Introduction to Java Programming
Welcome to our introductory Java course! In this article, we will embark on an exciting journey into the world of programming with Java. Whether you're a beginner curious about coding fundamentals or an experienced developer looking to brush up on your skills, this course is tailored for everyone. Our course is divided into two main parts: Introduction to Programming and Data Structures and Algorithms. Let's dive in!
Why Learn Java?
Java is a powerful, high-level programming language that's widely used in the software industry. Here are some compelling reasons to learn Java:
- Platform Independence: Write once, run anywhere. Java code can run on any platform that has a Java Virtual Machine (JVM).
- Strong Community Support: A vast community of developers means ample resources, libraries, and frameworks are available.
- Object-Oriented Structure: Java is based on object-oriented programming principles, promoting code reuse and modularity.
Course Structure and Schedule
This course is structured to provide a solid foundation in programming with Java. Over the next 12 classes, we will cover:
- What is Code and How to Code?
- Installing Code Editors
- Input, Output, Variables, and Data Types
- Flow Control Statements
- Functions and Methods
- Introduction to Object-Oriented Programming
- Error Handling in Java
- Data Structures
- Algorithms
- Hands-On Projects
- Review and Final Project
- Exam Preparation
Understanding Code
Programming is essentially the act of communicating instructions to a computer. Just as we use languages like Hindi or English to communicate with people, computers understand a different language—binary code (0s and 1s). This binary representation is fundamental for how computers process data and execute commands.
The Concept of High-Level Languages
To make coding more manageable, we use high-level programming languages like Java.
- High-Level Languages: These languages are closer to human languages and are easier to read and write compared to low-level machine languages. When we write Java code, it is compiled into a form that the computer understands, making it less tedious than translating everything into binary.
Getting Started with Java
Before coding, ensure you have the right tools installed:
- Java Development Kit (JDK): This kit includes everything you need to develop Java applications, including the Java compiler and runtime environment.
- Code Editor/Integrated Development Environment (IDE): We recommend using Visual Studio Code (VS Code) or IntelliJ IDEA. This software allows you to write, test, and debug your Java code easily.
You can find detailed installation instructions for VS Code in our previous videos. It’s crucial to set up your development environment correctly, as it will help you code efficiently.
Writing Your First Java Program
Now that you have your development environment set up and ready, let's write your first Java code!
Creating a Simple Java Program
Here's the process:
- Open your IDE and create a new file called
FirstClass.java
. - Write the following code:
public class FirstClass { public static void main(String[] args) { System.out.println("Hello, World!"); } }
- Save your file.
- Compile and run the code. You should see Hello, World! printed on your screen!
This simple program demonstrates how Java syntax works. Each part of your program has a specific role. The main
function is the entry point for your application, where execution begins.
Understanding Java Execution
When a Java program is run, it goes through two stages:
- Compilation: Your code (written in
.java
file) is compiled by the Java compiler into bytecode (with a.class
extension). Bytecode is platform-independent, meaning it can run on any system with Java Runtime Environment. - Execution: The bytecode is executed by the Java Virtual Machine (JVM), which converts the bytecode into native machine code that your computer can understand and execute.
Key Components of Java
Understanding code structure is crucial in mastering Java:
- Classes: Fundamental building blocks of Java code. Each program consists of one or more classes.
- Functions/Methods: Blocks of code that perform specific tasks. They can take parameters and return values.
Conclusion
In this introduction, we covered the basics of Java programming, the course outline, and how to set up a coding environment. Remember, coding requires practice and persistence before you become proficient. Don't be disheartened if things seem challenging initially!
As you proceed through the course, utilize the resources provided, engage with exercises, and participate in projects to solidify your understanding of Java and its applications.
In the next class, we will dive deeper into input and output operations in Java. Until then, keep learning and practicing!
