Introduction
In this comprehensive guide, you’ll learn how to build a modern and responsive frontend developer portfolio using Next.js, Tailwind CSS, and Sentry for performance monitoring. With the increasing competition in web development, having a standout portfolio is essential to grab the attention of potential employers, and this tutorial will equip you with the skills to do just that!
Why a Developer Portfolio?
A developer portfolio isn’t just an online resume; it’s a creative showcase of your skills and projects. It reflects your expertise, style, and can demonstrate your knowledge of modern web technologies—including frontend frameworks and performance optimization techniques.
What to Include in Your Portfolio:
- About Me: A brief introduction about yourself, your skills, and experience.
- Projects: Showcase various projects you’ve worked on (links, descriptions, and technologies used).
- Technologies: List of technologies or frameworks you’re proficient in.
- Testimonials: Feedback from clients or colleagues.
- Contact Information: Make it easy for potential clients or employers to reach you.
Tools and Technologies You'll Use:
- Next.js: A React framework for server-side rendering and static site generation.
- Tailwind CSS: A utility-first CSS framework for rapid UI development.
- Sentry: An application monitoring tool to track and manage errors in production.
- GSAP (GreenSock Animation Platform): For smooth animations.
Setting Up Your Environment
1. Initialize Next.js Project
Begin by creating a new Next.js application. Open your terminal and run:
npx create-next-app@latest my-portfolio
After the setup, navigate into your project directory:
cd my-portfolio
2. Install Tailwind CSS
Install Tailwind CSS by running:
npm install -D tailwindcss postcss autoprefixer
npx tailwindcss init -p
3. Configure Tailwind CSS
Open the tailwind.config.js
file and configure it like so:
tailwind.config = {
content: ["./pages/**/*.{js,ts,jsx,tsx}", "./components/**/*.{js,ts,jsx,tsx}"],
theme: {
extend: {},
},
plugins: [],
};
Add the Tailwind directives to your CSS file:
@tailwind base;
@tailwind components;
@tailwind utilities;
4. Install Sentry
To track performance and errors, you should setup Sentry. Run:
npm install @sentry/react @sentry/tracing
5. Setup Directory Structure
Create a clean structure for your components and pages:
components/
Header.jsx
Footer.jsx
Projects.jsx
About.jsx
utils/
constants/
Building the Portfolio Components
6. Header Component
Create a header component that includes your name and navigation links to different sections of your portfolio.
Example of how to create the Header.jsx
:
import React from 'react';
const Header = () => {
return (
<header className="flex justify-between items-center bg-white p-5 shadow-md">
<h1 className="text-2xl font-bold">Your Name</h1>
<nav>
<ul className="flex space-x-4">
<li><a href="#about">About</a></li>
<li><a href="#projects">Projects</a></li>
<li><a href="#contact">Contact</a></li>
</ul>
</nav>
</header>
);
};
export default Header;
7. Footer Component
A simple footer with your contact information.
Example of how to create the Footer.jsx
:
import React from 'react';
const Footer = () => {
return (
<footer className="bg-gray-800 text-white p-5 text-center">
<p>© 2024 Your Name. All Rights Reserved.</p>
</footer>
);
};
export default Footer;
8. Projects Component
This component will dynamically load your projects from a constants file. Structure each project with relevant information like project name, description, and links.
Adding Interactivity with GSAP Animations
9. Implementing Animation
Install GSAP using:
npm install gsap
Create animations for each section of your portfolio.
Example:
import { gsap } from 'gsap';
const animateElement = () => {
gsap.from(".your-class", { duration: 1, opacity: 0, y: -50 });
};
Monitoring with Sentry
10. Integrating Sentry
Set up Sentry in your _app.js
file and monitor errors as users interact with your portfolio.
import * as Sentry from '@sentry/react';
Sentry.init({
dsn: 'YOUR_SENTRY_DSN',
integrations: [new Sentry.BrowserTracing()],
tracesSampleRate: 1.0,
});
Conclusion
You now have a complete modern frontend developer portfolio built with Next.js and Tailwind CSS. You can customize the styles, add functionality, and showcase your skills effectively. Make sure you monitor performance with Sentry to keep everything running smoothly. Happy coding!
do you want to stay ahead of AI by learning modern frontend skills that even Devon can't beat this video is what
you need gone are the days when people were happy with plain old websites that Bots like vz. deev can now create with
ease now is the time to step up your game and build websites that outshine the rest so hi there and welcome to a
new course where you'll build and deploy not one not two but three stunning uiux websites turned into an ultimate course
designed to transform you into a front-end developer ready to get hired will'll kick things off with a crash
course on gap for building silky smooth animations and 3js for giving websites that 3D feel after which you will clone
the iconic Apple iPhone website then we'll develop a visually stunning landing page featuring exciting
animations Parallax effects and a Sleek Bento grid your users won't be able to look away and lastly you will showcase
your newly acquired skills with a minimalistic yet striking developer portfolio impressing potential employers
with both your creativity and Technical expertise and are you worried that this is a bit too advanced fear not I'll
break down everything from Gap and 3js for 3D effects to tailman CSS for styling all in a beginner friendly
step-by-step way so by the end of this course you'll have a unique set of front-end developer skills and the
confidence to build responsive web applications that leave a A Lasting Impression here are the projects you'll
build the first project is the iconic 3D Apple iPhone website clone yes we'll code an iPhone 15 Pro clone using
nothing less than Gap and 3js but we won't just clone the site we'll start with a detailed crash course on how to
create powerful animations using Gap after which I'll teach you the Core Concepts of 3js and 3D development and
then you'll build the Apple clone website that has custom animations with scroll triggers staggers timeline and
more a custom video slider with progress tracking animated 3D models with different configurations lights and
viewports and other sections with cool animations complete responsiveness and Optimal Performance then you'll develop
a modern UI X website called brainwave you'll build a beautiful hero section with different shapes reacting to your
cursor a Navar with a colorful button that transforms into an animated hamburger menu when you're on smaller
screens and look at that subtle smooth scroll Parallax effect that moves these elements down as we scroll moving on
we've got a nice Services section featuring gradient borders on the cards and unique curved rectangle shapes each
of these cards also reveals a hidden background image next we have a pattern that showcases the most important
elements in a circular layout after that is a very fresh and modern web development layout called Bento Box many
popular companies like apple linear and clerk use this layout to display their features effectively below there's an
always needed pricing section after that there's a great style layout presenting the applications road map with gradients
grid lines highquality images and geometry lines on both sides creating a modern look lastly there's a simple
footer where we connect to the website social media pages and to feature all of your work you'll develop a modern
developer portfolio with a modern hero section with spotlights and text animations latest Bento grid design
here we went a step further and animated some parts into this grid for example this globe is a real 3D Globe which you
can move around then we have our text tack right here some more information about yourself even some code blocks and
then this nicely animated bottom part that allows your users to copy your email moving below we have a small
selection of recent projects and what's cool about this and the previous section as well is that we're not only using
Tailwind we're also using exterity UI which gives us a lot of these outof thebox frameware motion animations and
that makes it look different from anything I've seen so far testimonials and companies you've worked with also a
section about your previous work experiences about being a front-end engineering intern mobile appdev
freelance project and so much more and don't forget if you scroll up you have this nice bar that pops up that allows
you to scroll to different sections moving forward we have my Approach which tells employers how you work starting
from planning and strategy to development and progress updates to development and launch so you're not
just doing development you're doing so much more than that and a short but sweet footer section alongside building
these great projects you will also learn learn nextjs with server and client side rendering routing and layouts for better
performance and SEO Tailwind CSS for a modern completely mobile responsive UI 3js Gap and exterity UI to create
mindblowing animations functional components and how you can reuse them to make your code more efficient the best
file and folder structure for organizing your code like a pro and much more we'll go beyond just building your websites
and I'll teach you how to deploy them to hostinger fast servers and get your custom domain name but we're not done
yet we'll also use Sentry to track your app's performance like how many people visit how fast it loads what users are
looking at how much each operation takes which parts of the code are slowing things down how fast each component is
loading and see if there are any issues with specific devices and what about the prerequisites well all you need is a
basic understanding of JavaScript and react plus I highly recommend taking our tailin CSS crash course to get the most
out of this course now with even better teaching methods where you'll get an open- Source codebase you can look at if
you get stuck a custom figma design so you know exactly what you're building and a Discord server to get all your
bugs resolved all of these things are free so get them immediately from the links in the description while the intro
is rolling and since my goal is to make sure you land a job your portfolio will not be like any other project it's a
career asset designed to Showcase everything you're capable of making you an attractive candidate for any job see
hosting your portfolio on a personalized domain makes you more professional and improves your credibility and search
engine visibility it also speaks about your skills in self-hosting scaling and managing websites effectively so before
we dive into coding we need to set the foundation for hosting all of our projects especially the modern portfolio
and right now they're offering in a great deal which you don't want to miss when it comes to hosting This Modern
portfolio I personally go for the Premium plan and here's why it's perfect for this and many other projects you can
host a 100 websites with the same account you get a free domain name which gives you a professional touch you get a
free SSL for https security which creates trust with anyone visiting your portfolio this deal won't last forever
and because we've partnered with hostinger you get an even bigger discount so once you visit the link in
the description click claim deal and add to card here we have to choose the period of our hosting with a crazy
discount going on right now I'll definitely choose 48 months to save the most money and down below you can choose
your payment method and enter your JavaScript Mastery coupon code which will give you an even bigger discount on
your hosting package once you've made your purchase we are ready to set everything up and get started with
building out our portfolio let's start with hostinger guided setup we are building a new website so we can choose
create and we're building a completely custom website so we Cano choose skip at the bottom next we need to choose our
domain as you can see I use hostinger for my Js mastery. proo platform so here you can type out your domain name I
would recommend something like your first name and last name.com something like John doe.com and if you purchase
the hosting for more than one year you'll get the domain completely for free choose it and click next the domain
is registering and while that is happening I quickly want to point your attention to our improved Discord server
where now we have dedicated forums for each video so if you're building this portfolio and you get stuck you just go
to the Forum and ask a question this is the best way to get help now already we are the next step where you can choose
where your audience is located at and you can see there's a lot of options click next and the setup will initialize
while that is happening just another quick note and that is that the full codebase of the video you're about to
build is live on GitHub so you can check it out right here and if you don't mind give it a star with that in mind we are
redirected to the dashboard of our hosting project so finally let's let's code it out and we'll deploy it at the
end of the course ever caught yourself endlessly scrolling through the Apple's website fantasizing of owning the latest
iPhone yeah we all been there but have you ever wanted to actually create something as slick as what Apple
showcases hi there and welcome to a new video where I'll teach you how to build stunning apps like apple does for its
products yes we'll code an iPhone 15 Pro clone using nothing less than Gap and 3js but we won't just clone the site
we'll start with a detailed crash course on how to create powerful animations using Gap after which I'll teach you the
Core Concepts of 3js and 3D development and then you'll build the Apple clone website that has custom animations with
scroll triggers staggers timeline and more a custom video slider with progress tracking animated 3D models with
different configurations lights and viewports and other sections with cool animations complete responsiveness and
Optimal Performance will go beyond just building and deploy your website to hostinger fast servers but we're not
done yet we'll also use Sentry to track its performance like how many people visit how fast it loads what users are
looking at and see if there are any issues with specific devices alongside the video I've also prepared the
ultimate 3js and gep guide that covers a step-by-step road map inside their tips examples and cool project ideas to take
your animation and 3D development skills to the next level the link to this completely free guide is in the
description so are you ready to dive in and learn Gap 3js and then put that expertise the work by cloning the iPhone
15 Pro website let's go green Soxs animation platform often referred to as just Gap is a high performance
JavaScript animation library that allows you to effortlessly animate any anything JavaScript can touch you can animate
anything from uis and svgs to creating immersive 3D experiences from exciting scrolling animations to changing shapes
of objects text effects and UI interactions there isn't a thing you can't animate with gap any exciting
website you see with animations Parallax effects or fancy scrolling tricks is likely made with gsap from making basic
HTML elements move smoothly to creating entire animation timelines more svgs customizing bounce and wiggle effects
simulating physics and more Gap is the all-in-one solution for all of your web application animation needs Gucci
Spotify Nike Shopify and even Google all use gsap but there's also framer motion known for its gesture-based interactions
and its declarative syntax which tightly integrates with react for simpler animations in react it's the Top Choice
both animation libraries have their own unique use cases but here's where Gap takes the throne Gap is known for its
exceptional performance even in complex animations it's optimizations keep the animation smooth making it the go-to
choice for applications in which performance matters Gap also offers a high level of flexibility and control
over animations allowing you to create complex sequences timelines and effects Gap is extensively tested and supported
across many browsers and devices ensuring consistent an animations for all users even on all the browsers and a
rich feature set allowing you to do anything from simple effects to Advanced physics-based animations now let's move
to the second most important technology in making our apps interactive it's 3js and it'll shape the future of the web
with advancements in virtual reality and wider access to more powerful devices 3D development is just warming up before
3js creating 3D graphics for the web was a complex task web developers had to rely on low-level apis like webg or
special plugins like Adobe Flash or Unity remember those which were a pain in the ass with limited browser support
and extreme use difficulty with the growing demand for immersive web experiences and increasing strength of
modern browsers developer needed a better solution for 3D apps 3js came in and filled this Gap by abstracting away
the complexities of webg and providing a userfriendly API for building 3D scenes directly in the browser using JavaScript
some of the key features are the abstraction of webgl 3js abstracts away all web gl's complexities providing a
higher level API that simplifies the process of creating and rendering 3D Graphics it has a high crossplatform
compatibility making apps work seamlessly across different devices and browsers
it provides a rich set of tools and utilities for creating immersive 3D experiences including support for
lighting shading textures animations and interaction it's also very fast using web gl's Hardware accelerated rendering
3js ensures smooth and efficient performance even for complex 3D scenes and finally it also has an active
community of developers contributing tutorials examples and plugins making it the go-to choice for developing 3D apps
and starting to learn 3js is like diving into a new and exciting world with many features and Concepts to learn to
properly learn 3js you'll have to move away from the 2D vision and start seeing websites in 3D in a 3D space objects
possess threedimensional properties like length width and height or in math X Y and Z unlike the two-dimensional World
which we typically interact with on screen 3D space adds depth allowing for a more realistic experience each point
in a 3D space is represented by a set of coordinates that specify its position from the origin now I want to teach you
the most important Concepts in 3D development first is the scene it's the canvas where all the objects lights and
cameras are placed the environment in which the 3D World exists and where interactions
happen then there's the renderer which converts the 3D scene into 2D images that can be displayed on the screen it's
responsible for rendering the scene onto the browser the camera plays an important role in defining the Viewpoint
from which the scene is viewed it determines what is visible in the rendered scene cameras have properties
like position rotation and field of view that determine their behavior and how the scene is rendered 3js supports
different types of cameras with special behaviors like a perspective camera which mimics the way human eyes perceive
the world creating a sense of depth and perspective and an autographic camera which renders objects without
perspective which is useful for technical drawings or 2D games lights illuminate the scene and affect the
appearance of objects 3js supports different types of Lights including ambient light which adds overall
illumination to the scene without a specific Source directional light which emits light from a specific Direction
like sunlight Point light which emits light in all directions from a single point in space Spotlight which emits
light in a cone shape from a specific position and Direction and there are many more lights have properties like
color intensity and position that control their behavior and influence on the scene so now that we have the scene
the camera and the lights we can finally see some things within the space and the first thing we can easily show would be
be a mesh a mesh is the basic building block of 3D objects in 3js it consists of vertices points in 3D space connected
to form faces meshes Define the shape and appearance of objects in the scene and each mesh is associated with a
geometry and a material geometry defines the structure and shape of a mesh by specifying its vertices faces and other
attributes 3js thankfully provides a wide range of built-in geometries like box geometries spheres planes cylinders
and more you can also create custom geometries by defining their vertices and faces manually which will later on
allow us to see such a detailed 3D Apple iPhone model then there's material material determines the appearance of a
mesh including its color texture and how it reacts to light 3js offers some built-in materials like mesh basic
material which is simple and is not affected by lighting mesh Fong material which reacts to some light sources and
then the standard material which is a physically based realistic rendering and there are many other materials as well
which you can customize by adjusting properties like color opacity highlights and more and these were all the basic
building blocks of 3js and 3D development of course you can find their detailed instructions alongside a cheat
sheet in the guide down below but but 3js also has some more complicated stuff like shaders tweaking with meshes and
animating things around I'll show you how to animate 3D models using Gap in this video but going into detail on all
of these things would make this video too long so if you want a full crash course on 3js just drop a comment and
let me know and before we dive into the main star of this video which is of course cloning the 3D Apple website with
3js and GAP let me provide a conclusion on this part of the crash course which is how to use 3js there are a couple of
ways in which we can use 3js depending on Project requirements and your preferences you can use 3js directly in
vanilla JavaScript without any additional libraries or Frameworks all you have to do is put a CDN script tag
for 3js but of course you can also integrate 3js with Frameworks such as react View and angular each one of these
has its own way of integrating 3js we'll use react 3 fiber which is a library that allows us to easily integrate 3js
into react applications and it even makes it possible to create 3D scenes with react components so just so you
know you'll learn react 3 fiber in this video as well so just to give you an example in vanilla JavaScript you would
have to first set up the scene by saying scene camera and renderer figure out where you want to render the canvas and
then add a cube to the scene and maybe even a plan animation in react 3 fiber it's much easier and much more similar
to what you're used to you simply import the canvas and the used frame from react 3 fiber and then you import the box or
some other element you want to show and call it using Hooks and show it with react components react through fiber
acts as a wrapper which makes it easier to add 3D scenes to our react applications enough with Theory let's
dive into action I prepared a quick workshop for you and I've linked it for for you in the description download it
open it within your Visual Studio code and then we can install all the necessary dependencies by running mpm
install this is going to take just a moment as we don't have too many dependencies as a matter of fact we can
look into what we do have and you'll notice that it's basically just react react Dom and react router Dom with some
Tailwind CSS so the entire setup is completely empty will explore everything from scratch so let's go ahead and run
the application by running mpm run Dev to see what we have on it and then we can hold the command key and click on
this link to open it up as you can see I prepared seven different demos that teach you seven most important Gap
Concepts so let's dive into the first one by clicking right here and let's explore gp. 2 gp. 2 method is used to
animate elements from their current state to a new state it's similar to gp. from but the difference is that the gsap
2 method animates elements from their current state to a new state while gsap from animates elements from a new state
to their current state and of course you can read more about it if you head over to the documentation page basically you
can see how it works right here you target a specific element by providing the identifier and then you specify the
properties that you want this element to take after the animation runs so we are changing the rotation and the exposition
throughout the duration of 1 second so now you've seen the demo right you could have done on your own without me but see
this box right here now we'll animate it together to solidify what we just learned so going back to the code we can
split our terminal and install a new package by running mpm install Gap as well as at Gap SL react which will allow
us to start adding those animations once that is done we can head over to our pages and then Gap 2 as that is the
first element we want to animate in this case I want you to animate the box that we have right here below so if you
scroll down you can see a div that has a class name of VW HW and then BG blue 500 which makes that a box and here we have
an ID of blue box so let me teach you how to animate using gab in react right below this Todo we can say use Gap
coming from Gap react this is a special hook which looks and behaves a lot like use effect essentially you define a
callback function and then a dependency array on when this has to run and within it you can use Gap to then animate the
elements you want so we do that by saying gap which you have to import from the original Gap package do2 and then
the first parameter to the do two method is the target you want to animate in this case we can Target it by ID by
saying blue box then the second parameter are variables which you want to animate so
you can expand an object and let's do something like X is 250 pixels so we animate from X of zero of course by
default to X is 250 so now if you go back to your page and reload you can see how nicely this animates and just so we
can see our animation Happ happening in real time at all times without needing to reload the page we can add a repeat
of minus one as well as a yo-yo property set to True which will make the animation reverse on every other cycle
so as you can see now it's going back and forth let's pull this to the side so we can continue animating while we're
writing the code at the same time there we go that's much better now let's also give it a bit of a rotation property of
360 and you can see now it completely rotate and you can see now it completely
rotates we can also increase the duration to something like 2 seconds if you do that it's going to move much
slower and we can also give it a special ease property which defines how the animation happens as you can see
immediately you get a lot of different properties you can choose from for example bounce in if you save it you can
see now it kind of bounces in and then stops you also have bounce out which going to make it bounce at the end
there's a lot of these different easing curves that you can decide to use there's also Circle back and so much
more in this case let's use elastic as you can see it looks like it's on a spring right now which you have to pull
and then move back and forward which gives it a very interesting effect but with this in mind you just learned how
to use one of the fundamental methods in gsap which is gap. 2 property which accepts an ID which is an identifier and
then properties you want to apply to the element you're targeting you also learned how to apply animations using
the used Gap hook and gsap within react so with that in mind let's go back to the homepage and navigate to the second
method of the day Gap from as the name suggests Gap from is used to animate elements from a new state to their
current state similar to GP 2 but the difference is that the from animates from the new to the current while GP 2
animates from their current to a new state of course you can find more information within the docs but for now
let's implement it in action I'm going to go back to gap. 2 and copy this entire
part then I'm going to navigate to Gap from and simply paste it right here below of course not forgetting to import
the used Gap as well as Gap now if we modify the method from to to from and modify the ID from blue to green and
save you can see that it started moving from the back and then going to the front in this case let's explore a bit
of a different ease one I like to use often which is power one.in out this one starts with a lot of power and then
slows down as you can see it right here but the most important thing is that now it starts from the position 250 pixels
in if I load see how it started from the right side moving to the left that is the only difference between from and to
the next method of the day is Gap from two which as the name suggests is used to animate elements from a new state to
a new state similar to from and two methods but the difference is that here you can Define both States so if we
scroll down we can copy the entire thing we have here and navigate to from two so let's paste what we copied import the
hook as well as Gap we can modify the method from from to from Two and you still pass a
specific identifier but this time you have two different objects you can modify we have the first object which is
the from object and then we have the two object which accounts for the two properties we want to implement so let's
try to animate it from an X of zero and a rot of Z with something like a border radius of 0% as
well and we're going to animate it to 250 repeat minus one and let's also add a border
radius of 100% And we can also modify the ease to something like bounce out to explore another one now if we modify the
identifier from Green Box to Red Box and save it now you can see how it turns from a square into a circle and then
comes back so we're modifying the rotation and the X and the Border radius to zero at the start and then at the end
we set it to 100 which essentially makes it a circle this is pretty useful when you want to modify both the start and
the end of an animation with that in mind those have been the three base Gap methods but let's explore what else we
have in store next one on the list is the GP Tim line and the gep timeline is used to create a timeline instance that
can be used to manage multiple animations again it's similar to two from and from 2 but it is used to manage
multiple animations while all of these manage just one element from their current state to a new state of course
we can open up the docs to learn a bit more about it and in this case you can see that they consider it a sequencing
tool that act as a container for the other animations we have explored it allows you to have complete
control and manage the timing of an animation so instead of doing something like this first do X100 then do y50 and
then change the opacity to zero you can create a timeline and then you can modify the elements to move on that
timeline you can also control the whole thing easily by pausing it resuming it reversing it and so much more so with
that said let's animate this yellow box and also include the play and pause button so we can navigate over to Gap
timeline and you can see at the bottom as we always do there is a div that has an ID of yellow box so first things
first when creating a timeline we have to Define it by saying const timeline is equal to gap which you have to import
from gap. timeline and then you call it like this next you can also pass the options
object to give it some properties like repeat minus one repeat delay of something like 1 second and yo-yo to
true we already learned what this does it simply makes the Box move left and right it simply makes the animation
repeat so we don't have to reload the page to check out the animation now that we have the timeline defined we can use
the same use gsap hook we have used not that long ago Define the Callback function as well as the dependen array
now within it we can use the timeline. two property instead of using the gap. two property and it works in the same
way you have seen it before it's just timeline. 2 you then Define the identifier which in this case is the
yellow box and the second parameter are the variables you pass to it such as the x is 250 rotation is 360 border radius
is 100 per duration is two and then ease is back do in out again this doesn't matter
too much right now but we're just playing with the way that the animation actually executes so if we save it now
you can see how it goes from one end to another and comes back now what we can do is we can also say
timeline do 2 again Target the same yellow box and then provide another another set of variables we want to
apply to this animation such as X500 scale of one rotation of 360 border radius of something like 8 pixels
duration of two and ease of back. inout now if I save this you can notice that it's yellow and then it goes further out
of the screen I'm going to zoom this out a bit so you can see the full animation first it goes to 200 50 Waits a bit and
moves to the end and then it returns now this is a bit similar to using the from and to or the FR 2 but what this allows
us to do is to add additional animations in the middle so we can say timeline. two once again where we target the
yellow box and this time we can for example do the Y of 250 scale of two rotation of
360 border radius of about 100% And duration of two as well as the ease back do in out now if we save this we have
added a third animation to the mix where now it will actually go down out of the screen move back and then it's going to
come back again so these animations are happening in a row one after another which gives you a lot of possibilities
to control the entire timeline now another another thing you can do here is of course play and pait because you have
complete control over the timeline so let's head over to our play and pause button which is right here and let's
expand this on click functionality we can define an if statement and say if timeline. paused is true then we want to
trigger the timeline. playay and also else vice versa then we want to run the timeline Dot pause so if we do this and
save now you can click play pause and completely pause the execution of the animation then you can trigger it again
and it works so I think you can see the benefits of running animations within a timeline you have more control now let's
move over to the fifth method in the Gap repertoire stagger Gap stagger is a feature that allows you to apply
animations with a staggered delay to a group of Elements by using the Stagger feature you can specify the amount of
time to stagger the animations between each element as well as customizing the easing and the duration of each
individual animation this enables you to create Dynamic and Visually appealing effects such as staggered Fades
rotations movements and more and of course you can find more within GP stagger documentation they even prepared
a little video but in essence it is as easy as using the Stagger property so let's explore it in Act ction by
navigating over to the Stagger file in this case if you scroll down you'll notice that we don't have a single box
with an ID of box rather we have multiple divs that all have the same class name stagger box so instead of
Simply using an ID we'll use the class name as an identifier so same thing goes we can use the use Gap hook create a
callback function and then we can use the gsap do2 method and in this case we can
import Gap from gsap now you might say hey we already learned two but we haven't used stagger well stagger is
just a property you can apply to any animation so we'll use two to show this out to explain the Stagger by targeting
the Stagger dashbox and then we can provide the options in this case we can do the same thing we've done before such
as y of 250 rotation of 360 border radius of 100% to turn it into a circle we can add a repeat of
minus one as well as a yo-yo of true now if we save this you can see they all follow the same animation as we're
targeting all of the elements simultaneously but what if we want them to animate one by one well in that case
we can provide a stagger property of 0.5 let's say so now we can save it and you can see that now after half a second
each one of this animates one after another but it doesn't end there we can also apply more complex stagger
Properties by defining stagger as an object here you can provide the amount which is the amount of time to stagger
the animations between each element and we can say something like 1.5 then you also have access to something known as a
grid grid selects the number of columns and rows in a grid so you can say something like 2
1 then there's also the axis and you can choose the axis along which the stacker the animations in this case we can say y
but you could also say X in which case they all happen at the same time next you can also apply the ease which in
this case we can do something interesting like Circ dot in out and how kind of they happen in a circle and
finally this is the most interesting one you can Define the from which is the starting position of the staggered
animation so instead of starting from the start or the end we can say Center so this will make it start from the
central staggered box as you can see now it happens from the start and moves until the end and
you can also see how they're doing two and then one and so much more so you have complete control over how you want
to stagger your elements this is great so now you know how stagger works and how you can use it when you have
multiple Elements which you want to animate and let's call it a dance of sorts the next feature on our list is
the scroll trigger which is use the trigger animations based on the scroll position so as you scroll you can
animate specific things once you reach a specific point in time so for example scroll down to see the animation and
then only after you reach a specific point we want the animation to start so let's first open up the documentation
page and I always advise you to read the doc for anything you're doing there's also a quick video right here and the
simplest way is to attach a specific Target which when it enters the viewport or the screen then the animation happens
or you of course have much more control over when what over when the animation triggers in this case we can go back and
go to Gap scroll trigger and let me show you how to implement it first of all it's very important to note that GP
scroll trigger is a plugin which means that we have have to initiate it at the top by saying import scroll
trigger from Gap all and then you can do gap which we have to import from gap. register plugin and then you pass that
scroll trigger this will make it work next with triggers you also have to define a ref a reference so we can say
scroll ref is equal to use ref coming from react this is not Gap related this is
just react then we can use the same old use Gap hook coming from Gap react which we
can Define like we have done so far now in this case if you scroll down you'll be able to see that we have some boxes
right here now if you scroll down let's attach that ref to our boxes so if we scroll here you can see that we have two
boxes the scroll pink and scroll orange and we can attach a r to this div wrapping both of them by
saying ref is equal to scroll ref like this now we can scroll to the top and we can start animating so first let's get
access to those boxes by saying const boxes is equal to gap. utils do to
array and to it we pass the scroll ref. current. so this is how we can get the children
using a react ref next we can also run the boxes dot for each so for each box we
want to do something and that something will be a simple gap. 2 animation where we target each individual box and for
each one we apply some specific properties in this case we can say x is something like
150 rotation is is 360 border radius is something like 100% scale is 1.5 we have learned that
already right so now if we scroll down you can see that they basically already turned into circles even though we
didn't see it at all because we were at the top when the animation was happening not too useful right typically you want
to have some animations when you scroll up to a specific point like if we're here we can see it sure but the user
will come from top and then we'll start viewing this at the bottom so this is where scroll trigger animations come in
to this gsap 2 property we can apply a scroll trigger which is an object to which you
can pass a trigger and then this one will be a box so one specific box comes into view we want to start the animation
let's see that once again if I start scrolling down already it's a circle but that's
because we're missing something important we're missing the start of the animation which we can Define as bottom
bottom so when the bottom of the Box hit the bottom of the viewport then it will animate and we can also Define when it
will end and it will end when the top of the box hits 20% of the viewport we also have something called scrub which we can
set to True which makes the animation smooth and below the scroll trigger we can also Define the ease of power one do
inout and the reason why the scroll trigger animations are not working is because I misspelled it as scroll
instead of scroll with a double l so if we fix this now check this out I'm going to scroll to the top reload the page and
start scrolling down so now as we enter the viewport slowly you'll see how this starts as a box because that's what it
Styles say by default and then slowly it starts moving to the right and it starts supplying the rotation border radius and
scale as we scroll check this out interesting right and also it goes back immediately now another thing is
that since we're mapping over those boxes we can apply a bit of math to each one so instead of just moving it x 150
for each one we can multiply that by boxes do index off a specific box so this will already
apply it a bit differently because the second one has an index of one and we can also give it some default value like
plus 5 so now if we start scrolling down you can see how nicely they animate and exit out of the screen we come back they
are back and of course you can keep playing with these value like top 30% which will make them go away much sooner
or like something like top 10% which will make them in the screen for a longer period of time it's up to you to
start playing with this and I just noticed we don't need a comma right here it's just bottom bottom so like this
yeah that's great another thing you can add when you're animating using the scroll trigger is if you go all the way
to where you end the used gep function which is here you can also provide a second parameter of scope is scroll
ref like this that way it will know exactly when the animation has to happen so now I'm sure you have already noticed
how useful GP is specifically simple properties like GP 2 staggering and so on but only when you pair it with scroll
triggers which will do a lot within this video and it's also covered in a lot of depth within our ultimate guide which
you can find in the description down below completely for free you notice how it all starts coming together when you
combine all of these properties and finally we have Gap text where I'm going to teach you how to animate text
elements with gap so to animate text you can use all the methods we have explored so far the only thing you have to do is
integrate the text plugin you can see it in the docs basically you can add the Gap 2 and then add the text you want to
animate you also have some special properties for animating text elements so let's head over to text and you can
see an H1 here that says Gap text but it's nowhere to be found on the screen it's kind of like it's being hidden
right and that's because it is at the start the opacity is zero so let's go ahead and animate it in we're going to
use the same old tips and tricks like use Gap coming from Gap react where we have our callback
function then we will Define gap. 2 which we have to import from Gap we target the text element by ID of text
and then we provide some properties like ease which will be power one.in out once again you can go with without this or
you can find the ones that you like and then add different eases and then I'm going to add opacity of one as well as y
of zero so this is nothing new right we're just changing some properties using gsap 2 and you can see how nicely
it slides in great now let me also teach you how to animate the paragraph you can see this par class name right here it
combines all of these properties that you can see so let's add a gap. from two in this case and we can Target the para
which is the paragraph and then as you know with from 2 you have to Define two different
objects and at the start this text is visible we didn't apply an opacity zero like we did here so you can apply it
here in from opacity 0 y of 20 and then of course it's going to break until you provide a second object where you can
reset the opacity to one and Y to zero so now you can see how nicely it fades in we can also apply other things we
learned such as the delay between the elements as well as the Stagger of 0.1 so now different paragraph elements
are going to animate one after another and with that we came to the end of this little Gap Workshop as I said we go into
more detail on all of these and many other more advanced Gap properties within the ultimate Gap and 3js guide
which you can find below so check it out and get warmed up for the main star of the show which is diving a bit into 3js
and then combining the 3js knowledge with your gep knowledge and building something on your own it's one thing to
watch me do things and then enter a couple of methods and it's a completely different thing to build something truly
useful and replicate what some of the best companies in the world do so how do you feel about these Gap features we'll
explore everything in more detail later in the video Gap also offers plugins for physics and motion that allow you to
create animations with realistic physics-based motion such as bouncing elasticity and acceleration and yes I
know we covered a lot of ground regarding Gap in a short amount of time which is exactly why I prepared a
complete GP guide and cheat sheet down below so download it and save it somewhere for reference it's completely
free also leave a comment down below below if you're interested in a more detailed and more advanced Gap crash
course but with that said that's enough Theory so let's get started to get started with building our amazing 3D
Apple website we'll begin from bare Beginnings by creating a new empty folder on our desktop I'm going to
rename it and call it applecore website and then drag and drop it into an empty Visual Studio code
window once you do that we are ready to set our project up to quickly get ready with our development environment we'll
use vit it will allow us to quickly spin up a react application with a lot of interesting things provided out of the
box like the instant server start optimized build hot module replacement and more but of course if you want to
build this project out with another technology like Vue angular or even better NEX GS I would be more than happy
for you to do that you're going to learn even more by building it in your techn you of choice so with that in mind we'll
use vit and with vit we'll also use Tailwind CSS which is a utility first CSS framework packed with utility
classes for easier styling so with that in mind let's go to Quick Search and let's search for vit or your own
preferred framework once you're there we can copy the command and quickly get started with our application back in our
editor I'm going to press control backtick which is a shorthand for for quickly opening up the terminal or you
can go to view and then terminal once you're there you can paste the First Command that we copied but I'm going to
change my project with Slash which will ensure to create all of the necessary files and folders right within the new
Apple website folder we created so press enter it's going to ask us whether we want to install the create V CLI to
which we can say why yes and then run mpm install to install all the necessary dependencies and once that is done let's
follow the next steps right here you want to install all of the necessary Tailwind config so let's copy this mpm
installed Tailwind CSS post CSS and auto prefixer paste it here and press enter let's also initialize a Tailwind
project next we need to update our template paths by copying this content navigating over to Tailwind doc
config.js and by the way Pro tip the way I just navigated is by using command or control
and then P you can start typing and you can immediately press enter to navigate in another file or of course you can use
your mouse and go right here with that in mind I'm going to update our content and then we can follow the next step
which is to add tailin CSS directives to our CSS by copying it going to our index.
