Introduction
Welcome to the world of data analysis with Python's Pandas library! In this comprehensive guide, we will explore the fundamental concepts you need to know to get started with data manipulation and analysis. Whether you're a beginner or looking to sharpen your skills, this article is designed to provide you with a solid foundation in using Pandas for your data projects.
What is Pandas?
Pandas is a powerful data manipulation and analysis library for Python. It offers flexible data structures that make it easy to work with structured data, such as time series, numerical data, and other formats. One of the key components of Pandas is the DataFrame, which allows you to work with two-dimensional data similar to a spreadsheet or SQL table.
Key Features of Pandas
- Data Structures: Pandas provides two main data structures: Series (one-dimensional) and DataFrame (two-dimensional).
- Data Manipulation: You can easily filter, group, and aggregate data using a variety of built-in methods.
- Data Analysis: Pandas offers many functions for analyzing and visualizing data, making insights easier to gather.
Setting Up Pandas
To start using Pandas, you'll need to ensure you have Python and the Pandas library installed on your system.
Installation
You can install Pandas using pip, the Python package manager. Run the following command:
pip install pandas
Creating a DataFrame
One of the first things you'll learn to do with Pandas is to create a DataFrame. Here’s how to create a DataFrame from a dictionary:
import pandas as pd
data = {
'Name': ['Alice', 'Bob', 'Charlie'],
'Age': [25, 30, 35],
'City': ['New York', 'Los Angeles', 'Chicago']
}
df = pd.DataFrame(data)
print(df)
This will output:
Name Age City
0 Alice 25 New York
1 Bob 30 Los Angeles
2 Charlie 35 Chicago
DataFrame Structure
A DataFrame consists of rows and columns:
- Columns are referenced by their name (e.g., 'Age', 'City').
- Rows can be indexed by their integer location.
Reading Data from CSV Files
CSV (Comma-Separated Values) files are a common way to store tabular data. Pandas makes it easy to read data from CSV files using the read_csv()
function.
Example of Reading a CSV File
df = pd.read_csv('data.csv')
print(df)
Writing Data to a CSV File
You can also write a DataFrame to a CSV file using the to_csv()
function:
df.to_csv('output.csv', index=False)
Fundamental DataFrame Operations
Now that we've created and read our DataFrame, let’s dive into some fundamental operations you can perform on it:
Accessing Data
- Selecting Columns: You can select a single column or multiple columns from a DataFrame:
- Single column:
df['Name']
- Multiple columns:
df[['Name', 'Age']]
- Single column:
- Selecting Rows: Use
.loc[]
to access rows by index and.iloc[]
for positional indexing.
Adding and Modifying Columns
You can add new columns to a DataFrame or modify existing ones:
df['Country'] = 'USA'
Filtering Data
Filtering data is crucial in data analysis. You can filter rows based on conditions:
filters = df['Age'] > 28
df_filtered = df[filters]
Grouping Data
Grouping data allows for aggregation and summarization:
grouped = df.groupby('City').mean()
Summary
In this article, we've introduced you to the basics of Pandas for data analysis including creating DataFrames, reading and writing CSV files, and performing fundamental operations. With these skills, you are now equipped to handle and analyze your data effectively. Remember to explore further and practice with real datasets to master Pandas!
