Introduction
Welcome to the world of Java programming! Today, we are delving into a critical aspect that often pops up during Java interview questions: short circuit operations. These operations are not just a technical detail but can significantly enhance the performance of your Java applications. We’ll explore what short circuit operations are, how they work, and why they are essential to understand for any Java developer.
What Are Short Circuit Operations?
Short circuit operations in Java refer to the behavior of Boolean expressions where the evaluation can stop as soon as the outcome is determined. In Java 8, these operations reduce unnecessary evaluations and improve performance, especially in conditions with multiple clauses.
When dealing with logical operations (such as AND and OR), short circuiting means that not all conditions need to be evaluated if the outcome can already be determined from the evaluated conditions.
Boolean Short Circuit Evaluation
To understand short circuit operations, let’s first look at Boolean short circuit evaluations. Here’s a simple example:
if (10 > 5 && 5 > 3) {
// Execute some code
}
In this expression, if the first condition (10 > 5) evaluates to true
, only then is the second condition checked. If the first evaluates to false
, Java doesn’t check the second condition, thereby saving time and computational resources.
Benefits of Short Circuiting
The primary benefits of using short circuit operations include:
- Performance Optimization: By skipping unnecessary evaluations, Java reduces the computational load and increases speed.
- Logical Integrity: Ensures that code executes logically, preventing potential runtime errors from evaluating unsupported conditions.
Types of Short Circuit Operations in Java
Java provides two primary logical operators that demonstrate short circuiting:
- AND (
&&
): Evaluates totrue
only if both operands aretrue
. - OR (
||
): Evaluates totrue
if at least one of the operands istrue
.
AND (&&) Condition
In an AND condition, if the first operand is false
, Java immediately concludes that the overall expression is false
, and further conditions are not checked.
Example:
if (10 < 5 && 5 > 3) {
// This code won't run because the first condition is false.
}
In this example, since 10 < 5
is false, the second condition 5 > 3
is never evaluated.
OR (||) Condition
With an OR condition, if the first operand is true
, the subsequent conditions aren't checked because the overall outcome will be true
.
Example:
if (10 > 5 || 5 < 3) {
// This code will run because the first condition is true.
}
Here, since 10 > 5
evaluates to true
, the second condition (5 < 3
) is irrelevant, and the evaluation is skipped.
Short Circuit Operations in Streams (Java 8)
In addition to Boolean expressions, Java 8 stream APIs also leverage short circuit operations. These are categorized into intermediate and terminal operations.
Intermediate Operations
Intermediate operations return a stream and are lazy-loaded, meaning they are not evaluated until a terminal operation is invoked.
Limit Operation
A common example is the limit()
method, which restricts the number of elements returned.
List<Employee> employees = getEmployees();
List<Employee> limitedEmployees = employees.stream()
.limit(3)
.collect(Collectors.toList());
In this example, if the list originally contains five employees, the limit()
method returns only the first three and ignores the rest. This operation saves processing time by stopping further evaluations once the required amount is reached.
Terminal Operations
Terminal operations conclude the stream processing and may trigger the short-circuiting for efficiency. Here are several key methods:
- findFirst(): Returns the first element that matches a condition.
- findAny(): Returns any element, which may vary with parallel streams, enhancing performance.
- anyMatch(): Checks if any of the elements match a specified predicate, returning early if a match is found.
- allMatch(): Validates whether all elements meet a condition and short circuits if a single mismatch occurs.
- noneMatch(): Checks if no elements meet a condition, terminating early upon finding a match.
Demonstration of Terminal Operations
Assume you have a list of employees and you want to find an employee based on a condition using anyMatch()
:
boolean hasCodeEmployee = employees.stream()
.anyMatch(emp -> emp.getName().contains("code"));
In this case, if the first employee's name contains "code", it skips evaluating others, showcasing the efficiency of short-circuiting.
Differences between findFirst() and findAny()
- findFirst() guarantees the retrieval of the first element in the encountered order, making it deterministic.