CSS removing everything and pasting just these three tailin directives once we have that we can add a simple
H1 to our app so let's navigate to our app.jsx remove everything from the return statement like this and simply
return a simple H1 while we're here let's also remove all of the other things that we don't need and we can
also remove the app CSS as well as removing that file as it's creating clutter
there we go we can also rename this to an es6 plus arrow function there we go so now that we have
this let's reopen up the terminal and run mpm run dev then you can hold command or control and then click right
here to open it up on Local Host 5173 and we are in we can see that the text is enlarged underscored and bolded
which means that Tailwind CSS classes are getting applied properly now let's turn this empty canvas into this a
modern 3D Apple website with gsap animations and 3D models and before we begin another Pro tip for new developers
out there you might notice that my browser is looking a bit weird this is not your typical Safari or Chrome
bookmark bar I'm using a new browser called Arc which is very convenient and allows you to focus on what matters it
is of course built on top of chromium so you always have all of the typical Chrome features you're used to and
they're available for Mac OS and as of recently for Windows 2o and now this is not a promotion I just wanted to share
it with you in case you're interested and before we begin setting up our file and folder structure we have to set up
our linting that will include es lint and prettier so in the description down below I created a special guide
containing the configuration that I personally use on all of my projects and the guide will look something like this
it is a 22 page PDF that covers es length prettier in Visual Studio code for both react and nextjs starting from
the introduction on what eslint even is and why do we need it and then moving on to a complete setup using nextjs but
what you're more interested in for this video is the setup for react all of that is included in this simple PDF and once
you get it you'll also be added to JSM weekly which is our official newsletter where every single week we share some
news from the web development World there is no ads just fresh information on react nextjs and web development in
general so download the guide implement the linting and we can continue now let's set up the base file and folder
structure and The Styling for our project first things first we want to navigate over to the Tailwind Doc
config.js and here we want to set up some basic colors so we can go under theme and under extend then extend the
colors that's going to be an object where we can add a color blue which is going to be #
2997 FF now every time you want to add a specific color you just say blue you don't mention these six random
characters and alongside adding the blue we also want to add a couple more colors and to quickly add them just just click
the read me link in the description navigate over to Snippets and then here you can find all the Snippets for this
video as you can see there aren't many the first one is the tail wi config JS alongside adding the blue we're also
adding a couple of variants of gray for our different text elements and a zinc color so let's copy this entire snippet
and let's simply override what we have here the second thing we want to add when it comes to styling is the
index.css so expand it copy it and then back within our code navigate to index.css
and override everything we have here you'll notice we have about 120 lines but all of these lines are helper
classes providing some text colors text variants and more so if we scroll all the way up you can see we're targeting
the body by making some default modifications and then adding some utility classes like nav height BTN
color container and more we'll refer to these throughout the build of this video but these are in no way all the Styles
we'll be using in this video you will write all of the Styles yourself these are just some helper classes to get us
going next in our file tree we also have the assets folder which for now simply contains the react. SVG icon we want to
remove that assets folder go back to the readme within links and then download our own assets containing all of the
assets for the build of this phenomenal Apple website including the 3D model we'll be using so click the link here
and simply download it from our Google Drive it's going to say we cannot scan it for viruses but I think you can trust
me on this once you download it simply delete this public folder right here and then drag and drop the new one into the
root of our directory click copy folder and here you'll notice that we have our 3D models as well as the Assets Now to
quickly use these assets within our code we're going to create a new folder called utils and within the utils folder
we'll create a new file called index.js back in a read me you can expand this
index.js copy it and paste it right here you can notice that here we're basically importing all of the images and then
exporting them so that we can quickly use them within our project and the last last last thing we need are the
constants these are some predefined arrays of information like content or text that we'll use within this project
for example see this enter a17 Pro gamechanging chip back in our app you can see where this text goes so what
we're doing here we're preparing the content for the entire application everything from nav items to footer
items and more so let's simply copy it and within the source folder create a new folder called
constants and create a new index.js file and paste everything we copied and as you can see there's zero logic happening
here just content which we can consume when we actually develop our website so with that in mind let's prepare for the
development by going into our index.html and let's modify our icon we can go from V SVG to a new icon coming
from our assets that's going to be public assets images apple. SVG so SLU SL assets
slimes slapp apple. SVG and we can also change the title of our application to Apple
iPhone and and save it now if we go back to the browser you can see that now we have this great looking Apple icon as
well as the title and we're ready to turn this into this so let's dive right into the development I'll start by
diving right into our app.jsx we want to remove this H1 and instead turn it into a main section and
give it a class name equal to BG Das black then within here we want to have a couple of components such as the Navar
the hero section and the highlights I think that's enough to get us going so let's create these three as
components by creating a new components folder and within there creating a new file called navb bar. jsx inside of
where we can run rafc and press enter this will quickly spin up a new react aror function
component called navbar and if this didn't work for you you need an extension called es7 plus Redux react
native Snippets so simply install it and it should work alongside the nav bar we're going to also get our hero section
by saying hero. jsx with rafc and we'll also get our highlights component where we can run our
afce now we have three three most important components well maybe second most important as our 3D model is the
star of the show so now let's go back into the app let's turn these three into a self-closing and self- calling
components and let's import them at the top by saying import navbar coming from slash components SL navbar and we can
duplicate this three times and rename the second one to hero and the third one to
Highlights finally let's see if we can see anything in our browser I'm going to pull our browser to the side and my code
Editor to the left now if I zoom this in a bit you can see that we have our navbar hero and highlights which gives
us a lot of room to start working on stuff so let's get started from top to bottom with the first component of the
day the nav bar to start developing it we can transform this div into an HTML 5 semantic header tag and within it we can
render a new nav tag this nav will render an image and that image will have a source equal to Apple IMG like this we
can import that Apple IMG at the top by saying import Apple IMG coming from d/ SL utils if we save it you can see the
Apple logo appear instead of the text that says navbar let's give it an all tag of Apple a width of about 14 and a
height of about 18 within this image we're going to have a div within this div we want to render all of our nav
items and that's going to be dynamic so we're going to have an array of something like let's say I don't know
phones we can also have MacBooks and we can have something like
tablets and we're gonna map over this array by seeing that map where we get each individual nav item and its index
and for each one we want to immediately return a div that will render that specific nav item this was supposed to
be a comma there we go so now we can see phones MacBooks and tablets we can also
give this div a key equal to the index that we're mapping over or in this case we could also just make that the nav
text itself because we know that it will always be unique finally going below this div we want to create another div
which will contain an image this image will be the search image so we can say source is equal to search image coming
from utils I just automatically imported it by pressing the enter key so you can see
it got it from here and we can also give it an Al tag of search as well as a width of 18 and a height of 18 as well
we can duplicate that below and we can also get the bag image from utils in case we want to buy something of course
I'm going to change the alt to bag if we save this you can also see the search and the bag all very minimalistic as
Apple always is is so now that we have the layout of our navigation bar let's start adding the
Styles I'm going to give our header a class name equal to w-o so it takes the full width of the screen padding y of
five to give it a bit of a padding top on small devices padding X of 10 to divide it a bit from the sides usually
padding X of five we're going to give it a Flex container like this where we want to
justify the items between and items Dash Center to vertically align them in the center of
the screen more importantly we want to make our inner nav a class name of flex which will immediately make all of the
items go one next to another we also want to give it a w full so they span across the entire screen and we also
want to give it a screen Max width so this will ensure that it takes the full width of the
screen now if we go into our index.css and at the top search for this screen Max width you can see that I misspelled
it as scrim so let me just say screen Max width and that way this will be applied and it will give it a Max width
of about 1, 120 pixels now let's style our class items by giving them a a class name of flex
that's going to make them go one next to another Flex off one justify Das Center and on Max Das small devices so devices
with a width of 600 and less we want to make it hidden because they don't all fit right so we can eventually expand it
but for now we cannot fit them all also it's not good to have this content right here and mess the content with the
actual jsx which we're presenting so with that in mind we can import this content from the constants by saying
import nav lists from SL constants that way instead of rendering this entire array here we can simply say
nav lists. map and this nav lists contains store Mac iPhone and support we cannot see it now because we have hidden
it on small devices but of course if we expand it you'll be able to see that it's there of course I need to zoom out
quite a lot there we go and go back great now let's style each one of our list items by giving it a class name
equal to padding X of five and it might be good to come back to larger devices so we can properly see it there we go
that's more Apple like let's also give it a text- smm so a bit smaller cursor Das pointer so we know they're clickable
text- gray there we go because we don't want it to take away from what's in the
middle of the screen we want them to buy the items on Hover we're going to make it a text of white and we're going to
give a small transition Dash all so that it nicely animates as you hover over it there we go
that is looking great now we can go back to a smaller screen and we can style the icons to the right side by styling this
div and giving it a class name equal to flex items Das Baseline gap of seven in between the elements on Max small
devices justify end and on Max s devices again Flex of one so this is going to make the items nicely appear on the
right side of course if you want to you can also add a hamburger menu but for now this is looking good to me and of
course it looks great on desktop devices as well this was just a super quick knv bar which means that we're now ready to
start focusing on the hero section so let's hold our Command or control and then click into the hero section to
start developing it our hero section will be just that a section so we can write section and then we can also give
it a class name of w- full for full width nav height which will make it come below the nav bar BG of black and
position of relative now within this section we're going to display a div with a class name equal to
h-5 over6 so this is a height of about 83% as you can see right here I can see it because I have a Tailwind CSS in
extension installed if you don't see this you might want to install it but now what does this mean you can see that
now it pushes the highlights below which means that the section is taking the full height of the screen let's also
give it a w- full a flex Dash Center and a flex Dash Co so the elements appear one on top of another within it let's
render a P tag and let's make it say iPhone 15 Pro there we go in the middle minimalistic
as Apple does it and let's give it a class name equal to Hero Das title now as you can see this hero title got
completely hidden away as soon as we added this class name why is that well if you're wondering what are the Styles
applied when we give a specific Tailwind class you can always copy the name of that class and then head over right here
paste it and find it within the index.css and here you can see that we are applying some things like text
Center font semi bold text 3 XL to make it bigger text Gray 100 but hey what is this why are we applying an opacity of
zero why are we hiding it well looking at the deployed site might give you a hint let me reload so you can see it at
the start the text was wasn't there but then it popped up that means that initially we want to hide this piece of
text but then we want to animate it in so let me teach you how to do that using Gap Gap is probably the best and a
widely robust JavaScript animation Library built for professionals let's scroll down a bit to see what they're
about they are going to allow you specifically yes you you because you're watching this video to effortlessly
animate anything JS can touch smooth performance and you can focus on the fun stuff IIM made literally anything it
looks great it feels great I think I'm sold right let's see how we can use it back in our code we can open up our
terminal split it to get a new one and run mpm install Gap as well as ADD gap for SL
react and press enter this just installed all the necessary libraries and it allowed us to import two things
import Gap from Gap and import use Gap coming from Gap SL react now right at the top we can call this use Gap hook
like this just as you would call any hook and to it you provide first of all the function containing your animations
and then dependencies when it is running so here we're going to have a callback function and then as the second
parameter we'll have an empty dependency array within here we can then use the base
Gap to animate something specifically we're going to say gap. 2 so we want to animate two specific values we know what
the default values are right right the text or rather the lack of text right here as the opacity is zero so given
that we know that fact we can now Target this element by a class or an ID and then give it a new value that it will
animate to so in this case I'm going to give this P tag an ID equal to Hero but you might as well Target it by a class
name if it's Unique then say hash hero as it's an ID and then here provide an object of the properties you want to
give it once it animates so that's going to be in our case opacity of one now if I save this you can see how
it nicely animated it was very quick though but you can also play with the delay or the duration in this case let
me set the delay to something like 1.5 seconds so if I do this you will notice that once you reload there's nothing for
one and a half seconds and then it animates in and with that my friend you have just implemented your first Gap
animation pretty simple right trust me we're going to get into much more complicated GP animations but be happy
that you've done your first one for now now the second thing is to get this video playing right here and yes it's a
video although you would never assume so so going below our P tag we can render a div and that div will render a class
name equal to on medium devices width of 10 over 12 which is about 83% and usually width of 9 over2 which
is about 75% within there we can render an HTML video tag like
this and we can give it a source this source is a self-closing tag that will have an SRC property of
video SRC like this which we can import at the top by saying import Hero video from do utils as well as small Hero
video why small well that's because we'll have two versions of the video depending if we're on desktop device or
tablet or if we're on the phone so let me show the that to you in action we can reload this screen and you can see that
here it's very small and narrow perfectly fits in your phone but if I go to a desktop device and reload you can
see that here we have a horizontal iPhone turning around how cool is that exactly like on the Apple website so to
achieve that we first have to figure out the width of the screen and for that we can use a use State hook and we can call
it video SRC so video SRC and set video SRC exactly what we have here under the source then here we want to figure out
what is the inner width of our window and we can do that by saying window. inner
width and if it's lower than 760 pixels then we make the video Source State equal to small Hero video else we make
it just a typical Hero video and of course we have to import use state from react now if we go back to our current
application and reload you can see that something happens in the middle but not too much happening yet so let's also
give it a type equal to video SL MP4 and in the video tag let's give it
auto play muted as we don't want any sound plays in line will be set to
true and we want to give it a key to this video which will be equal to video SRC if I save it you can see the video
started playing and it doesn't even look like a video we can also give it a class name equal to pointer-events
dnone so nobody can click it or mess with it so now if I save it looks great but if I expand my screen oh that's not
good right so let's make it dynamically modify the video depending on the width of the screen we can do that by saying
const handle video Source set like this and this will be a function that's simply going to look into the inner
width of the window by saying if window. inner width is lower than 760 pixels then we want to set the video source to
small Hero video and else we want to set the video source to be the big hero video or just
Hero video and now we can connect that to our use effect so let's create a new use effect hook like this with an empty
dependency array and don't forget to import it at the top then we can say window. addevent
listener and we're listening for resize of the screen and whenever we resize we want to call the handle video set source
and in react it's very important to clean up your event listeners as well which you can can do by returning a
function inside of which you can run window. remove event listener resize and that's going to be the handle
video Source set like this so this way you can notice that on big screen we're playing this wonderful looking
horizontal iPhone and on smaller screens we're playing this great looking smaller screen iPhone how cool is that so now we
can collapse these functions and we can scroll down to start adding our cold to action button to actually purchase this
gorgeous iPhone so let's head below the video and Below two more divs and let's create a new div which will have an ID
equal to CTA as in call to action and it will have a class name equal to flex flex-all so the elements appear in a
column items D Center to Center them on the screen opacity of zero and translate D
y-20 I think you already know why I added the opacity zero right we're going to animate it and I also added the
translate for the same reason this is simply going to translate it by 80 pixels to the bottom on the y axis so
later on we'll be able to animate it so that it flies up the screen so within this div we can create an anchor tag
that will have an href of hash highlights it's going to lead us to the Highlight
section and we can give it a class name equal to BTN here we can simply say buy now of
course we cannot see it yet and below this one we'll also add a P tag that will say from 199 bucks per month or 999
if you're purchasing right away we can give this P tag a class name equal to font
dormal and text- excl to make it a bit bigger but now I have a small task for you figure out how to animate this Viv
including the anchor tag and the P tag hint it's going to be happening within our used gab hook so pause this video
and give it a try what we can do here is once again call the gap. 2 function and then Target the
# CTA ID and we want to animate to the opacity of one and we want to also push it a bit up
by giving it a y AIS of minus 50 which will push it up and we can also give it a delay so that it happens hand inand
with the animation on of rh1 so we can say 1.5 seconds now you can see how both the
iPhone 15 and the buy button and the text animate in at the same time with the video so let's reload and see it all
in action the video shows up and then iPhone reveals itself and then we have the pieces to buy the text but hey we
don't want to buy it before we can actually see the iPhone right so let's bump up the delay to about 2 seconds
save reload the video shows up the iPhone comes up and then at the same time the
buy button and the iPhone text shows up how cool is that I'm very excited let's also check this out on the entire screen
by reloading checking it out and there we go the text is here as well who would
have said we have already developed the Animated Hero section of the Apple iPhone website but of course don't go
just yet as we have many more interesting things coming up such as this cool section where you can actually
move through different sections playing different videos and it all feels like it's a part of the website you can also
go down and then see the entire 3D model and you can move it around also you can change the colors and you can get to to
a bigger screen version as well how cool is that so with that in mind let's focus on developing the second section of day
which is this animated highlights section I'm going to bring my browser once again to the side close the hero
and the index and navigate into our highlight section to get started with our highlight section we can turn it
into a section and give it an ID of highlights so that we can can then scroll through it from our homepage that
way if we click buy later on we'll be able to see the Highlight section now let's also give a class name
to this section of w- screen which will give it a full width let's give it an overflow of hidden so we don't see any
scroll bars h- full for full height common Dash padding for paddings and BG D zinc for this nice looking background
color it's very similar to this full black but just a bit different let's create another div right within this
section with a class name equal to screen Max width and within it let's create another div that will contain our
H1 so here we can create an H1 with an ID of title so we can soon enough add animated let's give it a class name
equal to section dashe heading and let's say get the highlights of course if you buy the iPhone you get
the Highlights so we cannot see it right here as right now if we check the section heading in the class names
you'll notice that we apply the opacity zero and translate y20 so it's actually hidden so to get it showing you know
what we need to do we can use the use Gap hook like this from react call it provide a callback function and an empty
array then we can say gap. 2 where we animate a title and we animate it to the opacity of one and a y of zero to
reset its position and of course we can import Gap from Gap now if we save this you can see how it
not only shows up but it also comes from the bottom to the top great now we can style this div within which we have this
H1 by giving it a class name of MB of 12 for margin bottom we can also give it a w- full items Das end and justify Dash
between this way we'll have the highlights on the left and something else on the right
especially on bigger devices what I'm referring to are these two little pieces of text on the final
website which is this watch the film as well as watch the event links so let's add those by creating a new div directly
below the H1 that div will have a class name equal to flex flex-wrap items Das end and a gap of
five directly within it we can render a P tag and this P tag will say watch the film and then we can give this P tag a
class name of link and right below the text still within the P tag we want to render an image this image will have a
source equal to watch IMG coming from utils with an ALT tag of Watch and a class name equal to margin left of
two now if we save this and go back to our current version of the application we cannot yet see it as we're not really
animating it in so what we can do is also run the Gap do2 this time we're going to go for the
do link class name and we're going to animate it to opacity of one y of zero and save once we do that you can see
watch the film here now directly below we're going to duplicate it and create another link that's going to say watch
the event and here we can say write IMG coming from utils and this will be right now it looks like it comes below the
highlights but we want it to appear on the right side so what we can do here look looking at this div it looks like
the items end and justify between properties haven't been implemented because it doesn't have the flex display
so we can say on medium devices and larger we can give it a flex display and this will make the links appear on the
right side but now pay close attention you can see that they all appear at the same time whereas on the Finish side you
can see how they slowly animate in one after another so to achieve that look and that animation we can give it first
of all a duration of one to increase it a bit and then a stagger of
0.25 stagger will ensure that they only stagger one after another all of which have the same class name or the same
selector in this case that link we have a link here and we have a link here so now if we go back you can see highlights
first then the film then the event great so with that in mind the top of the Highlight section is done but of
course now the fun part begins we can focus on the video carousell component something that you don't really see
often so to create it let's go below the speed tag and Below two more divs and create our own custom video Carousel
component by going to components saying video carousel do jsx run
RFC and then go back and render it right here video Carousel and self close it of course we have to import it at the top
by saying import video Carousel from slide Carousel now you can see it right
here I'm going to collapse it as we'll be doing it for mobile devices as well which means that we can now dive into
the video Carousel and start implementing it this one will be quite complex trust me we're doing quite a lot
of stuff here a lot of animations and a lot of different videos which we need to scroll through at the same time so let's
first wrap everything into an empty react fragment and then have a div right below this div will have a class name
equal to flex and items St Center immediately within it we want to map over all of our highlight slides by
saying highlights slides like this coming from do do/ constants so if you look into it you'll notice that this is
essentially an array of a couple of different objects each one of which has an ID a list of text elements such as
the chip the video and the video duration we'll use all of this information to display all of these
videos one by one and it's not just the video it also has text that we show alongside that video so we want to map
over those slides and then for each one we get a list also an index and then we
automatically return something when I say automatically return I mean just have parentheses here and not currently
braces that means immediate return for for each one we want to return a div and we want to close it that div will have a
key equal to list. ID and it will have an ID of slider within it we can have a
div which we need to close and it will have a class name equal to video- Carousel underscore
container and we can save it so now if we scroll down we we cannot see anything yet but if you type something within it
you can see test test test test which means that we are properly displaying four different
slides now where we have the ID of slider we also want to give it a class name equal to on small devices padding
right of 20 and usually padding right of 10 that's just going to provide some spacing so you don't see the other slide
while the first one is not completed within this div we can run another div and that div will have a class
name equal to w- full h- full Flex D Center rounded -3 XL overflow hidden as we don't want to see those ugly scroll
bars we'll animate it to slide over different ones and then BG of black now you can see this black rectangle where
the video will go so right within this div let's create a video tag that will have a source within it like this and
that Source will have the SRC property of list. video with a type equal to video/
MP4 and now we get our first video right here but of course a video cannot yet play we need to make it play
automatically so let's give a couple of properties to this video such as ID of video so we can later on target it plays
in line like this will be set to true we're going to also give it a preload class of
Auto muted and that's it for now now as you can notice it's not going to start playing yet that's because
we're want to play it using some animations more on that later but for now let's create the layout around each
one of these slides we're going to go below below this video and Below one more div and create one last div that
will make this slide this div will have a class name equal to Absolute because we want to absolutely position that text
on top of our video with a property of top 12 left Dash inside of square brackets
5% and the zindex of 10 within that div we're going to map over our list texts by saying list do
text lists. map where we get each individual text and for each one we automatically return a P tag with a key
equal to let's do text and it's going to display text you can see enter A7 Pro
gamechanging chip groundbreaking performance let's style it a bit by giving it a class name equal to on
medium devices text- to Exel usually text- excl and f- medium there we go this is how it looks like
it's going to be much better once we actually start playing the video too first I'll create some refs or
references to specific elements so we can keep track of the video we're on I'm going to start by saying
const video ref is equal to use ref coming from react and at the start it will be set to an
empty array I will repeat this two more times call the second one video span ref and the third one video div ref we'll
have to manipulate and play with a lot of these different elements now that we have these refs
let's also create a state for the video by saying use State snippet and call it video and set video and at the start it
will be equal to an object don't forget to import Ed state from react this video will have some
properties like is end at the start set to false start play at the start set to false as well right the first one is not
yet playing we have to figure out which one is playing so for each video we'll have to know when to trigger this and
when to turn it to true then we have the video ID at the start set to zero is last video will be
set to false at the start and then is playing at the start set to false we'll need answers to all of these questions
and periodically we'll have to switch it on or off now just so it's easier to work with these pieces of data we can
immediately destructure those values by saying const and then getting the is end is last video start Play video ID and is
playing which is equal to video we have essentially just extracted those values or destructured the values so we can
then use them without saying video dot video dot video dot great so now let's create a use effect so we can start
playing those videos this use effect is of course coming from react so we have to import it and it will be a callback
function and we of course need to have a dependency array we will recall this use effect whenever the video ID changes or
whenever the start Play variable changes so what will we do in this video well first we have to figure out where are we
in our video playing Journey so we can say const current progress is equal to zero and then we have to get the span
element of the currently playing video by saying let span is equal to video span
ref. current more on that later finally if we have that span of a specific video ID then we can start animating it here
we'll animate the progress of the video remember for what that is if I go to the
finished website and reload you can see how nicely this progresses for the entire video duration and then opens up
a new one so we'll do that soon but first let's just focus on getting the videos to play in the first place to do
that we can say let anim which is short for animation is equal to gap which is coming from Gap dot 2 where we get a
span and then the video ID of that span and then as the second parameter we provide all of the options as you know
options don't necessarily have to be just properties that we animate like opacity or Y or xais it can also be
specific functions like on update what will happen once the animation updates which is a callback function the second
one we can have is oncomplete what happens if the animation is complete in this case since we're
working with a much more complex animation we need to keep track of all of these
values but this animation specifically has something to do with the progress tracking which we're not doing yet so
we'll get back to this very soon for now let's create another use effect on top of this one and this use effect will
deal specifically with the playing of the video so it can be retried whenever the start Play Changes whenever the
video ID changes the is playing or the loaded data that loaded data is a new use State Property which we can create
right here use State snippet loaded data set load the data at the start equal to an empty array more
this soon so what are we going to do in this use effect well here we can say say
something like if loaded data do length is greater than three then if not is playing we can say
video. current video id. pause so if we came to the end and if we're no longer playing then pause it
else if his playing is true then if start Play is also true then then video. current video id. playay so we find a
specific video we want to trigger and we play it this by itself won't yet start our first video and that's because we
haven't yet given it any refs so moving right here below to our video we have to give it a couple more properties first
of all let's give it a ref equal to here we're going to have a cook function where we get the element
and we want to say video ref. current I is equal to element like this so we're finding a specific index
in the video refs array and setting it to this current video element then on Play We want to call a callback function
where we want to set video where we get previous video information so prev video and then based of that information we
automatically return so you have to do parenthesis and then curly braces the spread of the previous video
so dot dot dot prev to get everything we have there but this time we turn on the is playing to be equal to true so on
play we spread all the information about the video but we said the is playing the true I know this is getting a bit more
complicated so if you have already already gotten stuck or if something is not clear don't worry you can code it
with me if it doesn't work like it should at the end of this video Carousel portion I will also provide you with a
complete code for the video Carousel so even if something breaks during the process you still got to watch it learn
from it and then you can paste it have it working and then explore exactly what we're doing now that we have only the
video it's kind of hard to see when the next one should be playing or what's happening with the video duration so
what do you say that we also implement the jsx for this progression tracking and this button too which we can use to
pause it and then we can continue with getting the video to play so with that in mind we can scroll down go below here
where we're rendering text go all the way until the bottom and then here we want to create another div this div will
have a class name equal to relative Flex Center and a margin top of 10 to divide it a bit from the content above
within it we want to have another div that will have a class name equal to flex Das Center padding y of five
padding X of seven background gray of 300 backdrop of blur and rounded Dash full this will create this rectangle
pill like sh shape so we can track our progression within there we want to go video. current. map where we get each
video but we don't need to do anything with it so we can just call it underscore as it's usually the practice
and then you can also get the index for each one of these video refs we want to immediately return a span
element and that span element will have a key equal to the index and we also need to give it a ref this ref will also
be equal to a cack function where we get the element and then we set video div ref. current index is equal to element
so we're going through a couple of these and whenever we have a new video we add it to this video div ref array we also
want to give it a class name equal to margin X of two to create some margins W of three for width h of three for height
you can see how the pill is changing shape BG gray of 200 there we go now we have four of these different dots
rounded Das full relative cursor Dash pointer within each one of these we want to create another
span element that span will have a class name equal to Absolute so it will be
absolutely positioned h- full for full height w- full for full width and rounded Das full to this one we also
want to give a similar ref as to the above one so we can simply paste the ref and change it from video div ref to
video span ref and we can self close this span right here there we go that should look
something like this wonderful and finally let's create the button to pause or reset it like we have it right
here while it's playing you can pause it and else if it's done you can reset the progress so going below this pan and
Below one more div we can create a button that will have a class name equal to
control- BTN you can see the button appear right here we can render an image and IMG tag right within it with a
source equal to if is last video then we render the replay IMG coming from utils else if it's not playing so if not is
playing then we render the play image else we render the pause image so we're using a double Turner right here for the
alt property we can check if it's the last video then we say replay else if it's not is playing then we say play
else we say pause so this little button has three different functionalities play pause and
replay and let's give it a Click by saying on click we want to check if it is the last video then we can call a
callback function which we will call handle process and pass it a string of video
reset this will temporarily break our application but don't worry about that then if it's not is playing like this we
can then call another callback function calling the same handled process function but this time with a play
action and for the last time we're going to call the same handle process but this time with the pause action so now you
might be wondering what is this special handle process function and from where will we import it well this is a
function which you will create so let's scroll up right here and let's create it right below this use effect by saying
const handled process which accepts the type of the action we want to make in the index and we're going to open up a
switch case so I like to use a switch whenever there's multiple cases sot switch based off of the type of the
action and if the case is video end in that case we're going to set video state by getting the previous video
State spreading it out by saying do do dot previous video is end whe set to true and we're
going to increment the video ID by by setting it to I + 1 looks like I missed a closing Arrow
right here there we go now we're good so this is if we're at the video end and don't forget the break the second case
is the video last so if we're the last video but not yet end here we also want to call set
video and we're going to also get the previous video which I guess we can shorten for just pre
previous video right here pre and then we want to immediately return something by first spreading the previous video
and then adding the is last video to true because it's the last one of course don't forget to break
it let's add another case which will be video reset where we can also basically duplicate this set video and instead of
saying is last video we will say is last video is false and we're going to reset the video ID to
zero let's break it and finally and most importantly we have a case of play so we want to play the video and here we can
again duplicate this set video paste it below and say is playing will be the opposite of previous that is
playing and that's basically the only thing we want to do we want to break it and at the end default we can return
the video so here we handled all of the different actions that we could ever do with that video that is great but still
not a lot is happening in the screen right right so let's use Gap to bring it all to action right at the top after we
destructure everything from our video we can use the used Gap hook like this coming from Gap react where we provide a
callback function and here in the dependency array we're going to add the is end and the video ID so that we
update stuff whenever the video ID changes here we want to animate the video to play when it is in the view by
saying gap. 2 we're going to select it by the ID of video and we want to provide it a couple of things first
we're going to provide it a scroll trigger like this so once this thing is in view then we trigger it once what is
in view well the video ID what do we do then well toggle actions so do something and we're going to say restart the video
this is when it first comes into the view and there are many different cases when it comes to the view from the top
or from the bottom in other cases for now we're going to say none none none we're only interested in making it play
once it first comes into view after the scroll trigger once we're complete with the video we want to have a callback
function and we want to set video to be equal to where we get the previous one immediate return do do dot pre by
spreading the previous state start Play will be set to true and is playing will also be set the
true now if we save this this by itself won't yet start playing the video which is fine but what will is the use of our
loaded data so the reason why we have this loaded data is because here in the original use effect we are checking if
the loaded data exists and only if it does only then do we start playing so what we have to do is create a new
function right here const handle loaded metadata equal to and this function will accept the index as well as the event we
pass into it and and it will simply call the set loaded data it will take all the previously loaded data so
pre and it will automatically return an array do do dot pre and then the new event we have added with this handle
loaded metadata we can now attach it to our video player so going to the video we can say
unloaded metadata and basically what this means means is this will get triggered with the event once the
metadata of the video has loaded so once it got the data we get that event and we call the handle loaded metadata and pass
the index as well as the event this Handler will call this function which will in return call our state Setter and
once it's in there this use effect will trigger the video play so let's save it and
reload and as you can see the video is now playing let's check that out one more time on full screen so if we go
here on full screen you can also see the videos which will soon play so let's reload and there we go the video starts
playing but nothing is happening with the animation here and once it ends we're not seeing the next video so let's
make that happen as well and once again if if you reach this point and if you're watching this and if this part is very
confusing don't worry about it at the end of this entire segment I'll provide you with the entire video Carousel code
so you'll be able to continue having the complete working code but still you will have learned a bit about how setting up
more complex video animations works now that we've got our first video playing let's also implement the way to track
the progress of that video we can do that right here I believe we even added a comment for ourselves there we go
animate the progress of the video so here we can say cons progress is equal to and we can use the math. seal method
and then get the anim do progress so this is directly built into gap which allows you to get the progress of the
animation how cool is that and then you can multiply that that by 100 to get the percent we can say if progress is not
equal to current progress then we want to update it by saying current progress is equal to
progress and of course this current progress has to be a let and then we use a gap. 2
functionality where we target a specific video div ref which is the container for this dot which will soon turn into a
progress Handler dot current of the specific video ID and then we're going to modify its width by saying width is
window. inner width is lower than 760 if that is the case then we're going
to make it something like 10 VW for mobile else if window. inner width is lower than
1,200 then we're going to make it something like 10 VW that's for tablet and else for laptop
we're going to make it for VW so if we do this you can immediately notice how it expands which is great but
still we have to animate the progress bar so let's go below and say gap. 2 span of that specific video ID we're
going to modify the width and we're going to do it dynamically by setting it to current
progress and then percent like this and we're going to change the background color to white so if we now save it you
can see how nicely if you reload it animates and moves further of course now it only lasts for the duration of the
animation which is a couple of seconds but now we want to actually modify the duration to be exactly as the duration
of the video so let's navigate to our oncomplete and check if is playing in that case we want to say gap. 2 video
div ref. current video ID we're going to modify the width to 12 pixels so only if it's playing its 12
usually it's going to be just a DOT so on complete we bring it back to a DOT and also gp. two we're now targeting
the span of a specific video ID like this we're going to change the background color to be equal to Hash AF
AF AF of course we must not end this right here we must end it right here below so if we now save it you can see
how quickly it starts and then gets back let's go below this on complete so that's going to be all the way here and
also exit this animation so exit curly brace and parentheses here want to say if video ID is equal to zero then we
want to restart the animation by saying anim do restart so this is a utility Handler
given by gsap and finally now we want to modify how long the animation lasts by saying
const anim update which is going to be used to update the progress bar here we can say anim
progress and bear with me we'll have to do some math we have to get the video. current video ID divided by
highlights slides video ID do video
duration so we divide those two times and that should give us the progress then we go down and say if is playing
then gap. ticker and ticker is used to update the progress bar do add anim update else if it's not playing gap.
tier. remove anim update and this anim update is coming from here but it needs to be one scope below so we
can actually access it there but here we cannot access the anim as I can see so we have to move all of this a bit to the
top right here so there's one closing here and then we have these two so now if I save it you can notice how it
expands and it's immediately all white and it stays that way of course this is still not the the same as on the
finished website where it slowly does it and then moves on to the next one so let's fix it one thing we have to do is
we have to give this video a few more Properties or rather a few more handlers we have to know when will it end so we
can say onended we're going to open up another callback function and we're going to check if the
index is not equal to three well in that case it's not yet the video end so we can call a Turner operator and call the
handle process where we pass the video end and then a specific index so we know which
one will we end and if we are at the third one we call the handle process and call it a video last so we know that we
need to restart in this case this can be an immediate return so we don't need curent
cly braces here at all and of course we don't need three equal signs if it's inequality if we save it now we should
be much closer to actually transitioning to the second bar which you can see it actually opens and it should go to the
third one as well but the videos are not yet reflecting that so let's go back to our animations right here to use
Gap that is here at the top and we're going to add a second Gap animation by saying gap. 2 and here we want to Target
the slider I believe we have given it an ID of slider yes you can see right here and we will modify it like this we will
apply a transform property where we will use the translate X and then we're going to make this a
dynamic template string and we're going to move it by minus 100 times the video ID so for each video the
first one is going to be zero so it's going to stay there for the second one it's going to be 1 two 3 and as we
update this video ID we're going to modify the percentage of how much we move away so that way new videos will
start showing up and of course we have to properly close it if we save this we're getting closer
but not there yet we can also modify the duration to two seconds and save it and reload we get the first video playing
it's looking good the second one but still nothing happens in this case we want to use a special ease function and
what are these ease functions well ease functions allow you to specify how you want your animation to happen it can
happen faster at the start and then slower at the end or you can give it some power at the start you can also
make it elastic like this you can play with it and do whatever you want quite interesting right in this case I found
the power two do inout to work the best with our video specifically great we're getting very very close to having all of
this working and I think I know why these are not getting properly cleared if we
scroll down all the way to where we're updating the specific animation here we are dividing
just the video ref with the video duration doesn't make too much sense what I wanted to do instead is get the
current time of the video and then divide that by the video duration only like that it will actually be able to
know how far did we come so if I reload now you'll be able to see that our video tracking progress update are now working
properly they go one to another and soon we'll be able to stop them as well and the reason why we're not moving from one
video to another right now believe it or not it's a simple typo instead of saying percent and then closing the parentheses
right here I first closed it and then added a percent sign so if we just modify these to and the reload the
screen we get the first video playing the progress works and then it still fails because I have an extra curly
brace so if I remove that extra curly brace now it works as you can see it goes to the last one it allows us to
reload or restart from scratch goes first second the pause doesn't work right now so let's fix it we are pausing
within our handle process where we have the play but I don't see the pause right here so basically we have to copy this
play and I think just renaming it to pause should do the trick because we're doing the toggle right
here is playing or is not playing so if we reload so if I click pause it works and I continue this looks
and feels wonderful it's not just the videos that make it special it's the pieces of text it's the
progress bar loading and this button right here one last thing we can do is I noticed that for some videos we need to
apply a specific class name right here so we can say class name to the video where we open up a dynamic block
of code and say if list. ID is equal to two then we can translate
x44 and close it and we don't need the other so we can just do the end end we can close it and then we can also say
pointer events none like this there we go if we now save it this looks great and as I promised if this is still not
working for you the full video Carousel will be added to that read me so you can copy it and override this code right
here if it didn't work before then it definitely will work now so with that in mind let's expand it to admire it in its
full Glory I'm going to scroll all the way to the top to check out our hero and now I'm going to bring the videos in
view it nicely opens up we get the second video playing too we can pause it we can play it it's going to go to the
third one and finally we can also restart start how cool is this I know I know
this was a more complicated one quite a complicated component to implement 200 lines of complex Logic for
video refs getting the progress bar to working and more but you got it even though it might not have worked at the
start you understand at least a bit better of how you would approach something as complex as this and that
counts too so great job on completing the video Carousel and with it completing the highlights section of the
video I hope you're excited for what's to come because the next thing is going to be the 3D model look at those
Reflections look at the Quality you can change the color and don't forget you can change the size so let's go ahead
and implement the 3D model next using 3js oh and just so I don't forget if you didn't download it at the start we have
created a special 3js and GP guide specifically I'm referring to the GP part right here if what you have now
developed was a bit tough and complicated I strongly advise you to just go through the guide take it step
by step and take the base Concepts in so then everything else will start making so much more sense I have also included
some special tips and tricks in there so GP and animations will be simpler for you in the future great with that said
let's get back to the 3D model so to get started with our 3D model let's create a new page right here or a new section
called Model like this and of course we have to import it from somewhere so let's import the model from components
model and of course therefore we have to create it right here by creating a new component called model. jsx with our R
afce right within it now back on our Local Host 5173 we can see the model right here at the bottom which means
that we can get started with developing it we're going to start as we usually do by turning this div into a section and
we're going to give it a class name equal to Common Das padding which will apply some common padding on all the
sides we can also create a div that's going to have a class name equal to screen- max-width
and within it we can create an H1 that will have an ID equal to heading because we're going to animate it very soon and
it will have a class name equal to heading or rather section dashe heading 2 and it can say something like take a
closer look look at what you might be wondering well of course our model now we cannot get see this piece of text
right here and that's because we will animate it using Gap so in this case we can scroll all the way to the top of
this model and say use Gap coming from Gap react which we simply call like a callback function right here and we can
specify an empty dependency array right here we can simply say gap. 2 of course Gap needs to be coming
from Gap and we can Target The Heading as we're used to and then we can also create a
new object saying what we want to animate we want to make it come to the position of zero and bring the opacity
to be equal to one that way our header will be showing there we go take a closer look now we can scroll below this
H1 and create a div which will serve as the container for our model so let's create a new div that will have a class
name equal to flex flex-all as we want the elements to appear in a column items Das Center and margin top of five right
here within that div we can create another div that will have a class name equal to w-o for full width h-
75 which will look something like this for the height and you want to specify by 75 VH which means vertical height so
that's about 34s of the screen on medium devices we can increase that a bit by saying h-h and that's going to be 90 VH
like this overflow hidden so there's no scroll bars and relative like this now we cannot yet see anything in there but
we have created some space to present our model within so to create our model or to show it we have of course will
render another custom component so we can separate the logic of the model of showing that 3D model from this entire
section so what we can do is create a new component called model view djsx and for now we can simply call rafc within
it and now we can use that model view and render it as a self-closing model view component like this there's two
different iPhones that we want to show here there's going to be the small one and the big one as you can see right
here and we'll want to render both of them so for that reason what we can do is we can immediately create references
for each one so let's go to the top and let's create a new use State snippet and this one will be size set size like this
at the start equal to small so we need to be able to choose which one we currently want to look at and of course
we need to import use state from react then we also want to get all of the other model details for the small or
the big so we can create another used State snippet and say model set model and at the start it will be equal to an
object containing the title which can be something like iPhone 15 Pro in natural titanium we can also specify different
colors so we can do that an array of hash 8 f8 a81 like this we can also do something
like hash ffe 7B 9 I found those values to work the best and hash 6f 6 c64 just so we have some colors to
choose from and and we can also specify the image this one will be yellow image which is coming from the utils this is
just the default state which we'll be able to modify later on and of course this model has to start with a lowercase
M like this now there is still a bit of setup we have to do we have to set up camera control for the model view so we
have to specify camera controls as well which is interesting and we will do them like this cons camera control small will
be equal to use ref coming from react and we will duplicate that and create a camera control large we need to have a
separate camera ref for each one of these two models and of course we use the size to Simply say which one are we
currently viewing here we specify the model details these are the different colors
for different meshes which we will use within our 3D models and here we add the texture which we're going to use you can
see how it looks like here it's a JPEG but it doesn't allow me to currently view it but you can view it within your
just typical finder or file explorer so with that in mind we have created camera controls for this model
view and also we need to create another ref to keep track of the model to access its properties when animating so we can
do that by saying cons small is equal to use ref where we can say new 3. group like this so you're creating a
new group of threejs elements and this three of course has to be imported from somewhere so for the first time in this
video we have to install 3js let's do it by saying mpm install three as well as ADD react D3
SLR and add react D3 SL fiber these are utility helpers that make the use of the 3js library
easier for react applications so let's simply import this three coming from three we can do that
at the top by saying import everything as three from three there we go and we can duplicate this and also
create one for the large model another thing we'll have to keep track of and I know there's a lot of things to keep
track of so this is the camera control this right here is the actual models themselves and then we need to keep
track of the rotation value of each model by saying const we can say small rotation set small rotation is equal to
use State and at the start it's going to be set to zero and we can modify this as well and set
large rotation as well so large rotation and set large rotation we have to keep track of the values of both of these
models at all times so that if we rotate it we can see and know where it is go back bring it to zero and then show the
other one great so this was a bit of a setup I know but now we can can actually pass all of the important information to
our model view which will soon render that model so let's pass it the index this one will of course be one because
it's the first one to show let's give it a group ref which is going to be equal to small a gap type
of view one so this is the first one control ref for camera control of camera control small set rotation state is
going to be equal to set small rotation and finally we want to pass the item which will be equal to the model
this model is universal as it can be a big one and a small one depending on what we choose in the state and also we
want to change the size right here by tracking what we have in the state great now if we go back to our current
application we can still see just the model view nothing more so now let's duplicate this below and let's make it
work for our big one by saying index is 2 large vi2 camera control
large also set large rotation and then the model and the size can remain the same now we can see two
model views and soon enough we'll render those models but for now we first have to show the canvas
so going right here below we can render a canvas component coming from react 3 fiber and within it we can render the
view. port like this and this view also has to be imported from react 3 Dray viewport is a way to render multiple
views of a model in the same canvas doing this will allow us to animate that model now let's also make this canvas
full screen so we can give it a last name equal to w- fo and h- fo and we can also style it a bit by giving it a style
property that can be position is fixed top is zero bottom is zero left is zero and right is zero with an overflow
of hidden there we go so we're just resetting the positioning and later on we'll also need to get access to the
event source and you can do that by saying Event Source is equal to document. getet element by
ID and we're going to get the root right here this is useful when you want to interact with the model that you work
with now this temporarily broke our application so let's go to inspect and go to the console and you can see right
here it says unod type error document. getl elements is not a function this right here should have been get element
by ID as it can only be singular so now we are back into the game before we start working on these 3D models let's
also show some additional information such as the title colors and the sizes that way as soon as we show the model
we'll be able to play with it and modify it so going back right here we can go below the canvas and below one more div
and create another div this one will have a class name equal to margin X of Auto and
w-o right within it we can create another P tag that will say model. tile and immediately you can see
iPhone 15 Pro in natural titanium and we can also style it a bit by giving it a class name equal to text Dash
SM font d light text- Center and margin bottom of five there we go that's better let's also create a div below that P tag
and that div will have a class name equal to flex Das Center and right within that div we'll have a UL that
will have a class name equal to color- container So within here we want to map map over all of our colors so we can
open up a new Dynamic block and say models. map and then to get access to these models we're going to import them
from constants and you may be wondering what is this well it's nothing more than an array containing a couple of objects
where each one contains some colors different meshes and also the title that is it so we want to map over those
models and get each individual item and then also the index of that color we're
showing and for each one we want to automatically return An Li which is a list item with a key equal to I a class
name equal to w-6 h-6 round-- full and margin X off two
and then most importantly we want to give it a style property of background color will be set to item.