हुआ है हेलो हेलो एवरीवन वेलकम टू बना दो वीडियो बाय सिटीजंस इन दिस वीडियो वो ड्वार्फ
टीम्स विल टीच ऊ एवरीथिंग यू नीड टो नो अबाउट बेसिक्स आफ डूइंग सो लेट्स गेट स्टार्टेड है
कि सुवटियो नहीं एक्सपेक्ट फ्रॉम दिस वीडियो बच्चे नेक्स्ट फ़्यू वर्ड्स ई विल आंसर थे क्वेश्चन वाउल्ड स्पेंड हिस डेथ
विल वांट टू डू एंड फाइनली विल एंड विद फंडामेंटल आफ ऑपरेशंस अट डू डू डू
कि कांडा से पाइथन पार्क के बीच फॉर डाटा एनालिसिस विच प्रोवाइड्स फ्लैक्सिबल एंड पॉवरफुल डाटा स्ट्रक्चर्स आफ डाटा स्टोरेज
एंड मेनिफेस्टेशन आईएस यूजफुल फॉर ट्रायल एंड विटामिन ई नो नथिंग बट थे
प्रोग्रामिंग लैंग्वेज में रिलेशनल डाटा स्ट्रक्चर्स इन ऑपरेशंस फॉर मेडिकल टेस्ट सीरीज
आफ विच प्रोवाइड्स ए multi-dimensional 1.5 विद साइंस मॉडल इन थे सिस्टम
डेट एंड एवरी थिंग डिस्ट्रीब्यूशन ऑपरेटिंग सिस्टम सब्सक्राइब टो
में नॉलेज सांसद क्वेश्चन वड़ापाव डेटाफ्रेम्स अपने डेटा प्रेम अ सिमिलर टो एसक्यूएल
टेबल फॉर 10 मिनिट्स ईच अट वर्क विद अश्लील और बैल इन केसेस आफ यस्टर ईयर्स एंड पॉवरफुल
सब्सक्राइब इंटीग्रल पार्ट आफ थिस क्वेश्चन ओं में नॉलेज टेक ए लुक अट थे डिफरेंट टाइप्स
आफ पांडवास डेटाफ्रेम्स हु इज द फर्स्ट वाइफ इन डेटाफ्रेम इज नार्मल डेटाफ्रेम्स साफ व डेटाफ्रेम
कंटेंट हस मल्टीप्ल कॉलम्स इन छह सिंह डाटा इज कोलंबस मल्टीपल रोज रेट 12%
सीरियस सीरियस Channel and subscribe the
Video then subscribe to the people डाटा ऑन
में नॉलेज क्वेश्चन फंडामेंटल डेटाफ्रेम ऑपरेशंस द फर्स्ट फंडामेंटल डेटाफ्रेम ऑपरेशन
दबंगों ने बिलों के गांठ इस हाउ टू क्रिएट अ पंडास डेटाफ्रेम स्टाफ व इंपोर्टेंट पार्ट डांस विद एंड शॉर्ट फॉर्म पीडीएफ
फॉर स्पीडी एनीव्हेयर यू सीरियस द प्रिवेंशन एंड डिटरमिन विच कंटेंस टू कॉलम्स एंड
बिगिंस विद ए स्माइल नंबर टू कॉलम्स एंड ड्रोस 123
456 subscribe The Channel
गिव वन श्रवण वाले स्टूडेंट उस फर्स्ट कोल्लम केरला कॉलम एंड फर्स्ट रोट द लास्ट फोन
9th इंडक्टेड इज द फर्स्ट कॉलम अंदर कॉलम शिफ्ट इस डाटा 0.1 नथिंग एल्स विच नथिंग बट थे टॉप आफ न अंपायर
फाइनली व्हेन यू प्रिंटआउट यह डाटा फ्रॉम विच लेट्स सी हाउ वे कैन कन्वर्ट सीएसवी
फाइल्स फ्रॉम ए हाउ टू रीड इन सीएसवी फाइल्स डेटाफ्रेम विसर्पी रीड रीड सीएसवी फंक्शन एनी पास
लोकेशन ऑफ द ईयर डेट आफ वैरीयस फेसट्स आफ इलेक्ट्रॉनिक्स एंड सी व्हाट आईएस लेटर फ्रेम लुक्स लाइक ए स्पिरिट फ्रॉम सीएसवी
फाइल सुपर डिफरेंट लुक्स लाइक तो सीएफएल ट्यूब स्किन अजीब सी
नॉर्म्स एंड कंटेंस मोर स्टूडेंट्स अलांग विद हेर एस ए नंबर आफ वर्सेज इन वैरीयस
डिफरेंट डाटा स्ट्रक्चर्स कैन अलसो बे थे फर्स्ट टो लीव वन टू थ्री 60
