
घी भी अगर क्वेश्चन है तो उस केस में प्रिंट करवाइए इक्वल टू कि अगर एबी से बढ़ा है उस केस में प्रिंट
करवाइए इस क्रेटर अगर एबी से छोटा है तो उस केस में प्रिंट करवाइए इस लेसन तो इस तरीके से वांट टू
थ्री कंडीशन हैं आपके पास इनको आपको इसकी फॉर्म में करना है उसको करके देखते हैं कोड कि हमें कैसे करेंगे सबसे पहले तो इसे
मिटा लेते हैं पहले हम क्या इनपुट ले रहे थे एक बार लेंगे एक सिमिलरली इन फूटे लेने के बाद इनपुट मिलेंगे
डिफेंस टिंट करके अब या करेंगे अब चेक करेंगे इस एग बिल्कुल टू बी उस केस में आप प्रिंट करवा दीजिए
इक्वल लेकिन अगर ऐसा नहीं है तो उस केस में क्या होगा उसके फिर दोबारा चेक कर लेते हैं कि एबी से बढ़ा है तो उसके इसमें
क्या प्रिंट करवा रहे होंगे एस ग्रेट कर लेकिन अगर ऐसा नहीं है मतलब ए भी से छोटा है तो उस केस में प्रिंट करवाएंगे
क्विज fluid है है तो यह हमने कोड लिखे है अगर एबी के कुल है तो प्रिंस करवाइए इक्वल मेल्स दोबारा
से चैक कर लेंगे अगर यह भी से बढ़ा है रिप्रिंट करवाइए ए ग्रेटर वैल्यू मतलब इसका सिर्फ मगर एबी से बढ़ा रही है तो
प्रिंट करवाइए इस लिस्ट अब इस कॉर्ड को रावण करते हैं रन करके हम अपना यह जो है वह पास करेंगे टू बी के पास करेंगे फाइल
तो एप्स लूथर वेरी क्लियर तो इससे में क्या समझ में आया कि अगर हमे सिर्फ दिया हो तो ऐज के बाद भी बहुत सारी ऐसी कंडीशन
होती हैं जो हम चेक करना चाहते हैं मतलब इस तो हमने चेक कर लिया ही रिक्वेस्टिड चेक कर लिया उसके बाद भी हमें चेक करने
ग्रेटर है लेस है क्या है तो ऐसे केस में हुए देश के अंदर सारी कंडीशन सूट नहीं पड़ेगी तो थोड़ा सा जो कोड है वह भी
क्लियर नहीं दिख रहा तो जावा में इसका सलूशन निकला जावा में इसका सलूशन जो निकला उसको हम कह वॉइस मेल सिर्फ स्टेटमेंट्स
एंड सैफ यानि अपने इष्ट चेक कर लिया कि अगर वह नहीं है तो फिर आप दोबारा से ऐड कर सकते हैं इसके अंदर आपको सब कुछ नहीं
लिखना किस तरीके से इंटैक्ट तरह का या आपने इस कंडीशन में चेक कर लिया कि स्टेटमेंट स्टेटमेंट टू फ्रंट हो जाएं अगर
कंडीशन वन सर्च है लेकिन अगर ऐसा नहीं है तो फिर आप चैक कर लें कंडीशन 2 इयर्स सिर्फ लिखकर तो इन कंडीशन फ्री और फॉर एनी
स्टेटमेंट रिलीफ टो एग्जीक्यूट हो रही होंगी उसके बाद आप कितने भी ऐसे लिख सकते हैं और फिर एंड में आप लिखे अपना फाइनल्स
अब आप इसमें बोल सकते हैं यदि आप बार-बार इस भी तो लिख सकते थे इसमें लिखते थे कंडीशन वन दोबारा ही लिख देते हैं कंडीशन
थ्रू और फिर से इस तरीके से हम अपनी दो कंडीशन चेक कर लेते लेकिन बार-बार आप इस लिखते हैं तो पहले वाली भी चेक होगी दूसरी
वाली भी चेक होगी लेकिन ऐड जब लिखते हैं तो पहले वाली अगर शुरू हो गई तो यह चेक नहीं होगी लेकिन अगर पहले वाली ट्रू नहीं
हुई तो यह होगी अगर पहले वाली भी शुरू नहीं हुई यह बैठक नहीं हुई तबीयत होगी तो कुछ इस तरीके से हमारा एब्स काम करता है