हाय एवरीवन एंड वेलकम टू अपना कॉलेज और इस वीडियो में हम शुरुआत करने वाले हैं अपने जावा कोर्स के साथ इस कोर्स को हमने दो
पार्ट्स में डिवाइड किया है जिसमें सबसे पहला पार्ट है इंट्रोडक्शन टू प्रोग्रामिंग यानी हम जावा को यूज करके
कोड क्या होता है कोडिंग प्रोग्रामिंग क्या होता है वो सब सीख रहे होंगे जितने भी फंडामेंटल्स जरूरी हैं जावा को जानने
समझने के लिए हम उन सबको सीखेंगे शुरुआत में और इसके सेकंड पार्ट में हम सीख रहे होंगे डाटा स्ट्रक्चर्स एंड एल्गोरिथम्स
को तो जो हमारा प्लान रहेगा इंट्रोडक्शन टू प्रोग्रामिंग का वो कुछ इस तरीके का रहेगा जिसमें फर्स्ट क्लास जो कि आज है हम
सीखेंगे कोड क्या होता है कोडिंग किस तरीके से होती है साथ के साथ इंस्टॉल करेंगे अपने कोड एडिटर्स को अगली क्लास
में इनपुट आउटपुट वेरिएबल एंड डेटा टाइप्स एंड इसी तरीके से आगे के कुछ टॉपिक्स को हम 12 दिन के अंदर 12 क्लासेस में कवर कर
रहे होंगे तो चलिए जावा सीखते हैं अपने शानदार कोर्स के साथ रियल लाइफ में अगर हमें किसी व्यक्ति से बात करनी होती है तो
एक सेट ऑफ इंस्ट्रक्शंस हम उसे देते हैं जो एक लैंग्वेज के माध्यम से देते हैं जैसे मैं आपसे बात कर रही हूं तो मैं
हिंदी लैंग्वेज में बात कर रही हूं हम इंग्लिश में भी बात कर सकते हैं लेकिन अगर हम एक कंप्यूटर या लैपटॉप या मोबाइल से
काम करवाना है तो उन्हें बताना पड़ेगा अब इंग्लिश या हिंदी तो उसे समझ में आता नहीं इसे समझ में आता है बाइनरी यानी ़ और वन
दीदी अब यह ज़ीरो और वन ही क्यों इसलिए यार क्योंकि हमारे लैपटॉप एंड कंप्यूटर इलेक्ट्रिकल डिवाइसेज होते हैं जो बिजली
से चलते हैं तो जब सर्किट के अंदर करंट जाता है उसे हम रिप्रेजेंट कर देते हैं वन से और जब सर्किट के अंदर कोई करंट नहीं
जाता तो उसे हम रिप्रेजेंट कर देते हैं जीरो से या इसे ऐसे समझो कि जब डिवाइसेज हाई वोल्टेज डिटेक्ट कर रही होती है तो
उसे + व से रिप्रेजेंट कर दिया या जब लो वोल्टेज डिटेक्ट कर रही है तो उसे हम जीरो से रिप्रेजेंट कर दें तो ऐसे में मान लो
कि जो हमारे रियल लाइफ डेसीमल नंबर्स होते हैं उनमें से रो को वी कैन रिप्रेजेंट विद रो इन बाइनरी डेसीमल के वन को वी कैन
रिप्रेजेंट विद वन इन बाइनरी डेसीमल के थ्री को वी कैन रिप्रेजेंट विद वनव इन बाइनरी एंड डेसीमल के फोर को वी कैन
रिप्रेजेंट विद 00 इन बाइनरी और इसी तरीके से हम आगे चले जाते हैं ऐसे ही ए बी सी डी ई एफज या स्पेशल कैरेक्टर्स जितनी भी
चीजें हैं उन्हें हम रो और वन के कॉमिनेशन में कंप्यूटर को समझा सकते हैं अब ऐसे में आपको दिमाग में एक चीज आएगी कि दीदी ये तो
बहुत कॉम्प्लिकेटेड लग रहा है कल को अगर मुझे दो नंबर का एडिशन भी करना हुआ जैसे 53 + 72 तो इसको तो 01 में कन्वर्ट करने
में बहुत सारा टाइम लग जाएगा तो मैंने कहा कि यहां आती है हमारी हाई लेवल लैंग्वेजेस जो हमें हेल्प करती हैं कैसे इस कोर्स में
जो हाई लेवल लैंग्वेज हम सीख रहे होंगे वो होगी जावा तो जावा में हम अपना कोड लिखते हैं और कंप्यूटर में एक कंपाइलर बैठा होता