color zero so this will be coming directly from that object within the models
array and then we can also give it an onclick so once we do that we want to set the model like
this and set the model to be that specific selected model be that blue yellow or whatever ever other color and
we can immediately close this li like this if we save it and scroll down you can see these four different beautiful
colors appear right here and we can also add a cursor Das pointer so that the user knows that they can actually click
it there we go it's not just for the show let's also go below this ul and let's create buttons for managing the
size we can do that by saying button with a class name equal to size- BTN Das container and within it we can simply
open a dynamic block of code and say sizes. map where we can destructure the label and the value of each size and
then automatically return a span that will have a key equal to label and it will simply display play
the label as well of course the sizes have to be imported from the constants now you can
see 61 and 67 let's style it a bit further by giving it a class name equal to size- BTN that's much better and
let's also give it a style property equal to background color and here if the size is equal to the selected value
then it can be white else it will be transparent that way we'll know which one is currently selected and also the
color if the size is triple equal to the value then it will be black because it has the white background else it'll be
white so now we can see which one is currently selected and let's also add an onclick where we can call a callback
function and set size to be that specific value so now if we save it you should be able to click and move between
those two states as well as select different colors and immediately see that the title changes as well that is
amazing but of course that's nothing without the models which are the main star of the show so we have to scroll
all the way up and navigate into our model view and that way we'll be able to make our colors and sizes make changes
to the 3D models will display on the screen so first things first let's accept all of the props we're passing
into this model view component we're going to get the index the group ref the Gap
type the control ref for the camera set rotation size the size and the item then we want to create a viewport for the
model by wrapping everything in a view coming from react 3 Dre each view has to have an index so we know which one it is
and we can pass it from the props the first one will have an index of zero and the second one of one we can also give
it an ID which will be a gep type also coming from props and let's also style our view a bit by giving it a class name
and just so we can initially see it I'm going to give it a border of two and border of red five 100 so now you can
see where it is positioned right now it is just a line in 2D but if I give it a w full and h- full it will take the full
height of the screen also we need to hide it if we're not showing it currently so we can turn this into a
dynamic template string like this and properly close it and only if index is equal to two then we want to
say right dash minus 100% that's going to look like this else nothing so this way we can
swap between the left and the right one next we want to play with the lighting a bit and the first source of light will
be the ambient light which lights up all the objects in the scene equally so we can say ambient light and we can get
give it an intensity of about 0.3 and right now it looks like it cannot properly read the light unknown
property intensity found but we'll fix that soon enough let's focus on what matters maybe even more than light well
who knows because we cannot have a camera without light right but still we need both the camera and the light to
get the picture so we'll use a type of camera that simulates the perspective of the human eye
and that is called the perspective camera coming from react 3 Dre like this we can give it a make default so
this is the default camera and give it a position that is an array of 0 04 so now we have the camera but it's still dark
we can then create some more custom lights by creating a new component called lights. jsx and from the read me
of this project you can copy the light jsx component and paste it right here you'll soon be able to notice that
this contains the environment and a couple of different lights we're using the reason why I
decided to provide these lights to you is because I was playing a lot with figuring out how to make this look the
best and with these light formers I managed to create custom lighting that are properly light up our 3D scene with
different lighting intensities positions and more and once again just to remind you more details on
these light formers custom lighting and 3D modeling with 3js in general you can find in the guide so with that in mind
it looks like that even though we have imported these lights right here still our 3js has some trouble figuring out
whether they actually work or not and this could be just an eslin issue so let's go back right here and let's call
our lights property like this by importing those lights at the top by saying import lights coming from slash
lights and if we scroll down it's still quite dark out there but don't worry as we'll figure it out soon right below the
lights I'm going to call a suspense which is used to provide some kind of a loader until the model loads like this
so you you can give it a fullback and then within the fullback you can define something that's going to load like
maybe a div that's going to say loading later on we'll make a custom loader but for now this will be good enough the
most important thing is now rendering the iPhone model now the way we can get to that iPhone is by checking out our
public models and then we have our scene. glb which contains the iPhone model now I have found found a
phenomenal GitHub repo that turns gtfs into jsx components so just click this link in the description and then simply
drag and drop your scene by revealing it in finder or opening in Explorer and then simply drag and drop it
in what it will do is it will automatically render it for you in the browser so you can check it out which is
very very cool for testing out different models but then you and more importantly what we care about is that it generated
an entire 3D jsx file with all of the meshes right here so let's simply copy that entire thing I think we can do it
somewhere here now here we can play with the model even more and modify the Shadows the types and stuff like that
but in this case I will just keep it as it is and import this entire thing copy it and I want to create a new
component called iphone. jsx and simply paste everything I have here don't worry about these eslint warnings for now with
that in mind let's go back to our code and right here within the suspense let's render this iPhone
model which of course has to be imported at the top by importing iPhone from iPhone
which will broke the screen because if you go into that iPhone you will see that right now it just exports it as a
function instead of saying something like function model and then at the bottom we can say export
default model that way we have something to import within our application and of course since it doesn't know where we
put this scene we have to say that it's coming from models scene. glb and if you scroll up in this same file you'll be
able to see that here we also have to say models scene glb if you do that our app is back to the working State and if
we scroll down you can see a very very tiny iPhone model in the middle I'm going to zoom it in and you can barely
see it so now let's actually play with the lights the positioning the camera and let's make it bigger I'm going to go
back to our model view right here close everything else so we can just focus on it but before that very quickly let's
get rid of these es lint warnings they're okay in this one but under lights they are quite ugly to look at
and especially within our iPhone model here they're all over the place so to fix it you can go into the eslint RC and
right here you can add a plugin called at react D3 and save it that way es Lin will
automatically know that these are real properties that exist and that you can call as you can see now it knows exactly
what intensity is and it's no longer complaining while we're here let's also remove this ugly border and let's make
this iPhone model the star of our show I'm going to wrap this entire suspense into something known as has orbit
controls which allow us to move the camera using the mouse so I'm going to say orbit controls coming from react 3
Dre and that will be a self-closing component right here so we don't yet have to wrap it but we do have to wrap
suspense within something known as a group so a group containing the suspense and the iPhone like
this this group will have a ref equal to group ref it will have a name if the
index like this is equal to one then it's small else it's going to be large and we can also give it a position which
is equal to an array of 0 0 0 to position the model in the center of the screen now let's play a bit with orbit
controls we want to make our orbit controls the default way of traversing over our model we want to give it a ref
equal to control ref we also want to give it enable Zoom to false as we don't want to zoom it in we just want to move
it around we're going to also disable panning so we cannot once again kind of move it
around and we're going to modify the rotate speed to about 0.4 so that it's slow and
steady then we're going to provide a Target to Target the center of the screen by saying new three and this
three has to be imported at the top by saying import everything as
three from three like this and now if we scroll down we want
to call it and construct a new Vector like this Vector 3 and give it 0 0 0 as x y and z a
so we position that in the center as well finally once we stop rotating we want to get the angle of the camera so
that we know where we are so we can say on end we can call a function that's going to set rotation
angle or rather set rotation State I think we called it to control ref do current dot get
aimal like this it's a weird one I know but we need to get this specific angle to know where we are in the space and
soon enough we'll be able to rotate our iPhone but for now let's scale it up so we can actually see it we can do that by
giving it a scale property and saying if index is triple equal to 1 then we want to say 15 15 15 else if it's the big one
we want to show we're going to make it 17 17 17 there we go that's more like it and you
can see that the orbit controls work because if we remove the orbit controls or if we comment them out you cannot
move it it stays stuck in place so these definitely come in handy and allow you to move the
model now we can also provide the item which contains all the information about that model and the size which also
contains the information about the size so this is our model as you can see we have it here and if you click here it
doesn't quite yet change the color but it will soon if we dive into it and create something known as a texture by
saying const texture is equal to use texture coming from react 3 Dre and we want to pass it props do item. image IMG
like this this contains the mesh we want to cover our phone with then we can use a use effect like
this and we're only going to call this when the material materials or the props that item change so once we make some
kind of a modification to these buttons that change the colors and the size within here we want to call the object
dot entries to get the key and the value pairs of all of our materials and we want to map over each one of these
materials where we get an individual material and then we check if that material exists we can check that by
checking if material zero is triple equal to a specific string that we can then compare with a specific color now
these are basically strings of IDs that contain about 10 to 15 characters and I definitely don't want you to type all of
these by yourself so in the read me down below you can find those five or six different material
colors as a matter of fact you can find the entire use effect belonging to the iPhone model so copy it and paste it
right here as you can see these are the material names that can be changed and they modify the color so material 1.
color is the new color coming from the props which we choose on the screen now we get an error because we didn't import
import use effect from react and we also need to import three so we can do that by saying import everything as three
coming from three and if we save that now we're back and would you look at that we have a yellow iPhone and it
actually looks quite good there we go we can change it to White and now our picker works as well this is wonderful
but we're going to make it even better if we go to the finished website you can see that on the blue iPhone we have a
very Vivid blue color if we change it to Yellow it is completely yellow and completely dark whereas on ours
currently yeah sure it does change a bit but for a white color it just looks too white right so what are we going to do
is take the texture from this image and then apply it to this specific front portion of the screen and the way I
found it is I use that same app that generated this jsx and then there I inspected to see which specific material
do I have to apply that texture to and you can use a control or command F and search for EI IU and find it here you
want to expand this mesh and then within it give it a mesh standard material with a
roughness of one and a map of texture that way we can apply black for black this one for the
white this one for the yellow blue and so on so you can see that now it makes complete sense and it looks much better
as we're applying a custom texture for each color great so with that in mind we can exit this iPhone and we can finalize
our model view by providing a proper loading because now if I reload this loading doesn't look too good so instead
let's create a new component called loader. jsx run rafc and then import it right here as a
custom component there we go and of course we have to import it at the top by saying
that that it's coming from loader loader now if we go to the loader now if we reload it will break because don't
forget we have to wrap it in HTML so that it knows that it's not 3D so we need to wrap it in
HTML and this HTML coming from react 3 Dre and then we can give it a div which we can absolutely position by giving it
a class name of absolute top zero left zero W full h- full Flex justify Das Center and items Das
Center within it we can render a new div that will say something like loading dot dot dot and we can also style this a bit
by giving it a class name of w-10 VW h-10 VW and rounded Dash full now if I scroll down and reload
it's looking just a tiny bit better but actually I created this loader file to give you the opportunity to play with
some interesting and exciting models maybe search the internet for some exciting 3D models of different loaders
and once again a complete list of websites where you can find different 3D models can be found in our 3js guide so
download it check it out and do something interesting with this model and once you do that go on
LinkedIn and x and tag us on there because I would be more than happy to share your work with that in mind you
can see that there's only one property that we didn't use which is the set rotation size and that's because I
misspelled it it was supposed to be set rotation state which we are using right here Below on end we properly set the
rotation state so with that in mind let's go back to our that's going to be a model. jsx and right now we have some
fixes to make you can see that our second model which is the big one is also visible on the bottom and if I
click right here nothing really happens right so let's play with gap where for the first time ever we're combining Gap
animations with 3js models to make something truly exciting like this thing right here where it rotates and we get
the second model to make that happen we can scroll all the way to the top create something known as a timeline we can
call it TL for short and call it a gap. timeline so that we can move across that timeline then we want to create a use
effect like this and it's only going to change when the size changes here we want to check
if we are going to trigger the size is equal to large in which case we're going to do something or if size is triple
equal to small when we can do something else so now we're going to do a special kind of Animation which is a timeline
animation so it looks like this broke our application most likely because we haven't yet imported use effect from
react yep that was it and now let's create a bit of a more complicated animation since it will be a bit more
complicated what do you say that we extracted logic to another file we can create a new file within the utils
folder which we can call animations.js within here we can say export const animate with gap
timeline and this is a special function which we will call now let's first talk a bit about what a timeline even is a
timeline is a powerful sequencing tool that acts as a container for tween and other timelines making it simple to
control them as a whole and precisely manage their timing without timelines building complex sequences would be far
more cumbersome because you need to use a delay for every animation like this you apply delays weight and so on but
with the timeline you can specify exactly what you want one after another but to make that happen instead of
calling gap. 2 you first create a timeline and then call the timeline. two exactly like what we have done right
here so what we can do next is we can call this animate with gsap timeline right here and of course we have to
import it from utils animations and we want to pass a couple of props to it we're going to pass the timeline itself
we're gonna pass the small model right here the small rotation as well as the view1 ID and the
view2 ID so we know when to move around and you might be wondering why are we doing small for the large one well if
the size is currently large we want to animate it to the small one and vice versa also we're going to give it an
object of transform where we're going to run a Translate X of minus
100% this will remove it from The View and we can also provide a duration of about 2 seconds so what we're doing
here is we get the current value of the small rotation then we rotate the small model by the value stored in that
rotation we animate the small model view to the left and we animate the large model view to the center but if the size
is small we're going to repeat the same thing right here but we're going to display the
large model and the large rotation and we're going to animate from V2 to vew one and the translate X is
going to be zero there we go so now we're passing all of the necessary props into this
animate with gap timeline and we can accept all those props right here first we passed in the timeline then the
rotation ref then the rotation State then the first Target then the second
target these are view1 and view2 and then all of the animation props now that we have these first we can call the
timeline do 2 and we want to go to rotation ref current. rotation so we get a specific rotation and we modify it by
saying Y is rotation State duration is 1 second and ease I found this one to work the best power
2.in out right below that one we call the timeline two once again and this time we timeline to the first Target and
we spread out all the animation props so these will be the things like why duration and more so animation props
coming from the props and we can provide the ease of power two. inout and also after that we can provide a string of
the lower than character this symbolizes to insert the animation at the start of the previous
animation finally we can duplicate this one right right here below and say timeline two second
target same thing and everything else will be the same so we animate from the first Target
to the second target now if we save this you can see that something started happening they're
moving so if we select the small one we get it back if we select the big one they're both gone now let's see why here
we have two and on the other one we have no models at all well we can go to our model and let's go directly into the
model view and here is where we're messing with which one are we showing so basically we modify the right position
of one to basically get it off of the screen but this right 100 is not going to work if it's not absolutely
positioned so here we can add position of absolute and now they show one on top of another look at this this is looking
a bit weird let me reload yeah there's still one on top of another and that's because this right is
not getting applied as it's outside of the dynamic block so it needs to be within that Dynamic block right here if
index is two then apply right else apply this one there we go so now it's hidden and if it go go right here it's showing
this is wonderful and you can see how nicely it rotates like if you mess with it it's going to rotate back to the
starting position and then move away this means that our models are now great we can modify the colors notice
how the screen also changes and you can go back to the different one that also changed the color the meshes the
textures the lighting it's all looking great and now that we can actually see the model we can also play with the
intensity a bit like bringing it to 0.5 maybe bring it to five there we go now you can notice a big difference or
even 10 right now everything is very very light no matter which color you choose so the colors really pop but to
get that a realistic look I kept it very very low to 0.3 and that way you can really see the titanium coming out of
this iPhone but of course this is something for you to play around with you can modify the perspective camera
the lights and so much more that's the beauty of 3js learning how to navigate your way around the 3D World and of
course if you want to learn a bit more about all of the different types of lights that exist all of the different
cameras that exist and all of the other properties that you can use to mess with your models definitely check out our
guide which is linked down below with that in mind let's close our model view animations and model and let's
expand our browser to full screen I'm going to scroll up and reload and let's see how all of this looks like in full
screen we have this beautiful titanium iPhone 15 Pro we scroll down this was a tough one right the Highlight section
with animated progress tracking bars this looks and feels good we can also pause it and we can scroll to reveal the
model now you can move it around see how it looks like in different light and make a decision on which one you want to
buy it's no longer should I buy it or not it's which color and which size should I get Apple's marketing division
is definitely very good so we can learn a lot from the way that they present their products on the website and this
is one of the perfect use cases for for smooth animations as we saw with the videos and also perfect use case for
showing up those 3D models there aren't a lot of 3D websites but if there is one that really should use 3D it's the one
where you can show the products in all of their full glory and now that you have done all of this created a 3D model
as well as these playing videos and a nice looking header when building websites as phenomenal as these ones
right here there's something that we often Overlook and that is performance right
you're going to say why should I care about performance when my websites look like this that's the most common thing
we think about as developers right but still no matter what you put into it they still have to be performant and
quick not to mention that the design should never take away from the form you need to be able to figure out if the
business objectives of the website are properly achieved such as is this titanium iPhone taking away from the
screen and are people actually clicking the buy button so today I wanted to take this video a step further and allow you
to get answers to all of those questions and learning this matters it matters a lot let me give you a comparison of two
rumes featuring projects from different developers take a moment to read through them and let me know which one sounds
more impressive the first one just built a website that looks like the iPhone 15 Pro page from Apple and used react 3js
and GAP cool right but the second developer developed that website as well as tracked the number of visitors and
how they're actually browsing the site are they taking the necessary actions that we want them to take the second
developer they're also tracking performance loading speeds clickthrough rates and all of of that good stuff so
let's be real which developer will get a job well it's going to be the one with business metrics and performance
optimizations so for that reason in this video even though you might not have expected it I want to teach you not just
how to build and deploy a website rather teach you how to get answers to all of those hard questions and we'll do that
using a completely free tool called Sentry which will give us access to all of this information and more it's super
simple to integrate so click the special link in the description so you can follow along and see exactly what I'm
seeing and then click try Sentry for free create your account by signing up with Google or GitHub and after you sign
up you'll be able to answer a couple of questions such as your organization's name if you have a company or if you
work within a company enter their name if not simply type JSM click I agree to terms in services and you can choose
whether you want to get email updates let's continue and now let's follow the
onboarding we can install Sentry by clicking start and we'll choose the platform we want to monitor in this case
it will be browser and how handy it is they also have a react wrapper press configure SDK and we can follow the
steps provided right here let me Zoom it in a bit and first things first we want to install Sentry as a dependency
so let's copy this command open up a terminal paste it and press enter then we can configure our SDK by
copying this entire block of code and heading over to our main. jsx here is where we can paste what we copied the
setup for Sentry we'll modify it just a tiny bit by removing this root render as we already have that right here and
we're going to keep the most important stuff right here that we copied it seems like a lot but you can also just remove
the comments and then you'll be able to see that there's basically a couple of specific properties that we are applying
right here to customize the way that we want to track our application there we go this is it next
we have to upload our source Maps which allow us to track the traces for errors trust me you're going to be pretty
amazed at what this does so let's copy it let's install it as we have done that before and to verify we can render this
somewhere by returning this button here we get asked to install the Sentry wizard so let's press Y and you'll be
asked to select what you want to do we can choose yes or no in this case it says you're not within a GitHub
repository the wizard will create an update files do you want to continue anyway I'm going to select yes are you
using Sentry SAS or selfhosted we'll be using Sentry SAS do you already have a Sentry account
yes we have just created it before the wizard will verify that and you can select your Sentry project and choose
your framework I'm going to choose vit and just like that it's going to install the Sentry vit plugin without
you having to do anything it's going to ask you for using cicd tool to build your application
we're going to say yes and we're going to say yes continue that's it we have successfully
set up Sentry for our project now that that got installed let's modify our Integrations right here
right now we're using the browser tracing integration but we also want to add Sentry do
metrics. metric aggregator integration like this and we also want to add a Sentry do
react router V6 browser tracing integration to which we can pass an object and say use
effect is equal to react. use effect this way it just knows that we're using react and how use effects work finally
we want to update our app jsx to wrap the app with Sentry so let's navigate over to app we're going to import
everything as Sentry from ad Sentry for/ react and then we can simply wrap our app by
saying Sentry do with profiler and then we wrap the app we
have believe it or not that's it that's the entire setup so right now it says this snippet contains an intentional
error and can be used as a test to make sure that everything is working as expected so let's simply copy this
return and instead of returning this entire main let's try to Simply return that button that contains a method that
doesn't exist I'm going to save it and run our application by running mpm
runev and opening it on Local Host 5173 immediately you can see break the world so now now if you click view
sample error it's going to process that sample event and it will give us all the
information about the event that happened type error object object has no update
from interesting this one doesn't belong to our app but soon enough we'll configure
Sentry to start viewing real errors so let's break our app this break the world is actually a button which you can click
and and it contains a method that doesn't exist within our application so if you inspect it and go to the console
you can see if I make this the normal size that it's going to say unod reference error method does not exist is
not defined so we really are breaking the world so once you do that Sentry should have gotten that event and should
enable our account so now if we go to issues you can see that we have a reference error method does not exist is
not defined Sentry will automatically group similar events together into an issue so let's click got it and we can
go into it and see more information about that issue a pretty crazy thing about this is that you can see the
browser the operating system as well as so much more information about that user their IP address the URL and more we can
also Mark it as handled or not handled we can also see which function triggered it and we can see full breath cramps of
how this error came to be all within this Sentry interface and not within our application so imagine if you gave your
app to the users and if they noticed an error it will be almost impossible to debug they would send you screenshots
pieces of code and so much more but with this you know exactly what is happening and believe it or not you you can also
see a replay of what the user was doing when the error happened you can do that by going to replays and you might need
to wait a bit or reload to ensure that it actually shows up but check this out we have most dead clicks which means
clicks that don't do anything and I did click on that button four times we have rage clicks as well uh because I was
clicking on it and nothing was happening so I was raging a bit guilty as charged and then we do have a full recording of
the duration with everything that was happening let's click into it and check it out if I click play we can see the
entire screen of exactly what I was doing on the screen and when I clicked it as well as when the specific errors
were happening how crazy is that I was also messing with my desktop and mobile view so we can see that as well and you
can see exactly what's happening right here on the timeline pretty cool stuff but of course the most
important thing we're concerned with is the errors tab where you can click it see the exact event ID and see all the
information about the error that happened so that we can resolve it and to resolve this error we can also scroll
down a bit and see more information you can see that the session replay since now it has been rendered actually
appears right here within that specific error so you don't even have to go to replace and then we have the complete
BREC cramp of the error as we have seen before and everything about the user's device browser and more and if you
scroll a bit up you'll be able to see this resources and maybe Solutions I like how at least they are being
realistic with AI sometimes you might get lucky but again maybe not so what we can do is we can click view suggestion
and their AI will of course of course if you give it consent try to solve the error for you and hey it looks like
you've encountered a reference error in your JavaScript code which we did so to fix this issue you'll need to define the
method does not exist in your code by adding it right here and they give you some more tips for debugging it in this
case instead of actually declaring it we know that this is faulty code which we don't need so for now I'm going to
Simply remove it from here and that will fix the error so I'm going to say yes this was surprisingly
helpful now let's see if the error has truly been fixed by going back to our app and reloading the screen yes we have
our Titanium video we have our highlight section and we have our iPhone model right here looks
great but we're not here just to fix errors let's explore the performance tab for you it might say zero it does
take a minute or two to load uh but very quickly you should be able to see 100 here I mean our website is loading
incredibly fast we can see that the timing is incredibly low and we can also head over to web
vitals and we can see the largest contentful paint within 300 milliseconds which is good and you get more
information about everything that is happening on your app I mean how cool is this and how convenient it is to have
all of this in one place we can also go to resources where you can track exactly what your website is transferring over
to the devices of different clients the main jsx is the main thing we're transferring now as well as some assets
and images next let's scroll down a bit and check the Discover tab here you can discover different
trends for your application like we can bring it to the last hour and you can see exact actly when your users were
doing what which is quite exciting so here you can see that one user had an error and there was a transaction for
the other user then you can track and see exactly what they're doing from what browser family what device and so much
more it is all here as we have explored before and you can also see complete Network events for everything they were
doing on the site next let's check out the dashboard you can choose from different templates that you can use
like the front- end template which shows you the erroring URLs and web vitals or you can just check out the general one
so let's see the general and here you can see the number of Errors the number of issues the number of affected users
which is interesting the handled versus unhandled errors uh yeah you can see this one is now counted as handled
because we have fixed the error you can see the Errors By browser so you know where everything is failing overall user
misery is also a very interesting metric to to track I guess and I mean all of this is working so well for tracking
what's happening on your application now let me go back and let's see if I can get some more information from the
frontend template by adding it to my dashboard and here if I zoom out just a tiny bit you can see more information
about the overall LCP FCP layout shifts happening page load times and more so you can see the top five issues by
unique users URLs where the issues are happening and if you have any slow page navigations of course you can customize
this by adding more vids for duration throughput crash rates health and so much more even LCP by country which is
good to know if you have users from different countries so I hope you like this little intro to Sentry and how we
can use it within in our project now as we continue developing our project we'll be able to come back to it and see if it
has recognize some new errors or if our apps performance dropped as we introduce some new animations so we'll be able to
track it all in real time and you'll see me use Sentry in many more videos in the future I recently found out about it and
I'm going to use it for all of my apps including our official course platform here there's a lot of things that could
go wrong just due to the sheer amount of features we're implementing within this project people that have purchased the
ultimate nextjs course have access to this platform and we want to ensure that nothing goes wrong on the platform we
have a lot of custom stuff like custom code blocks which you can copy uh all the way to complete files complete
configurations hints images and a lot more stuff like these callouts right here so you don't forget stuff so if
you're watching this video for building a gap and 3js react application you're going to love what we do in the ultimate
next gs14 course so check it out and you'll be able to access it within our new platform and getting back to sentury
as I said you'll see me using their software in many of the upcoming videos and we're going to dive deeper into
things like profiling user feedback that allows your users to quickly add a bug report cron actions alerts and so so
much more while my goal is to teach you how to build beautiful websites with this I want to take you a step further
and also teach you how to make them make sense from a business as well as a marketing and maybe even performance
optimization side of things so with that in mind let's focus on the next section of the day which is going to be this one
where you can explore the full story of how iPhone gets made with this video and some new images appear youring and of
course if you continue scrolling down more text and then these images animate back in so with that in mind let's get
started working on the next section by pulling this to the side and adding the next section right here within our
app.jsx to get started with our next section we can create a new components within the components folder called
features. jsx we can run our afce right within it and then we can navigate over right here import it features coming
from features and that's an automatic import from that/ components forward slash features now if we go back to our
website you should be able to see features right here at the bottom which means that we can dive right into the
features and start implementing it first things first we're going to turn this into a section with a class name equal
to H4 full for full height and common Dash padding we're going to also give it a BG off zinc as well as relative and
overflow Das hidden now you can see features has its own space right here let's also create a
div right here with a class name equal to screen-
max-width within it we'll have a div with a class name equal to margin bottom of 12 n w-
full and right within it we're going to have an H1 which will have an ID equal to we can
do features uncore title so we know what it is and you know why we're adding it that is because we want to animate it
let's also give it a class name equal to section dashe heading and it can say something like explore the full story
dramatic as Apple usually likes to do it so now we can add that first animation if we scroll up and say use Gap coming
from Gap react now you know how we use the used gab before you provide a callback
function right here and then you also add a dependency array now in this case I want to teach you how to make this
just a bit more optimized and we want to use a dry principle of good code architecture which means don't repeat
yourself now whenever we use the used gap which you can see it's a couple of times so far typically we use gap. two
and then specify what we want to happen and in these cases we didn't have to use the scroll trigger but sometimes we did
so if you see scroll trigger we used it in the video carousel right here where we have this used gab gep 2 with the
trigger video and then toggle actions now every time that we want to use a specific scroll trigger we need to
repeat this part trigger is video toggle actions is restart non Nan and so on so wouldn't it be better if we extracted
this function call where we repeat some stuff to a new function within our animations.js file we already have this
animate with gsap timeline but right here above it we can create a new export con animate with gap which is equal to
we get the target which we want to animate the animation props such as you know opacity one and then also scroll
props and here we're going to call the basic gap. two property of course Gap has to be
imported from gap which Target do we animate well we already have it under Target and then what are the animation
props such as opacity one right in this case we can just spread all of the animation props coming from the props
and then we can also add this scroll trigger that I talked about where the trigger will always be equal to the
Target in our case at least and then we also have these so-called toggle actions and what toggle actions are is they
determined how the length animation is controlled at four different places on enter so when we first enter it we want
to restart the animation then on leave once we leave this specific Target then we want to reverse the animation so this
is interesting reverse then when we enter back to it we want to restart and then when we leave back we want to do
reverse so this toggle action figures out what will happen with this specific animation on four different distinct
places whenever we scroll in when we come back when we scroll down and scroll up once again within here we can also
Define the starting position of the scroll trigger something like top 85% that means that when the top of the
trigger is 85% from the top of the viewport it's going to activate giving us enough space and time to trigger the
animation and we can also spread the rest of the scroll props great so now we have this animate with GP function with
scroll triggers and now we can go back not here but rather in the features and call animate with
gap and the only thing we have to do right now is provide the Target and in this case the title is the ID of the H1
so features uncore title and then we need to specify the Y is zero and opacity is one so basically we're simply
saying what do we want to animate and now if we scroll down we cannot see anything
yet and that's because I forgot to provide the hash to indicate that it's an ID so now if I reload oh nothing is
happening yet the ID seems to be correct and animate with gsap indeed provides
the gsap 2 functionality oh yeah but for scroll triggers to work we also need to register a scroll trigger plug-in and
you do that by importing the scroll trigger coming from gsap all and then you say gap. register
plugin and you simply pass that specific plugin you want to register this is how you use plugins within Gap so now if I
reload that is how you use plugins within Gap and the reason why we might not be able to see the animation yet is
because we cannot scroll to the top of it so we need to keep adding some more stuff so once we scroll down we'll be
able to see it so let's go back to Features below this H1 and Below another div we'll create a second div with a
class name equal to flex Flex Das call for column justify Das center items Das Center and overflow Dash hidden so we
don't see any scroll bars within it we can create another div which will help us position the title within it by
giving it a class name equal to margin top of 32 margin bottom of 24 and padding left of 242 within there we can
simply say H2 that is going to say iPhone and that iPhone you can see here so let's style it a bit by giving it a
class name equal to text- 5 XEL that's very big as well as on large devices text- 7 XEL which is even bigger and
font Das semi bolt there we go iPhone let's also duplicate this below and say forged in
titanium as here we want to show what it is made out of there we go iPhone forged in titanium and now you can also see the
explore the full story appear because we scrolled nicely there let's also go below this H2 and Below another div and
then create another div inside of which we'll show our animated videos and images let's give it a class name equal
to flex Das Center flex-all on small devices padding X of 10 then we can give it a div inside of
it which will help us position these elements by giving it a class name of relative
h-50 view height so that's 50% of the screen W full for full width flex and item stash
Center finally within here we want to display the video so we can say video with a
source that will have an SRC of explore video coming from utils and a type of video
MP4 if we scroll down we can see something that appears to be a non-playing video so let's give it a
property of plays in line let's give it an ID of explore video let's give it a class name of w-o
for full width h- full for full height object Das cover as well as object D Center we can also give it a preload of
none so it doesn't take away from the website's performance we will play it muted and we will Auto playay it and we
can also give it a ref to later on play with its settings so at the top we can create a new
ref const video ref is equal to use ref coming from react and we can pass that video ref right here and there we go as
you can see it started playing now what's going to happen when it ends it just stops but later on we want to make
sure that we animated it in and out once we go up and down before we do that let's also render the other two images
which we will animate so we can go below this video and Below another div and then create a container for our images
by saying div we'll have a class name equal to flex flex-all w- full and
relative within it we can create another div which will have a class name equal to feature Das video-
container and within it we can have another div that will contain one specific image so it will have a class
name equal to overflow Das hidden flex das1 and
h-50 VH within it we can render a self-closing image
tag that will have a source equal to explore one IMG an Al tag of something like titanium and a class name equal to
feature Das video Gore grow we'll use this to Target this
and give it some animations so now let's duplicate this entire div containing the image and let's make the second one
render the explore to IMG which we have to import say Titanium 2 and I think this is it if we save it and
reload we thought that we might be able to see the images but there's nothing yet and that's because they're hidden so
let's go ahead and animate them in we can scroll up and we can reuse this same animate with gap function where we can
provide a new Target of dot Gore grow and then we can expand it so it's easier to see which props are we passing and we
can also give it the animation props such as scale of one so it's going to get bigger opacity of one and ease of
power one so this is the way that it actually animates we can also give it some scroll properties and in this case
I'm going to use scrub of 5.5 I found this value to work the best and as soon as you save the file you can
see those two images appear they go out and they come back in wonderful now let's scroll a bit more
down and let's also add some text below those images so let's find the last image go
one two divs below and create a final div container that will have a class name equal to feature Das text-
container inside of it we can create another div that will have a class name equal to flex D1 and flex Das Center and
within it we can have a piece of text A P tag that has a class name equal
to feature Das text as well as Gore text because we'll animate it as well so this Gore stands for
Gap and here we can say iPhone 15 Pro is and then we can leave a space like so and save it you cannot see anything
yet because we didn't animate it in but at the end it should look something like this there we go iPhone
15 Pro is and then we want to create a span for the rest of the text so let's do that right below we can create a span
element with a class name equal to text- White and then you can follow along and type with me I'm going to say the first
iPhone to feature an AOS space grade
titanium design using the same aloy that
spacecrafts use for Missions to Mars okay let's go back we still cannot
see it so now might be a good idea to animate that in so if we right here below this animate with gap we can add a
new one and say animate with gap what do we want to animate in this case it will be a Gore text you see how easy it is
once you have a function you can reuse then you provide specific properties you want to animate such as y of zero
opacity of one we can also do an ease of power two. in out and a duration of one of course this has to be within an
object so let's fix it there we go and that is it so now if you reload you can see that the text
appears right below let's fix this it's an iPhone and now if I reload it's not there that's because we have to scroll a
bit more to see it don't worry we'll do that soon let's just go below this div and then duplicate this entire div with
flex one and flex center for the second piece of text there we go now we can see it but
of course we have to say something different now so in this case I will say something like let's do
titanium has one of the best strength to weight ratios of any metal making these are and here we can
say lightest Pro Models ever Apple surely does know how to Market and here we can
say you'll notice the difference the moment you pick one up there we go so now we have this nice
piece of text right here looking great so now this entire section is looking good the images are indeed animating in
the text comes in as well but this video once it continues playing it's just stuck so we have to play with it a bit
more to make it come to life let's scroll up and what do you say that we try using this animate with gap so we
can say animate with gap where we can provide the hash explore video and save it now if you reload it starts playing
it looks great there we go it animates but if you scroll up or down still nothing happens
so let's go to animate with gap let's copy this entire gap. 2 and for this one let's apply a manual animation without
using a reusable function because it will be just a bit different so the target will be the explore video of
course with the hash at the start we don't need to pass any animation props the scroll trigger will also be
explore video and the actions will be a bit different because we're dealing with the video so it's going to be play pause
if we go away and then reverse
restart you can play with these toggle actions a bit more and see how they behave finally the start will be minus
10% at the bottom and finally the third property will be uncomplete so what happens once it is
complete and here we want to say video. playay so essentially we will just restart the play and of course here we
also have to import Gap from Gap and now we can scroll down you can see that the video plays the images
animate and once it comes to the end if we go out and come back again nothing happens and that's because
this video. playay right here is not referring to anything rather it should be saying video
ref. current. playay because that's how we're targeting this video so now as you can see it's playing and once it
finishes if I scroll up or down it will start playing once again great now let's expand our browser and check this
out right here after here you see this great looking model you scroll down and you're greeted with explore the full
story of the iPhone forced in titanium we have this great looking video and two images that give you more detail now if
you scroll down you see some pieces of text and if you scroll up nothing happens to the video but if you go once
again down it starts playing again it's just a small little detail and of course you have a bit of creative freedom to
figure out how you want to play with these animations by choosing when you want to trigger them you can trigger
them on click or on scroll or in many different situations with that in mind our phenomenal feature section has now
been completed it's been a bit easier than the last two right well that's because now you know how to animate
better and let's be honest it also was a bit easier to implement than the last two but now let's move to the next one
which looks like this a 17 Pro chip a monster for gaming looks like this and on desktop devices it looks like this
the chip animates in and this great looking video starts playing so with that in mind let's start doing that
section next by going back to our development environment closing everything besides the app and creating
a new component within the components folder called how it works or you can also call it a chip so I'm going to say
how it works. jsx run RFC go back to the app and render the how it works section which of course we
have to import from the components how it works and now at the bottom there it is we can come into it and start
developing it to get started working on our how it works section we can can first turn it into a section and give it
a class name equal to Common padding as we typically do next we want to also give it a Max screen width so we can
create a new div and give it a class name equal to screen Max width now right within it we can create a new div that
will have an ID equal to Chip and yes this will be our processor chip image so we can give it a class name equal to
flex Das Center w- full and margin y of 20 within it we can render the self-closing image tag this image will
have a source equal to chip image or chip IMG coming from utils as well as an Al tag of Chip and a
width of about 180 and a height of about 180 as well there we go A7 Pro chip now what do you
say that we go ahead and animate it so right at the top we can use the use Gap hook and we can create a callback
function and then an empty dependency array right here we can say Gap Dot and for the first time ever we'll use the
from property instead of to property because we're animating it from a specific position so here we can say
what do we want to animate it's going to be the ID of Chip and we have to import Gap from Gap here we can give it some
scroll Properties by saying scroll trigger and the trigger will be the actual chip so once we reach it and
we're going to start on about 20% from the bottom and we want to animate from opacity of
zero scale of two duration of two as well and ease of
power two. inout now if we save it you can see it's gone and then it comes in as simple as
that let's reload that and see it one more time there we go that's looking great now let's go below the chip and
below the div and create another div with a class name equal to flex flex-all items D Center and right within
it we can create a new H2 property with a class name equal to hiw which means how it works title like this and we can
say something like let's do A7 Pro chip like this and then we can add a Break Tag and
then say a monster wi for gaming so now you can apparently game on your phone as well below it we want to
have a P tag with a class name equal to hiw subtitle and here we can say it's here
the biggest redesign in the history of apple gpus and say
there we go that's more like it now below it we want to show that huge video which will be playing right
here so let's scroll down let's go below the P tag and Below one more div and create a container for our video player
we can do that by saying div with a class name equal to margin top of 10 on medium devices margin top of 20 and a
margin bottom of 14 within it we can give it a div with a class name equal to
relative h- full for full height and a flex Das Center within it we can have one last div with a class name equal to
overflow Das hidden and within it we can render the image so this will be a self-closing
image with a source equal to frame IMG coming from utils an Al tag of frame and a class name equal to BG -
transparent relative and Z of 10 so now if we save it you can see this iPhone looking frame we want to place the video
right within it so right below this image we can have another div with a class name equal to hiw video
and right within it we can create a new video property with a source of SRC frame
video and a type of video MP4 now it appears at the bottom of it but let's style it a now currently it
appears at the bottom of it and that's because we should have put it below this div closing the image
so here we have the image and then we go one div down and then paste it right here now it fits perfectly within the
image let's also style this video property by giving it a class name equal to pointer-events
dnone let's give it a plays in line a preload of none a muted property Auto playay and we can also give it a ref
equal to video ref which we need to of course create at the top by saying const video ref is equal to use ref coming
from react which we of course have to import now if we save that you can see that the video starts autop playing of
course we'll kind of animate it so that it looks better even on larger devices now we want to add a bit of text at the
bottom so we can scroll below the video and then below one two more divs and here we want to do a P tag that says
honai star rail this is apparently a very good game that runs on this specific chip we can style it by giving
it a class name of text- gray font D semi bold text- Center and a margin top of three to divide it a bit
from the phone now right below it we want to have a couple of pieces of text that nicely slides in and this text is
quite similar to the text that we had in the previous section so let's go to the app go to features and let's just copy
these two pieces of text there we go div text container as a matter of fact we can copy the entire text container from
here to here and then paste it right within how it works below this P tag now now if we save it and go back
you won't be able to see it yet because it's hidden by default so let's style it a bit instead of saying feature text
container we can rename it to hiw which means how it works text container then it can have a property of flex flex das1
and in this case we can do justify Center and flex-all next instead of feature text we
can have an hiw text there we go and we can call it g fade in because we want to fade this text in and here we
can say something like A7 Pro is an entirely new class of iPhone chip that
delivers our space and then in white we can say something like best graphic performance by far we can delete this
thing after the span and then we have the second div which we can remove and just have a
P tag that says hiw text we can say something like mobile within the span games
will look and feel so immersive and then after the span we can say something like with incredibly
detailed environments and characters now if we save it you can see
this text right here but we cannot yet see this one that's because this one also has to say fade
in and then both will be gone and we'll be able to animate them once we scroll in before we do that let's also add this
one new proc class GPU with six course that's going to be below this div containing the P tag
it's going to be a special div with a class name equal to flex D1 Flex justify Das Center
flex-all and Gore fade in as well within it we can have a P tag that will say something like new and it will have a
class name equal to hiw text now we can duplicate this two more times the second one will say Pro class
GPU and the third one will say with six course now if I save it we cannot yet see it because it also will fade in in
the middle one will have a class name of big text because we want it to appear like this so now let's go ahead and fade
those in we can do that by scrolling up going below this Gap from and calling calling our reusable function animate
with gap we want to animate anything with a class of G fade in make sure to add a DOT at the start as it's a
class and then we can provide opacity of one y position of zero duration of one and ease of power 2.