युवा केंद्र अमित सेम कॉर्ड को अगर में एसिड की फॉर्म में चेंज करना है तो कैसे करेंगे इस तो हमने वैसा का वैसा रखना है
यहां पर हम बस इसे बना देंगे तो मेरा टैब यहां पर यह वाला प्रिंसेस हम हटा देंगे अब
हैं तो ध्यान से देखें तो क्या हुआ एस ए रिकॉर्ड भी ढूंढ उस कंडीशन एग अगर ऐसा नहीं है तो फिर आप दोबारा चेक करेंगे
ग्रेट आदमी ने इस ग्रेट हर एग्जाम दो बार चेक करेंगे इस तो इसे थोड़ा सा क्लीन कर देते हैं
और कर देते हैं अब ज्यादा के अंदर एक चीज होती है कि जो आपके करीयर ए ए होते हैं वह आप सेम लाइन पर लगते हैं ओपनिंग कर ली
प्रिंस और क्लोजिंग वाले जो बढ़िया होता है वह नेक्स्ट लाइन में आता है तो अगर आप इसको यहां भी कर देंगे तो भी कोर्ट में
कोई प्रॉब्लम नहीं आएगी बट ए कन्वेंशन है ज्यादा को लिखने का कि आप सेम लाइन पर करते हैं तो यह बेसिकली दिखाता है कि आप
एक अच्छे को डर है कोडिंग अच्छे-खासे टाइम से कर रहे हैं तो आपको पता है कि कौन सी लैंग्वेज है कौन से रूठ जाते हैं तो कुछ
इस तरीके से हमने ऐड का यूज कर लिया अब इस कॉर्ड को भी एक बार रन करके वेरीफाई जरुर कर लेते हैं इस बार पास करेंगे इनमें पांच
और भी में दो तो ए ग्रेटर तो कोण हमारा बिलकुल है सही अब थोड़े से कुछ क्वेश्चंस करते हैं थोड़ा सा प्रैक्टिस करते हैं जो
भी हमने सीखा उसको सब मिलाकर कुछ हमारे जो क्वेश्चन गिवर है 13 इस नोट नंबर प्रिंट बजरंगा अब यहां पर
ऑडिट की जगह बनाना चाहिए थोड़ा सा प्रिंटिंग मिस्टेक है इस थ्री इन वन नंबर प्रिंट ब्रिंगा और
यहां पर आएगा मल्टीपल चॉइस आफ करेक्ट मतलब एक से ज्यादा जो आपके ऑप्शन है वह भी सही हो सकते हैं अब सबसे पहले हम लाइक करें
सृजन ही वन नंबर प्रिंट बजेगा तो पहले ऑप्शन तो हम सबसे पहले अनलाइज़ करके देखते हैं कि पहला ऑप्शन क्या कहते है पहला
ऑप्शन क्या वही है जो क्वेश्चन में दिया हुआ है पहले ऑप्शन है इस फ्री मोड लो टू मतलब जो भी नंबर थ्री लौटे लो टू हम चेक
करेंगे कि अगर वह इवन है तो आप प्रिंट कर दीजिए बजरंगा बिल्कुल ठीक है तो ऑप्शन ए एस करेक्ट के स्टेटमेंट सेंट पिछली
सेंटेंस वाइज बिल्कुल ठीक है अब आते हैं ऑप्शन बी क्लियर 302 विडियो कुंवा गई1 है तो प्रिंट कर
दीजिए वजन का या अब इसमें तो कर ली पर एसएसआई नहीं यह कैसे सही हुआ अब बात यह है कि जावा के अंदर इसके पास कर ली प्रिंस
लगाना तब ज्यादा लॉजिकल रहता है जब आपको एक ही स्टेटमेंट प्रिंट करवानी हो पनीर इसमें तो कर ली प्रसिद्ध है यह कैसे ठीक
हुआ था तो जावा के अंदर कर ली प्रिंस इसके अंदर आपकी मल्टीपल स्टेटमेंट आ सकते हैं तो वह सारी की सारी प्रिंट होंगे अगर आप
एक ट्रू है लेकिन अगर आप की सिंगल स्टेटमेंट है और वह बिना डिलीवरी के लिखेंगे तो भी वह प्रिंट हो रही होगी तो