है जो इसे बाइनरी फॉर्मेट में कन्वर्ट करके देता है जो कंप्यूटर को समझ में आने लगती है और यहां से शुरुआत होती है हमारे
कोडिंग सफर की तो आपने बात तो करना सीख लिया पर हमें कंप्यूटर को कुछ कुछ सेट ऑफ इंस्ट्रक्शंस भी देने हैं यह इंस्ट्रक्शंस
देने के लिए हमें प्रोग्राम करना पड़ेगा तो हम यूज कर रहे होंगे जावा लैंग्वेज को टू गिव कमांड्स या फिर इंस्ट्रक्शंस टू द
कंप्यूटर टू परफॉर्म समथिंग अब ये चीज अगर समझ में नहीं आ रही तो एक एग्जांपल लेते हैं अब डे टू डे लाइफ में अगर हमें कोई
काम एग्जीक्यूट करना है तो उसके लिए कोई सेट ऑफ इंस्ट्रक्शंस देते हैं जैसे हम अभी एक फ्लो चार्ट से समझेंगे जैसे सबसे पहले
मैं शुरुआत करूंगी स्टार्ट से मुझे कुछ रॉ मटेरियल चाहिए है जैसे मैगी मसाला और पानी उसके साथ एक बर्तन और एक स्टोव चाहिए उसे
पकाने के लिए नेक्स्ट स्टेप होता है कि हम पानी मसाला मैगी उस बर्तन में डालें और उसके बाद उसे पकाने लगे उसे बॉईल करते हैं
और चेक करते हैं कि क्या हमारी मैगी बन गई है अगर मैगी नहीं बनी तो हम कहते हैं कि इसे और बॉईल करो और अगर बन गई तो कहते हैं
कि गैस बंद कर दो फिर हम मैगी को सर्व करते हैं और उसे खाते हैं फिर हमने एग्जिट कर दिया अपने फ्लो चार्ट से सिमिलरली जब
हम कंप्यूटर को कोई बात समझाना चाहते हैं तो हम ऐसे ही सेट ऑफ इंस्ट्रक्शंस को यूज करते हैं इनको कोड में लिखने से पहले हम
देख लेते हैं कि फ्लो चार्ट की हेल्प से इन्हें कैसे समझा रहे होंगे इससे हमें पता चल जाएगा कि रियल लाइफ में हमारे
कम्युनिकेशंस कितना सिमिलर हो रहे हैं और इसी सेट ऑफ इंस्ट्रक्शंस को जो हमारे राइट में लिखे हैं कंप्यूटर साइंस की भाषा में
हम सूडो कोड कह देते हैं अब हमें क्या करना है मान लेते हैं कि हमारा काम है कि दो नंबर्स हमें किसी से लेने हैं और उनका
सम कैलकुलेट करके हमें प्रिंट करवा देना है उस सेम लॉजिक को हम फ्लो चार्ट की हेल्प से कैसे रिप्रेजेंट करेंगे हम सबसे
पहले शुरुआत करेंगे तो इसीलिए हम ड्रॉ करेंगे सबसे पहले अपना स्टार्ट उसके बाद इनपुट के लिए हम अपने वेरिएबल को इनपुट ले
लेंगे पहले वेरिएबल को स्टोर कर देंगे नंबर वन में दूसरे वेरिएबल को स्टोर कर देंगे हम नंबर टू में यानी नंबर वन और
नंबर टू हमने यूजर से इनपुट ले लिए हैं उसके बाद दोनों का सम हम एक सम नाम के वेरिएबल में स्टोर करा देंगे यानी सम में
नंबर वन प्लस नंबर टू हमने ऐड करके डाल दिया उसके बाद हम प्रिंट करवा देंगे अपने सम को एंड फाइनली हम कर जाएंगे एग्जिट तो
कुछ इस तरीके से फ्लो रहेगा जो काम हमें करना है उस काम का अब सिमिलरली अगर हम सूडो कोड की बात करें तो सुडो कोड के अंदर
सबसे पहला स्टेप क्या होगा कि हम स्टार्ट कर रहे हैं उसके बाद हम इनपुट ले रहे हैं दो नंबर्स को उसके बाद हम सम कैलकुलेट कर
रहे हैं नंबर वन प्लस नंबर टू की हेल्प से फिर हम प्रिंट करवा रहे हैं उसी सम को एंड फाइनली हम अपने कोड से कर जाते हैं एग्जिट
तो सूडो कोड एक तरीके से जो भी फ्लो होता है प्रोग्राम का उसको सिंपल इंग्लिश की भाषा में रिप्रेजेंट करने का तरीका होता
है और इसी सूडो कोड को बाद में हम कन्वर्ट कर देते हैं एक्चुअल जावा या c+ प या पाइथन कोड के अंदर अब अपने कोड को लिखने
