Overview of the Boot Camp
In this boot camp, we will be building a live score application using React and Tailwind CSS. The session will guide participants through the essential concepts of React, including:
Key Topics Covered
- Introduction to React: Understanding what React is and its significance in web development. For those interested in expanding their knowledge of modern frontend development, check out our guide on Build a Modern Frontend Developer Portfolio Using Next.js, Tailwind CSS, and Sentry.
- JSX: Learning about JSX, the syntax extension for JavaScript that allows mixing HTML with JavaScript.
- Components: Exploring functional components and their role in React applications. If you're looking to implement your own design system, consider reading about Implementing Your Own Design System in Next.js.
- Props: Understanding how to pass data between components using props.
- State Management: Introduction to the useState hook for managing state in functional components.
- Conditional Rendering: Implementing if-else statements in JSX for dynamic rendering.
- Mapping Data: Using the map function to render lists of data efficiently.
Practical Coding Examples
Participants will engage in hands-on coding, including:
- Writing JSX code and understanding its structure.
- Creating functional components and passing props.
- Managing state with the useState hook.
- Implementing conditional rendering and mapping through arrays.
Installation Guidance
The session will also cover how to set up a React application locally, including:
- Verifying npm installation.
- Creating a new React app using npm commands.
- Installing necessary dependencies like Tailwind CSS.
Conclusion
By the end of this boot camp, participants will have a solid understanding of React fundamentals and be equipped to build their own live score application. For those interested in exploring other frameworks, you might find our summary on Getting Started with Svelte: The Ultimate Beginner's Guide helpful.
FAQs
-
What is React?
React is a JavaScript library for building user interfaces, particularly single-page applications. -
What is JSX?
JSX is a syntax extension for JavaScript that allows you to write HTML-like code within JavaScript. -
What are components in React?
Components are reusable pieces of code that return a React element to be rendered on the page. -
How do props work in React?
Props are used to pass data from one component to another, allowing for dynamic rendering. -
What is state management in React?
State management refers to how data is stored and managed within a React application, often using the useState hook. -
How do I create a new React application?
You can create a new React application using the commandnpx create-react-app my-app
in your terminal. -
What is Tailwind CSS?
Tailwind CSS is a utility-first CSS framework that allows for rapid UI development. To see a practical application of Tailwind CSS, check out our summary on Building the Ultimate Auto Space Parking Application. -
What is Headless UI?
Headless UI refers to a set of completely unstyled, fully accessible UI components designed to integrate beautifully with Tailwind CSS. Learn more in our summary on Understanding Headless, Boneless, and Skinless UI in Modern Development.
right now uh is my audio clear audio video everything uh it's all clear right just respond in the chat
yes yes okay great great okay uh so let's begin this uh session so okay uh so in this boot camp we will be building
one live uh score application okay so uh we will be building that by using a react uh javascript and that with
tailwind also we'll be using and we'll be fetching those score by using an API okay we will get into the description of
all this i will go on I will start with explaining you what all things we will be implementing first of all what things
we will be doing where we will be doing and how we will be doing okay everything will be explained no problem with that
okay so uh just respond in the chat if you all ready to go with this boot camp you all are excited for
this okay uh so first uh let me get into what all things we will be uh getting in this boot camp okay so first of all uh
we will be starting with first thing uh that is getting to understand what is reactors
okay just a Yep my code is visible now yeah so we will be start with uh getting to know what exactly is ReactJS
so this session will clearly be about ReactJS what is ReactJS and then what is uh JSX why we call it as JSX what are
things we have in JSX and then we willh get to know about different things in ReactJS such as what is prop okay before
prompt we will get to know what are functional components okay so one question remains same why do we use
React so much okay so for understanding that we have to understand what is functional component after we are done
with functional component we will move to props okay or else before that we can move to writing a code sample of in
JSX how we write a code in JSX we can do that uh then we can move to our props and then likewise we have many
different topics that we cover today okay uh don't worry i will also tell you how to install React okay in your local
system no problem with that how to create an application in React i will show you a terminal example of that okay
so installing uh React then CD then uh installing your dependencies in React and everything so uh we will do that too
text variable let me increase the weight for the text okay so let's begin with
understanding reactions okay so uh before what we had was we had normal JavaScript okay so before we had uh
normal JavaScript might be my handwriting would not be that great but uh if you do not understand any word or
anything just feel free to tell me and I will explain you so uh in normal JS what we can write is
just the script okay if you want to embed an HTML it was quite difficult in that so embedding u HTML and other
things was quite difficult in that so now to make our job easier developers were spending lot of time in doing so in
writing HTML different file and writing JavaScript and different files so now what we got is we got combination of
code so we got HTML plus of JavaScript into a single file that is
popularly known as JSX or else we can also use JS also in case of J okay jsx and JSX jsx and JS both will work
okay yeah okay so now what I told us we can have both HTML and uh JavaScript in a
single file so for that also we have rules and regulations so first let's get into JSX so I will show you a code
sample of how to write a JSX but uh like yeah so writing a JSX uh mostly yeah one more thing is uh before starting it we
will be implementing this all project complete project in code sandbox so that uh we can uh share the code with you can
also do in the local system but it will be quite difficult to share codes with you uh so if you want to do in code
sandbox or else uh if you want to do it in local system in VS code or whatever it's your will okay it's not an issue