- findAny(), however, allows for returning any of the elements in terms of performance, especially in parallel processing, leading to non-deterministic results.
Conclusion
Understanding Java short circuit operations is essential for writing efficient code. They not only improve performance but also ensure that your code executes correctly without unnecessary evaluations. As a Java developer, mastering these concepts will undoubtedly give you an edge in technical interviews and real-time applications.
In summary, short circuit operations enhance Java’s logical capabilities and stream handling, making your code cleaner and faster. If you have any further questions or need clarification on any aspect of short circuit operations, feel free to reach out. Happy coding!
hey guys welcome to code decode today in this video we're going to cover some interview questions on Java short
circuit operations so let's get started now what are short circuit operations so in Java 8 short circuit
operations are just like Boolean short circuit evaluations in Java now you'll ask me what were those Boolean short
circuit evaluations in Java so let's see with an example here now suppose in Java I had an if condition and if condition
evaluates to say if 10 is greater than five and and if 5 is greater than three if this is the case then do something so
this is a Java simple expression so this is a Boolean expression which will either evaluate to
true or false and on based of that the if condition if it is true will execute these statements or if if it evaluates
to false it will not evaluate this expression in this case if 10 it it says if 10 is greater than 5 yes it is
greater than five so it becomes true but it's not just enough then it has to see all the parameters apart from just the
first one all the conditions in the end end one so is five also greater than three yes it is true if both the
conditions evaluates to true then the output is true so how does this evaluates so if true and then true then
it becomes true if true and second evaluates to false the whole output is false if first is false and if this is
this is true or this is false it doesn't matter the output will remain false because at the first place it becomes
false so if the condition is opposite 10 less than five so is 10 less than 5 no so it's it's false so it what Java
compiler will do is it will not evaluate any further condition so all the conditions after this will be ignored by
compiler as soon it as it gets s false is the first parameter in end end condition so as soon as it gets false
the second or the third and the fourth oper doesn't even matter so in this case you will always get false so this is
short circuited and hence it is not evaluated so what is the benefit in doing this with Java compiler Java
compiler does not need to evaluate this and this and any n number of conditions here at the first place itself it gets
its result and it will not evaluate the if block hence it saves the compiler time so saves time increase performance
and many more things so this was the advantage of short circuiting so not just this was the operation that we used
to have for the short circuit we have one more operation right and the operation is or condition so if 10 is
greater than 5 or 5 is greater than three in this case do something what if this is true so if the first condition
is true the rest of the part is neglected by compiler why because or condition says if the first is true or
the second is true in any of the case either of the case you have to execute the code in the if Block in Boolean
short circuit Logic for example the first Boolean and second Boolean if first Boolean is false then remaining
part is ignored because of and operation the operation is short subed now because now similar in the case of first Boolean
or second Boolean if first bu itself is true remaining part is short circuited because the remaining evaluation will be
redundant it will be just the same result because the first one is true why to go to next one and see if it is false
or true if first is true rest everything will be short circuited in java8 stream short circuit operation this is not just
limited to Boolean types there are predefined short circuit operations and what are these short circuit operations
there's an intermediate and terminal operation so we have seen in java8 we had two types of operations the first
one was intermediate second was terminal what were intermediate and what were terminal operations we have already
covered this in real depth and the link to the video is given in the description below just for the revision purpose I'll
just reciprocate it once so intermediate operations were like filter map and these always returns you stream object
and these were lazy loaded they were not executed and the result is not returned until the terminal operation is
performed on The Stream So example is for each collect minmax and many more so these were two type of operations we had
to perform on stream that is terminal and the intermediate one now Java short circuit operations are are in both type
so intermediate we have an example of limit I'll cover this in a minute so limit is intermediate one and find first
find any any match all match and none match these are terminal operations I'll show you why these are short circuited
and how do they work in short circuit in Java it so let's get started with first the intermediate one now intermediate
one is just one method that is limit this method takes one argument and returns a stream so I