के लिए सबसे पहले जावा के अंदर हमें इंस्टॉल करनी पड़ेंगी कुछ-कुछ चीजें इसमें से सबसे पहली चीज जो हमें इंस्टॉल करनी है
व है जावा का जावा डेवलपमेंट किट ये एक ऐसा किट है जिसके अंदर वो सारी चीजें भरी हुई हैं जो तो आपको चाहिए आपके सिस्टम पर
जावा चलाने के लिए तो जब भी आपको जावा का कोड लिखना होता है जावा का प्रोग्राम लिखना होता है और उसे किसी भी सिस्टम पर
रन करना होता है तो हमें जावा डेवलपमेंट किट की जरूरत पड़ती है कुछ जरूरी टूल्स लेने के लिए उसके साथ-साथ हमें डाउनलोड
करना पड़ेगा एक कोड एडिटर या आईडी यानी इंटीग्रेटेड डेवलपमेंट एनवायरमेंट ये कोई भी हो सकता है हम अपने कोर्स में यूज कर
रहे होंगे विजुअल स्टूडियो कोड यानी वीएस कोड इसके अलावा आप इंटेलीज भी यूज कर सकते हैं आप इ क्लिप भी यूज कर सकते हैं यह कुछ
सॉफ्टवेयर्स हैं जैसे गेम के लिए हम गेम्स डाउनलोड कर रहे होते हैं वैसे ही कोड लिखने के लिए हम एक सॉफ्टवेयर डाउनलोड
करना पड़ता है अपने लैपटॉप या कंप्यूटर के ऊपर तो इन सॉफ्टवेयर्स को डाउनलोड करने के बाद हम अपना कोड इनके ऊपर लिख रहे होते
हैं और यह हमारे कोड को रन करके हमें कुछ दिखा रहे होते हैं कि एक्चुअल रिजल्टेंट आउटपुट हमारा क्या आने वाला है अब हमने
ऑलरेडी दो वीडियोस बनाए हुए हैं इंग्लिश और हिंदी में वीएस कोड को डाउनलोड करने की इनमें से सबसे पह पहला वीडियो है जो आपको
c+ प सीरीज में दूसरे इंडेक्स पर मिल रहा होगा उसके अंदर c+ प के अंदर हमने डाउनलोड करके दिखाया था आपको c+ प के कंपाइलर्स उन
सबको हमें डाउनलोड नहीं करना हमें सिर्फ डाउनलोड करना है जावा डेवलपमेंट किट को साथ के साथ विजुअल स्टूडियो कोड को अब
चाहे आप विज पर काम कर रहे हैं या आप मैक पर काम कर रहे हैं ये दोनों चीजें डाउनलोड करना सफिशिएंट है इसके लिए जाकर आप उन
दोनों वीडियोस को रेफर कर सकते हैं साथ के साथ अगर आपको इंटेलीज को डाउनलोड करना तो उसके लिए भी हमने एक ऑलरेडी वीडियो बनाया
हुआ है जावा वन शॉट के नाम से जिसके अंदर एक मिनट पर जब आप जाएंगे तो वहां पर जाकर आपको पूरा इंस्टॉलेशन प्रोसेस मिल जाएगा
कि जावा डेवलपमेंट किट को कैसे डाउनलोड करना है और इंटेलीज को कैसे डाउनलोड करना है उसके साथ-साथ हम आपको अभी दिखा भी देते
हैं कि जैसे ही आप अपने कम पर जाएंगे तो कम या जो भी ब्राउजर आप यूज करते हैं उस पर जाकर आपको सर्च करना है
जावा डेवलपमेंट किट के बारे में और कुछ इस तरीके से जो पहला ओरेकल का लिंक आ रहा होगा उस पर आप जाएंगे तो वहां पर
आपको डाउनलोड करने के लिए डिफरेंट डिफरेंट फॉर्मैट्स मिल जाएंगे अगर आप इसके अलावा एक और ऑप्शन है जिसमें पूरा का
पूरा जावा डेवलपर पैक आपको मिल रहा होता है तो माइक्रोसॉफ्ट ने क्या किया है विजुअल स्टूडियो कोड के साथ कुछ एक्सटेंशन
मिला दिए हैं जावा डेवलपमेंट किट मिला दिया है जिसकी वजह से एक ही लिंक में आपको सारी की सारी चीजें डाउनलोड करने को मिल
जाएंगी तो उसके लिए भी हम सर्च कर लेते हैं विजुअल स्टूडियो कोड इंस्टॉल पैक जावा तो यहां पर यह जो पहला लिंक आएगा