but only thing that I require from you is your intent to do this project okay along with me you have to go on with the
flow if you do not understand anything and while I am explaining you can clearly come uh in the chat and uh tell
me that yeah I'm not understanding that so uh we can uh clearly get back to that talk
yeah okay so uh let's get into JSX what exactly is JSX so as I explained earlier JSX is the combination of your
HTML and JavaScript jsx files is the combination of your HTML plus of JavaScript so in this uh
JSX files you can clearly write both of the HTML and JavaScript collectively you do not have to create another script tag
and you do not have to write call JS in that different script type okay so if I take one example
of JSX so let me show you an example yeah so we will be typing this no worries i'm explaining you the
theoretical part right now so that's why I'm showing you directly we will be absolutely typing this with no problem
okay so see uh this is one element one JavaScript element cost element what is cost what is let we will also refer that
no need to worry about that if you do not understand it now just after this after I explain the component I will
also explain just all things so con element H1 hello name now the thing to uh focus upon here is we have two
different components okay two different components what are the two different components we have H1 tag you can see we
have H1 tag so this H1 tag is clearly our HTML it's clearly our HTML and then con
element and the name so over here we would also have defined con name as something it might be a string value we
would have defined con name as something and we are printing that name from that variable over here so we have JavaScript
this is your JavaScript and your HTML so when we combine them both what we get is JSX that's what JSX is clearly about so
it is the lowest level of JSX that we can have okay so when we write a whole lot of whole lot of code in this uh boot
camp for the IPL project we will be seeing JavaScript HTML all everything in the same folder along with different
dependencies like XOS for and then API also we will be using I will explain you what all API we'll be using just in the
session itself okay now I explained you what JSX is now you would have got a doubt like what is const and what are
different things is we'll just get a little into that also so we have three types of u data types in JavaScript one
is cost another one is let and third one is okay so as per the name given cost okay so the cost will be a constant
value constant value so if you define a variable with con data type its value
would not be able to change in any future reference so suppose I define uh con a as 10 and then uh later in the
program later in the code I am trying to do is b is equals to 20 so absolutely you cannot do this why because we are
defining it with a cost okay now to remove this problem we have our let or where okay let or when so in case of let
suppose we are defining a is equals to 10 and in case of where also we are defining b is equals to
10 and then again in future we define a as 20 no problem with that we can get it we define b as 20 no problem with that
we can clearly get it we can update this there's no issue because it is not a cost it is let and where only difference
between let and where is one is a scope variable and one is not a scoped variable so what scope is so suppose we
have one bracket over here this is one scope so if you define let in a scope it will only be available in that scope but
where will be available in all scopes okay so this was just the basic of data types now let's get to components what
exactly is forming okay uh so let me show you uh an example if I can get a site
example yeah let's get out to the code sandbox example itself okay so yeah so you can see I think so you can see my
window okay so in this what we have is uh we have different three three four different
components okay so what are three to four different components is uh we can we have one sidebar over here so this
shows your folders and files all available in it you have one code section over here okay so this is your
code section that we have and then we have our output section over here okay so now we have three different sections
in a single page we can say if you consider we also have a header over here and sometimes we also have footers over
here so let's assume we have three three parts in this uh window one is our sidebar one is main coding window one is
a output window okay let's get to here so what are three parts we have one is our
sidebar and one is our coding window okay and the third one is our output window
so if I ask you it's my question to you if we want to make a web page similar web page as code sandbox by using just
HTML and CSS what do you think this web page would be like like will will we do that
all things in a single file or else we will be doing that in multiple file if you could answer me if we will do this
all code of all three components right coding and output in single file or else we will be doing
in multiple files okay yeah just respond in the chat uh
single file or multiple file single file yeah great uh no friends we will not be doing that in
multiple file because it is plain HTML and CSS i'm not talking about React okay if I talk about React in that case you
will be doing it in multiple files and multiple components okay so in in case of HTML you will be doing that in case
of single file no multiple files would be required okay like we can't do it in multiple sites so now here comes the uh
here comes the advantage of your ter okay here comes the advantage of your reacts so what react will do it
will break this all three parts into different components different components okay we
will have all three all three of this part into different components and we will create all these component
and component like we will have one sidebar component we will have one coding component we'll have one output
component just after this I will show you one example of a code sandbox so we'll create three components we'll add
some random text in that i will show you how we create all three components okay so once we create all three components
we combine them all into single file in app.js or some files and we view it as a single web page so what's better now is
your code readability your code readability gets better okay your code uh readability gets better as well as
your management of code gets better okay so it's like uh if we combine all three components in a single file so we would
be having around 2,000 and 3,000 lines of code in case of simple HTML and but if if we take ReactJS in that cases we
will divide it into three different components in that in that case uh the coding will be easier obviously
debugging part will also be easier okay so let's write what all advantages we have so we have easier