इस आपने कहीं भी लिख दिया और अगर वह ट्रू है तो उसके अगले वाली जो स्टेटमेंट है वह इसके साथ अपने आप जुड़ जाती है तो कुछ इस
तरीके से बिना कर ली प्रिंस इसके बावजूद आप एक सिंगर स्टेटमेंट को प्रिंट करवा सकते हैं लेकिन इसके अलावा बजिंगा के
अलावा अगर आपको कुछ और प्रिंट करना होता है अगली लाइन में अगर यह स्टेटमेंट सच हो जाती तो तो उसके लिए आपको कर ली प्रिंस जो
है वह लगाने पड़ते क्योंकि अगर आपने कैलीबरेशन नहीं लगाए हो कोट स्टेटमेंट एग्जीक्यूट होती इसके बाद तो यह जो हमारे
ऑप्शन भी है यह भी एक करेक्ट ऑप्शन अब्जॉर्प्शन सी की अगर बात करें तो इसमें एक फ्री मोड ऑन युटुब ओं सेम कंडीशन की
ठीक है फ्री को चेक करेंगे कि वह इवन है क्या लेकिन यहां पर यह एक स्पेशल टर्मिनेटर आपने देख लिया टर्मिनेटर यानी
अब आप यहां पर खत्म कर दीजिए इस लाइन को इस तरह मिनिस्टर की वजह से यह जो नेक्स्ट लाइन है
नेक्स्ट लाइन विल ऑलवेज बीइंग एजुकेटेड मतलब अगर इस ट्रू है नहीं है फर्क नहीं
पड़ता नैक लाइन में जो है प्रिंट हमेशा हो रहा होगा इसीलिए यह जो स्टेटमेंट है यह इन करेक्ट मतलब
क्वेश्चन में लिखा था इसीलिए हमारे जो ऑप्शंस इसमें से भी सही ऑप्शन गलत है क्योंकि
अगर यह नहीं होता तो यह बिल्कुल सही होता है झाल बात करते एक और क्वेश्चन कि इस क्वेश्चन को सॉल्व करते करते हम सीख
जाएंगे कि स्विच का क्या मतलब होता है जावा के अंदर हम क्वेश्चन कुछ इस तरीके से कि आप क्यों एक रोबोट दिया हुआ है उसके
पांव ऊपर तीन बटन है पहले बटन को प्रेस करते हैं तो वह कहता है हेलो दूसरे बटन को प्रेस करते हैं तो कहता है नमस्ते और
तीसरे बटन को प्रेस करते हैं तो वह कहता है वह अंजू अब ओन जूस फ्रेंच का नमस्ते हैं मुझे आइडिया नहीं है कि मैं इसको सही
प्रणाम्स कर रही हूं या नहीं पर अमरेली सॉरी अगर मैं गलत प्रिंस कर रही हूं तो तो कुछ इस तरीके से वांट टू थ्री बटन को
प्रेस करने के बाद आप के अलग-अलग जो ग्रीटिंग्स है वह प्रिंट को रहे होंगे इस चीज को सबसे पहले करते हैं इस ऐज की हेल्प
से उसके बाद देखेंगे कि स्विच की कहानी कैसे आ जाती है इसके बाद तो हमें पता है कि हमें क्या करना है सबसे पहले हम इनपुट
लेंगे अपना बटन तो इन बटन को इनपुट ले लिया कि एसी डॉट इन की हेल्प से उसके बाद क्या किया अगर हमारे बटन पर वन है उस केस
में आप प्रिंट कर दीजिए हेलो लेकिन अगर बटन पर टू है तो उस केस में
प्रिंट कर दीजिए को नमस्ते लेकिन अगर बटन पर
आपके पास फ्री है तो उस केस में हम प्रिंट कर देंगे वन जूस और अगर इसके अलावा अपने कोई बटन
प्रेस की है मतलब आप वह बटन प्रेस मत करिए वही इनवेलिड बटन है तो यहां पर लिख देंगे इन्वाइलेट बटन
को शेयर कर देते हैं तो लॉजिक क्या बना बटन को इनपुट लिया बटन अग्रवाल है तो प्रिंट करिए हेलो बटन अगर टू है तो प्रिंट
करिए नमस्ते बटन अगर फ्री है तो प्रिंटर ई वांट यू एंड गलत बटन आपने प्रेस किया तो इनवेलिड बटन आपके लिए प्रिंट हो जाएगा इस