already told you what does intermediate returns intermediate always returns you a stream object it returns you stream of
no more than n elements and N is going as an argument where n is the number of elements the stream should be limited to
and this function returns a new stream as an output so before going into the more theoretical part let's come quickly
see the demonstration part so this is the rest API demo we have already covered this while doing the
crud operations I have added the dev tool so that we don't have to restart it again and again in this example I'm
going to take an example of find all so what does find all returns you it returns you list of employees and how
many employees do we have so here we have list of five employees the ID is one to five and name as code decode
updated one updated two code and decode and the fifth one is null if I go to uh to reposit and say find all there will
be list of five employees to me now I don't want five employees I want to limit it only to two or three so what
should I do so let me just quickly run it and see what is the current result and show you all the five right
now the service server is running for me currently and with find all I have a result and result has five the list of
five employees it goes as first as code decode updated one updated two code decode and null is the name so these
were the five ones for me now what if I want to just limit it to two or three elements I don't want more of them the
find all will give me a list what I can do on a list I can open a stream as soon as you open a stream what you can do I
just want to limit it to only three employees or I just want to limit it to only two employees now this is an
intermediate operation this is going to return you a stream object so this will return you a stream object you have to
put some terminal operator so that you will be able to evaluate the limit so collectors
dot to list what will it return you it will still return you list of employees and
let's see now if I hit it so I should not get all the five of them I should just get two of them so see my operation
is short circuited so it is a stream so it says okay let me get get the first one let me get the second one but as
soon as your limit reaches two it will reject all of them and it will be short circuited and just two will be return
return back to you so in Java it limit method retrieves only the first n objects in the setting the maximum size
and ignores the remaining values after size of n so in our case our size was two so only first two objects were
returned and rest remaining all the three items were rejected the return type is stream of
object and that is why I have to put collect and collect it as a list as a terminal operator because this is an
intermediate one as soon as limit reaches the maximum number of item that is two in our case it doesn't consume
any more item and simply Returns the resulting stream so here as soon as two items of employees are reached all the
remaining three are rejected and hence just the size of two is returned to the collectors to collect in any format you
want so this is why we say that limit is a short circuit operation all the five elements of the stream are not executed
just the two of them are executed rest all the three are ignored and hence it is short circuited limit returns first n
elements and not just any n elements so this is very important this is very important from interview perspective the
limit operation returns you first n elements and not any of the N elements so if you have here five of the
operations you will not get null and code as an output when you limit it with two it will just R you first two so that
is why limit is the sequential operation and it returns you first n elements that is two in our case in an encounter order
that is in sequential order and not just any random order so this was the limit operation it was an intermediate
operation it is good choice when you're working on infinite stream or infinite Valu so limit is involved after calling
an terminal operation such as count or collect so I've already told you with this since this is an intermediate
operation terminal operation is required because this is lazy loaded this returns a stream of elements with size or
maximum limit given in our case we have given it as two this works well with sequential streams and it is not sest to
parallel streams or for the larger higher size limit the max size cannot be negative what if I give this as minus 2
rather than two it will give you illegal argument exception so it this is a runtime exception it's not compile
time so if I now try to hit it it will give you an exception it says illegal argument
exception this is how I proved you that the max siiz cannot be negative if the interviewer ask you what if the if you
try to limit it at minus 2 it will give you illegal argument exception now this was all about the
intermediate one now what are the terminal short cir methods we have the terminal short circuit methods we have
is is find first so what it does is it goes and find any of the first element which matches your condition so before
going to the theory let's quickly see in the demo part so this is your list of employees
you're going to open a stream to it you have each and every object in this stream of employee
I'm going to filter it with some condition EMP such that MP do get name on some condition and then I will do
like uh F first dot find first now this is since it's a terminal operation it does not need any kind of chaining