हाउ टू कंवर्ट डे सेंटर डेटाफ्रेम और भी आप टू-डू इज पे डेटाफ्रेम फंक्शन इन थे two-dimensional अरे
नेकलेस अलसो कन्वर्टेड डिक्शनरी अनुसार आंसर इंपॉर्टेंट पीरियड डेटाफ्रेम फंक्शन कल
सुबह जोगिंग सीनियर डिप्टी मैनेजर डिक्शनरी विचार व्यक्त की वैल्यू वन एंड कॉरस्पॉडिंग वैल्यू संशोधन
विधेयक-2012 फाइनल की वाली वाली वाली वाली
वाली सब्सक्राइब टो ओम श्री गणेश क्रिएट ए डेटाफ्रेम विक्रम
सिंह उसे डेटाफ्रेम अर्जेंट डेटाफ्रेम फंक्शन बेसिकली ओनली आफ्टर टू ईयर्स पास्ड इन योर
डाटा एंट्री वर्क वॉल्यूम सुदृढ़ सुबह रघुबीर सिंह गिल कॉल विद डाटा स्टोर्ड विदीन एंड पेंट फॉर 5 6 7 उदयराज वैकेंसी
विनोद सिंह टू डाइमेंशनल पिंप ट्यूब सिंह और डिक्शनरी इन यूजिंग 8051 डाइरैक्टली पास्ड इन थे नेम आफ कॉलम्स एंड
फाइनली Bigg Boss सीरियस सीरियस नथिंग बट ए डिफरेंट स्टोरी विद
डिक्शनरी subscribe and subscribe the Channel को
ब्लुटूथ सुबह अज रोकिन सीधे फास्टनर टू डाइमेंशनल अरे विच कंटेंस डिफरेंट रोल यूज एंड थ्रो आलू समझ सिलेक्टेड थ्रू और
विकिरण पास लिंक वॉल्यूम लेबल्स डिस्ट्रॉय थिस कंटिन्यूज इन थिस अलसो फॉलो उस अट ऑल स्कॉयर्ड स्टार्ट फ्रॉम जीरो एंड गो आफ
वर्सेज आफ कॉलम्स एंड सेंगोल फादर व्हो फॉलो उस ओं कि दास इस आज डिक्शनरी डर के पास है
नंदीश्वर लुक्स लाइक इंटरफेस डेटाफ्रेम सर्जिकल सी द की वायु विहार विल बी द कॉलिंग लेबल्स अंदर करेस्पांडेंट वैल्यूज
विल बी द रोल ऑफ कि Bigg Boss ट्वीट पर सनी लेबल्स हर रोज स्किन ऑटोमेटिकली जिस नंबर ऊसर 1000 थिंग
इज दिस इज़ द डेट आफ वरशिप थिस एंट्री वास पोस्टेड टो
गिव ए गुड द कॉलिंग लेबल इंटरव्यू पास धोकर उसपर कॉलम वैल्यूज एंड फाइनली आप सीरियस सीरियस
सीरियस विद डिफरेंट की गिवर है यह देखिए वाउल्ड यू बे लक्षण अंदर कॉरस्पॉडिंग और आलू ब्रदर्स विल अपीयर इन
द फॉर्म ऑफ सीरीज अव्यय कॉलम इस उंगली दिस व्यक्तियों को रस पानी वैल्यू सनातन रोल इज द रोल ब्राउज़र
अब स्कूल नॉट सी थे हिडेन ऑब्जेक्ट गेम्स
ऑफ बर्थ सर्टिफिकेट व्यक्ति सब्सक्राइब करें
इस पॉइंट को इस पॉइंट को subscribe करे
कि विगत 60 से पूछना थिंकपद रॉ जूस एंड फॉलो उस ओं कि वे कैन अलसो फाइंड द इंडक्टेड फ्रेम
सॉर्ट्स आफ नऊ व्हेन सैटर्न इन डिफरेंट फॉर्म्स टो एल्डर्स इन टावर डेफिनेटली नेक्स्ट
सब्सक्राइब वैल्यू एंड स्टॉप छह लुटेरों शातिर बदमाशों बर्फ रोजगार डिफरेंसेस एंड डिस्टैंसेस व्वे मींस पेट आफ्टर रॉनित रॉय
चेंज चेंज नेम इन कि स्वराज एजेंसी इन डेटाफ्रेम गिव द
कॉलिंग नेम द का इंटरव्यू फर्स्ट कॉलम नेम इज लास्ट नेम विदाउट एनी डबल कोर्स एंड रीमेनिंग कॉलम नेमस फॉर ब्लड ग्लूकोस राम
द वे अरे अफरैद टो कॉल थम व्हेन यू नीड टो कॉल थम ओं प्रिंटर नोटिस में पॉजिटिव हेल्थ प्रॉब्लम्स सुरक्षा विधेयक कॉलम नेम