पूरे के पूरे कोड को रन करते हैं रन करके सबसे पहले बटन हम प्रेस करना चाहते हैं 321 चूर प्रिंट हुआ अब दोबारा से अगर बटन
प्रेस करना चाहिए एक और तो इस बार प्रेस कर रहे होंगे बटन नंबर टू तो हमारे उन नमस्ते प्रिंट हुआ है है तो अब हमारा जो
कोड है इसमें हम नॉएडा इस बार लिखा है और यहां पर तो सिर्फ तीन बटन थे अगर बींस बटन होते रोबोट के ऊपर प्रथम बार बार बटन इज
इक्वल टू बटन इज इक्वल टू करते-करते परेशान हो जाते तो यहां पर जावा के अंदर एक प्यारा सा कौन से पाया है जिसको हम
स्विच का नाम देते हैं स्विच क्या होता है जैसे हमारे घर पर लाइट बोर्ड के ऊपर स्विच होते हैं जिसको आप बटन दबाकर किसी से पंखा
चल रहा होता है किसी से ऐसी चल रहा होता है किसी से हमारी लाइट जली होती है वैसे ही ज्यादा के अंदर स्वच्छ होता है इसको आप
एक मशीन की तरह समझ सकते हैं जिसके अंदर आपने इनपुट दे दिया कि आपने कौन सा बटन प्रेस करना है उस इनपुट के हिसाब से वह
कैसे लगेगा कि कौन सा टेस्ट मैच कर रहा है इस बटन प्रेस के साथ और जो भी के स्मार्ट करेगा उसके आगे वह आपको कुछ काम कर कर दे
रहा होगा अब स्विच कि अगर सिंटेक्स की बात करें तो वह कुछ ऐसा दिखेगा आपको तो स्विच के सेंटेंस में सबसे पहले हम लिखते हैं
स्वच्छ स्विच लिखने के बाद पर लगाते हैं पेरेंट्स पेरेंट्स के अंदर कोई भी वेजिटेबल रायता वेजिटेबल यानि यह वह बटन
है जिसको आपने प्रेस किया अब इस मटन की वैल्यू कोई नंबर हो सकता है जैसे 12345 से या फिर कोई कैरेक्टर भी हो सकता है आप भी
सी यहां पर हमने नंबर का के लिए अब यह जो बटन है यह भी के साथ वाली चीज़ होगी अगर आपने किया था तो वह
मैच के साथ बटन तो के साथ लेकिन बटन पर क्लिक करके सब्सक्राइब भी हो सकते थे लेकिन यहां पर
है नंबर वन प्रेस स्टेटमेंट एग्जीक्यूट हो जाएगी और उसके बाद यह ब्रेक ब्रेक ब्रेक
भी अब जैसे ही पर आ जाएंगे आप इस बीच से बाहर निकल जाएंगे तो जैसे ही स्टेटमेंट करेंगे
जो कर ली है अधम बाहर निकल जाएंगे लेकिन अगर यह ब्रेक यहां पर नहीं होता तो क्या होता नीचे की सारी स्टेटमेंट एग्जीक्यूट
होती चली जाती थी जब तक हमारे पास ब्रेक नहीं आ जाता इसीलिए हर केस में स्टेटमेंट के बाद एक ब्रेक होता है ताकि आप उस
स्टेटमेंट टो एग्जीक्यूट कर लें और वहां पर ब्रेक लगा दें और अब सिर्फ बाहर आ जाए इसलिए के स्टुअर्ट ब्रॉड तो यह स्टेटमेंट
एग्जीक्यूट और फिर एक के थ्रू अगर हुआ तो यह स्टेटमेंट एग्जीक्यूट और यह ब्रेक और एक स्पेशल केस होता है जो होता है डिपॉजिट
के अगर वो ट्रू हुआ तो यह स्टेटमेंट एग्जीक्यूट और आखिर में तो हमें ब्रेक की जरूरत ही नहीं है तो यह पता है कि
स्टेटमेंट जो है कर ली प्रिंस अपने आप खत्म हो गया तो अपने आप ब्रेक लग जाएगा तो कुछ इस तरीके से स्वच्छ होता है उसके अंदर