जावा इन विजुअल स्टूडियो कोड का उसके अंदर हमें जाना है थोड़ा सा स्क्रॉल करना है और यह इंस्टॉलिंग द कोडिंग पैक फॉर जावा विज
अगर आप विज यूज करते हैं तो या फिर इंस्टॉलिंग द कोडिंग पैक फॉर जावा मैक ओ अगर आप मैक यूज करते हैं तो तो इस तरीके
से आप जाकर विजुअल स्टूडियो कोड को सेटअप कर सकते हैं अपने सिस्टम के ऊपर एंड आगे के कोड जो हम लिखेंगे जावा के साथ वह हम
उसी कोड एडिटर पर लिख रहे होंगे अब बात करें कि अगर यह कोड एडिटर कैसा दिखता है तो डाउनलोड करने के बाद हमें विज स्टूडियो
कोड कुछ इस तरीके से दिख रहा होगा जिसमें हम क्रिएट करेंगे अपनी एक नई फाइल तो नई फाइल आप क्रिएट कर सकते हैं क्रिएट करने
के बाद अब इस फाइल में हम लिखने वाले हैं अपना पहला जावा कोड अब इस कोड को लिखने से पहले हम एक छोटी सी बात कर लें कि शुरू की
दो-तीन क्लासेस में हम जो जो कोड लिख रहे होंगे वो अगर आप प्रोग्रामिंग कोडिंग में नए हैं अगर आप पहली बार कोड को देख रहे
हैं तो आपको थोड़ी सी परेशानी आ सकती है समझने में लेकिन हमें हार नहीं माननी है हमें चीजों को समझने की कोशिश करनी है
शुरू शुरू में जैसे अगर हम अरेबिक या फिर फ्रेंच सीखने जाएं तो हमें चीजें काफी अलग लगेंगी और समझ नहीं आएंगी लेकिन थोड़े
टाइम के बाद हमें चीजें समझ आनी शुरू होगी तो वो फेथ वो ट्रस्ट हमें दिखाना पड़ेगा कोड के ऊपर हमें थोड़ा टाइम जब कोड को
देखते हुए हो जाएगा तब हमें चीजों के मतलब समझ में आने शुरू होंगे तो पहली क्लास के अंदर हम कोड लिखेंगे कुछ चीजें समझ नहीं
आएंगी बहुत सारी चीजें समझ आएंगी तो हमें उन चीजों को समझने पर ध्यान देना है सबसे पहले जावा कोड में जो हम लिखते हैं वो
बनाते हैं एक क्लास तो क्लास का नानाम हम लेते हैं फर्स्ट क्लास हमने इसका नाम दे दिया इसके अंदर हम बनाएंगे एक पब्लिक
स्टैटिक वॉइड मेन नाम का फंक्शन जिसमें स्ट्रिंग आर्गुमेंट आ रहे होंगे तो कुछ इस तरीके से जावा में कोई भी
कोड लिखने का यही टेंप्लेट रहता है जिसमें आप एक क्लास डिफाइन करते हैं और आप एक फंक्शन डिफाइन करते हैं अब ये क्लास और
फंक्शन क्या होता है यह हम थोड़ा सा देर बाद समझेंगे पर अभी के लिए मान लेते हैं कि जब भी जावा कोड लिखना होता है हमें यह
चीजें करनी होती है एंड इस फाइल को सेव करने का क्या तरीका रहेगा जो भी आपने जावा के अंदर फाइल के अंदर क्लास का नाम दिया
है वही नाम आप अपनी जावा फाइल को देंगे जैसे यहां पर क्लास का नाम दिया था फर्स्ट क्लास तो हम अपनी जावा फाइल को सेव करेंगे
एज फर्स्ट क्लास डॉट जावा तो जावा के अंदर जितनी भी फाइलें हम सेव करते हैं वो डॉट जावा के नाम से सेव होती है जैसे हम 11th
12th क्लास में या फिर जब भी कंप्यूटर साइंस पढ़ते थे स्कूल में तो ड txt.gz सबसे पहली चीज सबसे पहला कोड जो यूजुअली
सारी की सारी प्रोग्रामिंग लैंग्वेजेस में कोडर्स लिखते हैं वो होता है हेलो वर्ल्ड जिसमें आप पहली बार वर्ल्ड को हेलो कह रहे
हैं अपनी प्रोग्रामिंग लैंग्वेज को यूज करके तो उसे लिखते हैं system.out प्रिंट एलन हेलो
वर्ल्ड इसे सेव कर दिया अब हम इस कोड को रन करेंगे रन करने के बाद आपको पता चलेगा कि एक्चुअली कोड से होता क्या है जावा कोड