टुकुर चेंजेस कॉलम नेमस पॉजिटिव इसको आलरेडी फंड अनुसार ट्रिब्यूट आफ ए डेटाफ्रेम एंड इनीशिएलाइज्ड टुडे न्यू
कॉलम नेमस ऊ के स्वास्थ्य कि नशीद ने वह तो कॉलम्स हस बीन
चेंज्ड नॉट हैव एनी मोडिफिकेशंस इफ यू वांट झाल
एनी चेंजेज ठाट यू मेक लव विल इमीडीएटली बे रिफ्लेक्टेड इन द नेम ऑफ द कॉलिंग सफल डेट ऑफ रिसर्च कि फिर नो
है नेक्स्ट यह वे कैन सिलेक्ट रोशन कॉलम्स फ्रॉम ए डेटाफ्रेम इन कि जिस कैन बी डन यूजिंग ए लॉक एंड लॉक
फंक्शन दिलों की फंक्शंस उंधियू सेलेक्ट कॉलम्स एंड स्टाइल आफ फंक्शनिंग व्यस्त सिलेक्ट रोज फ्रॉम ए डेटाफ्रेम
बट लेट्स गेट मोर इनटू डीटेल्स अबाउट हाउ लॉग डायलॉग डिफरेंट यादव ब्लॉक फंक्शन विल वर्क और लेबल्स व्हिच मींस इफेक्टिव इन
लॉग एसेंस गिवर लुफ्त वैल्यू आफ ओसेन इन योर डाटा स्पेसिफिक लुक फॉर द आर्डर ऑफ द ब्रिटिश
लेबर लॉ विल वर्क पोजिशन आफ गिव एंड लुक फॉर इन लाइफ क्यूज़ आफ हिम
बट आप पोजिशन फॉर नॉलेज रणधीर बिट्टू सो यू कैन सी यू इन लॉग इन
अच्छी तरह फर्स्ट अल्फाज़ पोजीशन इट इज उस पर फायर ऑफिसर हैव बीन सिलेक्टेड एंड ड्रोस रिसर्च स्कॉलर मैसेज सेंड
डायरेक्टली फॉलिंग अस्लीप हड्डी यंत्र फंक्शन 10 लेबल्स सो व्हेन यू विल नॉट फंक्शन वह आपके पास सेलिब्रिटी
वाला फंक्शन नो द फंक्शन विल सिलेक्ट और दीवाली उस एंड थ्रो अर्थ और द थिंग
विच बंब एसिड बम और
अरे यार सुबह से क्यों व्हाट इस आपने क्यों चैटिंग ड्राई और रो विश्वास यह इंडेक्स फॉर
इलेक्ट्रिफिकेशन आफ रोड्स एंड कॉलम्स टो डेट ऑफ बिर्थ के नाम रोशन हम अच्छी तरीके से लॉग इन लॉग
इन नॉट मोर डिटेल एंड सॉइल
80 करोड़ इंडेक्सेस वन टू थ्री डू यू डू इसरो विक्रम
सिंह ने Twitter The अब स्कूल लेवल आफ 2014
श्रवण वेन्यूज आफ फ्लॉवर्स कैन फाइंड द वैल्यू उस अर्थ सेकंड पोजीशन और उसे कंटेंट एक्स्ट्रा रोशन कॉलम्स अव्य0
इंटैक्ट व्हिच मींस द फर्स्ट वर्ल्ड वर विल हियर एंड 110 016 151
210 अच्छी तरह इज द फर्स्ट टो एंटर इनटू थे जूसर 89 विकेट लिए लॉग इन लॉग इन
पिछला सॉन्ग अगेन लैपटॉप पैक क्रिएटिंग अंपायर रे विच हस कॉलम्स अमेज़न सी अंदर रोल लेबल्स
1230 लेबल्स वन टू थ्री सोंग्स रॉ टू डाइमेंशनल डाटा यूज करें
और 12345 609 यूज में से एक है
में नॉलेज चीज लव टू कंटिन्यु व्यक्तित्व डेटाफ्रेम
डिफिशिएंट इन जैसलमेर कैंडीडेट्स सिलेक्टेड फॉर एंडॉयड न्यू वाली इस अधिग्रहण से ऑलरेडी ए डेटाफ्रेम और रॉय
इंडेक्स एंड जतिन अब ट्यूशन लॉक वैगनर मेक न्यू रोले मॉडल फॉर एंडॉयड एंड टायर्ड बट डेटाफ्रेम आफ बजे अलार्म क्लॉक ऑन लेबल्स
एंड अदर इज नॉट एलिजिबल फॉर व्हेन विल पावर एंड यूज दिस फंक्शन अलौकिक गुणों रन शुरू ऑयल या फिर और एंड सी द नो रोले विल
फॉर फिजिकल क्रिएट वन बाय इत्सेल्फ विद वैल्यूज 1640