inout if we save it you can see all of the nicely scroll in now it looks like this is not properly positioned so let's
position it properly if we scroll down you'll notice that we have this div closing the video and then one more div
which closes this video context and then below it we have the honai star rail but what needed to happen here is we need to
close this previous div so here we close another div and then right below the end we can remove one closing sign this will
now properly position it also looking at this part at the bottom it seems like it's too close to what we have at the
top so let's see if it's in the right container we have this hiw text container and it looks like I ended it a
bit too soon it should have been ended right here at the bottom so if I fix this now this looks better so let's
expand it to see it on full screen screen I'm going to scroll up and reload and now I'm going to smoothly scroll
down you see the chip animate you see this video play looks much better on the big screen and then we have the text
right here oh let's see if this text is looking good it looks like the positioning is a bit off as you can see
right here it looks like we didn't properly close these two first pieces of text
together so let's do that by taking a look at this one and then we have to close it right below the speed
tag now if we do that you can see it looks great we cannot get to the second piece
of text because we don't have the footer yet but we will soon and it will look like
this so with that in mind let's quickly get the footer going as well it will be quite simple we just
have to go to the components and create a new footer jsx component where we can run
RFC and we can just render this footer right here of course it has to be imported at the top footer coming from
footer there it is at the bottom left we can dive into it and we can develop it by enclosing it within an HTML 5 footer
property with a class name equal to padding y of five on small devices padding X of 10 and
usually padding X of five as well let's also bring this back to the mobile view so we can see what's
happening within this footer we can have another div for the max screen width by saying class name is equal to screen-
max-width within it we can have another div for our first part of the footer within it
we can have a P tag that will say more ways to shop there we go more ways to shop Let's
style it a bit by giving it a class name of font D semibold text- gray and text- Xs for extra small then within this
speed tag we can have a span that will have a class name equal to underline inex Das blue to signify
it's a link we can add a space beforehand and say find an Apple
store we can also add a space exit the span so that looks like this right now and this space can be outside so it
doesn't get an underline right here there we go and then out of the span we can say
or and then once again within this same span so we can simply copy it and paste it we can say other
retailer and then exit out of the span near you there we go so this looks good we need to provide some spacing so after
or and after the span some space we can go here and duplicate this P tag one more time right below the first one and
properly close it and here we can say something like or call and then we can do some kind of a
phone number I'm just going to type some random stuff right here there we go this looks good to me now we want to go below
the speed tag and below this div and create just a self-closing div which will act as a divider line by giving it
a class name of BG neutral of 700 margin y of five h-1 pixel and w- full this will give us a nice divider
line and right below it we can show all of the footer links so to do that we can just say div with a class name equal to
flex on medium devices flex-r Flex Das column on smaller devices on medium devices items Dash
Center and justify Dash between within it we can have a P tag that will have the same class name as
the one above so we can copy that class name font semi bold text Gray and text Xs and we can say something like
copyright 2024 Apple Incorporated All Rights
Reserved there we go and finally we can have a div below this P tag with a class name of flex and here we can map over
our footer links so footer links coming from constants map where we get each
individual link and for each one we can return a P tag with a key equal to link same class name once again as the
previous one and within it we can render a link and then a space after the link that will look like this and you have
all of the links right here now you can notice that after some we don't have space so we can say if I
is not equal to footer links do length minus one so if it's not the last one then also add a
span with a class name of margin X of two so MX2 and add a straight line like this and
this will create some spacing but of course make sure that you're properly closing everything and in this case we
also have to get the index as we're mapping over it and now if we scroll down you can see this great looking
footer and of course you can turn these into links once you actually have somewhere to navigate and with that said
our footer is done but it's not just the footer it's our entire entire Gap 3js and Sentry powered website and now is
the time that we deploy it it's time to share this wonderful looking hero section that animates in this highlight
section which we worked so hard on this great looking 3js model section as well as the explore the full story and
materials the chipset and even the footer it's time to get it all deployed and on the internet so you can share it
with everyone to start deploying our Apple website head over to your hostinger dashboard find the project and
head to its file manager now you can go into the public HTML and remove this default PHP by deleting it then we'll be
able to upload our entire code base so back within our Visual Studio code you can stop our terminal from running by
pressing contrl C and then clear it next head over over to package.json and within here we can see that the build
script is mpm run build so let's type mpm run build which will run an optimized
production build of our application there we go in about 8 seconds it is done so now if you close this up and
head to the file explorer if you collapse it you'll be able to see a new this folder appear which if you right
click you can reveal in finder or open in file explorer once you open it you can go into it copy all of the files and
simply drag and drop them within this empty folder on our H panel this should take just about a minute maybe even less
and as soon as it is done we can go back to our specific projects dashboard and click our chosen domain name just like
that our project is now deployed and live on the internet it took less than a minute now now we can scroll through it
and admire it in its full Glory with this phenomenal highlight section not to mention that we also have this great
looking Apple iPhone logo and the Apple iPhone title and our domain is https secured let's continue scrolling down a
bit to take a closer look at our 3D model this is looking phenomenal let's not forget that we can also change the
colors which are immediately reflected right here and let's see how Gap and 3js work together by animating this model
there we go that's more like it now let's continue and see this section where we can see this titanium in more
detail learn more about the a17 pro chip and then see the footer with all the links of course let's not forget that
the entire project is completely mobile responsive so if I open up my inspect element and collapse this just a bit you
can see how in Mobile we get a completely different view with the Highlight section videos appearing like
this 3D model looking like this and all of the other sections seamlessly fitting into the
view wonderful so with that in mind huge thanks to you for coming to the end of this video and congratulations be proud
of yourself that you buil something like this and if you liked what we were building
here you will love what we do on JS mastery. proo specifically our ultimate next gs14 course I can proudly say that
it is the best course out there to make you the top one nextjs developer we follow what the best companies in the
world are doing and how they're using nextjs they're not using it like good old react because then they would fall
into a trap off you using the client side on everything and having bad performance accessibility and SEO
thankfully I'm going to teach you how to use it like your real Pro we'll do a deep down to fully understand how it
works behind the scenes then we'll build and deploy a very complex app and you'll also get a chance to participate in
active lessons where we give you complete hints and then you have to build a specific feature everything from
task to examples think resources and even hints on developing something so you can truly understand what you're
doing and of course you're going to build one of the best apps out there a modern version of Stack
workflow so with that said what are you waiting for go to jm.pro and check out our ultimate next js14 course I'll see
you there are you finished building those basic websites that even AI tools like vzer can quickly spin up now is
your time to flex and build a modern landing page with smooth animations that stand out from other let's be honest
outdated and boring sites this is our top uiux video yet and probably the best one you'll find on YouTube let's take a
look at the final application you'll build to Future proof your skills presenting brainwave a modern AI app
you'll build a beautiful hero section with different shapes reacting to your cursor a Navar with a colorful button
that transforms into an animated hamburger menu when you're on smaller screens and look at that subtle smooth
scroll Parallax effect that moves these elements down as we scroll moving on we've got a nice Services section
featuring gradient borders on the cards and unique curved rectangle shapes each of these cards also reveals a hidden
background image next we have a pattern that showcases the most important elements in a circular layout after that
is a very fresh and modern web development layout called bento box many popular companies like apple linear and
clerk use this layout to display their features effectively below there's an always needed pricing section after that
there is a grid style layout presenting the application's road map with gradients grid lines high quality images
and geometry lines on both sides creating a modern look lastly there's a simple footer where we connect to the
website social media pages of course everything's 100% mobile responsive which makes this a perfect tutorial to
learn not just to Cod a design like this but also the best practices of responsive front-end development which
will save you hours and make you a front-end machine this is the best time to go beyond the basics and make
something cool and creative to show that you're at least one step ahead of AI tools and for that we have to thank our
friends over at ui8 their creativity made this special design possible this video features just a couple of screens
out of 44 pre-made screens and hundreds of components check them out the link is in the description deson and if you're
still wondering if you should learn how to build this trust me you really should JavaScript knowledge is required and I
highly recommend taking our Tailwind CSS crash course before watching this video the first step in building our app is
setting up our hosting I want to teach you how to deploy this incredible app to the Internet so everyone can see what
you've built see the app you'll buil today isn't just a project it's a career asset it's designed to Showcase
everything you're capable of making you an attractive candidate for any job I highly recommend Stinger for this app
they're offering an amazing deal right now I'll go for the business plan here's why it's perfect for this project you
get up to five times increased performance free SSL for https security which builds trust with anyone visiting
your website you also get a free domain name giving your website that professional touch and a free CDN to
speed up your site it ensures that your website loads quickly improving the user experience and because we've partnered
with hostinger you get an even bigger discount so click the special link in the description click claim deal and add
to card here we have to choose the period of our hosting and I'll choose 48 months to save the most down below you
can choose your payment method and enter your JavaScript Mastery coupon code which gives you the biggest available
discount on your hosting package and once you purchase we are ready to set everything up right within hostinger
guided setup it's asking us what tool we want to use WordPress with AI or the hosting your website builder in this
case we are real developers so we're going to click skip as we want to develop our own website and I love this
UI as you can see I'm using hostinger to host our official JS mastery. proo website there you can check out our
ultimate nextjs course or if you're a bit more advanced the JSM master class but for our brain wave let's try to
think of something unique I'll go with jsmb brainwave and in this case theom domain is available and it's completely
free for the first year let's click next and once your domain registers you'll also be able to choose where your
website target audience is so that way it loads fastest for them I'm going to leave it as the default and click next
and just like that our hosting has been set up now let's actually develop our application so that at the end of this
video we can deploy it using our new hosting and domain name to start developing our fantastic project we'll
start from bare Beginnings by creating a new empty folder on our desktop we can call it
brainwave and simply drag and drop it into your empty Visual Studio code once you have it open up your terminal and we
can get started first I'll teach you how to set up your react project using V and then we'll set up Tailwind CSS which
will allow us to style our applications much more easily so to get started you can head over to Tailwind css.com click
get started then go to quick search and type vit click install tailin CSS with vit and let's follow the steps first we
have to create our project so let's just copy this Command right here paste it within our
console but then instead of my project type do slash which will create this project setup within our current
repository press y to install create V CLI and then as you can see right here run mpm install to install all the
necessary dep dependencies and then run mpm runev to run our project on Local Host
5173 and there we go we are live with v and react now let's follow the second step in our guide which is to install
tailpin CSS so let's copy this command stop our terminal from running clear it and paste what you copied mpm install DD
tailin CSS postcss Auto prefixer and then later on after that MPX tailin CSS in it- P once you do that you'll see
some new files appear next let's follow the other steps as well such as configuring our template paths so copy
this content part and let's head over to tailin config.js that is right here and modify the content that way it will know
where to find tailin CSS class names next we have to add our tailin CSS directives so copy this head over to
Source index.css remove every everything from here and simply paste the Tailwind CSS directives
then as the application says run mpm randev one more time and let's make some changes to our
app.jsx such as by adding this H1 that says hello world so if we go right here we can remove what we have right now and
just replace everything with a simple H1 we can remove the count the logos and everything else that's in here the only
thing we need is the import of the app CSS and this hello world back on our Local Host 5173 you can see that it is
bolded and underlined which means that Tailwind CSS is properly reading our utility class names such as text 3 Excel
font bold and underline and it's applying specific CSS properties if this popup is not appearing for you telling
you exactly which CSS styles are getting applied that must mean that you don't have the Tailwind CSS extension so
simply type Tailwind under extensions and install Tailwind CSS intellisense with that in mind you've successfully
set up Tailwind CSS with your simple V project now let's focus on formatting a bit to ensure that our codebase is
always clean and readable you can create a new folder within the root of your application called vs code within there
create a new file called settings. Json doing this we're specifically applying some settings just
for this project in the workspace it won't work for all of your other projects this is very handy to use if
you have different settings for different projects you're working on and for this project specifically I have
created a configuration that uses prettier that my entire team and I use when creating these projects so you can
find it in the readme of this project copy it and then paste it right here it's going to apply some prettier
properties like tab width single quotes and more now if you go back to your app.jsx and if you modify something or
just press save you'll notice how automatically it will format it based on its properties this will ensure that you
and I always have the same exact code the next thing we'll do is create a file and folder structure to make it easier
to develop our application in the future so you can head over to source and delete the app.css as we won't need it
and remove it from app.jsx all of the Styles we'll use will be within the index.css next we'll
update our Tailwind doc config.js with the colors we'll be using throughout this project this file will also be in
the read me down below so simply copy it and then override what we have right here you can see right here that we're
adding some special class names such as H1s H2S and so on as well as we are adding some special font families and
colors that's more or less it this will be very helpful later on as we won't necessarily have to say use the color
ac6 AF rather we'll be able to declare it in a simpler way another special thing that we've done here is this
plugins part which you can see here this allows us to Define some of the components that we can then later on use
within our application such as the H1s we want all of our H1s to have same exact Styles which is why we apply these
specific properties to all of the components that use the H1 class names next we want to go inside of the
index.css and also in the read me you can find the updated file and override this one right here here we provide some
special class names for rotations for some animations we'll have but more or less we simply import and set the fonts
and another thing we can do here is declare the color scheme to be dark as most of our application will have dark
colors now if we go within our appli it's going to look something like this so now that our Base Class names are set
up we can start focusing on the file and folder structure but don't worry you will still write all of the Styles these
are only some baseline classes so what we can do next is open up our terminal and open up a new
terminal tab inside of which we can install react router Dom by running mpm install
react-router-dom then we can go to source and main. jsx and we can wrap our entire application with the router by
wrapping our app right here with the router which is coming from react router Dom like this we have to close it of
course and indent it properly which prettier will do for us which is great now it's not router right here that we
have to import rather it's the browser router as router coming from react router Dom great this is exactly what we
want next if you go to your browser you can notice how you have this default v logo we want to make this a real fabicon
corresponding to application UI such as this one brainwave to do that you can delete the existing assets folder within
the source folder and then in the read me down below you can find the assets folder
containing all of the images and icons we'll be using throughout this project so simply download it unzip it and then
drag and drop it here within the source folder and if you expand it you'll see a lot of different images all sorted
within their own sections you can also see that we have our brainwave SVG which is the actual icon we'll use for the
favicon great now we want to head over within our index.html and we can modify our link
icon from v.svg to something like SRC or slsrc SL assets SLB brainwave
symbol. SVG we can also modify the title to say brain wve if we save it and go back you can
see that our meta taags have modified now we have this brainwave icon as well as the brain wave title which means that
we are ready to start setting up our application skeleton meaning the structure for where all of the
components will go great job on setting up a react project using V Tailwind CSS formatting and the base file and folder
structure to get started creating our layout we can navigate over to our app.jsx which will be our homepage here
we can declare our app using the es6 plus const function like this and then we can wrap our H1 with something known
as a react fragment that looks like this so we have a react fragment allowing us to add more elements right here and of
course we have to close our return now below our H1 we'll render something known as a button gradient and to get
access to this button gradient we can say import button gradient from assets SL SVG for/ button gradient
like this this button gradient is an SVG through which we're giving the gradient effect to the button if you hold command
and navigate into this button gradient you'll see different linear gradients they are going to be used within buttons
we're giving an ID to each one of these linear gradients so we can then later on consume them within our code so what do
you say that we start off with a button component so I can show you how we're going to consume this button
gradient we can create a new component within a new folder called components and within components we can create a
new file called button. jsx within our button we can run rafc which will create a new react Arrow function if this
didn't work for you that must mean that you don't have the es7 react Redux react native Snippets installed so install it
and try again now we can import this button within our app.jsx just to test it out so let's put it within a
div and that div will have a class name equal to we can add a bit of a padding top so like PT Dash and let's do a
4.75 REM whenever you want to provide specific values then you can use square brackets we can also on large devices
provide a padding top off about let's do 5.25 RM and overflow of hidden within there we
can render our button component which we can automatically import from components button it won't
be a self-closing component we can give it a class name of mt10 for margin top and an hre off hash login and it can say
something for now now if you go back to our Local Host 5 73 you can see this simple button
right here but we cannot yet see something we just see hello world that something is hidden because the button
is just rendering the text that says button so what we must do now is transform this button into a completely
reusable component this is why I wanted to start off this project with a simple button because if you can understand how
to create complex reusable components on something as simple as a button then you can do it for more complex things as
well so let's get started by making this button accept some of the props for example the class name or an href that
we're passing here we can destructure the props and accept a class name as well as href and later on we also might
want to accept a specific on click or some children that that button might accept or even pixel values or just
color is it white or is it dark once we accept all of these values we can then render that button and we can just
return it like we are doing here but sometimes let's say we want to make our button a link and sometimes we want to
make it a real rectangular button to make that happen we can render two subf functions called con render button which
is equal to just an arrow function where we have an instant return keep in mind these are parentheses not curly braces
where we just return a button component and this button component will render a span element rendering the
children like this and it will also render a special button SVG component so we can do it like this button SVG to
which we pass the color white now this button SVG of course has to be imported so import button SVG from SL assets SL
SVG SLB button SVG and finally we can return the render button component and call it like so and of course we have to
make sure that our path is correct so just add an additional dot right here to ensure that we have the correct path now
back in our browser you can see that we have something that resembles a clickable button but right now it is
within hello world so let's continue modifying the Styles further I'm going to put our browser to the right side of
our editor and that way we can see exactly what is happening now when we're rendering this button let's give it some
additional classes by creating a new property at the top const classes is equal to and we can start defining them
here but before we start defining them let's use them within our button by simply saying class name is equal to
classes now if we give it a class name of something like a button you can see that it's automatically getting applied
right here something now let's make that something a bit more interesting let's make it relative let's make it inline
Das Flex let's give it items Das Center justify - Center h-11 for height transition Das colors on
Hover we want to make it text- color-1 now if you're passing some specific pixel values like this pixel
coming from props coming from the parent component then we're going to render it else we're going to render a default of
px-7 next if the white is true then we want to render a text n of 8 and else we want to render a text n off one and
finally if we have the class names we're going to render them else we're going to render an empty string this way we
ensure that all of the properties properties that we pass from our parent component into our button indeed get
passed to our button you can see that from our app we're passing something like padding top we're passing a margin
top of 10 and if we give it a margin top of 20 right here you can see how it changes it this is how you make reusable
components now I will go a step further here and make it even more reusable I'm also going to Define const span classes
and I'm going to give it relative and Z index of 10 we can also now apply the class name to the span element that way
we can change and modify the text within it now what if we don't want to render a button such as this one what if we want
to render simply a link well we can create a new function for that by saying const render link and then we can have a
return statement an automatic one again where we have an anchor tag like this that will have an
HF of href because this is a clickable link and we still pass over the class names from classes and then the span and
the button will be exactly the same so we can simply copy it here and now that we have render link and render button we
can choose when we want to render each one so right here we can say return if the hre property exists then we can
render the render link like this else we can return the render button there we go in this case we did pass the href so of
course in this case we will render a link but if we now remove the hre if we make it a regular button you can see
that it will look something like this all completely reusable and we'll be able to expand this component even later
on and make it more Dynamic for now this is good enough I just wanted to teach you how to create reusable components
now let's create another component which is more important than the button for now which is the header component so we
can head over to components and create a new header.
jsx we can run ource we can remove this import from react as it's no longer needed and
within our app we can remove this button we no longer need it but rather we can just surrender the
header coming from do/ compon components forward slhe header and we can also remove this H1 the reason we have this
padding top is because the header is fixed and we don't want it to overlap with the content so at the top will'll
show the knob bar with that in mind we can head over to the header and start creating it we can start with the
structure by returning a div element like this that has a class name equal to fixed top- Z and this will pin it to the
the top zindex of 50 to appear on top background of N8 over 90 backdrop Das blur Das small
this is a CSS property that adds elements backdrop it will kind of give it that glassy look we also want to give
it a border dasb for Border bottom border Das n-6 this is a border Coler and on large
devices background of N8 over 90 and on large devices backdrop off blur DSM now we cannot see anything yet because this
header has no content but as soon as we add the header you can see it here within this div let's create another div
that div will have a class name equal to flex items Das Center padding X of five on large devices padding X off
7.5 on extra large devices say padding X of 10 on Max large devices like this we can give it a padding y of four for top
and bottom immediately within here we want to render an a tag an anchor tag that will render the image of source is
equal to brain wave and this brain wave has to be imported from our assets by saying import brain wave from do/
assets there we go that's better already ready let's also give it a width of about 190 and a height of about 40 with
an Al tag of brain wave this anchor tag can have a class name equal to block W of something like 12 r on extra large
devices margin right of eight and an H ra off hash hero like this this is an ID used for navigation we want to specify
what happens if we click it and in this case if we click it we navigate over to the hero section now I can notice this
is a bit too close to the left side of the screen and I can notice that I didn't put it within this inner div so
let's move it over to inside of the div we created not that long ago there we go that's much better now below this anchor
tag still within the other div we can create a nav element and this nav will have a class name equal to Hidden fixed
top property of five REM left zero right zero bottom zero as well background of N8 on large devices static on large
devices Flex on large devices margin X of Auto and en large devices BG of transparent and of course I have to
spell the class name properly with a capital N now within this nav we can create a div to create some layout this
div will have a class name equal to relative Z of2 Flex flex-all items Das Center justify Das
Center margin of Auto so M Auto and on large devices Flex row and within it we want to show all of our items such as 1
2 3 and so on right now we cannot see them because they're dark and for that reason to more easily map over our nav
items we can create a new folder within our source called constants and within the constants we
can create a new file called index.js in the read me down below where you found all of the other class names
you'll also be able to find this constants file so simply copy it and paste it over here now you might wonder
300 lines oh my God is this our entire application coded for us but not really no this is simply different lists of
content of text that we'll be using throughout our application such as when we're going over the pricing section I
don't want you to hardcode all of these values rather we'll extract them from here and then map over them similarly
we're doing that for our navigation bar so if you see the navigation immediately here we create different link items with
a title URL and the ID so now we can go back over to the header and we can map over them we can do that by scrolling
over to the top and importing in curly braces navigation coming from do SL constants now we can
use that navigation right here by using a dynamic block of code called navigation and then mapping over it by
saying that map where we get each individual item item and for each item we instantly return something and that
something is an anchor tag this anchor tag can render the item. tile and it will have a key equal to item. ID as
well as an href equal to item. URL so now we're mapping over all of these properties and we're using the URL
and the title but if you save it we cannot yet see it and why is that well that's because this nav is usually
hidden so if we expand our browser you'll be able to see it right here features pricing how to use roadmap new
account and sign in so we can keep this view right here while we're styling them let's also give this anchor tag a class
name this class name will be dynamic and it will first turn each element into a block then it will also give it a
relative a font dcode which will apply a special font family text- 2XL to make it a bit larger
uppercase of course because we want to be able to click on these links easily text- n-1 to change the
color transition Das colors and on Hover text- color-1 of course make sure to apply to
the hover element and now if you hover that's already much better we also want to turn on a special property called
item.on mobile so some of these properties will only be showing on mobile and if that is the case then we
can do LG hidden which means we can hide that item on desktop or we can just apply an empty string which will look
like this now we can continue with the styling by giving it a padding X of six to create some spacing we can also give
it a padding y of six on medium devices we can give it a padding y of eight on large devices we can give it a
minus margin right of 0.25 on large devices text- Xs and on large devices f- semibold as well this
will make it look like this a bit smaller next we have to figure out which link is currently clicked meaning are we
in features or are we in pricing to do that we can can use a special property called use location from react router
Dom by importing in curly braces use location coming from react router Dom then right at the top of our
component we can say const path name is equal to use location and this will give us access to the current URL we're on
with that in mind we can scroll down and within the Styles we can check if the item. URL is triple equal to the
path name. has in which case we can apply a zindex of two we can apply on large devices a text-
and-1 and if that is not the case we can then give a class on large devices of text- and-1 over 50 so now we can see
all of them are gr out besides pricing or features or how to use this works for all of the links next let's also give it
on large devices a leading of five which will increase the line height and on large devices and hover we can also give
it a text n of one and on extra large devices we can give it a padding X of 12 so now we have something that looks like
this great finally on the right side of the screen we want to have a sign up we're trying to replicate this we want
to finally use this reusable button comp component we created not that long ago so we can head over below our div and
below our nav and create another anchor tag that will have an href equal to Hash signup and it will have a class name
equal to button hidden margin right of 8 text- n-1 over 50 and this simply allows us to
apply this Coler we have declared in tailin CSS config transition Dash colors which will apply a nice
transition once we hover over it and on Hover we want to modify the text color to N1 as well as on large devices set it
to block within this anchor tag we can say new account there we go we can see it here
and right below this anchor tag we want to create a button or rather we want to use our button component we have created
not that long ago so we can at the top just import it but by saying import button from SL button this button will
have a class name which will be hidden usually but on larger devices and larger it will be
visible and it's going to have an href off hash login and it will say sign in if we save it you can see how nicely
it appears on the right side now we're almost done with this desktop now bar but I can notice that it's not going all
the way to the end of the screen so if we head over to our first div we can see if we properly applied all of the
classes toab zero oh we're also missing the left zero right here to ensure that it's positioned on the Zero from the
left and we're missing a w- full it's for it to take the full width of the screen this is more like it we have the
primary navigation we have the new account and sign in and if we expand it this is looking wonderful on mobile
devices exactly the same as our deployed application but unfortunately there's absolutely nothing on our Mobile screen
that's because we'll Implement a completely custom mobile menu which looks like this you can open it and you
can close it so to get started creating our mobile navigation I'm going to help you to create the circles behind the
actual navigation you can see these circles and these little icons let's make that happen first and to make that
happen we can go to our file explorer and from the read me down below you can download the design folder unzip it and
then drag and drop it within our components folder within our design folder you'll notice that we have a
header. jsx file and here we have things such as Rings Sidelines background circles hamburger menu and we're then
using all of them right here within our app I think you can understand what those are rings are the Rings you see
around background circles are those bowls that you can see around as well and Sidelines are those vertical lines
you can see here and here the reason I wanted to provide you with this code is because all of these are hardcoded
values that we can play with and find the ones that work accurately for us it's just absolute positioning more or
less but if you think this code is confusing and if you're wondering how to properly use the absolute property I
would highly recommend to check out the absolute positioning inside relative positioning guide by CSS tricks the link
will also be in the read me with that in mind we can now go back to our original header and we can import two things one
thing is to import the menu SVG coming from assets SL SVG for/ menu SVG and the other thing is to import the hamburger
menu inside of curly braces fromd design slhe header and now we can
neatly combine this mobile menu with the desktop menu we had before so let's create a new use State at the top by
using the US state snippet let's call it open navigation and set open navigation and at the start it will be set to false
then we can modify this div by turning it into a template string like this and then checking whether we are
currently open or Not by saying if open navigation like this then provide a BG of
N8 else provide a BG of N8 over 90 and a backdrop blur small like this so now we of course have to
import Ed State coming from react and if you do that you'll get an error saying that the hamburger menu does not exist
that's because we misspelled it right here hamburger menu it's missing an R right here Burger once you fixed that
and reload we're back in Action so now let's go below this div that's wrapping our items and right here let's render a
hamburger menu a self closing component and also we have to make it show by going to our nav and modify the class
Name by once again making it a template string close it with a template string as well and then in here we can check if
the navigation is currently open so if open navigation in that case we can make it
Flex else we can make it hidden and then we can remove hidden from here now it's obviously false but if we
turn it to true through you can see the navigation menu of course we're going to leave it
to fals by default but now we want to go over below our signin button and add a new button this button will render the
menu SVG so it will look something like this it will have the class name equal to margin left of Auto and on large
devices it will be hidden margin left Auto will align it horizontally right here we can also provide it a pixel
property of PX3 and to this menu SVG it needs to know whether it's opened or closed so we
can provide it the open navigation state of course we want to somehow turn it on or turn it off like a toggle switch so
right here we can create a function called const toggle navigation that is equal to a function
that checks if the navigation is currently open in that case we can set open navigation to be
false and else we can set open navigation to be true also we can declare a function called const handle
click and once we click something we want to set open navigation to false because the last thing you want is have
something opened and then once you click on it to then have to go and close it you want everything to be closed
automatically once you click so now back in here let's attach this tole navigation to our menu
button by giving this button an onclick property of toggle navigation if we save it and if we click here oh nothing seems
to be happening but if we manually switch it to true you can see that works so that must mean that something with
the toggle navigation function is not good let's see if we're properly using this onclick property within our button
component and would you look at that this reusable component button hasn't yet learned how to use the
onclick so we can simply pass it to this button by saying onclick is equal to on click now if we go back and click here
you can notice that it opens although it's not looking exactly the same as the finished application right
so let's see what's wrong with the layout this hurger menu should have been rendered below this div right here that
way we can close the div and then have the hurger menu this will fix it just a tiny bit I also think we forgot to pass
an onclick listener to each one of our navigation items so we can do that by saying onclick is equal to handle click
we'll most likely have to look into to our div that's wrapping our entire nav or our entire header so let's see fixed
top zindex that's good we can remove this color from here as we're changing it in the open navigation backdrop blur
SM we can also remove it from here and let's see if that fixes it and it does this is great we have our vertical lines
we have our linear Rings everything is looking great and we can open and close our mobile menu as you can see the only
thing we've done is we've used this header component which then goes over the Rings Sidelines and background
circles which are basically nothing more than absolutely positioned values sometimes beautiful designs like these
but you might think how the hell did they do that well they simply use absolute positioning so now you know the
tricks now another important thing we have to do with this mobile navigation is Implement a special package called
screen lock it's this package right here and the only thing we'll use it for is to disable page scrolling this is for
mobile devices this will ensure that once you open up the page you cannot Scroll once you're here rather once you
click somewhere then the page will scroll automatically to that element so let's install it by going to our
terminal and running mpm install scroll lock let's go back to our header and and let's
import disable page scroll and enable page scroll which can be imported from scroll
lock finally once we toggle the navigation off we want to enable the page scroll so we can once again
scroll make sure to put the p as uppercased as it has to be so enable page scroll and once we turn on this
mobile menu then we can disable page scroll like this also once we click on a specific
element if not open navigation so if it's closed then we simply want to return we are not allowed to click then
and here we can just enable page scroll and set navigation open to false because that means that we clicked and
we are ready to go somewhere else and Scroll once again and with that in mind you have successfully implemented your
first major component the header not only this beautiful mobile header that looks like this with absolute position
values that once you click it leads you to a specific section but also this great desktop header which looks like
this you have the logo on the left you have some links in the middle you have the new account and sign in on the right
right this looks clean but what's even cleaner is this phenomenal modern hero section with this nice looking image a
great heading and a call to action button so without any further Ado let's get started not only with creating our
hero section but by creating a reusable section component which we'll use for all of the sections to come to start
creating a reusable section component we can start start creating it like we would any other component by creating a
new component within the components folder called section do jsx where we can run our afce to get it up and
running this component will act as a common skeleton which will reuse in all section components this component will
create all the lines between different components so to start working on this component let's first accept some props
such as class names or class name the ID of the section the crosses whether we have them or not crosses offset soon
enough you'll see what this is custom paddings and children once we have that we can return
our div and to that div we can provide some class names by giving it a class name of
and we want to make it Dynamic so we're going to do it like this and and give it a position of relative check if we have
custom paddings and then also provide all the other styles such as padding y of 10 on
large devices padding y of 16 on extra- large devices padding y of 20 then if we have crosses so if crosses then on large
devices we can give it a padding y of 32 and extra large off padding y of 40 else just render an empty string finally if
we're passing some additional class names we can just render them here or else just render an empty string now
within the section we can render the children so we can say children right here meaning whatever we pass into it
will be rendered right here and we can also create the divs for the sidelines let me show you what those are so on the
finished website if we go right here you can see those lines this one right here with those two crosses so we want to
differentiate our sections in this very very interesting way so to do that I'm going to collapse our browser to mobile
view and I'm going to create a div that will usually be hidden so it will have a class name of hidden it will be absolute
top zero left five with a width of0 0.25 h- full and BG off stroke -1 pointer events none on median devices it will be
block meaning visible and larger and on large devices left 7.5 to move it a bit away from the left corner and an extra
large we're going to give it a left off 10 similarly we can duplicate this one and make it the same same but for right
devices right 7.5 and right 10 and we also want to handle those crosses that you saw before
you cannot see them on mobile devices but you can see them on desktop devices so let's create a new Dynamic block of
code where we check for crosses and if the crosses exist we render a react fragment that has a self-closing div
this div will have a class name equal to it will be a dynamic string of hidden absolute top-
Z left- 7.5 right- 7.5 as well h- 0.25 for the height BG d stro-1 and a
dynamic block of code if crosses offset exists then we render the crosses
offset like this also we're going to give it pointer events none and what this does is this disables all the
pointer events so we canot click it on large devices we will show it so it will be block usually it will be hidden on
extra large we'll give it a left of 10 and write of 10 usually like this now if we go back to our website still nothing
is happening because we haven't yet used this section and we will use it very very soon but before that we want to
import those crosses at the top by saying import section SVG from assets SL SVG SL section SVG and
then we can use it right here if we have crosses below this div by simply saying self-closing component of section SVG to
which we pass the crosses offset if it exists there we go so now we ready to finally
use this section and before we use it you can see that we have one warning right here and that is that the class
name is missing in props validation this is just an eslint warning which we can fix if we go to eslint rc. CJs if we go
to rules and then add an additional rule that's going to say react slpr types will be set to off like this and of
course we have to close the string here and now if we go back to our section you can notice that it's no longer
complaining it is now just giving us a proper warning that the ID is not being used and we do want to pass that ID over
to this div ID is equal to ID and finally we are ready to use this section within our application and what
other section will we use it for rather than our hero section so let's create a new component within the component
folder called hero. jsx just run rafc and let's immediately
consume it within our app.jsx just below the header we want to render a hero section looking like this
hero there we go that's pretty simple right now we can go into the hero and we can start developing it the interesting
thing here is that we're not going to wrap it in a typical div rather we're going to wrap it in our
section which we have created just before so we can import this section coming from that slash section and if we
save it you can notice how it pushes it down just a tiny bit and if I expand it you can also notice that it applies some
vertical lines although it's very hard to see right now it'll be easier to see as we pass some additional properties to
it for example I'm going to return this properly so it's easier to to see and I will pass our section some more props
that it wants such as a class name of pading top of 12 RM and minus margin top of
5.25 RM I found those values to work the best you can see how it shifts it and we can also continue adding some more
properties such as crosses as well as a crosses offset property of large
Translate Y of 5.25 RM like this we can also give it a property of custom paddings and we can
give it an ID of hero as this is our hero section if we save it and expand our browser you'll see that not a lot is
happening right now we cannot see any crosses or anything that's because we don't yet have enough content within our
hero section so let's change that let's wrap our section with another div and this div will have a class name
equal to container and relative within this div we'll create another div and that div will have a class name equal to
relative Z of One Max DW of 62 R MX Auto this is for margin horizontal Auto and this will simply align all of
the elements horizontally we can also give it a text Center to show the text in the center we can give it a margin
bottom of about four Ram to provide some spacing on medium devices we can give it a margin bottom of 20 and on large
devices we can give it a margin bottom of about six REM as well finally within this div we can create a new H1 that
will have a class name of H1 this is a special class we created before as well as a margin bottom of six and
within that H1 we can say explore the possibilities like this and we can also copy whatever else we say here explore
the possibilities off AI chatting with brainwave so let's simply copy it paste it here the deployed website will be
mentioned in the description down below so you'll be able to copy all the content from it and then within this H1
we also want to create a SP Anan element and this span will have a class name equal to inline Das block and also
relative here we can say brain wave now below brain wave we want to also render a self-closing image tag
this image will have a source equal to curve and this Curve will be coming from assets it will look something like this
and we can also give it a class name equal to absolute top- full left- Z w- full and on extra large devices we need
to reposition the bit to fit below brain wave to minus margin top of two there we go we can also give it a width of about
624 and a height of about 28 I found those values to work the best alongside with an Al tag off curve now we can head
below this image below the span and below the H1 and we can create a P tag which will form our
subheading that subheading will contain all the text below which will be this one you can just copy it and it can say
Unleash the Power of AI within brain wave let's style it a bit by giving it a class name of body -1 let's also give it
a Max dw-3 XL margin X of Auto margin bottom of six text- n-2 and on large devices margin bottom
of eight right below this P tag we want to show a call to action button so it will be a button component which we need
to import from that slash button I did that automatically right here at the top and that button since it's our component
we can pass it on HRA of forward slash pricing and we're going to give it a special property of white and then we
can say get started there we go now we have a white button now this button is not looking great right now you can see
the text is not centered so let's go into the button and let's see if you apply white why is it not centered and
usually it is it looks like our classes are just a tiny bit wrong so we do all the great stuff such as inline Flex
relative item Center justify Center as well then we apply padding and then we have this white property where we apply
text n 8 or text n one and then after that we apply a class name the issue is the typo I have right here where it says
inline it rather should be in line and there we go now our button is fixed as well now below that button we can start
creating the rest of the structure which is quite exciting so we can go below below the button and below the div and
create another div which will be the container for the rest of our hero section with a class name equal to
relative Max DW d23 REM margin X off auto on medium devices Max dw5 XL so it has a lot of width and on extra large
devices margin bottom of 22 within that div we'll create a another div to keep building out that
hero structure with a class name equal to relative Z of one padding of 0.5 rounded Dash to Excel and we also
want to apply this bg-- gradient within that div we want to create another div and that div will
have a class name equal to relative BG d n-8 rounded D1 REM like this and within that
div trust me this is the last one we're going to create a self-closing div that will have a class name equal to h- 1.4
Ram BG of n10 rounded dt- 0.8 remm that's going to give it this grayish rectangle and below it we
can have a d within which we'll show this great looking robot image with a source equal
to robot coming from do do/ assets we're going to also give it a class name equal to
w-o we're going to give it a width of about 1440p and a height of about 1,800 pixels
with an Al tag of hero as in hero section that's looking great now let's style the div wrapping the robot it will
have a class name equal to aspect -33 over 40 this is the aspect ratio and let's of course call it div as it is
then let's give it a rounded dasb of 0.9 REM like this let's also give it an overflow hidden so we cannot scroll