का मतलब क्या होता है ये कुछ लाइनें हमने अपनी फाइल में सेव कर दी अब यहां पर राइट में जाकर रन जावा अगर हम क्लिक
करें तो हमारे लिए कुछ ऐसा प्रिंट होगा हेलो वर्ल्ड अब यह चीज हम कैसे समझ सकते हैं जैसे हम देखते हैं कि कोडर्स
बहुत सारा कोड लिखते हैं और फिर उसके आउटपुट में क्या आता है एक वेबसाइट हमें दिखने लगती है वैसे ही यहां पर जावा का ये
जो छोटा सा कोड हमने लिखा है इसकी वजह से हमें अपनी स्क्रीन पर यह हेलो वर्ल्ड दिखना शुरू हो गया है तो यह कुछ जादू है
जो कोडिंग में होता है जिसमें कोड की हेल्प से आप स्क्रीन पर कुछ भी दिखा सकते हैं अब स्क्रीन पर दिखाने को हम आउटपुट
कहते हैं इसके अलावा अपने कोड की हेल्प से आप यूजर से कोई भी डाटा ले सकते हैं जिसको हम इनपुट कहते हैं इनपुट आउटपुट के बारे
में हम अगली क्लासेस में समझ रहे होंगे अभी के लिए हमने समझ लिया कि कुछ इस तरीके से जावा का कोड रन करता है और हमें एक
आउटपुट दिखा देता है अब थोड़ा थोड़ा सा समझते हैं कि जावा कोड एक्चुअली रन कैसे करता है जावा कोड को रन करने के लिए जो
हमारा कोड होता है वह दो स्टेप्स के थ्रू जाता है जिसमें से सबसे पहला स्टेप होता है कंपाइलेशन का और दूसरा स्टेप होता है
एग्जीक्यूशन का अब ये थ्योरी समझना थोड़ा सा इंपॉर्टेंट है क्योंकि ये थ्योरी कभी-कभी आपसे बाद में जाकर आपके प्लेसमेंट
इंटरव्यू में पूछ ली जाएगी या फिर अगर आपके कॉलेज में आप जावा एज अ सब्जेक्ट पढ़ते हैं तो वहां पर एग्जाम में आ जाएगी
अब जो जावा डेवलपमेंट किट हमने डाउनलोड किया है यानी जेडी के उसके अंदर जे आरई नाम का एक कंपोनेंट है जिसे हम कहते हैं
जावा रन टाइम एनवायरमेंट जे आरई के साथ-साथ इसके अंदर काफी सारे डेवलपमेंट टूल्स भी अवेलेबल हैं
और यह जो जे आरई है इसके अंदर भी एक और कंपोनेंट है जिसको हम कहते हैं जेवीएम जेवीएम यानी जावा वर्चुअल मशीन तो जावा
वर्चुअल मशीन जे आरई और जेडी के ये एक के अंदर एक लेवल वाइज फॉर्म होते हैं लेवल वाइज स्टोर्ड होते हैं और इसी की हेल्प से
हमारा पूरा का पूरा जावा जो कोड है वह रन हो पाता है अब इन दोनों स्टेजेस को अगर हम समझे तो कंपाइलेशन की स्टेज में क्या होता
है जो भी कोड हमने लिखा है उसे हम कह देते हैं सोर्स कोड सोर्स कोड को हम डॉट जावा से सेव करते
हैं और ये सारा का सारा कोड एक कंपाइलर के पास जाता है ये जो कंपाइलर है ये जेडीके के अंदर होता है और इस कंपाइलर के पास
सारा का सारा सोर्स कोड जाता है और यह कंपाइलर इस सोर्स कोड को कन्वर्ट कर देता है बाइट कोड के अंदर जिसका एक्सटेंशन होता
है डॉट क्लास और यह जो वाइट कोड है यह किसी भी ऑपरेटिंग सिस्टम पर रन कर सकता है एज़ लॉन्ग एज़ उस ऑपरेटिंग सिस्टम के अंदर
भी जे आरई जावा रन टाइम एनवायरमेंट हो तो अगर आपने कोड यह चीज आप c+ के साथ नहीं कर सकते इसीलिए
जावा को एक पोर्टेबल लैंग्वेज कहा जाता है क्योंकि इसमें बनाई हुई एप्स को आप बाइट कोड में कन्वर्ट करके किसी भी सिस्टम के
ऊपर रन कर सकते हैं तो यह हमने पहली स्टेज कवर कर ली जो थी कंपाइलेशन की स्टेज अब अगर अपनी दूसरी स्टेज की बात करें जो है