90 से आई लव यू कैन चेंज र वैल्यूज एंड डिफरेंट पोजीशन 100 ब्रिटिश गवर्नर यू कैन चेंज र वैल्यूज एंड लॉक अनलॉक
लेवल दो है तो आओ यार ड्यूटी चेंज सवार लुटेरे बिट्टू 211 1234 सिमिलरली व्हेन यू साय सदस्य फंक्शन 100% डायलॉग इस कोंट्रा
वाउचर डिलीवरी ले और अदल सेकंड एंड क्वाइट ओल्ड यूनिट्स विशेस
0210 वैल्यूज अट लेवल अगर आप चेंज चेंज यूर यूरो और डॉलर टू डू डू डू डू डू डू
एंड वैल्यूज आफ डीआर अंबेडकर अंतरराष्ट्रीय ओलंपिक
समिति ने Bigg Boss 0 ए सौवेणीर प्रिंट दिस अज्जू कंसीव विहार द कि अ अ दूसरे 2016 सुने आज लेकिन सीड तो
फर्स्ट ट कॉलेज अट व्हाट इज द कॉलिंग डी विद्या द इंडेक्स लेबल्स स्टार्टिंग फ्रॉम वन एंड वेट फॉर नेक्स्ट सीईए आफ्टर क्रिएट
आफ्टर एडिंग और लुटा बैटिंग अ कॉलम डिपॉजिट द कॉलिंग अमेज्ड तब आलू जीरो वन टू थ्री
इडिएट रोज-रोज डेट आफ सॉलि़ड स्टेट लॉटरी डियर वनस अगेन ड्यू टो ड्रॉ जूही डिफरेंट स्टूडेंट्स या द नंबर
आफ डिफरेंट लैंग्वेज प्रॉब्लम और रॉ टू इज द फंक्शन ऑफ डू
यू डू द वॉल्यूम और सब्सक्राइब हेलो व्युअर्स वन विच मिंस ठाट विच वेयर
मींस कॉलम्स विंटर्स 087 स्पेसिफिक रोज 100 करोडी ग्रेड कॉलम में डूब स्कूल
पिकअप द फोन है ना वह आप इन थिस यू डोंट नो द नेम ऑफ द कॉलम बट यू नो द पोजिशन अट विच यू वांट टो
ड्रॉप ऑइल नॉट यूज्ड ऑयल फिल्टर डुइज ड्रॉप कोलंबस पेंट पोजिशन टो स्पेसिफाई थे अपोजिशन यूके न्यूज़ तक वॉल्यूम फंक्शन
एंड दिस इज वेरी जैंटल कॉलम नेम सुंधा सिंगल प्वाइंट सिस्टम इन थे यूके डायरी एक्सेस कॉलम वेब डेवलपमेंट रिसर्च मींस
ट्यूशन गए राक्षस पोजीशन रिकॉर्डर पर मंडरा प्रेजेंट पोजिशन इन थे कॉलम व्हिकल्स एक्सेस वाली
1000cc नाट्य शिविर रोशन कॉलम0 एंड 110 012 सी व्हाट इज द वर्स्ट पोजीशन
व्वे रो रो रो रो रो रोल विचार इंडक्टिव
वित्त श्री भैरव भैरव न्यूज़ विड्रा पिंग जरूर इन थे प्रेजेंट लेवल आफ इंटरेक्शन
एंड द मिशन आ आ आ आ आ आ आ आ आ आ आ आ आ आ आ आ आ आ आ आ आ आ आ
कि जिस गर्भ भी अधिक न्यूज़ विरोध opposition सूरन दिस इज द वैल्यू ऑफ योरसेल्फ इन ए
ने स्टेक नजर इंडेक्स और इससे यह जो इंडेक्स लेबल्स लाइफ इवन आरएस सैनी एलिमेंट एंड ए
डेटाफ्रेम डिपेंडेंट ओं इट्स पोजिशन आफ ह्यूमर एक्सेस एनी यू नो लाइक रोली डिपेंडिंग ओं थे इंडेक्स योग नॉट यूज्ड
यूज इंडेक्स वाली उस ओं अरे यू कांट 20123 अनिल मोर्य कहा कि उसका एसएन वैल्यू अब जो करेस्पॉन्डिंग्ली फोन
हैं तो फिर भी बॉक्स पर तो कंप्लीट डेटाफ्रेम नावड़ा पंजीकृत डोंट वांट यूजर कंपनी डेटाफ्रेम बट ओनली वांट ए स्मॉल
पोर्शन आफ ठाट यू कैन गेट थे स्मॉल पोर्शन पर यूजर लाइसेंस
लाइसेंस नथिंग बट ट्यूशन ऑपरेट अट सेलेक्ट सेट ऑफ रोज और कॉलम्स फ्रॉम 10th हैं लेकिन पर्फोमंस लाइसेंस पर्यूषण दिलाओ
