Introduction
Programming can often become overwhelming, especially with Python where options abound. However, knowing the right functions can not only simplify your code but also save you significant time and effort. In this article, we're diving into 10 Python functions that make life easier for developers. From neatly printing complex data structures to handling tasks efficiently, each function has its own unique advantages. Let’s explore these functions and see how they can be implemented to enhance your coding experience.
1. The Print Function (pprint)
The first function on our list is the print function, more specifically, pprint
from the pprint
module, which stands for "pretty printer". This function provides a way to display complex data structures in a well-formatted way.
How to Use pprint
When working with large JSON files, a typical print statement can result in messy and difficult-to-read output. Here’s how pprint
makes it easier:
import pprint
data = {'name': 'John', 'age': 28, 'children': ['Mary', 'James'], 'address': {'city': 'New York', 'zipcode': '10001'}}
pprint.pprint(data)
This produces:
{'address': {'city': 'New York', 'zipcode': '10001'},
'age': 28,
'children': ['Mary', 'James'],
'name': 'John'}
Benefits
- Easy to read nested data structures.
- Saves time during debugging.
2. Default Dictionary
The defaultdict
is part of the collections
module and offers a valuable feature where a default value is automatically assigned when accessing a non-existent key.
Creating a Default Dictionary
Here’s a brief example:
from collections import defaultdict
word_count = defaultdict(int)
words = ['apple', 'banana', 'apple', 'orange']
for word in words:
word_count[word] += 1
print(word_count)
Benefits
- Eliminates key errors.
- Simplifies counting items in a list.
3. Using Pickle
The pickle
module allows you to serialize and deserialize Python objects easily, making it perfect for saving your complex data structures.
Example of Pickling an Object
import pickle
class Dog:
def __init__(self, name, age):
self.name = name
self.age = age
my_dog = Dog('Max', 10)
with open('dog.pkl', 'wb') as file:
pickle.dump(my_dog, file)
Benefits
- Saves any Python object into a file.
- Highly beneficial for sessions with complex data.
4. The Any Function
The any()
function checks if any item in an iterable (like lists) is True.
Usage Example
numbers = [0, 1, 2, 3]
print(any(numbers)) # Outputs: True
Benefits
- Reduces code complexity.
- Effective for validations across iterables.
5. The All Function
Likewise, the all()
function checks if all items in an iterable are True.
Usage Example
print(all([True, True, False])) # Outputs: False
Benefits
- Allows clear conditional checks.
- Simplifies readability and maintenance of code.
6. The Enumerate Function
The enumerate()
function adds a counter to an iterable and returns it as an enumerate object. This is very handy when you want to keep track of the index of items in a list.
Example of Enumerate
names = ['Alice', 'Bob', 'Charlie']
for index, name in enumerate(names):
print(index, name)
Benefits
- Provides access to both index and element in loops.
- Avoids the need for additional counters.
7. The Counter Function
Counter
from the collections module counts the occurrence of elements in an iterable.
Usage Example
from collections import Counter
fruits = ['apple', 'banana', 'apple']
count = Counter(fruits)
print(count) # Outputs: Counter({'apple': 2, 'banana': 1})
Benefits
- Quickly counts elements without manual iterations.
- Good for frequency analysis of data.
8. The Timeit Function
timeit
can measure the execution time of small bits of Python code.
Example Usage
import timeit
execution_time = timeit.timeit('list(range(10))', number=10000)
print(execution_time)
Benefits
- Helpful for performance testing different code implementations.
- Assists in optimizing code efficiently.
9. Chain Function
The chain()
function from the itertools
module allows you to iterate over multiple iterables as if they were a single iterable.
Example of Using Chain
from itertools import chain
list1 = [1, 2, 3]
list2 = ['a', 'b', 'c']
for item in chain(list1, list2):
print(item)
Benefits
- Efficient for working with many lists or tuples without creating additional memory overhead.
10. Dataclass Decorator
Introduced in Python 3.7, dataclasses
provide a decorator to automatically generate special methods for classes, focusing on handling simple data structures.
Usage Example
from dataclasses import dataclass
@dataclass
class Person:
name: str
age: int
person1 = Person('John', 30)
print(person1) # Outputs: Person(name='John', age=30)
Benefits
- Reduces boilerplate code.
- Automatically implements methods like
__init__
,__repr__
, and__eq__
.