आप इनपुट पास करते हैं अपना बटन प्रेस करते हैं उस पर वह अपने दिमाग में कैसे जगाता है और कुछ फ्रेंड करता है अब हमने
जो यह रोबोट वाली चीज की थी इसको हम स्विच की हेल्प से करते हैं तो पूरी की पूरी कॉर्ड को हम कमेंट कर देते हैं कमेंट को
है कान्वेंट स्कूल का वो पार्ट होते हैं इसको कंपाइलर इग्नोर करता है मतलब यह कोण का पार्ट है कि नहीं यह नार्मल इंग्लिश है
जिसको हम इग्नोर करने वाले हैं और हमारी कोड में यह बिल्कुल भी एग्जीक्यूट जो है नहीं हो रहा होगा तो हम कमेंट के बारे में
आगे वाली राशि में सीखेंगे आप इसको कम डिलीट डिलीट भी कर देंगे तो भी चलेगा हम बस कंपैरीजन के लिए रख रहे हैं तो कॉर्ड
में लिखेंगे स्विच स्विच के अंदर के पास करेंगे हमारा बटन और यहां पर लिखेंगे के इस वंश के स्वर्ण क्या कहते है कि आप
प्रिंट कर दीजिए हेलो हेलो हमने प्रिंट किया फिर कर गए हम ब्रेक फिर आता है हमारा केस-2 केस तू क्या कहती है कि आप प्रिंट
कर दीजिए नमस्ते नमस्ते हमने प्रिंट किया किया ब्रेक उसके बाद आता है कि स्त्री के स्त्री हमारा कहता है कि आप प्रिंट कर
दीजिए बावजूद व अंजू हमने प्रिंट किया उसके बाद किया ब्रेक व तो फिर आता है हमारा डिफ़ाल्ट यानि स्पेशल
केस कि डिफ़ाल्ट में हमने कि क्या प्रिंट किया इनवेलिड बटन इनवेलिड
बटन जब प्रिंट के तो यहां पर एक हमें जरूरत है ही नहीं तो कुछ इस तरीके से हमारी जो एक्शंस स्टेटमेंट सी वह स्विच की
फॉर्म में कन्वर्ट हो गई और आप ध्यान से देखेंगे तो यह बहुत क्लीन लग रहा है देखने में आपको स्विच वाला कॉर्ड तो इसीलिए
स्विच को हम यूज करते हैं जो बहुत सारे एग स्टेटमेंट हो जाते हैं और अपने कोड को हमें थोड़ा सा क्लीन करना होता है तो बीच
सेम चीज को इमरान करेंगे ज्यादा के अंदर एंड अब कौन सा बटन प्रेस करेंगे चलिए प्रेस करते हैं बटन नंबर 21 और इससे हमारे
पास प्रिंट हो गया है नमस्ते तो इस तरीके से जावा के अंदर हमने कवर कर लिए हैं इफैक्ट्स एंड सर्टिफिकेट्स और ब्रेक अब
इसके नीचे डिस्क्रिप्शन बॉक्स में आपको नोट्स मिल रहे होंगे साथ के साथ मिल रहा होगा एक इंपॉर्टेंट होमवर्क प्रॉब्लम
होमवर्क प्रॉब्लम है कि आपको अपना एक केलकुलेटर बनाना है अब होमवर्क प्रॉब्लम जरूरी इसलिए है क्योंकि आपने क्वांटिटी
में इनको खुद से करके देखना है और जब आप उस प्रॉब्लम को करेंगे तो बिल्कुल भी सलूशन को नहीं देखना खुद से ट्राय करना है
इटली से डेढ़ घंटा कि आप से उस चीज को पा रही है या नहीं और अगर उसमें आपको थोड़ी सी परेशानी आती है तो होमवर्क प्रॉब्लम का
सलूशन भी नीचे दिया हुआ है तो फिर आप उस सलूशन को रेफर करें उससे आप नहीं सीखे एंड जितना हम सीखेंगे उतना हम आगे बढ़ रहे
होंगे तो आए हो कि आज की क्लास हेल्पफुल रही होगी कन्फेशनल स्टेटमेंट को समझने में अनाज हवा को थोड़ा सा और समझने में आज के
लिए इतना ही मिलते हैं नेक्स्ट वीडियो में चिल्ड्रन एंड कीप लर्निंग कीप प्रैक्टिसिंग इन की पिक छोरी रे