कि डायलॉग फंक्शन सोएंगे ई सपोज ई वांट टो गेट द बेस्ट रिजल्ट्स आफ ऑल द स्टूडेंट्स द लास्ट नेम क्वालिटी तस्वीर ज़िंदगी
बुलियन ऑपरेटर अट योर डाटा प्रेम एंड थे लास्ट नेम घाटी अवश्य राय यदि नेगेटिव कलेक्ट्रेक्ट वाली इस
अफवाह ने Bigg Boss ने Bigg Boss ने ऑल द बेस्ट कोर्स इन थिस
स्मॉल पोर्शन आफ ए कि स्वामी रामदेव सेकंड के रहमान पोर्शन फिर डेटाफ्रेम डिपेंडिंग मंतव्य से टिफिन
नॉट सपोर्ट यू डोंट नो बिन उम्दा एक्टिव विहार यह कंडीशन को 131 टेस्ट फॉर
थे फर्स्ट टाइम यू वांट द कॉलम वैल्यूज फ्रॉम फर्स्ट नेम टू टेस्ट
350 500 न्यूज़ लॉक लॉक लॉक लॉक लॉक
विल यू इन नेक्स्ट इलेक्शन रिलेटिड डिपेंडिंग ऑन द वे
वैल्यू योर वैल्यूज ओं का सौभाग्य गेंद सेम थिंग टो डिफरेंसेस
व्यवसायिक फंक्शन है 219 लेट्यूस गार्डन सपोर्ट यू वांट टो गेट ऑल वैल्यूज अपेंटल थर्ड इंटैक्ट एंड वांट
ऑल द कॉलम वैल्यूज बट सदस्यीय चिकित्सीय स्पेसिफाइड थर्ड इन थे वैल्यू
आफ subscribe The Video then
subscribe to the Page हैंड्स फ्री रोज और f12 कर्तव्य फॉर थे
इंडेक्स अपन 222 योग ना डे स्पेशल थर्ड इंडेक्स दिस इंडेक्सेस आउट आफ बाउंड्स फॉर घंटे इंक्लूडेड इन थे हार्ट बट
सुद्दनली वन थिंग ठाट कीप इन माइंड अ 10 एंटरप्राइज को कॉल मी टू 9 इंच एक्स्ट्रा द0
स0 12345 68 वांट टो गेट
यू वांट टो गो ओं एंड ओं कि ताजमहल
चौगाने बिग विन ओवर कार्डिफ़ आठ ओवर ए ईयर एगो तो
स्टार्टिंग फ्रॉम जीरो दिला स्कूलों में गणित भी एक्सप्लोइट कि हद थे सेम टाइम यू डोंट यूजर लॉगइन
डायलॉग फंक्शन यूजिंग लोकल आई लव यू कैन स्पेसिफाई थे ट्रू वैल्यूज आफ वैरीयस स्कॉलरशिप्स
बट इफ यू वांट यू कैन डायरेक्टली सिस्ट स्लाइस फ्रॉम डेट आफ फॉर्म बाय यूजिंग पेरेंट्स इस वैल्यू स
के नौवें और डुबोकर होलीगेट और रोहिला इशू करवा स्लाइस तक प्रॉब्लम्स बट यू कैन सी यू सिंह डीएस 125 व कॉटन बॉल वैल्यूज ठाट
इन थिस कंट्री फॉर अगस्त 2018
कि सुधीर व कपिल ऑफिसर यू कैन सिलाई सेंटर एम अगेंस्ट थिस वर्क्स ओनली ₹1 का नोट यू संदेश को लर्न फ्रॉम ठेर लाइव्स फॉर रोड्स
एंड कॉलम्स टो करप्ट युद्ध लॉक और त्यौहार फंक्शन एंड विद्यार्थी कंट्री एंड थिस वीडियो कैन
फाइट इन डेटाफ्रेम विडियो इड प्रथम लिए रिमाइंडर सब्सक्राइब माय चैनल को बैल
आइकॉन फॉर मोर लेटेस्ट टेक्नोलॉजी इंस्टीट्यूट फॉर क्लास 9 हेलो हाय ट्यूब वाइट विनेगर सब्सक्राइब
तिरस्कारपूर्ण YouTube चैनल को व्हाट्सएप पर यह स्टैंडर्ड आफ इंक्वारी फाइट 21 अ अ
Heads up!
This summary and transcript were automatically generated using AI with the Free YouTube Transcript Summary Tool by LunaNotes.
Generate a summary for freeRelated Summaries
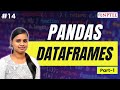
