Getting Started with Java: Writing Your First Code in VS Code
Introduction
In this tutorial, we explore how to set up Visual Studio Code for Java development and write your first Java program. We cover the basics of creating a project, using the terminal, and writing a simple 'Hello World' program.
Setting Up VS Code
- Open VS Code: Launch Visual Studio Code by typing
code
in your terminal. - Choose Theme: Select a color theme (dark mode is recommended for development). For more on customizing your development environment, check out our summary on Setting Up Your Development Environment.
- Explorer Panel: Familiarize yourself with the Explorer panel on the left side, where you can manage your project files.
Creating Your First Project
- Open Folder: Click on 'Open Folder' to create a new project folder on your desktop.
- Create Folder: Name the folder (e.g., 'course') and open it in VS Code.
- Create File: Create a new file named
Hello.java
. Remember, Java files must have a.java
extension.
Writing Your First Java Code
- Java Version Check: Use the terminal in VS Code to check your Java version by typing
java -version
andjavac -version
. If you're new to Java, you might want to read our guide on Java Basics. - Write Code: In
Hello.java
, write the following code:public class Hello { public static void main(String[] args) { System.out.println("Hello, World!"); } }
- Compile Code: Compile your code using the terminal command
javac Hello.java
. - Run Code: Execute your program with
java Hello
to see the output.
Using JShell for Quick Experiments
- JShell: Introduced in Java 9, JShell allows you to quickly test Java code snippets without creating a full program. You can type
jshell
in the terminal to start. For more on interactive coding, see our summary on Using JShell for Java. - Example: You can perform simple calculations or print statements directly in JShell.
Conclusion
This tutorial provided a foundational understanding of setting up Java in VS Code and writing your first program. In the next video, we will delve deeper into Java programming concepts and best practices. If you're interested in further learning, check out our summary on Advanced Java Programming Techniques.
FAQs
-
What is VS Code?
Visual Studio Code is a popular code editor that supports various programming languages, including Java. -
How do I install Java?
Download the Java Development Kit (JDK) from the official Oracle website and follow the installation instructions. -
What is JShell?
JShell is an interactive tool for quickly testing Java code snippets without the need for a full program. -
Why do I need to compile Java code?
Java is a compiled language, meaning you must compile your code into bytecode before running it on the Java Virtual Machine (JVM). -
What is the purpose of the
System.out.println
method?
This method is used to print text to the console in Java. -
Can I use other IDEs for Java development?
Yes, there are several IDEs available for Java development, including IntelliJ IDEA and Eclipse. -
What is the significance of the
.java
file extension?
The.java
extension indicates that the file contains Java source code.
now once we have our setup done it's time to write our first code that's right so finally we are going to run our
first Java code here now for that we have to open our editor so of course in the last video we have discussed that we
are going to use vs code so let's open vs code here so I will say code okay so our vs code is all set to write our
first code you can see it will give you a welcome screen when you do it and then it will ask you the preference do you
want a light color dark color uh dark high contrast or light high contrast I would say go for dark because it's good
for development it will launch train your eyes at least so let's close this get started and this is how your vs code
will look like okay simple stuff right now what we'll do is first of all uh where you will get your folder so you
will see your folders on the left hand side so this is where you will get your project all the files which you want to
work with so you'll be having that here now of course in the upcoming tutorials we'll be using a different IDE and I
want you to get used to different IDs when you work with it so on the left hand side we can see we have an Explorer
here and then we if you want to search something we have it here if you want to add some extensions which we are going
to do later uh we'll add certain extensions to work with at this point we don't need any extension so just keep it
blank I mean no need no need to install anything here in my machine I already have something on some other accounts
but on this account I don't think so anyway and then if you want to run and debug debug you will find the option
here now why do we run of course we know what to why to turn right but why do we debug the application yeah we'll see
that later at this point let's open our files of an Explorer here and this is where I want to create my first project
now how do we create a project now project will be a very broad term at this point what we are going to do is we
will create some files and of course when you merge these files and libraries you create a project at this point we
are not working with any external libraries at this point we are just going for a simple files okay hello
world right so for that what we'll do is we'll click on open folder because all this files will be part of one
particular folder so we'll click on open folder and it will give you certain options where you want to save this so I
will save this in on my desktop itself okay in fact on my desktop I want to create one more folder so I will say
open folder again inside desktop I would I want to create a folder here we'll say this is I will have a short name so it's
a course create and open so you can see we got our course folder here what I will do is I will zoom in inside my vs
code so that you can see everything properly and again the recommendation would be to watch this on a bigger
