Introduction
In the ever-evolving landscape of web development, terms like headless UI, boneless UI, and skinless UI have emerged as new ways to discuss component libraries. These abstract concepts, although playful in their naming, reflect serious methodologies about how we construct our user interface (UI) components today. This article delves into these categories, inspired by a captivating discussion featured in a recent article by Adam, and will explore their implications on frontend development.
What is Headless UI?
Headless UI refers to components that do not impose styling, allowing developers to define the visual aspect independently. This approach emphasizes functionality and logic over aesthetics, allowing for personalized design implementations.
- Features of Headless UI:
- Decoupled styling from functionality.
- Provides necessary behavior components without predefined aesthetics.
- Popular libraries include Tailwind's Headless UI, a go-to resource built for Tailwind users.
Example Code:
import { Popover } from '@headlessui/react';
const MyPopover = () => (
<Popover>
<Popover.Button>Open</Popover.Button>
<Popover.Panel>
<p>This is a headless popover!</p>
</Popover.Panel>
</Popover>
);
Exploring Boneless UI
Boneless UI is a newer term that refers specifically to styles that can be applied to headless UI components without the markup—hence, they lack the underlying HTML structure, focusing purely on presentation.
- Characteristics of Boneless UI:
- No built-in HTML or require markup.
- Styles aim to enhance headless components (think Tailwind CSS, Bootstrap).
Practical Use: By applying styles from a boneless library, a developer can spruce up a headless component efficiently. For example, using Tailwind CSS as a boneless layer enhances headless UI components through its extensive class system.
The Concept of Skinless UI
Skinless UI builds on the foundation of headless UI but excludes styling altogether, focusing solely on markup and functionality without any additional skin or UI enhancement.
- Understanding Skinless UI:
- Markup is provided, but without any styling cues.
- Libraries that offer this will enable you to create elements that behave functionally but require detailed styling efforts from the developer.
Example Code:
const SkinlessPopover = () => (
<div>
<button>Open Skinless</button>
<div>This will look default unless styled.</div>
</div>
);
Lifeless UI
A related term is lifeless UI, describing UI abstractions that lack visible rendering but provide logic. It signifies components that work behind the scenes, orchestrating functionalities without presenting elements themselves.
- Aspects of Lifeless UI:
- No UI elements are rendered.
- Often includes libraries focused on state management or logic organization.
Example Usage: Using a lifeless UI approach with a range input helps manage state without the need for traditional UI components:
const lifelessRange = useRange({ initialValue: 0 });
Transitioning Between UI Types
As developers navigate between these varying UI strategies, it’s crucial to understand when to utilize each:
- Use Headless UI when you need deep customization of component behavior without any styling constraints.
- Apply Boneless UI styles to tailor the presentation of headless components succinctly while allowing for dynamic features.
- Opt for Skinless UI when you want to dictate both markup and custom styles altogether, allowing maximum creativity in design.
- Lifeless UI is best for advanced developers looking to maximize their control over intricate interactions without predefined UI components.
Conclusion
The introduction of terms like headless, boneless, skinless, and lifeless UI reflects a significant evolution in how developers conceptualize and implement user interfaces in web development. By understanding these different layers, developers can make informed decisions that enable them to build customized and effective UI libraries. As we continue to experiment with these ideas, it’ll be fascinating to see how the frontend community adapts and embraces them moving forward. So, let’s keep these discussions rolling and innovate together!