coding absolutely our job will be much more easier we have debugging okay debugging which will also
be easier and once debugging porting is easier we will have like this port this
two part is most important other like others uh we have other advantage too but um these are the main two advantages
okay so we divide uh this code into three different components so let's see in the code sandbox how we can do that
is so see uh this is how to set up this and all we'll be explaining no need to worry about this once we start the main
project uh we will get down into this too so in uh source directory I will be making a file known as text dot jsx okay
we have one file text jsx in that yeah yeah so let's begin with component
function test right now one more thing to take care of is whenever you are in a JSX
file so whenever you want to uh have an HTML okay whenever you whenever you want to have an HTML every time we add that
HTML under a return tag so we have return small bracket then we can go on now one more thing that we have is this
is this can also be used in case of HTML so the thing is inside a return tag we can just have one single tag okay one
single tag so it's like yeah so suppose we have defined a return tag uh return we have defined a
return in JS6 we have written so uh suppose we are writing H1 tag over here okay H1 tag and then again we are
writing one more H1 tag over here so in this case what will happen is we will have a issue issue will be we will have
get an error so to avoid this error what we do is either Either we have two solutions to this
either we can use a div tag that will contain all the code inside it okay we can use this or else what we can do is
we can have this tag so once you have this tag you can write whatever code inside it
not outside or other than this you can write inside it so how many tags you want to define inside it you can no
problem with that but remember multiple tags defining inside a return is not a good thing you will get a clear sort of
error so we are done with that and we are missing one part that is our small bracket we are done with the small
bracket and yeah ready to go now let's define our H1 okay hello we are making
our component see uh as for like I am telling you basics if I am starting some
code uh so I I suppose that you are also going on you are also coding with me because it will give you a more clear
understanding okay so we are not adding any CSS right now we are just uh Laura Mson just random things
okay so this is one component we made test.jsx let's make another component that is test
1.jsx okay yeah uh so one more thing that we are missing out is every time you create
a react component do not uh forget to write export default function okay so either you can write export default
function over here or else at the end you can do export default test okay so both of things are valid so do either of
one not both either of one so at the end either you can export uh the component or else you can do it at the starting
itself when you are defining the function yeah right so let's get to test
two default function test one we are ready with the function let's return it
up okay we are ready and again H1 tag we use H1 tag here we will be writing uh this
is test one of the window and then we will be having a paragraph tag and here we'll write some
random thing this is okay now we have two components uh let's test it out let's see how this is
looking so we already have a code in our app dot.jsx we'll remove that up or else uh yeah we'll remove that I
do not want to create confusion for you so first of all before importing it into app.t JS6 we will do
import so import test
from so we have test over here and we are done then we'll be importing our second test test one
from then test one okay now as I told you in the return we will be having just one tag having all the codes inside so
in before like in test and test we use this tag right now in app dot js what we are using is div so this both will work
fine no issue with that now let's import this up test and close it
then again test and then close it so we messed this
up yeah okay now you can see what we did is we created two different components one was test so in test we had hello we
are making our component first thing and Laura and in test two we had uh 1 minute yeah so yeah we'll discuss this
also why this is happening so in test one what we had is this is test one of the component and this is paragraph so
now let's see output so the output what's the problem in the output we are having the same component getting
returned twice okay why this is happening because we imported test okay and while writing over here we just
wrote test and test two times so that's why it's happening if we write test one over here and then
our our output will change okay so we wrote test in test one we wrote hello we are making our components and option in
test one this is test one of the component and this is par graph okay are you getting this like uh did you got a
small intro of what components is okay yeah great i know I know like many of you
would be knowing what all components are but we are starting from basic so let's not ignore some of the basic parts or
like from the first lecture we will just cover somewhat of the basic part and then in the next from next class we will
start building our application so so that you do not miss out some basic concept okay so if you know about these
all concepts it's great no problem with that just okay any doubts on this
part no right okay uh so next thing uh that we'll be starting with is props
okay okay so next thing is our props so what props is used for so props is used for passing values between your
functions passing values between function okay so uh
suppose that you have two functions two different functions in two different files okay so now what happens is you
want to pass a value you want to pass a particular value from one function or else from one part to another part okay
one part to another part suppose it is part two so this both are in different files suppose this is file 1
jsx and this is file 2.jsx so what we are doing is we are
sending one variable value from file 1.jsx to file 2.jsx jsx so to do so what we use is props okay so for this we are
using props so uh let's uh see one code example how we can implement this is
just get back to the code and watch so uh in text what we'll be doing is we'll be defining one as
name hello we are making our component and then we can use name over here we write here so what we did is uh
directly while we were defining the function while we were writing export default function test we directly add
one variable name over here inside a curly braces so the syntax is very important if you directly write
name just a minute yeah if you directly uh write name so in that case it will not work
well uh just give me a moment yeah so what I was saying is if you directly write name so it might be