Conclusion
The aforementioned functions are powerful tools designed to enhance productivity and efficiency while coding in Python. Each function comes with unique advantages that simplify complex coding tasks, making them essential in any developer's toolkit. By incorporating these functions into your workflow, you’ll soon notice different aspects of coding becoming more manageable.
Don't forget to share in the comments which functions resonate most with you or if you have any other tips and tricks! Happy coding!
today we'll be looking at 10 python functions that will make your life easier these can save you a ton of time
and a lot of headache and they get more and more interesting as we go through them now let's start with number one
which is the print function now in order to see the value of this let's look at what happens when we try to load in a
large Json file like this a lot of the times we'll have some Json from a request we'll have a bunch of data that
we want to view in the console when we print that using a normal print statement we'll get something very messy
like this this I don't know about you but this is very difficult to read I'd have to go through and try to manually
parse for different fields and there must be a better way in fact there is with the print function now PR print
stands for pretty printer or pretty print and if I simply use PR print. pprint I can specify my data and
optionally the maximum width although I don't need this if I don't want and now when I run my code I'll actually get a
nicely formatted object that allows me to more easily read through in this case on but you can print anything that you
want with the pr print function for example nested lists work really well as well and you can really easily see what
it looks like with nice formatting and indentation in the terminal now the next function on my list is the default
dictionary function this comes from the collections Library which is built into Python Meaning you don't need to install
it and this allows you to create a dictionary object that has a default value what that means is that when you
try to access a key that does not exist rather than getting a key error you're going to get whatever the default value
is which is dictated by the return of whatever the function is that you pass to the Constructor so this is how we
create a new dictionary default dictionary we then pass inside of here a function in this case I'm using the in
function and the int function simply return zero whenever it's called I could instead use the string function so Str
Str I could use something like the list function if I wanted an empty list I could even use another dictionary if I
wanted to do that I can pass my own function as well so just to make this clear what I can do is Define a function
so I can say Define and then we'll just go with default Funk like that and what I can do is just return a value say like
20 if that's what I wanted the value to be and now rather than passing the int I would just pass my default function
notice I'm not calling it and now anytime I try to access a key that does not exist we're going to call this
function get this value and use that as the default value in order to illustrate that to you I'm just going to get rid of
the below example here and I'm going to try to print out the word count at the key0 now currently the key Zer does not
exist inside of my word count dictionary this is just an empty dictionary and you'll see that when I run this I get
the value 20 let's clear this and go back now notice what happens if I were to change this to a normal dictionary so
just initializing it like that when I run the code now we get a key error because zero doesn't exist let's go back
to the other example I had which is a good reason to use this uh default dictionary so let's go back here and you
can see that in this example what we're doing is keeping track of the frequency of different words that we have in this
words list and rather than having to check if a word already exists as a key in the dictionary we can simply in
increment the countr by one every time we process a word the reason for that is that if the word does not exist the
value by default will simply be zero so we don't need to handle that case so just to quickly run this here you can
see that now we have all of the counts of the various strings that we had inside of our words list the next
function on my list is actually a combination of two and it involves using pickle now this is pickle. dump and
pickle. load now a lot of times when you're working in Python you want to directly save a python object this could
be a dictionary this could be a list it could actually be an instance of a class like in this case here now it's very
difficult to do that and usually what you'll have to do is convert that python object into something like Json or into
something like a list some type that can easily be saved in a file you have to save it there and then later when you're
loading it back in you have to parsu that data and then convert it back into the python object that you want now that
is a bit of a pain and fortunately python has a solution for us which is to use pickle pickle allows us to save any
python object in a file so to illustrate that I have this example where I've created an instance of this dog now this
is a custom class that I've ridden myself and what I want to do is save this dog instance so what I'm going to
do is open a new file here that has a pickle extension so pkl I'm also going to open it in the right bytes mode
because we're going to be saving this as bytes not as text what I can do to save this dog object here is I can simply
dump it using pickle so I can say pickle. dump I take my data which is my dog object but it could be any python
type that I want and then I save it to this file now later on I can go ahead and open this file we're just doing this
for example purposes so I can open data. pickle in the read bytes mode that's what RB stands for and then I can load
in this object by doing pickle. load and then loading in the file when I do this is going to give me the exact same dog