Heads up!
This summary and transcript were automatically generated using AI with the Free YouTube Transcript Summary Tool by LunaNotes.
Generate a summary for freeRelated Summaries
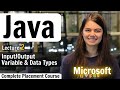
Java Basics: Outputs, Variables, and User Input Explained
Learn Java's fundamentals: how to give output, use variables, data types, and take user input effectively.
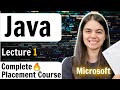
Java Course Introduction: Mastering Coding Fundamentals and Data Structures
Kickstart your Java programming journey with our guided course covering basics to algorithms for aspiring developers.
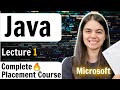
Java Programming Course: Introduction, Structure, and Setup Guide
Learn about Java programming fundamentals, data structures, and how to set up your coding environment.
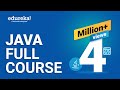
Java Programming: A Comprehensive Guide to Understanding Java and Its Concepts
Explore Java programming concepts including OOP, exception handling, and collections. Learn how to build robust applications!
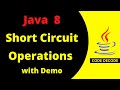
Understanding Java Short Circuit Operations: A Comprehensive Guide
Explore Java short circuit operations, including examples and benefits for improved performance in programming.
Most Viewed Summaries
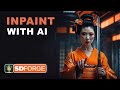
Mastering Inpainting with Stable Diffusion: Fix Mistakes and Enhance Your Images
Learn to fix mistakes and enhance images with Stable Diffusion's inpainting features effectively.
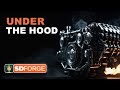
A Comprehensive Guide to Using Stable Diffusion Forge UI
Explore the Stable Diffusion Forge UI, customizable settings, models, and more to enhance your image generation experience.
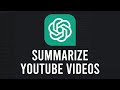
How to Use ChatGPT to Summarize YouTube Videos Efficiently
Learn how to summarize YouTube videos with ChatGPT in just a few simple steps.
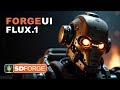
Ultimate Guide to Installing Forge UI and Flowing with Flux Models
Learn how to install Forge UI and explore various Flux models efficiently in this detailed guide.
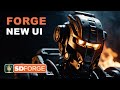
How to Install and Configure Forge: A New Stable Diffusion Web UI
Learn to install and configure the new Forge web UI for Stable Diffusion, with tips on models and settings.