एग्जीक्यूशन की स्टेज तो एग्जीक्यूशन की स्टेज में क्या होता है यह जो बाइट कोड हमारे पास
था अब जावा वर्चुअल मशीन इस बाइट कोड को लेती है और उसे नेटिव कोड में कन्वर्ट करती है नेटिव कोड यानी वो कोड जो मशीन को
समझ में आए अभी हमने बात की थी कि सबसे लो लेवल कोड जो मशीन को समझ में आता है वो आता है रो और वन का तो नेटिव कोड वैसा ही
कुछ कोड होता है जो मशीन को समझ में आता है अब ये बाइट कोड नेटिव कोड में जब कन्वर्ट हो गया तो चाहे आपकी मशीन विंडोज
हो या मैक हो अब उसे आपका जावा कोड समझ में आने लग जाएगा उसी तरीके से जैसे आपको हेलो वर्ल्ड प्रिंट करवाना था तो आपकी
मशीन में वो कोड कन्वर्ट होते-होते नेटिव कोड में कन्वर्ट हुआ और फिर आपके सिस्टम में आपको हेलो वर्ल्ड दिखने लग गया अब अगर
हम अपने कोड के कंपोनेंट्स की बात करें तो इसमें से सबसे पहला कंपोनेंट आता है फंक्शन फंक्शंस या मेथड्स कोड का वो पार्ट
होते हैं जिसको हम कुछ काम परफॉर्म करवाने के लिए बोलते हैं जैसे अगर हमें दो नंबर्स को ऐड करवाना था तो वो सारा का सारा कोड
हम एक फंक्शन के अंदर लिखते अगर हमें हेलो वर्ल्ड प्रिंट करवाना था तो उस सारे के सारे कोड को हमने एक फंक्शन के अंदर लिखा
और वो फंक्शन क्या था वो फंक्शन था यह वॉइड मेन नाम की चीज जहां पर फंक्शन का नाम था मेन और उसका रिटर्न टाइप था वॉइड
वॉइड यानी खाली यह फंक्शन कुछ रिटर्न नहीं करता इसने सिर्फ हमारे लिए हेलो वर्ल्ड को प्रिंट करवाया था तो जो भी कोड हमें लिखना
होता है जावा के अंदर वो हम फंक्शंस के अंदर लिखते हैं और इन फंक्शंस को हम क्लास के अंदर लिखते हैं तो क्लास एक बड़ी
एंटिटी है जिसके अंदर काफी सारे फंक्शंस आ सकते हैं क्लास को कैसे समझे जैसे हमारा एक बड़ा सा किचन होता है घर के अंदर
जिसमें बहुत सारे छोटे-छोटे डब्बे होते हैं जिसके अंदर कुछ-कुछ स्टोर्ड होता है जैसे किचन के अंदर एक डब्बा चीनी का है एक
डब्बा नमक का है वैसे ही जावा के अंदर बड़ी सी एक क्लास होती है जिसके अंदर छोटे-छोटे फंक्शंस होते हैं एक फंक्शन में
कुछ काम हो रहा है दूसरे फंक्शन में कुछ और काम हो रहा है एंड जावा की क्लासेस में जो मेन नाम का फंक्शन होता है वो पूरे के
पूरे कोड का पहला पार्ट होता है जो एग्जीक्यूट होता है तो जावा के अंदर जो मेन फंक्शन में पहली चीज लिखी है सबसे
पहले वो एग्जीक्यूट होगी बाद में सारी की सारी कोड की चीजें हमारे मेरे पास एग्जीक्यूट होकर आ रही होंगी तो आज की
क्लास में हमने काफी सारी चीजें समझने की कोशिश करी कि जैसे कोड क्या होता है कोडिंग क्या होती है मशीन को चीजें कैसे
समझ में आती है और हम अपने जावा कोड को एक्चुअली कैसे लिखते हैं आई होप कि नेक्स्ट क्लास से पहले आप अपना कोड एडिटर
यानी विजुअल स्टूडियो कोड या फिर जो भी एडिटर आपको पसंद है साथ के साथ जावा डेवलपमेंट किट को आप डाउनलोड कर लेंगे
ताकि आगे की क्लासेस में हम क्लास के साथ-साथ कोड को लिखकर देख सके रन करके देख सके कि हमें कोई प्रॉब्लम तो नहीं आ रही
या फिर सारे की सारी चीजें हम क्या अच्छे से सीख पा रहे हैं कर पा रहे हैं क्योंकि कोडिंग में प्रैक्टिस करना ज्यादा