instance that I had before and I'll show you that by printing out the loaded data and then calling a method that's only
available on the dog type so let's run the code here and you can see that we get our main dog object and we get my
name is Max and I am a 10-year-old golden retriever so that is how you can save any valid python object using
pickle it's very useful especially when you don't want to be converting say a complex class into something like Json
before you save it now before we get into these next functions I do want to let you know that I do have an entire
software development course where I partner with course careers and a few other instructors that are experts in
their respective field the way that this course works is you go through the software development fundamentals which
is taught by myself and then you actually take a specialization course in front end backend or devops all of this
is included in the price it's a really great value we've already had a lot of success Landing ing students jobs if
you're interested in landing a job in 2024 and Beyond or you just want to level up your software development
skills definitely check it out it is a ton of content tons of practice and we have entire sections dedicated to
helping you fine-tune your resume portfolio and actually how you look for jobs and apply to them successfully this
is really the guidance that a lot of people need in order to break into the industry and even if you're not
interested in purchasing the course we have an entire free introduction course it's about an hour and a half long you
can access by clicking the link in the description anyways with that said let's get into our next function now moving on
the next function on my list is the any function now the any function simply tells you if any of the values in an
iterable object result in true or equivalent to true now if we're looking at the numbers list right here we have 0
1 2 3 4 zero would be a falsy value 1 two and three would all be equivalent to true if they were to be converted to a
Boolean so when I print any on numbers it just looks at all of the numbers and if even just one of the values inside of
this iterable is true then it returns true so in this case we would get true now another way that we can use the any
function is by actually having a comprehension inside of it so what I'm doing here is I'm looping through all of
the numbers in my next numbers list and I'm checking if any of them are greater than zero now this is going to give me
an array inside of here that has true and false for all of the respective values that are inside of numbers and
then we're checking if any of those are true well then this whole thing would return true in this case none of them
are so we would get false next we can do this with strings same thing I can check if any of the strings are empty and if
they are then we can return true notice that this is a much faster way to check for the presence of a value and also
allows you to write some more readable code because you can write expressions in one line whereas normally to do this
to check if any of the values are true or equal to something you need to write an entire for Loop go through set a flag
see if something is true now another example usage here is something like checking for the existence of files so
what I'm doing here is looking if any of these files here exist inside of the current path if they do then I will
return true right so another useful thing you may potentially want to do now this leads me really nicely into the
next example which is the all function the all function works pretty much the exact same way as the any function
except it checks if all of the values are true so if all of them are true we get true if even one of them is false
then we would get false I'm not going to run this code I think it's fairly straightforward but any and all can be
quite useful and can allow you to write some more readable code and to evaluate things in a single line rather than
having to write out these more detailed for Loop or while loop checks moving on to the next function we have enumerate
now enumerate is a simple function that allows you to iterate by both an index as well as a value when you're looking
through any iterable object most commonly used for list but really can be used for anything that's iterable so a
string a tupple some other type type as well now what this will do is give you a topple that contains the index first and
then the value of whatever you're iterating over so when we look at a numerate we can pass to it an any
iterable object in this case I'm passing it a list now we can also optionally pass it a start value but for now let's
look at it without that what this is effectively going to do is generate an iterator that will return these values
so zero Alice one Bob two Charlie which means you can Loop over it by doing four index name and then the index will be
equal to whatever the index is and then the name will be equal to well whatever the value is now optionally you can pass
a start value so if I pass something like start equals 1 if this autocomplete will go away what's going to happen now
is rather than starting at an index of zero you will start an index of one which means now you would get actually
kind of the true positions starting at one rather than starting at zero so obviously you can see why this would be
useful when you need both of those pieces of information and in this case now if I were to run my code you can see
that we get a nice list here where we have one Alice two Bob and then three Charlie there's a lot of other use cases
for enumerate but typically used in the context of a for Loop when you need to access the index as well as the value
when you're iterating over something moving on the next function that I have is counter and this comes from the
collections Library just like one of the previous ones that we looked at now what counter allows us to do is create an
object that counts all of the instances or frequencies of specific words characters objects doesn't really matter
what it is inside of an iterable object so in this case I have a list of strings and maybe I want to count the frequency