terminal operations because only one terminal operation is allowed so we don't want to collect it or do something
and since it is going to return just one employee you should not have the list of employees here just one employee is
going to be returned to you and here also going to change it to just one employee and I'm going to filter out
only those employees whose name contains code in the name so does any of so does any of my employees name contains code
in it so let's see yes there were three names which contains code in it that is 1 2 and three now it says find the first
one who contains name as code so what should be the first one that should be returned that is code decode updated one
but find first returns you an optional so what you need to do is you need to do get now let's see is there any fine
first employee which returns you this so yes the first one is code decode updated one so we had three values which
contains code that is code decode updated one code decode updated two and third is code simple simple code so with
this terminal operation find first what it does is it goes in n getes to each and every of the stream object so it
goes first with in the sequence order it goes code decode updated one and check does the name contain code yes it does
so all the 2 3 4 5 all of these stream elements are short circuited and with the first El itself you get your
response in your employee and return back to your client now suppose these were just five rows what if I had
hundreds and thousands of rows that you have to check then in that case these short circuit operations increases your
performance because it doesn't have to go to all thousands and thousands of rows the very first row returns you the
cre and it returns you the object so this is fine first for you so the fine first written the very
first element wrapped in the optional object so what we did was we had to do get in the case of optional object in
the Stream before traversing the others one the terminal operation terminated on finding the very first element and hence
the rest are short circuited and hence this is short circuit operation okay now the next short
circuit operation is find any so what is the difference between find First and find any so it returns
you an optional instance which wraps only and only one element of the stream so even fine first used to written me
only one and one element the first one so fine first behave fine first operation as per the documentation in
Java the behavior of this expression is explicitly non-deterministic what does that mean you cannot determinately say
that yes you're going to return as a result as code decode updated one there can be chances you can get code decode
updated two or code so there were three so here there were three rows code decode one updated two and
code so any of the three can return if you use find any this is to allow maximum performance in parallel
operation the cost is that multiplied invocation of the same resource may not return the same result so it's
explicitly say you if you run it multiple times you're not going to get the same result and hence it is not
important in nature do we know what important is ID imp poent is which when operated multiple times give you same
result it doesn't give you same result and hence you can say the difference between find any and find first is find
first is itm poent in nature this is not itm poent in nature if you want to get a stable result then always use find first
rather than find any so find any in here it is going to give you code decode updated one because it is a sequential
order what if I use a parallel stream see I have used a parallel stream here and what is the response I got here
as ID three code as the output so this is the third one this is the third row which got as an output so this is the
feature of find any so if you would have used find first here this might not have been the
case fine first is always going to return you the very first in the sequential
order so this is the find first result and that was your find any result so this is a very big difference between
the find First and find any you will find this difference only lonely case when you use the parallel streams
now for sequential stream there won't be any difference between find First and find any I have shown you just now but
for parallel stream find any will return any element rather than waiting for the first element so this is a very big
difference between find First and find any this is a very important from the interview perspective now the third one
is any match and this is going to return return your Boolean condition so on basis of this Boolean condition you can
evaluate your further steps so there are multiple cases where you need to find any if any of these matches then only
proceed with your business logic else stop then and there so this is to test whether any element matches the provided
predicate so we are going to pass a predicate here so let's quickly see the Demo First now from repo find all the
elements and stream it out and rather than filtering and using terminal operation
you don't need any kind of intermediate operation right now rather you can use any match
and pass your condition here itself so any match employee such that employee. getet name do contains code if it does
return you Boolean return this Boolean as true and false okay now what I'm doing is find all and while you doing
find all stream it up so you have in stream five objects now in these five objects find any employee whose name