hopefully we've all heard about headless UI now you know the idea of UI pieces that don't prescribe UI they just help
you with the interaction part but have you heard of boneless UI what about skinless UI what if I told you these
were all terms that are being invented now as a way to try and talk about how we construct our UI libraries and it
also happens to fit the Halloween theme really well doesn't it this article by Adam is stunning I'm super excited to
dive into this article with y'all about how to build in this weird modern UI world and how to think about component
Library huge shout out to Adam for writing this before we dive in though quick word from today's sponsor today's
sponsor is upload thing if you didn't know this isn't my full-time job I love youtubing and I certainly put 40 hours a
week in but upload thing is where I'm putting most of my actual work time it's the best way to do file uploads
especially for us nextjs developers it's so easy to get set up we actually were running speedruns for a bit to see how
quick people could set up upload thing the record time was under 5 seconds from a brand new project to uploads done
safely and facing users 5 Seconds what I'm so proud of what we built for almost all use cases we already are the best
way to do file uploads and anything that we're not we're working on it by the way I'm not paying myself to put this in
this is here because we didn't have an ad if you would like to buy an ad in a spot like this might be surprised by the
price hit us up YouTube at t3g let's Dive Right In on this I'm excited headless boneless skinless and lifeless
UI UI abstractions continue to evolve year-over-year let's talk about a couple of them what they do and a little about
why and tease them a bit with some silly names I like the call out here that nobody actually says these terms after I
just said all of these terms we'll see where we end up should be fun anyways I love the little animations
Adam's the best he immediately calls out that he won't be covering components with that have heads bodies skins and
Bones like Shakra Shaden radic and mui these are all fully loaded I wouldn't even say radic necessarily is it's it's
it provides a lot of Primitives but fair chat's already saying I'm going to start using these terms please help me make
them stick yeah these are good terms I guess yeah radic has both components and Primitives to be fair these Solutions
are fully loaded which means they have batteries included effectively and they have their own mix of abstractions for
bones skins and life post is more interested in the layered abstractions that the front end Community is using
now so they can compose the layers themselves moving quickly but with higher customizability in order to build
their own Design Systems and fulfill their own unique application logic so here are the terms as defined by Adam
reminder that none of these are are real terms and since nobody's using them yet but I can see them catching on we got
headless which is working components that don't have styles you bring those yourself there's boneless which is just
the Styles so you bring your own life effectively you provide the thing that the Styles attached to as well as the
logic behind it skinless which is just the HTML part you have to bring everything else and bodyless so this is
the state part so like the use selector hook or use input that you would have in something like react it doesn't provide
any of the markup or styling at all it's just the state part so let's start with headless UI pretty common term curious
does this link to headless UI like the library it does cool headless UI is one of the libraries for doing headless uis
that was built by the Tailwind team in order to make it easier to build your own component library with Tailwind
pretty cool stuff but rad is slowly and as Adam says here this is the one term in the post that's actually common
jargon amongst frontend developers there's even a whole library that tries to embody the category that's a good pun
good work yeah headless UI this is what a headless UI offering looks like in code so you see you have the import for
popover pop over button and panel all from headless UI and then you use their components but these don't provide
Styles they are just workflow components effectively so you provide the Styles via class names yourself they're
components that come bundled with functionality they have bones and they have very minimal style headless doesn't
quite match what you get in my opinion since these totally have a brain muscle and structure the mismatch is what led
to the author creating the terms boneless skinless and bodyless here's an incomplete list of headless libraries
which also includes radic Primitives by the way so I wasn't totally wrong calling that out but these are all
things that don't really give you much UI because they're designed to let you provide the UI and The Styling
yourself and we have boneless UI this abstraction layer is all about composable styles styles without markup
skin without Bones on their own boneless libraries don't do much but when applied to Bones you can compose nice you eyes I