of all of these strings or how many times all of these fruits exist now rather than doing that manually using a
dictionary and using a for loop I can simply invoke the counter from the collections module so what I do is say
count is equal to counter and then I pass my iterable object this does not need to be a list and now when I print
out the count I'm going to have the frequency of all of these different objects if I want to accessor the
frequency of a single object then I can use that with a key so I can say count and then something like apple and now
we're going to get the value directly which is three that's about it there's a few other operations you can do with the
counter but this is useful when you very quickly want to get the frequency of all of the different objects that exist
inside of an iterable object the next function on my list is the time it function now this comes from the time in
module which is built into Python and as it suggests it allows us to time how long certain code takes to run now
there's two ways that we can invoke the time it function we can pass a string to it which is what we're doing right here
with some code that we want to execute or we can pass a function which is the way that I'm doing it here now by
default the time it function will run this code 1 million times and then take the average on how long it takes to
execute however as you can see here I can specify the number of times that I'd like to run it myself so in this case
100,000 times if I want to speed up the operation now here we're just going to compare using a list comprehension to
generate squares compared to using the map function and let's see which one actually runs faster so when I execute
this here you can see that the list comprehension took 0.3 seconds and the map function took 0.4 seconds so we'd
want to use the list comprehension if we clear that and decide to run it again you can see in this case actually it
took less time if we wanted to have a more consistent result then we would just run this more times and again if we
removed the number value here by default it will run it 1 million times this is a very fast way to time different
implementations of code so if you want to check for yourself what way you should do something or you have some
code that's maybe perform its dependent then definitely try using the time it module moving on we have the chain
function which comes from the eer tools module now eer tools has a bunch of very useful functions so I'd recommend that
you check it out but chain is one that you'll want to know about now in order to understand the value of chain I want
to talk about what happens when we do something like a list concatenation so if you want to combine two list together
in Python there's a few different ways to do that but a common approach is to Simply add them together when you do
that all the values from list two will be added to the end of list one but what this is going to do is immediately
create a new list for you that has all of those different values that means that all of those values are going to be
stored in memory immediately and you have them there even though you might not necessarily need them at the current
point in time now what the chain function will do is effectively the exact same thing as this list
concatenation but rather than creating a new object in memory and immediately storing all of these new values it's
going to create an iterator that will step over the values from in this case list one and list two now when we use an
iterator the values are not immediately available to us what that means is that we're going to access them when we
actually need them now if you haven't looked at iterators before I understand this can be a little bit confusing but a
lot of objects in Python you'll notice you need to use this list function on if you want to actually view what they are
and print them out in the console you first have to convert them to a list so something like the map object or the
filter object or in this case the chain object and the reason for that is that they're not actually a list or they're
not a tuple they don't have all of the values immediately available instead they just return the values when they're
needed that means that you're not storing all of these values in memory at the time you create this new object
You're simply making an object that allows you to access them when you need them let me show you what I mean by this
so when I run the code here you can see that we get the same thing however if instead of converting this to a list I
just use the chain function here you'll notice that when I print this I get this iter tools. chain object that's telling
me that this is actually an iterator and it doesn't yet have all of the values it's waiting until I actually need them
now in order to verify that for you I'm going to use this function called Next now next will give you the next value
from an iterable object so I can say print and then sorry this is going to be combined chain and when I do this it's
going to give me the first value from the iterator so you can see that we get that we get one and then we get 1 2 3
and then we get the iter tools object because I'm still printing this out so let me stop printing that now I can use
the next function as many times as I want and I'll get the next value in that sequence so I get one 2 and then
eventually if I keep going I would get a b c so I'm not sure if you're understanding where I'm going here but
the point is that rather than giving us all of these at once and store ing them in memory where we might not actually
yet need them it just gives it to us one at a time which means as we're iterating over this with something like a for Loop
we only actually need to have one new value in memory the value that we're currently processing not a combination
of all of the different values now this isn't important in a small example but if you can imagine that list one and
list two had 1 million values each if we concatenated them together we'd immediately be storing 2 million values