work or not like sometimes you might get an issue but it also works without the curly braces no issue with that we'll
also see that in our project so right now let's work with a work with inside the curly braces
now while we call this so over here we will be writing name is equals to uh let's suppose I'm uh writing name as
runoff okay writing name as runoff let's save it up let it load
again text main we have some visual just wait a minute it's opening Just give me a moment uh I think so the
server is slow yeah so what exactly will happen is uh in the output you will be getting hello we are making a component
and with some space you will be getting the name that we pass from this component so what we did is we uh we
created one uh variable name as name and then we passed that name from app dot.js js6 to test.js okay so these are both
different files or as different components so we are passing it up from one component to another component okay
so that is what the use of props is okay is everything fine like is the screen zoomed in or something
okay uh yeah it's still working like it is still working so let's get to the next topic once it's back output is back
uh see it's going soon it's Yeah are you able to see it now clear like I have the font and all close
down this terminal is that fine okay then uh so process done then we
will be uh seeing next thing that is our use state okay so now use state
and use effect like we have two important things so first of all main important thing is use effect okay so
let's get into understanding what use effect is so now suppose that you have a one
component okay you have one window so in that window you are having one button okay you have one button so when you
click that button what happens is the background color we will write it as PG background color changes from
white to the background color changes from white to blue white to blue now this is what we call it as change of
state change of state so change of state is kind of change in some property of your application okay so suppose you
would have used many applications where you have dark mode and light mode combined okay so there are many
applications or web pages where if you want to switch from light mode to dark you can clearly do that so you just have
one toggle button or else one thing once you click that your all of the sides theme get changed to dark mode or else
white mode so that is also a function of state so state is clearly a property okay so a change of property is called
as change of state it might be change of background it might be change of text it might be change of your routes like we
have many things inside a state so we'll get to know about this once we are coding in our project okay so what is
state and uh how we change the state we will get to know about that okay right now just understand what state is state
is the change of one property so suppose that what happening what's happening is you have a sign in that side uh you have
a text being displayed so that text says hello okay so that text says hello and you have one button downloads so that
button says proceed so when user comes in comes on your site or this is a web page when
user comes uh on your uh site so in that case you will be showing hello to him or her okay so after he he or she clicks on
the proceed button this text should be changed obviously this should be changed it would either proceed to sign up
button or else signup page or another thing so to render that or else to show that we use a use state in that case
also again I'm telling change of a property or other things inside a web page is what we are we call it as change
of state Okay yeah yeah vic prompt is clearly passing off your value from one
component to other okay whenever you want to pass a value from one component to another in that case we use a uh
property okay i showed you how to define a prop okay but let me not yeah so as I show showed you the
example in text JSS what we did is we created a variable here inside the curly braces name if we want to return
multiple props too we can do that too i will show you just an example for that or we can write name and text and over
here too we can have name can have text that will
focus text hello okay we can also do this what we need to do one more thing is
import yeah uh yeah we'll see that later like importing different things we'll see that later let's get to the basic
thing right now okay yeah so what state is state is change in some properties is what we have
state yeah let's get to see the state thing okay now uh I will show you a code okay the basic uh right implementation
also we will see where I will show you a small code example so let's make
one file so file will be state jsx
yeah export default function we can write it as
state okay now before uh using the state what we have to do is we have to import one
thing that is use state from react without that uh our code will not be running so import in bracket we'll be
writing use state from react okay so if you are not importing user state from react in that case the user state will
not be working okay yeah so uh let's again uh what we'll do is we'll create an increment
counter uh example okay so in that uh let's define counter and set counter now we have
post counter comma set counter is equals to use state okay we are defining the counter right now as
default as zero okay right now it's zero it does not has any value so next will be our HTML component
in that we will be adding one button so what that button will do is whenever we click that button our counter will go on
increasing so let's write return this one just let's use a div it will be much better yep so paragraph
tag count and in braces count and then we will be having a
button now the function of this button will just be to increase the counter okay so let's write
agreement increment and then button on click now on click is a
property okay so whenever we click that button in that case yeah in that case uh we will be
using okay uh so on click uh we have to increase the count every time whenever
we click this button uh by one so we using our arrow tag yeah then we will not be now using
count uh counter we'll be using set counter because what happens is you have defined counter counter is a variable
that stores the value and the set counter will be used for updating this values okay so you will not be using
counter as uh in that case you have to use set counter inside that we'll be using count so let me show you that we
will be using set counter then small braces and then it will be count + 1
okay we have written the code what what's happening is first of all we are importing the user state from the react
after that what we are doing is we are defining counter and set counter so by default its value is set to be zero okay
once we are done with that what we are doing is we are showing it up and on click whenever we click that button we