through it and on medium devices let's modify the aspect ratio so aspect Dash 688 over 490 I found those values to
work the best and on large devices we also want to play with it a bit by saying aspect
d124 over 490 this will make it look good on all different kinds of devices and screen sizes now let's go one two
three divs down and below it we're creating one last final div and within that div we're creating
the image that will be the hero background so here we can say Source equal to Hero background coming from do/
assets let's give it a class name equal to w-o and looking at it right now I think
I provided the class names of this image to the image above so we want to take this width and the height and the AL tag
from the robot over to this below one so we want to do it like this with 1440p height 18800 alt is hero and that's
going to give us this great looking background for now this background appears below the robot but soon enough
it will look like this it's going to come from behind it to do that let's add more properties to our robot such as
width of 10,24 and height of 490 and Al tag of AI now let's add the class names to this
div wrapping the hero Background by giving it a class name equal to Absolute minus stop minus or Dash
54% this will make it appear below or rather behind this image we can give it a left- 1 over2
which will move it a bit away but then we can give it a width of 234 per which would make it a bit larger we
can also then use the translate property so minus translate specifically dx1 over 2 that's going to look like
this and now it's going to appear right behind it then on medium devices we want to play a bit where we show it so it's
going to be minus stop- 46% like this on medium devices W will be
138% and on large devices minus stop Dash 104% there we go so now we have this
beautiful looking hero section as you can see and if we expand it you'll see that
it's looking great but not perfect as it says curve right here we want to remove that part where it says curve rather
that is just the actual component or the image there we go and if we compare it with the finished product we're still a
bit off right it looks a bit different so going back now we can make the fixes for desktop devices here we're missing
the positioning in RS you can use RS you can use pixels whatever you prefer this one is 84 pixels and it seems to me that
the image is definitely not looking good so it's not positioned correctly as you can see right here so let's scroll down
to where we have our AI robot and let's provide it some class names other than w full for example we can give it a scale
of Dash 1.7 a translate of Y of 8% which will bring it a bit up and then we want to
play with that scale and translate on different devices such as on medium we can set the scale to be one on medium we
also can do the minus translate dy- 10% and on large devices we can do a
minus translate of Y of 33% so now if we save it you can see
that it looks much closer to the final design although we're definitely missing some space between our header we're much
closer than we were before as you can see on the final website we have these moving circles which we already
implemented before as well as these circular rings that go in the middle of the screen so let's implement it to
start implementing it we'll get some help from the package called first we have to install it react just Parallax
so react Parallax and then we'll use the same Rings we have used before from design but this time in the hero you can
see gradient bottom line we have different rings and all of that is being displayed within background circles and
these little things within thises that are mounted these are the WS that you can see flowing around so now to
implement those rings and circles and everything we can head over to the hero section that we have developed and we
can import everything we need we can import the background circles bottom line as well as
gradient coming from.des slh hero we can also import something known as hero icons coming
from do/ constants and let's not forget the Parallax we can import the scroll
Parallax coming from react just Parallax and it looks like we have to fix the import for our
hero we are right now within hero so it's going to be just slash design hero there we go now to make it work we can
give a ref to our div of the hero section by saying ref is equal to Parallax ref
and we can Define that ref right here by saying const Parallax ref is equal to use ref which is a hook which we have to
import at the start equal to null once we have that ref we can navigate over below our image of the robot of the AI
and then there we can render the scroll Parallax coming from that package which we just
installed there we can say is absolutely positioned which is one of its proper and we can render a UL or an unordered
list within it we can give it a class name equal to Hidden absolute positioning as we said
it will be a minus left of 5.5 Ram a bottom of 7.5 REM padding X of one padding y of one
background of N9 over 40 back backrop blur border and Border D n-1
over10 let's not forget to make it a bit rounded of to XL and on extra large devices it will be Flex now within there
we'll be able to show all of these different icons that you can see here floating around so if we go back within
this UL we can open up a dynamic code and say hero icons. map where we get each individual
icon and an index for that icon and we can immediately return An Li that has a class name of padding of five so
P5 and a key equal to index and within it we render an image that has a source equal to Icon a width of about 24 a
height of about 25 and its alt tag will be equal to just icon now if we save this you can see this menu appeared
later on we can make it clickable as well and you can see how it flows we have this Parallax effect which is
really cool and it is done by the library we installed two devs down below that Parallax we want to render a self-
closing gradient component which looks like this and this will give it this nice looking gradient to make it really
pop and then going below this background hero that we have here specifically one div below the image we want to render
the background circles which we also created not that long ago and this will make it move like this now this hero is
looking much closer to the finished website let's see where is it missing some top margin from if we check out our
hero section here we're giving it a padding top of 12 REM but if we go within the
section let's see if we're actually implementing that class so if we really make make sure that everything is nicely
divisible we have relative we then start with custom paddings so if custom paddings are applied appli them else
apply all of these Styles right here and then at the end apply class names now make sure that this code is exactly as
it is here and then it will work most likely had a typo somewhere and if it still doesn't work you can refer to the
finished section. jsx code so simply copy it and overwrite it but once that is done you'll be able to see that now
our hero is looking amazing also notice that we're seeing brain wave two times so if I go back to my hero section and
look at brain wave we don't have to see it for the second time we have it within the span
element and we have to provide some space like this so now it looks great amazing job building this hero section
you can see that now it's very similar to exactly how it looks like on the deployed application we'll do some more
improvements soon but even for now this is just absolutely amazing great work now the only thing that our hero is
missing for it to be perfect are these little jumping add-ons these cards that go up and down where we can see what we
can do within this AI app such as code generation and also this AI is generating also I notice we have a bit
of a different text spacing so if we go to our deployed application you can see that a I is at the top whereas here it's
at the bottom which has a bit of a nicer text hierarchy so going back to where we are saying possibilities of AI right
before off we can render the N sign and say NBSB and then a semicolon and we can also put that after AI so let's just do
it right here before and after AI that should be better so now if we save it you can see off space AI space and then
chatting with but make sure that there's no space between semicolon and chatting with then it's going to look exactly
like this which is now much more similar to this and also it looks like I misspelled possibilities great with that
said we can focus on what matters which is implementing those flying add-ons to our robot screen so first let's focus on
this AI is generating text I'm going to go back to our code and if you look at here we have a bit of a warning where it
says that the bottom line is never used so just before we Implement those little add-ons we can scroll to the bottom and
right before we're closing the section we can also use this bottom line component now let's go back to the
components and create our first flying component this one will be called generating dot jsx it's going to be a
simple rafc component that's going to say generating and it's gonna render only a single image and a piece of text
this image will have a class name equal to W5 h-5 and margin right of four and we can
also give it a source equal to loading so this will be our loader and this loader we can import loading from
do/ assets and then we can also give it an Al tag of something like loading and we can also say AI is
generating now if we save that it's going to be nowhere to be found but now as soon as we style this
div by giving it a class name equal to it's going to be a dynamic template string and it's going to have classes of
flex items Das Center h-3.5 REM padding X of 6 background of N8 over 80
and rounded Das 1.7 REM finally it needs to render all of the class names that we passed to it so we can see class name
here and then render class name or an empty string if we don't have any and we can also give it a text Das base this
will just provide it a typical font size and line height so it looks good now we want to use this within our hero section
so let's go to our hero and let's scroll up above our scroll Parallax and here we can render the self-closing generating
component which we of course have to import at the top by saying import generating from slash generating and it
won't be within curly braces that's my bad if we save it we still cannot see it and that's because we have to pass some
additional props or styles to it specifically we have to make it absolutely positioned by giving it a
class name of absolute left -4 right D4 and bottom-5 now on medium devices we can
modify the left to 1/ two and on medium devices we can make it right- Auto on medium devices we can also modify the
bottom to eight and on medium devices we can modify the width to about 31 Ram like this and on medium devices we can
also use the dash translate or minus translate X1 /2 this is just to ensure that we properly position it on top of
our image which you can see right now looks absolutely perfect later on we can even make this SVG spin so it feels like
it's generating something and now the last thing we have to do is this code eration card and we
can create it by creating a new component within the components folder and we can call it a
notification. jsx we can run RFC and we can immediately use it within our hero it's also going to be within
its own Parallax so let's recreate another Parallax scroll Parallax that means that it's going to
move up and down it will be absolutely positioned and there we can render the self-closing
notification component coming from do/ notification and we can also automatically pass some properties to it
we can pass a class name equal to usually hidden but on larger devices will be able to see it absolute minus
right- 5.5 remm bottom of 11 REM w -8 RAM for width and on extra-
large devices Flex meaning visible we can also give it a title of code generation this will be what the
notification will say and now we can go into the notification and start creating it we can accept the props we passing
into it such as the class name and the title and we can start styling our div by giving it a class name equal to
it's going to be a dynamic template string where we first render the class name or an empty string if it doesn't
exist in this case it will because we're making it absolute we can also give it a property of flex items Das Center
padding off four padding right off six background of N9 over
40 backdrop Das blur to make it feel like it's glassy border border dn1 over 10 which is the
Coler rounded - 2XL gap of five and that's it already you can see this notification appear
finally we can wrap it within a div that has a class name of flex -1 and there we can render an
H6 this H6 will have a class name equal to margin bottom of one font Das semi bold and justify between and it will
render the dynamic title we're passing from props now it says code generation right below this age6 we're going to
create a Dev where we have a class name equal to flex items Das Center and justify between and now that I look at
it this H6 doesn't need to have a justify between it's going to have a text base to modify its font size and
line height within this inner div we're going to have a UL that will have a class name equal to
flex minus margin of 0.5 and then within this we can map over our images of the users generating
something with AI by saying notification images which we can import from constants that map where we get each
individual item and the index and we can immediately return An Li that renders a self-closing image with a
source equal to item a class name equal to w- fo a width of 20 and a height of 20 as well so let's fix it and let's
also give it an ALT tag of item now if I save this you can see all of these different user avatars
let's style them a bit by giving this Li a key equal to index and a class name equal to flex
w-6 h-6 border of two border dn-12 and most importantly rounded Das
full which will turn it into full circles and overflow hidden so we cannot actually scroll through them there we go
this is much better notice how in the final version we have this image of what they're generating so we can add that to
our application as well by adding an image Above This div with a flex of one this will be self-closing image with a
source equal to notification one coming from assets with a width of 62 a height of 62 and then I'll tag of image there
we go go that's better already and we can also make it a bit rounded by giving it a class name equal to rounded
DXL there we go the last thing we need is a text that says 1 minute ago so to do that we can scroll down and right
below the UL we can create a div that will have a class name equal to body -2 and text-
n-13 and it's it can say 1 M ago there we go now this is looking more like it it's Fuller and these things
scroll up and down if you want to make the AI is generating also scroll up and down you can simply wrap it in this
scroll Parallax in case you want to do that there we go so generating you wrap it into a scroll Parallax and save it
and you can see that now it moves as well but now it feels like too much is happening I want to leave this here and
only let those two move up and down so I'm going to bring this back to what it was great finally the last part of the
hero that they're missing is helping people create beautiful content ad and then these different logos right here so
that's going to appear below the hero section or rather still within it but a bit below so to create that last hero
component we can go to components and create a new file called company logos. jsx where we can run
RFC and we can go back to the hero and immediately use it within here that's going to be all the way at the bottom
below background circles below a div and then right here company logos imported from that/
company logos and we can also provide it a class name equal to Hidden on smaller devices
relative Z of 10 margin top of 20 and on large devices block so it's actually visible and now you should be able to
see it somewhere here at the bottom but it's quite dark so you cannot see it so let's go into the company logos and
let's make it appear exactly how it should be we can do that by accepting these class names that we're providing
and then applying them directly to our div class name is equal to class name that will make it appear right here at
the bottom then within this div we also want to render an H5 that will say helping people create
beautiful content at and then we want to start listing those logos so we can remove
this company logos we can style this H5 a bit by giving it a class name equal to tagline margin B bom of six text- center
and text- n-1 over 50 that's much better and below the H5 we can render a UL that's going to have a class name equal
to flex and it's going to render or map over company logos coming from constants map where we get each
individual logo an index of that logo and we return An Li for each one of these that renders a self-closed image
with a source of logo a width of about 134 a height of about 28 and then we can also say alt is going to be that actual
logo if we save it you can see all of those logos at the bottom and to style it a bit we can give this Li a class
name of flex items Das Center justify Das Center Flex D1 and H -8.5 RM and of course we have to also
give it a key equal to index if we save it you can now see those beautiful logos and now we can say
that our hero section is looking wonderful this is one of the most modern designs I have seen in the long time
these circles or bols move as you scroll but still they're not taking away your attention you have those circles or
rings actually bringing your attention to this button and to this image here these cards nicely float showing you
what you can do with this software and then you get instant approval by seeing other companies using this software as
well now that this hero is spared with our wonderful Navar this is looking great and let's not forget that it's
also looking amazing for mobile devices you can open it up you can close it scroll down and here we remove or get
rid of some of the Clutter you don't want to show all of these cards if you don't have enough space on desktop
devices we have space here we don't so we hide them so that is it for the hero section phenomenal job on coming this
far but don't forget this entire time we haven't been just building the hero section or the Navar we set the
foundation for everything else we're going to build we have the reusable section component which now creates
these rectangular lines on desktop devices and we have also set up the entire file and folder structure so that
now we can simply render an additional section below the hero section specifically the next one will
be this one we're going to call it benefits where it says what you can do with this app such as ask anything
improve every day connect anywhere or much more it looks great on mobile devices but it looks even better on desk
stop so let's go ahead and create the second big section of the day which is our benefit section and snap I just
changed our Visual Studio code theme to make the video a bit more interesting I switched it to a theme called one dark
Pro whereas before I used this cpin theme what do you think of this one let me know in the comments down below which
one you prefer but with that said let's continue with the video we want to focus focus on another big section of this
video which is the benefit section so we want to add this tagline as well as these beautiful cards indicating
different benefits of our application so to implement it we can create a new component within the components folder
called benefits. jsx in there we can run our AFC and
immediately we can import those benefits right below our hero so we can say a self-closing benefits which we can
automatically import from components now if we go into the benefits we can start creating them and
we should already see benefits right here at the bottom although it's quite hard to notice but we will be able to
notice it once we wrap our benefits into a section component coming from do slash section this will give it some space and
nicely position it on the screen we can also give it an ID equal to Features so we can later on scroll through it
dynamically from the navigation bar and what do you say that within this section we also have a div with a class name
equal to container relative and a z of two within this div we can render a special heading
component this is a component that we haven't created yet so we can create it right away by creating a new heading JSM
X component and run our fce then we can simply import it within our benefits just like this and now we can
see heading and to this heading we can pass to props we can pass a class name prop allowing us to further style our
heading and we can also pass it a title prop in this case it can say something like chat smarter not harder with
brainwave and we can paste it right here there we go so now if we go back into the heading we can accept these class
names as well as a title coming from props and we can use them when creating this heading so right here this heading
will be a very reusable component it will allow us to use it as a title as a subtitle as a large text tagline and it
will take many more forms so right here we can check if we're getting the title and then within this div we can say
title and and render an H2 that will render this title and we can also give a class name to this H2 to be equal to H2
now if we save this and go back you can see chat smarter not harder with brainwave we also can do something with
with those class names and specifically we can use them within our div so this div can accept a class name equal to
it's going to be a dynamic template string where we can render the class name
dynamically and we can also pass it some additional Styles such as Max DW of 50 REM margin X of Auto margin bottom of
12 on large devices margin bottom of 20 so now if we save it we can pass some additional class names every time that
we call our heading and this is useful because you might want to call headings in different
places so for example in this benefit section we can pass it a media query of medium Max dw- medium and on large Max
dw-2 excl so now as we scale this text you can see how it will also change right
here below great this is it for the heading but now we can focus on our benefits section so right below this
heading still within the div we can render another div and this div will have a class name equal to flex
flex-wrap gap-10 and margin bottom of 10 as well within here we can map over our benefits
which we can import from do/ constants so if you now dive into these benefits by command clicking them you can see
that this is a simple array with a couple of different objects specifically each object is a card with an ID a title
text background URL icon and more so what we can say is we can now say benefits. map and we want to get each
individual benefit we can call it an item and automat automatically return something for each one of those items
let's make sure that we properly close it right here there we go and I think we're missing one more div right here
there we go we also want to give this div a key equal to item. ID within that div we can create another div and within
that div we can have an H5 that simply renders the item. tile so now if we save this
and scroll down you can notice that we have five or six different pieces of text each one of these is a card even
though right now it's just a piece of text we will create that card and we can do that by giving a class name to this
outermost div this div will have a class name of block so each element is a block relative for relative positioning
padding off 0.5 to provide some spacing BG Das no repeat like this BG Dash and now this is a special one we can give it
a length by saying length 100% underscore 100% so now the background will take 100% of the size of this div
and on medium devices we can also say Max dw-2 24 REM now the way we'll apply that
background image to each one of these cards is by using the inline Styles so we can say background image is it's
going to be a template string of URL and then in parentheses dollar sign curly braces item. background URL so for each
one of these cards as you can see we're getting a different background URL now let's style this inner div right here by
giving it a class name equal to relative z-2 Flex flex-coil
m-h d22 REM and this will just give these cards a bit more size even though it looks silly right now it will look
better very soon we can also give it a p- 2.4 REM for some padding that's much better already and we can say
pointer-events dnone as these won't be clickable cards to this H5 we can give a class name equal to H5
and margin bottom of five okay and below the AG five we can also render a P tag that will render the item. text and we
can style it a bit by giving it a class name equal to body das2 margin bottom of six and text-
n-3 these n colors are just special colors we have defined in our Tailwind config you can call them anything you
like if we go into our config you can see right here if we go to colors that we just call them different things of
course we could have named them something else as well now let's go below this P tag and let's render a div
and this div will have a class name equal to flex items Das Center and margin top of Auto within here we're
going to render the icon for this card by giving this image a source of item that icon
URL a width of about 48 a height of about 48 and then Al tag of item. tile if we now save it you can see that each
card just got a special icon looking good let's also provide a P tag to say Explore More so simple P that says
Explore More and Below we can also render an arrow which is a self-closing arrow component which we can import at
the top by saying import Arrow from do SL assets SL SVG SL Arrow now if we scroll down you can see
Explore More and arrow but it's not really aligned so let's style this B tag by giving get a class name equal to
margin left of Auto font DH code to change the font family text- XS font Das bold text-
and-1 for de Coler uppercase and tracking Dash wider this will give it a bit of a bigger letter spacing finally
it looks like I messed up the item center right here if we do that it's looking much much better now if we go a
bit down two divs down sometimes we might want to have a light item which will be a bit different from other items
and in that case if item. light is true meaning end end then we can render the gradient light which is another SVG
coming from the top so we can import gradient light like this coming
fromd design slash benefits if we save it you can now see this gradient appear over the cards we
choose and the way we chose it is we simply put it right here card light true and these ones don't have the light true
this one does have it so you can just modify it and that's going to give this nice effect uh of a gradient light
appearing behind some cards just for some visual interest finally we can go below this gradient light and create
another div this div will be interesting if we go to the finished website you can really see a lot of stuff here but if we
expand it you can see how if you hover over the cards you get this nice looking image to appear well this will be for
that image URL but we have to be smart with how we're going to place this image onto the card so that once we hover over
it it happens or rather it appears so let's give this div a class name equal to Absolute inset of 0.5 which will
apply a CSS inser property and a background of N8 we can also give it a
style equal to clip path of URL benefits I don't specifically remember why I had to add this clip path but I think it
helps showing those background images on Hover So within this div we can have another div which will render that
actual image so if we properly close it and inside we check if the item has an image URL like this only then render a
self-closing image property that has a source equal to item. image URL also a width of about 380 pixels a
height of about 362 pixels an Al tag of item. tile and if we save it you can now see
those images everywhere but we want to give it a class name of w- full for full width h- full for full height and object
Das cover so it nicely fits right there now to hide it or to only show it on Hover we can style this div by giving it
a class name of absolute inset -0 opacity -0 but then transition the
opacity on Hover opacity will be 10 so now if we hover you can see how it nicely appears in a lower opacity so it
doesn't take away from the card and right below these two divs we can also render a property called clip path which
we can import at the top by saying import clip path from d/
assets SL SVG slash clip path this is just a simple SVG which ensures that we also see the ends of our cards oh yeah
this will actually apply that clip path of benefits so now if we go a bit up and you see this clip path of URL it will
actually allow us to see full rectangles for these cards and with that in mind we're done with the benefit section we
Reus this amazing section that allowed us to quickly create it you'll see how it looks like on desktop devices soon we
used a couple of svgs and we neatly mapped over our benefits which are simply a collection of different data
pieces and we also created a heading which will make much more reusable later on and we helped ourselves with a couple
of svgs so now if we close this and the heading the benefit section is done so let's see how does it look like on the
finished website on desktop there we go this is looking great if I compare it with the finished
one you can see that we definitely have a bit of a large size for our card headings so if I go back here and if I
check out our H5 oh it looks like I said H2 right here I should have said H5 so if we go back this is now much
more like it it looks amazing chat smarter not harder with brainwave and we have these nice looking cards of course
you can Implement an onclick right here and then you'll be able to navigate somewhere for example to show more
details about a specific benefit or a feature again fully responsive so if we go to a smaller view you can see we have
six and if we go right here we'll have only one per row and it looks and feels incredibly natural for mobile devices as
well so with that in mind our benefit section is now done and the next section we'll Implement will be this AI chat
collaboration section which looks great on desktop you can see a big heading on the left side with some illustrations on
the right side and if we go right here it looks great on mobile as well so let's get started with collaboration to
get started with the collaboration section we can first create a new component within the components folder
called collaboration. jsx and we can run our afce and immediately imported within our
app by opening a new tag and saying collaboration coming from components then if we scroll down you can see
collaboration right here we can command click into it and start implementing it by first wrapping everything into a
section coming from section which is going to just give it some space and we can give a special property of crosses
to this section which will also on larger devices apply these crosses above the section this just applies some
visual interest so that it's not boring when you scroll you sometimes have those crosses as well for different sections
and if you remember it is so simple to do it now because right here within the section we have implemented a special
crosses part which then adds this div with crosses which is basically just a collection of svgs with that in mind
right within our section we can create a div that div will act as a container so you can have a class name equal to
container and en large devices Flex so now if we scroll you cannot see anything within that div we can have
another div and that div will have a class name of Max dw-2 REM so this is for the max width
and right within it we can render an H2 that can say AI chat app for seamless
collaboration there we go and we can also style it a bit by giving it a class name equal to H2 margin bottom of four
and medium devices margin bottom of eight there we go now if we go below that H2 we can render a UL or an
unordered list and on a finish website that's going to look something like this where we need to map over a couple of
different elements such as integration Automation and topnotch security so we can give this UL a class name of Max
dw-22 REM and margin bottom of 10 and on medium devices margin bottom of 14 right within
it we can map over our collab content coming from constants by saying collab content do map where we map over each
individual item and for each one we return An Li now this collab content is just a simple array with three objects
that have different titles that's it and we can also pass special collab text such as this one right here which we
will pass into the second property so let's give this Li a class name equal to margin bottom of three and padding y of
three and a key equal to item. ID now if we save it and go back you cannot see anything and that's because we have to
provide some content within the LI in our case that's going to be a div and that div will have a class name equal to
flex and items Das center right within that div we're going to render an image which will have a
source equal to check this will be that check mark which is coming from do/ assets with a width of about 24 and a
height of 24 as well now if we go down we can also render an H6 El element and render the item. tile for each of these
checkboxes that's going to look something like this so now we can also give this image an Al tag of check for
accessibility purposes and this H6 can have a class name equal to body -2 and margin left of five to provide some
space between the elements that's looking much better now if there is a text uh such as in a case of the first
property we can then check if item. text exists then we can render a P tag that's going to have a class name equal to body
-2 margin top of three and text- n-4 and it can render the item. text so if we save it now we can
see the text right here this text should go outside of this div not within this uh specific specific div that contains
the checkbox and the text so let's put it just below and that's going to make it look much better now we also have to
implement this button try it now so that button will come below the UL and that button will now be simple to implement
as we have our own custom reusable button component which can say try it now see how simple that was we now have
a usable section and a reusable button as you develop your apps and uis it's all about creating that custom UI that
you can then use and leverage which is great and tailin config of course makes it easy uh not that long ago we had a
custom class and this container here is a custom class that applies a width but also applies some media queries for
different widths and it's very useful to expand on the typical taable in config with colors font families and so on so
you can more easily create reusable classes great with that in mind we can start focusing on the second part of our
collaboration component which is this illustration right here so to create it we can go below the button and below
this div and start focusing on the right side of the screen at least on desktop devices on mobile everything is just
below so let's create this div that will have a class name equal to on large devices margin left of Auto on extra
large devices W d38 RM like this and we can now put something within it specifically I'm
going to put a P tag that will render the collab text coming from constants this is a special text which is just
basically a string but it's better to extract it into constants and then use it like this than
to type it manually right here now let's also style it a bit by giving it a class name equal to body
-2 margin bottom of four text- n-4 on medium devices margin bottom of 16 on large devices margin bottom of 32
and on large devices W of 22 Ram also on large devices margin X of Auto so we provide some margin or spacing between
the elements great also to divide this text from this button we can also maybe add a margin top of something like two
or maybe even more such as four now that we have that we can start focusing on the right side of the screen or as I
said on mobile that's below it's this illustration that you can see but the coolest thing is this is not just a
single image if you try to drag and drop you can see that each one of these icons is a separate thing so this is not just
an illustration that you just place on the screen rather we're going to develop this circle and the way that the
elements go around this brain wve logo it won't be easy but we'll do it together right below this P tag we can
create a div that will act as the wrapper for this illustration with a class name equal to
relative left of 1 over two Flex w-22 REM aspect d square because it's a square
illustration border border D n-6 rounded Das full minus Translate dx-1
two scale of 75 and on medium devices scale of 100 to make it a bit bigger so if we do this you can now see this Outer
Circle appear right here we have just created a circle that's it basically a div acting as a border that then has the
round full property which gives it a maximum border radius now I think you can see where we're going with this
right within it we can create another div that will have a class name equal to flex
w-6 aspect d square margin of Auto border border - n-6 and rounded Dash full if you do that
you can see the second Circle appear within that div we can create another div and that div will have just a
different width so we can give it a class name equal to
w-6 REM we can also give it an aspect of square margin Auto padding Dash something like 0.2 REM B- conic D
gradient and rounded Das full and then within this one we'll apply another div that will then render an image this
image will be a source equal to brain wave symbol coming from assets with a width of about 48 height
of about 48 and an Al tag of something like brain wave which is the name of our app now we
can style this div to centrally position it by giving it a class name of flex items Das Center justify Das Center W-4
h- full background of N8 and rounded Das full to make it a circle so now it is nicely positioned at the center but I
think we're missing one Circle even though we said rounded full we don't yet see anything right here and that's
because I think we misspelled this conic gradient it's BG conic if I spell it properly now this looks exactly like it
should as you've seen it might have seem simple but we're going to really build it not just slap an image and call it a
day so now that we have all of this we have to go uh one two three divs down and create a
UL uh this UL will wrap over collab apps so collab apps is basically just an array of different icons so you can see
the title of the icon as well as the icon itself and we can say collab apps. map where we get each individual app and
its index and we can immediately return An Li a list item this list item will have a key equal to app. ID within this
list item we're going to have a div and within this div we'll render an image that will actually render a source of
the app. ion if we do that and scroll down you can see all of these icons appear now let's properly position them
on the screen we can start doing that by styling our Li so let's give it a class name equal to let's make it a dynamic
template string and we want to absolutely position it on the screen so position absolute we're going to give it
a top- z and now they're going to just fly away somewhere we won't be able to see them on the screen at all what we
also want to do is give it a left- 1/2 and h-1 over2 as well minus ml margin left- 1.6 remm like this this is going
to position it on a specific angle and we can also give it An Origin bottom which is going to do a transform origin
bottom property and now we want to rotate it which is interesting so we want to say rotate
Dash and now we want to make this Dynamic we want to apply a rotation by using dollar and curly braces index
times 45° so we're going to rotate it by 45 degrees and we're going to get something
that looks like this this is great now of course we have to play with the scale a bit right so we can style this image
within not the div just the image for now by giving get a class name of m- Auto as well as a width of app. width
and a height of app. height okay this will change the width and the height and we can also change
the alt what we're doing this by saying ab. tile this is more like it but they're still not touching this Outer
Circle let's first style this div and then we can play with that further by giving it a class name equal to let's
make it Dynamic and let's give it relative positioning let's also give it a minus
stop Dash 1.6 REM there we go that's much better let's give it a flex property W Dash so this is for the width
of 3.2 Ram let's give it also a height of 3.2 Ram that's much better let's give it a
background like BG and7 let's also give it a border and a border n 1 over 15 so it's going to be
barely noticeable but I think you can see it right around those elements like they're floating they're kind of like
planets in a universe flying around the Sun so we can give it round the dash Exel to make it a circle and we can also
apply minus so minus rotate Dash and now that's going to be index times 45 so now you can notice how they rotate right if
I go back you can see how these are not properly positioned right now but if I rotate it back to where we had it it's
looking good but now you you might think are we just not canceling out these two rotations well let me show you what
happens if I remove this rotation as well as this minus rotation they're not properly positioned at all
so we have to rotate them on the LI axis but then rotate them again for them to be properly positioned so you can see
them all in a normal view from top to bottom great and with that in mind you can see how this looks like on mobile
it's great but if we expand it you can also see it on desktop but are we missing something if we go to the
finished website you can see it looks good what if I expand it even further you can see that here you have a
tried now line that goes from this one to here kind of like you're going to trigger some effect and there's also a
line going from here till the end showing that you can collaborate effectively it's these little details
that matter so to implement this we can go below the UL and we can render the left curve curve like this as well as
the right curve right here and these are two elements that we can import at the top by saying
import left curve as well as right curve coming fromd design slash
collaboration and you can see that now if we go back to the current website we cannot see them really on mobile and
maybe we should also provide some margin top on this let's do that so if I go here to where we have our circles I can
provide a margin top of something like two or we did four before so let's do four or maybe let's do a margin bottom
of this piece of text right here oh we do have it margin bottom of four it's weird why is it overlapping let's try to
increase it a bit to something like five or maybe even six or eight there we go that's a bit better so now if I expanded
you should be able to see those lines appear on our current version as well although they're barely noticeable you
can see them right here and how do those work you might ask well if we go into one of these you can see that it's
basically a div with an image that renders the curve which I don't think we can see because it's an SVG but
basically it's just a line that you position the screen and then we use absolute positioning to properly
position it once again this project has a lot of absolute positioning but hey it works right and you need absolute
positioning if you want to make something like this but you have to make sure that it works great on mobile
devices as well great with that said we have completed the collaboration section which is yet another section in our
design let's see what comes after that is it the pricing well not yet we're going to do the pricing soon but now we
have a very exciting section that looks like this generative AI made for creators we have this huge over the
entire screen I'm not even sure how I would call it but we are reusing this AI generating which is pretty cool uh we
have some more additional checkboxes I would call it some features right these are different features that
our app has oh and I got excited this is exciting we are not just seeing double this huge over the entire screen image
we have a big one and then we have smaller one this is I believe called a bento box design if I'm not mistaken yep
Bento Box it looks something like this Apple has done it not that long ago where we have more rectangles some
bigger and some smaller you can see it here on the Apple website and you can see just how similar it is to what we
have on the screen right now A big one and some small ones so without any further Ado let's dive into creating our
next section of the day which is Services what are we offering to get started with the services section we can
create a new component called services. jsx run rafc and then simply use it right here
beneath our collaboration Services there we go and we can just self close it and import it from compos
components Services then we can go right here and we can start implementing them this is maybe one of the most exciting
sections of the application maybe besides the hero section I mean you cannot really beat this and also the
mobile menu is pretty cool so now in the services we can of course wrap everything in a reusable section
component which we need to import oh the import doesn't want to do it for now for some reason so we can manually imported
by saying import section from slash section there we go and scroll down now the ID of this one will be how to use or
meaning which Services we provide and right within it we can have a div that will have a class name equal to
container great now within that container we want to render a heading component so heading like this this and
we also need to looks like self imported at the top by importing heading from
heading and to that heading we can pass a title of something like generative AI made for creators that's kind of our
title there we go and we can also give it a text equal to brain wave unlocks the potential
of AI powered applications now this text won't do
anything on its own but what will is once we go into this heading and make it more reusable allowing it to accept text
as another property and then to do something with that text such as going in here and saying text and end we can
then render a paragraph that will render that text with the appropriate class
name of body- two margin top of four and text- n-4 so now you can see the heading and
you can also see the subtext great going back to Services beneath our header we want to create another div that will
have a class name equal to relative this div will contain another div that will have a class name equal to
once again relative Z of one to appear a bit on top a flex so you can Flex some items within it items Das Center height
of 39 REM like this margin bottom of five padding of eight border border of n 1/ 10 rounded - 3XL
overflow Dash hidden on large devices padding of 20 on extra large devices
h-46 REM now this didn't do a lot on its own it just created space for something to
show here and that something will look a bit like this this is what we're creating space for pretty exciting right
so what do we do within that div well we can create another div and within that div we can render a
self-closing image that has a source equal to service one and looks like Auto Imports are really not doing me service
today maybe if I close the file and then reopen it maybe now no still doesn't like me never mind so let's Auto Import
it from the top by saying import service one we can also get the service two service 3 as well as check mark or check
from d/ assets great so now if we save this you should be able to see this great
looking image right here but it doesn't really look that good on its own so let's kind of style it to be a part of
that div specifically we can give it a class name equal to
w-f h- full OB object - cover on medium devices object d right we can also give it a width of about 800 pixels and a
height of about 730 pixels and we can give it an Al tag of
smartest AI great now this is still just an image so let's style this div containing it by
giving it a class name equal to 2 absolute top- 0 left- Z
w-o h-o there we go that's more like it pointer-events Das none to indicate it's
not clickable on medium devices w-3 over5 and on extra large devices W off auto there we go that's much better this
looks more closer to what we have on the finished version and now below this div containing this service we can render
another div that will have a class name equal to relative Z of One Max dasw of 17 REM and
margin left of Auto within there we can render an H4 that can say smartest Ai and we can also render a p
tab that will say let's copy it from the finished website brainwave unlocks the
potential of AI powered applications there we go that's good and we can also render a UL that will have a class name
equal to body -2 and within here we can map over brainwave Services of course if we spell
it properly and if we get out of imports but looks like we don't have have any
luck today so I'll go right here to the top and manually import it geez I just remember the times when I had to
manually import everything uh brainwave services and then brainwave Services icons coming
from. SL constants that's going to look something like this it's basically just photo
generating photo enhance and seamless integration and services icons I is capitalized there we go so now you can
see the three of them photo generating photo enhance seamless integration but let's put them within different Li items
by saying brainwave services do map where we get each item in the index and we can automatically
return An Li that will have a key equal to index and within that Li we can render
an image that will have a source equal to check and beneath the image we can render a P tag that will say
item this will look like this now of course we have to style the H4 the P tag the Li and the image so let's start from
top to bottom with the H4 by giving it a class name of H4 and then margin bottom of four as
well we're creating some text hierarchy right here then we can create another class name that will have a body of two
margin bottom of let's do three REM and then text- n-3 so we're making it a bit smaller and
we're also muting the color a bit then we can style our Li by giving it a class name equal to flex items Das start
padding y of four to create Some Space Border Das t for top and Border d n-6 as you can see this provided this
very light color border on top and we can also style the image by giving it the width of 24 and height of 24 as
well and finally we can style the P tag by giving it a class name of margin left of four and we have just converted what
was previously just an image into something that looks more like this great now we want to do a couple more of
these right it's not just one if we now expand it this is looking so wonderful on desktop devices but now we want to
have two more of these um and as you can notice they're all similar they have a title subheading and then some text in
this case or no these are just titles and subheadings without text this right here is just the image but once again
this is not the entire image right here you will create this popup manually as well as the image and even this icon and
this track right here that you can see all of that will be created manually we're not just sticking a sticker on it
same thing for this too why is this useful well if you have a landing page where you know for a fact that this will
not change then you could have just used that as the entire image same thing for this here everything right right here
could have been just a single image although if it's image it's larger in size but if you have it like this this
is not taking too much computational power or internet right um and text loads much faster than an image which
allows you to use smaller images and Achieve better performance so with that in mind I can now collapse this you can
still see how it looks on mobile devices and we can start creating those two different cards oh but let's not forget
on the original card if you expand we have this AI is generating right here at the bottom and on our current version we
don't have it so let's go right here below the UL and below the div create a new
generating like this self-closing component which we can import generating from SL generating we have
created this component before but we have to provide it some class names to position it exactly where
we want it to be right now it's here we don't want that it's there so we're going to make it absolute so it doesn't
just jump into the view this gives us power to position it where we want such as left four right four bottom four if
you do that you can see now it's here we can also give it a a border n of 1 over 10 as well as a border in General on
large devices now you want to play with the positioning a bit on large devices left of one over two and this is just a
trial and error so you can just figure out how to position it properly LG right Auto LG bottom 8 and also LG minus
translate X1 over2 so now it looks great on medium it looks great on larger tablet even here and it's even here on
mobile wonderful now let's go a div below and let's start creating our second service what we can even do is we
can just collapse this entire div for the first service I think it's this one there we go so now this makes sense
we're going to have three divs within this relative service one service two and service three and the bottom two are
going to be within a grid so we're going to play Not only with flex but also with grids we can give it a class name equal
to relative Z of one grid gap between of elements of five and enlarge devices grid calls
two and now we can start creating our first one by wrapping it in a div that will have a class name equal to
relative m-h Das something like 39 REM I found works the best
border border D n-1 over10 rounded -3 excl and overflow of hidden and now you
can see that we have created the second card within this div we can create another div that will have a class name
equal to Absolute and inset zero and within it we can render the image for this second image or for the second
service that will have a source equal to service 2 that looks like this with a class name h- fo w-o
and object D cover now that makes it bigger let's also give it a width of
630 a height of 750 like this and let's give it the alt of something
like robot that should be good there we go now let's go below it and let's also add this text on top of
it now this text will be similar to the text we used for the first one so we can copy it this one just below the service
one it's going to be this one right here a div that contains the H4 and the P tag so let's copy it collapse it one more
time and use it right here below this div containing the
image and paste it and don't forget to close this div that we copied as well if we save it you can see it doesn't look
as good so we have to still play with it a bit let's modify the styles of that div let's make it absolute so we get