I just can't unsee the Bon less which is a skateboard trick where you use one leg to jump it's just all I could think of
whenever I read this hello just show oh rest in peace Ben rers now I didn't see it was Ben he's
one of my all-time favorites he passed away a few years ago oh yeah that's the Boneless it's when
you pop the board into your hand and then use your leg to get most of the the jump that makes me sad I love Ben I
always want to call these rers UI just in tribute love that dude anyways I'll stop thinking about skateboarding and
focus on UI libraries here's an example of a boneless UI doesn't do much but once you apply to Bones you can compose
an ice UI so here's some Tailwind where the Tailwind Library provided skin supplied to some bones so the bones
would be the div and the a tags and then the Tailwind is the the skin on top so the Val wait Tailwind is oh yeah so
boneless yeah so Tailwinds the the skin but there is no bones they don't have components they don't even have HTML
it's just the CSS so boneless UI could also be like UI skin almost think about it it's a part on the outside that has
to be applied to that structure but it's uses out the structure underneath it things like bootstrap Tailwind Bulma
open props all of these Solutions are boneless libraries they don't tell you even how to apply they just give you the
pieces that you use as classes and that's really it then there's skinless UI it's like headless UI but it's
entirely unstyled and it's functioning bones no brains no heart no skin while checking out headless UI libraries if
they're unstyled I would put them in this category instead so a skinless popover UI offering from react area
would just look like this in code all that's a style you're telling it with and height I'm joking this is very
unstyled but you have to take over if you want to actually make it styled like this this won't do anything until you
start applying Styles it'll be like a default div effectively these libraries often order CSS starting places or
helper layouts in positioning but the goal is to let you have full styling control you can even see little bits of
styling in line in the bones hinting or providing meaningful minimal defaults as to The Styling that you'll likely use
like the class names or the flex call here or what I mentioned before with the SVG stuff like those are hints of
styling but you have to apply most of it yourself I'm not super familiar with rqi I've been hearing it more and more yeah
as they say here it's entirely unstyled so the things are just using the browser defaults until you apply Styles yourself
often through things like Tailwind makes a lot of sense there's react ARA which is similar to headless UI or rtic UI but
it's by the crew over at Adobe it's in a really good State overall my team was harassing me so we're going to talk
about area kit too it's one of the these libraries specifically it is providing unstyled primitive components which
means it's skinless right cuz it has the logic part yeah should have mentioned it before but all of chat spamming here
area kits just super high quality headless Best in Class it's great seems phenomenal I yeah it it's something I
have yet to play with but I know Diego decently well phenomenal developer very excited to play with this at some point
I just I I'm so on Shad Cen which does all of the gluing for me it's hard for me to make the move but I could
absolutely see myself playing with this apparently this is what will convince me access to manipulate the state of area
components in a performant way through component stores combo box use combo box store and
I can pass that to different things and control it externally this I could use form store I
can set default values I can call control on it separately and I can dump it on things too
you store State you pass it one of their stores very interesting I can see why you nerds with your crazy UI patterns
and weird search for stuff like Axiom would take a ton of advantage of this interesting yeah all their
components can either be past direct State and do things in controlled ways or you can pass it their custom select
store that does it out of bound very interesting how many times have I done const open set open equals UST State
false with radic Fair Point Fair Fair Point and now we have lifeless UI another category that would usually get
lumped into headless but I feel there's more precise ways to describe a subset of these headless kits like Spirits
Phantoms or ghosts that know stuff but can't actually manifest it into the real world so they're lifeless UI
abstractions tan stack has their lifeless offerings apparently interesting like their types safe hooks
they don't actually render or Supply any UI elements and their focus is maximum inversion of control in mind I I will
say this is Tanner is the one who really caused my brain to light up about this possibility he's one of the first big
like react Library authors that never gave you real components occasionally Tanner would give you things like a a
rapper component that was like a context provider or a thing for like react windows so that it would virtualize