will be updating it up by one every time you click it will it will get updated by one so let's remove this test thing and
we'll see the import yeah uh we have used the state as
a name okay so round then we are closing it
up okay so let's see yeah we can see uh output right now we are having our count as zero okay clearly we are having our
count as zero why because we have set it as zero suppose if we set it at 20 if we set it at 20 then by default we will
have 20 over here so no need to start with 20 we will start with zero and on clicked whenever we click on this button
we will increment it one by one see I clicked it up it's not getting updated uh let me see what's the issue
still it's not getting updated so what's the problem can anyone tell me what is the error what is the problem that I
that I made in this code if anyone can tell me it will be great help for me like I know what's the error but I want
you to debug and tell me what's the error I made so the for easing your task I will tell you the error is in button
tag if anyone could tell me in the chat what is the error yeah guys first tell me tell
me so count is not initialized no count is initialized on click is put no problem anyone else what's the
error set counter counter counter count three great what we did is we wrote count we wrote count instead of counter
what we defined was counter but not count suppose I would have defined count over here in that case it would be
working but we define counter so here also we have to write counter not count okay so let's get done with it refresh
our site now as we are clicking the increment ment it's it goes on
increasing 5 6 7 8 9 and whatever times to 1 okay so suppose that uh like out of curiosity you also want to add one more
thing that is uh same button one more button now it will what
it will do is it will set your counter to zero so again on click okay we are doing that
same okay set counter and then bracket it will be zero what we are doing is we are setting
up our counter in first case we are incrementing it by one and in the second case we are setting it as zero so if I
click on this it will automatically set your counter as zero okay it will automatically set your counter as
zero so same increment will work like that and once we set zero it will get back to zero okay so this was use state
thing okay so this comes under hooks so hooks is same like changing states changing effect and
all so if you have any doubt on this use effect thing uh just get in the chat write if you have anything that you did
not understand in this part if if if everything is clear then uh we can move to next
thing counter plus yeah you can write counter++ no issue with that let me show you
that we figure out counter++ also it's working same just counter ++ okay uh I don't think so
it will work that well uh for else we can do is counter = to one let's see if this will work
now this will also not work so like clearly we have to do counter plus one only counter ++ is not working see if
you get any doubts if you get anything out of curiosity just check it okay do not wait for anything do not wait for
any validation just go on just add it add it in the code and check it okay like why is not working and different
things just add it to the code and check it yeah uh so next thing uh that we have is yeah so uh we explained what is
component what is prop and uh then what is our use effect uh use state okay so uh next thing that we can go on with is
conditional printing like if else statement also so yeah let me show that example also so
if you would have studied JavaScript okay normal plain JavaScript in that what we have is if else statements if
else conditional statements okay if else conditional statements so in that if a condition is true in that
case we will execute that code okay so same way in case of JSX what we can have conditional rending
conditional rendering so what does uh this conditional uh rendering does is if the
condition is true in that case only it will show you the HTML code or else it will show you the HTML code on the web
page if the condition is not true if the condition is false in that case uh that code is not going to be shown okay so
what we have is conditional ending conditional ending if the statement is true in that case we will be having our
uh our HTML to be shown but in case uh it's false we will not be showing it on the string okay so if we uh get into the
example I will directly show you the code u we will not type that in because it's a easy part so in the state itself
uh let's uh see that code yeah so uh this is the code before that we have to define one more thing
is con this log n is equals
to okay so we are defining this up now this will be the code now we are getting some errors over here let's see
what's the error obviously we will not be showing it like that as we're talking to
social yeah it's fine now okay so what what was the error that we had was we are not adding this inside
a div okay so what happens is is logged in so we defined is logged in so if the is loud then thing is true so here we
are using a turnary operator okay yeah so here we are doing a turnary operator so if the condition is true
like if the condition in the code is true that is is logged in so if it is true in that case we will be showing
welcome or else if it is false in that case we will be showing showing please log so this is your conditional ending
you can also use the if thing if you want so we are using turnary in this case
okay so suppose what we do is uh we do this as false what we do is we set it as false in that case what
will happen is we are having our second text being displayed that is please log in okay in that case we are having
second thing uh as being displayed yes please log in okay everyone got this conditional
ending any issue spelling mistake uh it was spelling mistake i corrected it no
problem older in place of count it's done uh thought you were too late to answer this
++ counter someone is telling me to try plus+ counter let me show you if plus+ counter will work or
not yeah everyone is done with this code right uh I can remove this code right or else we will add one more file that and
we will see if the plus+ counter is working or not so counter dot jsxs see once we are done with this I
will tell you why this all things are not working plus plus+ counter or counter ++ let me directly add in the
more yeah okay this is the code so someone is telling me to try plus counter let's try
++ counter add in app.jsx we'll be importing this
import we have imported counter so we'll just update it counter okay
i think so there is some error counter JSX fine use state everything is fine set
comment with the counter Set the user state set count
++ counter JSX working with the states
dat yeah uh what's the issue here okay yeah so the error that we were
making was we were not exporting the function that's why we were having this error so blank screen