more uh kind of power of where we want to position it let's do inset of zero row let's add a flex Flex Das call
justify Dash end so it appears at the end padding off 8 BG gradient to B like this this is a linear
gradient from n 8/0 to N8 over 90 with a large devices padding of 15 this applies this gradient
so it looks very nice but this is still not similar to how it looks on the finished website right on the finished
one it appears at the bottom not at the top and I think that's because I messed up the justify end so if I fix it now
it's at the bottom and here we're going to say something like photo editing and we can say whatever this
text says right here by copying it and pasting it get right here there we go now we have to do this photo chat icon I
think I have coded this one before it's just a photo chat so if we go to the top and if we import photo or I think I
misspelled it as fod fod chat message coming fromd
design SLS Services you can see how this photo Chad let's let's fix it it's Photo Chat Photo Chat right here um and you
can see what this is it's basically a div but I provided it to you because it's just a div where we have to do some
absolute positioning nothing more than that and then it uses this double bubble double bubble Wing which is an SVG
nothing more than that um this is great and we'll also get some more things from this design uh folder uh called gradient
we have used it before video bar this will be that bar at the bottom and also video chat
message these are all mostly just components that are absolutely positioned to be somewhere in the screen
and they use some kind of svgs or pngs to show some content with that in mind we can now
scroll down we can also use the dollar sign or rather the N sign sorry appos and then semicolon to do the
aposto this is the way you properly do it because like this you get a warning saying that you need to escape it and
then right here below this p and below the div we can render the photo chat message as a self-closing one and it
will appear on top so with that in mind we're done with the second of these services so I
believe I can properly collapse it so this is the first one this is the second one and now we can create the third one
but I don't think I have collapsed it properly um remember that I said that these two services will be within this
outer div that has a display of grid so I have to collapse this inner one not the outer one and then go right here
below it now I can create a new div that will have a class name all padding of four BG of
N7 bed-3 XL overflow D hidden on large devices m- h-46
Ram this will create some space right here at the bottom for the new card let's also create another div within it
for the layout with a class name of padding y of 12 and padding X of four and an extra large devices padding X of
eight as well within that div we can create an H4 this H4 will say video generation and below that H4 we can
create a P tag that can say something like this thing right here this will be the world's most powerful AI video
generation art engine what will you create okay that's a good one of course let's style it a bit by giving this H4 a
class name equal to H4 and margin bottom of four let's also style this P tag by giving it a class name of body
das2 margin bottom of two REM and text- n-3 for the color there we go that's great and now here we'll have to map
over all of these Services they're not just an image we actually map over them and for example this one can be selected
so to Implement that right below the speed tag we can create a UL element and this UL will have a class name equal to
flex items Das Center and justify Dash between then within it we can render the brainwave Services
icons map where we get each individual item and its index and we can automatically return An Li for each one
of these that Li will keep track of a div and within that div we can render an
image that renders that item which is just an icon so should I say source is item and we save it and this gives you
five of these icons now of course we can further style this by making this one selected and by giving them some kind of
a background behind I'm not sure if you can see it but there's a circle happening behind each icon so let's
style it by giving this Li a class name it can be a dynamic One Flex items Das Center justify Dash
Center and now we want to keep track of the index and if the index is triple equal to two meaning the third element
then we're going to get it a w of three REM and h-3 REM as well and a padding off 0
Let's do three a BG Das conic Dash gradient rounded Das
2XL on medium devices width of 4.5 RAM and on medium devices height of 4.5 Ram as well well else if it's not the
currently selected one then we want to give it a flex W of 10 h of 10 BG of n6 rounded Dash to Excel or now that I
see it this rounded could have gone outside of this so we could have put rounded at the start rounded -2 Excel
that way we don't have to repeat ourselves two times then let's continue we also want
to give it a medium device width of 15 and on medium devices height of 15 as well now if we save it the reason why
we're getting an error is because we didn't provide a key is equal to Index this looks very colorful but on the
final one it's not the actual full circle that's colorful rather it's just the border so what we can do is we can
also color this inner div by giving it a class last name where we can check if index is two
then we give it Flex items - Center justify Das Center w-f h-f BG D
n-7 and rounded Dash let's do one REM and save it of course we have to do the other part of the turnery and say
nothing but now as you can see it seems that we have completely hidden that color that appeared before and that
could be because we provided too much padding here this padding controls how much of this circle we're going to see
around it so if we do something like padding of 0 point let's do 25 instead of three you can see that now we have
just enough of this background uh conic gradient to show which is exactly what we wanted also for this image we can
give it a width of about 24 height of 24 and an Al tag of item so it knows what it is and with that we have our icons
the only thing we're missing is well the only this is a cool thing we're missing is another Chad bubble with an icon as
well as this photo and then we have this track bar bar so to implement it we can go below this ul and Below one more div
and we can create a final div right here this div will contain an image this image will have a source
equal to service 3 and we can see this image right here it can also have a class name equal to w- full h- full and
object D cover also we can give it a width of about 520 and a height of about 400 and we can give it an ALT of scary
robot I guess um and below this image we can render the video chat message as well as the
video bar which are very simple components that we just absolutely position on the screen we can style this
Diva bit by giving it a class name equal to relative this will make all of the absolutely position elements within it
properly position themselves so now we can see this chat box we can also give it an
h-20 R for the height we can give it a background of N8 a
rounded of excel an overflow of hidden on medium devices h of 25 Ram great and if we go one two three
divs down we can also render a gradient component which you cannot see on mobile really but now if you're ready for it we
can expand and this is how it looks like I think you can see the gradient right here if we go
back you can also see it on the deployed website yeah that's great and on the test website we have this wonderful
looking full image we have these two smaller ones that we play a lot with absolute positioning and it's crazy how
well everything works and looks like across different screen sizes so now if I start kind of slowly putting it like
this you can see how it collapses even more and then finally we have everything on one screen and it
still looks very very nice this bento box design has been something that I really liked recently a lot of Dev tool
companies and even tech companies like apple have started using this bento box design more and more one that it's
specifically remember that has a new website design is Clerk and if you expand it and if you check this out you
can see right here how they have this same design right it's a bit different it's not exactly the same but it's a box
in a way where you have different sizes of rectangles so here you have two and there here you have one and once you
hover you have some nice animations happening in the screen you could also play with art
design and animate so that once you hover on some you make some animations happen very modern and very visually
stunning and interesting design with that in mind we can collapse our browser and go back to our
app.jsx to start working on the next section the next section is maybe not as interesting but definitely maybe one of
the most important ones which is the pricing section here you have to be really careful of how you position it
what shows on mobile what shows on desktop in which way it shows and how does it look like so here you can see
how the premium and the middle part is kind of a bit exposed to the outside it shows kind of like on top of the basic
in Enterprise you have to be careful and you also modify the colors of the buttons depending on which one you want
them to use so this section is maybe not as interesting but it's definitely important so let's collapse it and let's
get started I think you already know the drill right we'll go to our components create a new component called pricing
dosx run rafc and then simply import and use it right here by saying pricing self
closing and there we go it's at the bottom now let's navigate into the pricing and let's start developing it
first we'll wrap everything in a section coming from SL section and we'll give it a class
name equal to overflow Das hidden then we can also give it an ID equal to pricing so that we can nicely
scroll from the Navar to our pricing section we can create a div with a class name equal to container
relative and Z index of two within it we can create another div and this one will have a class name usually it will be
hidden relative justify Dash Center margin bottom of about 6.5 REM to divide it
from the bottom and on large devices Flex meaning it will be visible and what we're developing right now is some that
you cannot see here but if we expand it you'll be able to see it it's this sphere right here that we're creating
it's a very cool visual element so we'll have to keep our browser about this size right here so we can also see it within
our development environment so moving down into this div we can render an image that will have a source equal to
small sphere coming from aets with a class name equal to relative and a z of one there we go we can also
give it a width of about 255 and a height of about 255 as well with an Al tag of
sphere there we go that's more like it now we also want to add some Stars around it so below this image we can
create a div and that div will contain an image that will have a source equal to Stars coming from assets
you can see how those stars look like right now they push the sphere to the left but we can first modify the class
name so that it takes the full width by saying w- fo a width of about 950 and a height of about 400 and an Al tag of
stars more so circles than stars but it will do and now we have to style this div so that it actually appear is
absolutely positioned so that the sphere doesn't mind the Stars around it by saying absolute you can see how now
immediately the sphere is not concerned with the Stars around it it gets taken away from the general layout then we can
play a bit with the positioning by saying top-1 over2 left -1 over2 d-60
REM minus Translate - X-1 /2 and minus translate dy-1 /2 as well and pointer-events
dnone there we go that's more like it now if we go two divs down we want to create our heading and that will be
quite convenient right now because we already have our heading component so let's pass two things into it a tag
equal to get started with brainwave and a title of pay once Ed
forever sounds a bit sketchy but who knows maybe it's good now you can only see the pay once used forever but you
cannot see the tag so let's go into the heading and make it a bit more reusable so that it actually accepts a tag at the
top and now it has to do something with that tag within this div we can recognize if a tag EX exists and if it
does we can render a tag line component like this the Stag line of course doesn't yet exist so what do you say
that we go ahead and create it we can do that by moving into our components and creating a new component called tagline
do jsx we can run rafc and we can quickly use it or import
it from the tagline so we no long longer have the error for some reason the Auto Import isn't working so I can do it
manually right here at the top by saying import tagline from SL tagline and it should be a lowercase L there we go now
at the bottom we can see tagline which means that we can jump into the tagline and start developing it this component
to make it reusable will also accept class name as well as children let's properly spell the class name and
let's start creating the layout of this tagline just to remind you it should look something like this it should have
brackets on the left and the right side and some text in the middle so to achieve this look we can first render
the children in between off a div so we can have a div with a class name that's going to be dynamic that will have the
tagline class Flex items Das Center and it will render the dynamic class name if it exists or it will be an empty
string like this now here we'll use a special component called brackets which we have to import from assets this is
basically an SVG so we can say import brackets from d slash assets SL SVG SL brackets and then we can call it as a
function and pass it the side such as the left side and after that we can also pass the
right side if we do that you can see that we have here left and right and the way it works it's basically a react
component that returns two different svgs depending on the prop that we send over and in the middle we want to render
a div and that div will have a class name equal to margin X of three to divide it a bit from the sides and text-
n-3 inside of which we can render the children now if we go back we can now pass some children into this tagline and
we can pass something like just a tag right what we're passing from the pricing section and that's already
looking better now let's position it to the center by giving it a class name equal to let's give it a margin bottom
of four on medium devices justify Dash Center and if we save it that's already better great now let's go back to the
pricing section and below this heading we want to render another div and this div will have a class name equal to
relative within here we want to show our pricing list and that pricing list will be yet another component so let's create
a new compon component called pricing list. jsx and some of you might wonder how often do we have to create
components well it's more an art than a specific answer but when a specific component starts getting too big you can
split it into multiple or if you want to reuse it across multiple places now we can import this pricing list right here
by saying pricing list and we can import it at the top by saying import pricing list coming from SL
pricing list we'll also import two additional elements called Left line and right line coming from
ddesign SL pricing and we can use those right below the pricing list left line and right
line there we go if I save it you can see that it's complaining that it cannot get it from D that/ design let's see why
is that we're in the pricing and we want to go to the components oh yeah it it is just slash design pricing there we go so
if we go here this is basically this line that you can see here and the line on the right it's barely visible but
later on it's going to act as a Connect from the left side to the right side just a small visual
detail now we can finally dive into the pricing list and start implementing our pricing section A pricing list will
essentially be just one card which will be repeated three times with some small changes so right here we can create a
div that will wrap all three of them with a class name equal to flex gap-1 REM like this and on Max LG
devices flex-wrap so they nicely wrap within it we want to map over the pricing coming from constants by saying
pricing. map where we get each individual item and for each one we want to return a div within that div we can
return an H4 and that H4 will say item. tile if we save it you can see basic premium and
Enterprise and this reminds us of this these are the three pricing cards so if you look into the pricing you'll see
that I created three different objects that contain different things such as title description price and features we
can map over so let's use all of that data to make it happen first we can give this div an ID equal to item.
ID and and we can also give it a class name equal to and while we're developing this we can also collapse our browser
because the pricing section is also visible on smaller devices we can give it a w of 19 RAM on Max LG devices w-
full h- full for full height padding X of six and you can start seeing how they're forming then we're going to have
a background of N8 for the Coler border border dm-6 rounded -2 REM like this you can
slowly start seeing how it's forming on large devices W Auto which we cannot see right now on even elements we can do a
padding y of 14 so this will only be on the middle one which is the premium on odd elements padding y off8 this will
give it different sizes just to create a visual hierarchy where the premium one will seem the most important and if you
want to you can also change the colors of these three cards for now I will simply leave it white as it is next
let's create a class name for this H4 something like H4 and margin bottom of four will do that's much better let's
see why this div is complaining oh it's missing a key prop it should have been a key instead of an ID and below this H4
we can do a P tag that will render the item. description there we go and as a matter of fact let's create the full
structure first and then we can focus on The Styling below the P tag we can create a div and this div if the price
exists so if the item. price is there it will render a div which we close and that div will say
dollar sign and then in a div below we can render the item. price of course you know know the drill whenever you have
two adjacent elements they have to be wrapped in a react fragment or another div so we can put them here and properly
close them there we go now we have the price 09 and this one doesn't have the price because it's on
demand below this div let's also render a button this will be a button component that we have created before coming from
SLB button and it will simply say if there is a price so if item. price then it will say
get started else it will say something like contact us there we go already a nice
looking button also some of these buttons can have different styling so what we can do is give this button a
class name and give it a w- fo as well as margin bottom of six we
can also give it an HRA property where if it has a price then it will lead to the pricing section else it's going to
say mail to info add and then here we can do something else like JSM dopro or let's do a real one that's going to be
contact at JS mastery. proo there we go now if we save this buttons will do different things but we also want to
change how they look like so we can say not not item. price this double exclamation mark basically turns
whatever value into a Boolean value so if it's Trudy it's going to turn it into true if it's falsy it's going to turn it
into false and since those two have a price it will turn them into white and this one will remain colored finally
below the button we want to render a ul and that UL will map over the item. features. map where we get each
individual feature and an index of that feature and for each one we return An Li that has a key of
index and it also renders an image which is our check image so source is check coming from d/ assets with a width of 24
height 24 and then I'll tag off check there we go let's also add a P tag that will
simply render the existing feature there we go so this is our card but now is the time that we style it so let's scroll
all the way to the top to our description and let's style the description first by giving it a class
name equal to body -2 m-h D4 REM to give it a bit of height margin bottom of three text- n-1 over
50 there we go then we want to style this div containing the price by giving it a class name equal to flex items Das
Center h-h 5.5 REM and margin bottom of six then the div with the dollar sign will have a class name of
H3 and the div with the price will have a class name equal to text- 5.5 REM and leading Das
none as well as font Das bold that's a big price 0 and 9.99 then we want to scroll down to the UL specifically to
the Li and give it a class name equal to flex items Das Center or rather I think we're going to do start here makes more
sense padding y of five border DT for top and Border D n-6 there we go that's more like it and
finally let's style the P tag by giving it a class name of body -2 and margin left off
four there we go this is our pricing card of course it doesn't look that interesting on mobile but if we now go
back and zoom it out you can see we have a nice looking card but this definitely isn't centered like it is on the
finished website so we have to center it but our cards are looking good another thing we can do is push this Center card
a bit up so that it appears on top of the other two by scrolling up and saying odd margin y off
four as you can see now it seems like it's a bit bigger then we can also play with the colors a bit you can see how
much better it looks like here and we can do do that by using some special properties specifically square brackets
and then n sign greater than H4 so we're targeting the H4 and we're saying first text- Coler
das2 this will now give the first one a Coler of two then we can Target a second one by copying this and saying second
text- Coler two which won't work so the second doesn't exist only the first one but
what we can do is we can say even right so we can say even which is the middle one right and we're going to give it a
Coler of one and then I think maybe you can guess it if we repeat it one more time we can say odd text color of three
or rather there's a special property of last and this is going to give the last one a special call
great now let's Center this pay once used Forever by going back to the pricing and I believe that's within
heading here we can also add a property on medium devices to do a text Dash Center and that will properly Center it
now we're almost done with the pricing section besides one small detail at the bottom which is see the full details in
case you want to explore what else are you getting with each one of these pricing options so if you go back to
pricing and go below this div containing the right line we can render another div with a class name equal to flex justify
Dash Center and margin top of 10 and within here we can render an anchor tag that says see the full
details and there we go we can also apply a couple of class names to this anchor tag such as
x-xs font Das code font Das bold and we can also collapse our browser to see it like this there we go
see the full details let's also give it a tracking Dash wider which will make it span a bit more and give it uppercase
border dasb and we can give it an href pointing to
pricing great with that in mind we are done with the pricing section which looks and feels great on all different
screen sizes after the pricing let's see what other section do we have this time we have what we're working on section
and this is an interesting one as you can see once again a lot of stuff is moving here we're going to reuse our
tagline component I think you can see the similarity right so if you're wondering why this was a custom
component this is why we are reusing it here then we have the different boxes saying what the app can
do and once again all of these are custom elements which we will develop and then we have the road map so you can
see that we'll essentially develop one of these cards and then create the layout and then just call it four
different times which is quite exciting so let's go back to our app let's collapse it and let's start developing
our next section called roadmap so we can create a new component called Road
map. jsx run RFC and then simply import it right here by saying road
map import it from components and just use it and there we have it so to get started with it you know the drill we're
going to definitely reuse our section component which we can import from that/ section now that we have it we can also
give it a class name equal to overflow Dash hidden and an ID equal to road map then we can have a div within
it that will act as a container by giving it a class name of container on medium devices and larger will also give
it a padding bottom of 10 and we can immediately display a heading property this heading of of course we can import
from heading that's slhe heading of course and we can give it a tag equal to ready
to get started and a title of what we are working on and immediately looks so
great we just wrote one single line right below it we can create a div and that div will act as the container for
all four of the road map cards by giving it a class name equal to relative grid a gap of six on medium devices grid
Das calls D2 on medium devices gap of four between those grid items and on medium devices a padding bottom of seven
R and now we're going to map over our road map of course first we have to import it by saying import
road map coming from do/ constants and this road map looks
something like this once again it is an array of four different cards that have a title text date status image URL and a
colorful true or false property so we can say map where we get each individual item and for each one we can not
instantly return rather open up a function block to First figure out the status of the road map and Status can
say equal to if item. status is triple equal to done then we can set it to done with an
uppercase D else it will be in progress we will use this right here you can see how this one says done this one says
done this is in progress and so on so now we can say return and then we can return a div what do you say that we
first create the structure for it just like we did before and then later we can apply The Styling let me know down in
the comments what you prefer and also let me know how it is following these videos where I type all of this code do
you type in real time do I speak too fast or too slow just let me know and I will try to make it better but with that
in mind within this div will first give it a key because we're mapping over it of item. ID within that div will create
a second div for layout and within that second div we'll create a third div for the layout as
well within that div we can then display an image that image will have a source equal to
grid which we have to import from data/ assets and if we save it looks like we didn't close something properly maybe we
had too many divs oh yeah I'm missing the ending sign right here that's good and now we should be able to see the
image but yeah you can see the Grid in the background it's barely noticeable but it's there we can also give it a
class name equal to w- full a width of about 550 and a height of about 550 and then I'll tag of grid below that image
and the div It's contained within we can create another div this one will of course close and we'll give it a class
name equal to relative and give it a zindex of one just to know where we are within that
div we'll create another div that will have a class name equal to flex items dcent enter and justify Dash
between and within it we'll render the tag line coming from tagline which will render the item. DAT there we go now we
can see four different dates appear right here below the tagline we can render another div and within that div
we can render an image this image will have a different Source depending on its state so if source is equal to if item.
status is triple equal to done then we can render a check two else we will render a loading one and make sure to
import them from the assets we can also give it a class name equal to Mr of 2.5 like this we can give
it a width of about 16 and a height of about 16 and the AL tag can be static
now we cannot see it on mobile but if I expand a bit we should be able to see that icon no not yet later on it should
look like this we're rendering the check mark oh that's because we are on the dark background so we cannot yet see it
even though it's there later on we'll add some color to it and below that image we can render a div that will have
a class name equal to tagline and it will render the status so now you can see in progress
and right next to it is the icon that we cannot see right now finally if we go one and two divs down we can render
another div this div will have a class name equal to margin bottom of 10 and within it we'll render another image
this is the most important one that will have a source equal to item. image URL it's also going to have a class name
equal to w-o a width of about 630 and a height of about
420 and an Al tag of item. tile that's going to look something like this we have four of these different
images now in not so good of a layout so let's go here collapse it and yeah this is definitely not that close but we're
getting there below this div containing the image we can render an H4 that will render the item.
tile there we go Chad bot customization and below the H4 we can also render a P tag which will render
the item do text there we go that's looking good immediately we can give this H4 a class name of H4 and margin
bottom four and we can give this P tag a class name equal to body -2 in text-
n-4 there we go so I think you can see what's happening here this all of this is a flex layout each card but right now
it's a flex row whereas it should have been a flex column so let's scroll all the way to the top and let's start
implementing our class names by adding some additional styles to our divs we can start from top to bottom
with this div that has the key of item id we can give it a class name equal to it's going to be a dynamic one on medium
devices it will be Flex on even elements and medium elements we can run a
translate of Y 7 Ram to kind of move it a bit this will allow us to have that layout where not all are just appearing
in a single line whereas desktop devices one is up one is down and they seem kind of dynamically flowing on the screen
let's also give it a padding of about let's do 0.25 and rounded Das 2.5 remm next we
want to figure out if it's a colorful item or just a regular item so we can open up a dynamic code block and say if
item. colorful question mark and then we can say BG dasc conic d
gradient or else we can say BG D n-6 and save it and of course let's remove this last Dash and there we go
now you can see it so if I expand it you can see that all four of these or all three of these look a bit pale whereas
this one really stands out as that's the last thing we have done within our road map so going a bit back we can continue
styling it by styling this inner card by giving it a class name equal to relative
p-8 for padding BG D n-8 rounded dash2 remm overflow Das hidden and on extra
large devices we want to bump up the padding to 15 the div within it will have a class
name equal to Absolute top- z left- z and a Max DW of full this is the background grid so
we're wanting to absolutely position it so it doesn't take away from the display of the other elements then we can scroll
down to this div with relative or rather to this div with flex finally we can give it a Max dw- 27 R for the max width
margin bottom of eight and on medium devices margin bottom of 20 then we want to go below the tagline and give it a
class name equal to flex items Das Center padding X of four padding y of
one BG off N1 rounded and text- n-8 we can scroll down specifically to this div wrapping
this image we can put position it a bit better by giving it a minus margin y of 10 and minus margin X of 15 and even
after applying those Styles it looks like the layout is still not there I've had some issues with my divs not closing
automatically as you can see I had to close them manually which I haven't done in a long time so it messed up my UI and
this was a long component so in case something is messed up with your component as well no worries you can go
to the description down below go to the read me and then copy and then paste it right here the only thing we have to
change is this tagline to lowercase L if we save that you can see that all of the changes we've done have been
successfully implemented so if we scroll a bit up we have this grade section that has this item colorful which applies a
BG con gradient and then we have three additional ones which have no gradient but all of this looks amazing and we
haven't yet checked it out on desktop so if I expand it you can see how great this looks like it's similar to a Bento
Box that we have seen before but this time it goes one two and then again moving three four right here it's a very
Dynamic and interesting layout great and also at the bottom we have our road
map finally the last thing we have to do is the footer we can see how that footer looks like it's a very simple and
minimalistic one and once again we utilize those crosses which we have implemented before so let's collapse our
browser go back to our app.jsx and implement the footer of course we have to first create
it within the components footer. jsx run RFC and then simply import it right here like it was always there now you can see
the footer which you can navigate into and we can start implementing it it will be as easy as turning it into
a section coming from do/ section that will have crosses turned on with a class name off exclamation mark PX of0 so
we're just resetting the styles to zero right here and using exclamation mark for important and same thing for padding
y of 10 because this section is a bit different from our typical sections as it's a footer within it we can have a
div that will have a class name equal to container Flex on small devices justify Dash
between justify Das Center usually items Das Center gap of 10 on Max SM devices which
is minwidth of 640 we want to apply a flex column because this is on very small devices and if we save it you
cannot see anything yet but now we can add a P tag that can say something like we can get a new date like this by using
a dynamic block and saying new date and then do get full year this way you don't have to go back and manually updated and
we can say all rights reserved there we go go if we save it there there it is we can also add a copyright sign which you
can just Google and then paste over here that's just the C and we can add a DOT after the year there we go we can also
style this B tag by giving it a class name equal to caption text- n-4 and enlarge devices block okay it's
very muted not in your face but it's there and then we want to go below the P tag and form the UL give it a class name
of flex gap of five and flex-wrap and there we're want to map over our social icons by saying socials imported
from constants map where we map over each item and each item is an anchor tag
containing an image with a source s equal to item. ion URL with a width of about
16 a height of 16 so it's very small and then I'll tag of item. tile now you can see all of these social icons and we of
course have to give this Anor tag a key equal to item. ID an href equal to item. URL so we can navigate to it and a
Target core blank so this will open it in a new tab and not override the current
URL finally let's style this anchor tag a bit by giving it a class name of flex items - Center justify Das Center w-10
h-10 BG of N7 rounded Das fold to make it into a circle transition Dash colors and then hover
BGN of six so now if you hover over them you'll have this nice looking transition this is great and with that believe it
or not our footer has been implemented which means that we are ready to expand our browser and check this out the
crosses are here the road map is here the footer is here the Navar is here and you can click on different parts of the
website to scroll through them you can also click on brainwave to to check out our amazing
homepage congratulations you came to the end of this video and you have developed the entire brainwave application now
comes the exciting part we are ready to share our project with the world which means we need to deploy it to the
Internet so to do that open up your hostinger dashboard and navigate over to your hosting and the main name for this
project once you're there go to file manager here this will open up an H panel where you'll be able to drag and
drop the files of your application there we go you can head over to public HTML delete the default PHP and we are ready
to upload but the question is upload what well in this case we have to run mpm run build and you can see this
within your package Jon if you go to scripts there is a build script so go to a terminal
stop it from running and then run mpm run build this will create an optimized production build of our application in
just under a second it will create it under a new folder called this which you can see here right click it and click
revealing finder or opening file explorer and the only thing you have to do is expanded and then copy and paste
everything from here to our H panel this process should take just under a minute or under a second and you can go
back to our dashboard finally click your domain name just like that in a second we have deployed and hosted our
application and all of the amazing things we have implemented this is looking great as you
scroll down you can notice that everything is here and everything works wonderful with we have our pricing we
have our road map section which we have implemented not that long ago and our footer and if I click you can see how
fast it loads and also we must not forget that it is https secured which you can see by going to security SSL and
you can see that the SSL certificate has been applied and with that done phenomenal job on coming to the end of
this video I'm very proud of you since you came to the end you you might be interested in our ultimate nextjs course
hosted on JS mastery. proo the link will be in the description now you have built a vit application but you're definitely
ready to dive into everything that NEX gs14 has to offer and this is not just react some people think it is but it's
more many companies use it but you can end up using it just like good old react which is not going to work because your
performance won't be good so in this case I want to teach you how to have your performance be at the top levels
and through a specially crafted process where we go through a series of Deep dive lectures with a lot of
illustrations explaining the ins and outs of nextjs where you build and deploy a very complex app and with
active lessons where you not only watch the tutorial but also participate in building it by by being given a askas
examples resources and even hints to develop it finally you'll build the complete stack ORF flow clone but an
even better version of it a modern Dev overflow application which look which looks and feels amazing so with that in
mind if you want to dive in and the text stack of 2024 and Beyond just go to JS master. proo and join over 3,000
developers that have already enrolled with that said thank you so much for watching this video and I'll see you in
the next one have a wonderful day ever scroll through a website and been blown away by its seamless
animations and Sleek design what if that website was your portfolio leaving every visitor impressed and eager to see more
of your work sounds cool right welcome to a new video where you'll build and deploy a minimalistic yet modern
developer portfolio with Sleek animations using none other than nextjs with a modern hero section with
spotlights and text animations latest Bento grid design here we went a step further and animated some parts into
this grid for example this globe is a real 3D Globe which you can move around then we have our text stack right here
some more information about yourself even some code blocks and then this nicely animated bottom part that allows
your users to copy your email moving below we have a small selection of recent projects and what's cool about
this and the previous section as well is that we're not only using Tailwind we're also using exterity UI which gives us a
lot of these outof the boox frameware motion animations and that makes it look different from anything I've seen so far
testimonials and companies you've worked with also a section about your previous work experiences about being a front-end
engineering intern mobile appdev freelance project and so much more and don't forget if you scroll up you have
this nice bar that pops up that allows you to scroll to different sections moving forward we have my Approach which
tells employers how you work starting from planning and strategy to development and progress updates to
development and launch so you're not just doing development you're doing so much more than that and a short but
sweet footer section and while you're building this portfolio you'll learn next GS with server and client side
rendering routing and layouts for better performance and SEO Tailwind CSS for a modern complete completely mobile
responsive UI exterity UI for sick animations using frame or motion and Sentry monitoring how many people have
visited your portfolio now with even better teaching methods where you'll get a free open- source code base you can
look at if you get stuck and a custom figma design so you know exactly what you're building yep that's it simple yet
stylish so are you ready to make this your new portfolio alongside this portfolio project I've also prepared a
comprehensive guide on making sure your portfolio has all the sections that it needs and that it stands out and for the
first time ever there's now a dedicated Discord Forum where you can get help if you get stuck building this portfolio
the links are in the description and it's all completely free now a developer portfolio is not like any other project
it is a career asset designed to Showcase everything you're capable of making you an attractive candidate for
any job and that's why I'll teach you how to go a step further see hosting your portfolio on a personalized domain
makes you more professional memorable and improves your credibility and search engine visibility so before we dive into
coding let's get that domain name I highly recommend hostinger for hosting your modern portfolio and right now
they're offering a great deal which you don't want to miss when it comes to hosting This Modern portfolio I
personally go for the Premium plan and here's why it's perfect for this and many other projects you can host a 100
websites with the same account you get a free domain name which gives you a professional touch you get a free SSL
for https security which creates trust with anyone visiting your portfolio this deal won't last forever and because I've
partnered with hostinger you get an even bigger discount once you click the link in the description click claim deal and
add to card here we'll have to choose the period of our hosting with the crazy discount going on right now I'll
definitely choose 48 months to save the most money down below you can choose your payment method and enter your
JavaScript Mastery coupon code which will give you an even bigger discount on your hosting package and once you buy it
we are ready to set everything up and get started with building out our portfolio let's start with hostinger
guided setup we are building a new website so we can choose create and we're building a completely custom
website so we can choose skip at the bottom next we need to choose our domain as you can see I use hostinger for my Js
mastery. proo platform so here you can type out your domain name I would recommend something like your first name
and last name.com something like john.com and if you purchase the hosting for more than one year you'll get the
domain completely for free choose it and click next the main is registering and while that is happening I quickly want
to point your attention to our improved Discord server where now we have dedicated forums for each video so if
you're building this portfolio and you get stuck you just go to the Forum and ask a question this is the best way to
get help now already we are the next step where you can choose where your audience is located at and you can see
there's a lot of options click next and the setup will initialize while that is happening just another quick note and
that is that the full code base of the video you're about to build is live on GitHub so you can check it out right
here and if you don't mind give it a star with that in mind we are redirected to the dashboard of our hosting project
so finally let's code it out and we'll deploy it at the end of the course to get started building our minimal yet
modern nextjs developer portfolio we'll start as we always do from bare Beginnings by opening up an empty Visual
Studio code window and creating a new folder on our desktop and then simply drag and drop it into your Visual Studio
code once we're there we can initialize our project now before we begin let me show you which software we'll use to
develop it of course we'll use react the library for web and Native user interfaces that allows us to use GSX
syntax which merges JavaScript with Styles and HTML directly on top of react will also use the official react
framework for the web nextjs used by the world's largest companies enables you to create highquality web applications with
the power of react components so here we'll get the Speed and Performance while still writing very similar reactjs
code to style our application we use Tailwind CSS a utility first CSS framework packed with classes that will
speed up our styling process but then we'll power it up with framer motion a production ready animation library for
react which allows us to play with a lot of different animations but we won't be using frame or motion natively creating
all of these gestures variants and more on our own we'll use a Stern UI to make her websites look 10 times more modern
they allow us to copy and paste the most trending component components and use them directly in our websites without
having to worry about styling and animations it's completely free and open source and in today's video we'll be
using many of their most popular components almost our entire website will be powered by exterity components
everything from Scrolls to this globe you're seeing right here animations and so much more after that we'll have to
monitor our application and check for brakes so we can fix them faster we'll do that using Sentry and finally we'll
use hostinger to get our domain name and very fast servers so we can deploy our application and share it with everyone
with that in mind let's initialize our project to initialize it we'll use next js's starter so simply go to NEX js. org
and copy this starting command back in our Visual Studio code paste it and add slash at the end which will make sure to
initialize it in the current repository we're in press enter and we'll have to go through a couple of questions we
would like to use typescript in this case and if you have never used it no worries I'm going to show you how it
works we will use es length to make sure our codebase is clean tailin CSS that's a big yes no Source directory it's not
needed app router yes no need to customize things and in a matter of seconds our base file and folder
structure will be set up let's run our application by running mpm runev hold control or command and press
this Local Host 3000 URL it will open up this starting nextjs template and I put it right here side by side with my code
editor so we can see the changes that we make live and also I will open up a new terminal as that way we can easily
install additional packages later on for now let's quickly explore the code base we have the app folder which is where
we'll spend most of our time here we have our favicon which we can delete as we'll add a new one later on and we have
our globals where we import some base components and utilities we also have some predefined nextjs Styles which we
can completely clear for the time being after that we can quickly check the layout and the layout is responsible for
our font in this case we'll be using inter and here we can add our title and description of the application I'll say
Adrian's portfolio you can say of course your name and I will say something like a modern and
minimalist JS Mastery portfolio feel free to put whatever you want here these changes should be visible in your
browser tab for this website immediately after we can head to the page. TSX and delete everything within the return of
this page so let's scroll all the way down and instead let's return an HTML 5 semantic main tag which is similar to
the div give it a div within it and for now say something like H1 that will say hello
portfolio if we save that you should be able to see it on the screen and let's also give it a couple of class names to
check whether our Tailwind CSS is working let's give it a class name equal to
relative and by the way as I'm typing you'll be able to see which CSS properties are being applied given a
specific Tailwind CSS class name you can also get access to that by simply hovering over it and this will only work
if you install a Tailwind CSS intellisense extension so simply search for it install it and it should work it
will also show you some things like classes like if do BG black 100 or BG black it will show you the exact color
you're using as well as all of the CSS properties getting applied later on we'll also use es7 plus react redax
react native Snippets we'll use this to quickly spin up our components so make sure to install it as well now in this
course I'll also show you how we can customize our Tailwind CSS config and we'll do that by referring to to our
complete figma design at all times from here we'll be able to extract colors fonts paddings and spacing and more you
can download this entire design for free the link is in the description with that said let me show you how to add our
first custom color we can go to Tailwind doc config.txt our existing theme we can
remove this background image for now and we'll extend colors specifically we'll extend the black
color by modifying the default color like this to Hash 0000 so this is a complete Black and then we can add
different variants of that black color such as black 100 and we can make it hash
000031 9 this value of course we can extract from the figma design now what this allows us to do is go to base p.
TSX or any other page for that matter and refer to BG black 100 which will now give us that color that way we don't
have to repeat 0000 319 every time and do that for all the other colors we can just declare it once and then use it
like this every other time let's also give it a flex justify Dash Center to center it horizontally items Dash Center
to center it vertically we'll switch the orientation by saying Flex Dash call as in column and we'll make it overflow
hidden so we don't have those scroll bars margin X will be set to Auto so this will allow us to give some space to
the middle part of the screen and then we can also use Tailwinds builtin sizing functionalities for mobile
responsiveness such as SM which stands for small and give it a padding X off of 10 so this will apply a padding left and
right meaning X because it's on the horizontal axis only if the media minwidth is 640 pixels so this means 640
and up and we can also give it a padding X of five on all other screen sizes let's also style this div inside it by
giving it a class name of Max dw- 7xl this is for Max w and w-o and within here we can add our
content so what do you say that we start with our first section of the day the hero section with this top title primary
title another subtitle and a call to action button to show our work we can start by creating a new folder in the
root of our directory called components and within components we can create a new file called hero. TSX hero
typically stands for the primary section that you see above the fold once you first visit the page and here we can use
that extension if you type rafc which will allow us to quickly create a react Arrow function with an
export that's coming from that extension es7 Plus react redex Snippets if we do that we can go back
here and we can easily import it by saying hero coming from comp components hero it auto imported it right
here so let's give this div a class name equal to padding bottom of 20 and padding top of 36 and let's create a div
which will act as a wrapper for our first components coming from eity if you take a look at our final design maybe
it's not so obvious on the mobile mode but in our design at the top you should be able to see these spotlight effects
which are beams of light shining to the middle of the screen it has a very nice visual effect so let's figure out how to
use it you can navigate over to exterity and they have this pretty cool sear which now you can find across many
websites where if you press command K it will open up a search no matter where on the page you are and this is a huge Pro
tip as a matter of fact this is so important that I will expand my screen and show to you how I use this feature
on a day-to-day basis like in this case I want to go here search for spotlight press enter and here I am but it's not
only for UI like if we go back to the official nextjs documentation press command or control K and search for
something like server actions and immediately here you are and then I would use command F to find something on
the screen like use server so this matters a lot using your keystroke like controller command F and controller
command K to quickly Traverse the docks and find exactly what you're looking for so going back to exterity let's look
into Spotlight a spotlight effect with Tailwind CSS good for drawing attention to a particular element on the page here
we can see how it looks like and right below we can see the installation I believe most of these have already
gotten installed when we installed Tailwind but just to be safe and because I know we have to install framer motion
let's copy it go to our terminal and paste mpm install framer motion and all of these other packages then we need to
add a util file which I believe was already added for us by tailn CSS but we can quickly check it out it should be in
utils cn. DS so if we go here and search for utils no I cannot seem to find it so let's create a new folder called lib as
in library and within it let's create a new utils.py
this is just a helper function to make everything work together with Tailwind after that the next step is to copy the
source code they don't even need us to expand it we can just simply press copy right here and let's see where it needs
to go it's uis spotlight. DSX so let's create a new folder Within components called UI and this is where
all of our exterity or shaten components would go so simply create a new file called spotlight.