screen so that you know you can actually understand each and every line because on mobile sometime you will be seeing a
font size and a small font okay I don't want to welcome I don't want to see this page every time I open my vs code so I
will just uncheck this and click on close here and let's create our first file and this is where you are going to
write our first code right so we have already seen how do we install jdk how do we check the version on the terminal
can you do that in this so vs code itself as a terminal here so you don't have to open the external terminal of
course if you are using Windows you will be having an option of command prompt but let's click on Terminal here and you
can see we have an option of terminal click on this one okay this is where you can enter your
commands whatever commands you have the same command will write here so we'll say Java version and you can see I have
Java 17 in this machine and Java 18 is already released but then Java 17 is the LTS version so when you say LTS it means
long term support right so we'll use that and we'll also check if my Java C is working you can see javasa is also
there now Java C basically stands for Java compiler as we discussed before so we need both we need Java to run the
application we need Java C to compile the code right okay cool so we got the terminal as well I will just minimize
this a bit okay so now let's create our first file so what we are going to do is we'll click on this particular file here
and we'll name this as Hello dot Java now this is something you have to remember whenever you create a Java
project you have to make sure that you give an extension or Java files basically you have to give an extension
with DOT Java now different languages have different extensions when you talk about C we say dot C when you say C plus
plus language we say dot CPP when you say JavaScript we use dot JS in the same way in Java we say dot Java simple stuff
right so you got our file here now vs code will give you some suggestions will ask you to install some extensions for
Java and we'll click on recommendation okay it is asking us tension pack for Java which will help us it will give you
some options and extra stuff again this is just an advantage which you get from vs code again not required if you don't
want to install this extension I think I should go for it we also have intelli code for AI assistance but yeah we'll
stick to this extension again in your machine you don't have to install this there's no compulsion you can still
write your Java code but maybe it will give you some suggestions and stuff in between let's see let's close this and
let's write our code so extension will be installing behind this thing you don't have to worry about it so
basically how do we write our Java code it's very simple actually uh first of all the question is what you want to do
in this code do you want to create a game or do you want to to build a rocket which will go to mass they will not be
doing those extra stuff what I will do now is I will create a very simple file which will print hello world I know
that's how you start a programming language so it is irrespective of which language you're learning the first thing
you do is you print hello world so here I want to print hello world now how do we do that see uh if you in case if you
are coming from other languages let's say python right so what you do is if you want to print hello world in Python
you can write only one line and maybe Java is not that famous for Simplicity at the start of course when you build a
bigger project Java is much more structured compared to the languages but then to start with it's not that easy so
just bear with me okay so what we are going to do now is if you want to just experiment with Java if you want to play
some if you want to test some code or stuff we have an option which was introduced in Java 9. so basically you
can write your code in one line you can get the output example so even before we jump to hello world let me show you
something so so we have a we have a concept of JSL in Java so it was introduced in Java 9 as I mentioned so
you can open your terminal and here let me just increase the size a bit so what I will do is I will click on J shell or
I will type J shell and it will give you a j shell prompt okay now J in this case is Java so you can it make it should
make sense now okay so what you can do in JSL is if you want to experiment something example why do we write code
why do we build applications so to solve problems right now it can be a very simple problem as well example if you
want to add two numbers it's very simple you can say two plus three and if I say enter you can say we got five right so
we got the answer which is five what if you want to do a subtraction you can do that so 9 minus 6 and you can see we got
three so this is working but what if we want to print something so in this case what you can do is whenever in Java if
you want to do something we use something called Methods at this point you don't have to remember methods on
what the definition is just remember that there is a concept called Methods using which you can do something the
only thing you have to remember is if you want to do something you need methods in in the same way if you want
to do something here I want to print something right so we have to use a method called print it is a inbuilt
method in Java so you don't have to worry about it just use it it will work so whenever you call methods you have to
pass the values so I want to say hey print but then it will say okay I will print but what you want me to print so
you can mention that in this bracket so in this round brackets you can mention I want to print hello world now if you
want to just print a number let's start with that first so let me just print a number which is six and uh in Java
there's one more thing you have to put a semicolon at the end it should work in this but then remember this at the end
put a semicolon that's how you mentioned hey something is getting ended so we're going to semicolon there and now what