was due to this problem okay so once we are done with the export default function counter we are done with this
return p counter ++ increment and we are importing this counter show
that okay yeah we see yeah so it's not just me after coding for such long time also I
sometime forget this export default so it might happen to you Okay so it's not a like very common mistake but it
happens so export default function if you're not writing that before define the function or else if you're not
writing at the end in that case you will have a blank screen now see we did increment plus counter okay now in case
of other languages like Java and all if if you define in case of Java if we take an example in that case if you define
int count in counter and then we do ++ counter or counter++ in that case it will get updated but in JS what happens
is we are not working with normal we are not working with normal variables we are working with use state okay so in case
of use state the plus+ counter thing and counter++ thing is not going to work okay are you getting me that the plus+
counter and counter++ is not going to work this thing just temporarily updates the value of counter but it is not
getting saved to the user state so that is why this will not work neither plus+ counter nor counter ++ so we just have
to use counter + one okay only this thing will be working in case of use state we not talking about normal
variables we are talking about use state link okay yeah you can use no problem with
the RFC we are starting in the beginning i know that we can use RFC we are starting with the beginning so that's
why I want to explain you each and every concept each and every part of the code okay so you do not get confused i know
many of you know what to use what not to use great but I do not want to confuse anyone who's starting from basics okay
yeah so we did the use state thing and we did the conditional rending
okay so next thing that we'll be uh using is map map function or is loop okay so you would know what loop is what
exactly is loop okay so loop is just a circulation or else you go on
moving inside a code block until or unless the condition is true so famously like we have for loop in JavaScript also
we have while loop and then do while okay so we have this all three loops but this will work fine in normal JS it
works great other than that what we have is we have map we have one thing that is known as map and for
each both are same both have same functionality they they differ somewhat but most probably we will be using map
and for each we will not be using for while and do while while we are writing JSX ports okay so just map and for each
will be used so let's get into what is map so we will be rightly writing one code for map and we'll see how things
are getting rendered in that case so uh just let's make a map dot jsx
okay so if you want you can do rcs no problem uh in some cases in board sandbox it
might work or not it's changing me yeah I don't think so it's working in code sandbox so let's
go with the normal export called function
map okay so we done with the function and now we have to create one array so you would be knowing what array is okay
so we will take one sample array i will directly take it up so let's make a
con so this will be your uh list of elements so list of elements like
[Music] quotes okay we took a list like we took an array now what we have to do is we
have to show this all three elements of the array by using map okay so one way is by defining list item for each
elements list item for apple list item banana list item cherry so this good but if you have a large list of items that
you want to show so in that case what you have to do is you have to use map okay so let's begin
our HTML code return can add this let's use div itself is here and then we'll define
unordered list inside the unordered list we'll be defining our map okay list now a r dot
map r dot map then inside map we will be having uh two things yeah one will be the variable
that will go on at each element so suppose we are defining item we defining a item variable now what will happen is
item variable will start with apple first then it will move to banana and then it will move to grapes along with
item what we have is we have one more thing that is known as your index so index is your unique number or is the
index of the element inside that array okay so once we are done with this yeah so this arrow we will be
having this arrow and then the small braces we will have the small braces and then we will have our list items so
every time like uh before when we were using maps we would not be required with key like it's required but it was not
that mandatory but now we have key so every time every element in map will have a key and that key will be set to
index okay so key will be set to index and then you can write item item inside your curly
braces okay so what we did is we created an array of fruits inside an array of fruits what we did is we created an
ordered list and then uh for an ordered list by using our map what we are doing is we are showing each of the fruits one
by one okay let's show it on the screen and let's import our
val so map okay yeah so in the output window you
can clearly see what's happening is we are having our anord list of apple banana and grapes so the map is mostly
used for fetching all the elements inside an array or as object okay why uh one by one so if you have a large values
large number of values more number of values in that case map will be very helpful for you so in practical
applications what we will be doing is uh we will be having a large list of JSON files large objects and by using map uh
we will be showing all the items inside the list okay so it has a very great application map and for each has a very
great application so let me give you one application example okay you might need one so what happens is suppose you would
have visited some sites where we have many number of cards okay suppose we have six number of
cards and this six number of cards has same information like this has image then title description image title
description image title description and same with all this detail so suppose if you are uh writing code for each cards
so you have to write six code blocks six code blocks and it will be taking a lot of time for you and lot of space so now
what we can do is we can add the detail of all this in a JSON object JSON object or JavaScript
object okay so after adding this in JSON object you can use map map function how to do this we will clearly see it in the
project because we have that application of printing cards and by using map you have to just write uh one block of code
around 6 7 8 lines of code and then you can show all of this six card at once so instead of writing six different blocks
of cardboards you have to just write one block of code okay so by doing so you can like save your time you can save
your effort let's run both and for each will be used for this okay okay as you can see like we are