TSX and paste what you just copied you don't necessarily need to understand everything that's happening here but if
you need to change something you can we will actually modify a couple of these components later on and immediately I
can notice how it's trying to find utils CN but I called it lib utils so it might be better to follow along and do exactly
what they say by creating a utils folder and then cnts inside of it so let's do that instead of lib I will call it
utils and then instead of u. DS I will call it cnts that way our Spotlight will no
longer complain and now we can use it within the hero section so let's use our first
Spotlight component by importing it from /ui and this is kind of the beauty of chaten and exterity and that is that you
have full control over the components you use not like in material UI or some other UI kits where you have to install
the entire Library here you only import the code you actually want to use and another benefit is that to all of the
components from shaten or aity you can pass native tailin CSS classes so we can position it exactly how we want let's
give it a class name of minus top-40 minus left d10 and we can then also further
position it on medium devices minus left 32 on medium devices again minus top-20 and then full height by saying h-
screen we're just making sure it looks good on all different devices and we can give it a
fill equal to White now it might be a bit hard to see it on mobile devices but before we expand it
let's duplicate it two more times and let's also change it up a bit so we have multiple spotlights for the second one
we will say top dash 10 left- full and then we don't need any other medium properties rather I'm going to give it
h-80 VH so this is 80% of the vertical height of the screen and w- 50 vertical width of the screen and fill purple to
have a bit of a different color finally for the last one I'm going to give it a top of
28 not minus top and I will give it a left of 80 to just position all of them a bit differently and I will also remove
all of the previous Styles and give it h-8 VH and w- 50 vertical width and a fill of blue that should give us enough
variety now even if you expand this you won't be able to see anything right here we can even try to maybe inspect
elemented but yeah basically nothing is there so why is that well if we go back to exterity documentation and scroll
down you'll notice that we forgot to copy the highlighted Spotlight animation into the Tailwind doc config.js file so
let's copy this entire config go to our Tailwind config and paste it right here of course this way we have lost our
colors but we'll bring it back later on don't worry about that also if you press command K and go to add utilities you'll
notice that we also need this flatten color pette we need to add that to the Tailwind config as well and now we would
have to copy this once again and then override our tailin config and we would have to continue adding new stuff every
time that we choose a new new component and for that reason I've already prepared some code in this completely
open- Source repo so we can get started more easily so if you scroll down to the read me section you'll be able to go to
code to copy and here you'll see that we have this twin. config.txt all of this code is not
something we write by hand so don't worry about that it is just the code coming from exterity and also it
includes some more colors that I have extracted from the design other than that we also have the global. CSS which
will make our styling just a bit easier so let's copy it and paste it to our global. CSS within the
app and later on we'll come back for the data as as well as the assets we'll use throughout the project now as soon as
you have updated these files you'll notice that we have to install some of these additional packages such as mini
SVG data URI so copy its name and run mpm install mini SVG data URI we'll also need tailin CSS animate
so let's get that one as well mpm install tailin CSS animate and just like that we are back
in action and I'm not sure if you can see it on the screen but I can see a light beam coming from top left moving
to bottom right and on full screen it's even more visible it is subtle but it is there and it'll be much more visible
once we expand our hero section to the full height of the screen and right below the div containing these pot
lights we want to create another div and that one will act as a UI grid and for that one we'll use a component called
grid and Dot backgrounds in this case we want to use this grid background so let's switch over to code right here and
let's copy these two divs with a P tag in between let's properly open it up and let's also indent it if we save
that you should be able to see a grid appear right here so let's quickly modify a couple of classes by making it
h- screen so it takes the full height of the screen W full on dark BG black 100 that is our specific variant of black
typically BG white on dark mode we have this BG let's switch this over to 0.3 I found that to work better with our grid
and right here in this inner div we can also change BG black to BG black 100 and then we don't need anything in it we
just want to use the the background so we can just make this self closing now obviously this is not what
we initially anticipated right we can see that it actually has a white background instead of a dark one and
that's because exterity supports both white and dark mode but our entire application will be dark so let's use a
theme provider to switch it to dark to achieve that we'll be using a package called Next themes and if we scroll down
you can see that it has half a million weekly downloads and I'll show you how to use
it first let's install it by running mpmi next themes and then if you just Google next themes you should be able to
find a shaten dark mode and you can see that that's exactly what they do right here so we want to follow the setup
first thing we have to do after installation is create a theme provider so let's copy this code go to app and
create a new provider. TS x file and then simply paste it right here after that you want to wrap your root layout
so copy this theme provider layout go to the layout file and simply wrap your children with it so we can do it right
here within the body we can of course indent it properly and import theme Provider from that SL provider next if
you want to you can add a toggle that will then allow you to switch between a dark and a light mode but in this case
we can set the default full theme to dark and save now if we go back you'll see that our grid turns dark so now
let's go back to the hero section and below it let's create a div that will wrap our heading make sure to do it
below this div containing our grid and let's give it a class name equal to flex justify Dash Center and
before we continue adding some other classes to it let's first add an inter div that will have a class name of Max
dw- 89 VW I found this value to work the best and then right within it we can render an H1 that will say something
like Dynamic web magic with next.js and let's make this H2 because this will be a subheading so if we save this you
can see it right here at the bottom so let's go ahead and make it a bit bigger by giving it a class name and say
uppercase give it a tracking Das wiist this will make it span out give it a text- XS text-
Center text- Blue 100 and Max DW of 80 now let's also style our first div that's wrapping all of this by giving it
a relative a margin y of 20 a zindex of 10 and now we can style this inner div by giving it a medium device
Max dw-2 XL on large devices Max DW of 60 VW Flex flex-all items Das Center and justify
Das Center there we go here it is now we want this text to appear over our grid not below it which means that we have to
make our grid container a position of absolute so right here at the end we can say absolute top- z left- z and we can
remove the relative as we're making it absolute right up the bat that will make it appear on top and let's also make
this wi grid a bit less noticeable by giving it a 003 color of course you can play with the values and find one that
works best for you now if we scroll down we want to render our primary text to make it look exactly like it does in the
design and it's not just about adding the text it's giving it its animation so for that reason we can go back to
exterity and we can search for text generate effect and you can see how that will look like so let's copy the source
code and add it over to components UI text generate
effect. CSX paste it here and now we can use it right below the H2 by saying text generate effect and to it we need to
pass two props and you can even see the props we can pass here the first one is the class name and the second one is
words this is a pretty simple and straightforward way to say hey what you want to render here so we can say
something like transforming Concepts into seamless
experiences and no this was not written for me by chat GPT there we go let's also style it by giving it a class name
of text- Center text- 40 pixels that's for the size on medium devices text- 5 Xcel and on large devices even bigger
text off six Excel and now if you reload you can immed mediately notice how nicely it flows in right below this one
let's render a P tag that will have a class name equal to text- Center and here we can say something
like hi I'm Adrien and you can use your name here let's also use this apostrophe sign
instead of just using apostrophy here and we can say something like a nextjs developer
based in Croatia let's also give it some Styles such as on medium devices tracking Das wider margin bottom off
four to divide it a bit text Dash small on medium devices text- large and on large devices text- toxel now let's
enter into the text generate effect this code that we copied and let me show you how we can modify it directly let's
first scroll down and here we have a motion span we can make this class name Dynamic just like
so and we can say if index is greater than three of course that has to be within a dollar sign in curly
braces for it to be dynamic so if that is the case then we want to give it a text purple and else we can give it the
rest of the Styles dark text white and typically text black so we can paste that right here and in
all cases opacity zero this will now turn the experiences into purple let's also scroll a bit down and instead of
margin top four let's give it a margin y4 which will divide it from the bottom part two and let's remove the text 2
Excel from here this will make it bigger so now you know that you can make modifications even to the code that you
copy finally in our final application we also have have this phenomenal looking button with this nice effect so let's
implement it too if you can guess this will be yet another exterity component so going to exterity Let's search for a
button go to tailan CSS buttons and we can pick one that we like this one has lit up borders and
this one has border magic so let's just copy it and create a new component within UI called Magic
button. TSX and here you can paste the code or rather it will copy just the return value of this button so we first
have to copy it back and create a new react error function component and now we can paste that
button right within it and indent it properly now if you go here we can call
that button right below our P tag by wrapping it in an anchor tag with an hre pointing to forward
slab and we can render a magic button coming from that slashmagic buttton we can pass it a title equal to
show my work and if you do that immediately you'll be able to see this border magic
button so let's go into it and let's make it a bit more Dynamic first let's make it accept some props such as a
title an icon a position a handle click so what will
happen once we click it and some other classes which we might want to pass and then since we're using
typescript we can now Define all of those here title of a type string icon of a type react. node or react node I
think it is position of a type string handle click which is going to be
optional and it will be of a type function that doesn't return anything so void and other classes which will also
be optional and it will be of a type string if we do decide to pass it so
immediately we already are passing the title so we can accept it right here show my work great let's also style it a
bit better we can give it a value of w- full for full width and change the roundness to rounded LG which will make
it a bit more rectangular and then we can remove those Focus rings so going all the way here after Focus outline we
can remove those other focuses I don't think we'll be needing them we can style it a bit further
though by giving it a medium devices width of 60 on medium devices margin top of 10 and I think that'll do it for now
and we can also style this in our span right here by changing this to rounded LG instead of rounded full now it's
rectangular we can change the padding to something like seven to give it a bit more padding padding y I don't think
we'll need in this case and here we can render some of the other classes if we are passing them but for that to work
we'll have to turn this into a dynamic template string and then if we are passing any icons we
can render it either on the left side or on the right side so if the position is triple equal to left then we will render
the icon right here else if the position is triple equal to right we will render it right
here now we're not passing any so we cannot see it but this is it for our button and now to this button we can
pass an icon by saying icon is equal to and now you could use a regular PNG or SVG here but I'll show you a better way
there is a great package called react icons which features icons from all of the different most popular
packages so you can simply install it by running mpm install react icons and after that you can use it by
simply importing an icon so let's import it I'm going to use use an fa location Arrow which is coming from
fa6 and we can also declare its position let's do right in this case so now we have this great looking icon but we do
need to provide a bit of spacing here so let's go into this button and let's add a gap of two right here which will
create some spacing this is looking great and with that our hero section is done so check this out it looks even
better on desktop I'll reload it and notice how the text slowly comes in and we can also see some spotlights appear
although I can mostly see just one this blue one coming from top left and if we compare it with the final version you'll
be able to see well first of all that I forgot to add user experiences which we can do right here seamless user
experiences but besides that you can see that here we also have this great looking navigation bar I'm not even sure
if we can call it a bar as it hides when you don't want it to be there and it shows up when you want to scroll so it's
a pretty cool take on a regular navigation bar let's go ahead and implement it back in exterity we can
search for floating navbar and we can copy the code add a new UI component
floating na. TSX and and paste it right here this one is a bit longer as it contains some navigation logic so let's
go back to page this time and let's import it right here floating nav to it we'll want to pass one prop though and
that is nav items and you can see that clearly right here the navigation items you want to render in the nav bar and
this shows you how important it is to read the docs and typically all components you use have some kind of
props you want to pass to them of course we're using tab script here so it's very useful to just get an alert that
something's not right right within your Visual Studio code and here you can also see the type it will be an object with a
name link and an icon so let's try to pass one nav items is equal to an array of these different items for now let's
simply say home with a link of forward slash and a react node of something like fa home if we save this go back and
reload we cannot see anything yet and I wouldn't worry too much about that for the time being as we technically cannot
even scroll anywhere and the way that the floating nav works is that it only appears when you scroll down and then
move up a bit so it allows you to navigate faster to where you want to go so we'll be coming back to styling this
nav further and passing it the right now I items a bit later on once we add one of the coolest sections of this course
so let's create a new component in the components folder called grid. TSX and here you can run
RFC and we can import that grid right below the hero once it's there we can navigate to it and we can use another
exterity component this one it will be a Bento grid a skewed grid layout with title description and header components
you can go to it by searching command K and then searching for bento grid on desktop devices it has this very cool
layout where elements in different rows can take different widths so let's install it by copying the code and
creating a new UI component called Bento grid. TSX and we can paste it right here and this component actually exports two
components a Bento grid and a Bento grid item so let's import them within our own grid component by turning this div into
a section giving it an ID of about section so we can easily scroll through it and then immediately importing a
Bento grid component inside of which we can render an array of objects with some kind of a
title let's say Title One and also a description which can be
description one and now we can map over that array where we get each individual item and also an index of that item and
for each one we automatically return by automatically I mean within parentheses not opening another function block here
a bentto grid item that is that second component which we have imported from exterity now this bentto grid item does
accept a couple of props to it and we can see that to props there we go title header icon and more so let's give it
first an ID since we're mapping over it of item. ID let's give it a key equal to item. ID as well so we don't need an
index at all in that case we can also pass it a title equal to item. tile and we can pass it a description
equal to item. description and we also need to actually give it an ID right here let's do ID of
one now we cannot seem to see what's happening now there is nothing there so let's get into this Bento grid item and
see if we can modify the code to make it work with our design the only thing I want to do for now is give it a border
of two and border of red 500 oh I think it already had a border applied so we don't need this border of
two and this red 500 will ensure that we can see it once it appears on the screen but right now there seems to be an issue
with the ID it says that we're not accepting it within a benog grid item so let's accept it we can say
ID and Define the type of that ID equal to number so we know we'll pass a number and we can also make it something to one
right here so now it's better but that on its own will not make it work so to fix it let's modify it Styles directly
by changing the rapper div class names instead of rounded Excel we can make it a bit more rounded give it a position of
relative and immediately you should be able to see the card appear right here and that's good right now because we
know that at least we are rendering whatever is in here but now where are we actually going to keep this data we
won't really create different objects right here directly within this file that wouldn't look good would it
something like this where we would have multiple of these cards right here within our code with different titles
descriptions and more especially considering that there's going to be a lot of text within each one of these
cards some will even contain special elements within it so for that reason what I often like to do is create a
separate folder within the root of our directory called Data within that data we can create a new
index.ts and then within it we can declare those types of data arrays such as this one so let's declare it by
saying export const grid items is equal to an array of these elements so now we can go back here and just import grid
items save it and immediately the same cards appear but now our code is looking much cleaner
while the data is extracted here so even a non-technical person can come here and edit something if they really want to
without messing with your code of course unless they do something like this which means they're going to break it anyway
but jokes aside our project will contain a lot of data like text elements for I'm very flexible with time zone
Communications I prioritize client collaboration and data for all of these project cards
testimonials work experience and more so to save us some time under the read me of this project code to copy we'll have
this data index.ts which you can simply copy and paste right here it will contain all of the data for this project
allowing you to very simply come here and modify something like if you have your project cards these are right right
here there we go if you haven't built a 3D solar system but rather something else you can just change the title
description image and it will show up whenever we render it within the code and within a lot of these we'll also be
using some kind of images so to ensure that you have access to all of these images needed to build this project
properly the last final thing we'll get from this read me are the assets so you can click here and download it once to
download it unzip it delete the existing public folder and then simply drag and drop the
new one to the root of our directory and don't press add folder to workspace rather just copy folder which will bring
it right here and you'll have all the assets with that said we can close our index file and back within our grid
you'll notice that now we have a series of different title elements such as I prioritize client collaboration my text
stack with I constantly try to improve and other aspects now to each one of these Bento grid items we can also pass
some more stuff to customize it further and to get to that truly extraordinary Bento grid that you saw in the design
this is the one I'm talking about so here we'll have different Technologies we'll have a Macbook mockup we'll have a
button to copy your email address and even some code blocks so let's pass some additional props and then dive into the
Bento grid and customize it to our needs alongside the title in the description I want to also pass a class name which
will come from item. class name so how this works is if you go to grid items you'll notice that I created some of the
additional class names that will apply to each one of these grid items uh primarily it's giving them different
columns and rows pans so they take different amounts of space and you can see immediately medely this created a
very nice Bento like grid after that we also want to give it an image in case there is one by saying item. image and
you can notice that we're repeating this item do something many times so what we can do instead is destructure those
values out of the grid items by using the destructuring syntax and then saying ID title description class name IMG
we'll also have image class name title class name and also spare image in case there is one and all of this should be
autocorrected for you by typescript because it knows exactly which properties we have and if we try to
destructure something that we don't have immediately it will say test does not exist on this grid item type great so
now instead of saying item do something every time we can remove that item and just pass it
this below image we'll also pass the IMG class name equal to IMG class name and we can also pass a title class name
equal to title class name and finally we can pass the spare image equal to spare image or
IMG now you'll notice that it's complaining about this image and some other properties which we're not
accepting on the bentto grid item so let's go ahead and accept them nicely so we can put them to use we'll need a
class Name ID title description um we don't need this icon we don't need the header what we do need
is the IMG the IMG class name the title class name and the spare IMG and of course we need to Define the types of
these properties so we can basically copy them here and now we can Define the type for each one most or basically all
in this case will simply be a string and all of them will be optional because we don't necessarily need to have them on
every one of these cards and in typescript you indicate that by adding a question mark at the end and this is not
a comma it's a semicolon also we can properly end this thing right here we don't need a double close there we go so
now we have those properties which we can use and also we have some things to remove like the header icon and so much
more so let's get started with properly styling our Bento grid and modifying it to suit our needs right now as you can
see it is broken so first things first let's remove this border red 500 that we added before and let's also add an
additional style property right here this will be a dynamic style you can also do this using the CN property in
this case I'm just going to write it like this and give it a background of RGB 4
729 I found this value to work the best here and I also want to give it a background color which will be a
gradient now we need to get this gradient from somewhere but before we go ahead and get it let's simply remove
this header an icon as right now they're not really doing a lot so so we can just remove them if we save that we should be
able to see some cards but now let's give each one a gradient for that you can head over to cssg
gradient. and you can generate any kind of gradient that you want so you can go to something that matches our theme
something like this and you can even change this blue one to something a bit darker it's completely up to you and
then you'll get the background and the gradient which you can then copy to the clipboard and paste it right here as the
styles of course you'll have to turn these into Strings and remove the semicolon and
turn it into a comma instead also it will be background and background color if we do that you should be able to see
some kind of a background appear and in my case I'll use some of the values I created before that look the best to me
I will also add this to the read me down below so you can copy it and have have the same gradient as I have immediately
within this div right here we want to create a new div that will look something like this div that will have a
class name and here we want to make it a bit Dynamic saying that it's a template string where if the ID is equal to six
then we will apply it a style of flex and justify Center so only for the sixth item
and typically it's going to have an h- full for all other items so that would look like this just a bit different for
the last one and we can also give it an inner div with a class name equal to w-o h- full and
absolute we want to put an image right here so if an image exists then we want to render an IMG tag that will have a
source equal to IMG an ALT tag equal to IMG as well and it will have a class name equal to CN IMG class name so these
are the class names we're passing from props as well as some basic ones like object Das cover and object Das Center
and we do get a warning that using the basic IMG tag could result in some slower speeds but we need to do this to
have a proper and easy deployment later on and we'll still ensure the images are optimized so if you do this you'll
notice that the card one and card six got this nice looking image we can also go down below this div containing the
image just below the inner one and create a new one for the secondary image to this one we can give a class name
equal to let's make it Dynamic and we going to make it absolute right-
zero minus bottom-5 and only if ID is five so only for the fifth one we also want to give
it a w- full as well as opacity of 80 and now within here if there is a spare or secondary image image only then
do we want to render it by saying image and it'll be basically the same as above so let's copy the properties but
instead of source image it will be spare image alt will be spare image and we don't need this CN right here we're just
going to make it like this object cover object Center and to this one we'll also give W full and h- full and if we do
this you'll also notice that this image will appear right here but right now you cannot see the beauty of it because
these cards are not yet fully done so they don't have the necessary Dimensions to properly show it but as we continue
building it it will make more sense finally we can go below this div and create another div but only if we're on
the sixth item so if ID is triple equal to six only then do we want to render and of course we'll use this animation
from exterity UI only then do we want to render an animation and of course we'll use an
animation from exterity UI you can see this is the one we're going for and we're going to use it right here in our
sixth card so let's uh copy the file we don't have to copy this stuff in Tailwind config we already have that but
we do need a source code for the background animation so let's copy it and back within our code we can create a
new UI component called gradient bg. DSX and simply paste it right here and now we can use it within here
so let's call it background gradient animation and within it we can render a self-closing div with the following
class names class name is equal to Absolute z- 50 so it appears on top items Das Center justify Das Center
text- White font Dash bold and this should be enough for us to already see it so if we go back it looks like we'll
have to properly import the lib utils so let me put this to the side and go to our background gradient animation and
import CN from I think it's coming from add slash utils and then forward SL CN there we go
that's the correct one and now if we go back you can see this gradient that we have right here so let's continue with
this div so we can make it look a bit better and more similar to what we want to achieve and this should be justify
Center spelled properly and for now we're going to leave it this big we can definitely come
later on to make it look like an actual card right here and not take full window we'll do that later on as we have we
have to continue styling the other parts of this card so let's scroll below this id6 and create a new
div this div will have a class name equal to
CN where we first render the title class name so this will let us know that we're creating a title within
it and we can also give it some additional properties like group- hover so when we hover over that card
slbo translate X2 so we want to move the text within it by two pixels we want to give it some kind of a
transition as well as a duration of 200 for that transition we'll make it a position of relative and also on medium
devices we'll give it a full height typically Min age of 40 flex and flex coal so the elements appear one
below another padding X of five typically padding of five and on large devices padding of 10
you can see that now the title will appear a bit differently if we save that not a lot happens but now we can render
another div within it within which we'll render the description so now we have the inside
scoop I constantly try to improve and all of that other stuff as well let's style that description by by
giving it a class name equal to and I actually think we're already using this title and description below as you can
see here so we can essentially copy the class names for this description and then delete it from
here there we go it should look something like this font Sans instead of font normal it's going to be extra light
text Dash I found this color to work the best C1 C2 D3 it's a gray color text SM instead of XS we can remove this dark on
medium devices text XS on large devices text- base and Z index of 10 so it appears on top so now
our description looks like this right below the description we can render our title so let's copy this div containing
the title and let's place it right here below the description and if you're Wonder wondering what this group hover
Bento translate is it was already coming from an exterity component so you don't have to worry about that great so now we
have this title as well let's style it a bit font Sans font bold we'll also give it text- LG to make
it larger we don't need to provide it any kind of colors I think that should be done by default we can remove the
margin bottom and margin top and instead we can give it this on large devices text- 3XL to make it very
large Max DW of 96 and Z of 10 so it appears on top of other elements so you can see how this
looks like right now looks great even on mobile even though the Bento grid is designed to be viewed on larger devices
now some of these cards have some images and gradients and some exciting stuff happening but some don't have anything
and the goal is for all of them to have something unique so for this one for the second one for example we want to show
this globe okay and for the third one we want to show the tech stack so now let's start by adding these special elements
for each one of the cards starting with the 3D Globe which you can actually move around right it doesn't just move on its
own you can actually hover over it select it and move it around believe it or not this will be another Eastern UI
component so simply search for GitHub Globe you can see it right here and let's scroll down to the source code
this one globe. TSX copy it and create a new UI component called grid globe. TSX where you'll simply paste that data
you'll see there's some red stuff right here and primarily it wants a couple of imports to three Globe react 3 fiber 3js
and even this globe Json okay so for this globe they actually provided a globe. Json file which you can simply
click here and then copy which will provide some information about the data of this
globe after you do that you'll have to add it to data folder so if we go to data you can create a new globe. Json
and then simply paste this data right here looks like it didn't copy the file for me so let me do it one more time
here oh this is display I need to display the source blob it took some time to load as it's a long one and then
we want to go to the raw file and now we can copy it and paste it here it has a lot of lines this is just geometry and
data to display the globe so that is done but now we need to install additional dependencies as you can see
it's three three Globe react 3 fiber and more so let's copy it and let's simply install all of them here and in a couple
of seconds our Visual Studio code should stop complaining because it will get all of the necessary packages there we go
it's looking good and now we can simply use that Globe right here if we go below id6 and below the title and description
let's indent it properly we can now create another Dynamic part if ID is two then we want to display a grid
Globe looking like this and we'll have to import it properly to where we added it let's see where did I put
it grid Globe components UI oh here it is not sure why didn't allow me to automatically imported let's see export
function Globe okay so if I say Globe will that find it yep Globe coming from grid globe and you can automatically
import it it does say that it's missing some props so let's go into it and it wants a globe config in this case so
let's see if we have that Globe config somewhere well it seems like we only have the type for it and we have to
create it ourselves let's see if they provide information on how to do that Globe config props they do give us
something but they don't give us the entire Globe config oh but if we go to code right here in this globe demo there
is a globe config there so let me see maybe I copy the wrong one or are they really different yeah we copy this
export function Globe which is coming from here and this one doesn't have
it but this one on top code has it so let's copy this one right here and this is the usage of this grid Globe we have
created so what might make more sense is to call this one just a globe so this is the actual ual Globe component and now
we want to create a new file called grid globe. TSX which will be the representation of the globe component
within the grid and here we can paste what we just copied which contains this grid config that they have or Globe
config and now we can import the Globe coming from UI or coming from just do slash globe and now we should have
everything so going back to our Bento grid we want to import the grid Globe looks like it doesn't want to Auto
Import it but we know that it should look something like this import grid Globe coming from SLG grid Globe oh and
looks like it's a named export so here instead of saying grid Globe like default we have to put it in parenthesis
and we have to be specific it's called Globe demo so we can call Globe demo and use it right here now if we do that
and go back you should be able to see with cell soap the globe is interactive okay so this is coming from their
example but we want to go into the globe demo and remove some of the stuff that they have said here about soap we don't
really care about that so in this outer div let's clean it up a bit and say Flex items Dash Center justify Dash Center
absolute minus left D5 top 36 this is just for positioning
it on medium devices top 40 w- full and h- full for full width and height and immediately this looks a
bit closer to what we want to achieve from the second one we can remove the H full as well as medium h40 we don't need
that and then we can remove all of these text divs containing H2 P Tags and More that's going to make it look like this
and here we want to remove this minus bottom 20 since we removed the height from this container we have to add it a
bit of a different height something like h-96 which is going to give it about 400 pixels of height going to make it look
like this and that gives us access to this full globe and now that we can see it we can go back to the Beno grid and
we can fix the styles of our container to make it not jump out of the card which matters a lot for this image at
the top for the gradient but even for this globe right here which right now is floating above the card and we want it
to be within so let's go to our primary container div and let's check the class names row
span one that's good relative we want to give it an overflow of hidden and save and already
this makes it look so much better we can still move across it but now it fits nicely within a card great now the next
thing we want to focus on is the ID3 which will be the text stack list div that will look something like this
react Express typescript or you'll be able to type any of the Technologies you are proficient in so let's say if ID is
triple equal to 3 then we want to render a div which will act as the container so it will start with something like this
and it will have a class name equal to flex gap of one on large devices a bit of a bigger gap of five w- fit absolute
minus R-3 and on large devices minus wr-2 within it we can render the text
stack list by creating a div with a class name name equal to flex Flex Das call as they will appear one below
another gap of three and on large devices a gap of eight right within here we want to
render our left list first so we can say left lists and this left list is simply an array of Technologies which you want
to put on the left list so you can do an array of something like react.js we can also do something like next.js and maybe
something like typescript and now we can map over those by saying that map where we get each individual
item and we can simply return something like a span for each one of these like this so if you scroll down you should be
able to see reactjs and then the others are cut off but they're here we can also give this span a key equal to item and
we can give it a class name that class name will have a padding y of
two but on large devices padding y of four and on large devices padding X of three typically we also want to have a
padding X off three we also are going to give it a text XS like this on large devices text-
Bas opacity -50 on l devices opacity d00 rounded dlg text- Center and a BG of hash
10132 e so even if you go back to full screen right now you can see that the first card looks quite good the globe
card looks great as well but here the Technologies are still out of the picture so I noticed that I accidentally
closed this div too soon this div right here is not just for the title it's for the whole card so let's copy this ending
tag below the div title and let's move it over all the way until the end that way we're properly closing all
the divs and now our cards will appear properly and you'll be able to see the teex on the left side great with that
said we can go below this left side mapping and we can create just a single self-closing span this one will have a
class name equal to this one will have a padding y of four and padding X of three rounded dlg text- Center and a BG off
hh10 132e if we save it you should be able to see a new container appear and now we're
going to go below this div right here and ENT duplicate this entire div containing this list so let's copy it
and paste it below but this time we can use different Technologies so let's say something like
this is going to depend on you of course but maybe we can do vuejs if that's what you're into we can also do something
like AWS or maybe mongod DB if we save it you can see those appear right
here but it's not as close to what our final design looks like you can see we have this empty span here and on the
right side the empty span is on top to create this Dynamic looking effect so going back here I'm going to take this
span on the right side which before was on the bottom and I'm going to put it right here above the map so now you can
see how it moved it to the right side great now also I noticed we can make some additional fixes to this primary
class name such as removing this P4 we don't need it here as our card already have enough padding we can
remove this dark BG black and dark borders and BG white all of this is already accounted for by our theme we
also don't need the border border transparent and we can keep justify between Flex Flex call and space y4 but
we can add a tiny border by saying border and then order- white SL 0.1 so this will provide a very
very lightish border just so we can see it here and now these cards look even more uniform we have this great looking
layout and the first three cards are more or less done this is looking great now let's continue working on the rest
if I scroll down to where we close this ID3 we're going to keep creating some additional stuff for the one with an ID
of six so if ID is six and this is the one with the gradient if I remember correct where
we'll allow people to copy our email so let me just show you how that looks like do you want to start a project together
copy my email email is copied and then even some confetti on desktop so here we can create a div with a class name equal
to margin top of five and relative we want to create an additional div within it with a class name equal to it will be
dynamic absolute minus bottom-5 right- 0 and let's fix this bottom of five right within it we want
to render a l animation and we can do that by installing a package called react Das l l provides a
lot of react icons and animations we can use and we also need its types so we need to say mpm install --
save-dev at types SL react DL this will make it know exactly what we need to import and now here we can render L like
this and give it options equal to an object of loop now it's going to Loop only when we copy it
so we need to have access to the state of copy so why don't we create a state
right here in the Bento grid item I will create a new use State snippet call it copied and set copied at the start set
to false and we of course need to import Ed state from react now let's also properly close it right here just so
it's not complaining and we're going to say Loop only when we copy it so when copied then Loop autoplay when copied
once again so Auto playay animation data will be equal to animation data and this will be another Json object which you
can add to your data folder so let's go to data and create a new confetti. Json and you can find this
Json file within the read me of this project simply paste it here and it's going to look something like this it has
all the confetti data so now we can import it right at the Top by saying import animation data coming
from add slash data SLC confetti. Json and we can now use this animation data right here at the
bottom great finally there is one property which we need to provide that's going to make it look better and that is
renderer settings which is an object where we can add a special property of preserve
aspect ratio and we can say x mid y mid and then slice I found this to work the best
for this sort of Animation we have to import L from react L of course and now if we go back we'll also
have to make this a use client component because we have used a use state so here we can say use client and this will make
it work now we can scroll down go below this div wrapping the animation because how will we trigger animation if we
don't have a button to trigger it so let's render a magic button which we have already
created before and to it we can pass some props such as a title which if we have copied it'll be something like
email copied else it's going to say copy my email icon will be IO copy outline
coming from react icons Position will be set to left and of course this IO copy outline has to actually be a
self-closing component we can pass some other classes to make it look better such as important BG so we're
overwriting the BG right here 161 a31 and we can give it a handle click equal to handle copy so this is a
function which we can create to manage the copying of the email so we can add it at the top by saying const handle
copy is equal to and here you can Define your email by saying
Navigator do clipboard. WR text and here you can paste your email contact JS mastery.