you can do is uh let's try to run this code now adults right adults are very important in your life in your program
in life basically you still got there it says cannot find a simple call point it's not working okay cool no problem so
let me just clear the screen now clearcom is not working ignore that you will say so let me open jayshaw once
again and let's because I just want to keep it on top so what I will do now is if I want to print something and I want
to print a value 6 at the end I want a semicolon as well now Java says if you want to call print print belongs to
something right so it when I say it is available inside Java so the statement you have to always mention is system dot
out dot println see there will be a point where you I will talk about what is system what is out at this point it's
very difficult to understand so just bear with me this is a statement which you have to re which you have to use if
you want to print something on the console okay so if I want to print something here just say system.out.print
and whatever value you want to pass say enter you can say we got 6. but what if you want to print hello world I don't
want to print six so if you want to repeat the same statement of course you can type or you can click on the up
Arrow it will give you the option now here if you want to print hello world you have to put that in double quotes so
whenever you want to do something with text see numbers are easy to use you can simply say six but if you want to work
with a text you have to put that in double quotes the way I've done it here so in this double quotes you have to
mention hello world right that's how you start a programming programming Journey right I will say enter and you can say
we got hello world what if I want to print something else which is very simple you can say double quotes not
been ready the Disco and if I say enter you can see we got the text so whatever you want to print just put that in
double quotes and it should work and you can see we are getting the output as well so cool so we are able to do this
with the help of J shell but will it work in this if I try to copy this in fact why to copy when we can type it so
let's type system dot out dot print Allen and here we'll say hello world and let's give a
semicolon at the end it was working on J shell but when you work with Java files because this is how you build a project
right Json is not to build projects it's just for the experiment if you are learning a language which is good but if
you want to make it work you can do if you want to build a big application say dot Java file and write your code there
so let's compile this code now how do we compile okay so there are two steps you have to follow first when you write a
code you have to compile the code and then you can run the code so to compile the code you can say Java C in the
terminal and let me just increase the font a bit so you have to say Java C and then you have to mention the file name
so first you have to be make sure that you are in a correct folder which is course you can see that this is the
folder we have and then you have to mention Java C and then the file name the file name in this case is hello.java
click on enter oh we got an error okay so it says something is missing you know something else was expected and you are
giving this is not working so this is not how you write applications or code in Java file we have to do something
else but at this point we have understood right how do you work with jshl so you can if you want to do
something with JSL just write a code it will work if you want to add two numbers it will work you can't do that here you
have to do something extra and what is that something that will say in the next video
Heads up!
This summary and transcript were automatically generated using AI with the Free YouTube Transcript Summary Tool by LunaNotes.
Generate a summary for freeRelated Summaries
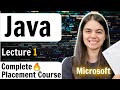
Java Course Introduction: Mastering Coding Fundamentals and Data Structures
Kickstart your Java programming journey with our guided course covering basics to algorithms for aspiring developers.
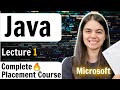
Java Programming Course: Introduction, Structure, and Setup Guide
Learn about Java programming fundamentals, data structures, and how to set up your coding environment.
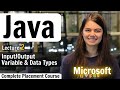
Java Basics: Outputs, Variables, and User Input Explained
Learn Java's fundamentals: how to give output, use variables, data types, and take user input effectively.
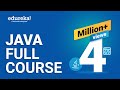
Java Programming: A Comprehensive Guide to Understanding Java and Its Concepts
Explore Java programming concepts including OOP, exception handling, and collections. Learn how to build robust applications!
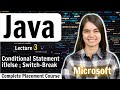
Mastering Java: Understanding Conditional Statements for Beginners
Dive into Java's conditional statements, including 'if', 'switch', and 'break', to enhance your programming skills.
Most Viewed Summaries
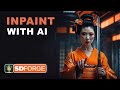
Mastering Inpainting with Stable Diffusion: Fix Mistakes and Enhance Your Images
Learn to fix mistakes and enhance images with Stable Diffusion's inpainting features effectively.
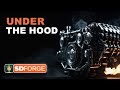
A Comprehensive Guide to Using Stable Diffusion Forge UI
Explore the Stable Diffusion Forge UI, customizable settings, models, and more to enhance your image generation experience.
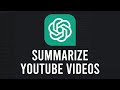
How to Use ChatGPT to Summarize YouTube Videos Efficiently
Learn how to summarize YouTube videos with ChatGPT in just a few simple steps.
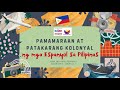
Pamaraan at Patakarang Kolonyal ng mga Espanyol sa Pilipinas
Tuklasin ang mga pamamaraan at patakarang kolonyal ng mga Espanyol sa Pilipinas at ang mga epekto nito sa mga Pilipino.
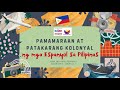
Pamamaraan at Patakarang Kolonyal ng mga Espanyol sa Pilipinas
Tuklasin ang mga pamamaraan at patakaran ng mga Espanyol sa Pilipinas, at ang epekto nito sa mga Pilipino.