in memory rather than doing that and duplicating all these values into a new list we can use something like chain
where it sets up the iterator to Loop through list one and list two without creating any new values it simply gives
us the value from list one or list two depending on where we are in the iteration when we need it to process it
now obviously if you convert this to a list then you kind avoid the point of using chain I just did that so I can
show you what it looks like when we print it out hopefully that makes a bit of sense if you want more on iterators I
have a video here that I will leave on the screen the next function that I have for you is is the data class function
now this is a new function I believe in Python 3.11 but it might be in an earlier version but to be safe let's say
3.11 and this acts as a decorator to decorate a class now what the data class decorator will do is automatically
Implement some methods for you that are common when you're writing a data class a data class is a class that typically
doesn't have a ton of different operations but instead is used to organize or store specific data in this
case we might have a person class where we want to store a name age and job now we could have some functionality on this
class as well but typically what we're going to need for this class is something like an initialization we're
going to need a representation we're going to need maybe a way to check for equivalence between these two objects
now the data class uh decorator pretty much does all of that for us it actually implements all of those methods based on
the different attributes that we Define with their respective types so all I do is say that I want a name that's a
string an age that's an INT and a job that's a string and when we have this data class decorator on top it
automatically implements the initialization so underscore uncore nit underscore uncore the repper soore
repper uncore uncore and the equal method this allows us to utilize this object uh very quickly without having to
Define all of those ourselves now just to show that to you if I run the code here you can see that when we print out
the person we get a nice representation of that we also could check for equivalence between two people so if we
make a new person here we can say person two like that and we can say person uh is equal to person two and in this case
they should be the same in this case they are because they have all the same values but if I change one of the names
to for example something like John when we run this now we get false now just to show you exactly what this actually does
I have an expanded version of this example so if we look at this here this is the equivalent code to what you saw
right here okay so it's the exact same what we're looking at this is the implementation of the initialization
this is the implementation of the repper so we need to kind of write all of this out and do the nice formatting of
printing out these values and this is the implementation of the equal checking is this the same instance do these have
the same values Etc so this just saves us a lot of time and kind of gives us that boiler play code there's a few
options that you can pass to data class but I'm not going to get into that here if you want more information on the data
class decorator I will leave a video on the screen that you can check out so that covers the 10 python functions that
will make your life easier let me know in the comments down below which one was your favorite or if I missed any that
you think are useful with that said I will see you in the next video [Music]
Heads up!
This summary and transcript were automatically generated using AI with the Free YouTube Transcript Summary Tool by LunaNotes.
Generate a summary for freeRelated Summaries
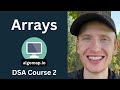
Understanding Static Arrays, Dynamic Arrays, and Strings in Python
Explore the differences between static arrays, dynamic arrays, and strings in Python, their operations and complexities.
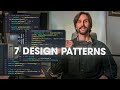
Understanding 7 Essential Software Design Patterns
Learn about 7 critical software design patterns that improve your programming skills. Discover how to effectively implement them!
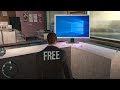
25 Must-Have Free PC Programs for All Gamers
Discover 25 essential free programs that enhance gaming on your PC, from launchers to utilities for modding and system management.
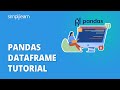
Python Pandas Basics: A Comprehensive Guide for Data Analysis
Learn the essentials of using Pandas for data analysis in Python, including DataFrames, operations, and CSV handling.
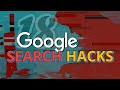
18 Essential Google Search Tips for Developers
This video provides developers with 18 effective tips and tricks to enhance their Google search skills, enabling them to quickly find solutions to coding errors and programming queries. Learn how to use specific search operators, quotation marks, and other techniques to refine your search results and become a more efficient developer.
Most Viewed Summaries
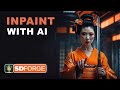
Mastering Inpainting with Stable Diffusion: Fix Mistakes and Enhance Your Images
Learn to fix mistakes and enhance images with Stable Diffusion's inpainting features effectively.
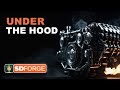
A Comprehensive Guide to Using Stable Diffusion Forge UI
Explore the Stable Diffusion Forge UI, customizable settings, models, and more to enhance your image generation experience.
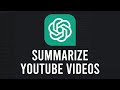
How to Use ChatGPT to Summarize YouTube Videos Efficiently
Learn how to summarize YouTube videos with ChatGPT in just a few simple steps.
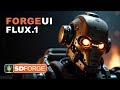
Ultimate Guide to Installing Forge UI and Flowing with Flux Models
Learn how to install Forge UI and explore various Flux models efficiently in this detailed guide.
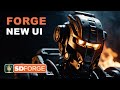
How to Install and Configure Forge: A New Stable Diffusion Web UI
Learn to install and configure the new Forge web UI for Stable Diffusion, with tips on models and settings.