proo for me for you it might be something else and then we want to set copied to true
there we go so now if you scroll down you can see this do you want to start a project together copy my email but it's
not doing anything at the moment and also it's not appearing within a gradient which is what we want so let's
go into the magic button and let's style it a bit further specifically we're going to give it a
handle click right here equal to handle click which we're passing from props or rather it's going to be an onclick to
this element so now if we reload the page you can see that if you click copy my email you will get the confetti
animation and it will say email copied now the last thing we have to figure out is how to put it within this gradient so
let's do that next we can scroll up to where we specified a ID of six first and where we're using this background graded
animation we can dive into it and then scroll down to this outside wrapper div which is this one here instead of H
screen we're going to make it h full and instead of w screen we'll make it w full and this will make it take the full
width and height of the container instead of the screen also instead of relative we can make it absolute which
will actually show the elements within it now we can go back to the Beno grid and we can check out if we're properly
aligning it in the middle because right now it seems to be aligned to the left side so if if we check this out oh looks
like I have a mistake here just if I Center this should be within this curly brace like this so that way we have the
ID is six and flex and justify Center so we have to properly close it here and then typically H full and this will
Center it in the middle so now this is looking great and we can actually copy it let's reload and we do get the
animation again great so now if we expand this into full screen we can see all of these sections in their full
Glory we have I prioritize Clan collaboration fostering op and communication and if you want to change
any of these text elements you can do so very easily just copy it search it remove an empty space at the end to find
it properly and you should be able to find it within the data object where you can completely change any kind of text
you want then I constantly try to improve my tech stack very flexible Tech Enthusiast with a passion for
development inside scoop building an animation library and then we have a copy email right here this is looking
amazing so with that we have our hero section and we also have our benog grid telling people what we do the next
section on our list will be what our portfolio is all about which is displaying the recent projects we worked
on using this very engaging card that folds back and allows you to visit the live website that you're seeing on the
screen here I very much like this effect so let's go ahead and implement it together I'll collapse this right here
go back to the current version of the project exit all of our currently open files oh and I just noticed we don't
need this div right here which we were adding before so you can simply comment it out within the background
animation exit the files and just create a new section the next section will be called the
recent projects so under components create a new recent projects. TSX run RFC import it right here as recent
projects imported and you should be able to see a text that says recent projects which means that we are ready to start
developing it great work so far to get started started with our recent projects we can remove this text and give this a
class name of padding y of 20 to divide it a bit from top and bottom immediately after we can create our first H1 that
will have a class name equal to heading and right within it we can say a small selection of and then we can provide a
space like this and then create a span that will say resent projects now if we save it you can see
it like this but we can also give this pan a class name equal to text- purple just to provide some visual interest
right below this H1 we can create a div inside of which we'll show our project cards so let's give it a class name
equal to flex flex-wrap items Das Center justify Das Center padding of four gap of 16 between the Cards and margin top
of 10 to divide it from the title and right within here we can map over our projects by saying projects importing it
from at SL theta. map where we get each individual project and we can automatically return
something for each project such as a div within which we can return a project. tile
let's save it and you can see the titles for four of my projects now let's dive into the projects by command clicking it
to see what each project has it's the ID title desk for description IMG icons and a link and now I would invite you to go
ah to go ahead and change titles descriptions and images for your own projects to truly make this your own
portfolio or we can keep building it out together and then you can modify it at the end with that in mind let's
destructure all of these properties from the projects such as the ID title description image icon list and Link we
can copy that and then we can automatically destructure them right here so we don't have to say projects
every time I'm going to add commas at the end of each one of these like this and I will put them in the same line so
that will look something like this we get the ID title desk image icon list and a link and we close it and then
present a div with a key of ID for each one and we no longer have to say project. tile or project. ID now it is
just title great so now let's give this div a card-like appearance by giving it a class name equal to on large devices
m-h of 32.5 RM I found this prec prise value to work the best we can also give it a typical height of 25 REM
Flex items Dash Center so we Center them justify Dash Center so we Center them horizontally on small devices W of 96
and typically W of 90 VW so 90% of the screen and now we have space for all of these projects and right within here we
want to render another erity UI component this time it will be the 3D pin why a pin because it will pin on
this card like this and you'll be able to click it to go to the desired website this card or this design is absolutely
perfect for portfolio projects because it basically screams okay here I have some data and I can enter into that
website to learn a bit more about it okay it even points to the L of where it will take you a very engaging
design so let's copy The Code by clicking here and let's create a new PIN container right here under UI pin
container. TSX inside of which we can paste what we just copied and it looks like it's using some kind of a pin
container coming from UI 3D pin so let's see what that is about oh it says we need to copy this component UI 3D pin so
so let's do that by adding a new component to the UI section called 3D D pin. TSX where we can paste this and now
if we go back we can just import it from sl3d pin and if we go back into projects we should be able to use it by simply
wrapping everything in here we can just say pin container coming from UI 3D pin and we can wrap it like this and in here
let's render the title for now if I do that and scroll down you'll be able to see that it kind of immediately works
but each one of these is in its own kind of space we just want to have a simple card right here so let's enter this spin
container and let's scroll down a bit here we here we want to remove this BG black as we already have a background we
want to continue Down and Under pin perspective we want to change w96 to W full and then I just noticed we don't
even need this first pin container we have created as this is just an example of how you can use it so you kind of
wrap it with something and then you put some stuff within it so for now I will delete this pin container and we'll use
just the 3D pin so now within this pin container let's provide more content let's first give it a title and this is
where it will point to so I think for now let's make it a title of our project and let's let's also give it an href and
this hre will be where it will point to so that will be the link of your project okay let's see if it accepts that title
href title href it does seem like it accepts both so why is it complaining oh property children is missing of course
so let's add the children I'll create a div right within it within that div we'll create another div and that will
be the wrapper for the image so here we can render the image with a source SLB g.png with an Al tag of BG IMG so if I
save this so if I save this you cannot see anything yet but let's also add another image right below this div and
this one will be the image of the project so source and it will be equal to
IMG with an Al tag of cover or it can also be the L tag the actual title of the project and a class name of
Z10 absolute and bottom zero great let's save it and even if you're reload there appears to be nothing there let's see
why that is if I go into pin container it wants children right here and where is it using that children it seems like
it's trying to render it here so why is it not showing up where we need it let's look into that soon just after we add
the classes to this div within the pin container we can give it a class name equal to relative
Flex items Das Center justifi Das Center on small devices w- 96 typically W of 80
VW overflow Dash hidden so there's no scroll h of 20 VH for the height of the card and there we go so it was actually
missing the height to be able to see the content inside of it and now we have four of these great project images of
course you'll be able to use your own later on we can also give it on large devices a bit more height like h-30 VH
and margin bottom of 10 now within this inner div we can give it a class name equal to
relative w-o h-o overflow D hidden and enlarge
devices rounded -3 XL and then we can give it a BG G of # 131 62d so now it's going to look like a card it's hard to
notice it now but we'll be able to notice it later finally let's add a title right below this div containing
the images it will be an H1 that will say title as simple as that now let's style it as a title by giving it a class
name equal to font Das bold on large devices text- 2XL on medium devices text- xcl typically text- Bas and line
clamp one so it means that it will not go into two lines even if it needs to expand below that age1 we can render a P
tag that will render a des a description and we can give it a class name equal to on large devices text Excel let's fix
this class name first on large devices font dormal font Das light text- smm
typically and line clamp of two so you can extend to two lines there we go so this is now looking very nice and
uniform and finally let's create the bottom part of this card which should look something like this where we have
these Technologies and also check the live side button we can do that by creating a new div below this P tag that
will have a class name equal to flex items Das Center justify Dash between because we want to have content on both
left and the right side margin top of seven and margin bottom of three within it another div with a class name equal
to flex and items Das Center and finally within here we can map over our icons or
Technologies for that project so we can say something like icon lists which we destructuring
from each one of these projects map or we get an icon and then for each icon we return a
div that'll have a key equal to Icon because each one is unique a class name equal to border border Das white over in
square brackets 0.2 and right within it we can display an image IMG that will have a source equal to
icon with an Al tag of Icon and a class name of [Music]
p-2 so if now we saved this you can see all of these Technologies typically these should be circles right so let's
give it a rounded Das full property that's good BG Das black on large devices W of 10 for
the width on large devices h of 10 and in smaller deves W8 and H of 8 and this should be enough for all of them to
become circles we can also give it a flex justify Center and items Center which will
Center it vertically and horizontally within those circles let's also give it a special style property which will
say dynamically translate x minus 5
* index time 2 and then outside of that PX and then we get the index right here
so this will move each one by some multiple of the index let's properly spell index here and let's save it it
looks like I didn't properly close it so inside of style I am missing a transform property that we then give that we then
assign to translate and if I save this if we reload they're appearing one on top of another
which gives for a very cool visual effect like they're all together within this project very cool finally right
outside of this div containing all the icon lists we can create another div which will say check out live site so
let's create a p immediately check live site and below it we can render a fa location Arrow
import it and give it a class name of Ms for margin in line start of three and we can give it a color of hash
CBA cf9 that's going to look something like this check Liv site we're using the same
purplish color we're using right here and now we can style this div with a class name of
flex justify Das Center and and items Das Center there we go finally let's style the P tag by giving it a class
name off flex on large devices text- XL on medium devices text- Xs and typically text- smm
and also text- purple for the color this will make it a bit smaller and it will look good now you can notice that when I
hover over the project there seems to be quite a long title right here appearing so instead of the title maybe we show
the URL right here in the pin container so the link can also be the title which kind of makes more sense in this use
case because it tells you where we're going after we click on this card which is pretty cool I think that's how it
should be used and believe it or not with that we're done with what you could argue is the most important section of
our portfolio the project section so if I open it up on full screen this is how it looks like and this is how it looks
like on the final design it is a big difference right here the projects are much
larger and here they're kind of more neatly packed together so let's quickly fix that here we have this div which is
containing everything and within it we have the div containing only the projects so to fix it let's first look
into this outer div below the H1 this one one here it's wrapping everything it has the padding but we can just give it
a bit of a different horizontal Gap rather than a vertical Gap so Gap X of 24 we can have more space but Gap y of
just eight so a bit lower like this this will change the Gap Dynamics and now we can also modify this div within the
projects by giving it a small property of H of 41 REM and typically h of 32 REM so we can overwrite our previous 25 to
give it a bit more space Also on small devices we can give it a width of 570 pixels so a bit more than before and
this will make it more spread out across the screen but of course now we have to fill
that space somehow so we can also modify this card within the Pin C container by giving it on small devices a width of
570 pixels this will make them much bigger and much more powerful typically with of
0v VW on small devices we can give it a height of 40 VH and typically a height of 30 VH on large devices we can remove
this one right here and keep it margin bottom of 10 great so now they're looking much more
similar as they do on the final website so now that we have a couple of sections ready and this is maybe the most
important one uh we can also implement the floating Navar or at least the links so we can actually scroll down to the
project section to do that we can go back to the original homepage and you can see the structure for our links the
only thing we have to do now is remove this array and use the constants nav items coming from at
fordata this one includes links to about projects testimonials and contact so let's save it and as soon as you
do if you scroll down and then up you should be able to see different parts we can remove the login at the End by
heading into the floating nav and then scrolling down and removing this login
button there we go and we'll also have to make some small modifications as now it looks a bit narrow so let's go to the
top of the jsx and to the class names let's change rounded Das full to rounded LG let's remove this dark border as well
as the dark background and BG white we don't need that and we can change padding right to padding
X of 10 we can also remove these and add add a padding y of five so now it has a bit
more space but it doesn't have any background or a border so let's add a border
white over 0.2 inside of square brackets this will give it a nice tiny border and we can
remove this border transparent as well let's give it BG of something like black 100 okay that's good and if you want to
you can make this rounded full it's it's really a personal preference depending on whe whether you want to have a pill
like shape or a rectangular like shape I think this is good now let's see if all our links are pointing to right things
if I click about it points to about which is great if I click projects it doesn't point to
projects so let's go back to the recent projects and let's give this div an ID equal to
projects if we do that and now scroll to projects you can see that it works great and this is a pretty cool nav bar
because it only shows when you actually need it like if you're scrolling down means you want to see more but if you go
back up it means you want to see something specific so we're going to make it work for testimonials and
contact later on as well great with that said we have now completed the most important sections of your portfolio the
dynamic homepage that has animations the about section which is incredibly unique I mean I haven't seen something like
this in a long time and also a small selection of your recent projects where people can click them and check out
their livite and with this many features completed on your developer portfolio it's important to ensure a smooth user
experience optimized performance and just in general showcasing that you're using all the best practices of
developing applications for that reason we'll use Sentry an Enterprise level application monitoring software
that allows you to fix your portfolio very quickly if it breaks and specifically in this case will also
enable your users to share when they experience something unexpected with the site we'll integrate this model that
will allow them to provide you direct feedback and this matters a lot because you're showing to whoever is looking at
your portfolio that you know how to implement best practices to your apps so let's get started with integrating
Sentry click the link in the description so you can follow along and see exactly what I'm seeing and then either sign in
or get started sign up using your preferred method and if you've used it before you should be able to see the
issues happening on your other projects so you can fix it and check out the projects you've done but if you haven't
you should be able to see the onboarding and there's a trick to get the onboarding even if you've used sentury
before just copy your current URL and instead of projects say onboarding that'll show you this welcome to Sentry
integration guide click install Sentry choose your framework in this case nextjs and configure the
SDK we can follow the commands and install the Sentry wizard by pasting this command in the terminal it will ask
us whether we want to proceed to which we can say yes and now it's going to ask us a series of questions this wizard
will create an update files okay that's good to know so let's continue we'll use the Sentry SAS
version we do have a Sentry account it'll try to automatically log Us in we can select our Sentry
project and it will install all the necessary dependencies this is a pretty cool
feature that allows you to avoid add blockers if you want to use it that's okay but for now we're just going to
press no and proceed with the default option we'll use yes for this one yes for cicd
tools and then you'll be given the century o token which you need to copy and let's immediately store it somewhere
for later on for example in the env. looc file and we can paste it right here senty o token and then we can continue
and the project has been successfully set up that means that Sentry has set up everything for us
everything from their client configuration guides to this instrumentation file and even a global
error example which allows us to trigger an error to see if everything works so now let's click the next button right
here and it looks like I connected this Central instance to one of my previous projects but that's okay I don't mind it
in your case most likely you have a new clean project right here as a matter of fact it's even better that I have old of
these previous issues events and all the data right here loaded as typically it can take about 15 minutes for all the
data to show up so for you it might take some time so right now I can guide you through everything that I already have
here and then you can get back to this page later on and see it for yourself as well one thing we need to do is we need
to go to this page that was created for US Century example page I think is the URL yes so if you go to your portfolio
and navigate over to Sentry example page you should be able to see a page that looks like this and you can
click throw error and you can see the error was thrown sentury example frontend error
this now means that we should be able to catch that error under our issues and if I reload here it is sentury example
front end error you should be able to see just this one if you have a new clean project
now what Sentry allows you to do is to track the operating system the browser the device and a lot more information
about the error that was thrown such as the entire session replay of what the user was seeing when the error happened
this is incredibly useful because when your users are browsing your portfolio in production it's hard to know what's
going to happen on their side but with this you can very easily see the error and debug it because you're also getting
the complete breadcrumbs of the event and all of the information coming from the console some of the other things we
have here are queries where you can see what your users are doing also requests they're making web vitals which matters
a lot for a portfolio so here you can track how much time it takes for the website to load replays that we have
discussed so far where you can see if people are clicking something that is not working or if they're clicking
something many times which is considered a rage click that's not good means that your user experience is not as it should
be and in the dashboard you can also see a lot about what they're doing what kind of experience they're having which Pages
load the slowest people from which countries are visiting your portfolio and much more but what I want to explore
further right now is this user feedback feature which allows you to create bug reports so that users can let you know
about sneaky issues right away and every report will automatically include related replays tags and errors so let's
set it up I believe we have already installed this and we have to add a special integration called century.
feedback integration so copy this and let's search for this century. init within our code so if I go here and
search for century. init you can see it in three places client Edge and
server in this case they give us an alert saying that it must be added to client config so let's go to the
client and I believe we can just add Integrations if we don't already have any oh we do have a replay integration
so now we can just add a feedback integration and the color scheme can be dark now if we go back to our portfolio
and go to Local Host 3000 would you look at that there is a report a bug button which matches her
Coler scheme very well where people can leave their name email description of the bug for example
let's see if we can find a bug on our current website I mean this is technically not a
bug as somebody only needs to copy your email once but let's say they copy it and they forgot they copied it and they
want to click this again no more confetti again right again not a bug but you can Implement a refresh after every
couple of seconds so let's say this user doesn't like that and we can say email copy button doesn't work and we're going
to leave many exclamation marks like an angry user we can also add a screenshot but I believe it's not
necessary let's see how that works oh very cool so I can just select it from my screen immediately and it will Target
this part of the page okay and we can set send a bug report oh that's it thank you for your
report let's see how much time it takes for us to get the user feedback I reload and here it is email copy button
doesn't work let's click it you can see the screen they were seeing when they gave that their URL and also the entire
replay of course the numbers and letters are hidden here for privacy but you can see exactly what the user was seeing on
the screen when the happened as well as their browser name extension version and more I mean this
is amazing typically you would have to go back and forth with the user asking them what their browser was what their
operating system was and more and here you just get this error in this case I'm not going to mark it as spam but I will
mark it as resolve as as I said it's not really a bug but with that said Sentry is amazing I reloaded the page I can
move up and down and Sentry is tracking some of the events right here that's a pretty cool thing so if you want to
track how many people clicked show my work or clicked contact you can do that as well all of the events will be shown
under replays and requests but with that said let's continue building out our phenomenal portfolio next on our list
kind words from satisfied clients to start developing it I'll scroll down navigate over to the homepage and create
a new section under components called clients. TSX where we can run our afce and then we can simply use that
component right here clients and we can import it go into
it and start developing it our client section will start in the same way that our project section started so we can
quickly go to recent projects and just copy the first div H1 and even the opening tag of the div below and then
paste it right here and properly close all the divs that is one and I believe we need one more there we go so now
let's indent it properly and let's save it instead of saying a small selection of recent
projects we can say something like kind words from and then we can say something like
satisfied clients and also we can add a space right here I believe there we go that's good right below we have Flex we
don't have to wrap it here because we don't have any elements within it but we will make it Flex Das CO as we want to
show elements below items Das Center and then we don't need anything else in this case and within this div we can start
showing those cards of course these are the ones I'm talking about and if you can guess it correctly they're coming
from eity so let's go here and let's search for infinite moving cards this is what we need and we can copy their code
base infinite moving cards so copy or let's see it right here yeah this is the card so we can create a new component
under UI called infinite moving cards. TSX and paste it right here at the top we need to fix this to
slash utils slcn and now we can use it right here within this div by creating a new
infinite moving cards close it it is a self-closing component and it accepts a couple of props such as items which need
a quote a name and a title a direction and speed and some optional parameters so let's say items is equal to
testimonials coming from data direction is equal to right and speed is equal to
slow let's save it and if we go back right off the bat you can see some cards moving right here this is the default
styling coming from aity UI we can wrap it within an additional div right here so let's put it inside and let's give it
a couple more properties such as a class name equal to h- 50 VH like this on medium devices
h-30 REM rounded Das MD that'll make it look like this Flex
Flex Das coal so they appear one below another anti- alast this will help with the way that they move items Das Center
relative and overflow Das hidden and now with that we can move into the
infinite moving cards and we can style them to our liking so let's go to the jsx part of things right here and let's
scroll down to this UL right here and change the Gap to 16 to give it a bit more
space then we can go into each individual list item and let's change the medium width from 450 to about 60
VW so 60 vertical height this will give it a bit more space Also we can change the general width to about 90 VW much
more than before we can also make this border slate 800 so a bit darker and we can modify the padding x 8 to just
padding of five and on medium devices padding of 16 so it's styled a bit differently now as before we can
generate a specific linear gradient okay um and in our case a bit of a darker color might work better so I believe we
have already used this linear gradient someware so if I search for linear gradient Let's see we used it in the
Beno grid yep so we can copy this same one we have used before and we can paste it right here
under background and save and with that background color we also just had a background so let's copy that as well
make a new proper of background and make it this and then this is going to be a background
color if we save it you can see now it kind of matches our design a bit more and by default if you hover over it it
stops that's great and in this case we can change the key to idx because sometimes our names are repeating of
these testimonials okay let's modify the text a bit this span element right here let's
change the color to text White and also text LG to make it a bit larger there we go let's also add a new
div right here above the name above the span of name for the profile photo so that will be a div with a class name
equal to me so this is margin inline end of three with an image so IMG of source is Slash
profile. SVG of course you can make this Dynamic for each of the users and we can give it an ALT tag of profile we can
change the text color to text white one more time and instead of font normal it can be font
bold and also let's make it text XL so extra large finally we can change the text of
the item title to text- wh-200 you can see that now it looks completely different you can see this
profile photo right here you can see the name of the person but it looks like it's jumping out a bit so it looks like
not everything fits on the screen whereas on the finished website this is how it looks like so let's play with
that a bit we can go to the topmost div this one right here and we're going to change the max W7 XL to w- screen which
will make it expand over the entire screen there we go that's much better so now we can see that a lot of this
actually fits but still it looks like this bottom part is jumping out of the card whereas on the finished one it fits
and it has much more width so let's scroll down I think it should be either in the UI or the LI right here the LI
has a w of 90 VW which seems good and a Max W full so yeah this looks good to me but if we scroll down
here we have two separate spans and we want to add them together so I'll create another span which will wrap those two
which is the name and the title and this one will have a class name equal to flex Flex Co and a gap of
one and now that I think about it this pan should have been a div so let's just make it a div not a David just a div and
it looks like you have to reload every time with this testimonial section to see the changes so if I do that now it's
looking better but still not quite there yet it's possible that our text is just a bit too large so right here on item.
quotes span we can give it a text- smm and then on medium devices text- LG I think this will make more sense there we
go and now we have a card which is much more similar if not exactly the same as on our finished website this is great it
looks like we just need some more spacing between this section and the bottom section as
well so if I go back this is looking great and we can go back to our infinite moving cards and I believe we don't even
need this div that's wrapping the infinite moving cards because we already have it within it so we can remove it
here and let's make sure we don't have an extra div save it reload and let's see how it looks
like that's good looks the same as before right here on Max LG devices we can also give it a margin top of
10 that's good and now we can start crafting a section right below it which will be within this same div so
let's do it right here a div within which we want to wrap over our companies so we can do that by
saying companies do Ma app and make sure to import it from the data object where we
get each individual company with its ID image name as well as name image and I think that's it right within here we can
create a div and it looks like I didn't properly open or close this function block so let's do that right
away and let's give this div a key equal to ID and a class name of flex on medium devices Max
dw- 60 typically Max DW of 32 and a gap of two between the
elements and this section you would use if you have worked with some companies before so we can add an
image and add a source equal to IMG with an ALT equal to name and a class name equal to on medium
devices W of 10 otherwise W5 and if we save that you should be
able to see some company logos appear but we can also duplicate that image and show their whole name IMG so companies
typically have a shorter logo and then a larger name of that company and we can make it a bit larger such as on medium
devices W of 24 and typically W of 20 so now you can see what I meant and and we can also style this outside div to make
it look better by giving it a class name equal to flex that's going to make them show in a
row Flex Das wrap so they show in multiple rows if necessary items Dash Center to Center them vertically justify
Dash Center to Center them horizontally a gap of four to create some
spacing on medium devices a gap of 16 so even Lar larger and on Max LG margin top of 10 so this means it will show on
smaller devices to divide it from the testimonials and if you haven't worked with any company so far you can leave
this section off or you can maybe put the logos or names of your applications you have developed be creative but as a
last result you can just remove it with that in mind we have concluded our client section so let's check it out
on full screen there we go I think I need to reload for the speed to take effect there we go so it's much slower
and if you want to read it you can just hover over it to stop it and of course here you would put your own testimonials
you've received from other people that have either worked with you or you've worked for that's always a great idea
whenever you're working on a project collect testimonials from people that you work with it gives other people a
sense that you know what you're doing and that people actually liked working with you which matters a lot so yeah now
we have the satisfied client section and we should be able to easily scroll to it well we cannot WR
now but if we give this client section an ID of not projects but rather testimonials and now if we select it
right here you can see how nicely it Scrolls to it this is great the next section on your list is my work
experience with these cards that have a moving light appearing on them and you notice how this section didn't take too
much time to develop that's because we are reusing just one single component and then moving through it same
situation happens right here so let's go ahead and let's get this section developed we can start by going back to
page and creating a new component which we can call something like experience so let's go to components and create new
experience. TSX section where we can run RFC import it and use it right here experience coming from
components get into it and we should be able to see experience at the bottom so let's get started first let's copy the
heading from the client section that includes the div with the H1 and the first opening div as before
we can paste it right here and properly close the two divs there is one and there is two now if we save this instead
of kind words from our clients we can say something like my and then in a span we can say work
experience there we go make sure to include a space right here and then below that we have a div with the rest
of the content so this one doesn't necessarily have to be flexed we can just give it a w full for full width
margin top to divide it a bit from the top and then we'll make it a grid okay on larger devices we will have four
columns so grid call 4 and on mobile we'll have just grid calls one and then a gap of 10 between each
one of these work experiences we're talking about these cards of course so let's map over them I'm going to say
work experience. map where we're going to map over each one each card and for each one
return a button so in a way we can make them clickable if you want to this will temporarily break her application but
that's okay as we want to use an exterity moving border component okay a border that moves around the container
it can be used for buttons or cards so let's copy its usage right here and let's create a new component
called moving borders. TSX and paste it right here you'll notice that it has an export
called moving border and at the top of that it has an export called button so let's go back here and let's import the
button from UI moving borders and we can provide something within that button such as a
div and within the div we can provide the image that will have a source equal to
card. thumbnail an I'll tag of card. thumbnail 2 and a class
name equal to on large devices W of 32 on medium W of 20 and typically a w of 16
so now if we save this and go back scroll all the way down you should be able to see four cards that look like
this they have images within them and you can see this animation happening which looks great since we're mapping
over those buttons we need to give them an ID so let's expand it and let's say key is equal to card. ID you can also
provide some other properties such as the Border radius maybe a bit larger of 1.75 REM let's
see okay not too big of a difference and we can also give it some additional class names by saying class name is
equal to flex D1 text- white border Das neutral -200 and on dark
border of slate 800 which is what we care about you can also play with the durations a bit by making it random by
saying duration is equal to math. floor math. random and then times 10,000 plus 10,000 I found those values
to be very random and they seem to work great for these cards so you can see now it's moving a bit slower and they're
moving at different paces and it just looks a bit more engaging again if you prefer the regular one that's okay but
but we can also make a decision later on once we conclude the look and feel of the card so let's do that by giving this
div a class name equal to flex on large devices Flex Das row otherwise flex-all on large devices items - Center
padding -3 padding y-6 on medium devices padding of five on larg devices padding of 10
and always a gap of two this didn't do anything just yet but as we add more elements within this div it will
position them nicely such as this div we can give it a class name equal to on large devices margin s so that's inline
start all five and within it we can render an H1 within which we can render a card title and below that we can also
render a P tag that will render a card. DEC which stands for description there we go but this looks quite bad right now
so let's style them a bit by giving the H1 a class name of text- start text- XL on medium devices text- 2XL and font Das
bold so this will make it even larger there we go and we can also style the P tag by giving it a class name of
text- start text- wh-100 to change the color a bit margin top of three to divide it from the title
and font D semi bold to not make it as bolded as the title great so now we need to dive into this button and style it
further or it's not going to be a button in our case it will be a card starting with this component class name we can
remove the h16 and w40 and instead we can add on medium
devices call- span das2 this will make it span across two lines on larger devices and immediately as we removed
the w40 now it takes much more width so it fills out the entire space and already looks so much better and that
was the only change we needed to make so you can see how exterity UI is pretty cool in a way that it allows you to not
install the code but just copy and paste it and therefore you can change it still if I had to give feedback to exterity I
would prefer to have a CLI integration like shaten where I can just install this component and it automatically gets
added to my codebase it would make things just a bit easier rather than having to go here and then copy all of
these parts but with that said we're now done with our experience section so let's check it out in the full screen
and it looks amazing this was actually so simple to implement you can see how some of the
starting Parts like this navigation bar or this hero section or especially this Bento grid took us a bit more time but
this project section and the testimonials and even the work experience was super simple to make so
with that in mind the next section that we can work on after the experience is the my Approach which uses these very
interesting cards to show different phases one two and three look at that animation let's Implement that next back
on our page we can create a new component called approach or process whatever you
prefer. TSX run rafc and simply import and use it right here approach
it's going to say Approach at the bottom which means we can get into it and start implementing it for that we'll use a
stni canvas reveal effect a dot background that expands on Hover as seen on Clerk's website that's good so we can
scroll all the way down and here we can get the canvas reveal effect so let's copy it and create a new UI component
called canvas reveal effect. TSX and just baste it
here that is good here we have that canvas reveal effect component and then we can scroll all the
way up and directly within the approach we can copy the code of how we can use this card and the canvas reveal effect
so let's copy it and paste it right here within this div you'll notice we need a few things we
need a card and also a canvas reveal effect the canvas reveal effect we already have coming from this file but
where is the card coming from we copied just the jsx part right here but what we didn't see is that right below it was
the card as well as the icon so it might be better to just copy the entire thing and paste it right here so now we have
everything and we can just just do export default and call it approach as we did before so con approach is equal
to an arrow function that we can export default at the bottom so export default approach
but now we also have a card component right within here let's collapse everything so it's easier to see it icon
exterity icon we have a card and finally we have the approach and we can import this components UI canvas reveal effect
as we have already copied that and it's coming from add for/ components forward slash and I think it was the canvas
let's see canvas reveal effect yes so it's slui SL canvas reveal effect and now we should have everything
to show our cards within this approach so going back to our portfolio and reloading the page
you can see three cards appear right here with some names so let's turn it from this to this where it says my
Approach with different phases first things first we can wrap everything as a section and give it a class name equal
to w-o and padding y of 20 then within it we can create a new H1 where we can give it a class name
equal to heading and it will say my and then a span with a class name equal to text- purple
something like this that can say approach and of course we have to properly close it there we go that was
supposed to be better if we could actually see it but we cannot see it yet I reloaded and it appeared right here
which is good now let's modify the styling on this div in instead of padding y of 20 I'm going to do margin y
of 20 Flex Flex column unlock devices Flex row this is all good we don't need the
BG wide and dark and so on we just need a gap of four that will provide a bit less gap
between the elements and then we're rendering an exterity card it has a title it has an icon and within it it
renders a canvas reveal effect and that happens three times you could potentially just map over the data and
then show different things or you can just keep it as it is for now I'll just keep it as it is and put everything in a
new line so we can easily see what is happening there we go radial gradient for the cued fade okay and then this is
the last card also has a title icon and a card great so now we can modify the data and the look and feel of these
cards let's do the data first the title of the first one will be something like planning and
strategy if we save it nothing happens yet but if I hover over it you can see how nice that looks
like the icon will be just a regular icon but we're going to also pass it an order equal to phase one and then we can
go into this exterity icon accept this order as a prop you can call it anything like a title as well and give it a type
of a type string and then we can use it right here below so no longer will this be just an
SVG like it is right now it will be a div that will render a button coming from exterity UI so we can go here and
search for button and you can choose any kind of button from here I like the one with the
Border magic which is this one right here and you can just press copy when you do that you can put the button right
within the div like this but it looks like I got the invert one instead of the magic so let me just go to the magic one
there we go and copy it and paste it yeah that's the one it has a couple
of pans and some more stuff happening so if we go here you can see the Border magic as simple as
that and here we can render the order by order I mean kind of like 1 two three for the first one we're passing
phase one and for others we're not passing anything yet if you want to you can style it further but this looks good
enough for me so let's close it and let's go back up let's pass other phases so order is equal to phase 2
and then here we can give an order equal to phase three there we go next we can give each
card a description and you need to add it to a card not here to the canvas reveal effect so expand it and then say
description is equal to and then you can add it right here now you can get it either from the
deployed website or even better from the final figma design which you can find in the description simply copy it and I
will repeat this process for all of the cards added one description this is phase two and we will also add the
description right here equal to Let's copy it and let's also add the title as well so I will copy the title overwrite
it and one last is remaining card with a title of development and launch and a description right
here description is equal to this one right here this is looking great now by itself this will not read the
description as the card doesn't know what a description is so let's dive into the card and modify it to accept a new
prop of description let's add it description is equal to
a type of a string and right below the title right here we can replicate this H2 change it
to description and we'll have to modify a few
Styles let's make it text- smm instead of text- XL and save it okay this is better let's also give it a style
property with a color of hash E4 ecff and also a text Dash Center class name if we do this and you hover this
will look better we can also Center the title by giving it a text Dash Center property and we can maybe make it even
larger by giving it a text- 3XL property right here instead of Simply Excel the top so now that will
look something like this that's better and and we can change the dimensions of the card a bit by scrolling up all the
way to this primary div here we can say on large devices give it a height of 35 RM we can remove this relative and give
it a rounded -3 XL this will make the cards smaller initially but they'll be big on larger
devices this is good we can also try to Center this phase button by going right here and you can see the icon we'll need
to use absolute positioning to Center this so let's say absolute right here after the translate
y4 let's give it a top Dash 50% left- 50% it has to be inside of square
brackets and now we can also use a translate so Translate Dash x dash and then
50% like this oh we have to do minus 50% right here and also translate Dy Das minus 50%
like this as well and now it is properly centered this is looking great we also don't need this radial gradient for CU
fade as I don't really see too big of a difference yeah I think we can remove this part if you want to you can change
the colors the dot size and more but for now I'm okay with those and with that said our approach phase seems to be done
at least on mobile but let's check out desktop or full screen in this case Yep this is looking great love
it we have this nice animation and you can specify different phases within your project that you're working on if you
want to we could make these just a bit larger so it's easier to see phase one two and three so let's go to our
button yeah that's right here and let's make it a text- 2XL we can add that to the span element
right here text- to XL we can remove the text small and we can also make it bold since we have
increase the size so font Das bold instead of font medium and here we can also give it a bit more padding padding
X of five and Y of two to accommodate for the larger font size there we go this is beautiful the next thing we can
focus on is this minimalistic yet quite effective footer so let's do it next I Collapse my screen and I'll go back to
the homage and create a new section which we can create in the components folder
called footer. TS XX run RFC and import it right here by saying footer coming from
components it will immediately appear at the bottom which means we can get into it
and start developing it first things first we can turn this div into an HTML 5 semantic footer tag and give it a
class name of w full so it extends for the full width of this screen padding top of 20 and padding bottom of 10 and
an ID of contact so we can very quickly scroll to it from the navigation bar that's going to look something like
this right within it we can create another div for an image and this will be the footer grid
so we can say image with a source equal to footer Das grid. SVG with an ALT tag of grid and a class name equal to
w- h- full in opacity of 50 if we save this it might be a bit hard to spot it especially since we haven't yet added
classes to the outside div so let's do that by giving it a class name of w- full
absolute left- Z and minus bottom -72 in a Min AG of 96 like this so if we do this it might
still be hard to spot it and on large devices you should be able to see just a bit of a grid right
here if you want to see it better you can increase the opacity to 100% there we go but this might be a bit
too much so I'll bring it back to 50 and collapse the browser we can go below this div wrapping the image and create
another div that's going to be for our content with a class name equal to flex flex-all and items Das
Center within it we can create a new H1 that will say something like ready to take and then within a
span your outside digital presence to the next level okay let's fix this digital and we should be able to see it
right here we can also make the span with a color of text purple so class name is text- purple and we can give it
a class name equal to heading on large devices Max dw- 45
VW great so mobile it looks great and right below it we can create a P tag that will say reach out to me today
and let's discuss how I can help you achieve your goals so always make it about the other person also it's not
good to use apostrophes so use these characters instead let's see how that looks like and let's style it a bit by
giving it a class name equal to text- white-2 100 so it's not full white on medium devices margin top of 10
otherwise margin y of five and text- Center if we save it that looks much better and we are ready to start
creating the button portion of the page so we can create an anchor tag that will have an href equal to mail 2 and then
put your email here such as contact jm.pro and here you can render that magic button which we have used before
importing it from UI magic button with a title equal to let's get in touch an icon equal to fa location Arrow
like this you need to import it and a position equal to right and that's going to give us this
nice looking button which we have seen before we can go all the way below this div and create one last class div for
our bottom of the footer inside of which we'll have our P tag with a copyright so we can say
copyright and we can try to find that copyright sign so we can say copyright sign there we go we can copy
it and paste it and say something like 2024 and then put your name there we go let's style that a bit further by giving
get a class name equal to on medium devices text- base otherwise text- small on medium devices font Das normal and
typically font Das light as we don't want it to stand out too much let's also style this div outside of the P tag by
giving it a class name of flex margin top of 16 to divide it from the button on medium devices Flex row
typically Flex column and also we want to center it by giving it a justify between so there's a space between and
also we want to give it item stash Center so now there's this copyright in the center but as soon as we add another
div right here it will move to the left side and this div will have a class name equal
to flex items Das Center and on meding devices get gap of three but on bigger devices a gap of
six and within here you can display your social media profiles by saying social media. map where you get each individual
profile and for each profile you can automatically return a div with a key equal to
profile.id and within it you can have an image with a source equal to profile. mg an Al tag of
profile.id a width of 20 and a height of 20 and of course you need to import social media from at slata as those are
our constants so if you do that and scroll down you should be able to see those
three icons but we can improve that further by giving this div a class name of w-10 a h-10 this will give them the
width and the height we can also give it cursor Das pointer so people know they
can click them also Flex justify Dash center items Das Center why because we will put them within circles so we can
give it a backdrop filter a backdrop blur LG saturate 180 this is an interesting one and BG
opacity of 75 and BG black 200 so this will make it look like it's within some kind of circles right here after we give
it a border so we can say rounded - LG border and Border black 300 so just a bit of a difference from what we have
here and now we can see it's within some kind of blurry or glassy rectangles and let just fix this items Center as well
so it looks good there we go that's better and that is our footer of course it looks much better on full screen so
you can see it right here and this button leads you directly to your email client which is great and with that
believe it or not we came to the end of this phenomenal portfolio build congratulations for sticking till
the end and soon enough we'll deploy to the Internet so that everyone can see your work
but first let's fix some bugs I noticed that if I scroll anywhere and if it adds this about section here or if I go to
testimonials whatever and then I try to go back a weird thing happens the top is cut off and then if I go to the bottom
there's too much stuff at the bottom here too much empty space so how can we fix that let's go to our home page and
right here you can see overflow hidden and we can remove that part so if I save this click show my work and scroll up it
seems to be good now let's try going somewhere else like testimonials yep that works as well
let's try maybe contact there is some more space at the bottom still but at least the most important part which is
the top part was fixed so now we can always go here and even if I reload while in the about it Scrolls and I can
still go back and I I think the best solution to this would be just to remove this div
containing the grid image it wasn't that visible anyway so just removing it will immediately fix the problem and make her
footer look great while at the same time fixing all the issues we've had with a hero section now since we remove that
overflow thing we have this weird horizontal scroll happening so let's go ahead and fix that back in the homepage
we're going to add it another type of overflow overflow Das clip if I save
this you'll notice that the Bottom bar disappears but the top bar still is here so that means that we cannot scroll to
the right side anymore we can just scroll up and down and the behavior works exactly as it should great so we
have fixed that the actual navbar does work on desktop devices but what I've noticed on mobile is if I go here and
then choose mobile you can see that for some reason it is completely empty it seems like there's nothing there so
let's fix that too in our floating nav if you scroll down a bit to where we're showing our list items you can see that
now we're hiding the nav item on extra small devices and on small we show them but in our case we don't have the icons
for all items so we want to show the name always so let's just make it text Dash smm and let's also give it a
important cursor Das pointer to make it seem clickable scroll down and then up you can see the full navigation and we
can go down or we can go all the way up but it does seem like our footer is hidden a bit so we might want to give it
some space it's good that we checked it out on mobile too so if we go to the footer we can remove the padding top of
20 and instead add a margin bottom of about 100 pixels which should give us enough space for the full content to
show at least on mobile so if we check this out you can see that now we can see
everything clearly the rest of the page looks amazing and let's go back to
desktop and you'll see that now we have some extra spacing here which kind of is okay but we don't need this much so we
can go back right here and say on medium devices and up we can do something like margin bottom of just five let's say
that's about 20 pixels instead of 100 so there we go this now is much better and our application is bug free um if you do
encounter some bugs you can report them or what I would recommend even more is just adding a new post under the modern
portfolio Discord right here where you can just say what's bothering you and of course make sure to read the guidelines
to be able to ask the question in a proper way with that in mind let's get our portfolio deployed and we're back on
our hostinger dashboard from here we can go to our file manager you can go into the public uncore HTML and there's only
a default PHP file which we can delete this is where our build folder will go so let's go back to our
application stop it from running by pressing contrl C and then clearing it and we can go to our next. config.js and
expand this next config object here we can say output and in this case we'll choose export which will
give us an exported build output in the out directory so let's say output and that's
going to be export great now we can open up the
terminal and say mpm run build immediately you can notice that it's building out everything and it will
return it in an out folder it will take a couple of seconds you don't have to worry about these
messages because on the bottom you can see compiled successfully so if we scroll to the bottom you'll see that we
have a type error and this happens so for that reason there is one thing I like to do for builds and that is to go
to next config and say typescript ignore build errors is set to true it is possible that we had an
occasional cscript warning but this will just skip it and run our build regardless and just before we spin up
our mpm run build once again let's just go right here to our file tree to the app and remove this Sentry example page
as we don't need that this is the one that we use to trigger that example error and another another thing we need
to delete is this Sentry API as we won't be using it so once you delete it open up your terminal and rerun mpm run
build let's give it some time and I'll be right back and there we go pre-rendered as static content so now if
we go right here you can see the out folder which you can rightclick and then click reveal in finder or open in file
explorer if you're in Windows enter the out folder copy everything that is in there and simply drag and
drop it to public HTML this should take less than a minute and with that in mind we can simply go
back to our H panel and go to your new URL in literally seconds your website has been deployed to the internet you
have seen that nice animation happen and don't forget you have https security as you can see there is a proper SSL
certificate added to your domain so let's give it one last look show me your work we have this 3D Globe people can
copy your email see a selection of your projects see what other people have said about you your work experience process
and they can easily send you an email oh and what if they're checking your website out from mobile it also looks
amazing everything fits nicely with one screen the animations are there especially on mobile as you as you put
your finger over a specific project testimonials look good and everything just feels like a native mobile
application phenomenal job on coming to the end of this video not many people do but you did this means that you might be
interested in diving a bit deeper so go to JS mastery. proo and check out our ultimate nextjs course here we dive into
so much much more depth teaching you how to truly use nygs and get from this performance to this we have a lot of
deep Dives where you can see exactly how things work behind the scenes you build and deploy a very complex applications
and we even have some active lessons which allow you not to just follow along with the video but we give you a task an
example and then resources and hints so you can truly build something on your own and learn it and of course the app
you'll build in the course is pretty crazy it is a full stack modern Dev overflow application basically stack
overflow on steroids with AI other people have loved it I'm sure you love it too so check it out with that in mind
take a bit of a break you deserved it congrats on your new portfolio I want to see you make some changes and tag me on
LinkedIn Twitter Instagram wherever you are and show me what you have done and I will share your portfolio with everybody
so they can see your work once again great work and have a wonderful day
Heads up!
This summary and transcript were automatically generated using AI with the Free YouTube Transcript Summary Tool by LunaNotes.
Generate a summary for freeRelated Summaries
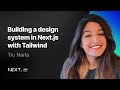
Implementing Your Own Design System in Next.js
Learn how to efficiently create a reusable design system in Next.js using Tailwind CSS and other modern tools.
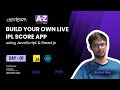
Building a Live Score Application with React and Tailwind CSS: Boot Camp Overview
In this boot camp session, participants will learn to build a live score application using React and Tailwind CSS. The session covers the fundamentals of React, including JSX, components, props, and state management, along with practical coding examples and installation guidance.
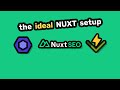
Essential Nuxt Modules for Optimizing Your Next.js Project
Discover the must-have Nuxt modules for linting, testing, SEO optimization, and more to enhance your project.
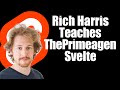
Getting Started with Svelte: The Ultimate Beginner's Guide
Learn the best starting points to get started with Svelte, including tutorials and tips for beginners.
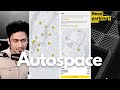
Building the Ultimate Auto Space Parking Application
Learn how to create an advanced parking application with Auto Space using modern technologies.
Most Viewed Summaries
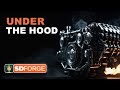
A Comprehensive Guide to Using Stable Diffusion Forge UI
Explore the Stable Diffusion Forge UI, customizable settings, models, and more to enhance your image generation experience.
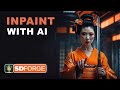
Mastering Inpainting with Stable Diffusion: Fix Mistakes and Enhance Your Images
Learn to fix mistakes and enhance images with Stable Diffusion's inpainting features effectively.
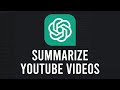
How to Use ChatGPT to Summarize YouTube Videos Efficiently
Learn how to summarize YouTube videos with ChatGPT in just a few simple steps.
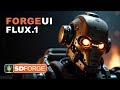
Ultimate Guide to Installing Forge UI and Flowing with Flux Models
Learn how to install Forge UI and explore various Flux models efficiently in this detailed guide.
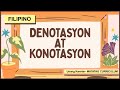
Pag-unawa sa Denotasyon at Konotasyon sa Filipino 4
Alamin ang kahulugan ng denotasyon at konotasyon sa Filipino 4 kasama ang mga halimbawa at pagsasanay.