इंपॉर्टेंट है तो हम जितना प्रैक्टिस करेंगे उतना हम कोड को और अच्छे से समझ पाएंगे आज के लिए इतना ही मिलते हैं
नेक्स्ट वीडियो में टिल देन कीप लर्निंग एंड कीप प्रैक्टिसिंग
Heads up!
This summary and transcript were automatically generated using AI with the Free YouTube Transcript Summary Tool by LunaNotes.
Generate a summary for freeRelated Summaries
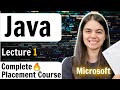
Java Programming Course: Introduction, Structure, and Setup Guide
Learn about Java programming fundamentals, data structures, and how to set up your coding environment.
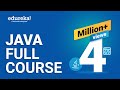
Java Programming: A Comprehensive Guide to Understanding Java and Its Concepts
Explore Java programming concepts including OOP, exception handling, and collections. Learn how to build robust applications!
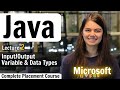
Java Basics: Outputs, Variables, and User Input Explained
Learn Java's fundamentals: how to give output, use variables, data types, and take user input effectively.
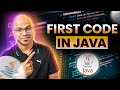
Getting Started with Java: Writing Your First Code in VS Code
In this tutorial, we explore how to set up Visual Studio Code for Java development and write your first Java program. We cover the basics of creating a project, using the terminal, and writing a simple 'Hello World' program.
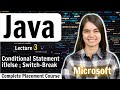
Mastering Java: Understanding Conditional Statements for Beginners
Dive into Java's conditional statements, including 'if', 'switch', and 'break', to enhance your programming skills.
Most Viewed Summaries
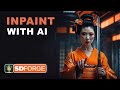
Mastering Inpainting with Stable Diffusion: Fix Mistakes and Enhance Your Images
Learn to fix mistakes and enhance images with Stable Diffusion's inpainting features effectively.
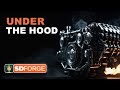
A Comprehensive Guide to Using Stable Diffusion Forge UI
Explore the Stable Diffusion Forge UI, customizable settings, models, and more to enhance your image generation experience.
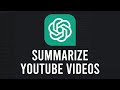
How to Use ChatGPT to Summarize YouTube Videos Efficiently
Learn how to summarize YouTube videos with ChatGPT in just a few simple steps.
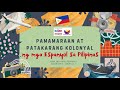
Pamaraan at Patakarang Kolonyal ng mga Espanyol sa Pilipinas
Tuklasin ang mga pamamaraan at patakarang kolonyal ng mga Espanyol sa Pilipinas at ang mga epekto nito sa mga Pilipino.
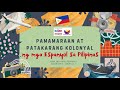
Pamamaraan at Patakarang Kolonyal ng mga Espanyol sa Pilipinas
Tuklasin ang mga pamamaraan at patakaran ng mga Espanyol sa Pilipinas, at ang epekto nito sa mga Pilipino.