returning the HTML itself so we can clearly clearly use this for return
HTML okay any doubts to this point great there's nothing here okay uh so this was all basic basic
things that we uh what we got to know in uh JavaScript more we will be getting to know while we code in the project so
next thing that I want to show you is how to create uh react application if you want to do so in your own system
okay so uh we will do that in our normal terminal window okay just give me a second i will show you how to do that in
terminal so I'm just doing so that you will create a application in your own window and you can practice it up
okay so I'm starting with a command prompt i have opened my cmd just a minute i will share my screen of the cmd
so we share also you can see my CMD screen right great uh so uh what we will be
doing is uh we'll be writing our npm command so the thing is before starting this I want you to verify if your npm is
installed okay so you should verify that if your npm and react other things are installed after verifying this only you
have to start with this so let's begin with writing uh the command from creating our input
so it will be npm let's I will uh actually for data and show you all different formats okay
now yeah so what we have is npm create vit at so we are also using uh with vit okay so normally you can also create a
react without vit but we use it for easier easiness okay so npm uh node package module so before starting this
you should have your react just installed you should have your npm installed okay so uh just check it out
if it is installed or not if what you can do is uh you can directly write npm dash version okay so if you get the
version number in that case it is installed if you're not getting it so it means it is not installed and you have
to install it up so I will show you how to check it up just after this okay so what we can uh do
is yeah you can do it on VS code or else in terminal whatever place you want okay because VS code has inbuilt terminals so
it's not an issue yeah so npm create uh at the rate latest now this is a normal command after this comes your name of
the application name of the application so before uh we had just npm create name of the application and react
app so before this but it was depreciated so we have to use it uh now like npm create vit at the rate latest
iore app and uh dash whatever it is template and react okay and remember one thing you should not have a capital
letters in this name of your applications or else it will throw an error suppose if I show
exact course it should throw and error goes to the package so yeah so what's happening
is I have used a capital I so that's why what happening is we are getting an error of using small i okay so now we
will get back to small i we will enter and we will yeah so it's done it's installed now next step is doing cd cd
is your change directory we are changing our directory to IPL score app okay we have got into IPL
score app just to cd space the name of your application or the folder or directory
okay yeah now uh after doing that we will be doing npm
install so you can either write npm space i or else npm install directly so we are going with npm let's do that and
it will install all the dependencies okay it will take uh some time because uh it is installing all
your dependencies i know installation process is not going to take 2 days obviously it
will get installed in 10 to like max to max if you have very bad internet it will take
two 2 3 minutes or else it will just take 1 minute okay it's not going to take you 2 days for installing
this yeah so what we did is npm I Okay so after we are done with the npm I we will be running our application
npf run def okay npm rundev now our application is running at localhost 5173 let me show you let me stop
presenting my terminal i will show you that on Google host just give me a
moment okay so we have local host normally we have 3,000 but here in VIP we use
5173 yeah so this is your normal page that you can see after installation it will be like
this okay again it has the state thing that I told you the counter thing okay so uh once you're done with that uh we
will see like you can change the ports and all other installations we will see also the next thing that we have to
install is our t CSS but before that I also have to tell you one thing yeah the thing is uh to check if you
have npm installed or not okay so we will get back to our terminal again and we'll uh see if our mpm and reacts
installed or not it most importantly we have npm to be seen okay yeah I'm back at the terminal so
what I did is npm- version npm- version or else you can do npm- v also it will also tell you the version
of the npm installed so if you are getting a version any version 10.2.4 11 something whatever latest or
old version you have if you have older versions uh just get it updated uh if you have a real version no issue with
that okay yeah so you can use npm uh dash mean uh for checking if we have npm
installed or okay uh let's check with if we have the react installed or not react is not recognized
uh so we might be making a mistake what is the mistake that we are making react version it will also be recognized can
anyone tell me what's the mistake I'm making while checking the version of the React anyone from your
side uh can you show the map board once just a minute i will show you once we are back uh to the speed i'll show
that react is pre-installed uh yeah I know React is pre-installed okay show
that like what would be the command uh to check it if React is installed or not okay so what you can use is npm list
react uh so what I'm using it inside the core app so obviously I am making a react application so I know that it is
it is installed it is a common sense to know if I have made a react application then it it will always be installed okay
so it's not a thing to be checked but if you want then obviously if you want to see the version of the react then you
can check it out by using npm list react okay yeah yeah i know reacts dot dot version is obviously a joke i know it
will not be working but just for your clarity that you do not do it i am telling you what are mistakes not to
make okay so npm list react C while in this boot camp I will be doing such commands i will be writing such commands
and quotes that will obviously be senseless only to tell you not to do that okay so if I write that there would
be only two purpose either I'm making a great mistake or else I'm trying to test it okay so if you get to see those
mistake just write in the comment sections or in the live chat that yeah this is a mistake I'm making I will
correct it out okay yeah so npm list react will work fine now any someone wanted to see the
code the map let me share it with you yeah so let's get to the workspace
back and yeah I think so you can see the code for your map once you write multiple HTML