contains code so what it does is it goes in all the five and it says okay the first one
itself contains code if that is the case it finds any who contains code return it as true and rest all the four records
are not going to be valuated now there must have been like thousands and thousands of rows if the first itself
return you true your performance increases a very good extent because all the rest 10 thousands and thousands of
rows are not going to be valuated in your stream now let's see and run it it should get true so you you are getting
true and hence this is a short circuit operation why because that this is a terminal method which will return you as
soon as it finds the match and in our case it find the match with employee whose name is code as the
first one itself and hence it returns you and and rest of the four rows are not going to Traverse at all so that was
about any match now all match now this is as good as any match was as good as or condition the first one is evaluated
true true it returns you the response now here it tests whether all the elements should match the predicate now
how it will be short circuited what if the first element itself doesn't match your condition and it says it all should
match and this is as good as and and operation this was as good as or operation now this is as good as and and
operation so if the first one is false rest all will be redundant and hence will be short circuited so let's see the
demo all match the predicate whose employees name contains new suppose and it will return you false why because it
goes to the first one it says does it it should all of them should match so does it contains new no it doesn't so the
first one itself says my name does not contains new and it says every every name should have new in it it doesn't so
the first one it plays it it returns you new false and it returns you false in the postman itself so hence the all the
four were short circuited now it says None matches this is opposite of all matches it says test whether no element
of the stream match the provided predicate it may return early if the false result when any element matches
the predicate so none matches let's see the demo NM says no one should match my condition if I give you code
the first one itself is the code so it says the first one matched so it says no none of you should match so the if since
the first one match it will give you false at the first place so let's see if this
works yes it works with a false condition why because the first one matched so as soon as the first one
matched all of these remaining rows are neglected so this was all about the terminal and non-terminal operations or
the intermediate operations I have few more things to cover on Java optional because it is very important from the
interview perspective so the way how you can create the optional the way how you can see whether the value is present and
all the operations on optional you can do but if you want me to cover optional in the next video just let me know in
the comment section then only I will cover Java optional interview questions for you thank you
Heads up!
This summary and transcript were automatically generated using AI with the Free YouTube Transcript Summary Tool by LunaNotes.
Generate a summary for freeRelated Summaries
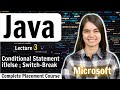
Mastering Java: Understanding Conditional Statements for Beginners
Dive into Java's conditional statements, including 'if', 'switch', and 'break', to enhance your programming skills.
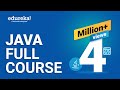
Java Programming: A Comprehensive Guide to Understanding Java and Its Concepts
Explore Java programming concepts including OOP, exception handling, and collections. Learn how to build robust applications!
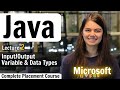
Java Basics: Outputs, Variables, and User Input Explained
Learn Java's fundamentals: how to give output, use variables, data types, and take user input effectively.
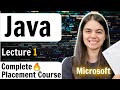
Java Course Introduction: Mastering Coding Fundamentals and Data Structures
Kickstart your Java programming journey with our guided course covering basics to algorithms for aspiring developers.
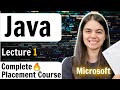
Java Programming Course: Introduction, Structure, and Setup Guide
Learn about Java programming fundamentals, data structures, and how to set up your coding environment.
Most Viewed Summaries
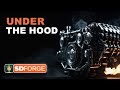
A Comprehensive Guide to Using Stable Diffusion Forge UI
Explore the Stable Diffusion Forge UI, customizable settings, models, and more to enhance your image generation experience.
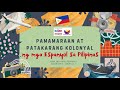
Pamaraan at Patakarang Kolonyal ng mga Espanyol sa Pilipinas
Tuklasin ang mga pamamaraan at patakarang kolonyal ng mga Espanyol sa Pilipinas at ang mga epekto nito sa mga Pilipino.
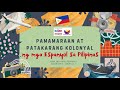
Pamamaraan at Patakarang Kolonyal ng mga Espanyol sa Pilipinas
Tuklasin ang mga pamamaraan at patakaran ng mga Espanyol sa Pilipinas, at ang epekto nito sa mga Pilipino.
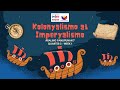
Kolonyalismo at Imperyalismo: Ang Kasaysayan ng Pagsakop sa Pilipinas
Tuklasin ang kasaysayan ng kolonyalismo at imperyalismo sa Pilipinas sa pamamagitan ni Ferdinand Magellan.
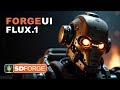
Ultimate Guide to Installing Forge UI and Flowing with Flux Models
Learn how to install Forge UI and explore various Flux models efficiently in this detailed guide.