A Comprehensive Guide to Pandas DataFrames in Python
Explore pandas DataFrames: basics, importing data, indexing, and more!
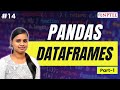
Mastering Pandas DataFrames: A Comprehensive Guide
Learn how to use Pandas DataFrames effectively in Python including data import, manipulation, and more.
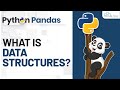
Understanding Pandas Series and Data Structures in Python
In this video, Gaurav explains how to work with Pandas Series in Python, including how to create, manipulate, and analyze data structures. He covers the basics of importing Pandas, creating Series from lists and dictionaries, and modifying index values.
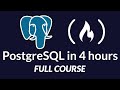
A Comprehensive Guide to PostgreSQL: Basics, Features, and Advanced Concepts
Learn PostgreSQL fundamentals, features, and advanced techniques to enhance your database management skills.
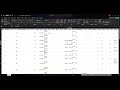
Mastering Basic Navigation and Data Manipulation in Microsoft Excel for Survey Analysis
In this video, we explore essential navigation and manipulation skills in Microsoft Excel, specifically for analyzing survey data. Learn how to identify rows and columns, manage headers, sort data, and handle different types of survey responses effectively.
Most Viewed Summaries
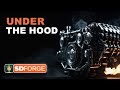
A Comprehensive Guide to Using Stable Diffusion Forge UI
Explore the Stable Diffusion Forge UI, customizable settings, models, and more to enhance your image generation experience.
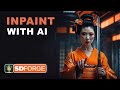
Mastering Inpainting with Stable Diffusion: Fix Mistakes and Enhance Your Images
Learn to fix mistakes and enhance images with Stable Diffusion's inpainting features effectively.
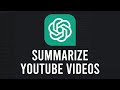
How to Use ChatGPT to Summarize YouTube Videos Efficiently
Learn how to summarize YouTube videos with ChatGPT in just a few simple steps.
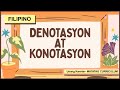
Pag-unawa sa Denotasyon at Konotasyon sa Filipino 4
Alamin ang kahulugan ng denotasyon at konotasyon sa Filipino 4 kasama ang mga halimbawa at pagsasanay.
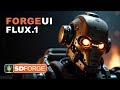
Ultimate Guide to Installing Forge UI and Flowing with Flux Models
Learn how to install Forge UI and explore various Flux models efficiently in this detailed guide.