components so you will see what all things you have to change in this okay worry we are not yet done with this all
things I just wanted to take first session as a basic session so that you can get a revision of what react is what
different component is tomorrow we'll be starting with our installation of tailwind CSS we'll directly start from
that part and then we will start with coding okay so we'll uh first begin with the front end part and that we'll be
writing the home screen code and other codes i will tell you what all the components we have and slowly slowly
we'll moving forward we'll create the footer we'll create the header uh then we'll having our section created and how
to fetch those live sports what API to use what uh JS to write and anything everything we will starting from
tomorrow slowly slowly not fast we will be going with a good pace not too fast not too slow it was the first session
that's why we went slow and two basic not from tomorrow so I just wanted to give you explanation of the basics so
that you can get to it okay you can get in the base and tomorrow we can tightly start with the application okay
yeah see if you are having a doubt about npx create react app my app obviously you can use npx npm whatever you want
okay so no problem with that and I think if you want to try if will this will work or not just try it in the term
okay if you want I can show you if it will work or not you want to see that no problem i
will show you if it will work or not just let me share your terminal okay my terminal is on the
screen let me get one directory back and you want to test if npx create app will work
or not create react have
my Okay yes I think so you can see my screen yeah you can see it's working
right great it's working let's write yes to proceed it's working so as I told you if you are getting a curious doubt if
you're getting a curious doubt out of your curiosity that will this command work or will this command not work just
directly be open to try it out in terminal okay was if you're not curious if you're not
trying out things then obviously will not be learned okay so uh someone came with a doubt that if npx will not npx
will work or not it is working now you have two ways to create a react application there are multiple ways you
have you know two ways now now you can do whatever you want whichever you feel easier so npx1 will be more easier no
problem with that now uh only thing I want to highlight is you can clearly see a warning over here
saying that create react app is depreciated okay you are not getting the same warning in uh this command okay it
was just uh no warning over here so it will work no issue it will work but in future uh you might get some issues or
problem with that for now it will work so it's better to use the latest command npx is working great no problem but
let's switch at that last command that we used at the first place
okay uh it is taking too much time let's close this up i just wanted to show you if it is if it will work or none okay
yeah uh any doubts till this point any issue that you are having ing
anyone okay the code uh will be shared with you uh even notes can also be shared with you it's usual we will share
it up [Music] the link and it copy the
link yeah so uh we will be sharing this up okay yeah whatever code you want to use uh
and if you want to use like VBS code or the code sandbox whatever you want to use you can because it's not a
constraint yeah as I told you you can use whatever you want it's your free will wherever
you feel it you will get to know soon you get to know where we areing this
okay great uh I think you have your attendance form over here just fill it up here yeah you will get to know where
we are putting and all different things don't worry about that you will get informed
yeah the command to install T we will see in the next class it will be similar if there will be any change I will just
notify you while we will be implementing that okay yeah you can do the attendance in the
form i think so the form is updated for that okay uh no doubts related to the code or any concept that I taught today
if you have just write it in the chat great if you understand everything clearly for today's lecture it will be
great y so uh everyone remember tomorrow we'll be starting the application implementation okay so we'll be starting
with bit and all so I want you all to be present tomorrow like you should not miss the tomorrow
session okay then uh we'll be closing this live session now yeah hope so you got all the
concepts right great okay if you have any doubt uh just cons like we can resolve it in tomorrow's lecture okay
and uh have a nice day ahead thank you bye-bye
Heads up!
This summary and transcript were automatically generated using AI with the Free YouTube Transcript Summary Tool by LunaNotes.
Generate a summary for freeRelated Summaries
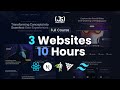
Build a Modern Frontend Developer Portfolio Using Next.js, Tailwind CSS, and Sentry
Learn to create a stunning developer portfolio with animations, responsive layout, and performance tracking.
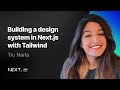
Implementing Your Own Design System in Next.js
Learn how to efficiently create a reusable design system in Next.js using Tailwind CSS and other modern tools.
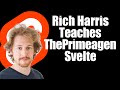
Getting Started with Svelte: The Ultimate Beginner's Guide
Learn the best starting points to get started with Svelte, including tutorials and tips for beginners.
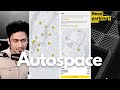
Building the Ultimate Auto Space Parking Application
Learn how to create an advanced parking application with Auto Space using modern technologies.
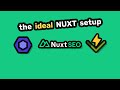
Essential Nuxt Modules for Optimizing Your Next.js Project
Discover the must-have Nuxt modules for linting, testing, SEO optimization, and more to enhance your project.
Most Viewed Summaries
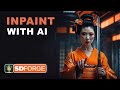
Mastering Inpainting with Stable Diffusion: Fix Mistakes and Enhance Your Images
Learn to fix mistakes and enhance images with Stable Diffusion's inpainting features effectively.
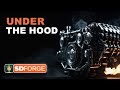
A Comprehensive Guide to Using Stable Diffusion Forge UI
Explore the Stable Diffusion Forge UI, customizable settings, models, and more to enhance your image generation experience.
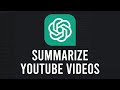
How to Use ChatGPT to Summarize YouTube Videos Efficiently
Learn how to summarize YouTube videos with ChatGPT in just a few simple steps.
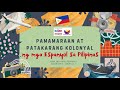
Pamaraan at Patakarang Kolonyal ng mga Espanyol sa Pilipinas
Tuklasin ang mga pamamaraan at patakarang kolonyal ng mga Espanyol sa Pilipinas at ang mga epekto nito sa mga Pilipino.
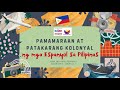
Pamamaraan at Patakarang Kolonyal ng mga Espanyol sa Pilipinas
Tuklasin ang mga pamamaraan at patakaran ng mga Espanyol sa Pilipinas, at ang epekto nito sa mga Pilipino.