stuff outside of the current view but nothing that was styled ever I also see that we have some tanack contributors in
chat wonder if tanac shock should use the same terminology I'm not opposed either way
I trust y'all to make up the decision because you guys normalize this way of thinking it's on us developers to wire
up these functions to appropriate elements and Styles as if we're inserting a spirit into a body of bones
and skin this is what a tan stack range input lifeless UI offering looks like in code so there's the tanac react Ranger
which is their range element and it's not even an element it's is the capital r Ranger even a
component here oh here it is onchange it's a ranger instance of an HTML div elment so that's
literally just being used for the type definition here and that's it yeah you don't get real components from it you
just call their hook and then you connect it to things using their use Ranger hook to do The Binding to the
HTML and now we have this ref that is pointing to the ranger ref which binds all of the
behavior there's an example set of these with tan stack stuff floating UI I cover floating UI a bunch before as well as
zag JS which I've SE people in chat freaking out about cui components powered by finite State machines very
interesting yeah use machine and then you bind the machine two elements you call like api. getet root props when you
see stuff like this where you're dumping props and values into default HTML elements that's when you know you're
you're stumbling into the lifeless UI stuff a timely for the month in tongue and cheek abstract article about ghostly
abstractions good summary here as Neel said this post doesn't even cover how much JS work that the HTML and CSS are
covering for you like popover anchor dialogue view transition scroll driven animations and custom select so more and
more you don't even need the JS to do those things the HTML and CSS can but having the right abstractions makes
gluing it all together much easier and I like this framing a lot it's increasingly time for me to update my
old video one of my most popular where I compared all of the different modern CSS Solutions it's been 2 years since I
published that Jesus Christ time flies all we' been doing YouTube for a bit over two years that's this pre mustache
Theo God who who ever trusted this smug for opinions I count time in pre and post mustache Theo love it if you
enjoyed this definitely give Adam a follow legendary Devon the space deep on the Chrome team and web standard stuff
one my favorite people to talk about these things with at random events great dude great article definitely worth the
follow and until next time peace nerds
Heads up!
This summary and transcript were automatically generated using AI with the Free YouTube Transcript Summary Tool by LunaNotes.
Generate a summary for freeRelated Summaries
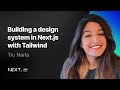
Implementing Your Own Design System in Next.js
Learn how to efficiently create a reusable design system in Next.js using Tailwind CSS and other modern tools.
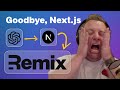
Why OpenAI Migrated from Next.js to Remix: An In-Depth Analysis
Explore the reasons behind OpenAI's shift from Next.js to Remix and how it impacts performance and development.
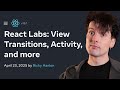
Exciting New Features from React Labs: View Transitions and Activity Components
The React team has unveiled a range of exciting experimental features, including view transitions and a new activity component that enhances UI performance. These updates promise to simplify animations and state management for developers, making React even more powerful and user-friendly.
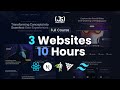
Build a Modern Frontend Developer Portfolio Using Next.js, Tailwind CSS, and Sentry
Learn to create a stunning developer portfolio with animations, responsive layout, and performance tracking.
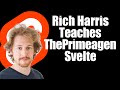
Getting Started with Svelte: The Ultimate Beginner's Guide
Learn the best starting points to get started with Svelte, including tutorials and tips for beginners.
Most Viewed Summaries
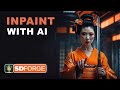
Mastering Inpainting with Stable Diffusion: Fix Mistakes and Enhance Your Images
Learn to fix mistakes and enhance images with Stable Diffusion's inpainting features effectively.
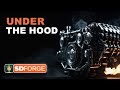
A Comprehensive Guide to Using Stable Diffusion Forge UI
Explore the Stable Diffusion Forge UI, customizable settings, models, and more to enhance your image generation experience.
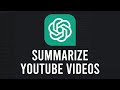
How to Use ChatGPT to Summarize YouTube Videos Efficiently
Learn how to summarize YouTube videos with ChatGPT in just a few simple steps.
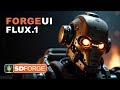
Ultimate Guide to Installing Forge UI and Flowing with Flux Models
Learn how to install Forge UI and explore various Flux models efficiently in this detailed guide.
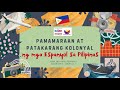
Pamaraan at Patakarang Kolonyal ng mga Espanyol sa Pilipinas
Tuklasin ang mga pamamaraan at patakarang kolonyal ng mga Espanyol sa Pilipinas at ang mga epekto nito sa mga Pilipino.